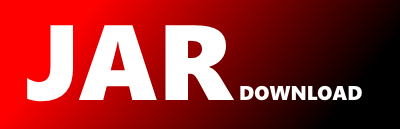
software.amazon.awssdk.services.cloudwatch.model.CompositeAlarm Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details about a composite alarm.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CompositeAlarm implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACTIONS_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ActionsEnabled").getter(getter(CompositeAlarm::actionsEnabled)).setter(setter(Builder::actionsEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsEnabled").build()).build();
private static final SdkField> ALARM_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AlarmActions")
.getter(getter(CompositeAlarm::alarmActions))
.setter(setter(Builder::alarmActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ALARM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmArn").getter(getter(CompositeAlarm::alarmArn)).setter(setter(Builder::alarmArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmArn").build()).build();
private static final SdkField ALARM_CONFIGURATION_UPDATED_TIMESTAMP_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("AlarmConfigurationUpdatedTimestamp")
.getter(getter(CompositeAlarm::alarmConfigurationUpdatedTimestamp))
.setter(setter(Builder::alarmConfigurationUpdatedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmConfigurationUpdatedTimestamp")
.build()).build();
private static final SdkField ALARM_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmDescription").getter(getter(CompositeAlarm::alarmDescription))
.setter(setter(Builder::alarmDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmDescription").build()).build();
private static final SdkField ALARM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmName").getter(getter(CompositeAlarm::alarmName)).setter(setter(Builder::alarmName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmName").build()).build();
private static final SdkField ALARM_RULE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmRule").getter(getter(CompositeAlarm::alarmRule)).setter(setter(Builder::alarmRule))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmRule").build()).build();
private static final SdkField> INSUFFICIENT_DATA_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InsufficientDataActions")
.getter(getter(CompositeAlarm::insufficientDataActions))
.setter(setter(Builder::insufficientDataActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InsufficientDataActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OK_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OKActions")
.getter(getter(CompositeAlarm::okActions))
.setter(setter(Builder::okActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OKActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StateReason").getter(getter(CompositeAlarm::stateReason)).setter(setter(Builder::stateReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateReason").build()).build();
private static final SdkField STATE_REASON_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StateReasonData").getter(getter(CompositeAlarm::stateReasonData))
.setter(setter(Builder::stateReasonData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateReasonData").build()).build();
private static final SdkField STATE_UPDATED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StateUpdatedTimestamp").getter(getter(CompositeAlarm::stateUpdatedTimestamp))
.setter(setter(Builder::stateUpdatedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateUpdatedTimestamp").build())
.build();
private static final SdkField STATE_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StateValue").getter(getter(CompositeAlarm::stateValueAsString)).setter(setter(Builder::stateValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateValue").build()).build();
private static final SdkField STATE_TRANSITIONED_TIMESTAMP_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("StateTransitionedTimestamp")
.getter(getter(CompositeAlarm::stateTransitionedTimestamp))
.setter(setter(Builder::stateTransitionedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateTransitionedTimestamp").build())
.build();
private static final SdkField ACTIONS_SUPPRESSED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActionsSuppressedBy").getter(getter(CompositeAlarm::actionsSuppressedByAsString))
.setter(setter(Builder::actionsSuppressedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressedBy").build())
.build();
private static final SdkField ACTIONS_SUPPRESSED_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActionsSuppressedReason").getter(getter(CompositeAlarm::actionsSuppressedReason))
.setter(setter(Builder::actionsSuppressedReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressedReason").build())
.build();
private static final SdkField ACTIONS_SUPPRESSOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActionsSuppressor").getter(getter(CompositeAlarm::actionsSuppressor))
