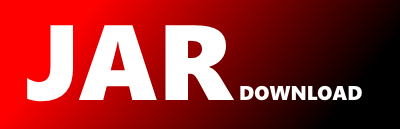
software.amazon.awssdk.services.cloudwatch.model.PutMetricStreamRequest Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutMetricStreamRequest extends CloudWatchRequest implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(PutMetricStreamRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField> INCLUDE_FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("IncludeFilters")
.getter(getter(PutMetricStreamRequest::includeFilters))
.setter(setter(Builder::includeFilters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeFilters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricStreamFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> EXCLUDE_FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExcludeFilters")
.getter(getter(PutMetricStreamRequest::excludeFilters))
.setter(setter(Builder::excludeFilters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExcludeFilters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricStreamFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FIREHOSE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FirehoseArn").getter(getter(PutMetricStreamRequest::firehoseArn)).setter(setter(Builder::firehoseArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FirehoseArn").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(PutMetricStreamRequest::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField OUTPUT_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputFormat").getter(getter(PutMetricStreamRequest::outputFormatAsString))
.setter(setter(Builder::outputFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputFormat").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(PutMetricStreamRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> STATISTICS_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StatisticsConfigurations")
.getter(getter(PutMetricStreamRequest::statisticsConfigurations))
.setter(setter(Builder::statisticsConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatisticsConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricStreamStatisticsConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INCLUDE_LINKED_ACCOUNTS_METRICS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IncludeLinkedAccountsMetrics")
.getter(getter(PutMetricStreamRequest::includeLinkedAccountsMetrics))
.setter(setter(Builder::includeLinkedAccountsMetrics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeLinkedAccountsMetrics")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD,
INCLUDE_FILTERS_FIELD, EXCLUDE_FILTERS_FIELD, FIREHOSE_ARN_FIELD, ROLE_ARN_FIELD, OUTPUT_FORMAT_FIELD, TAGS_FIELD,
STATISTICS_CONFIGURATIONS_FIELD, INCLUDE_LINKED_ACCOUNTS_METRICS_FIELD));
private final String name;
private final List includeFilters;
private final List excludeFilters;
private final String firehoseArn;
private final String roleArn;
private final String outputFormat;
private final List tags;
private final List statisticsConfigurations;
private final Boolean includeLinkedAccountsMetrics;
private PutMetricStreamRequest(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.includeFilters = builder.includeFilters;
this.excludeFilters = builder.excludeFilters;
this.firehoseArn = builder.firehoseArn;
this.roleArn = builder.roleArn;
this.outputFormat = builder.outputFormat;
this.tags = builder.tags;
this.statisticsConfigurations = builder.statisticsConfigurations;
this.includeLinkedAccountsMetrics = builder.includeLinkedAccountsMetrics;
}
/**
*
* If you are creating a new metric stream, this is the name for the new stream. The name must be different than the
* names of other metric streams in this account and Region.
*
*
* If you are updating a metric stream, specify the name of that stream here.
*
*
* Valid characters are A-Z, a-z, 0-9, "-" and "_".
*
*
* @return If you are creating a new metric stream, this is the name for the new stream. The name must be different
* than the names of other metric streams in this account and Region.
*
* If you are updating a metric stream, specify the name of that stream here.
*
*
* Valid characters are A-Z, a-z, 0-9, "-" and "_".
*/
public final String name() {
return name;
}
/**
* For responses, this returns true if the service returned a value for the IncludeFilters property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasIncludeFilters() {
return includeFilters != null && !(includeFilters instanceof SdkAutoConstructList);
}
/**
*
* If you specify this parameter, the stream sends only the metrics from the metric namespaces that you specify
* here.
*
*
* You cannot include IncludeFilters
and ExcludeFilters
in the same operation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIncludeFilters} method.
*
*
* @return If you specify this parameter, the stream sends only the metrics from the metric namespaces that you
* specify here.
*
* You cannot include IncludeFilters
and ExcludeFilters
in the same operation.
