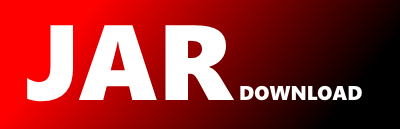
software.amazon.awssdk.services.cloudwatch.model.PutCompositeAlarmRequest Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutCompositeAlarmRequest extends CloudWatchRequest implements
ToCopyableBuilder {
private static final SdkField ACTIONS_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ActionsEnabled").getter(getter(PutCompositeAlarmRequest::actionsEnabled))
.setter(setter(Builder::actionsEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsEnabled").build()).build();
private static final SdkField> ALARM_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AlarmActions")
.getter(getter(PutCompositeAlarmRequest::alarmActions))
.setter(setter(Builder::alarmActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ALARM_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmDescription").getter(getter(PutCompositeAlarmRequest::alarmDescription))
.setter(setter(Builder::alarmDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmDescription").build()).build();
private static final SdkField ALARM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmName").getter(getter(PutCompositeAlarmRequest::alarmName)).setter(setter(Builder::alarmName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmName").build()).build();
private static final SdkField ALARM_RULE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AlarmRule").getter(getter(PutCompositeAlarmRequest::alarmRule)).setter(setter(Builder::alarmRule))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmRule").build()).build();
private static final SdkField> INSUFFICIENT_DATA_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InsufficientDataActions")
.getter(getter(PutCompositeAlarmRequest::insufficientDataActions))
.setter(setter(Builder::insufficientDataActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InsufficientDataActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OK_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OKActions")
.getter(getter(PutCompositeAlarmRequest::okActions))
.setter(setter(Builder::okActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OKActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(PutCompositeAlarmRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ACTIONS_SUPPRESSOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActionsSuppressor").getter(getter(PutCompositeAlarmRequest::actionsSuppressor))
.setter(setter(Builder::actionsSuppressor))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressor").build()).build();
private static final SdkField ACTIONS_SUPPRESSOR_WAIT_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ActionsSuppressorWaitPeriod")
.getter(getter(PutCompositeAlarmRequest::actionsSuppressorWaitPeriod))
.setter(setter(Builder::actionsSuppressorWaitPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressorWaitPeriod")
.build()).build();
private static final SdkField ACTIONS_SUPPRESSOR_EXTENSION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ActionsSuppressorExtensionPeriod")
.getter(getter(PutCompositeAlarmRequest::actionsSuppressorExtensionPeriod))
.setter(setter(Builder::actionsSuppressorExtensionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsSuppressorExtensionPeriod")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACTIONS_ENABLED_FIELD,
ALARM_ACTIONS_FIELD, ALARM_DESCRIPTION_FIELD, ALARM_NAME_FIELD, ALARM_RULE_FIELD, INSUFFICIENT_DATA_ACTIONS_FIELD,
OK_ACTIONS_FIELD, TAGS_FIELD, ACTIONS_SUPPRESSOR_FIELD, ACTIONS_SUPPRESSOR_WAIT_PERIOD_FIELD,
ACTIONS_SUPPRESSOR_EXTENSION_PERIOD_FIELD));
private final Boolean actionsEnabled;
private final List alarmActions;
private final String alarmDescription;
private final String alarmName;
private final String alarmRule;
private final List insufficientDataActions;
private final List okActions;
private final List tags;
private final String actionsSuppressor;
private final Integer actionsSuppressorWaitPeriod;
private final Integer actionsSuppressorExtensionPeriod;
private PutCompositeAlarmRequest(BuilderImpl builder) {
super(builder);
this.actionsEnabled = builder.actionsEnabled;
this.alarmActions = builder.alarmActions;
this.alarmDescription = builder.alarmDescription;
this.alarmName = builder.alarmName;
this.alarmRule = builder.alarmRule;
this.insufficientDataActions = builder.insufficientDataActions;
this.okActions = builder.okActions;
this.tags = builder.tags;
this.actionsSuppressor = builder.actionsSuppressor;
this.actionsSuppressorWaitPeriod = builder.actionsSuppressorWaitPeriod;
this.actionsSuppressorExtensionPeriod = builder.actionsSuppressorExtensionPeriod;
}
/**
*
* Indicates whether actions should be executed during any changes to the alarm state of the composite alarm. The
* default is TRUE
.
