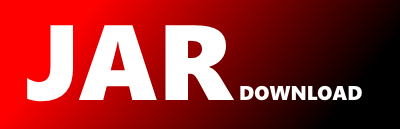
software.amazon.awssdk.services.cloudwatch.model.MetricDatum Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Encapsulates the information sent to either create a metric or add new values to be aggregated into an existing
* metric.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MetricDatum implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField METRIC_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetricName").getter(getter(MetricDatum::metricName)).setter(setter(Builder::metricName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricName").build()).build();
private static final SdkField> DIMENSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Dimensions")
.getter(getter(MetricDatum::dimensions))
.setter(setter(Builder::dimensions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Dimensions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Dimension::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("Timestamp").getter(getter(MetricDatum::timestamp)).setter(setter(Builder::timestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timestamp").build()).build();
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("Value")
.getter(getter(MetricDatum::value)).setter(setter(Builder::value))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Value").build()).build();
private static final SdkField STATISTIC_VALUES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StatisticValues")
.getter(getter(MetricDatum::statisticValues)).setter(setter(Builder::statisticValues))
.constructor(StatisticSet::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatisticValues").build()).build();
private static final SdkField> VALUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Values")
.getter(getter(MetricDatum::values))
.setter(setter(Builder::values))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Values").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> COUNTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Counts")
.getter(getter(MetricDatum::counts))
.setter(setter(Builder::counts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Counts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField UNIT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Unit")
.getter(getter(MetricDatum::unitAsString)).setter(setter(Builder::unit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Unit").build()).build();
private static final SdkField STORAGE_RESOLUTION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StorageResolution").getter(getter(MetricDatum::storageResolution))
.setter(setter(Builder::storageResolution))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageResolution").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(METRIC_NAME_FIELD,
DIMENSIONS_FIELD, TIMESTAMP_FIELD, VALUE_FIELD, STATISTIC_VALUES_FIELD, VALUES_FIELD, COUNTS_FIELD, UNIT_FIELD,
STORAGE_RESOLUTION_FIELD));
private static final long serialVersionUID = 1L;
private final String metricName;
private final List dimensions;
private final Instant timestamp;
private final Double value;
private final StatisticSet statisticValues;
private final List values;
private final List counts;
private final String unit;
private final Integer storageResolution;
private MetricDatum(BuilderImpl builder) {
this.metricName = builder.metricName;
this.dimensions = builder.dimensions;
this.timestamp = builder.timestamp;
this.value = builder.value;
this.statisticValues = builder.statisticValues;
this.values = builder.values;
this.counts = builder.counts;
this.unit = builder.unit;
this.storageResolution = builder.storageResolution;
}
/**
*
* The name of the metric.
*
*
* @return The name of the metric.
*/
public final String metricName() {
return metricName;
}
/**
* Returns true if the Dimensions property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasDimensions() {
return dimensions != null && !(dimensions instanceof SdkAutoConstructList);
}
/**
*
* The dimensions associated with the metric.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasDimensions()} to see if a value was sent in this field.
*
*
* @return The dimensions associated with the metric.
*/
public final List dimensions() {
return dimensions;
}
/**
*
* The time the metric data was received, expressed as the number of milliseconds since Jan 1, 1970 00:00:00 UTC.
*
*
* @return The time the metric data was received, expressed as the number of milliseconds since Jan 1, 1970 00:00:00
* UTC.
*/
public final Instant timestamp() {
return timestamp;
}
/**
*
* The value for the metric.
*
*
* Although the parameter accepts numbers of type Double, CloudWatch rejects values that are either too small or too
* large. Values must be in the range of -2^360 to 2^360. In addition, special values (for example, NaN, +Infinity,
* -Infinity) are not supported.
*
*
* @return The value for the metric.
*
* Although the parameter accepts numbers of type Double, CloudWatch rejects values that are either too
* small or too large. Values must be in the range of -2^360 to 2^360. In addition, special values (for
* example, NaN, +Infinity, -Infinity) are not supported.
*/
public final Double value() {
return value;
}
/**
*
* The statistical values for the metric.
*
*
* @return The statistical values for the metric.
