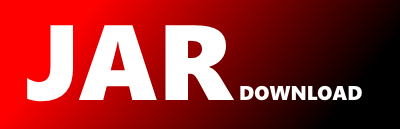
software.amazon.awssdk.services.cloudsearch.model.AnalysisOptions Maven / Gradle / Ivy
Show all versions of cloudsearch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudsearch.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Synonyms, stopwords, and stemming options for an analysis scheme. Includes tokenization dictionary for Japanese.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AnalysisOptions implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SYNONYMS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Synonyms").getter(getter(AnalysisOptions::synonyms)).setter(setter(Builder::synonyms))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Synonyms").build()).build();
private static final SdkField STOPWORDS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Stopwords").getter(getter(AnalysisOptions::stopwords)).setter(setter(Builder::stopwords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Stopwords").build()).build();
private static final SdkField STEMMING_DICTIONARY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StemmingDictionary").getter(getter(AnalysisOptions::stemmingDictionary))
.setter(setter(Builder::stemmingDictionary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StemmingDictionary").build())
.build();
private static final SdkField JAPANESE_TOKENIZATION_DICTIONARY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("JapaneseTokenizationDictionary")
.getter(getter(AnalysisOptions::japaneseTokenizationDictionary))
.setter(setter(Builder::japaneseTokenizationDictionary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JapaneseTokenizationDictionary")
.build()).build();
private static final SdkField ALGORITHMIC_STEMMING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlgorithmicStemming").getter(getter(AnalysisOptions::algorithmicStemmingAsString))
.setter(setter(Builder::algorithmicStemming))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlgorithmicStemming").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SYNONYMS_FIELD,
STOPWORDS_FIELD, STEMMING_DICTIONARY_FIELD, JAPANESE_TOKENIZATION_DICTIONARY_FIELD, ALGORITHMIC_STEMMING_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Synonyms", SYNONYMS_FIELD);
put("Stopwords", STOPWORDS_FIELD);
put("StemmingDictionary", STEMMING_DICTIONARY_FIELD);
put("JapaneseTokenizationDictionary", JAPANESE_TOKENIZATION_DICTIONARY_FIELD);
put("AlgorithmicStemming", ALGORITHMIC_STEMMING_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String synonyms;
private final String stopwords;
private final String stemmingDictionary;
private final String japaneseTokenizationDictionary;
private final String algorithmicStemming;
private AnalysisOptions(BuilderImpl builder) {
this.synonyms = builder.synonyms;
this.stopwords = builder.stopwords;
this.stemmingDictionary = builder.stemmingDictionary;
this.japaneseTokenizationDictionary = builder.japaneseTokenizationDictionary;
this.algorithmicStemming = builder.algorithmicStemming;
}
/**
*
* A JSON object that defines synonym groups and aliases. A synonym group is an array of arrays, where each
* sub-array is a group of terms where each term in the group is considered a synonym of every other term in the
* group. The aliases value is an object that contains a collection of string:value pairs where the string specifies
* a term and the array of values specifies each of the aliases for that term. An alias is considered a synonym of
* the specified term, but the term is not considered a synonym of the alias. For more information about specifying
* synonyms, see Synonyms in the Amazon CloudSearch Developer Guide.
*
*
* @return A JSON object that defines synonym groups and aliases. A synonym group is an array of arrays, where each
* sub-array is a group of terms where each term in the group is considered a synonym of every other term in
* the group. The aliases value is an object that contains a collection of string:value pairs where the
* string specifies a term and the array of values specifies each of the aliases for that term. An alias is
* considered a synonym of the specified term, but the term is not considered a synonym of the alias. For
* more information about specifying synonyms, see Synonyms in the Amazon CloudSearch Developer Guide.
*/
public final String synonyms() {
return synonyms;
}
/**
*
* A JSON array of terms to ignore during indexing and searching. For example, ["a", "an", "the", "of"]
* . The stopwords dictionary must explicitly list each word you want to ignore. Wildcards and regular expressions
* are not supported.
*
*
* @return A JSON array of terms to ignore during indexing and searching. For example,
* ["a", "an", "the", "of"]
. The stopwords dictionary must explicitly list each word you want
* to ignore. Wildcards and regular expressions are not supported.