*
*
* @return Indicates whether actions should be executed during any changes to the alarm state of the composite
* alarm. The default is TRUE
.
*/
public final Boolean actionsEnabled() {
return actionsEnabled;
}
/**
* For responses, this returns true if the service returned a value for the AlarmActions property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAlarmActions() {
return alarmActions != null && !(alarmActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to the ALARM
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: ]
*
*
* Amazon SNS actions:
*
*
* arn:aws:sns:region:account-id:sns-topic-name
*
*
* Lambda actions:
*
*
* -
*
* Invoke the latest version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name
*
*
* -
*
* Invoke a specific version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:version-number
*
*
* -
*
* Invoke a function by using an alias Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:alias-name
*
*
*
*
* Systems Manager actions:
*
*
* arn:aws:ssm:region:account-id:opsitem:severity
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAlarmActions} method.
*
*
* @return The actions to execute when this alarm transitions to the ALARM
state from any other state.
* Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: ]
*
*
* Amazon SNS actions:
*
*
* arn:aws:sns:region:account-id:sns-topic-name
*
*
* Lambda actions:
*
*
* -
*
* Invoke the latest version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name
*
*
* -
*
* Invoke a specific version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:version-number
*
*
* -
*
* Invoke a function by using an alias Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:alias-name
*
*
*
*
* Systems Manager actions:
*
*
* arn:aws:ssm:region:account-id:opsitem:severity
*/
public final List alarmActions() {
return alarmActions;
}
/**
*
* The description for the composite alarm.
*
*
* @return The description for the composite alarm.
*/
public final String alarmDescription() {
return alarmDescription;
}
/**
*
* The name for the composite alarm. This name must be unique within the Region.
*
*
* @return The name for the composite alarm. This name must be unique within the Region.
*/
public final String alarmName() {
return alarmName;
}
/**
*
* An expression that specifies which other alarms are to be evaluated to determine this composite alarm's state.
* For each alarm that you reference, you designate a function that specifies whether that alarm needs to be in
* ALARM state, OK state, or INSUFFICIENT_DATA state. You can use operators (AND, OR and NOT) to combine multiple
* functions in a single expression. You can use parenthesis to logically group the functions in your expression.
*
*
* You can use either alarm names or ARNs to reference the other alarms that are to be evaluated.
*
*
* Functions can include the following:
*
*
* -
*
* ALARM("alarm-name or alarm-ARN")
is TRUE if the named alarm is in ALARM state.
*
*
* -
*
* OK("alarm-name or alarm-ARN")
is TRUE if the named alarm is in OK state.
*
*
* -
*
* INSUFFICIENT_DATA("alarm-name or alarm-ARN")
is TRUE if the named alarm is in
* INSUFFICIENT_DATA state.
*
*
* -
*
* TRUE
always evaluates to TRUE.
*
*
* -
*
* FALSE
always evaluates to FALSE.
*
*
*
*
* TRUE and FALSE are useful for testing a complex AlarmRule
structure, and for testing your alarm
* actions.
*
*
* Alarm names specified in AlarmRule
can be surrounded with double-quotes ("), but do not have to be.
*
*
* The following are some examples of AlarmRule
:
*
*
* -
*
* ALARM(CPUUtilizationTooHigh) AND ALARM(DiskReadOpsTooHigh)
specifies that the composite alarm goes
* into ALARM state only if both CPUUtilizationTooHigh and DiskReadOpsTooHigh alarms are in ALARM state.