*/
public final StatisticSet statisticValues() {
return statisticValues;
}
/**
* Returns true if the Values property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasValues() {
return values != null && !(values instanceof SdkAutoConstructList);
}
/**
*
* Array of numbers representing the values for the metric during the period. Each unique value is listed just once
* in this array, and the corresponding number in the Counts
array specifies the number of times that
* value occurred during the period. You can include up to 150 unique values in each PutMetricData
* action that specifies a Values
array.
*
*
* Although the Values
array accepts numbers of type Double
, CloudWatch rejects values
* that are either too small or too large. Values must be in the range of -2^360 to 2^360. In addition, special
* values (for example, NaN, +Infinity, -Infinity) are not supported.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasValues()} to see if a value was sent in this field.
*
*
* @return Array of numbers representing the values for the metric during the period. Each unique value is listed
* just once in this array, and the corresponding number in the Counts
array specifies the
* number of times that value occurred during the period. You can include up to 150 unique values in each
* PutMetricData
action that specifies a Values
array.
*
* Although the Values
array accepts numbers of type Double
, CloudWatch rejects
* values that are either too small or too large. Values must be in the range of -2^360 to 2^360. In
* addition, special values (for example, NaN, +Infinity, -Infinity) are not supported.
*/
public final List values() {
return values;
}
/**
* Returns true if the Counts property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasCounts() {
return counts != null && !(counts instanceof SdkAutoConstructList);
}
/**
*
* Array of numbers that is used along with the Values
array. Each number in the Count
* array is the number of times the corresponding value in the Values
array occurred during the period.
*
*
* If you omit the Counts
array, the default of 1 is used as the value for each count. If you include a
* Counts
array, it must include the same amount of values as the Values
array.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCounts()} to see if a value was sent in this field.
*
*
* @return Array of numbers that is used along with the Values
array. Each number in the
* Count
array is the number of times the corresponding value in the Values
array
* occurred during the period.
*
* If you omit the Counts
array, the default of 1 is used as the value for each count. If you
* include a Counts
array, it must include the same amount of values as the Values
* array.
*/
public final List counts() {
return counts;
}
/**
*
* When you are using a Put
operation, this defines what unit you want to use when storing the metric.
*
*
* In a Get
operation, this displays the unit that is used for the metric.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link StandardUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return When you are using a Put
operation, this defines what unit you want to use when storing the
* metric.
*
* In a Get
operation, this displays the unit that is used for the metric.
* @see StandardUnit
*/
public final StandardUnit unit() {
return StandardUnit.fromValue(unit);
}
/**
*
* When you are using a Put
operation, this defines what unit you want to use when storing the metric.
*
*
* In a Get
operation, this displays the unit that is used for the metric.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link StandardUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return When you are using a Put
operation, this defines what unit you want to use when storing the
* metric.
*
* In a Get
operation, this displays the unit that is used for the metric.
* @see StandardUnit
*/
public final String unitAsString() {
return unit;
}
/**
*
* Valid values are 1 and 60. Setting this to 1 specifies this metric as a high-resolution metric, so that
* CloudWatch stores the metric with sub-minute resolution down to one second. Setting this to 60 specifies this
* metric as a regular-resolution metric, which CloudWatch stores at 1-minute resolution. Currently, high resolution
* is available only for custom metrics. For more information about high-resolution metrics, see High-Resolution Metrics in the Amazon CloudWatch User Guide.
*
*
* This field is optional, if you do not specify it the default of 60 is used.
*
*
* @return Valid values are 1 and 60. Setting this to 1 specifies this metric as a high-resolution metric, so that
* CloudWatch stores the metric with sub-minute resolution down to one second. Setting this to 60 specifies
* this metric as a regular-resolution metric, which CloudWatch stores at 1-minute resolution. Currently,
* high resolution is available only for custom metrics. For more information about high-resolution metrics,
* see High-Resolution Metrics in the Amazon CloudWatch User Guide.
*
* This field is optional, if you do not specify it the default of 60 is used.
