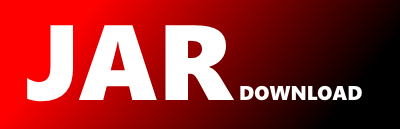
software.amazon.awssdk.services.cloudsearchdomain.CloudSearchDomainClient Maven / Gradle / Ivy
Show all versions of cloudsearchdomain Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudsearchdomain;
import java.nio.file.Path;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.sync.RequestBody;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.cloudsearchdomain.model.CloudSearchDomainException;
import software.amazon.awssdk.services.cloudsearchdomain.model.DocumentServiceException;
import software.amazon.awssdk.services.cloudsearchdomain.model.SearchException;
import software.amazon.awssdk.services.cloudsearchdomain.model.SearchRequest;
import software.amazon.awssdk.services.cloudsearchdomain.model.SearchResponse;
import software.amazon.awssdk.services.cloudsearchdomain.model.SuggestRequest;
import software.amazon.awssdk.services.cloudsearchdomain.model.SuggestResponse;
import software.amazon.awssdk.services.cloudsearchdomain.model.UploadDocumentsRequest;
import software.amazon.awssdk.services.cloudsearchdomain.model.UploadDocumentsResponse;
/**
* Service client for accessing Amazon CloudSearch Domain. This can be created using the static {@link #builder()}
* method.
*
*
* You use the AmazonCloudSearch2013 API to upload documents to a search domain and search those documents.
*
*
* The endpoints for submitting UploadDocuments
, Search
, and Suggest
requests are
* domain-specific. To get the endpoints for your domain, use the Amazon CloudSearch configuration service
* DescribeDomains
action. The domain endpoints are also displayed on the domain dashboard in the Amazon
* CloudSearch console. You submit suggest requests to the search endpoint.
*
*
* For more information, see the Amazon
* CloudSearch Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface CloudSearchDomainClient extends AwsClient {
String SERVICE_NAME = "cloudsearch";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "cloudsearchdomain";
/**
*
* Retrieves a list of documents that match the specified search criteria. How you specify the search criteria
* depends on which query parser you use. Amazon CloudSearch supports four query parsers:
*
*
* simple
: search all text
and text-array
fields for the specified
* string. Search for phrases, individual terms, and prefixes.
* structured
: search specific fields, construct compound queries using Boolean operators, and use
* advanced features such as term boosting and proximity searching.
* lucene
: specify search criteria using the Apache Lucene query parser syntax.
* dismax
: specify search criteria using the simplified subset of the Apache Lucene query parser
* syntax defined by the DisMax query parser.
*
*
* For more information, see Searching Your Data in the
* Amazon CloudSearch Developer Guide.
*
*
* The endpoint for submitting Search
requests is domain-specific. You submit search requests to a
* domain's search endpoint. To get the search endpoint for your domain, use the Amazon CloudSearch configuration
* service DescribeDomains
action. A domain's endpoints are also displayed on the domain dashboard in
* the Amazon CloudSearch console.
*
*
* @param searchRequest
* Container for the parameters to the Search
request.
* @return Result of the Search operation returned by the service.
* @throws SearchException
* Information about any problems encountered while processing a search request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.Search
*/
default SearchResponse search(SearchRequest searchRequest) throws SearchException, AwsServiceException, SdkClientException,
CloudSearchDomainException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of documents that match the specified search criteria. How you specify the search criteria
* depends on which query parser you use. Amazon CloudSearch supports four query parsers:
*
*
* simple
: search all text
and text-array
fields for the specified
* string. Search for phrases, individual terms, and prefixes.
* structured
: search specific fields, construct compound queries using Boolean operators, and use
* advanced features such as term boosting and proximity searching.
* lucene
: specify search criteria using the Apache Lucene query parser syntax.
* dismax
: specify search criteria using the simplified subset of the Apache Lucene query parser
* syntax defined by the DisMax query parser.