*/
public final List includeFilters() {
return includeFilters;
}
/**
* For responses, this returns true if the service returned a value for the ExcludeFilters property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasExcludeFilters() {
return excludeFilters != null && !(excludeFilters instanceof SdkAutoConstructList);
}
/**
*
* If you specify this parameter, the stream sends metrics from all metric namespaces except for the namespaces that
* you specify here.
*
*
* You cannot include ExcludeFilters
and IncludeFilters
in the same operation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExcludeFilters} method.
*
*
* @return If you specify this parameter, the stream sends metrics from all metric namespaces except for the
* namespaces that you specify here.
*
* You cannot include ExcludeFilters
and IncludeFilters
in the same operation.
*/
public final List excludeFilters() {
return excludeFilters;
}
/**
*
* The ARN of the Amazon Kinesis Data Firehose delivery stream to use for this metric stream. This Amazon Kinesis
* Data Firehose delivery stream must already exist and must be in the same account as the metric stream.
*
*
* @return The ARN of the Amazon Kinesis Data Firehose delivery stream to use for this metric stream. This Amazon
* Kinesis Data Firehose delivery stream must already exist and must be in the same account as the metric
* stream.
*/
public final String firehoseArn() {
return firehoseArn;
}
/**
*
* The ARN of an IAM role that this metric stream will use to access Amazon Kinesis Data Firehose resources. This
* IAM role must already exist and must be in the same account as the metric stream. This IAM role must include the
* following permissions:
*
*
* -
*
* firehose:PutRecord
*
*
* -
*
* firehose:PutRecordBatch
*
*
*
*
* @return The ARN of an IAM role that this metric stream will use to access Amazon Kinesis Data Firehose resources.
* This IAM role must already exist and must be in the same account as the metric stream. This IAM role must
* include the following permissions:
*
* -
*
* firehose:PutRecord
*
*
* -
*
* firehose:PutRecordBatch
*
*
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see
* Metric streams output formats.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputFormat} will
* return {@link MetricStreamOutputFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #outputFormatAsString}.
*
*
* @return The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
* @see MetricStreamOutputFormat
*/
public final MetricStreamOutputFormat outputFormat() {
return MetricStreamOutputFormat.fromValue(outputFormat);
}
/**
*
* The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see
* Metric streams output formats.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #outputFormat} will
* return {@link MetricStreamOutputFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #outputFormatAsString}.
*
*
* @return The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
* @see MetricStreamOutputFormat
*/
public final String outputFormatAsString() {
return outputFormat;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of key-value pairs to associate with the metric stream. You can associate as many as 50 tags with a metric
* stream.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* You can use this parameter only when you are creating a new metric stream. If you are using this operation to
* update an existing metric stream, any tags you specify in this parameter are ignored. To change the tags of an
* existing metric stream, use TagResource or
* UntagResource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of key-value pairs to associate with the metric stream. You can associate as many as 50 tags with
* a metric stream.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions
* by granting a user permission to access or change only resources with certain tag values.
*
*
* You can use this parameter only when you are creating a new metric stream. If you are using this
* operation to update an existing metric stream, any tags you specify in this parameter are ignored. To
* change the tags of an existing metric stream, use TagResource or UntagResource.
*/
public final List tags() {
return tags;
}
/**
* For responses, this returns true if the service returned a value for the StatisticsConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasStatisticsConfigurations() {
return statisticsConfigurations != null && !(statisticsConfigurations instanceof SdkAutoConstructList);
}
/**
*
* By default, a metric stream always sends the MAX
, MIN
, SUM
, and
* SAMPLECOUNT
statistics for each metric that is streamed. You can use this parameter to have the
* metric stream also send additional statistics in the stream. This array can have up to 100 members.
*
*
* For each entry in this array, you specify one or more metrics and the list of additional statistics to stream for
* those metrics. The additional statistics that you can stream depend on the stream's OutputFormat
. If
* the OutputFormat
is json
, you can stream any additional statistic that is supported by
* CloudWatch, listed in
* CloudWatch statistics definitions. If the OutputFormat
is opentelemetry1.0
or
* opentelemetry0.7
, you can stream percentile statistics such as p95, p99.9, and so on.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatisticsConfigurations} method.
