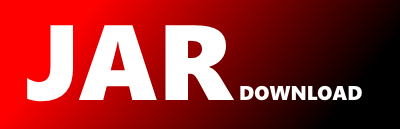
software.amazon.awssdk.services.cloudwatch.model.GetMetricStreamResponse Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetMetricStreamResponse extends CloudWatchResponse implements
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(GetMetricStreamResponse::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(GetMetricStreamResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField> INCLUDE_FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("IncludeFilters")
.getter(getter(GetMetricStreamResponse::includeFilters))
.setter(setter(Builder::includeFilters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeFilters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricStreamFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> EXCLUDE_FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExcludeFilters")
.getter(getter(GetMetricStreamResponse::excludeFilters))
.setter(setter(Builder::excludeFilters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExcludeFilters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricStreamFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FIREHOSE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FirehoseArn").getter(getter(GetMetricStreamResponse::firehoseArn)).setter(setter(Builder::firehoseArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FirehoseArn").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(GetMetricStreamResponse::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("State")
.getter(getter(GetMetricStreamResponse::state)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationDate").getter(getter(GetMetricStreamResponse::creationDate))
.setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationDate").build()).build();
private static final SdkField LAST_UPDATE_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdateDate").getter(getter(GetMetricStreamResponse::lastUpdateDate))
.setter(setter(Builder::lastUpdateDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdateDate").build()).build();
private static final SdkField OUTPUT_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputFormat").getter(getter(GetMetricStreamResponse::outputFormatAsString))
.setter(setter(Builder::outputFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputFormat").build()).build();
private static final SdkField> STATISTICS_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatisticsConfigurations")
.getter(getter(GetMetricStreamResponse::statisticsConfigurations))
.setter(setter(Builder::statisticsConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatisticsConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricStreamStatisticsConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INCLUDE_LINKED_ACCOUNTS_METRICS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IncludeLinkedAccountsMetrics")
.getter(getter(GetMetricStreamResponse::includeLinkedAccountsMetrics))
.setter(setter(Builder::includeLinkedAccountsMetrics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeLinkedAccountsMetrics")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
INCLUDE_FILTERS_FIELD, EXCLUDE_FILTERS_FIELD, FIREHOSE_ARN_FIELD, ROLE_ARN_FIELD, STATE_FIELD, CREATION_DATE_FIELD,
LAST_UPDATE_DATE_FIELD, OUTPUT_FORMAT_FIELD, STATISTICS_CONFIGURATIONS_FIELD, INCLUDE_LINKED_ACCOUNTS_METRICS_FIELD));
private final String arn;
private final String name;
private final List includeFilters;
private final List excludeFilters;
private final String firehoseArn;
private final String roleArn;
private final String state;
private final Instant creationDate;
private final Instant lastUpdateDate;
private final String outputFormat;
private final List statisticsConfigurations;
private final Boolean includeLinkedAccountsMetrics;
private GetMetricStreamResponse(BuilderImpl builder) {
super(builder);
this.arn = builder.arn;
this.name = builder.name;
this.includeFilters = builder.includeFilters;
this.excludeFilters = builder.excludeFilters;
this.firehoseArn = builder.firehoseArn;
this.roleArn = builder.roleArn;
this.state = builder.state;
this.creationDate = builder.creationDate;
this.lastUpdateDate = builder.lastUpdateDate;
this.outputFormat = builder.outputFormat;
this.statisticsConfigurations = builder.statisticsConfigurations;
this.includeLinkedAccountsMetrics = builder.includeLinkedAccountsMetrics;
}
/**
*
* The ARN of the metric stream.
*
*
* @return The ARN of the metric stream.
*/
public final String arn() {
return arn;
}
/**
*
* The name of the metric stream.
*
*
* @return The name of the metric stream.
*/
public final String name() {
return name;
}
/**
* For responses, this returns true if the service returned a value for the IncludeFilters property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasIncludeFilters() {
return includeFilters != null && !(includeFilters instanceof SdkAutoConstructList);
}
/**
*
* If this array of metric namespaces is present, then these namespaces are the only metric namespaces that are
* streamed by this metric stream.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIncludeFilters} method.
*
*
* @return If this array of metric namespaces is present, then these namespaces are the only metric namespaces that
* are streamed by this metric stream.
*/
public final List includeFilters() {
return includeFilters;
}
/**
* For responses, this returns true if the service returned a value for the ExcludeFilters property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasExcludeFilters() {
return excludeFilters != null && !(excludeFilters instanceof SdkAutoConstructList);
}
/**
*
* If this array of metric namespaces is present, then these namespaces are the only metric namespaces that are not
* streamed by this metric stream. In this case, all other metric namespaces in the account are streamed by this
* metric stream.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExcludeFilters} method.
*
*
* @return If this array of metric namespaces is present, then these namespaces are the only metric namespaces that
* are not streamed by this metric stream. In this case, all other metric namespaces in the account are
* streamed by this metric stream.
*/
public final List excludeFilters() {
return excludeFilters;
}
/**
*
* The ARN of the Amazon Kinesis Data Firehose delivery stream that is used by this metric stream.