*
*
* -
*
* ALARM(CPUUtilizationTooHigh) AND NOT ALARM(DeploymentInProgress)
specifies that the alarm goes to
* ALARM state if CPUUtilizationTooHigh is in ALARM state and DeploymentInProgress is not in ALARM state. This
* example reduces alarm noise during a known deployment window.
*
*
* -
*
* (ALARM(CPUUtilizationTooHigh) OR ALARM(DiskReadOpsTooHigh)) AND OK(NetworkOutTooHigh)
goes into
* ALARM state if CPUUtilizationTooHigh OR DiskReadOpsTooHigh is in ALARM state, and if NetworkOutTooHigh is in OK
* state. This provides another example of using a composite alarm to prevent noise. This rule ensures that you are
* not notified with an alarm action on high CPU or disk usage if a known network problem is also occurring.
*
*
*
*
* The AlarmRule
can specify as many as 100 "children" alarms. The AlarmRule
expression
* can have as many as 500 elements. Elements are child alarms, TRUE or FALSE statements, and parentheses.
*
*
* @return An expression that specifies which other alarms are to be evaluated to determine this composite alarm's
* state. For each alarm that you reference, you designate a function that specifies whether that alarm
* needs to be in ALARM state, OK state, or INSUFFICIENT_DATA state. You can use operators (AND, OR and NOT)
* to combine multiple functions in a single expression. You can use parenthesis to logically group the
* functions in your expression.
*
* You can use either alarm names or ARNs to reference the other alarms that are to be evaluated.
*
*
* Functions can include the following:
*
*
* -
*
* ALARM("alarm-name or alarm-ARN")
is TRUE if the named alarm is in ALARM state.
*
*
* -
*
* OK("alarm-name or alarm-ARN")
is TRUE if the named alarm is in OK state.
*
*
* -
*
* INSUFFICIENT_DATA("alarm-name or alarm-ARN")
is TRUE if the named alarm is in
* INSUFFICIENT_DATA state.
*
*
* -
*
* TRUE
always evaluates to TRUE.
*
*
* -
*
* FALSE
always evaluates to FALSE.
*
*
*
*
* TRUE and FALSE are useful for testing a complex AlarmRule
structure, and for testing your
* alarm actions.
*
*
* Alarm names specified in AlarmRule
can be surrounded with double-quotes ("), but do not have
* to be.
*
*
* The following are some examples of AlarmRule
:
*
*
* -
*
* ALARM(CPUUtilizationTooHigh) AND ALARM(DiskReadOpsTooHigh)
specifies that the composite
* alarm goes into ALARM state only if both CPUUtilizationTooHigh and DiskReadOpsTooHigh alarms are in ALARM
* state.
*
*
* -
*
* ALARM(CPUUtilizationTooHigh) AND NOT ALARM(DeploymentInProgress)
specifies that the alarm
* goes to ALARM state if CPUUtilizationTooHigh is in ALARM state and DeploymentInProgress is not in ALARM
* state. This example reduces alarm noise during a known deployment window.
*
*
* -
*
* (ALARM(CPUUtilizationTooHigh) OR ALARM(DiskReadOpsTooHigh)) AND OK(NetworkOutTooHigh)
goes
* into ALARM state if CPUUtilizationTooHigh OR DiskReadOpsTooHigh is in ALARM state, and if
* NetworkOutTooHigh is in OK state. This provides another example of using a composite alarm to prevent
* noise. This rule ensures that you are not notified with an alarm action on high CPU or disk usage if a
* known network problem is also occurring.
*
*
*
*
* The AlarmRule
can specify as many as 100 "children" alarms. The AlarmRule
* expression can have as many as 500 elements. Elements are child alarms, TRUE or FALSE statements, and
* parentheses.