*
*
* For more information, see Searching Your Data in the
* Amazon CloudSearch Developer Guide.
*
*
* The endpoint for submitting Search
requests is domain-specific. You submit search requests to a
* domain's search endpoint. To get the search endpoint for your domain, use the Amazon CloudSearch configuration
* service DescribeDomains
action. A domain's endpoints are also displayed on the domain dashboard in
* the Amazon CloudSearch console.
*
*
*
* This is a convenience which creates an instance of the {@link SearchRequest.Builder} avoiding the need to create
* one manually via {@link SearchRequest#builder()}
*
*
* @param searchRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudsearchdomain.model.SearchRequest.Builder} to create a request.
* Container for the parameters to the Search
request.
* @return Result of the Search operation returned by the service.
* @throws SearchException
* Information about any problems encountered while processing a search request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.Search
*/
default SearchResponse search(Consumer searchRequest) throws SearchException, AwsServiceException,
SdkClientException, CloudSearchDomainException {
return search(SearchRequest.builder().applyMutation(searchRequest).build());
}
/**
*
* Retrieves autocomplete suggestions for a partial query string. You can use suggestions enable you to display
* likely matches before users finish typing. In Amazon CloudSearch, suggestions are based on the contents of a
* particular text field. When you request suggestions, Amazon CloudSearch finds all of the documents whose values
* in the suggester field start with the specified query string. The beginning of the field must match the query
* string to be considered a match.
*
*
* For more information about configuring suggesters and retrieving suggestions, see Getting
* Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* The endpoint for submitting Suggest
requests is domain-specific. You submit suggest requests to a
* domain's search endpoint. To get the search endpoint for your domain, use the Amazon CloudSearch configuration
* service DescribeDomains
action. A domain's endpoints are also displayed on the domain dashboard in
* the Amazon CloudSearch console.
*
*
* @param suggestRequest
* Container for the parameters to the Suggest
request.
* @return Result of the Suggest operation returned by the service.
* @throws SearchException
* Information about any problems encountered while processing a search request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.Suggest
*/
default SuggestResponse suggest(SuggestRequest suggestRequest) throws SearchException, AwsServiceException,
SdkClientException, CloudSearchDomainException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves autocomplete suggestions for a partial query string. You can use suggestions enable you to display
* likely matches before users finish typing. In Amazon CloudSearch, suggestions are based on the contents of a
* particular text field. When you request suggestions, Amazon CloudSearch finds all of the documents whose values
* in the suggester field start with the specified query string. The beginning of the field must match the query
* string to be considered a match.
*
*
* For more information about configuring suggesters and retrieving suggestions, see Getting
* Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* The endpoint for submitting Suggest
requests is domain-specific. You submit suggest requests to a
* domain's search endpoint. To get the search endpoint for your domain, use the Amazon CloudSearch configuration
* service DescribeDomains
action. A domain's endpoints are also displayed on the domain dashboard in
* the Amazon CloudSearch console.
*
*
*
* This is a convenience which creates an instance of the {@link SuggestRequest.Builder} avoiding the need to create
* one manually via {@link SuggestRequest#builder()}
*
*
* @param suggestRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudsearchdomain.model.SuggestRequest.Builder} to create a
* request. Container for the parameters to the Suggest
request.
* @return Result of the Suggest operation returned by the service.
* @throws SearchException
* Information about any problems encountered while processing a search request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.Suggest
*/
default SuggestResponse suggest(Consumer suggestRequest) throws SearchException, AwsServiceException,
SdkClientException, CloudSearchDomainException {
return suggest(SuggestRequest.builder().applyMutation(suggestRequest).build());
}
/**
*
* Posts a batch of documents to a search domain for indexing. A document batch is a collection of add and delete
* operations that represent the documents you want to add, update, or delete from your domain. Batches can be
* described in either JSON or XML. Each item that you want Amazon CloudSearch to return as a search result (such as
* a product) is represented as a document. Every document has a unique ID and one or more fields that contain the
* data that you want to search and return in results. Individual documents cannot contain more than 1 MB of data.
