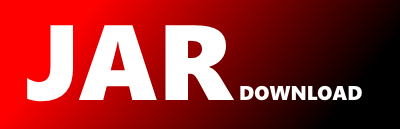
software.amazon.awssdk.services.cloudwatch.model.MetricDataQuery Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* This structure is used in both GetMetricData
and PutMetricAlarm
. The supported use of this
* structure is different for those two operations.
*
*
* When used in GetMetricData
, it indicates the metric data to return, and whether this call is just
* retrieving a batch set of data for one metric, or is performing a math expression on metric data. A single
* GetMetricData
call can include up to 500 MetricDataQuery
structures.
*
*
* When used in PutMetricAlarm
, it enables you to create an alarm based on a metric math expression. Each
* MetricDataQuery
in the array specifies either a metric to retrieve, or a math expression to be performed
* on retrieved metrics. A single PutMetricAlarm
call can include up to 20 MetricDataQuery
* structures in the array. The 20 structures can include as many as 10 structures that contain a
* MetricStat
parameter to retrieve a metric, and as many as 10 structures that contain the
* Expression
parameter to perform a math expression. Of those Expression
structures, one must
* have True
as the value for ReturnData
. The result of this expression is the value the alarm
* watches.
*
*
* Any expression used in a PutMetricAlarm
operation must return a single time series. For more
* information, see Metric Math Syntax and Functions in the Amazon CloudWatch User Guide.
*
*
* Some of the parameters of this structure also have different uses whether you are using this structure in a
* GetMetricData
operation or a PutMetricAlarm
operation. These differences are explained in
* the following parameter list.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MetricDataQuery implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(MetricDataQuery::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField METRIC_STAT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("MetricStat").getter(getter(MetricDataQuery::metricStat)).setter(setter(Builder::metricStat))
.constructor(MetricStat::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricStat").build()).build();
private static final SdkField EXPRESSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Expression").getter(getter(MetricDataQuery::expression)).setter(setter(Builder::expression))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Expression").build()).build();
private static final SdkField LABEL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Label")
.getter(getter(MetricDataQuery::label)).setter(setter(Builder::label))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Label").build()).build();
private static final SdkField RETURN_DATA_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ReturnData").getter(getter(MetricDataQuery::returnData)).setter(setter(Builder::returnData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReturnData").build()).build();
private static final SdkField PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Period").getter(getter(MetricDataQuery::period)).setter(setter(Builder::period))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Period").build()).build();
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountId").getter(getter(MetricDataQuery::accountId)).setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, METRIC_STAT_FIELD,
EXPRESSION_FIELD, LABEL_FIELD, RETURN_DATA_FIELD, PERIOD_FIELD, ACCOUNT_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final MetricStat metricStat;
private final String expression;
private final String label;
private final Boolean returnData;
private final Integer period;
private final String accountId;
private MetricDataQuery(BuilderImpl builder) {
this.id = builder.id;
this.metricStat = builder.metricStat;
this.expression = builder.expression;
this.label = builder.label;
this.returnData = builder.returnData;
this.period = builder.period;
this.accountId = builder.accountId;
}
/**
*
* A short name used to tie this object to the results in the response. This name must be unique within a single
* call to GetMetricData
. If you are performing math expressions on this set of data, this name
* represents that data and can serve as a variable in the mathematical expression. The valid characters are
* letters, numbers, and underscore. The first character must be a lowercase letter.
*
*
* @return A short name used to tie this object to the results in the response. This name must be unique within a
* single call to GetMetricData
. If you are performing math expressions on this set of data,
* this name represents that data and can serve as a variable in the mathematical expression. The valid
* characters are letters, numbers, and underscore. The first character must be a lowercase letter.
*/
public final String id() {
return id;
}
/**
*
* The metric to be returned, along with statistics, period, and units. Use this parameter only if this object is
* retrieving a metric and not performing a math expression on returned data.
*
*
* Within one MetricDataQuery object, you must specify either Expression
or MetricStat
but
* not both.
*
*
* @return The metric to be returned, along with statistics, period, and units. Use this parameter only if this
* object is retrieving a metric and not performing a math expression on returned data.