*/
public final String stopwords() {
return stopwords;
}
/**
*
* A JSON object that contains a collection of string:value pairs that each map a term to its stem. For example,
* {"term1": "stem1", "term2": "stem2", "term3": "stem3"}
. The stemming dictionary is applied in
* addition to any algorithmic stemming. This enables you to override the results of the algorithmic stemming to
* correct specific cases of overstemming or understemming. The maximum size of a stemming dictionary is 500 KB.
*
*
* @return A JSON object that contains a collection of string:value pairs that each map a term to its stem. For
* example, {"term1": "stem1", "term2": "stem2", "term3": "stem3"}
. The stemming dictionary is
* applied in addition to any algorithmic stemming. This enables you to override the results of the
* algorithmic stemming to correct specific cases of overstemming or understemming. The maximum size of a
* stemming dictionary is 500 KB.
*/
public final String stemmingDictionary() {
return stemmingDictionary;
}
/**
*
* A JSON array that contains a collection of terms, tokens, readings and part of speech for Japanese Tokenizaiton.
* The Japanese tokenization dictionary enables you to override the default tokenization for selected terms. This is
* only valid for Japanese language fields.
*
*
* @return A JSON array that contains a collection of terms, tokens, readings and part of speech for Japanese
* Tokenizaiton. The Japanese tokenization dictionary enables you to override the default tokenization for
* selected terms. This is only valid for Japanese language fields.
*/
public final String japaneseTokenizationDictionary() {
return japaneseTokenizationDictionary;
}
/**
*
* The level of algorithmic stemming to perform: none
, minimal
, light
, or
* full
. The available levels vary depending on the language. For more information, see Language Specific Text Processing Settings in the Amazon CloudSearch Developer Guide
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #algorithmicStemming} will return {@link AlgorithmicStemming#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #algorithmicStemmingAsString}.
*
*
* @return The level of algorithmic stemming to perform: none
, minimal
, light
* , or full
. The available levels vary depending on the language. For more information, see Language Specific Text Processing Settings in the Amazon CloudSearch Developer
* Guide
* @see AlgorithmicStemming
*/
public final AlgorithmicStemming algorithmicStemming() {
return AlgorithmicStemming.fromValue(algorithmicStemming);
}
/**
*
* The level of algorithmic stemming to perform: none
, minimal
, light
, or
* full
. The available levels vary depending on the language. For more information, see Language Specific Text Processing Settings in the Amazon CloudSearch Developer Guide
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #algorithmicStemming} will return {@link AlgorithmicStemming#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #algorithmicStemmingAsString}.
*
*
* @return The level of algorithmic stemming to perform: none
, minimal
, light
* , or full
. The available levels vary depending on the language. For more information, see Language Specific Text Processing Settings in the Amazon CloudSearch Developer
* Guide
* @see AlgorithmicStemming
*/
public final String algorithmicStemmingAsString() {
return algorithmicStemming;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(synonyms());
hashCode = 31 * hashCode + Objects.hashCode(stopwords());
hashCode = 31 * hashCode + Objects.hashCode(stemmingDictionary());
hashCode = 31 * hashCode + Objects.hashCode(japaneseTokenizationDictionary());
hashCode = 31 * hashCode + Objects.hashCode(algorithmicStemmingAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AnalysisOptions)) {
return false;
}
AnalysisOptions other = (AnalysisOptions) obj;
return Objects.equals(synonyms(), other.synonyms()) && Objects.equals(stopwords(), other.stopwords())
&& Objects.equals(stemmingDictionary(), other.stemmingDictionary())
&& Objects.equals(japaneseTokenizationDictionary(), other.japaneseTokenizationDictionary())
&& Objects.equals(algorithmicStemmingAsString(), other.algorithmicStemmingAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AnalysisOptions").add("Synonyms", synonyms()).add("Stopwords", stopwords())
.add("StemmingDictionary", stemmingDictionary())
.add("JapaneseTokenizationDictionary", japaneseTokenizationDictionary())
.add("AlgorithmicStemming", algorithmicStemmingAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Synonyms":
return Optional.ofNullable(clazz.cast(synonyms()));
case "Stopwords":
return Optional.ofNullable(clazz.cast(stopwords()));
case "StemmingDictionary":
return Optional.ofNullable(clazz.cast(stemmingDictionary()));
case "JapaneseTokenizationDictionary":
return Optional.ofNullable(clazz.cast(japaneseTokenizationDictionary()));
case "AlgorithmicStemming":
return Optional.ofNullable(clazz.cast(algorithmicStemmingAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function