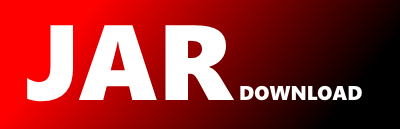
software.amazon.awssdk.services.cloudwatch.model.PutMetricAlarmRequest Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutMetricAlarmRequest extends CloudWatchRequest implements
ToCopyableBuilder {
private static final SdkField ALARM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmName").getter(getter(PutMetricAlarmRequest::alarmName)).setter(setter(Builder::alarmName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmName").build()).build();
private static final SdkField ALARM_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmDescription").getter(getter(PutMetricAlarmRequest::alarmDescription))
.setter(setter(Builder::alarmDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmDescription").build()).build();
private static final SdkField ACTIONS_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ActionsEnabled").getter(getter(PutMetricAlarmRequest::actionsEnabled))
.setter(setter(Builder::actionsEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsEnabled").build()).build();
private static final SdkField> OK_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OKActions")
.getter(getter(PutMetricAlarmRequest::okActions))
.setter(setter(Builder::okActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OKActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ALARM_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AlarmActions")
.getter(getter(PutMetricAlarmRequest::alarmActions))
.setter(setter(Builder::alarmActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> INSUFFICIENT_DATA_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InsufficientDataActions")
.getter(getter(PutMetricAlarmRequest::insufficientDataActions))
.setter(setter(Builder::insufficientDataActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InsufficientDataActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField METRIC_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetricName").getter(getter(PutMetricAlarmRequest::metricName)).setter(setter(Builder::metricName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricName").build()).build();
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Namespace").getter(getter(PutMetricAlarmRequest::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Namespace").build()).build();
private static final SdkField STATISTIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Statistic").getter(getter(PutMetricAlarmRequest::statisticAsString)).setter(setter(Builder::statistic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Statistic").build()).build();
private static final SdkField EXTENDED_STATISTIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExtendedStatistic").getter(getter(PutMetricAlarmRequest::extendedStatistic))
.setter(setter(Builder::extendedStatistic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExtendedStatistic").build()).build();
private static final SdkField> DIMENSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Dimensions")
.getter(getter(PutMetricAlarmRequest::dimensions))
.setter(setter(Builder::dimensions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Dimensions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Dimension::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Period").getter(getter(PutMetricAlarmRequest::period)).setter(setter(Builder::period))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Period").build()).build();
private static final SdkField UNIT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Unit")
.getter(getter(PutMetricAlarmRequest::unitAsString)).setter(setter(Builder::unit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Unit").build()).build();
private static final SdkField EVALUATION_PERIODS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EvaluationPeriods").getter(getter(PutMetricAlarmRequest::evaluationPeriods))
.setter(setter(Builder::evaluationPeriods))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluationPeriods").build()).build();
private static final SdkField DATAPOINTS_TO_ALARM_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("DatapointsToAlarm").getter(getter(PutMetricAlarmRequest::datapointsToAlarm))
.setter(setter(Builder::datapointsToAlarm))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatapointsToAlarm").build()).build();
private static final SdkField THRESHOLD_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("Threshold").getter(getter(PutMetricAlarmRequest::threshold)).setter(setter(Builder::threshold))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Threshold").build()).build();
private static final SdkField COMPARISON_OPERATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ComparisonOperator").getter(getter(PutMetricAlarmRequest::comparisonOperatorAsString))
.setter(setter(Builder::comparisonOperator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComparisonOperator").build())
.build();
private static final SdkField TREAT_MISSING_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TreatMissingData").getter(getter(PutMetricAlarmRequest::treatMissingData))
.setter(setter(Builder::treatMissingData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TreatMissingData").build()).build();
private static final SdkField EVALUATE_LOW_SAMPLE_COUNT_PERCENTILE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EvaluateLowSampleCountPercentile")
.getter(getter(PutMetricAlarmRequest::evaluateLowSampleCountPercentile))
.setter(setter(Builder::evaluateLowSampleCountPercentile))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluateLowSampleCountPercentile")
.build()).build();
private static final SdkField> METRICS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Metrics")
.getter(getter(PutMetricAlarmRequest::metrics))
.setter(setter(Builder::metrics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Metrics").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricDataQuery::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(PutMetricAlarmRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField THRESHOLD_METRIC_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ThresholdMetricId").getter(getter(PutMetricAlarmRequest::thresholdMetricId))
.setter(setter(Builder::thresholdMetricId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ThresholdMetricId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ALARM_NAME_FIELD,
ALARM_DESCRIPTION_FIELD, ACTIONS_ENABLED_FIELD, OK_ACTIONS_FIELD, ALARM_ACTIONS_FIELD,
INSUFFICIENT_DATA_ACTIONS_FIELD, METRIC_NAME_FIELD, NAMESPACE_FIELD, STATISTIC_FIELD, EXTENDED_STATISTIC_FIELD,
DIMENSIONS_FIELD, PERIOD_FIELD, UNIT_FIELD, EVALUATION_PERIODS_FIELD, DATAPOINTS_TO_ALARM_FIELD, THRESHOLD_FIELD,
COMPARISON_OPERATOR_FIELD, TREAT_MISSING_DATA_FIELD, EVALUATE_LOW_SAMPLE_COUNT_PERCENTILE_FIELD, METRICS_FIELD,
TAGS_FIELD, THRESHOLD_METRIC_ID_FIELD));
private final String alarmName;
private final String alarmDescription;
private final Boolean actionsEnabled;
private final List okActions;
private final List alarmActions;
private final List insufficientDataActions;
private final String metricName;
private final String namespace;
private final String statistic;
private final String extendedStatistic;
private final List dimensions;
private final Integer period;
private final String unit;
private final Integer evaluationPeriods;
private final Integer datapointsToAlarm;
private final Double threshold;
private final String comparisonOperator;
private final String treatMissingData;
private final String evaluateLowSampleCountPercentile;
private final List metrics;
private final List tags;
private final String thresholdMetricId;
private PutMetricAlarmRequest(BuilderImpl builder) {
super(builder);
this.alarmName = builder.alarmName;
this.alarmDescription = builder.alarmDescription;
this.actionsEnabled = builder.actionsEnabled;
this.okActions = builder.okActions;
this.alarmActions = builder.alarmActions;
this.insufficientDataActions = builder.insufficientDataActions;
this.metricName = builder.metricName;
this.namespace = builder.namespace;
this.statistic = builder.statistic;
this.extendedStatistic = builder.extendedStatistic;
this.dimensions = builder.dimensions;
this.period = builder.period;
this.unit = builder.unit;
this.evaluationPeriods = builder.evaluationPeriods;
this.datapointsToAlarm = builder.datapointsToAlarm;
this.threshold = builder.threshold;
this.comparisonOperator = builder.comparisonOperator;
this.treatMissingData = builder.treatMissingData;
this.evaluateLowSampleCountPercentile = builder.evaluateLowSampleCountPercentile;
this.metrics = builder.metrics;
this.tags = builder.tags;
this.thresholdMetricId = builder.thresholdMetricId;
}
/**
*
* The name for the alarm. This name must be unique within the Region.
*
*
* @return The name for the alarm. This name must be unique within the Region.
*/
public final String alarmName() {
return alarmName;
}
/**
*
* The description for the alarm.
*
*
* @return The description for the alarm.
*/
public final String alarmDescription() {
return alarmDescription;
}
/**
*
* Indicates whether actions should be executed during any changes to the alarm state. The default is
* TRUE
.