*/
public final String alarmRule() {
return alarmRule;
}
/**
* For responses, this returns true if the service returned a value for the InsufficientDataActions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasInsufficientDataActions() {
return insufficientDataActions != null && !(insufficientDataActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any other
* state. Each action is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: ]
*
*
* Amazon SNS actions:
*
*
* arn:aws:sns:region:account-id:sns-topic-name
*
*
* Lambda actions:
*
*
* -
*
* Invoke the latest version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name
*
*
* -
*
* Invoke a specific version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:version-number
*
*
* -
*
* Invoke a function by using an alias Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:alias-name
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInsufficientDataActions} method.
*
*
* @return The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any
* other state. Each action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: ]
*
*
* Amazon SNS actions:
*
*
* arn:aws:sns:region:account-id:sns-topic-name
*
*
* Lambda actions:
*
*
* -
*
* Invoke the latest version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name
*
*
* -
*
* Invoke a specific version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:version-number
*
*
* -
*
* Invoke a function by using an alias Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:alias-name
*
*
*/
public final List insufficientDataActions() {
return insufficientDataActions;
}
/**
* For responses, this returns true if the service returned a value for the OKActions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasOkActions() {
return okActions != null && !(okActions instanceof SdkAutoConstructList);
}
/**
*
* The actions to execute when this alarm transitions to an OK
state from any other state. Each action
* is specified as an Amazon Resource Name (ARN).
*
*
* Valid Values: ]
*
*
* Amazon SNS actions:
*
*
* arn:aws:sns:region:account-id:sns-topic-name
*
*
* Lambda actions:
*
*
* -
*
* Invoke the latest version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name
*
*
* -
*
* Invoke a specific version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:version-number
*
*
* -
*
* Invoke a function by using an alias Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:alias-name
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOkActions} method.
*
*
* @return The actions to execute when this alarm transitions to an OK
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
* Valid Values: ]
*
*
* Amazon SNS actions:
*
*
* arn:aws:sns:region:account-id:sns-topic-name
*
*
* Lambda actions:
*
*
* -
*
* Invoke the latest version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name
*
*
* -
*
* Invoke a specific version of a Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:version-number
*
*
* -
*
* Invoke a function by using an alias Lambda function:
* arn:aws:lambda:region:account-id:function:function-name:alias-name
*
*
*/
public final List okActions() {
return okActions;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an alarm. To be
* able to associate tags with the alarm when you create the alarm, you must have the
* cloudwatch:TagResource
permission.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter are ignored.
* To change the tags of an existing alarm, use TagResource or
* UntagResource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of key-value pairs to associate with the alarm. You can associate as many as 50 tags with an
* alarm. To be able to associate tags with the alarm when you create the alarm, you must have the
* cloudwatch:TagResource
permission.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions
* by granting a user permission to access or change only resources with certain tag values.
*
*
* If you are using this operation to update an existing alarm, any tags you specify in this parameter are
* ignored. To change the tags of an existing alarm, use TagResource or UntagResource.
*/
public final List tags() {
return tags;
}
/**
*
* Actions will be suppressed if the suppressor alarm is in the ALARM
state.
* ActionsSuppressor
can be an AlarmName or an Amazon Resource Name (ARN) from an existing alarm.
*
*
* @return Actions will be suppressed if the suppressor alarm is in the ALARM
state.
* ActionsSuppressor
can be an AlarmName or an Amazon Resource Name (ARN) from an existing
* alarm.
*/
public final String actionsSuppressor() {
return actionsSuppressor;
}
/**
*
* The maximum time in seconds that the composite alarm waits for the suppressor alarm to go into the
* ALARM
state. After this time, the composite alarm performs its actions.
*
*
*
* WaitPeriod
is required only when ActionsSuppressor
is specified.
*
*
*
* @return The maximum time in seconds that the composite alarm waits for the suppressor alarm to go into the
* ALARM
state. After this time, the composite alarm performs its actions.
*
* WaitPeriod
is required only when ActionsSuppressor
is specified.
*
*/
public final Integer actionsSuppressorWaitPeriod() {
return actionsSuppressorWaitPeriod;
}
/**
*
* The maximum time in seconds that the composite alarm waits after suppressor alarm goes out of the
* ALARM
state. After this time, the composite alarm performs its actions.