.setter(setter(Builder::actionsSuppressor))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressor").build()).build();
private static final SdkField ACTIONS_SUPPRESSOR_WAIT_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ActionsSuppressorWaitPeriod")
.getter(getter(CompositeAlarm::actionsSuppressorWaitPeriod))
.setter(setter(Builder::actionsSuppressorWaitPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressorWaitPeriod")
.build()).build();
private static final SdkField ACTIONS_SUPPRESSOR_EXTENSION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ActionsSuppressorExtensionPeriod")
.getter(getter(CompositeAlarm::actionsSuppressorExtensionPeriod))
.setter(setter(Builder::actionsSuppressorExtensionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressorExtensionPeriod")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACTIONS_ENABLED_FIELD,
ALARM_ACTIONS_FIELD, ALARM_ARN_FIELD, ALARM_CONFIGURATION_UPDATED_TIMESTAMP_FIELD, ALARM_DESCRIPTION_FIELD,
ALARM_NAME_FIELD, ALARM_RULE_FIELD, INSUFFICIENT_DATA_ACTIONS_FIELD, OK_ACTIONS_FIELD, STATE_REASON_FIELD,
STATE_REASON_DATA_FIELD, STATE_UPDATED_TIMESTAMP_FIELD, STATE_VALUE_FIELD, STATE_TRANSITIONED_TIMESTAMP_FIELD,
ACTIONS_SUPPRESSED_BY_FIELD, ACTIONS_SUPPRESSED_REASON_FIELD, ACTIONS_SUPPRESSOR_FIELD,
ACTIONS_SUPPRESSOR_WAIT_PERIOD_FIELD, ACTIONS_SUPPRESSOR_EXTENSION_PERIOD_FIELD));
private static final long serialVersionUID = 1L;
private final Boolean actionsEnabled;
private final List alarmActions;
private final String alarmArn;
private final Instant alarmConfigurationUpdatedTimestamp;
private final String alarmDescription;
private final String alarmName;
private final String alarmRule;
private final List insufficientDataActions;
private final List okActions;
private final String stateReason;
private final String stateReasonData;
private final Instant stateUpdatedTimestamp;
private final String stateValue;
private final Instant stateTransitionedTimestamp;
private final String actionsSuppressedBy;
private final String actionsSuppressedReason;
private final String actionsSuppressor;
private final Integer actionsSuppressorWaitPeriod;
private final Integer actionsSuppressorExtensionPeriod;
private CompositeAlarm(BuilderImpl builder) {
this.actionsEnabled = builder.actionsEnabled;
this.alarmActions = builder.alarmActions;
this.alarmArn = builder.alarmArn;
this.alarmConfigurationUpdatedTimestamp = builder.alarmConfigurationUpdatedTimestamp;
this.alarmDescription = builder.alarmDescription;
this.alarmName = builder.alarmName;
this.alarmRule = builder.alarmRule;
this.insufficientDataActions = builder.insufficientDataActions;
this.okActions = builder.okActions;
this.stateReason = builder.stateReason;
this.stateReasonData = builder.stateReasonData;
this.stateUpdatedTimestamp = builder.stateUpdatedTimestamp;
this.stateValue = builder.stateValue;
this.stateTransitionedTimestamp = builder.stateTransitionedTimestamp;
this.actionsSuppressedBy = builder.actionsSuppressedBy;
this.actionsSuppressedReason = builder.actionsSuppressedReason;
this.actionsSuppressor = builder.actionsSuppressor;
this.actionsSuppressorWaitPeriod = builder.actionsSuppressorWaitPeriod;
this.actionsSuppressorExtensionPeriod = builder.actionsSuppressorExtensionPeriod;
}
/**
*
* Indicates whether actions should be executed during any changes to the alarm state.
*
*
* @return Indicates whether actions should be executed during any changes to the alarm state.
*/
public final Boolean actionsEnabled() {
return actionsEnabled;
}
/**
* For responses, this returns true if the service returned a value for the AlarmActions property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAlarmActions() {
return alarmActions != null && !(alarmActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to the ALARM state from any other state. Each action is
* specified as an Amazon Resource Name (ARN).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAlarmActions} method.
*
*
* @return The actions to execute when this alarm transitions to the ALARM state from any other state. Each action
* is specified as an Amazon Resource Name (ARN).
*/
public final List alarmActions() {
return alarmActions;
}
/**
*
* The Amazon Resource Name (ARN) of the alarm.
*
*
* @return The Amazon Resource Name (ARN) of the alarm.
*/
public final String alarmArn() {
return alarmArn;
}
/**
*
* The time stamp of the last update to the alarm configuration.
*
*
* @return The time stamp of the last update to the alarm configuration.
*/
public final Instant alarmConfigurationUpdatedTimestamp() {
return alarmConfigurationUpdatedTimestamp;
}
/**
*
* The description of the alarm.
*
*
* @return The description of the alarm.
*/
public final String alarmDescription() {
return alarmDescription;
}
/**
*
* The name of the alarm.
*
*
* @return The name of the alarm.
*/
public final String alarmName() {
return alarmName;
}
/**
*
* The rule that this alarm uses to evaluate its alarm state.
*
*
* @return The rule that this alarm uses to evaluate its alarm state.