*
*
* @return Indicates whether actions should be executed during any changes to the alarm state. The default is
* TRUE
.
*/
public final Boolean actionsEnabled() {
return actionsEnabled;
}
/**
* For responses, this returns true if the service returned a value for the OKActions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasOkActions() {
return okActions != null && !(okActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to an OK
state from any other state. Each action
* is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
| arn:aws:automate:region:ec2:reboot
* | arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOkActions} method.
*
*
* @return The actions to execute when this alarm transitions to an OK
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* | arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
*/
public final List okActions() {
return okActions;
}
/**
* For responses, this returns true if the service returned a value for the AlarmActions property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAlarmActions() {
return alarmActions != null && !(alarmActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to the ALARM
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
| arn:aws:automate:region:ec2:reboot
* | arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
* | arn:aws:ssm:region:account-id:opsitem:severity
|
* arn:aws:ssm-incidents::account-id:response-plan:response-plan-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAlarmActions} method.
*
*
* @return The actions to execute when this alarm transitions to the ALARM
state from any other state.
* Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
* | arn:aws:ssm:region:account-id:opsitem:severity
|
* arn:aws:ssm-incidents::account-id:response-plan:response-plan-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* | arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
*/
public final List alarmActions() {
return alarmActions;
}
/**
* For responses, this returns true if the service returned a value for the InsufficientDataActions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasInsufficientDataActions() {
return insufficientDataActions != null && !(insufficientDataActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any other
* state. Each action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
| arn:aws:automate:region:ec2:reboot
* | arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* >arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInsufficientDataActions} method.
*
*
* @return The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any
* other state. Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* >arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* | arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
*/
public final List insufficientDataActions() {
return insufficientDataActions;
}
/**
*
* The name for the metric associated with the alarm. For each PutMetricAlarm
operation, you must
* specify either MetricName
or a Metrics
array.
*
*
* If you are creating an alarm based on a math expression, you cannot specify this parameter, or any of the
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters. Instead, you specify all this information in the Metrics
* array.
*
*
* @return The name for the metric associated with the alarm. For each PutMetricAlarm
operation, you
* must specify either MetricName
or a Metrics
array.
*
* If you are creating an alarm based on a math expression, you cannot specify this parameter, or any of the
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters. Instead, you specify all this information in the
* Metrics
array.
*/
public final String metricName() {
return metricName;
}
/**
*
* The namespace for the metric associated specified in MetricName
.
*
*
* @return The namespace for the metric associated specified in MetricName
.
*/
public final String namespace() {
return namespace;
}
/**
*
* The statistic for the metric specified in MetricName
, other than percentile. For percentile
* statistics, use ExtendedStatistic
. When you call PutMetricAlarm
and specify a
* MetricName
, you must specify either Statistic
or ExtendedStatistic,
but
* not both.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statistic} will
* return {@link Statistic#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statisticAsString}.
*
*
* @return The statistic for the metric specified in MetricName
, other than percentile. For percentile
* statistics, use ExtendedStatistic
. When you call PutMetricAlarm
and specify a
* MetricName
, you must specify either Statistic
or
* ExtendedStatistic,
but not both.
* @see Statistic
*/
public final Statistic statistic() {
return Statistic.fromValue(statistic);
}
/**
*
* The statistic for the metric specified in MetricName
, other than percentile. For percentile
* statistics, use ExtendedStatistic
. When you call PutMetricAlarm
and specify a
* MetricName
, you must specify either Statistic
or ExtendedStatistic,
but
* not both.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statistic} will
* return {@link Statistic#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statisticAsString}.
*
*
* @return The statistic for the metric specified in MetricName
, other than percentile. For percentile
* statistics, use ExtendedStatistic
. When you call PutMetricAlarm
and specify a
* MetricName
, you must specify either Statistic
or
* ExtendedStatistic,
but not both.
* @see Statistic
*/
public final String statisticAsString() {
return statistic;
}
/**
*
* The percentile statistic for the metric specified in MetricName
. Specify a value between p0.0 and
* p100. When you call PutMetricAlarm
and specify a MetricName
, you must specify either
* Statistic
or ExtendedStatistic,
but not both.
*
*
* @return The percentile statistic for the metric specified in MetricName
. Specify a value between
* p0.0 and p100. When you call PutMetricAlarm
and specify a MetricName
, you must
* specify either Statistic
or ExtendedStatistic,
but not both.
*/
public final String extendedStatistic() {
return extendedStatistic;
}
/**
* For responses, this returns true if the service returned a value for the Dimensions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDimensions() {
return dimensions != null && !(dimensions instanceof SdkAutoConstructList);
}
/**
*
* The dimensions for the metric specified in MetricName
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDimensions} method.
*
*
* @return The dimensions for the metric specified in MetricName
.
*/
public final List dimensions() {
return dimensions;
}
/**
*
* The length, in seconds, used each time the metric specified in MetricName
is evaluated. Valid values
* are 10, 30, and any multiple of 60.
*
*
* Period
is required for alarms based on static thresholds. If you are creating an alarm based on a
* metric math expression, you specify the period for each metric within the objects in the Metrics
* array.
*
*
* Be sure to specify 10 or 30 only for metrics that are stored by a PutMetricData
call with a
* StorageResolution
of 1. If you specify a period of 10 or 30 for a metric that does not have
* sub-minute resolution, the alarm still attempts to gather data at the period rate that you specify. In this case,
* it does not receive data for the attempts that do not correspond to a one-minute data resolution, and the alarm
* might often lapse into INSUFFICENT_DATA status. Specifying 10 or 30 also sets this alarm as a high-resolution
* alarm, which has a higher charge than other alarms. For more information about pricing, see Amazon CloudWatch Pricing.
*
*
* An alarm's total current evaluation period can be no longer than one day, so Period
multiplied by
* EvaluationPeriods
cannot be more than 86,400 seconds.
*
*
* @return The length, in seconds, used each time the metric specified in MetricName
is evaluated.
* Valid values are 10, 30, and any multiple of 60.
*
* Period
is required for alarms based on static thresholds. If you are creating an alarm based
* on a metric math expression, you specify the period for each metric within the objects in the
* Metrics
array.
*
*
* Be sure to specify 10 or 30 only for metrics that are stored by a PutMetricData
call with a
* StorageResolution
of 1. If you specify a period of 10 or 30 for a metric that does not have
* sub-minute resolution, the alarm still attempts to gather data at the period rate that you specify. In
* this case, it does not receive data for the attempts that do not correspond to a one-minute data
* resolution, and the alarm might often lapse into INSUFFICENT_DATA status. Specifying 10 or 30 also sets
* this alarm as a high-resolution alarm, which has a higher charge than other alarms. For more information
* about pricing, see Amazon CloudWatch Pricing.
*
*
* An alarm's total current evaluation period can be no longer than one day, so Period
* multiplied by EvaluationPeriods
cannot be more than 86,400 seconds.
*/
public final Integer period() {
return period;
}
/**
*
* The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are Bytes
* because NetworkIn tracks the number of bytes that an instance receives on all network interfaces. You can also
* specify a unit when you create a custom metric. Units help provide conceptual meaning to your data. Metric data
* points that specify a unit of measure, such as Percent, are aggregated separately.
*
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published for the
* metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit, so the alarm works
* as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the alarm's
* behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that is not
* published for this metric. Doing so causes the alarm to be stuck in the INSUFFICIENT DATA
state.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link StandardUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are
* Bytes because NetworkIn tracks the number of bytes that an instance receives on all network interfaces.
