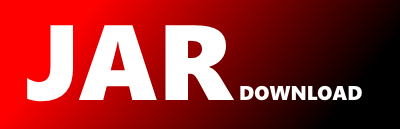
software.amazon.awssdk.services.cloudwatch.model.ListMetricsRequest Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListMetricsRequest extends CloudWatchRequest implements
ToCopyableBuilder {
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Namespace").getter(getter(ListMetricsRequest::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Namespace").build()).build();
private static final SdkField METRIC_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetricName").getter(getter(ListMetricsRequest::metricName)).setter(setter(Builder::metricName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricName").build()).build();
private static final SdkField> DIMENSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Dimensions")
.getter(getter(ListMetricsRequest::dimensions))
.setter(setter(Builder::dimensions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Dimensions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DimensionFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextToken").getter(getter(ListMetricsRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken").build()).build();
private static final SdkField RECENTLY_ACTIVE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RecentlyActive").getter(getter(ListMetricsRequest::recentlyActiveAsString))
.setter(setter(Builder::recentlyActive))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecentlyActive").build()).build();
private static final SdkField INCLUDE_LINKED_ACCOUNTS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeLinkedAccounts").getter(getter(ListMetricsRequest::includeLinkedAccounts))
.setter(setter(Builder::includeLinkedAccounts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeLinkedAccounts").build())
.build();
private static final SdkField OWNING_ACCOUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OwningAccount").getter(getter(ListMetricsRequest::owningAccount)).setter(setter(Builder::owningAccount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwningAccount").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAMESPACE_FIELD,
METRIC_NAME_FIELD, DIMENSIONS_FIELD, NEXT_TOKEN_FIELD, RECENTLY_ACTIVE_FIELD, INCLUDE_LINKED_ACCOUNTS_FIELD,
OWNING_ACCOUNT_FIELD));
private final String namespace;
private final String metricName;
private final List dimensions;
private final String nextToken;
private final String recentlyActive;
private final Boolean includeLinkedAccounts;
private final String owningAccount;
private ListMetricsRequest(BuilderImpl builder) {
super(builder);
this.namespace = builder.namespace;
this.metricName = builder.metricName;
this.dimensions = builder.dimensions;
this.nextToken = builder.nextToken;
this.recentlyActive = builder.recentlyActive;
this.includeLinkedAccounts = builder.includeLinkedAccounts;
this.owningAccount = builder.owningAccount;
}
/**
*
* The metric namespace to filter against. Only the namespace that matches exactly will be returned.
*
*
* @return The metric namespace to filter against. Only the namespace that matches exactly will be returned.
*/
public final String namespace() {
return namespace;
}
/**
*
* The name of the metric to filter against. Only the metrics with names that match exactly will be returned.
*
*
* @return The name of the metric to filter against. Only the metrics with names that match exactly will be
* returned.
*/
public final String metricName() {
return metricName;
}
/**
* For responses, this returns true if the service returned a value for the Dimensions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDimensions() {
return dimensions != null && !(dimensions instanceof SdkAutoConstructList);
}
/**
*
* The dimensions to filter against. Only the dimensions that match exactly will be returned.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDimensions} method.
*
*
* @return The dimensions to filter against. Only the dimensions that match exactly will be returned.
*/
public final List dimensions() {
return dimensions;
}
/**
*
* The token returned by a previous call to indicate that there is more data available.
*
*
* @return The token returned by a previous call to indicate that there is more data available.
*/
public final String nextToken() {
return nextToken;
}
/**
*
* To filter the results to show only metrics that have had data points published in the past three hours, specify
* this parameter with a value of PT3H
. This is the only valid value for this parameter.
*
*
* The results that are returned are an approximation of the value you specify. There is a low probability that the
* returned results include metrics with last published data as much as 40 minutes more than the specified time
* interval.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #recentlyActive}
* will return {@link RecentlyActive#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #recentlyActiveAsString}.