*/
public final String alarmRule() {
return alarmRule;
}
/**
* For responses, this returns true if the service returned a value for the InsufficientDataActions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasInsufficientDataActions() {
return insufficientDataActions != null && !(insufficientDataActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInsufficientDataActions} method.
*
*
* @return The actions to execute when this alarm transitions to the INSUFFICIENT_DATA state from any other state.
* Each action is specified as an Amazon Resource Name (ARN).
*/
public final List insufficientDataActions() {
return insufficientDataActions;
}
/**
* For responses, this returns true if the service returned a value for the OKActions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasOkActions() {
return okActions != null && !(okActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to the OK state from any other state. Each action is specified
* as an Amazon Resource Name (ARN).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOkActions} method.
*
*
* @return The actions to execute when this alarm transitions to the OK state from any other state. Each action is
* specified as an Amazon Resource Name (ARN).
*/
public final List okActions() {
return okActions;
}
/**
*
* An explanation for the alarm state, in text format.
*
*
* @return An explanation for the alarm state, in text format.
*/
public final String stateReason() {
return stateReason;
}
/**
*
* An explanation for the alarm state, in JSON format.
*
*
* @return An explanation for the alarm state, in JSON format.
*/
public final String stateReasonData() {
return stateReasonData;
}
/**
*
* Tracks the timestamp of any state update, even if StateValue
doesn't change.
*
*
* @return Tracks the timestamp of any state update, even if StateValue
doesn't change.
*/
public final Instant stateUpdatedTimestamp() {
return stateUpdatedTimestamp;
}
/**
*
* The state value for the alarm.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stateValue} will
* return {@link StateValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateValueAsString}.
*
*
* @return The state value for the alarm.
* @see StateValue
*/
public final StateValue stateValue() {
return StateValue.fromValue(stateValue);
}
/**
*
* The state value for the alarm.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stateValue} will
* return {@link StateValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateValueAsString}.
*
*
* @return The state value for the alarm.
* @see StateValue
*/
public final String stateValueAsString() {
return stateValue;
}
/**
*
* The timestamp of the last change to the alarm's StateValue
.
*
*
* @return The timestamp of the last change to the alarm's StateValue
.
*/
public final Instant stateTransitionedTimestamp() {
return stateTransitionedTimestamp;
}
/**
*
* When the value is ALARM
, it means that the actions are suppressed because the suppressor alarm is in
* ALARM
When the value is WaitPeriod
, it means that the actions are suppressed because
* the composite alarm is waiting for the suppressor alarm to go into into the ALARM
state. The maximum
* waiting time is as specified in ActionsSuppressorWaitPeriod
. After this time, the composite alarm
* performs its actions. When the value is ExtensionPeriod
, it means that the actions are suppressed
* because the composite alarm is waiting after the suppressor alarm went out of the ALARM
state. The
* maximum waiting time is as specified in ActionsSuppressorExtensionPeriod
. After this time, the
* composite alarm performs its actions.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #actionsSuppressedBy} will return {@link ActionsSuppressedBy#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #actionsSuppressedByAsString}.
*
*
* @return When the value is ALARM
, it means that the actions are suppressed because the suppressor
* alarm is in ALARM
When the value is WaitPeriod
, it means that the actions are
* suppressed because the composite alarm is waiting for the suppressor alarm to go into into the
* ALARM
state. The maximum waiting time is as specified in
* ActionsSuppressorWaitPeriod
. After this time, the composite alarm performs its actions. When
* the value is ExtensionPeriod
, it means that the actions are suppressed because the composite
* alarm is waiting after the suppressor alarm went out of the ALARM
state. The maximum waiting
* time is as specified in ActionsSuppressorExtensionPeriod
. After this time, the composite
* alarm performs its actions.
* @see ActionsSuppressedBy
*/
public final ActionsSuppressedBy actionsSuppressedBy() {
return ActionsSuppressedBy.fromValue(actionsSuppressedBy);
}
/**
*
* When the value is ALARM
, it means that the actions are suppressed because the suppressor alarm is in
* ALARM
When the value is WaitPeriod
, it means that the actions are suppressed because
* the composite alarm is waiting for the suppressor alarm to go into into the ALARM
state. The maximum
* waiting time is as specified in ActionsSuppressorWaitPeriod
. After this time, the composite alarm
* performs its actions. When the value is ExtensionPeriod
, it means that the actions are suppressed
* because the composite alarm is waiting after the suppressor alarm went out of the ALARM
state. The
* maximum waiting time is as specified in ActionsSuppressorExtensionPeriod
. After this time, the
* composite alarm performs its actions.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #actionsSuppressedBy} will return {@link ActionsSuppressedBy#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #actionsSuppressedByAsString}.
