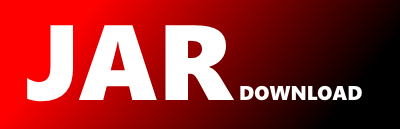
software.amazon.awssdk.services.cloudwatch.model.MetricAlarm Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatch.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents an alarm.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MetricAlarm implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ALARM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::alarmName)).setter(setter(Builder::alarmName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmName").build()).build();
private static final SdkField ALARM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::alarmArn)).setter(setter(Builder::alarmArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmArn").build()).build();
private static final SdkField ALARM_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::alarmDescription)).setter(setter(Builder::alarmDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmDescription").build()).build();
private static final SdkField ALARM_CONFIGURATION_UPDATED_TIMESTAMP_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.getter(getter(MetricAlarm::alarmConfigurationUpdatedTimestamp))
.setter(setter(Builder::alarmConfigurationUpdatedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmConfigurationUpdatedTimestamp")
.build()).build();
private static final SdkField ACTIONS_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(MetricAlarm::actionsEnabled)).setter(setter(Builder::actionsEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsEnabled").build()).build();
private static final SdkField> OK_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(MetricAlarm::okActions))
.setter(setter(Builder::okActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OKActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ALARM_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(MetricAlarm::alarmActions))
.setter(setter(Builder::alarmActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlarmActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> INSUFFICIENT_DATA_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(MetricAlarm::insufficientDataActions))
.setter(setter(Builder::insufficientDataActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InsufficientDataActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATE_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::stateValueAsString)).setter(setter(Builder::stateValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateValue").build()).build();
private static final SdkField STATE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::stateReason)).setter(setter(Builder::stateReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateReason").build()).build();
private static final SdkField STATE_REASON_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::stateReasonData)).setter(setter(Builder::stateReasonData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateReasonData").build()).build();
private static final SdkField STATE_UPDATED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(MetricAlarm::stateUpdatedTimestamp)).setter(setter(Builder::stateUpdatedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateUpdatedTimestamp").build())
.build();
private static final SdkField METRIC_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::metricName)).setter(setter(Builder::metricName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricName").build()).build();
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Namespace").build()).build();
private static final SdkField STATISTIC_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::statisticAsString)).setter(setter(Builder::statistic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Statistic").build()).build();
private static final SdkField EXTENDED_STATISTIC_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::extendedStatistic)).setter(setter(Builder::extendedStatistic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExtendedStatistic").build()).build();
private static final SdkField> DIMENSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(MetricAlarm::dimensions))
.setter(setter(Builder::dimensions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Dimensions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Dimension::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(MetricAlarm::period)).setter(setter(Builder::period))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Period").build()).build();
private static final SdkField UNIT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::unitAsString)).setter(setter(Builder::unit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Unit").build()).build();
private static final SdkField EVALUATION_PERIODS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(MetricAlarm::evaluationPeriods)).setter(setter(Builder::evaluationPeriods))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluationPeriods").build()).build();
private static final SdkField DATAPOINTS_TO_ALARM_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(MetricAlarm::datapointsToAlarm)).setter(setter(Builder::datapointsToAlarm))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatapointsToAlarm").build()).build();
private static final SdkField THRESHOLD_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.getter(getter(MetricAlarm::threshold)).setter(setter(Builder::threshold))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Threshold").build()).build();