* The entire batch cannot exceed 5 MB. To get the best possible upload performance, group add and delete operations
* in batches that are close the 5 MB limit. Submitting a large volume of single-document batches can overload a
* domain's document service.
*
*
* The endpoint for submitting UploadDocuments
requests is domain-specific. To get the document
* endpoint for your domain, use the Amazon CloudSearch configuration service DescribeDomains
action. A
* domain's endpoints are also displayed on the domain dashboard in the Amazon CloudSearch console.
*
*
* For more information about formatting your data for Amazon CloudSearch, see Preparing Your Data
* in the Amazon CloudSearch Developer Guide. For more information about uploading data for indexing, see Uploading Data in the
* Amazon CloudSearch Developer Guide.
*
*
* @param uploadDocumentsRequest
* Container for the parameters to the UploadDocuments
request.
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* A batch of documents formatted in JSON or HTML.
*
* '
* @return Result of the UploadDocuments operation returned by the service.
* @throws DocumentServiceException
* Information about any problems encountered while processing an upload request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.UploadDocuments
*/
default UploadDocumentsResponse uploadDocuments(UploadDocumentsRequest uploadDocumentsRequest, RequestBody requestBody)
throws DocumentServiceException, AwsServiceException, SdkClientException, CloudSearchDomainException {
throw new UnsupportedOperationException();
}
/**
*
* Posts a batch of documents to a search domain for indexing. A document batch is a collection of add and delete
* operations that represent the documents you want to add, update, or delete from your domain. Batches can be
* described in either JSON or XML. Each item that you want Amazon CloudSearch to return as a search result (such as
* a product) is represented as a document. Every document has a unique ID and one or more fields that contain the
* data that you want to search and return in results. Individual documents cannot contain more than 1 MB of data.
* The entire batch cannot exceed 5 MB. To get the best possible upload performance, group add and delete operations
* in batches that are close the 5 MB limit. Submitting a large volume of single-document batches can overload a
* domain's document service.
*
*
* The endpoint for submitting UploadDocuments
requests is domain-specific. To get the document
* endpoint for your domain, use the Amazon CloudSearch configuration service DescribeDomains
action. A
* domain's endpoints are also displayed on the domain dashboard in the Amazon CloudSearch console.
*
*
* For more information about formatting your data for Amazon CloudSearch, see Preparing Your Data
* in the Amazon CloudSearch Developer Guide. For more information about uploading data for indexing, see Uploading Data in the
* Amazon CloudSearch Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UploadDocumentsRequest.Builder} avoiding the need
* to create one manually via {@link UploadDocumentsRequest#builder()}
*
*
* @param uploadDocumentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudsearchdomain.model.UploadDocumentsRequest.Builder} to create a
* request. Container for the parameters to the UploadDocuments
request.
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* A batch of documents formatted in JSON or HTML.
*
* '
* @return Result of the UploadDocuments operation returned by the service.
* @throws DocumentServiceException
* Information about any problems encountered while processing an upload request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.UploadDocuments
*/
default UploadDocumentsResponse uploadDocuments(Consumer uploadDocumentsRequest,
RequestBody requestBody) throws DocumentServiceException, AwsServiceException, SdkClientException,
CloudSearchDomainException {
return uploadDocuments(UploadDocumentsRequest.builder().applyMutation(uploadDocumentsRequest).build(), requestBody);
}
/**
*
* Posts a batch of documents to a search domain for indexing. A document batch is a collection of add and delete
* operations that represent the documents you want to add, update, or delete from your domain. Batches can be
* described in either JSON or XML. Each item that you want Amazon CloudSearch to return as a search result (such as
* a product) is represented as a document. Every document has a unique ID and one or more fields that contain the
* data that you want to search and return in results. Individual documents cannot contain more than 1 MB of data.
