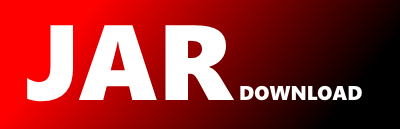
software.amazon.awssdk.services.cloudwatch.model.GetMetricWidgetImageRequest Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetMetricWidgetImageRequest extends CloudWatchRequest implements
ToCopyableBuilder {
private static final SdkField METRIC_WIDGET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetricWidget").getter(getter(GetMetricWidgetImageRequest::metricWidget))
.setter(setter(Builder::metricWidget))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricWidget").build()).build();
private static final SdkField OUTPUT_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputFormat").getter(getter(GetMetricWidgetImageRequest::outputFormat))
.setter(setter(Builder::outputFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputFormat").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(METRIC_WIDGET_FIELD,
OUTPUT_FORMAT_FIELD));
private final String metricWidget;
private final String outputFormat;
private GetMetricWidgetImageRequest(BuilderImpl builder) {
super(builder);
this.metricWidget = builder.metricWidget;
this.outputFormat = builder.outputFormat;
}
/**
*
* A JSON string that defines the bitmap graph to be retrieved. The string includes the metrics to include in the
* graph, statistics, annotations, title, axis limits, and so on. You can include only one MetricWidget
* parameter in each GetMetricWidgetImage
call.
*
*
* For more information about the syntax of MetricWidget
see GetMetricWidgetImage: Metric Widget Structure and Syntax.
*
*
* If any metric on the graph could not load all the requested data points, an orange triangle with an exclamation
* point appears next to the graph legend.
*
*
* @return A JSON string that defines the bitmap graph to be retrieved. The string includes the metrics to include
* in the graph, statistics, annotations, title, axis limits, and so on. You can include only one
* MetricWidget
parameter in each GetMetricWidgetImage
call.
*
* For more information about the syntax of MetricWidget
see GetMetricWidgetImage: Metric Widget Structure and Syntax.
*
*
* If any metric on the graph could not load all the requested data points, an orange triangle with an
* exclamation point appears next to the graph legend.
*/
public final String metricWidget() {
return metricWidget;
}
/**
*
* The format of the resulting image. Only PNG images are supported.
*
*
* The default is png
. If you specify png
, the API returns an HTTP response with the
* content-type set to text/xml
. The image data is in a MetricWidgetImage
field. For
* example:
*
*
* <GetMetricWidgetImageResponse xmlns=<URLstring>>
*
*
* <GetMetricWidgetImageResult>
*
*
* <MetricWidgetImage>
*
*
* iVBORw0KGgoAAAANSUhEUgAAAlgAAAGQEAYAAAAip...
*
*
* </MetricWidgetImage>
*
*
* </GetMetricWidgetImageResult>
*
*
* <ResponseMetadata>
*
*
* <RequestId>6f0d4192-4d42-11e8-82c1-f539a07e0e3b</RequestId>
*
*
* </ResponseMetadata>
*
*
* </GetMetricWidgetImageResponse>
*
*
* The image/png
setting is intended only for custom HTTP requests. For most use cases, and all actions
* using an Amazon Web Services SDK, you should use png
. If you specify image/png
, the
* HTTP response has a content-type set to image/png
, and the body of the response is a PNG image.
*
*
* @return The format of the resulting image. Only PNG images are supported.
*
* The default is png
. If you specify png
, the API returns an HTTP response with
* the content-type set to text/xml
. The image data is in a MetricWidgetImage
* field. For example:
*
*
* <GetMetricWidgetImageResponse xmlns=<URLstring>>
*
*
* <GetMetricWidgetImageResult>
*
*
* <MetricWidgetImage>
*
*
* iVBORw0KGgoAAAANSUhEUgAAAlgAAAGQEAYAAAAip...
*
*
* </MetricWidgetImage>
*
*
* </GetMetricWidgetImageResult>
*
*
* <ResponseMetadata>
*
*
* <RequestId>6f0d4192-4d42-11e8-82c1-f539a07e0e3b</RequestId>
*
*
* </ResponseMetadata>
*
*
* </GetMetricWidgetImageResponse>
*
*
* The image/png
setting is intended only for custom HTTP requests. For most use cases, and all
* actions using an Amazon Web Services SDK, you should use png
. If you specify
* image/png
, the HTTP response has a content-type set to image/png
, and the body
* of the response is a PNG image.