*/
public final Integer storageResolution() {
return storageResolution;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(metricName());
hashCode = 31 * hashCode + Objects.hashCode(hasDimensions() ? dimensions() : null);
hashCode = 31 * hashCode + Objects.hashCode(timestamp());
hashCode = 31 * hashCode + Objects.hashCode(value());
hashCode = 31 * hashCode + Objects.hashCode(statisticValues());
hashCode = 31 * hashCode + Objects.hashCode(hasValues() ? values() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCounts() ? counts() : null);
hashCode = 31 * hashCode + Objects.hashCode(unitAsString());
hashCode = 31 * hashCode + Objects.hashCode(storageResolution());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MetricDatum)) {
return false;
}
MetricDatum other = (MetricDatum) obj;
return Objects.equals(metricName(), other.metricName()) && hasDimensions() == other.hasDimensions()
&& Objects.equals(dimensions(), other.dimensions()) && Objects.equals(timestamp(), other.timestamp())
&& Objects.equals(value(), other.value()) && Objects.equals(statisticValues(), other.statisticValues())
&& hasValues() == other.hasValues() && Objects.equals(values(), other.values())
&& hasCounts() == other.hasCounts() && Objects.equals(counts(), other.counts())
&& Objects.equals(unitAsString(), other.unitAsString())
&& Objects.equals(storageResolution(), other.storageResolution());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MetricDatum").add("MetricName", metricName())
.add("Dimensions", hasDimensions() ? dimensions() : null).add("Timestamp", timestamp()).add("Value", value())
.add("StatisticValues", statisticValues()).add("Values", hasValues() ? values() : null)
.add("Counts", hasCounts() ? counts() : null).add("Unit", unitAsString())
.add("StorageResolution", storageResolution()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MetricName":
return Optional.ofNullable(clazz.cast(metricName()));
case "Dimensions":
return Optional.ofNullable(clazz.cast(dimensions()));
case "Timestamp":
return Optional.ofNullable(clazz.cast(timestamp()));
case "Value":
return Optional.ofNullable(clazz.cast(value()));
case "StatisticValues":
return Optional.ofNullable(clazz.cast(statisticValues()));
case "Values":
return Optional.ofNullable(clazz.cast(values()));
case "Counts":
return Optional.ofNullable(clazz.cast(counts()));
case "Unit":
return Optional.ofNullable(clazz.cast(unitAsString()));
case "StorageResolution":
return Optional.ofNullable(clazz.cast(storageResolution()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Although the parameter accepts numbers of type Double, CloudWatch rejects values that are either too
* small or too large. Values must be in the range of -2^360 to 2^360. In addition, special values (for
* example, NaN, +Infinity, -Infinity) are not supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder value(Double value);
/**
*
* The statistical values for the metric.
*
*
* @param statisticValues
* The statistical values for the metric.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statisticValues(StatisticSet statisticValues);
/**
*
* The statistical values for the metric.
*
* This is a convenience that creates an instance of the {@link StatisticSet.Builder} avoiding the need to
* create one manually via {@link StatisticSet#builder()}.
*
* When the {@link Consumer} completes, {@link StatisticSet.Builder#build()} is called immediately and its
* result is passed to {@link #statisticValues(StatisticSet)}.
*
* @param statisticValues
* a consumer that will call methods on {@link StatisticSet.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #statisticValues(StatisticSet)
*/
default Builder statisticValues(Consumer statisticValues) {
return statisticValues(StatisticSet.builder().applyMutation(statisticValues).build());
}
/**
*
* Array of numbers representing the values for the metric during the period. Each unique value is listed just
* once in this array, and the corresponding number in the Counts
array specifies the number of
* times that value occurred during the period. You can include up to 150 unique values in each
* PutMetricData
action that specifies a Values
array.
*
*
* Although the Values
array accepts numbers of type Double
, CloudWatch rejects values
* that are either too small or too large. Values must be in the range of -2^360 to 2^360. In addition, special
* values (for example, NaN, +Infinity, -Infinity) are not supported.
*
*
* @param values
* Array of numbers representing the values for the metric during the period. Each unique value is listed
* just once in this array, and the corresponding number in the Counts
array specifies the
* number of times that value occurred during the period. You can include up to 150 unique values in each
* PutMetricData
action that specifies a Values
array.