*
*
* @return By default, a metric stream always sends the MAX
, MIN
, SUM
, and
* SAMPLECOUNT
statistics for each metric that is streamed. You can use this parameter to have
* the metric stream also send additional statistics in the stream. This array can have up to 100
* members.
*
* For each entry in this array, you specify one or more metrics and the list of additional statistics to
* stream for those metrics. The additional statistics that you can stream depend on the stream's
* OutputFormat
. If the OutputFormat
is json
, you can stream any
* additional statistic that is supported by CloudWatch, listed in
* CloudWatch statistics definitions. If the OutputFormat
is opentelemetry1.0
* or opentelemetry0.7
, you can stream percentile statistics such as p95, p99.9, and so on.
*/
public final List statisticsConfigurations() {
return statisticsConfigurations;
}
/**
*
* If you are creating a metric stream in a monitoring account, specify true
to include metrics from
* source accounts in the metric stream.
*
*
* @return If you are creating a metric stream in a monitoring account, specify true
to include metrics
* from source accounts in the metric stream.
*/
public final Boolean includeLinkedAccountsMetrics() {
return includeLinkedAccountsMetrics;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(hasIncludeFilters() ? includeFilters() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasExcludeFilters() ? excludeFilters() : null);
hashCode = 31 * hashCode + Objects.hashCode(firehoseArn());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(outputFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasStatisticsConfigurations() ? statisticsConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(includeLinkedAccountsMetrics());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutMetricStreamRequest)) {
return false;
}
PutMetricStreamRequest other = (PutMetricStreamRequest) obj;
return Objects.equals(name(), other.name()) && hasIncludeFilters() == other.hasIncludeFilters()
&& Objects.equals(includeFilters(), other.includeFilters()) && hasExcludeFilters() == other.hasExcludeFilters()
&& Objects.equals(excludeFilters(), other.excludeFilters()) && Objects.equals(firehoseArn(), other.firehoseArn())
&& Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(outputFormatAsString(), other.outputFormatAsString()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && hasStatisticsConfigurations() == other.hasStatisticsConfigurations()
&& Objects.equals(statisticsConfigurations(), other.statisticsConfigurations())
&& Objects.equals(includeLinkedAccountsMetrics(), other.includeLinkedAccountsMetrics());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PutMetricStreamRequest").add("Name", name())
.add("IncludeFilters", hasIncludeFilters() ? includeFilters() : null)
.add("ExcludeFilters", hasExcludeFilters() ? excludeFilters() : null).add("FirehoseArn", firehoseArn())
.add("RoleArn", roleArn()).add("OutputFormat", outputFormatAsString()).add("Tags", hasTags() ? tags() : null)
.add("StatisticsConfigurations", hasStatisticsConfigurations() ? statisticsConfigurations() : null)
.add("IncludeLinkedAccountsMetrics", includeLinkedAccountsMetrics()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "IncludeFilters":
return Optional.ofNullable(clazz.cast(includeFilters()));
case "ExcludeFilters":
return Optional.ofNullable(clazz.cast(excludeFilters()));
case "FirehoseArn":
return Optional.ofNullable(clazz.cast(firehoseArn()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "OutputFormat":
return Optional.ofNullable(clazz.cast(outputFormatAsString()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "StatisticsConfigurations":
return Optional.ofNullable(clazz.cast(statisticsConfigurations()));
case "IncludeLinkedAccountsMetrics":
return Optional.ofNullable(clazz.cast(includeLinkedAccountsMetrics()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you are updating a metric stream, specify the name of that stream here.
*
*
* Valid characters are A-Z, a-z, 0-9, "-" and "_".
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
*
* If you specify this parameter, the stream sends only the metrics from the metric namespaces that you specify
* here.
*
*
* You cannot include IncludeFilters
and ExcludeFilters
in the same operation.
*
*
* @param includeFilters
* If you specify this parameter, the stream sends only the metrics from the metric namespaces that you
* specify here.
*
* You cannot include IncludeFilters
and ExcludeFilters
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder includeFilters(Collection includeFilters);
/**
*
* If you specify this parameter, the stream sends only the metrics from the metric namespaces that you specify
* here.
