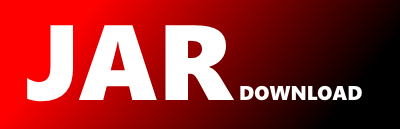
software.amazon.awssdk.services.cloudwatch.model.GetInsightRuleReportRequest Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetInsightRuleReportRequest extends CloudWatchRequest implements
ToCopyableBuilder {
private static final SdkField RULE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetInsightRuleReportRequest::ruleName)).setter(setter(Builder::ruleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuleName").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetInsightRuleReportRequest::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetInsightRuleReportRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(GetInsightRuleReportRequest::period)).setter(setter(Builder::period))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Period").build()).build();
private static final SdkField MAX_CONTRIBUTOR_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(GetInsightRuleReportRequest::maxContributorCount)).setter(setter(Builder::maxContributorCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxContributorCount").build())
.build();
private static final SdkField> METRICS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(GetInsightRuleReportRequest::metrics))
.setter(setter(Builder::metrics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Metrics").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ORDER_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetInsightRuleReportRequest::orderBy)).setter(setter(Builder::orderBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrderBy").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RULE_NAME_FIELD,
START_TIME_FIELD, END_TIME_FIELD, PERIOD_FIELD, MAX_CONTRIBUTOR_COUNT_FIELD, METRICS_FIELD, ORDER_BY_FIELD));
private final String ruleName;
private final Instant startTime;
private final Instant endTime;
private final Integer period;
private final Integer maxContributorCount;
private final List metrics;
private final String orderBy;
private GetInsightRuleReportRequest(BuilderImpl builder) {
super(builder);
this.ruleName = builder.ruleName;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.period = builder.period;
this.maxContributorCount = builder.maxContributorCount;
this.metrics = builder.metrics;
this.orderBy = builder.orderBy;
}
/**
*
* The name of the rule that you want to see data from.
*
*
* @return The name of the rule that you want to see data from.
*/
public String ruleName() {
return ruleName;
}
/**
*
* The start time of the data to use in the report. When used in a raw HTTP Query API, it is formatted as
* yyyy-MM-dd'T'HH:mm:ss
. For example, 2019-07-01T23:59:59
.
*
*
* @return The start time of the data to use in the report. When used in a raw HTTP Query API, it is formatted as
* yyyy-MM-dd'T'HH:mm:ss
. For example, 2019-07-01T23:59:59
.
*/
public Instant startTime() {
return startTime;
}
/**
*
* The end time of the data to use in the report. When used in a raw HTTP Query API, it is formatted as
* yyyy-MM-dd'T'HH:mm:ss
. For example, 2019-07-01T23:59:59
.
*
*
* @return The end time of the data to use in the report. When used in a raw HTTP Query API, it is formatted as
* yyyy-MM-dd'T'HH:mm:ss
. For example, 2019-07-01T23:59:59
.
*/
public Instant endTime() {
return endTime;
}
/**
*
* The period, in seconds, to use for the statistics in the InsightRuleMetricDatapoint
results.
*
*
* @return The period, in seconds, to use for the statistics in the InsightRuleMetricDatapoint
results.
*/
public Integer period() {
return period;
}
/**
*
* The maximum number of contributors to include in the report. The range is 1 to 100. If you omit this, the default
* of 10 is used.
*
*
* @return The maximum number of contributors to include in the report. The range is 1 to 100. If you omit this, the
* default of 10 is used.
*/
public Integer maxContributorCount() {
return maxContributorCount;
}
/**
* Returns true if the Metrics property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasMetrics() {
return metrics != null && !(metrics instanceof SdkAutoConstructList);
}
/**
*
* Specifies which metrics to use for aggregation of contributor values for the report. You can specify one or more
* of the following metrics:
*
*
* -
*
* UniqueContributors
-- the number of unique contributors for each data point.
*
*
* -
*
* MaxContributorValue
-- the value of the top contributor for each data point. The identity of the
* contributor may change for each data point in the graph.
*
*
* If this rule aggregates by COUNT, the top contributor for each data point is the contributor with the most
* occurrences in that period. If the rule aggregates by SUM, the top contributor is the contributor with the
* highest sum in the log field specified by the rule's Value
, during that period.
*
*
* -
*
* SampleCount
-- the number of data points matched by the rule.
*
*
* -
*
* Sum
-- the sum of the values from all contributors during the time period represented by that data
* point.
*
*
* -
*
* Minimum
-- the minimum value from a single observation during the time period represented by that
* data point.
*
*
* -
*
* Maximum
-- the maximum value from a single observation during the time period represented by that
* data point.
*
*
* -
*
* Average
-- the average value from all contributors during the time period represented by that data
* point.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasMetrics()} to see if a value was sent in this field.
*
*
* @return Specifies which metrics to use for aggregation of contributor values for the report. You can specify one
* or more of the following metrics:
*
* -
*
* UniqueContributors
-- the number of unique contributors for each data point.
*
*
* -
*
* MaxContributorValue
-- the value of the top contributor for each data point. The identity of
* the contributor may change for each data point in the graph.
*
*
* If this rule aggregates by COUNT, the top contributor for each data point is the contributor with the
* most occurrences in that period. If the rule aggregates by SUM, the top contributor is the contributor
* with the highest sum in the log field specified by the rule's Value
, during that period.
*
*
* -
*
* SampleCount
-- the number of data points matched by the rule.
*
*
* -
*
* Sum
-- the sum of the values from all contributors during the time period represented by
* that data point.
*
*
* -
*
* Minimum
-- the minimum value from a single observation during the time period represented by
* that data point.
*
*
* -
*
* Maximum
-- the maximum value from a single observation during the time period represented by
* that data point.
*
*
* -
*
* Average
-- the average value from all contributors during the time period represented by
* that data point.
*
*
*/
public List metrics() {
return metrics;
}
/**
*
* Determines what statistic to use to rank the contributors. Valid values are SUM and MAXIMUM.
*
*
* @return Determines what statistic to use to rank the contributors. Valid values are SUM and MAXIMUM.
*/
public String orderBy() {
return orderBy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(ruleName());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(period());
hashCode = 31 * hashCode + Objects.hashCode(maxContributorCount());
hashCode = 31 * hashCode + Objects.hashCode(metrics());
hashCode = 31 * hashCode + Objects.hashCode(orderBy());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetInsightRuleReportRequest)) {
return false;
}
GetInsightRuleReportRequest other = (GetInsightRuleReportRequest) obj;
return Objects.equals(ruleName(), other.ruleName()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(period(), other.period())
&& Objects.equals(maxContributorCount(), other.maxContributorCount())
&& Objects.equals(metrics(), other.metrics()) && Objects.equals(orderBy(), other.orderBy());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("GetInsightRuleReportRequest").add("RuleName", ruleName()).add("StartTime", startTime())
.add("EndTime", endTime()).add("Period", period()).add("MaxContributorCount", maxContributorCount())
.add("Metrics", metrics()).add("OrderBy", orderBy()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RuleName":
return Optional.ofNullable(clazz.cast(ruleName()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "Period":
return Optional.ofNullable(clazz.cast(period()));
case "MaxContributorCount":
return Optional.ofNullable(clazz.cast(maxContributorCount()));
case "Metrics":
return Optional.ofNullable(clazz.cast(metrics()));
case "OrderBy":
return Optional.ofNullable(clazz.cast(orderBy()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function