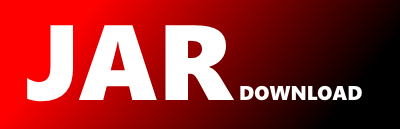
software.amazon.awssdk.services.cloudwatch.model.GetMetricDataRequest Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetMetricDataRequest extends CloudWatchRequest implements
ToCopyableBuilder {
private static final SdkField> METRIC_DATA_QUERIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MetricDataQueries")
.getter(getter(GetMetricDataRequest::metricDataQueries))
.setter(setter(Builder::metricDataQueries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricDataQueries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricDataQuery::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(GetMetricDataRequest::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(GetMetricDataRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextToken").getter(getter(GetMetricDataRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken").build()).build();
private static final SdkField SCAN_BY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ScanBy")
.getter(getter(GetMetricDataRequest::scanByAsString)).setter(setter(Builder::scanBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScanBy").build()).build();
private static final SdkField MAX_DATAPOINTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxDatapoints").getter(getter(GetMetricDataRequest::maxDatapoints))
.setter(setter(Builder::maxDatapoints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxDatapoints").build()).build();
private static final SdkField LABEL_OPTIONS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("LabelOptions").getter(getter(GetMetricDataRequest::labelOptions)).setter(setter(Builder::labelOptions))
.constructor(LabelOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LabelOptions").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(METRIC_DATA_QUERIES_FIELD,
START_TIME_FIELD, END_TIME_FIELD, NEXT_TOKEN_FIELD, SCAN_BY_FIELD, MAX_DATAPOINTS_FIELD, LABEL_OPTIONS_FIELD));
private final List metricDataQueries;
private final Instant startTime;
private final Instant endTime;
private final String nextToken;
private final String scanBy;
private final Integer maxDatapoints;
private final LabelOptions labelOptions;
private GetMetricDataRequest(BuilderImpl builder) {
super(builder);
this.metricDataQueries = builder.metricDataQueries;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.nextToken = builder.nextToken;
this.scanBy = builder.scanBy;
this.maxDatapoints = builder.maxDatapoints;
this.labelOptions = builder.labelOptions;
}
/**
* For responses, this returns true if the service returned a value for the MetricDataQueries property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMetricDataQueries() {
return metricDataQueries != null && !(metricDataQueries instanceof SdkAutoConstructList);
}
/**
*
* The metric queries to be returned. A single GetMetricData
call can include as many as 500
* MetricDataQuery
structures. Each of these structures can specify either a metric to retrieve, a
* Metrics Insights query, or a math expression to perform on retrieved data.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMetricDataQueries} method.
*
*
* @return The metric queries to be returned. A single GetMetricData
call can include as many as 500
* MetricDataQuery
structures. Each of these structures can specify either a metric to
* retrieve, a Metrics Insights query, or a math expression to perform on retrieved data.
*/
public final List metricDataQueries() {
return metricDataQueries;
}
/**
*
* The time stamp indicating the earliest data to be returned.
*
*
* The value specified is inclusive; results include data points with the specified time stamp.
*
*
* CloudWatch rounds the specified time stamp as follows:
*
*
* -
*
* Start time less than 15 days ago - Round down to the nearest whole minute. For example, 12:32:34 is rounded down
* to 12:32:00.
*
*
* -
*
* Start time between 15 and 63 days ago - Round down to the nearest 5-minute clock interval. For example, 12:32:34
* is rounded down to 12:30:00.
*
*
* -
*
* Start time greater than 63 days ago - Round down to the nearest 1-hour clock interval. For example, 12:32:34 is
* rounded down to 12:00:00.
*
*
*
*
* If you set Period
to 5, 10, or 30, the start time of your request is rounded down to the nearest
* time that corresponds to even 5-, 10-, or 30-second divisions of a minute. For example, if you make a query at
* (HH:mm:ss) 01:05:23 for the previous 10-second period, the start time of your request is rounded down and you
* receive data from 01:05:10 to 01:05:20. If you make a query at 15:07:17 for the previous 5 minutes of data, using
* a period of 5 seconds, you receive data timestamped between 15:02:15 and 15:07:15.
*
*
* For better performance, specify StartTime
and EndTime
values that align with the value
* of the metric's Period
and sync up with the beginning and end of an hour. For example, if the
* Period
of a metric is 5 minutes, specifying 12:05 or 12:30 as StartTime
can get a
* faster response from CloudWatch than setting 12:07 or 12:29 as the StartTime
.
*
*
* @return The time stamp indicating the earliest data to be returned.
*
* The value specified is inclusive; results include data points with the specified time stamp.
*
*
* CloudWatch rounds the specified time stamp as follows:
*
*
* -
*
* Start time less than 15 days ago - Round down to the nearest whole minute. For example, 12:32:34 is
* rounded down to 12:32:00.
*
*
* -
*
* Start time between 15 and 63 days ago - Round down to the nearest 5-minute clock interval. For example,
* 12:32:34 is rounded down to 12:30:00.
*
*
* -
*
* Start time greater than 63 days ago - Round down to the nearest 1-hour clock interval. For example,
* 12:32:34 is rounded down to 12:00:00.