*
*
* You cannot include IncludeFilters
and ExcludeFilters
in the same operation.
*
*
* @param includeFilters
* If you specify this parameter, the stream sends only the metrics from the metric namespaces that you
* specify here.
*
* You cannot include IncludeFilters
and ExcludeFilters
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder includeFilters(MetricStreamFilter... includeFilters);
/**
*
* If you specify this parameter, the stream sends only the metrics from the metric namespaces that you specify
* here.
*
*
* You cannot include IncludeFilters
and ExcludeFilters
in the same operation.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter.Builder} avoiding the need to
* create one manually via {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter#builder()}
* .
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter.Builder#build()} is called
* immediately and its result is passed to {@link #includeFilters(List)}.
*
* @param includeFilters
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #includeFilters(java.util.Collection)
*/
Builder includeFilters(Consumer... includeFilters);
/**
*
* If you specify this parameter, the stream sends metrics from all metric namespaces except for the namespaces
* that you specify here.
*
*
* You cannot include ExcludeFilters
and IncludeFilters
in the same operation.
*
*
* @param excludeFilters
* If you specify this parameter, the stream sends metrics from all metric namespaces except for the
* namespaces that you specify here.
*
* You cannot include ExcludeFilters
and IncludeFilters
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder excludeFilters(Collection excludeFilters);
/**
*
* If you specify this parameter, the stream sends metrics from all metric namespaces except for the namespaces
* that you specify here.
*
*
* You cannot include ExcludeFilters
and IncludeFilters
in the same operation.
*
*
* @param excludeFilters
* If you specify this parameter, the stream sends metrics from all metric namespaces except for the
* namespaces that you specify here.
*
* You cannot include ExcludeFilters
and IncludeFilters
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder excludeFilters(MetricStreamFilter... excludeFilters);
/**
*
* If you specify this parameter, the stream sends metrics from all metric namespaces except for the namespaces
* that you specify here.
*
*
* You cannot include ExcludeFilters
and IncludeFilters
in the same operation.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter.Builder} avoiding the need to
* create one manually via {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter#builder()}
* .
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter.Builder#build()} is called
* immediately and its result is passed to {@link #excludeFilters(List)}.
*
* @param excludeFilters
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamFilter.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #excludeFilters(java.util.Collection)
*/
Builder excludeFilters(Consumer... excludeFilters);
/**
*
* The ARN of the Amazon Kinesis Data Firehose delivery stream to use for this metric stream. This Amazon
* Kinesis Data Firehose delivery stream must already exist and must be in the same account as the metric
* stream.
*
*
* @param firehoseArn
* The ARN of the Amazon Kinesis Data Firehose delivery stream to use for this metric stream. This Amazon
* Kinesis Data Firehose delivery stream must already exist and must be in the same account as the metric
* stream.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder firehoseArn(String firehoseArn);
/**
*
* The ARN of an IAM role that this metric stream will use to access Amazon Kinesis Data Firehose resources.
* This IAM role must already exist and must be in the same account as the metric stream. This IAM role must
* include the following permissions:
*
*
* -
*
* firehose:PutRecord
*
*
* -
*
* firehose:PutRecordBatch
*
*
*
*
* @param roleArn
* The ARN of an IAM role that this metric stream will use to access Amazon Kinesis Data Firehose
* resources. This IAM role must already exist and must be in the same account as the metric stream. This
* IAM role must include the following permissions:
*
* -
*
* firehose:PutRecord
*
*
* -
*
* firehose:PutRecordBatch
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleArn(String roleArn);
/**
*
* The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see
* Metric streams output formats.
*
*
* @param outputFormat
* The output format for the stream. Valid values are json
, opentelemetry1.0
,
* and opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
* @see MetricStreamOutputFormat
* @return Returns a reference to this object so that method calls can be chained together.
* @see MetricStreamOutputFormat
*/
Builder outputFormat(String outputFormat);
/**
*
* The output format for the stream. Valid values are json
, opentelemetry1.0
, and
* opentelemetry0.7
. For more information about metric stream output formats, see
* Metric streams output formats.