* The entire batch cannot exceed 5 MB. To get the best possible upload performance, group add and delete operations
* in batches that are close the 5 MB limit. Submitting a large volume of single-document batches can overload a
* domain's document service.
*
*
* The endpoint for submitting UploadDocuments
requests is domain-specific. To get the document
* endpoint for your domain, use the Amazon CloudSearch configuration service DescribeDomains
action. A
* domain's endpoints are also displayed on the domain dashboard in the Amazon CloudSearch console.
*
*
* For more information about formatting your data for Amazon CloudSearch, see Preparing Your Data
* in the Amazon CloudSearch Developer Guide. For more information about uploading data for indexing, see Uploading Data in the
* Amazon CloudSearch Developer Guide.
*
*
* @param uploadDocumentsRequest
* Container for the parameters to the UploadDocuments
request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* A batch of documents formatted in JSON or HTML.
*
* '
* @return Result of the UploadDocuments operation returned by the service.
* @throws DocumentServiceException
* Information about any problems encountered while processing an upload request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.UploadDocuments
* @see #uploadDocuments(UploadDocumentsRequest, RequestBody)
*/
default UploadDocumentsResponse uploadDocuments(UploadDocumentsRequest uploadDocumentsRequest, Path sourcePath)
throws DocumentServiceException, AwsServiceException, SdkClientException, CloudSearchDomainException {
return uploadDocuments(uploadDocumentsRequest, RequestBody.fromFile(sourcePath));
}
/**
*
* Posts a batch of documents to a search domain for indexing. A document batch is a collection of add and delete
* operations that represent the documents you want to add, update, or delete from your domain. Batches can be
* described in either JSON or XML. Each item that you want Amazon CloudSearch to return as a search result (such as
* a product) is represented as a document. Every document has a unique ID and one or more fields that contain the
* data that you want to search and return in results. Individual documents cannot contain more than 1 MB of data.
* The entire batch cannot exceed 5 MB. To get the best possible upload performance, group add and delete operations
* in batches that are close the 5 MB limit. Submitting a large volume of single-document batches can overload a
* domain's document service.
*
*
* The endpoint for submitting UploadDocuments
requests is domain-specific. To get the document
* endpoint for your domain, use the Amazon CloudSearch configuration service DescribeDomains
action. A
* domain's endpoints are also displayed on the domain dashboard in the Amazon CloudSearch console.
*
*
* For more information about formatting your data for Amazon CloudSearch, see Preparing Your Data
* in the Amazon CloudSearch Developer Guide. For more information about uploading data for indexing, see Uploading Data in the
* Amazon CloudSearch Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UploadDocumentsRequest.Builder} avoiding the need
* to create one manually via {@link UploadDocumentsRequest#builder()}
*
*
* @param uploadDocumentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudsearchdomain.model.UploadDocumentsRequest.Builder} to create a
* request. Container for the parameters to the UploadDocuments
request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* A batch of documents formatted in JSON or HTML.
*
* '
* @return Result of the UploadDocuments operation returned by the service.
* @throws DocumentServiceException
* Information about any problems encountered while processing an upload request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudSearchDomainException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudSearchDomainClient.UploadDocuments
* @see #uploadDocuments(UploadDocumentsRequest, RequestBody)
*/
default UploadDocumentsResponse uploadDocuments(Consumer uploadDocumentsRequest,
Path sourcePath) throws DocumentServiceException, AwsServiceException, SdkClientException, CloudSearchDomainException {
return uploadDocuments(UploadDocumentsRequest.builder().applyMutation(uploadDocumentsRequest).build(), sourcePath);
}
/**
* Create a {@link CloudSearchDomainClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CloudSearchDomainClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CloudSearchDomainClient}.
*/
static CloudSearchDomainClientBuilder builder() {
return new DefaultCloudSearchDomainClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default CloudSearchDomainServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}