private static final SdkField COMPARISON_OPERATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::comparisonOperatorAsString)).setter(setter(Builder::comparisonOperator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComparisonOperator").build())
.build();
private static final SdkField TREAT_MISSING_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::treatMissingData)).setter(setter(Builder::treatMissingData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TreatMissingData").build()).build();
private static final SdkField EVALUATE_LOW_SAMPLE_COUNT_PERCENTILE_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(MetricAlarm::evaluateLowSampleCountPercentile))
.setter(setter(Builder::evaluateLowSampleCountPercentile))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluateLowSampleCountPercentile")
.build()).build();
private static final SdkField> METRICS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(MetricAlarm::metrics))
.setter(setter(Builder::metrics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Metrics").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricDataQuery::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ALARM_NAME_FIELD,
ALARM_ARN_FIELD, ALARM_DESCRIPTION_FIELD, ALARM_CONFIGURATION_UPDATED_TIMESTAMP_FIELD, ACTIONS_ENABLED_FIELD,
OK_ACTIONS_FIELD, ALARM_ACTIONS_FIELD, INSUFFICIENT_DATA_ACTIONS_FIELD, STATE_VALUE_FIELD, STATE_REASON_FIELD,
STATE_REASON_DATA_FIELD, STATE_UPDATED_TIMESTAMP_FIELD, METRIC_NAME_FIELD, NAMESPACE_FIELD, STATISTIC_FIELD,
EXTENDED_STATISTIC_FIELD, DIMENSIONS_FIELD, PERIOD_FIELD, UNIT_FIELD, EVALUATION_PERIODS_FIELD,
DATAPOINTS_TO_ALARM_FIELD, THRESHOLD_FIELD, COMPARISON_OPERATOR_FIELD, TREAT_MISSING_DATA_FIELD,
EVALUATE_LOW_SAMPLE_COUNT_PERCENTILE_FIELD, METRICS_FIELD));
private static final long serialVersionUID = 1L;
private final String alarmName;
private final String alarmArn;
private final String alarmDescription;
private final Instant alarmConfigurationUpdatedTimestamp;
private final Boolean actionsEnabled;
private final List okActions;
private final List alarmActions;
private final List insufficientDataActions;
private final String stateValue;
private final String stateReason;
private final String stateReasonData;
private final Instant stateUpdatedTimestamp;
private final String metricName;
private final String namespace;
private final String statistic;
private final String extendedStatistic;
private final List dimensions;
private final Integer period;
private final String unit;
private final Integer evaluationPeriods;
private final Integer datapointsToAlarm;
private final Double threshold;
private final String comparisonOperator;
private final String treatMissingData;
private final String evaluateLowSampleCountPercentile;
private final List metrics;
private MetricAlarm(BuilderImpl builder) {
this.alarmName = builder.alarmName;
this.alarmArn = builder.alarmArn;
this.alarmDescription = builder.alarmDescription;
this.alarmConfigurationUpdatedTimestamp = builder.alarmConfigurationUpdatedTimestamp;
this.actionsEnabled = builder.actionsEnabled;
this.okActions = builder.okActions;
this.alarmActions = builder.alarmActions;
this.insufficientDataActions = builder.insufficientDataActions;
this.stateValue = builder.stateValue;
this.stateReason = builder.stateReason;
this.stateReasonData = builder.stateReasonData;
this.stateUpdatedTimestamp = builder.stateUpdatedTimestamp;
this.metricName = builder.metricName;
this.namespace = builder.namespace;
this.statistic = builder.statistic;
this.extendedStatistic = builder.extendedStatistic;
this.dimensions = builder.dimensions;
this.period = builder.period;
this.unit = builder.unit;
this.evaluationPeriods = builder.evaluationPeriods;
this.datapointsToAlarm = builder.datapointsToAlarm;
this.threshold = builder.threshold;
this.comparisonOperator = builder.comparisonOperator;
this.treatMissingData = builder.treatMissingData;
this.evaluateLowSampleCountPercentile = builder.evaluateLowSampleCountPercentile;
this.metrics = builder.metrics;
}
/**
*
* The name of the alarm.
*
*
* @return The name of the alarm.
*/
public String alarmName() {
return alarmName;
}
/**
*
* The Amazon Resource Name (ARN) of the alarm.
*
*
* @return The Amazon Resource Name (ARN) of the alarm.
*/
public String alarmArn() {
return alarmArn;
}
/**
*
* The description of the alarm.
*
*
* @return The description of the alarm.
*/
public String alarmDescription() {
return alarmDescription;
}
/**
*
* The time stamp of the last update to the alarm configuration.
*
*
* @return The time stamp of the last update to the alarm configuration.
*/
public Instant alarmConfigurationUpdatedTimestamp() {
return alarmConfigurationUpdatedTimestamp;
}
/**
*
* Indicates whether actions should be executed during any changes to the alarm state.
*
*
* @return Indicates whether actions should be executed during any changes to the alarm state.
*/
public Boolean actionsEnabled() {
return actionsEnabled;
}
/**
*
* The actions to execute when this alarm transitions to the OK
state from any other state. Each action
* is specified as an Amazon Resource Name (ARN).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The actions to execute when this alarm transitions to the OK
state from any other state.
* Each action is specified as an Amazon Resource Name (ARN).
*/
public List okActions() {
return okActions;
}
/**
*
* The actions to execute when this alarm transitions to the ALARM
state from any other state. Each
* action is specified as an Amazon Resource Name (ARN).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The actions to execute when this alarm transitions to the ALARM
state from any other state.