*
*
* @return When the value is ALARM
, it means that the actions are suppressed because the suppressor
* alarm is in ALARM
When the value is WaitPeriod
, it means that the actions are
* suppressed because the composite alarm is waiting for the suppressor alarm to go into into the
* ALARM
state. The maximum waiting time is as specified in
* ActionsSuppressorWaitPeriod
. After this time, the composite alarm performs its actions. When
* the value is ExtensionPeriod
, it means that the actions are suppressed because the composite
* alarm is waiting after the suppressor alarm went out of the ALARM
state. The maximum waiting
* time is as specified in ActionsSuppressorExtensionPeriod
. After this time, the composite
* alarm performs its actions.
* @see ActionsSuppressedBy
*/
public final String actionsSuppressedByAsString() {
return actionsSuppressedBy;
}
/**
*
* Captures the reason for action suppression.
*
*
* @return Captures the reason for action suppression.
*/
public final String actionsSuppressedReason() {
return actionsSuppressedReason;
}
/**
*
* Actions will be suppressed if the suppressor alarm is in the ALARM
state.
* ActionsSuppressor
can be an AlarmName or an Amazon Resource Name (ARN) from an existing alarm.
*
*
* @return Actions will be suppressed if the suppressor alarm is in the ALARM
state.
* ActionsSuppressor
can be an AlarmName or an Amazon Resource Name (ARN) from an existing
* alarm.
*/
public final String actionsSuppressor() {
return actionsSuppressor;
}
/**
*
* The maximum time in seconds that the composite alarm waits for the suppressor alarm to go into the
* ALARM
state. After this time, the composite alarm performs its actions.
*
*
*
* WaitPeriod
is required only when ActionsSuppressor
is specified.
*
*
*
* @return The maximum time in seconds that the composite alarm waits for the suppressor alarm to go into the
* ALARM
state. After this time, the composite alarm performs its actions.
*
* WaitPeriod
is required only when ActionsSuppressor
is specified.
*
*/
public final Integer actionsSuppressorWaitPeriod() {
return actionsSuppressorWaitPeriod;
}
/**
*
* The maximum time in seconds that the composite alarm waits after suppressor alarm goes out of the
* ALARM
state. After this time, the composite alarm performs its actions.
*
*
*
* ExtensionPeriod
is required only when ActionsSuppressor
is specified.
*
*
*
* @return The maximum time in seconds that the composite alarm waits after suppressor alarm goes out of the
* ALARM
state. After this time, the composite alarm performs its actions.
*
* ExtensionPeriod
is required only when ActionsSuppressor
is specified.
*
*/
public final Integer actionsSuppressorExtensionPeriod() {
return actionsSuppressorExtensionPeriod;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(actionsEnabled());
hashCode = 31 * hashCode + Objects.hashCode(hasAlarmActions() ? alarmActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(alarmArn());
hashCode = 31 * hashCode + Objects.hashCode(alarmConfigurationUpdatedTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(alarmDescription());
hashCode = 31 * hashCode + Objects.hashCode(alarmName());
hashCode = 31 * hashCode + Objects.hashCode(alarmRule());
hashCode = 31 * hashCode + Objects.hashCode(hasInsufficientDataActions() ? insufficientDataActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasOkActions() ? okActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(stateReason());
hashCode = 31 * hashCode + Objects.hashCode(stateReasonData());
hashCode = 31 * hashCode + Objects.hashCode(stateUpdatedTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(stateValueAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateTransitionedTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressedByAsString());
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressedReason());
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressor());
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressorWaitPeriod());
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressorExtensionPeriod());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CompositeAlarm)) {
return false;
}
CompositeAlarm other = (CompositeAlarm) obj;
return Objects.equals(actionsEnabled(), other.actionsEnabled()) && hasAlarmActions() == other.hasAlarmActions()