*/
public final String outputFormat() {
return outputFormat;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(metricWidget());
hashCode = 31 * hashCode + Objects.hashCode(outputFormat());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetMetricWidgetImageRequest)) {
return false;
}
GetMetricWidgetImageRequest other = (GetMetricWidgetImageRequest) obj;
return Objects.equals(metricWidget(), other.metricWidget()) && Objects.equals(outputFormat(), other.outputFormat());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetMetricWidgetImageRequest").add("MetricWidget", metricWidget())
.add("OutputFormat", outputFormat()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MetricWidget":
return Optional.ofNullable(clazz.cast(metricWidget()));
case "OutputFormat":
return Optional.ofNullable(clazz.cast(outputFormat()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* For more information about the syntax of MetricWidget
see GetMetricWidgetImage: Metric Widget Structure and Syntax.
*
*
* If any metric on the graph could not load all the requested data points, an orange triangle with an
* exclamation point appears next to the graph legend.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder metricWidget(String metricWidget);
/**
*
* The format of the resulting image. Only PNG images are supported.
*
*
* The default is png
. If you specify png
, the API returns an HTTP response with the
* content-type set to text/xml
. The image data is in a MetricWidgetImage
field. For
* example:
*
*
* <GetMetricWidgetImageResponse xmlns=<URLstring>>
*
*
* <GetMetricWidgetImageResult>
*
*
* <MetricWidgetImage>
*
*
* iVBORw0KGgoAAAANSUhEUgAAAlgAAAGQEAYAAAAip...
*
*
* </MetricWidgetImage>
*
*
* </GetMetricWidgetImageResult>
*
*
* <ResponseMetadata>
*
*
* <RequestId>6f0d4192-4d42-11e8-82c1-f539a07e0e3b</RequestId>
*
*
* </ResponseMetadata>
*
*
* </GetMetricWidgetImageResponse>
*
*
* The image/png
setting is intended only for custom HTTP requests. For most use cases, and all
* actions using an Amazon Web Services SDK, you should use png
. If you specify
* image/png
, the HTTP response has a content-type set to image/png
, and the body of
* the response is a PNG image.
*
*
* @param outputFormat
* The format of the resulting image. Only PNG images are supported.
*
* The default is png
. If you specify png
, the API returns an HTTP response
* with the content-type set to text/xml
. The image data is in a
* MetricWidgetImage
field. For example:
*
*
* <GetMetricWidgetImageResponse xmlns=<URLstring>>
*
*
* <GetMetricWidgetImageResult>
*
*
* <MetricWidgetImage>
*
*
* iVBORw0KGgoAAAANSUhEUgAAAlgAAAGQEAYAAAAip...
*
*
* </MetricWidgetImage>
*
*
* </GetMetricWidgetImageResult>
*
*
* <ResponseMetadata>
*
*
* <RequestId>6f0d4192-4d42-11e8-82c1-f539a07e0e3b</RequestId>
*
*
* </ResponseMetadata>
*
*
* </GetMetricWidgetImageResponse>
*
*
* The image/png
setting is intended only for custom HTTP requests. For most use cases, and
* all actions using an Amazon Web Services SDK, you should use png
. If you specify
* image/png
, the HTTP response has a content-type set to image/png
, and the
* body of the response is a PNG image.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder outputFormat(String outputFormat);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudWatchRequest.BuilderImpl implements Builder {
private String metricWidget;
private String outputFormat;
private BuilderImpl() {
}
private BuilderImpl(GetMetricWidgetImageRequest model) {
super(model);
metricWidget(model.metricWidget);
outputFormat(model.outputFormat);
}
public final String getMetricWidget() {
return metricWidget;
}
public final void setMetricWidget(String metricWidget) {
this.metricWidget = metricWidget;
}
@Override
@Transient
public final Builder metricWidget(String metricWidget) {
this.metricWidget = metricWidget;
return this;
}
public final String getOutputFormat() {
return outputFormat;
}
public final void setOutputFormat(String outputFormat) {
this.outputFormat = outputFormat;
}
@Override
@Transient
public final Builder outputFormat(String outputFormat) {
this.outputFormat = outputFormat;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public GetMetricWidgetImageRequest build() {
return new GetMetricWidgetImageRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}