* Each action is specified as an Amazon Resource Name (ARN).
*/
public List alarmActions() {
return alarmActions;
}
/**
*
* The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any other
* state. Each action is specified as an Amazon Resource Name (ARN).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The actions to execute when this alarm transitions to the INSUFFICIENT_DATA
state from any
* other state. Each action is specified as an Amazon Resource Name (ARN).
*/
public List insufficientDataActions() {
return insufficientDataActions;
}
/**
*
* The state value for the alarm.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stateValue} will
* return {@link StateValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateValueAsString}.
*
*
* @return The state value for the alarm.
* @see StateValue
*/
public StateValue stateValue() {
return StateValue.fromValue(stateValue);
}
/**
*
* The state value for the alarm.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stateValue} will
* return {@link StateValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateValueAsString}.
*
*
* @return The state value for the alarm.
* @see StateValue
*/
public String stateValueAsString() {
return stateValue;
}
/**
*
* An explanation for the alarm state, in text format.
*
*
* @return An explanation for the alarm state, in text format.
*/
public String stateReason() {
return stateReason;
}
/**
*
* An explanation for the alarm state, in JSON format.
*
*
* @return An explanation for the alarm state, in JSON format.
*/
public String stateReasonData() {
return stateReasonData;
}
/**
*
* The time stamp of the last update to the alarm state.
*
*
* @return The time stamp of the last update to the alarm state.
*/
public Instant stateUpdatedTimestamp() {
return stateUpdatedTimestamp;
}
/**
*
* The name of the metric associated with the alarm.
*
*
* @return The name of the metric associated with the alarm.
*/
public String metricName() {
return metricName;
}
/**
*
* The namespace of the metric associated with the alarm.
*
*
* @return The namespace of the metric associated with the alarm.
*/
public String namespace() {
return namespace;
}
/**
*
* The statistic for the metric associated with the alarm, other than percentile. For percentile statistics, use
* ExtendedStatistic
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statistic} will
* return {@link Statistic#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statisticAsString}.
*
*
* @return The statistic for the metric associated with the alarm, other than percentile. For percentile statistics,
* use ExtendedStatistic
.
* @see Statistic
*/
public Statistic statistic() {
return Statistic.fromValue(statistic);
}
/**
*
* The statistic for the metric associated with the alarm, other than percentile. For percentile statistics, use
* ExtendedStatistic
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statistic} will
* return {@link Statistic#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statisticAsString}.
*
*
* @return The statistic for the metric associated with the alarm, other than percentile. For percentile statistics,
* use ExtendedStatistic
.
* @see Statistic
*/
public String statisticAsString() {
return statistic;
}
/**
*
* The percentile statistic for the metric associated with the alarm. Specify a value between p0.0 and p100.
*
*
* @return The percentile statistic for the metric associated with the alarm. Specify a value between p0.0 and p100.
*/
public String extendedStatistic() {
return extendedStatistic;
}
/**
*
* The dimensions for the metric associated with the alarm.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The dimensions for the metric associated with the alarm.
*/
public List dimensions() {
return dimensions;
}
/**
*
* The period, in seconds, over which the statistic is applied.
*
*
* @return The period, in seconds, over which the statistic is applied.
*/
public Integer period() {
return period;
}
/**
*
* The unit of the metric associated with the alarm.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link StandardUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return The unit of the metric associated with the alarm.
* @see StandardUnit
*/
public StandardUnit unit() {
return StandardUnit.fromValue(unit);
}
/**
*
* The unit of the metric associated with the alarm.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link StandardUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return The unit of the metric associated with the alarm.
* @see StandardUnit
*/
public String unitAsString() {
return unit;
}
/**
*
* The number of periods over which data is compared to the specified threshold.
*
*
* @return The number of periods over which data is compared to the specified threshold.
*/
public Integer evaluationPeriods() {
return evaluationPeriods;
}
/**
*
* The number of datapoints that must be breaching to trigger the alarm.
*
*
* @return The number of datapoints that must be breaching to trigger the alarm.
*/
public Integer datapointsToAlarm() {
return datapointsToAlarm;
}
/**
*
* The value to compare with the specified statistic.
*
*
* @return The value to compare with the specified statistic.
*/
public Double threshold() {
return threshold;
}
/**
*
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified statistic
* value is used as the first operand.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return The arithmetic operation to use when comparing the specified statistic and threshold. The specified
* statistic value is used as the first operand.