*
* Within one MetricDataQuery object, you must specify either Expression
or
* MetricStat
but not both.
*/
public final MetricStat metricStat() {
return metricStat;
}
/**
*
* The math expression to be performed on the returned data, if this object is performing a math expression. This
* expression can use the Id
of the other metrics to refer to those metrics, and can also use the
* Id
of other expressions to use the result of those expressions. For more information about metric
* math expressions, see Metric Math Syntax and Functions in the Amazon CloudWatch User Guide.
*
*
* Within each MetricDataQuery object, you must specify either Expression
or MetricStat
* but not both.
*
*
* @return The math expression to be performed on the returned data, if this object is performing a math expression.
* This expression can use the Id
of the other metrics to refer to those metrics, and can also
* use the Id
of other expressions to use the result of those expressions. For more information
* about metric math expressions, see Metric Math Syntax and Functions in the Amazon CloudWatch User Guide.
*
* Within each MetricDataQuery object, you must specify either Expression
or
* MetricStat
but not both.
*/
public final String expression() {
return expression;
}
/**
*
* A human-readable label for this metric or expression. This is especially useful if this is an expression, so that
* you know what the value represents. If the metric or expression is shown in a CloudWatch dashboard widget, the
* label is shown. If Label is omitted, CloudWatch generates a default.
*
*
* You can put dynamic expressions into a label, so that it is more descriptive. For more information, see Using Dynamic
* Labels.
*
*
* @return A human-readable label for this metric or expression. This is especially useful if this is an expression,
* so that you know what the value represents. If the metric or expression is shown in a CloudWatch
* dashboard widget, the label is shown. If Label is omitted, CloudWatch generates a default.
*
* You can put dynamic expressions into a label, so that it is more descriptive. For more information, see
* Using
* Dynamic Labels.
*/
public final String label() {
return label;
}
/**
*
* When used in GetMetricData
, this option indicates whether to return the timestamps and raw data
* values of this metric. If you are performing this call just to do math expressions and do not also need the raw
* data returned, you can specify False
. If you omit this, the default of True
is used.
*
*
* When used in PutMetricAlarm
, specify True
for the one expression result to use as the
* alarm. For all other metrics and expressions in the same PutMetricAlarm
operation, specify
* ReturnData
as False.
*
*
* @return When used in GetMetricData
, this option indicates whether to return the timestamps and raw
* data values of this metric. If you are performing this call just to do math expressions and do not also
* need the raw data returned, you can specify False
. If you omit this, the default of
* True
is used.
*
* When used in PutMetricAlarm
, specify True
for the one expression result to use
* as the alarm. For all other metrics and expressions in the same PutMetricAlarm
operation,
* specify ReturnData
as False.
*/
public final Boolean returnData() {
return returnData;
}
/**
*
* The granularity, in seconds, of the returned data points. For metrics with regular resolution, a period can be as
* short as one minute (60 seconds) and must be a multiple of 60. For high-resolution metrics that are collected at
* intervals of less than one minute, the period can be 1, 5, 10, 30, 60, or any multiple of 60. High-resolution
* metrics are those metrics stored by a PutMetricData
operation that includes a
* StorageResolution of 1 second
.
*
*
* @return The granularity, in seconds, of the returned data points. For metrics with regular resolution, a period
* can be as short as one minute (60 seconds) and must be a multiple of 60. For high-resolution metrics that
* are collected at intervals of less than one minute, the period can be 1, 5, 10, 30, 60, or any multiple
* of 60. High-resolution metrics are those metrics stored by a PutMetricData
operation that
* includes a StorageResolution of 1 second
.
*/
public final Integer period() {
return period;
}
/**
*
* The ID of the account where the metrics are located, if this is a cross-account alarm.
*
*
* Use this field only for PutMetricAlarm
operations. It is not used in GetMetricData
* operations.
*
*
* @return The ID of the account where the metrics are located, if this is a cross-account alarm.
*
* Use this field only for PutMetricAlarm
operations. It is not used in
* GetMetricData
operations.