* You can also specify a unit when you create a custom metric. Units help provide conceptual meaning to
* your data. Metric data points that specify a unit of measure, such as Percent, are aggregated
* separately.
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published for
* the metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit, so the
* alarm works as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the
* alarm's behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that is
* not published for this metric. Doing so causes the alarm to be stuck in the
* INSUFFICIENT DATA
state.
* @see StandardUnit
*/
public final StandardUnit unit() {
return StandardUnit.fromValue(unit);
}
/**
*
* The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are Bytes
* because NetworkIn tracks the number of bytes that an instance receives on all network interfaces. You can also
* specify a unit when you create a custom metric. Units help provide conceptual meaning to your data. Metric data
* points that specify a unit of measure, such as Percent, are aggregated separately.
*
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published for the
* metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit, so the alarm works
* as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the alarm's
* behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that is not
* published for this metric. Doing so causes the alarm to be stuck in the INSUFFICIENT DATA
state.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link StandardUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are
* Bytes because NetworkIn tracks the number of bytes that an instance receives on all network interfaces.
* You can also specify a unit when you create a custom metric. Units help provide conceptual meaning to
* your data. Metric data points that specify a unit of measure, such as Percent, are aggregated
* separately.
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published for
* the metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit, so the
* alarm works as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the
* alarm's behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that is
* not published for this metric. Doing so causes the alarm to be stuck in the
* INSUFFICIENT DATA
state.
* @see StandardUnit
*/
public final String unitAsString() {
return unit;
}
/**
*
* The number of periods over which data is compared to the specified threshold. If you are setting an alarm that
* requires that a number of consecutive data points be breaching to trigger the alarm, this value specifies that
* number. If you are setting an "M out of N" alarm, this value is the N.
*
*
* An alarm's total current evaluation period can be no longer than one day, so this number multiplied by
* Period
cannot be more than 86,400 seconds.
*
*
* @return The number of periods over which data is compared to the specified threshold. If you are setting an alarm
* that requires that a number of consecutive data points be breaching to trigger the alarm, this value
* specifies that number. If you are setting an "M out of N" alarm, this value is the N.
*
* An alarm's total current evaluation period can be no longer than one day, so this number multiplied by
* Period
cannot be more than 86,400 seconds.
*/
public final Integer evaluationPeriods() {
return evaluationPeriods;
}
/**
*
* The number of data points that must be breaching to trigger the alarm. This is used only if you are setting an
* "M out of N" alarm. In that case, this value is the M. For more information, see Evaluating an Alarm in the Amazon CloudWatch User Guide.
*
*
* @return The number of data points that must be breaching to trigger the alarm. This is used only if you are
* setting an "M out of N" alarm. In that case, this value is the M. For more information, see Evaluating an Alarm in the Amazon CloudWatch User Guide.
*/
public final Integer datapointsToAlarm() {
return datapointsToAlarm;
}
/**
*
* The value against which the specified statistic is compared.
*
*
* This parameter is required for alarms based on static thresholds, but should not be used for alarms based on
* anomaly detection models.
*
*
* @return The value against which the specified statistic is compared.
*
* This parameter is required for alarms based on static thresholds, but should not be used for alarms based
* on anomaly detection models.
*/
public final Double threshold() {
return threshold;
}
/**
*
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified statistic
* value is used as the first operand.
*
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
, and
* GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return The arithmetic operation to use when comparing the specified statistic and threshold. The specified
* statistic value is used as the first operand.
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
,
* and GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
* @see ComparisonOperator
*/
public final ComparisonOperator comparisonOperator() {
return ComparisonOperator.fromValue(comparisonOperator);
}
/**
*
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified statistic
* value is used as the first operand.
*
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
, and
* GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return The arithmetic operation to use when comparing the specified statistic and threshold. The specified
* statistic value is used as the first operand.
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
,
* and GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
* @see ComparisonOperator
*/
public final String comparisonOperatorAsString() {
return comparisonOperator;
}
/**
*
* Sets how this alarm is to handle missing data points. If TreatMissingData
is omitted, the default
* behavior of missing
is used. For more information, see Configuring How CloudWatch Alarms Treats Missing Data.
*
*
* Valid Values: breaching | notBreaching | ignore | missing
*
*
*
* Alarms that evaluate metrics in the AWS/DynamoDB
namespace always ignore
missing data
* even if you choose a different option for TreatMissingData
. When an AWS/DynamoDB
metric
* has missing data, alarms that evaluate that metric remain in their current state.
*
*
*
* @return Sets how this alarm is to handle missing data points. If TreatMissingData
is omitted, the
* default behavior of missing
is used. For more information, see Configuring How CloudWatch Alarms Treats Missing Data.
*
* Valid Values: breaching | notBreaching | ignore | missing
*
*
*
* Alarms that evaluate metrics in the AWS/DynamoDB
namespace always ignore
* missing data even if you choose a different option for TreatMissingData
. When an
* AWS/DynamoDB
metric has missing data, alarms that evaluate that metric remain in their
* current state.
*
*/
public final String treatMissingData() {
return treatMissingData;
}
/**
*
* Used only for alarms based on percentiles. If you specify ignore
, the alarm state does not change
* during periods with too few data points to be statistically significant. If you specify evaluate
or
* omit this parameter, the alarm is always evaluated and possibly changes state no matter how many data points are
* available. For more information, see Percentile-Based CloudWatch Alarms and Low Data Samples.
*
*
* Valid Values: evaluate | ignore
*
*
* @return Used only for alarms based on percentiles. If you specify ignore
, the alarm state does not
* change during periods with too few data points to be statistically significant. If you specify
* evaluate
or omit this parameter, the alarm is always evaluated and possibly changes state no
* matter how many data points are available. For more information, see Percentile-Based CloudWatch Alarms and Low Data Samples.
*
* Valid Values: evaluate | ignore
*/
public final String evaluateLowSampleCountPercentile() {
return evaluateLowSampleCountPercentile;
}
/**
* For responses, this returns true if the service returned a value for the Metrics property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasMetrics() {
return metrics != null && !(metrics instanceof SdkAutoConstructList);
}
/**
*
* An array of MetricDataQuery
structures that enable you to create an alarm based on the result of a
* metric math expression. For each PutMetricAlarm
operation, you must specify either
* MetricName
or a Metrics
array.
*
*
* Each item in the Metrics
array either retrieves a metric or performs a math expression.
*
*
* One item in the Metrics
array is the expression that the alarm watches. You designate this
* expression by setting ReturnData
to true for this object in the array. For more information, see MetricDataQuery.
*
*
* If you use the Metrics
parameter, you cannot include the MetricName
,
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters of PutMetricAlarm
in the same operation. Instead, you
* retrieve the metrics you are using in your math expression as part of the Metrics
array.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMetrics} method.
*
*
* @return An array of MetricDataQuery
structures that enable you to create an alarm based on the
* result of a metric math expression. For each PutMetricAlarm
operation, you must specify
* either MetricName
or a Metrics
array.
*
* Each item in the Metrics
array either retrieves a metric or performs a math expression.
*
*
* One item in the Metrics
array is the expression that the alarm watches. You designate this
* expression by setting ReturnData
to true for this object in the array. For more information,
* see
* MetricDataQuery.
*
*
* If you use the Metrics
parameter, you cannot include the MetricName
,
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters of PutMetricAlarm
in the same operation. Instead,
* you retrieve the metrics you are using in your math expression as part of the Metrics
array.
*/
public final List metrics() {
return metrics;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an alarm.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter are ignored.