*
*
*
* ExtensionPeriod
is required only when ActionsSuppressor
is specified.
*
*
*
* @return The maximum time in seconds that the composite alarm waits after suppressor alarm goes out of the
* ALARM
state. After this time, the composite alarm performs its actions.
*
* ExtensionPeriod
is required only when ActionsSuppressor
is specified.
*
*/
public final Integer actionsSuppressorExtensionPeriod() {
return actionsSuppressorExtensionPeriod;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(actionsEnabled());
hashCode = 31 * hashCode + Objects.hashCode(hasAlarmActions() ? alarmActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(alarmDescription());
hashCode = 31 * hashCode + Objects.hashCode(alarmName());
hashCode = 31 * hashCode + Objects.hashCode(alarmRule());
hashCode = 31 * hashCode + Objects.hashCode(hasInsufficientDataActions() ? insufficientDataActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasOkActions() ? okActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressor());
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressorWaitPeriod());
hashCode = 31 * hashCode + Objects.hashCode(actionsSuppressorExtensionPeriod());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutCompositeAlarmRequest)) {
return false;
}
PutCompositeAlarmRequest other = (PutCompositeAlarmRequest) obj;
return Objects.equals(actionsEnabled(), other.actionsEnabled()) && hasAlarmActions() == other.hasAlarmActions()
&& Objects.equals(alarmActions(), other.alarmActions())
&& Objects.equals(alarmDescription(), other.alarmDescription()) && Objects.equals(alarmName(), other.alarmName())
&& Objects.equals(alarmRule(), other.alarmRule())
&& hasInsufficientDataActions() == other.hasInsufficientDataActions()
&& Objects.equals(insufficientDataActions(), other.insufficientDataActions())
&& hasOkActions() == other.hasOkActions() && Objects.equals(okActions(), other.okActions())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(actionsSuppressor(), other.actionsSuppressor())
&& Objects.equals(actionsSuppressorWaitPeriod(), other.actionsSuppressorWaitPeriod())
&& Objects.equals(actionsSuppressorExtensionPeriod(), other.actionsSuppressorExtensionPeriod());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PutCompositeAlarmRequest").add("ActionsEnabled", actionsEnabled())
.add("AlarmActions", hasAlarmActions() ? alarmActions() : null).add("AlarmDescription", alarmDescription())
.add("AlarmName", alarmName()).add("AlarmRule", alarmRule())
.add("InsufficientDataActions", hasInsufficientDataActions() ? insufficientDataActions() : null)
.add("OKActions", hasOkActions() ? okActions() : null).add("Tags", hasTags() ? tags() : null)
.add("ActionsSuppressor", actionsSuppressor()).add("ActionsSuppressorWaitPeriod", actionsSuppressorWaitPeriod())
.add("ActionsSuppressorExtensionPeriod", actionsSuppressorExtensionPeriod()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ActionsEnabled":
return Optional.ofNullable(clazz.cast(actionsEnabled()));
case "AlarmActions":
return Optional.ofNullable(clazz.cast(alarmActions()));
case "AlarmDescription":
return Optional.ofNullable(clazz.cast(alarmDescription()));
case "AlarmName":
return Optional.ofNullable(clazz.cast(alarmName()));
case "AlarmRule":
return Optional.ofNullable(clazz.cast(alarmRule()));
case "InsufficientDataActions":
return Optional.ofNullable(clazz.cast(insufficientDataActions()));
case "OKActions":
return Optional.ofNullable(clazz.cast(okActions()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ActionsSuppressor":
return Optional.ofNullable(clazz.cast(actionsSuppressor()));
case "ActionsSuppressorWaitPeriod":
return Optional.ofNullable(clazz.cast(actionsSuppressorWaitPeriod()));
case "ActionsSuppressorExtensionPeriod":
return Optional.ofNullable(clazz.cast(actionsSuppressorExtensionPeriod()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function