*
* Although the Values
array accepts numbers of type Double
, CloudWatch rejects
* values that are either too small or too large. Values must be in the range of -2^360 to 2^360. In
* addition, special values (for example, NaN, +Infinity, -Infinity) are not supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder values(Collection values);
/**
*
* Array of numbers representing the values for the metric during the period. Each unique value is listed just
* once in this array, and the corresponding number in the Counts
array specifies the number of
* times that value occurred during the period. You can include up to 150 unique values in each
* PutMetricData
action that specifies a Values
array.
*
*
* Although the Values
array accepts numbers of type Double
, CloudWatch rejects values
* that are either too small or too large. Values must be in the range of -2^360 to 2^360. In addition, special
* values (for example, NaN, +Infinity, -Infinity) are not supported.
*
*
* @param values
* Array of numbers representing the values for the metric during the period. Each unique value is listed
* just once in this array, and the corresponding number in the Counts
array specifies the
* number of times that value occurred during the period. You can include up to 150 unique values in each
* PutMetricData
action that specifies a Values
array.
*
* Although the Values
array accepts numbers of type Double
, CloudWatch rejects
* values that are either too small or too large. Values must be in the range of -2^360 to 2^360. In
* addition, special values (for example, NaN, +Infinity, -Infinity) are not supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder values(Double... values);
/**
*
* Array of numbers that is used along with the Values
array. Each number in the Count
* array is the number of times the corresponding value in the Values
array occurred during the
* period.
*
*
* If you omit the Counts
array, the default of 1 is used as the value for each count. If you
* include a Counts
array, it must include the same amount of values as the Values
* array.
*
*
* @param counts
* Array of numbers that is used along with the Values
array. Each number in the
* Count
array is the number of times the corresponding value in the Values
* array occurred during the period.
*
* If you omit the Counts
array, the default of 1 is used as the value for each count. If
* you include a Counts
array, it must include the same amount of values as the
* Values
array.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder counts(Collection counts);
/**
*
* Array of numbers that is used along with the Values
array. Each number in the Count
* array is the number of times the corresponding value in the Values
array occurred during the
* period.
*
*
* If you omit the Counts
array, the default of 1 is used as the value for each count. If you
* include a Counts
array, it must include the same amount of values as the Values
* array.
*
*
* @param counts
* Array of numbers that is used along with the Values
array. Each number in the
* Count
array is the number of times the corresponding value in the Values
* array occurred during the period.
*
* If you omit the Counts
array, the default of 1 is used as the value for each count. If
* you include a Counts
array, it must include the same amount of values as the
* Values
array.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder counts(Double... counts);
/**
*
* When you are using a Put
operation, this defines what unit you want to use when storing the
* metric.
*
*
* In a Get
operation, this displays the unit that is used for the metric.
*
*
* @param unit
* When you are using a Put
operation, this defines what unit you want to use when storing
* the metric.
*
* In a Get
operation, this displays the unit that is used for the metric.
* @see StandardUnit
* @return Returns a reference to this object so that method calls can be chained together.
* @see StandardUnit
*/
Builder unit(String unit);
/**
*
* When you are using a Put
operation, this defines what unit you want to use when storing the
* metric.
*
*
* In a Get
operation, this displays the unit that is used for the metric.
*
*
* @param unit
* When you are using a Put
operation, this defines what unit you want to use when storing
* the metric.
*
* In a Get
operation, this displays the unit that is used for the metric.
* @see StandardUnit
* @return Returns a reference to this object so that method calls can be chained together.
* @see StandardUnit
*/
Builder unit(StandardUnit unit);
/**
*
* Valid values are 1 and 60. Setting this to 1 specifies this metric as a high-resolution metric, so that
* CloudWatch stores the metric with sub-minute resolution down to one second. Setting this to 60 specifies this
* metric as a regular-resolution metric, which CloudWatch stores at 1-minute resolution. Currently, high
* resolution is available only for custom metrics. For more information about high-resolution metrics, see High-Resolution Metrics in the Amazon CloudWatch User Guide.
*
*
* This field is optional, if you do not specify it the default of 60 is used.
*
*
* @param storageResolution
* Valid values are 1 and 60. Setting this to 1 specifies this metric as a high-resolution metric, so
* that CloudWatch stores the metric with sub-minute resolution down to one second. Setting this to 60
* specifies this metric as a regular-resolution metric, which CloudWatch stores at 1-minute resolution.