&& Objects.equals(alarmActions(), other.alarmActions()) && Objects.equals(alarmArn(), other.alarmArn())
&& Objects.equals(alarmConfigurationUpdatedTimestamp(), other.alarmConfigurationUpdatedTimestamp())
&& Objects.equals(alarmDescription(), other.alarmDescription()) && Objects.equals(alarmName(), other.alarmName())
&& Objects.equals(alarmRule(), other.alarmRule())
&& hasInsufficientDataActions() == other.hasInsufficientDataActions()
&& Objects.equals(insufficientDataActions(), other.insufficientDataActions())
&& hasOkActions() == other.hasOkActions() && Objects.equals(okActions(), other.okActions())
&& Objects.equals(stateReason(), other.stateReason())
&& Objects.equals(stateReasonData(), other.stateReasonData())
&& Objects.equals(stateUpdatedTimestamp(), other.stateUpdatedTimestamp())
&& Objects.equals(stateValueAsString(), other.stateValueAsString())
&& Objects.equals(stateTransitionedTimestamp(), other.stateTransitionedTimestamp())
&& Objects.equals(actionsSuppressedByAsString(), other.actionsSuppressedByAsString())
&& Objects.equals(actionsSuppressedReason(), other.actionsSuppressedReason())
&& Objects.equals(actionsSuppressor(), other.actionsSuppressor())
&& Objects.equals(actionsSuppressorWaitPeriod(), other.actionsSuppressorWaitPeriod())
&& Objects.equals(actionsSuppressorExtensionPeriod(), other.actionsSuppressorExtensionPeriod());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CompositeAlarm").add("ActionsEnabled", actionsEnabled())
.add("AlarmActions", hasAlarmActions() ? alarmActions() : null).add("AlarmArn", alarmArn())
.add("AlarmConfigurationUpdatedTimestamp", alarmConfigurationUpdatedTimestamp())
.add("AlarmDescription", alarmDescription()).add("AlarmName", alarmName()).add("AlarmRule", alarmRule())
.add("InsufficientDataActions", hasInsufficientDataActions() ? insufficientDataActions() : null)
.add("OKActions", hasOkActions() ? okActions() : null).add("StateReason", stateReason())
.add("StateReasonData", stateReasonData()).add("StateUpdatedTimestamp", stateUpdatedTimestamp())
.add("StateValue", stateValueAsString()).add("StateTransitionedTimestamp", stateTransitionedTimestamp())
.add("ActionsSuppressedBy", actionsSuppressedByAsString())
.add("ActionsSuppressedReason", actionsSuppressedReason()).add("ActionsSuppressor", actionsSuppressor())
.add("ActionsSuppressorWaitPeriod", actionsSuppressorWaitPeriod())
.add("ActionsSuppressorExtensionPeriod", actionsSuppressorExtensionPeriod()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ActionsEnabled":
return Optional.ofNullable(clazz.cast(actionsEnabled()));
case "AlarmActions":
return Optional.ofNullable(clazz.cast(alarmActions()));
case "AlarmArn":
return Optional.ofNullable(clazz.cast(alarmArn()));
case "AlarmConfigurationUpdatedTimestamp":
return Optional.ofNullable(clazz.cast(alarmConfigurationUpdatedTimestamp()));
case "AlarmDescription":
return Optional.ofNullable(clazz.cast(alarmDescription()));
case "AlarmName":
return Optional.ofNullable(clazz.cast(alarmName()));
case "AlarmRule":
return Optional.ofNullable(clazz.cast(alarmRule()));
case "InsufficientDataActions":
return Optional.ofNullable(clazz.cast(insufficientDataActions()));
case "OKActions":
return Optional.ofNullable(clazz.cast(okActions()));
case "StateReason":
return Optional.ofNullable(clazz.cast(stateReason()));
case "StateReasonData":
return Optional.ofNullable(clazz.cast(stateReasonData()));
case "StateUpdatedTimestamp":
return Optional.ofNullable(clazz.cast(stateUpdatedTimestamp()));
case "StateValue":
return Optional.ofNullable(clazz.cast(stateValueAsString()));
case "StateTransitionedTimestamp":
return Optional.ofNullable(clazz.cast(stateTransitionedTimestamp()));
case "ActionsSuppressedBy":
return Optional.ofNullable(clazz.cast(actionsSuppressedByAsString()));
case "ActionsSuppressedReason":
return Optional.ofNullable(clazz.cast(actionsSuppressedReason()));
case "ActionsSuppressor":
return Optional.ofNullable(clazz.cast(actionsSuppressor()));
case "ActionsSuppressorWaitPeriod":
return Optional.ofNullable(clazz.cast(actionsSuppressorWaitPeriod()));
case "ActionsSuppressorExtensionPeriod":
return Optional.ofNullable(clazz.cast(actionsSuppressorExtensionPeriod()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function