*
*
* @return The ARN of the Amazon Kinesis Data Firehose delivery stream that is used by this metric stream.
*/
public final String firehoseArn() {
return firehoseArn;
}
/**
*
* The ARN of the IAM role that is used by this metric stream.
*
*
* @return The ARN of the IAM role that is used by this metric stream.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The state of the metric stream. The possible values are running
and stopped
.
*
*
* @return The state of the metric stream. The possible values are running
and stopped
.
*/
public final String state() {
return state;
}
/**
*
* The date that the metric stream was created.
*
*
* @return The date that the metric stream was created.
*/
public final Instant creationDate() {
return creationDate;
}
/**
*
* The date of the most recent update to the metric stream's configuration.
*
*
* @return The date of the most recent update to the metric stream's configuration.
*/
public final Instant lastUpdateDate() {
return lastUpdateDate;
}
/**
*
* The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputFormat} will
* return {@link MetricStreamOutputFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #outputFormatAsString}.
*
*
* @return The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
* @see MetricStreamOutputFormat
*/
public final MetricStreamOutputFormat outputFormat() {
return MetricStreamOutputFormat.fromValue(outputFormat);
}
/**
*
* The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputFormat} will
* return {@link MetricStreamOutputFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #outputFormatAsString}.
*
*
* @return The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
* @see MetricStreamOutputFormat
*/
public final String outputFormatAsString() {
return outputFormat;
}
/**
* For responses, this returns true if the service returned a value for the StatisticsConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasStatisticsConfigurations() {
return statisticsConfigurations != null && !(statisticsConfigurations instanceof SdkAutoConstructList);
}
/**
*
* Each entry in this array displays information about one or more metrics that include additional statistics in the
* metric stream. For more information about the additional statistics, see
* CloudWatch statistics definitions.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatisticsConfigurations} method.
*
*
* @return Each entry in this array displays information about one or more metrics that include additional
* statistics in the metric stream. For more information about the additional statistics, see
* CloudWatch statistics definitions.
*/
public final List statisticsConfigurations() {
return statisticsConfigurations;
}
/**
*
* If this is true
and this metric stream is in a monitoring account, then the stream includes metrics
* from source accounts that the monitoring account is linked to.
*
*
* @return If this is true
and this metric stream is in a monitoring account, then the stream includes
* metrics from source accounts that the monitoring account is linked to.
*/
public final Boolean includeLinkedAccountsMetrics() {
return includeLinkedAccountsMetrics;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(hasIncludeFilters() ? includeFilters() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasExcludeFilters() ? excludeFilters() : null);
hashCode = 31 * hashCode + Objects.hashCode(firehoseArn());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(state());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdateDate());
hashCode = 31 * hashCode + Objects.hashCode(outputFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasStatisticsConfigurations() ? statisticsConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(includeLinkedAccountsMetrics());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetMetricStreamResponse)) {
return false;
}
GetMetricStreamResponse other = (GetMetricStreamResponse) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& hasIncludeFilters() == other.hasIncludeFilters() && Objects.equals(includeFilters(), other.includeFilters())
&& hasExcludeFilters() == other.hasExcludeFilters() && Objects.equals(excludeFilters(), other.excludeFilters())
&& Objects.equals(firehoseArn(), other.firehoseArn()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(state(), other.state()) && Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(lastUpdateDate(), other.lastUpdateDate())
&& Objects.equals(outputFormatAsString(), other.outputFormatAsString())
&& hasStatisticsConfigurations() == other.hasStatisticsConfigurations()
&& Objects.equals(statisticsConfigurations(), other.statisticsConfigurations())
&& Objects.equals(includeLinkedAccountsMetrics(), other.includeLinkedAccountsMetrics());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetMetricStreamResponse").add("Arn", arn()).add("Name", name())
.add("IncludeFilters", hasIncludeFilters() ? includeFilters() : null)
.add("ExcludeFilters", hasExcludeFilters() ? excludeFilters() : null).add("FirehoseArn", firehoseArn())
.add("RoleArn", roleArn()).add("State", state()).add("CreationDate", creationDate())
.add("LastUpdateDate", lastUpdateDate()).add("OutputFormat", outputFormatAsString())
.add("StatisticsConfigurations", hasStatisticsConfigurations() ? statisticsConfigurations() : null)
.add("IncludeLinkedAccountsMetrics", includeLinkedAccountsMetrics()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "IncludeFilters":
return Optional.ofNullable(clazz.cast(includeFilters()));
case "ExcludeFilters":
return Optional.ofNullable(clazz.cast(excludeFilters()));
case "FirehoseArn":
return Optional.ofNullable(clazz.cast(firehoseArn()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "State":
return Optional.ofNullable(clazz.cast(state()));
case "CreationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "LastUpdateDate":
return Optional.ofNullable(clazz.cast(lastUpdateDate()));
case "OutputFormat":
return Optional.ofNullable(clazz.cast(outputFormatAsString()));
case "StatisticsConfigurations":
return Optional.ofNullable(clazz.cast(statisticsConfigurations()));
case "IncludeLinkedAccountsMetrics":
return Optional.ofNullable(clazz.cast(includeLinkedAccountsMetrics()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function