* To change the tags of an existing alarm, use TagResource or
* UntagResource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an
* alarm.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions
* by granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter are
* ignored. To change the tags of an existing alarm, use TagResource or UntagResource.
*/
public final List tags() {
return tags;
}
/**
*
* If this is an alarm based on an anomaly detection model, make this value match the ID of the
* ANOMALY_DETECTION_BAND
function.
*
*
* For an example of how to use this parameter, see the Anomaly Detection Model Alarm example on this page.
*
*
* If your alarm uses this parameter, it cannot have Auto Scaling actions.
*
*
* @return If this is an alarm based on an anomaly detection model, make this value match the ID of the
* ANOMALY_DETECTION_BAND
function.
*
* For an example of how to use this parameter, see the Anomaly Detection Model Alarm example on this
* page.
*
*
* If your alarm uses this parameter, it cannot have Auto Scaling actions.
*/
public final String thresholdMetricId() {
return thresholdMetricId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(alarmName());
hashCode = 31 * hashCode + Objects.hashCode(alarmDescription());
hashCode = 31 * hashCode + Objects.hashCode(actionsEnabled());
hashCode = 31 * hashCode + Objects.hashCode(hasOkActions() ? okActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAlarmActions() ? alarmActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasInsufficientDataActions() ? insufficientDataActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(metricName());
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(statisticAsString());
hashCode = 31 * hashCode + Objects.hashCode(extendedStatistic());
hashCode = 31 * hashCode + Objects.hashCode(hasDimensions() ? dimensions() : null);
hashCode = 31 * hashCode + Objects.hashCode(period());
hashCode = 31 * hashCode + Objects.hashCode(unitAsString());
hashCode = 31 * hashCode + Objects.hashCode(evaluationPeriods());
hashCode = 31 * hashCode + Objects.hashCode(datapointsToAlarm());
hashCode = 31 * hashCode + Objects.hashCode(threshold());
hashCode = 31 * hashCode + Objects.hashCode(comparisonOperatorAsString());
hashCode = 31 * hashCode + Objects.hashCode(treatMissingData());
hashCode = 31 * hashCode + Objects.hashCode(evaluateLowSampleCountPercentile());
hashCode = 31 * hashCode + Objects.hashCode(hasMetrics() ? metrics() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(thresholdMetricId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutMetricAlarmRequest)) {
return false;
}
PutMetricAlarmRequest other = (PutMetricAlarmRequest) obj;
return Objects.equals(alarmName(), other.alarmName()) && Objects.equals(alarmDescription(), other.alarmDescription())
&& Objects.equals(actionsEnabled(), other.actionsEnabled()) && hasOkActions() == other.hasOkActions()
&& Objects.equals(okActions(), other.okActions()) && hasAlarmActions() == other.hasAlarmActions()
&& Objects.equals(alarmActions(), other.alarmActions())
&& hasInsufficientDataActions() == other.hasInsufficientDataActions()
&& Objects.equals(insufficientDataActions(), other.insufficientDataActions())
&& Objects.equals(metricName(), other.metricName()) && Objects.equals(namespace(), other.namespace())
&& Objects.equals(statisticAsString(), other.statisticAsString())
&& Objects.equals(extendedStatistic(), other.extendedStatistic()) && hasDimensions() == other.hasDimensions()
&& Objects.equals(dimensions(), other.dimensions()) && Objects.equals(period(), other.period())
&& Objects.equals(unitAsString(), other.unitAsString())
&& Objects.equals(evaluationPeriods(), other.evaluationPeriods())
&& Objects.equals(datapointsToAlarm(), other.datapointsToAlarm())
&& Objects.equals(threshold(), other.threshold())
&& Objects.equals(comparisonOperatorAsString(), other.comparisonOperatorAsString())
&& Objects.equals(treatMissingData(), other.treatMissingData())
&& Objects.equals(evaluateLowSampleCountPercentile(), other.evaluateLowSampleCountPercentile())
&& hasMetrics() == other.hasMetrics() && Objects.equals(metrics(), other.metrics())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(thresholdMetricId(), other.thresholdMetricId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PutMetricAlarmRequest").add("AlarmName", alarmName())
.add("AlarmDescription", alarmDescription()).add("ActionsEnabled", actionsEnabled())
.add("OKActions", hasOkActions() ? okActions() : null)
.add("AlarmActions", hasAlarmActions() ? alarmActions() : null)
.add("InsufficientDataActions", hasInsufficientDataActions() ? insufficientDataActions() : null)
.add("MetricName", metricName()).add("Namespace", namespace()).add("Statistic", statisticAsString())
.add("ExtendedStatistic", extendedStatistic()).add("Dimensions", hasDimensions() ? dimensions() : null)
.add("Period", period()).add("Unit", unitAsString()).add("EvaluationPeriods", evaluationPeriods())
.add("DatapointsToAlarm", datapointsToAlarm()).add("Threshold", threshold())
.add("ComparisonOperator", comparisonOperatorAsString()).add("TreatMissingData", treatMissingData())
.add("EvaluateLowSampleCountPercentile", evaluateLowSampleCountPercentile())
.add("Metrics", hasMetrics() ? metrics() : null).add("Tags", hasTags() ? tags() : null)
.add("ThresholdMetricId", thresholdMetricId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AlarmName":
return Optional.ofNullable(clazz.cast(alarmName()));
case "AlarmDescription":
return Optional.ofNullable(clazz.cast(alarmDescription()));
case "ActionsEnabled":
return Optional.ofNullable(clazz.cast(actionsEnabled()));
case "OKActions":
return Optional.ofNullable(clazz.cast(okActions()));
case "AlarmActions":
return Optional.ofNullable(clazz.cast(alarmActions()));
case "InsufficientDataActions":
return Optional.ofNullable(clazz.cast(insufficientDataActions()));
case "MetricName":
return Optional.ofNullable(clazz.cast(metricName()));
case "Namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "Statistic":
return Optional.ofNullable(clazz.cast(statisticAsString()));
case "ExtendedStatistic":
return Optional.ofNullable(clazz.cast(extendedStatistic()));
case "Dimensions":
return Optional.ofNullable(clazz.cast(dimensions()));
case "Period":
return Optional.ofNullable(clazz.cast(period()));
case "Unit":
return Optional.ofNullable(clazz.cast(unitAsString()));
case "EvaluationPeriods":
return Optional.ofNullable(clazz.cast(evaluationPeriods()));
case "DatapointsToAlarm":
return Optional.ofNullable(clazz.cast(datapointsToAlarm()));
case "Threshold":
return Optional.ofNullable(clazz.cast(threshold()));
case "ComparisonOperator":
return Optional.ofNullable(clazz.cast(comparisonOperatorAsString()));
case "TreatMissingData":
return Optional.ofNullable(clazz.cast(treatMissingData()));
case "EvaluateLowSampleCountPercentile":
return Optional.ofNullable(clazz.cast(evaluateLowSampleCountPercentile()));
case "Metrics":
return Optional.ofNullable(clazz.cast(metrics()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ThresholdMetricId":
return Optional.ofNullable(clazz.cast(thresholdMetricId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder okActions(Collection okActions);
/**
*
* The actions to execute when this alarm transitions to an OK
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
*
*
* @param okActions
* The actions to execute when this alarm transitions to an OK
state from any other state.
* Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder okActions(String... okActions);
/**
*
* The actions to execute when this alarm transitions to the ALARM
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
* | arn:aws:ssm:region:account-id:opsitem:severity
|
* arn:aws:ssm-incidents::account-id:response-plan:response-plan-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
*
*
* @param alarmActions
* The actions to execute when this alarm transitions to the ALARM
state from any other
* state. Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
* | arn:aws:ssm:region:account-id:opsitem:severity
|
* arn:aws:ssm-incidents::account-id:response-plan:response-plan-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder alarmActions(Collection alarmActions);
/**
*
* The actions to execute when this alarm transitions to the ALARM
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
* | arn:aws:ssm:region:account-id:opsitem:severity
|
* arn:aws:ssm-incidents::account-id:response-plan:response-plan-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
*
*
* @param alarmActions
* The actions to execute when this alarm transitions to the ALARM
state from any other
* state. Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
* | arn:aws:ssm:region:account-id:opsitem:severity
|
* arn:aws:ssm-incidents::account-id:response-plan:response-plan-name
*
*
* Valid Values (for use with IAM roles):
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Recover/1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder alarmActions(String... alarmActions);
/**
*
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any other
* state. Each action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* >arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
*
*
* @param insufficientDataActions
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from
* any other state. Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* >arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder insufficientDataActions(Collection insufficientDataActions);
/**
*
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any other
* state. Each action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* >arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
|
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
*
*
* @param insufficientDataActions
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from
* any other state. Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: arn:aws:automate:region:ec2:stop
|
* arn:aws:automate:region:ec2:terminate
|
* arn:aws:automate:region:ec2:recover
|
* arn:aws:automate:region:ec2:reboot
|
* arn:aws:sns:region:account-id:sns-topic-name
|
* arn:aws:autoscaling:region:account-id:scalingPolicy:policy-id:autoScalingGroupName/group-friendly-name:policyName/policy-friendly-name
*
*
* Valid Values (for use with IAM roles):
* >arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Stop/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Terminate/1.0
* |
* arn:aws:swf:region:account-id:action/actions/AWS_EC2.InstanceId.Reboot/1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder insufficientDataActions(String... insufficientDataActions);
/**
*
* The name for the metric associated with the alarm. For each PutMetricAlarm
operation, you must
* specify either MetricName
or a Metrics
array.
*
*
* If you are creating an alarm based on a math expression, you cannot specify this parameter, or any of the
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters. Instead, you specify all this information in the
* Metrics
array.
*
*
* @param metricName
* The name for the metric associated with the alarm. For each PutMetricAlarm
operation, you
* must specify either MetricName
or a Metrics
array.
*
* If you are creating an alarm based on a math expression, you cannot specify this parameter, or any of
* the Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters. Instead, you specify all this information in the
* Metrics
array.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder metricName(String metricName);
/**
*
* The namespace for the metric associated specified in MetricName
.
*
*
* @param namespace
* The namespace for the metric associated specified in MetricName
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder namespace(String namespace);
/**
*
* The statistic for the metric specified in MetricName
, other than percentile. For percentile
* statistics, use ExtendedStatistic
. When you call PutMetricAlarm
and specify a
* MetricName
, you must specify either Statistic
or ExtendedStatistic,
* but not both.
*
*
* @param statistic
* The statistic for the metric specified in MetricName
, other than percentile. For
* percentile statistics, use ExtendedStatistic
. When you call PutMetricAlarm
* and specify a MetricName
, you must specify either Statistic
or
* ExtendedStatistic,
but not both.
* @see Statistic
* @return Returns a reference to this object so that method calls can be chained together.
* @see Statistic
*/
Builder statistic(String statistic);
/**
*
* The statistic for the metric specified in MetricName
, other than percentile. For percentile
* statistics, use ExtendedStatistic
. When you call PutMetricAlarm
and specify a
* MetricName
, you must specify either Statistic
or ExtendedStatistic,
* but not both.
*
*
* @param statistic
* The statistic for the metric specified in MetricName
, other than percentile. For
* percentile statistics, use ExtendedStatistic
. When you call PutMetricAlarm
* and specify a MetricName
, you must specify either Statistic
or
* ExtendedStatistic,
but not both.
* @see Statistic
* @return Returns a reference to this object so that method calls can be chained together.
* @see Statistic
*/
Builder statistic(Statistic statistic);
/**
*
* The percentile statistic for the metric specified in MetricName
. Specify a value between p0.0
* and p100. When you call PutMetricAlarm
and specify a MetricName
, you must specify
* either Statistic
or ExtendedStatistic,
but not both.
*
*
* @param extendedStatistic
* The percentile statistic for the metric specified in MetricName
. Specify a value between
* p0.0 and p100. When you call PutMetricAlarm
and specify a MetricName
, you
* must specify either Statistic
or ExtendedStatistic,
but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder extendedStatistic(String extendedStatistic);
/**
*
* The dimensions for the metric specified in MetricName
.
*
*
* @param dimensions
* The dimensions for the metric specified in MetricName
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dimensions(Collection dimensions);
/**
*
* The dimensions for the metric specified in MetricName
.
*
*
* @param dimensions
* The dimensions for the metric specified in MetricName
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dimensions(Dimension... dimensions);
/**
*
* The dimensions for the metric specified in MetricName
.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudwatch.model.Dimension.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.cloudwatch.model.Dimension#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudwatch.model.Dimension.Builder#build()} is called immediately and
* its result is passed to {@link #dimensions(List)}.
*
* @param dimensions
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudwatch.model.Dimension.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #dimensions(java.util.Collection)
*/
Builder dimensions(Consumer... dimensions);
/**
*
* The length, in seconds, used each time the metric specified in MetricName
is evaluated. Valid
* values are 10, 30, and any multiple of 60.
*
*
* Period
is required for alarms based on static thresholds. If you are creating an alarm based on
* a metric math expression, you specify the period for each metric within the objects in the
* Metrics
array.
*
*
* Be sure to specify 10 or 30 only for metrics that are stored by a PutMetricData
call with a
* StorageResolution
of 1. If you specify a period of 10 or 30 for a metric that does not have
* sub-minute resolution, the alarm still attempts to gather data at the period rate that you specify. In this
* case, it does not receive data for the attempts that do not correspond to a one-minute data resolution, and
* the alarm might often lapse into INSUFFICENT_DATA status. Specifying 10 or 30 also sets this alarm as a
* high-resolution alarm, which has a higher charge than other alarms. For more information about pricing, see
* Amazon CloudWatch Pricing.
*
*
* An alarm's total current evaluation period can be no longer than one day, so Period
multiplied
* by EvaluationPeriods
cannot be more than 86,400 seconds.
*
*
* @param period
* The length, in seconds, used each time the metric specified in MetricName
is evaluated.
* Valid values are 10, 30, and any multiple of 60.
*
* Period
is required for alarms based on static thresholds. If you are creating an alarm
* based on a metric math expression, you specify the period for each metric within the objects in the
* Metrics
array.
*
*
* Be sure to specify 10 or 30 only for metrics that are stored by a PutMetricData
call with
* a StorageResolution
of 1. If you specify a period of 10 or 30 for a metric that does not
* have sub-minute resolution, the alarm still attempts to gather data at the period rate that you
* specify. In this case, it does not receive data for the attempts that do not correspond to a
* one-minute data resolution, and the alarm might often lapse into INSUFFICENT_DATA status. Specifying
* 10 or 30 also sets this alarm as a high-resolution alarm, which has a higher charge than other alarms.
* For more information about pricing, see Amazon
* CloudWatch Pricing.
*
*
* An alarm's total current evaluation period can be no longer than one day, so Period
* multiplied by EvaluationPeriods
cannot be more than 86,400 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder period(Integer period);
/**
*
* The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are Bytes
* because NetworkIn tracks the number of bytes that an instance receives on all network interfaces. You can
* also specify a unit when you create a custom metric. Units help provide conceptual meaning to your data.