* @see ComparisonOperator
*/
public ComparisonOperator comparisonOperator() {
return ComparisonOperator.fromValue(comparisonOperator);
}
/**
*
* The arithmetic operation to use when comparing the specified statistic and threshold. The specified statistic
* value is used as the first operand.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return The arithmetic operation to use when comparing the specified statistic and threshold. The specified
* statistic value is used as the first operand.
* @see ComparisonOperator
*/
public String comparisonOperatorAsString() {
return comparisonOperator;
}
/**
*
* Sets how this alarm is to handle missing data points. If this parameter is omitted, the default behavior of
* missing
is used.
*
*
* @return Sets how this alarm is to handle missing data points. If this parameter is omitted, the default behavior
* of missing
is used.
*/
public String treatMissingData() {
return treatMissingData;
}
/**
*
* Used only for alarms based on percentiles. If ignore
, the alarm state does not change during periods
* with too few data points to be statistically significant. If evaluate
or this parameter is not used,
* the alarm is always evaluated and possibly changes state no matter how many data points are available.
*
*
* @return Used only for alarms based on percentiles. If ignore
, the alarm state does not change during
* periods with too few data points to be statistically significant. If evaluate
or this
* parameter is not used, the alarm is always evaluated and possibly changes state no matter how many data
* points are available.
*/
public String evaluateLowSampleCountPercentile() {
return evaluateLowSampleCountPercentile;
}
/**
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return
*/
public List metrics() {
return metrics;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(alarmName());
hashCode = 31 * hashCode + Objects.hashCode(alarmArn());
hashCode = 31 * hashCode + Objects.hashCode(alarmDescription());
hashCode = 31 * hashCode + Objects.hashCode(alarmConfigurationUpdatedTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(actionsEnabled());
hashCode = 31 * hashCode + Objects.hashCode(okActions());
hashCode = 31 * hashCode + Objects.hashCode(alarmActions());
hashCode = 31 * hashCode + Objects.hashCode(insufficientDataActions());
hashCode = 31 * hashCode + Objects.hashCode(stateValueAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateReason());
hashCode = 31 * hashCode + Objects.hashCode(stateReasonData());
hashCode = 31 * hashCode + Objects.hashCode(stateUpdatedTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(metricName());
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(statisticAsString());
hashCode = 31 * hashCode + Objects.hashCode(extendedStatistic());
hashCode = 31 * hashCode + Objects.hashCode(dimensions());
hashCode = 31 * hashCode + Objects.hashCode(period());
hashCode = 31 * hashCode + Objects.hashCode(unitAsString());
hashCode = 31 * hashCode + Objects.hashCode(evaluationPeriods());
hashCode = 31 * hashCode + Objects.hashCode(datapointsToAlarm());
hashCode = 31 * hashCode + Objects.hashCode(threshold());
hashCode = 31 * hashCode + Objects.hashCode(comparisonOperatorAsString());
hashCode = 31 * hashCode + Objects.hashCode(treatMissingData());
hashCode = 31 * hashCode + Objects.hashCode(evaluateLowSampleCountPercentile());
hashCode = 31 * hashCode + Objects.hashCode(metrics());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MetricAlarm)) {
return false;
}
MetricAlarm other = (MetricAlarm) obj;
return Objects.equals(alarmName(), other.alarmName()) && Objects.equals(alarmArn(), other.alarmArn())
&& Objects.equals(alarmDescription(), other.alarmDescription())
&& Objects.equals(alarmConfigurationUpdatedTimestamp(), other.alarmConfigurationUpdatedTimestamp())
&& Objects.equals(actionsEnabled(), other.actionsEnabled()) && Objects.equals(okActions(), other.okActions())
&& Objects.equals(alarmActions(), other.alarmActions())
&& Objects.equals(insufficientDataActions(), other.insufficientDataActions())
&& Objects.equals(stateValueAsString(), other.stateValueAsString())
&& Objects.equals(stateReason(), other.stateReason())
&& Objects.equals(stateReasonData(), other.stateReasonData())
&& Objects.equals(stateUpdatedTimestamp(), other.stateUpdatedTimestamp())