*
*
*
*
* If you set Period
to 5, 10, or 30, the start time of your request is rounded down to the
* nearest time that corresponds to even 5-, 10-, or 30-second divisions of a minute. For example, if you
* make a query at (HH:mm:ss) 01:05:23 for the previous 10-second period, the start time of your request is
* rounded down and you receive data from 01:05:10 to 01:05:20. If you make a query at 15:07:17 for the
* previous 5 minutes of data, using a period of 5 seconds, you receive data timestamped between 15:02:15
* and 15:07:15.
*
*
* For better performance, specify StartTime
and EndTime
values that align with
* the value of the metric's Period
and sync up with the beginning and end of an hour. For
* example, if the Period
of a metric is 5 minutes, specifying 12:05 or 12:30 as
* StartTime
can get a faster response from CloudWatch than setting 12:07 or 12:29 as the
* StartTime
.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The time stamp indicating the latest data to be returned.
*
*
* The value specified is exclusive; results include data points up to the specified time stamp.
*
*
* For better performance, specify StartTime
and EndTime
values that align with the value
* of the metric's Period
and sync up with the beginning and end of an hour. For example, if the
* Period
of a metric is 5 minutes, specifying 12:05 or 12:30 as EndTime
can get a faster
* response from CloudWatch than setting 12:07 or 12:29 as the EndTime
.
*
*
* @return The time stamp indicating the latest data to be returned.
*
* The value specified is exclusive; results include data points up to the specified time stamp.
*
*
* For better performance, specify StartTime
and EndTime
values that align with
* the value of the metric's Period
and sync up with the beginning and end of an hour. For
* example, if the Period
of a metric is 5 minutes, specifying 12:05 or 12:30 as
* EndTime
can get a faster response from CloudWatch than setting 12:07 or 12:29 as the
* EndTime
.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* Include this value, if it was returned by the previous GetMetricData
operation, to get the next set
* of data points.
*
*
* @return Include this value, if it was returned by the previous GetMetricData
operation, to get the
* next set of data points.
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The order in which data points should be returned. TimestampDescending
returns the newest data first
* and paginates when the MaxDatapoints
limit is reached. TimestampAscending
returns the
* oldest data first and paginates when the MaxDatapoints
limit is reached.
*
*
* If you omit this parameter, the default of TimestampDescending
is used.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scanBy} will
* return {@link ScanBy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scanByAsString}.
*
*
* @return The order in which data points should be returned. TimestampDescending
returns the newest
* data first and paginates when the MaxDatapoints
limit is reached.
* TimestampAscending
returns the oldest data first and paginates when the
* MaxDatapoints
limit is reached.
*
* If you omit this parameter, the default of TimestampDescending
is used.
* @see ScanBy
*/
public final ScanBy scanBy() {
return ScanBy.fromValue(scanBy);
}
/**
*
* The order in which data points should be returned. TimestampDescending
returns the newest data first
* and paginates when the MaxDatapoints
limit is reached. TimestampAscending
returns the
* oldest data first and paginates when the MaxDatapoints
limit is reached.
*
*
* If you omit this parameter, the default of TimestampDescending
is used.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scanBy} will
* return {@link ScanBy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scanByAsString}.
*
*
* @return The order in which data points should be returned. TimestampDescending
returns the newest
* data first and paginates when the MaxDatapoints
limit is reached.
* TimestampAscending
returns the oldest data first and paginates when the
* MaxDatapoints
limit is reached.
*
* If you omit this parameter, the default of TimestampDescending
is used.
* @see ScanBy
*/
public final String scanByAsString() {
return scanBy;
}
/**
*
* The maximum number of data points the request should return before paginating. If you omit this, the default of
* 100,800 is used.
*
*
* @return The maximum number of data points the request should return before paginating. If you omit this, the
* default of 100,800 is used.
*/
public final Integer maxDatapoints() {
return maxDatapoints;
}
/**
*
* This structure includes the Timezone
parameter, which you can use to specify your time zone so that
* the labels of returned data display the correct time for your time zone.
*
*
* @return This structure includes the Timezone
parameter, which you can use to specify your time zone
* so that the labels of returned data display the correct time for your time zone.
*/
public final LabelOptions labelOptions() {
return labelOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasMetricDataQueries() ? metricDataQueries() : null);
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(scanByAsString());
hashCode = 31 * hashCode + Objects.hashCode(maxDatapoints());
hashCode = 31 * hashCode + Objects.hashCode(labelOptions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetMetricDataRequest)) {
return false;
}
GetMetricDataRequest other = (GetMetricDataRequest) obj;
return hasMetricDataQueries() == other.hasMetricDataQueries()
&& Objects.equals(metricDataQueries(), other.metricDataQueries())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(nextToken(), other.nextToken()) && Objects.equals(scanByAsString(), other.scanByAsString())
&& Objects.equals(maxDatapoints(), other.maxDatapoints()) && Objects.equals(labelOptions(), other.labelOptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetMetricDataRequest")
.add("MetricDataQueries", hasMetricDataQueries() ? metricDataQueries() : null).add("StartTime", startTime())
.add("EndTime", endTime()).add("NextToken", nextToken()).add("ScanBy", scanByAsString())
.add("MaxDatapoints", maxDatapoints()).add("LabelOptions", labelOptions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MetricDataQueries":
return Optional.ofNullable(clazz.cast(metricDataQueries()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "ScanBy":
return Optional.ofNullable(clazz.cast(scanByAsString()));
case "MaxDatapoints":
return Optional.ofNullable(clazz.cast(maxDatapoints()));
case "LabelOptions":
return Optional.ofNullable(clazz.cast(labelOptions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function