*/
public final String accountId() {
return accountId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(metricStat());
hashCode = 31 * hashCode + Objects.hashCode(expression());
hashCode = 31 * hashCode + Objects.hashCode(label());
hashCode = 31 * hashCode + Objects.hashCode(returnData());
hashCode = 31 * hashCode + Objects.hashCode(period());
hashCode = 31 * hashCode + Objects.hashCode(accountId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MetricDataQuery)) {
return false;
}
MetricDataQuery other = (MetricDataQuery) obj;
return Objects.equals(id(), other.id()) && Objects.equals(metricStat(), other.metricStat())
&& Objects.equals(expression(), other.expression()) && Objects.equals(label(), other.label())
&& Objects.equals(returnData(), other.returnData()) && Objects.equals(period(), other.period())
&& Objects.equals(accountId(), other.accountId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MetricDataQuery").add("Id", id()).add("MetricStat", metricStat())
.add("Expression", expression()).add("Label", label()).add("ReturnData", returnData()).add("Period", period())
.add("AccountId", accountId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "MetricStat":
return Optional.ofNullable(clazz.cast(metricStat()));
case "Expression":
return Optional.ofNullable(clazz.cast(expression()));
case "Label":
return Optional.ofNullable(clazz.cast(label()));
case "ReturnData":
return Optional.ofNullable(clazz.cast(returnData()));
case "Period":
return Optional.ofNullable(clazz.cast(period()));
case "AccountId":
return Optional.ofNullable(clazz.cast(accountId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Within one MetricDataQuery object, you must specify either Expression
or
* MetricStat
but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder metricStat(MetricStat metricStat);
/**
*
* The metric to be returned, along with statistics, period, and units. Use this parameter only if this object
* is retrieving a metric and not performing a math expression on returned data.
*
*
* Within one MetricDataQuery object, you must specify either Expression
or MetricStat
* but not both.
*
* This is a convenience that creates an instance of the {@link MetricStat.Builder} avoiding the need to create
* one manually via {@link MetricStat#builder()}.
*
* When the {@link Consumer} completes, {@link MetricStat.Builder#build()} is called immediately and its result
* is passed to {@link #metricStat(MetricStat)}.
*
* @param metricStat
* a consumer that will call methods on {@link MetricStat.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #metricStat(MetricStat)
*/
default Builder metricStat(Consumer metricStat) {
return metricStat(MetricStat.builder().applyMutation(metricStat).build());
}
/**
*
* The math expression to be performed on the returned data, if this object is performing a math expression.
* This expression can use the Id
of the other metrics to refer to those metrics, and can also use
* the Id
of other expressions to use the result of those expressions. For more information about
* metric math expressions, see Metric Math Syntax and Functions in the Amazon CloudWatch User Guide.
*
*
* Within each MetricDataQuery object, you must specify either Expression
or
* MetricStat
but not both.
*
*
* @param expression
* The math expression to be performed on the returned data, if this object is performing a math
* expression. This expression can use the Id
of the other metrics to refer to those
* metrics, and can also use the Id
of other expressions to use the result of those
* expressions. For more information about metric math expressions, see Metric Math Syntax and Functions in the Amazon CloudWatch User Guide.
*
* Within each MetricDataQuery object, you must specify either Expression
or
* MetricStat
but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder expression(String expression);
/**
*
* A human-readable label for this metric or expression. This is especially useful if this is an expression, so
* that you know what the value represents. If the metric or expression is shown in a CloudWatch dashboard
* widget, the label is shown. If Label is omitted, CloudWatch generates a default.
*
*
* You can put dynamic expressions into a label, so that it is more descriptive. For more information, see Using Dynamic
* Labels.
*
*
* @param label
* A human-readable label for this metric or expression. This is especially useful if this is an
* expression, so that you know what the value represents. If the metric or expression is shown in a
* CloudWatch dashboard widget, the label is shown. If Label is omitted, CloudWatch generates a
* default.
*
* You can put dynamic expressions into a label, so that it is more descriptive. For more information,
* see Using
* Dynamic Labels.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder label(String label);
/**
*
* When used in GetMetricData
, this option indicates whether to return the timestamps and raw data
* values of this metric. If you are performing this call just to do math expressions and do not also need the
* raw data returned, you can specify False
. If you omit this, the default of True
is
* used.