*
*
* @param outputFormat
* The output format for the stream. Valid values are json
, opentelemetry1.0
,
* and opentelemetry0.7
. For more information about metric stream output formats, see Metric streams output formats.
* @see MetricStreamOutputFormat
* @return Returns a reference to this object so that method calls can be chained together.
* @see MetricStreamOutputFormat
*/
Builder outputFormat(MetricStreamOutputFormat outputFormat);
/**
*
* A list of key-value pairs to associate with the metric stream. You can associate as many as 50 tags with a
* metric stream.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* You can use this parameter only when you are creating a new metric stream. If you are using this operation to
* update an existing metric stream, any tags you specify in this parameter are ignored. To change the tags of
* an existing metric stream, use TagResource
* or
* UntagResource.
*
*
* @param tags
* A list of key-value pairs to associate with the metric stream. You can associate as many as 50 tags
* with a metric stream.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user
* permissions by granting a user permission to access or change only resources with certain tag values.
*
*
* You can use this parameter only when you are creating a new metric stream. If you are using this
* operation to update an existing metric stream, any tags you specify in this parameter are ignored. To
* change the tags of an existing metric stream, use TagResource or UntagResource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* A list of key-value pairs to associate with the metric stream. You can associate as many as 50 tags with a
* metric stream.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* You can use this parameter only when you are creating a new metric stream. If you are using this operation to
* update an existing metric stream, any tags you specify in this parameter are ignored. To change the tags of
* an existing metric stream, use TagResource
* or
* UntagResource.
*
*
* @param tags
* A list of key-value pairs to associate with the metric stream. You can associate as many as 50 tags
* with a metric stream.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user
* permissions by granting a user permission to access or change only resources with certain tag values.
*
*
* You can use this parameter only when you are creating a new metric stream. If you are using this
* operation to update an existing metric stream, any tags you specify in this parameter are ignored. To
* change the tags of an existing metric stream, use TagResource or UntagResource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* A list of key-value pairs to associate with the metric stream. You can associate as many as 50 tags with a
* metric stream.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* You can use this parameter only when you are creating a new metric stream. If you are using this operation to
* update an existing metric stream, any tags you specify in this parameter are ignored. To change the tags of
* an existing metric stream, use TagResource
* or
* UntagResource.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudwatch.model.Tag.Builder} avoiding the need to create one manually
* via {@link software.amazon.awssdk.services.cloudwatch.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudwatch.model.Tag.Builder#build()} is called immediately and its
* result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudwatch.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* By default, a metric stream always sends the MAX
, MIN
, SUM
, and
* SAMPLECOUNT
statistics for each metric that is streamed. You can use this parameter to have the
* metric stream also send additional statistics in the stream. This array can have up to 100 members.
*
*
* For each entry in this array, you specify one or more metrics and the list of additional statistics to stream
* for those metrics. The additional statistics that you can stream depend on the stream's
* OutputFormat
. If the OutputFormat
is json
, you can stream any
* additional statistic that is supported by CloudWatch, listed in
* CloudWatch statistics definitions. If the OutputFormat
is opentelemetry1.0
or
* opentelemetry0.7
, you can stream percentile statistics such as p95, p99.9, and so on.
*
*
* @param statisticsConfigurations
* By default, a metric stream always sends the MAX
, MIN
, SUM
, and
* SAMPLECOUNT
statistics for each metric that is streamed. You can use this parameter to
* have the metric stream also send additional statistics in the stream. This array can have up to 100
* members.
*
* For each entry in this array, you specify one or more metrics and the list of additional statistics to
* stream for those metrics. The additional statistics that you can stream depend on the stream's
* OutputFormat
. If the OutputFormat
is json
, you can stream any
* additional statistic that is supported by CloudWatch, listed in
* CloudWatch statistics definitions. If the OutputFormat
is
* opentelemetry1.0
or opentelemetry0.7
, you can stream percentile statistics
* such as p95, p99.9, and so on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statisticsConfigurations(Collection statisticsConfigurations);
/**
*
* By default, a metric stream always sends the MAX
, MIN
, SUM
, and
* SAMPLECOUNT
statistics for each metric that is streamed. You can use this parameter to have the
* metric stream also send additional statistics in the stream. This array can have up to 100 members.