*
*
* @return To filter the results to show only metrics that have had data points published in the past three hours,
* specify this parameter with a value of PT3H
. This is the only valid value for this
* parameter.
*
* The results that are returned are an approximation of the value you specify. There is a low probability
* that the returned results include metrics with last published data as much as 40 minutes more than the
* specified time interval.
* @see RecentlyActive
*/
public final RecentlyActive recentlyActive() {
return RecentlyActive.fromValue(recentlyActive);
}
/**
*
* To filter the results to show only metrics that have had data points published in the past three hours, specify
* this parameter with a value of PT3H
. This is the only valid value for this parameter.
*
*
* The results that are returned are an approximation of the value you specify. There is a low probability that the
* returned results include metrics with last published data as much as 40 minutes more than the specified time
* interval.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #recentlyActive}
* will return {@link RecentlyActive#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #recentlyActiveAsString}.
*
*
* @return To filter the results to show only metrics that have had data points published in the past three hours,
* specify this parameter with a value of PT3H
. This is the only valid value for this
* parameter.
*
* The results that are returned are an approximation of the value you specify. There is a low probability
* that the returned results include metrics with last published data as much as 40 minutes more than the
* specified time interval.
* @see RecentlyActive
*/
public final String recentlyActiveAsString() {
return recentlyActive;
}
/**
*
* If you are using this operation in a monitoring account, specify true
to include metrics from source
* accounts in the returned data.
*
*
* The default is false
.
*
*
* @return If you are using this operation in a monitoring account, specify true
to include metrics
* from source accounts in the returned data.
*
* The default is false
.
*/
public final Boolean includeLinkedAccounts() {
return includeLinkedAccounts;
}
/**
*
* When you use this operation in a monitoring account, use this field to return metrics only from one source
* account. To do so, specify that source account ID in this field, and also specify true
for
* IncludeLinkedAccounts
.
*
*
* @return When you use this operation in a monitoring account, use this field to return metrics only from one
* source account. To do so, specify that source account ID in this field, and also specify
* true
for IncludeLinkedAccounts
.
*/
public final String owningAccount() {
return owningAccount;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(metricName());
hashCode = 31 * hashCode + Objects.hashCode(hasDimensions() ? dimensions() : null);
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(recentlyActiveAsString());
hashCode = 31 * hashCode + Objects.hashCode(includeLinkedAccounts());
hashCode = 31 * hashCode + Objects.hashCode(owningAccount());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListMetricsRequest)) {
return false;
}
ListMetricsRequest other = (ListMetricsRequest) obj;
return Objects.equals(namespace(), other.namespace()) && Objects.equals(metricName(), other.metricName())
&& hasDimensions() == other.hasDimensions() && Objects.equals(dimensions(), other.dimensions())
&& Objects.equals(nextToken(), other.nextToken())
&& Objects.equals(recentlyActiveAsString(), other.recentlyActiveAsString())
&& Objects.equals(includeLinkedAccounts(), other.includeLinkedAccounts())
&& Objects.equals(owningAccount(), other.owningAccount());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListMetricsRequest").add("Namespace", namespace()).add("MetricName", metricName())
.add("Dimensions", hasDimensions() ? dimensions() : null).add("NextToken", nextToken())
.add("RecentlyActive", recentlyActiveAsString()).add("IncludeLinkedAccounts", includeLinkedAccounts())
.add("OwningAccount", owningAccount()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "MetricName":
return Optional.ofNullable(clazz.cast(metricName()));
case "Dimensions":
return Optional.ofNullable(clazz.cast(dimensions()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "RecentlyActive":
return Optional.ofNullable(clazz.cast(recentlyActiveAsString()));
case "IncludeLinkedAccounts":
return Optional.ofNullable(clazz.cast(includeLinkedAccounts()));
case "OwningAccount":
return Optional.ofNullable(clazz.cast(owningAccount()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function