*
*
* When used in PutMetricAlarm
, specify True
for the one expression result to use as
* the alarm. For all other metrics and expressions in the same PutMetricAlarm
operation, specify
* ReturnData
as False.
*
*
* @param returnData
* When used in GetMetricData
, this option indicates whether to return the timestamps and
* raw data values of this metric. If you are performing this call just to do math expressions and do not
* also need the raw data returned, you can specify False
. If you omit this, the default of
* True
is used.
*
* When used in PutMetricAlarm
, specify True
for the one expression result to
* use as the alarm. For all other metrics and expressions in the same PutMetricAlarm
* operation, specify ReturnData
as False.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder returnData(Boolean returnData);
/**
*
* The granularity, in seconds, of the returned data points. For metrics with regular resolution, a period can
* be as short as one minute (60 seconds) and must be a multiple of 60. For high-resolution metrics that are
* collected at intervals of less than one minute, the period can be 1, 5, 10, 30, 60, or any multiple of 60.
* High-resolution metrics are those metrics stored by a PutMetricData
operation that includes a
* StorageResolution of 1 second
.
*
*
* @param period
* The granularity, in seconds, of the returned data points. For metrics with regular resolution, a
* period can be as short as one minute (60 seconds) and must be a multiple of 60. For high-resolution
* metrics that are collected at intervals of less than one minute, the period can be 1, 5, 10, 30, 60,
* or any multiple of 60. High-resolution metrics are those metrics stored by a
* PutMetricData
operation that includes a StorageResolution of 1 second
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder period(Integer period);
/**
*
* The ID of the account where the metrics are located, if this is a cross-account alarm.
*
*
* Use this field only for PutMetricAlarm
operations. It is not used in GetMetricData
* operations.
*
*
* @param accountId
* The ID of the account where the metrics are located, if this is a cross-account alarm.
*
* Use this field only for PutMetricAlarm
operations. It is not used in
* GetMetricData
operations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder accountId(String accountId);
}
static final class BuilderImpl implements Builder {
private String id;
private MetricStat metricStat;
private String expression;
private String label;
private Boolean returnData;
private Integer period;
private String accountId;
private BuilderImpl() {
}
private BuilderImpl(MetricDataQuery model) {
id(model.id);
metricStat(model.metricStat);
expression(model.expression);
label(model.label);
returnData(model.returnData);
period(model.period);
accountId(model.accountId);
}
public final String getId() {
return id;
}
public final void setId(String id) {
this.id = id;
}
@Override
@Transient
public final Builder id(String id) {
this.id = id;
return this;
}
public final MetricStat.Builder getMetricStat() {
return metricStat != null ? metricStat.toBuilder() : null;
}
public final void setMetricStat(MetricStat.BuilderImpl metricStat) {
this.metricStat = metricStat != null ? metricStat.build() : null;
}
@Override
@Transient
public final Builder metricStat(MetricStat metricStat) {
this.metricStat = metricStat;
return this;
}
public final String getExpression() {
return expression;
}
public final void setExpression(String expression) {
this.expression = expression;
}
@Override
@Transient
public final Builder expression(String expression) {
this.expression = expression;
return this;
}
public final String getLabel() {
return label;
}
public final void setLabel(String label) {
this.label = label;
}
@Override
@Transient
public final Builder label(String label) {
this.label = label;
return this;
}
public final Boolean getReturnData() {
return returnData;
}
public final void setReturnData(Boolean returnData) {
this.returnData = returnData;
}
@Override
@Transient
public final Builder returnData(Boolean returnData) {
this.returnData = returnData;
return this;
}
public final Integer getPeriod() {
return period;
}
public final void setPeriod(Integer period) {
this.period = period;
}
@Override
@Transient
public final Builder period(Integer period) {
this.period = period;
return this;
}
public final String getAccountId() {
return accountId;
}
public final void setAccountId(String accountId) {
this.accountId = accountId;
}
@Override
@Transient
public final Builder accountId(String accountId) {
this.accountId = accountId;
return this;
}
@Override
public MetricDataQuery build() {
return new MetricDataQuery(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}