* Metric data points that specify a unit of measure, such as Percent, are aggregated separately.
*
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published for the
* metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit, so the alarm
* works as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the alarm's
* behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that is not
* published for this metric. Doing so causes the alarm to be stuck in the INSUFFICIENT DATA
state.
*
*
* @param unit
* The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are
* Bytes because NetworkIn tracks the number of bytes that an instance receives on all network
* interfaces. You can also specify a unit when you create a custom metric. Units help provide conceptual
* meaning to your data. Metric data points that specify a unit of measure, such as Percent, are
* aggregated separately.
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published
* for the metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit,
* so the alarm works as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the
* alarm's behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that
* is not published for this metric. Doing so causes the alarm to be stuck in the
* INSUFFICIENT DATA
state.
* @see StandardUnit
* @return Returns a reference to this object so that method calls can be chained together.
* @see StandardUnit
*/
Builder unit(String unit);
/**
*
* The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are Bytes
* because NetworkIn tracks the number of bytes that an instance receives on all network interfaces. You can
* also specify a unit when you create a custom metric. Units help provide conceptual meaning to your data.
* Metric data points that specify a unit of measure, such as Percent, are aggregated separately.
*
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published for the
* metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit, so the alarm
* works as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the alarm's
* behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that is not
* published for this metric. Doing so causes the alarm to be stuck in the INSUFFICIENT DATA
state.
*
*
* @param unit
* The unit of measure for the statistic. For example, the units for the Amazon EC2 NetworkIn metric are
* Bytes because NetworkIn tracks the number of bytes that an instance receives on all network
* interfaces. You can also specify a unit when you create a custom metric. Units help provide conceptual
* meaning to your data. Metric data points that specify a unit of measure, such as Percent, are
* aggregated separately.
*
* If you don't specify Unit
, CloudWatch retrieves all unit types that have been published
* for the metric and attempts to evaluate the alarm. Usually, metrics are published with only one unit,
* so the alarm works as intended.
*
*
* However, if the metric is published with multiple types of units and you don't specify a unit, the
* alarm's behavior is not defined and it behaves unpredictably.
*
*
* We recommend omitting Unit
so that you don't inadvertently specify an incorrect unit that
* is not published for this metric. Doing so causes the alarm to be stuck in the
* INSUFFICIENT DATA
state.
* @see StandardUnit
* @return Returns a reference to this object so that method calls can be chained together.
* @see StandardUnit
*/
Builder unit(StandardUnit unit);
/**
*
* The number of periods over which data is compared to the specified threshold. If you are setting an alarm
* that requires that a number of consecutive data points be breaching to trigger the alarm, this value
* specifies that number. If you are setting an "M out of N" alarm, this value is the N.
*
*
* An alarm's total current evaluation period can be no longer than one day, so this number multiplied by
* Period
cannot be more than 86,400 seconds.
*
*
* @param evaluationPeriods
* The number of periods over which data is compared to the specified threshold. If you are setting an
* alarm that requires that a number of consecutive data points be breaching to trigger the alarm, this
* value specifies that number. If you are setting an "M out of N" alarm, this value is the N.
*
* An alarm's total current evaluation period can be no longer than one day, so this number multiplied by
* Period
cannot be more than 86,400 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder evaluationPeriods(Integer evaluationPeriods);
/**
*
* The number of data points that must be breaching to trigger the alarm. This is used only if you are setting
* an "M out of N" alarm. In that case, this value is the M. For more information, see Evaluating an Alarm in the Amazon CloudWatch User Guide.
*
*
* @param datapointsToAlarm
* The number of data points that must be breaching to trigger the alarm. This is used only if you are
* setting an "M out of N" alarm. In that case, this value is the M. For more information, see Evaluating an Alarm in the Amazon CloudWatch User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder datapointsToAlarm(Integer datapointsToAlarm);
/**
*
* The value against which the specified statistic is compared.
*
*
* This parameter is required for alarms based on static thresholds, but should not be used for alarms based on
* anomaly detection models.
*
*
* @param threshold
* The value against which the specified statistic is compared.
*
* This parameter is required for alarms based on static thresholds, but should not be used for alarms
* based on anomaly detection models.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder threshold(Double threshold);
/**
*
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified statistic
* value is used as the first operand.
*
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
, and
* GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
*
*
* @param comparisonOperator
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified
* statistic value is used as the first operand.
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
,
* and GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
* @see ComparisonOperator
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComparisonOperator
*/
Builder comparisonOperator(String comparisonOperator);
/**
*
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified statistic
* value is used as the first operand.
*
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
, and
* GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
*
*
* @param comparisonOperator
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified
* statistic value is used as the first operand.
*
* The values LessThanLowerOrGreaterThanUpperThreshold
, LessThanLowerThreshold
,
* and GreaterThanUpperThreshold
are used only for alarms based on anomaly detection models.
* @see ComparisonOperator
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComparisonOperator
*/
Builder comparisonOperator(ComparisonOperator comparisonOperator);
/**
*
* Sets how this alarm is to handle missing data points. If TreatMissingData
is omitted, the
* default behavior of missing
is used. For more information, see Configuring How CloudWatch Alarms Treats Missing Data.
*
*
* Valid Values: breaching | notBreaching | ignore | missing
*
*
*
* Alarms that evaluate metrics in the AWS/DynamoDB
namespace always ignore
missing
* data even if you choose a different option for TreatMissingData
. When an
* AWS/DynamoDB
metric has missing data, alarms that evaluate that metric remain in their current
* state.
*
*
*
* @param treatMissingData
* Sets how this alarm is to handle missing data points. If TreatMissingData
is omitted, the
* default behavior of missing
is used. For more information, see Configuring How CloudWatch Alarms Treats Missing Data.
*
* Valid Values: breaching | notBreaching | ignore | missing
*
*
*
* Alarms that evaluate metrics in the AWS/DynamoDB
namespace always ignore
* missing data even if you choose a different option for TreatMissingData
. When an
* AWS/DynamoDB
metric has missing data, alarms that evaluate that metric remain in their
* current state.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder treatMissingData(String treatMissingData);
/**
*
* Used only for alarms based on percentiles. If you specify ignore
, the alarm state does not
* change during periods with too few data points to be statistically significant. If you specify
* evaluate
or omit this parameter, the alarm is always evaluated and possibly changes state no
* matter how many data points are available. For more information, see Percentile-Based CloudWatch Alarms and Low Data Samples.
*
*
* Valid Values: evaluate | ignore
*
*
* @param evaluateLowSampleCountPercentile
* Used only for alarms based on percentiles. If you specify ignore
, the alarm state does
* not change during periods with too few data points to be statistically significant. If you specify
* evaluate
or omit this parameter, the alarm is always evaluated and possibly changes state
* no matter how many data points are available. For more information, see Percentile-Based CloudWatch Alarms and Low Data Samples.
*
* Valid Values: evaluate | ignore
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder evaluateLowSampleCountPercentile(String evaluateLowSampleCountPercentile);
/**
*
* An array of MetricDataQuery
structures that enable you to create an alarm based on the result of
* a metric math expression. For each PutMetricAlarm
operation, you must specify either
* MetricName
or a Metrics
array.
*
*
* Each item in the Metrics
array either retrieves a metric or performs a math expression.
*
*
* One item in the Metrics
array is the expression that the alarm watches. You designate this
* expression by setting ReturnData
to true for this object in the array. For more information, see
*
* MetricDataQuery.