* Currently, high resolution is available only for custom metrics. For more information about
* high-resolution metrics, see High-Resolution Metrics in the Amazon CloudWatch User Guide.
*
* This field is optional, if you do not specify it the default of 60 is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder storageResolution(Integer storageResolution);
}
static final class BuilderImpl implements Builder {
private String metricName;
private List dimensions = DefaultSdkAutoConstructList.getInstance();
private Instant timestamp;
private Double value;
private StatisticSet statisticValues;
private List values = DefaultSdkAutoConstructList.getInstance();
private List counts = DefaultSdkAutoConstructList.getInstance();
private String unit;
private Integer storageResolution;
private BuilderImpl() {
}
private BuilderImpl(MetricDatum model) {
metricName(model.metricName);
dimensions(model.dimensions);
timestamp(model.timestamp);
value(model.value);
statisticValues(model.statisticValues);
values(model.values);
counts(model.counts);
unit(model.unit);
storageResolution(model.storageResolution);
}
public final String getMetricName() {
return metricName;
}
@Override
public final Builder metricName(String metricName) {
this.metricName = metricName;
return this;
}
public final void setMetricName(String metricName) {
this.metricName = metricName;
}
public final Collection getDimensions() {
if (dimensions instanceof SdkAutoConstructList) {
return null;
}
return dimensions != null ? dimensions.stream().map(Dimension::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder dimensions(Collection dimensions) {
this.dimensions = DimensionsCopier.copy(dimensions);
return this;
}
@Override
@SafeVarargs
public final Builder dimensions(Dimension... dimensions) {
dimensions(Arrays.asList(dimensions));
return this;
}
@Override
@SafeVarargs
public final Builder dimensions(Consumer... dimensions) {
dimensions(Stream.of(dimensions).map(c -> Dimension.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setDimensions(Collection dimensions) {
this.dimensions = DimensionsCopier.copyFromBuilder(dimensions);
}
public final Instant getTimestamp() {
return timestamp;
}
@Override
public final Builder timestamp(Instant timestamp) {
this.timestamp = timestamp;
return this;
}
public final void setTimestamp(Instant timestamp) {
this.timestamp = timestamp;
}
public final Double getValue() {
return value;
}
@Override
public final Builder value(Double value) {
this.value = value;
return this;
}
public final void setValue(Double value) {
this.value = value;
}
public final StatisticSet.Builder getStatisticValues() {
return statisticValues != null ? statisticValues.toBuilder() : null;
}
@Override
public final Builder statisticValues(StatisticSet statisticValues) {
this.statisticValues = statisticValues;
return this;
}
public final void setStatisticValues(StatisticSet.BuilderImpl statisticValues) {
this.statisticValues = statisticValues != null ? statisticValues.build() : null;
}
public final Collection getValues() {
if (values instanceof SdkAutoConstructList) {
return null;
}
return values;
}
@Override
public final Builder values(Collection values) {
this.values = ValuesCopier.copy(values);
return this;
}
@Override
@SafeVarargs
public final Builder values(Double... values) {
values(Arrays.asList(values));
return this;
}
public final void setValues(Collection values) {
this.values = ValuesCopier.copy(values);
}
public final Collection getCounts() {
if (counts instanceof SdkAutoConstructList) {
return null;
}
return counts;
}
@Override
public final Builder counts(Collection counts) {
this.counts = CountsCopier.copy(counts);
return this;
}
@Override
@SafeVarargs
public final Builder counts(Double... counts) {
counts(Arrays.asList(counts));
return this;
}
public final void setCounts(Collection counts) {
this.counts = CountsCopier.copy(counts);
}
public final String getUnit() {
return unit;
}
@Override
public final Builder unit(String unit) {
this.unit = unit;
return this;
}
@Override
public final Builder unit(StandardUnit unit) {
this.unit(unit == null ? null : unit.toString());
return this;
}
public final void setUnit(String unit) {
this.unit = unit;
}
public final Integer getStorageResolution() {
return storageResolution;
}
@Override
public final Builder storageResolution(Integer storageResolution) {
this.storageResolution = storageResolution;
return this;
}
public final void setStorageResolution(Integer storageResolution) {
this.storageResolution = storageResolution;
}
@Override
public MetricDatum build() {
return new MetricDatum(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}