*
*
* For each entry in this array, you specify one or more metrics and the list of additional statistics to stream
* for those metrics. The additional statistics that you can stream depend on the stream's
* OutputFormat
. If the OutputFormat
is json
, you can stream any
* additional statistic that is supported by CloudWatch, listed in
* CloudWatch statistics definitions. If the OutputFormat
is opentelemetry1.0
or
* opentelemetry0.7
, you can stream percentile statistics such as p95, p99.9, and so on.
*
*
* @param statisticsConfigurations
* By default, a metric stream always sends the MAX
, MIN
, SUM
, and
* SAMPLECOUNT
statistics for each metric that is streamed. You can use this parameter to
* have the metric stream also send additional statistics in the stream. This array can have up to 100
* members.
*
* For each entry in this array, you specify one or more metrics and the list of additional statistics to
* stream for those metrics. The additional statistics that you can stream depend on the stream's
* OutputFormat
. If the OutputFormat
is json
, you can stream any
* additional statistic that is supported by CloudWatch, listed in
* CloudWatch statistics definitions. If the OutputFormat
is
* opentelemetry1.0
or opentelemetry0.7
, you can stream percentile statistics
* such as p95, p99.9, and so on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statisticsConfigurations(MetricStreamStatisticsConfiguration... statisticsConfigurations);
/**
*
* By default, a metric stream always sends the MAX
, MIN
, SUM
, and
* SAMPLECOUNT
statistics for each metric that is streamed. You can use this parameter to have the
* metric stream also send additional statistics in the stream. This array can have up to 100 members.
*
*
* For each entry in this array, you specify one or more metrics and the list of additional statistics to stream
* for those metrics. The additional statistics that you can stream depend on the stream's
* OutputFormat
. If the OutputFormat
is json
, you can stream any
* additional statistic that is supported by CloudWatch, listed in
* CloudWatch statistics definitions. If the OutputFormat
is opentelemetry1.0
or
* opentelemetry0.7
, you can stream percentile statistics such as p95, p99.9, and so on.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamStatisticsConfiguration.Builder} avoiding
* the need to create one manually via
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamStatisticsConfiguration#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamStatisticsConfiguration.Builder#build()}
* is called immediately and its result is passed to {@link
* #statisticsConfigurations(List)}.
*
* @param statisticsConfigurations
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudwatch.model.MetricStreamStatisticsConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #statisticsConfigurations(java.util.Collection)
*/
Builder statisticsConfigurations(Consumer... statisticsConfigurations);
/**
*
* If you are creating a metric stream in a monitoring account, specify true
to include metrics
* from source accounts in the metric stream.
*
*
* @param includeLinkedAccountsMetrics
* If you are creating a metric stream in a monitoring account, specify true
to include
* metrics from source accounts in the metric stream.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder includeLinkedAccountsMetrics(Boolean includeLinkedAccountsMetrics);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudWatchRequest.BuilderImpl implements Builder {
private String name;
private List includeFilters = DefaultSdkAutoConstructList.getInstance();
private List excludeFilters = DefaultSdkAutoConstructList.getInstance();
private String firehoseArn;
private String roleArn;
private String outputFormat;
private List tags = DefaultSdkAutoConstructList.getInstance();
private List statisticsConfigurations = DefaultSdkAutoConstructList.getInstance();
private Boolean includeLinkedAccountsMetrics;
private BuilderImpl() {
}
private BuilderImpl(PutMetricStreamRequest model) {
super(model);
name(model.name);
includeFilters(model.includeFilters);
excludeFilters(model.excludeFilters);
firehoseArn(model.firehoseArn);
roleArn(model.roleArn);
outputFormat(model.outputFormat);
tags(model.tags);
statisticsConfigurations(model.statisticsConfigurations);
includeLinkedAccountsMetrics(model.includeLinkedAccountsMetrics);
}
public final String getName() {
return name;
}
public final void setName(String name) {