*
*
* If you use the Metrics
parameter, you cannot include the MetricName
,
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters of PutMetricAlarm
in the same operation. Instead, you
* retrieve the metrics you are using in your math expression as part of the Metrics
array.
*
*
* @param metrics
* An array of MetricDataQuery
structures that enable you to create an alarm based on the
* result of a metric math expression. For each PutMetricAlarm
operation, you must specify
* either MetricName
or a Metrics
array.
*
* Each item in the Metrics
array either retrieves a metric or performs a math expression.
*
*
* One item in the Metrics
array is the expression that the alarm watches. You designate
* this expression by setting ReturnData
to true for this object in the array. For more
* information, see MetricDataQuery.
*
*
* If you use the Metrics
parameter, you cannot include the MetricName
,
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters of PutMetricAlarm
in the same operation.
* Instead, you retrieve the metrics you are using in your math expression as part of the
* Metrics
array.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder metrics(Collection metrics);
/**
*
* An array of MetricDataQuery
structures that enable you to create an alarm based on the result of
* a metric math expression. For each PutMetricAlarm
operation, you must specify either
* MetricName
or a Metrics
array.
*
*
* Each item in the Metrics
array either retrieves a metric or performs a math expression.
*
*
* One item in the Metrics
array is the expression that the alarm watches. You designate this
* expression by setting ReturnData
to true for this object in the array. For more information, see
*
* MetricDataQuery.
*
*
* If you use the Metrics
parameter, you cannot include the MetricName
,
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters of PutMetricAlarm
in the same operation. Instead, you
* retrieve the metrics you are using in your math expression as part of the Metrics
array.
*
*
* @param metrics
* An array of MetricDataQuery
structures that enable you to create an alarm based on the
* result of a metric math expression. For each PutMetricAlarm
operation, you must specify
* either MetricName
or a Metrics
array.
*
* Each item in the Metrics
array either retrieves a metric or performs a math expression.
*
*
* One item in the Metrics
array is the expression that the alarm watches. You designate
* this expression by setting ReturnData
to true for this object in the array. For more
* information, see MetricDataQuery.
*
*
* If you use the Metrics
parameter, you cannot include the MetricName
,
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters of PutMetricAlarm
in the same operation.
* Instead, you retrieve the metrics you are using in your math expression as part of the
* Metrics
array.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder metrics(MetricDataQuery... metrics);
/**
*
* An array of MetricDataQuery
structures that enable you to create an alarm based on the result of
* a metric math expression. For each PutMetricAlarm
operation, you must specify either
* MetricName
or a Metrics
array.
*
*
* Each item in the Metrics
array either retrieves a metric or performs a math expression.
*
*
* One item in the Metrics
array is the expression that the alarm watches. You designate this
* expression by setting ReturnData
to true for this object in the array. For more information, see
*
* MetricDataQuery.
*
*
* If you use the Metrics
parameter, you cannot include the MetricName
,
* Dimensions
, Period
, Namespace
, Statistic
, or
* ExtendedStatistic
parameters of PutMetricAlarm
in the same operation. Instead, you
* retrieve the metrics you are using in your math expression as part of the Metrics
array.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricDataQuery.Builder} avoiding the need to create
* one manually via {@link software.amazon.awssdk.services.cloudwatch.model.MetricDataQuery#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricDataQuery.Builder#build()} is called
* immediately and its result is passed to {@link #metrics(List)}.
*
* @param metrics
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricDataQuery.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #metrics(java.util.Collection)
*/
Builder metrics(Consumer... metrics);
/**
*
* A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an alarm.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter are
* ignored. To change the tags of an existing alarm, use TagResource
* or
* UntagResource.
*
*
* @param tags
* A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an
* alarm.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user
* permissions by granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter
* are ignored. To change the tags of an existing alarm, use TagResource or UntagResource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an alarm.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter are
* ignored. To change the tags of an existing alarm, use TagResource
* or
* UntagResource.
*
*
* @param tags
* A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an
* alarm.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user
* permissions by granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter
* are ignored. To change the tags of an existing alarm, use TagResource or UntagResource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an alarm.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter are
* ignored. To change the tags of an existing alarm, use TagResource
* or
* UntagResource.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudwatch.model.Tag.Builder} avoiding the need to create one manually
* via {@link software.amazon.awssdk.services.cloudwatch.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudwatch.model.Tag.Builder#build()} is called immediately and its
* result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudwatch.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* If this is an alarm based on an anomaly detection model, make this value match the ID of the
* ANOMALY_DETECTION_BAND
function.
*
*
* For an example of how to use this parameter, see the Anomaly Detection Model Alarm example on this
* page.
*
*
* If your alarm uses this parameter, it cannot have Auto Scaling actions.
*
*
* @param thresholdMetricId
* If this is an alarm based on an anomaly detection model, make this value match the ID of the
* ANOMALY_DETECTION_BAND
function.
*
* For an example of how to use this parameter, see the Anomaly Detection Model Alarm example on
* this page.
*
*
* If your alarm uses this parameter, it cannot have Auto Scaling actions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder thresholdMetricId(String thresholdMetricId);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudWatchRequest.BuilderImpl implements Builder {
private String alarmName;
private String alarmDescription;
private Boolean actionsEnabled;
private List okActions = DefaultSdkAutoConstructList.getInstance();
private List alarmActions = DefaultSdkAutoConstructList.getInstance();
private List insufficientDataActions = DefaultSdkAutoConstructList.getInstance();
private String metricName;
private String namespace;
private String statistic;
private String extendedStatistic;
private List dimensions = DefaultSdkAutoConstructList.getInstance();
private Integer period;
private String unit;
private Integer evaluationPeriods;
private Integer datapointsToAlarm;
private Double threshold;
private String comparisonOperator;
private String treatMissingData;
private String evaluateLowSampleCountPercentile;
private List metrics = DefaultSdkAutoConstructList.getInstance();
private List tags = DefaultSdkAutoConstructList.getInstance();
private String thresholdMetricId;
private BuilderImpl() {
}
private BuilderImpl(PutMetricAlarmRequest model) {
super(model);
alarmName(model.alarmName);
alarmDescription(model.alarmDescription);
actionsEnabled(model.actionsEnabled);
okActions(model.okActions);
alarmActions(model.alarmActions);
insufficientDataActions(model.insufficientDataActions);
metricName(model.metricName);
namespace(model.namespace);
statistic(model.statistic);
extendedStatistic(model.extendedStatistic);
dimensions(model.dimensions);
period(model.period);
unit(model.unit);
evaluationPeriods(model.evaluationPeriods);
datapointsToAlarm(model.datapointsToAlarm);
threshold(model.threshold);
comparisonOperator(model.comparisonOperator);
treatMissingData(model.treatMissingData);
evaluateLowSampleCountPercentile(model.evaluateLowSampleCountPercentile);