&& Objects.equals(metricName(), other.metricName()) && Objects.equals(namespace(), other.namespace())
&& Objects.equals(statisticAsString(), other.statisticAsString())
&& Objects.equals(extendedStatistic(), other.extendedStatistic())
&& Objects.equals(dimensions(), other.dimensions()) && Objects.equals(period(), other.period())
&& Objects.equals(unitAsString(), other.unitAsString())
&& Objects.equals(evaluationPeriods(), other.evaluationPeriods())
&& Objects.equals(datapointsToAlarm(), other.datapointsToAlarm())
&& Objects.equals(threshold(), other.threshold())
&& Objects.equals(comparisonOperatorAsString(), other.comparisonOperatorAsString())
&& Objects.equals(treatMissingData(), other.treatMissingData())
&& Objects.equals(evaluateLowSampleCountPercentile(), other.evaluateLowSampleCountPercentile())
&& Objects.equals(metrics(), other.metrics());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("MetricAlarm").add("AlarmName", alarmName()).add("AlarmArn", alarmArn())
.add("AlarmDescription", alarmDescription())
.add("AlarmConfigurationUpdatedTimestamp", alarmConfigurationUpdatedTimestamp())
.add("ActionsEnabled", actionsEnabled()).add("OKActions", okActions()).add("AlarmActions", alarmActions())
.add("InsufficientDataActions", insufficientDataActions()).add("StateValue", stateValueAsString())
.add("StateReason", stateReason()).add("StateReasonData", stateReasonData())
.add("StateUpdatedTimestamp", stateUpdatedTimestamp()).add("MetricName", metricName())
.add("Namespace", namespace()).add("Statistic", statisticAsString())
.add("ExtendedStatistic", extendedStatistic()).add("Dimensions", dimensions()).add("Period", period())
.add("Unit", unitAsString()).add("EvaluationPeriods", evaluationPeriods())
.add("DatapointsToAlarm", datapointsToAlarm()).add("Threshold", threshold())
.add("ComparisonOperator", comparisonOperatorAsString()).add("TreatMissingData", treatMissingData())
.add("EvaluateLowSampleCountPercentile", evaluateLowSampleCountPercentile()).add("Metrics", metrics()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AlarmName":
return Optional.ofNullable(clazz.cast(alarmName()));
case "AlarmArn":
return Optional.ofNullable(clazz.cast(alarmArn()));
case "AlarmDescription":
return Optional.ofNullable(clazz.cast(alarmDescription()));
case "AlarmConfigurationUpdatedTimestamp":
return Optional.ofNullable(clazz.cast(alarmConfigurationUpdatedTimestamp()));
case "ActionsEnabled":
return Optional.ofNullable(clazz.cast(actionsEnabled()));
case "OKActions":
return Optional.ofNullable(clazz.cast(okActions()));
case "AlarmActions":
return Optional.ofNullable(clazz.cast(alarmActions()));
case "InsufficientDataActions":
return Optional.ofNullable(clazz.cast(insufficientDataActions()));
case "StateValue":
return Optional.ofNullable(clazz.cast(stateValueAsString()));
case "StateReason":
return Optional.ofNullable(clazz.cast(stateReason()));
case "StateReasonData":
return Optional.ofNullable(clazz.cast(stateReasonData()));
case "StateUpdatedTimestamp":
return Optional.ofNullable(clazz.cast(stateUpdatedTimestamp()));
case "MetricName":
return Optional.ofNullable(clazz.cast(metricName()));
case "Namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "Statistic":
return Optional.ofNullable(clazz.cast(statisticAsString()));
case "ExtendedStatistic":
return Optional.ofNullable(clazz.cast(extendedStatistic()));
case "Dimensions":
return Optional.ofNullable(clazz.cast(dimensions()));
case "Period":
return Optional.ofNullable(clazz.cast(period()));
case "Unit":
return Optional.ofNullable(clazz.cast(unitAsString()));
case "EvaluationPeriods":
return Optional.ofNullable(clazz.cast(evaluationPeriods()));
case "DatapointsToAlarm":
return Optional.ofNullable(clazz.cast(datapointsToAlarm()));
case "Threshold":
return Optional.ofNullable(clazz.cast(threshold()));
case "ComparisonOperator":
return Optional.ofNullable(clazz.cast(comparisonOperatorAsString()));
case "TreatMissingData":
return Optional.ofNullable(clazz.cast(treatMissingData()));
case "EvaluateLowSampleCountPercentile":
return Optional.ofNullable(clazz.cast(evaluateLowSampleCountPercentile()));
case "Metrics":
return Optional.ofNullable(clazz.cast(metrics()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function