this.name = name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final List getIncludeFilters() {
List result = MetricStreamFiltersCopier.copyToBuilder(this.includeFilters);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setIncludeFilters(Collection includeFilters) {
this.includeFilters = MetricStreamFiltersCopier.copyFromBuilder(includeFilters);
}
@Override
public final Builder includeFilters(Collection includeFilters) {
this.includeFilters = MetricStreamFiltersCopier.copy(includeFilters);
return this;
}
@Override
@SafeVarargs
public final Builder includeFilters(MetricStreamFilter... includeFilters) {
includeFilters(Arrays.asList(includeFilters));
return this;
}
@Override
@SafeVarargs
public final Builder includeFilters(Consumer... includeFilters) {
includeFilters(Stream.of(includeFilters).map(c -> MetricStreamFilter.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final List getExcludeFilters() {
List result = MetricStreamFiltersCopier.copyToBuilder(this.excludeFilters);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setExcludeFilters(Collection excludeFilters) {
this.excludeFilters = MetricStreamFiltersCopier.copyFromBuilder(excludeFilters);
}
@Override
public final Builder excludeFilters(Collection excludeFilters) {
this.excludeFilters = MetricStreamFiltersCopier.copy(excludeFilters);
return this;
}
@Override
@SafeVarargs
public final Builder excludeFilters(MetricStreamFilter... excludeFilters) {
excludeFilters(Arrays.asList(excludeFilters));
return this;
}
@Override
@SafeVarargs
public final Builder excludeFilters(Consumer... excludeFilters) {
excludeFilters(Stream.of(excludeFilters).map(c -> MetricStreamFilter.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final String getFirehoseArn() {
return firehoseArn;
}
public final void setFirehoseArn(String firehoseArn) {
this.firehoseArn = firehoseArn;
}
@Override
public final Builder firehoseArn(String firehoseArn) {
this.firehoseArn = firehoseArn;
return this;
}
public final String getRoleArn() {
return roleArn;
}
public final void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
@Override
public final Builder roleArn(String roleArn) {
this.roleArn = roleArn;
return this;
}
public final String getOutputFormat() {
return outputFormat;
}
public final void setOutputFormat(String outputFormat) {
this.outputFormat = outputFormat;
}
@Override
public final Builder outputFormat(String outputFormat) {
this.outputFormat = outputFormat;
return this;
}
@Override
public final Builder outputFormat(MetricStreamOutputFormat outputFormat) {
this.outputFormat(outputFormat == null ? null : outputFormat.toString());
return this;
}
public final List getTags() {
List result = TagListCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final List getStatisticsConfigurations() {
List result = MetricStreamStatisticsConfigurationsCopier
.copyToBuilder(this.statisticsConfigurations);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setStatisticsConfigurations(
Collection statisticsConfigurations) {
this.statisticsConfigurations = MetricStreamStatisticsConfigurationsCopier.copyFromBuilder(statisticsConfigurations);
}
@Override
public final Builder statisticsConfigurations(Collection statisticsConfigurations) {
this.statisticsConfigurations = MetricStreamStatisticsConfigurationsCopier.copy(statisticsConfigurations);
return this;
}
@Override
@SafeVarargs
public final Builder statisticsConfigurations(MetricStreamStatisticsConfiguration... statisticsConfigurations) {
statisticsConfigurations(Arrays.asList(statisticsConfigurations));
return this;
}
@Override
@SafeVarargs
public final Builder statisticsConfigurations(
Consumer... statisticsConfigurations) {
statisticsConfigurations(Stream.of(statisticsConfigurations)
.map(c -> MetricStreamStatisticsConfiguration.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final Boolean getIncludeLinkedAccountsMetrics() {
return includeLinkedAccountsMetrics;
}
public final void setIncludeLinkedAccountsMetrics(Boolean includeLinkedAccountsMetrics) {
this.includeLinkedAccountsMetrics = includeLinkedAccountsMetrics;
}
@Override
public final Builder includeLinkedAccountsMetrics(Boolean includeLinkedAccountsMetrics) {
this.includeLinkedAccountsMetrics = includeLinkedAccountsMetrics;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public PutMetricStreamRequest build() {
return new PutMetricStreamRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}