metrics(model.metrics);
tags(model.tags);
thresholdMetricId(model.thresholdMetricId);
}
public final String getAlarmName() {
return alarmName;
}
public final void setAlarmName(String alarmName) {
this.alarmName = alarmName;
}
@Override
public final Builder alarmName(String alarmName) {
this.alarmName = alarmName;
return this;
}
public final String getAlarmDescription() {
return alarmDescription;
}
public final void setAlarmDescription(String alarmDescription) {
this.alarmDescription = alarmDescription;
}
@Override
public final Builder alarmDescription(String alarmDescription) {
this.alarmDescription = alarmDescription;
return this;
}
public final Boolean getActionsEnabled() {
return actionsEnabled;
}
public final void setActionsEnabled(Boolean actionsEnabled) {
this.actionsEnabled = actionsEnabled;
}
@Override
public final Builder actionsEnabled(Boolean actionsEnabled) {
this.actionsEnabled = actionsEnabled;
return this;
}
public final Collection getOkActions() {
if (okActions instanceof SdkAutoConstructList) {
return null;
}
return okActions;
}
public final void setOkActions(Collection okActions) {
this.okActions = ResourceListCopier.copy(okActions);
}
@Override
public final Builder okActions(Collection okActions) {
this.okActions = ResourceListCopier.copy(okActions);
return this;
}
@Override
@SafeVarargs
public final Builder okActions(String... okActions) {
okActions(Arrays.asList(okActions));
return this;
}
public final Collection getAlarmActions() {
if (alarmActions instanceof SdkAutoConstructList) {
return null;
}
return alarmActions;
}
public final void setAlarmActions(Collection alarmActions) {
this.alarmActions = ResourceListCopier.copy(alarmActions);
}
@Override
public final Builder alarmActions(Collection alarmActions) {
this.alarmActions = ResourceListCopier.copy(alarmActions);
return this;
}
@Override
@SafeVarargs
public final Builder alarmActions(String... alarmActions) {
alarmActions(Arrays.asList(alarmActions));
return this;
}
public final Collection getInsufficientDataActions() {
if (insufficientDataActions instanceof SdkAutoConstructList) {
return null;
}
return insufficientDataActions;
}
public final void setInsufficientDataActions(Collection insufficientDataActions) {
this.insufficientDataActions = ResourceListCopier.copy(insufficientDataActions);
}
@Override
public final Builder insufficientDataActions(Collection insufficientDataActions) {
this.insufficientDataActions = ResourceListCopier.copy(insufficientDataActions);
return this;
}
@Override
@SafeVarargs
public final Builder insufficientDataActions(String... insufficientDataActions) {
insufficientDataActions(Arrays.asList(insufficientDataActions));
return this;
}
public final String getMetricName() {
return metricName;
}
public final void setMetricName(String metricName) {
this.metricName = metricName;
}
@Override
public final Builder metricName(String metricName) {
this.metricName = metricName;
return this;
}
public final String getNamespace() {
return namespace;
}
public final void setNamespace(String namespace) {
this.namespace = namespace;
}
@Override
public final Builder namespace(String namespace) {
this.namespace = namespace;
return this;
}
public final String getStatistic() {
return statistic;
}
public final void setStatistic(String statistic) {
this.statistic = statistic;
}
@Override
public final Builder statistic(String statistic) {
this.statistic = statistic;
return this;
}
@Override
public final Builder statistic(Statistic statistic) {
this.statistic(statistic == null ? null : statistic.toString());
return this;
}
public final String getExtendedStatistic() {
return extendedStatistic;
}
public final void setExtendedStatistic(String extendedStatistic) {
this.extendedStatistic = extendedStatistic;
}
@Override
public final Builder extendedStatistic(String extendedStatistic) {
this.extendedStatistic = extendedStatistic;
return this;
}
public final List getDimensions() {
List result = DimensionsCopier.copyToBuilder(this.dimensions);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setDimensions(Collection dimensions) {
this.dimensions = DimensionsCopier.copyFromBuilder(dimensions);
}
@Override
public final Builder dimensions(Collection dimensions) {
this.dimensions = DimensionsCopier.copy(dimensions);
return this;
}
@Override
@SafeVarargs
public final Builder dimensions(Dimension... dimensions) {
dimensions(Arrays.asList(dimensions));
return this;
}
@Override
@SafeVarargs
public final Builder dimensions(Consumer... dimensions) {
dimensions(Stream.of(dimensions).map(c -> Dimension.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Integer getPeriod() {
return period;
}
public final void setPeriod(Integer period) {
this.period = period;
}
@Override
public final Builder period(Integer period) {
this.period = period;
return this;
}
public final String getUnit() {
return unit;
}
public final void setUnit(String unit) {
this.unit = unit;
}
@Override
public final Builder unit(String unit) {
this.unit = unit;
return this;
}
@Override
public final Builder unit(StandardUnit unit) {
this.unit(unit == null ? null : unit.toString());
return this;
}
public final Integer getEvaluationPeriods() {
return evaluationPeriods;
}
public final void setEvaluationPeriods(Integer evaluationPeriods) {
this.evaluationPeriods = evaluationPeriods;
}
@Override
public final Builder evaluationPeriods(Integer evaluationPeriods) {
this.evaluationPeriods = evaluationPeriods;
return this;
}
public final Integer getDatapointsToAlarm() {
return datapointsToAlarm;
}
public final void setDatapointsToAlarm(Integer datapointsToAlarm) {
this.datapointsToAlarm = datapointsToAlarm;
}
@Override
public final Builder datapointsToAlarm(Integer datapointsToAlarm) {
this.datapointsToAlarm = datapointsToAlarm;
return this;
}
public final Double getThreshold() {
return threshold;
}
public final void setThreshold(Double threshold) {
this.threshold = threshold;
}
@Override
public final Builder threshold(Double threshold) {
this.threshold = threshold;
return this;
}
public final String getComparisonOperator() {
return comparisonOperator;
}
public final void setComparisonOperator(String comparisonOperator) {
this.comparisonOperator = comparisonOperator;
}
@Override
public final Builder comparisonOperator(String comparisonOperator) {
this.comparisonOperator = comparisonOperator;
return this;
}
@Override
public final Builder comparisonOperator(ComparisonOperator comparisonOperator) {
this.comparisonOperator(comparisonOperator == null ? null : comparisonOperator.toString());
return this;
}
public final String getTreatMissingData() {
return treatMissingData;
}
public final void setTreatMissingData(String treatMissingData) {
this.treatMissingData = treatMissingData;
}
@Override
public final Builder treatMissingData(String treatMissingData) {
this.treatMissingData = treatMissingData;
return this;
}
public final String getEvaluateLowSampleCountPercentile() {
return evaluateLowSampleCountPercentile;
}
public final void setEvaluateLowSampleCountPercentile(String evaluateLowSampleCountPercentile) {
this.evaluateLowSampleCountPercentile = evaluateLowSampleCountPercentile;
}
@Override
public final Builder evaluateLowSampleCountPercentile(String evaluateLowSampleCountPercentile) {
this.evaluateLowSampleCountPercentile = evaluateLowSampleCountPercentile;
return this;
}
public final List getMetrics() {
List result = MetricDataQueriesCopier.copyToBuilder(this.metrics);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setMetrics(Collection metrics) {
this.metrics = MetricDataQueriesCopier.copyFromBuilder(metrics);
}
@Override
public final Builder metrics(Collection metrics) {
this.metrics = MetricDataQueriesCopier.copy(metrics);
return this;
}
@Override
@SafeVarargs
public final Builder metrics(MetricDataQuery... metrics) {
metrics(Arrays.asList(metrics));
return this;
}
@Override
@SafeVarargs
public final Builder metrics(Consumer... metrics) {
metrics(Stream.of(metrics).map(c -> MetricDataQuery.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final List getTags() {
List result = TagListCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final String getThresholdMetricId() {
return thresholdMetricId;
}
public final void setThresholdMetricId(String thresholdMetricId) {
this.thresholdMetricId = thresholdMetricId;
}
@Override
public final Builder thresholdMetricId(String thresholdMetricId) {
this.thresholdMetricId = thresholdMetricId;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public PutMetricAlarmRequest build() {
return new PutMetricAlarmRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}