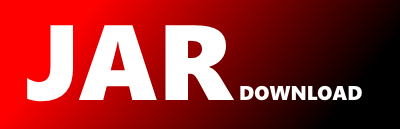
software.amazon.awssdk.services.cloudwatch.model.GetInsightRuleReportResponse Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetInsightRuleReportResponse extends CloudWatchResponse implements
ToCopyableBuilder {
private static final SdkField> KEY_LABELS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("KeyLabels")
.getter(getter(GetInsightRuleReportResponse::keyLabels))
.setter(setter(Builder::keyLabels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyLabels").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField AGGREGATION_STATISTIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AggregationStatistic").getter(getter(GetInsightRuleReportResponse::aggregationStatistic))
.setter(setter(Builder::aggregationStatistic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AggregationStatistic").build())
.build();
private static final SdkField AGGREGATE_VALUE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("AggregateValue").getter(getter(GetInsightRuleReportResponse::aggregateValue))
.setter(setter(Builder::aggregateValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AggregateValue").build()).build();
private static final SdkField APPROXIMATE_UNIQUE_COUNT_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ApproximateUniqueCount").getter(getter(GetInsightRuleReportResponse::approximateUniqueCount))
.setter(setter(Builder::approximateUniqueCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApproximateUniqueCount").build())
.build();
private static final SdkField> CONTRIBUTORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Contributors")
.getter(getter(GetInsightRuleReportResponse::contributors))
.setter(setter(Builder::contributors))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Contributors").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InsightRuleContributor::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> METRIC_DATAPOINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MetricDatapoints")
.getter(getter(GetInsightRuleReportResponse::metricDatapoints))
.setter(setter(Builder::metricDatapoints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricDatapoints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InsightRuleMetricDatapoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(KEY_LABELS_FIELD,
AGGREGATION_STATISTIC_FIELD, AGGREGATE_VALUE_FIELD, APPROXIMATE_UNIQUE_COUNT_FIELD, CONTRIBUTORS_FIELD,
METRIC_DATAPOINTS_FIELD));
private final List keyLabels;
private final String aggregationStatistic;
private final Double aggregateValue;
private final Long approximateUniqueCount;
private final List contributors;
private final List metricDatapoints;
private GetInsightRuleReportResponse(BuilderImpl builder) {
super(builder);
this.keyLabels = builder.keyLabels;
this.aggregationStatistic = builder.aggregationStatistic;
this.aggregateValue = builder.aggregateValue;
this.approximateUniqueCount = builder.approximateUniqueCount;
this.contributors = builder.contributors;
this.metricDatapoints = builder.metricDatapoints;
}
/**
* For responses, this returns true if the service returned a value for the KeyLabels property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasKeyLabels() {
return keyLabels != null && !(keyLabels instanceof SdkAutoConstructList);
}
/**
*
* An array of the strings used as the keys for this rule. The keys are the dimensions used to classify
* contributors. If the rule contains more than one key, then each unique combination of values for the keys is
* counted as a unique contributor.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasKeyLabels} method.
*
*
* @return An array of the strings used as the keys for this rule. The keys are the dimensions used to classify
* contributors. If the rule contains more than one key, then each unique combination of values for the keys
* is counted as a unique contributor.
*/
public final List keyLabels() {
return keyLabels;
}
/**
*
* Specifies whether this rule aggregates contributor data by COUNT or SUM.
*
*
* @return Specifies whether this rule aggregates contributor data by COUNT or SUM.
*/
public final String aggregationStatistic() {
return aggregationStatistic;
}
/**
*
* The sum of the values from all individual contributors that match the rule.
*
*
* @return The sum of the values from all individual contributors that match the rule.
*/
public final Double aggregateValue() {
return aggregateValue;
}
/**
*
* An approximate count of the unique contributors found by this rule in this time period.
*
*
* @return An approximate count of the unique contributors found by this rule in this time period.
*/
public final Long approximateUniqueCount() {
return approximateUniqueCount;
}
/**
* For responses, this returns true if the service returned a value for the Contributors property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasContributors() {
return contributors != null && !(contributors instanceof SdkAutoConstructList);
}
/**
*
* An array of the unique contributors found by this rule in this time period. If the rule contains multiple keys,
* each combination of values for the keys counts as a unique contributor.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContributors} method.
*
*
* @return An array of the unique contributors found by this rule in this time period. If the rule contains multiple
* keys, each combination of values for the keys counts as a unique contributor.
*/
public final List contributors() {
return contributors;
}
/**
* For responses, this returns true if the service returned a value for the MetricDatapoints property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMetricDatapoints() {
return metricDatapoints != null && !(metricDatapoints instanceof SdkAutoConstructList);
}
/**
*
* A time series of metric data points that matches the time period in the rule request.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMetricDatapoints} method.
*
*
* @return A time series of metric data points that matches the time period in the rule request.
*/
public final List metricDatapoints() {
return metricDatapoints;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasKeyLabels() ? keyLabels() : null);
hashCode = 31 * hashCode + Objects.hashCode(aggregationStatistic());
hashCode = 31 * hashCode + Objects.hashCode(aggregateValue());
hashCode = 31 * hashCode + Objects.hashCode(approximateUniqueCount());
hashCode = 31 * hashCode + Objects.hashCode(hasContributors() ? contributors() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasMetricDatapoints() ? metricDatapoints() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetInsightRuleReportResponse)) {
return false;
}
GetInsightRuleReportResponse other = (GetInsightRuleReportResponse) obj;
return hasKeyLabels() == other.hasKeyLabels() && Objects.equals(keyLabels(), other.keyLabels())
&& Objects.equals(aggregationStatistic(), other.aggregationStatistic())
&& Objects.equals(aggregateValue(), other.aggregateValue())
&& Objects.equals(approximateUniqueCount(), other.approximateUniqueCount())
&& hasContributors() == other.hasContributors() && Objects.equals(contributors(), other.contributors())
&& hasMetricDatapoints() == other.hasMetricDatapoints()
&& Objects.equals(metricDatapoints(), other.metricDatapoints());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetInsightRuleReportResponse").add("KeyLabels", hasKeyLabels() ? keyLabels() : null)
.add("AggregationStatistic", aggregationStatistic()).add("AggregateValue", aggregateValue())
.add("ApproximateUniqueCount", approximateUniqueCount())
.add("Contributors", hasContributors() ? contributors() : null)
.add("MetricDatapoints", hasMetricDatapoints() ? metricDatapoints() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "KeyLabels":
return Optional.ofNullable(clazz.cast(keyLabels()));
case "AggregationStatistic":
return Optional.ofNullable(clazz.cast(aggregationStatistic()));
case "AggregateValue":
return Optional.ofNullable(clazz.cast(aggregateValue()));
case "ApproximateUniqueCount":
return Optional.ofNullable(clazz.cast(approximateUniqueCount()));
case "Contributors":
return Optional.ofNullable(clazz.cast(contributors()));
case "MetricDatapoints":
return Optional.ofNullable(clazz.cast(metricDatapoints()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function