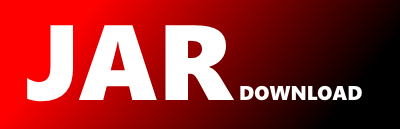
software.amazon.awssdk.services.cloudwatchlogs.model.LogStream Maven / Gradle / Ivy
Show all versions of cloudwatchlogs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatchlogs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a log stream, which is a sequence of log events from a single emitter of logs.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LogStream implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField LOG_STREAM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logStreamName").getter(getter(LogStream::logStreamName)).setter(setter(Builder::logStreamName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logStreamName").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("creationTime").getter(getter(LogStream::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("creationTime").build()).build();
private static final SdkField FIRST_EVENT_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("firstEventTimestamp").getter(getter(LogStream::firstEventTimestamp))
.setter(setter(Builder::firstEventTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("firstEventTimestamp").build())
.build();
private static final SdkField LAST_EVENT_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("lastEventTimestamp").getter(getter(LogStream::lastEventTimestamp))
.setter(setter(Builder::lastEventTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastEventTimestamp").build())
.build();
private static final SdkField LAST_INGESTION_TIME_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("lastIngestionTime").getter(getter(LogStream::lastIngestionTime))
.setter(setter(Builder::lastIngestionTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastIngestionTime").build()).build();
private static final SdkField UPLOAD_SEQUENCE_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("uploadSequenceToken").getter(getter(LogStream::uploadSequenceToken))
.setter(setter(Builder::uploadSequenceToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("uploadSequenceToken").build())
.build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(LogStream::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField STORED_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("storedBytes").getter(getter(LogStream::storedBytes)).setter(setter(Builder::storedBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("storedBytes").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOG_STREAM_NAME_FIELD,
CREATION_TIME_FIELD, FIRST_EVENT_TIMESTAMP_FIELD, LAST_EVENT_TIMESTAMP_FIELD, LAST_INGESTION_TIME_FIELD,
UPLOAD_SEQUENCE_TOKEN_FIELD, ARN_FIELD, STORED_BYTES_FIELD));
private static final long serialVersionUID = 1L;
private final String logStreamName;
private final Long creationTime;
private final Long firstEventTimestamp;
private final Long lastEventTimestamp;
private final Long lastIngestionTime;
private final String uploadSequenceToken;
private final String arn;
private final Long storedBytes;
private LogStream(BuilderImpl builder) {
this.logStreamName = builder.logStreamName;
this.creationTime = builder.creationTime;
this.firstEventTimestamp = builder.firstEventTimestamp;
this.lastEventTimestamp = builder.lastEventTimestamp;
this.lastIngestionTime = builder.lastIngestionTime;
this.uploadSequenceToken = builder.uploadSequenceToken;
this.arn = builder.arn;
this.storedBytes = builder.storedBytes;
}
/**
*
* The name of the log stream.
*
*
* @return The name of the log stream.
*/
public final String logStreamName() {
return logStreamName;
}
/**
*
* The creation time of the stream, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC.
*
*
* @return The creation time of the stream, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC.
*/
public final Long creationTime() {
return creationTime;
}
/**
*
* The time of the first event, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC.
*
*
* @return The time of the first event, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC.
*/
public final Long firstEventTimestamp() {
return firstEventTimestamp;
}
/**
*
* The time of the most recent log event in the log stream in CloudWatch Logs. This number is expressed as the
* number of milliseconds after Jan 1, 1970 00:00:00 UTC. The lastEventTime
value updates on an
* eventual consistency basis. It typically updates in less than an hour from ingestion, but in rare situations
* might take longer.
*
*
* @return The time of the most recent log event in the log stream in CloudWatch Logs. This number is expressed as
* the number of milliseconds after Jan 1, 1970 00:00:00 UTC. The lastEventTime
value updates
* on an eventual consistency basis. It typically updates in less than an hour from ingestion, but in rare
* situations might take longer.
*/
public final Long lastEventTimestamp() {
return lastEventTimestamp;
}
/**
*
* The ingestion time, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC.
*
*
* @return The ingestion time, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC.
*/
public final Long lastIngestionTime() {
return lastIngestionTime;
}
/**
*
* The sequence token.
*
*
* @return The sequence token.
*/
public final String uploadSequenceToken() {
return uploadSequenceToken;
}
/**
*
* The Amazon Resource Name (ARN) of the log stream.
*
*
* @return The Amazon Resource Name (ARN) of the log stream.
*/
public final String arn() {
return arn;
}
/**
*
* The number of bytes stored.
*
*
* Important: On June 17, 2019, this parameter was deprecated for log streams, and is always reported as
* zero. This change applies only to log streams. The storedBytes
parameter for log groups is not
* affected.
*
*
* @return The number of bytes stored.
*
* Important: On June 17, 2019, this parameter was deprecated for log streams, and is always reported
* as zero. This change applies only to log streams. The storedBytes
parameter for log groups
* is not affected.
* @deprecated Starting on June 17, 2019, this parameter will be deprecated for log streams, and will be reported as
* zero. This change applies only to log streams. The storedBytes parameter for log groups is not
* affected.
*/
@Deprecated
public final Long storedBytes() {
return storedBytes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(logStreamName());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(firstEventTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(lastEventTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(lastIngestionTime());
hashCode = 31 * hashCode + Objects.hashCode(uploadSequenceToken());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(storedBytes());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LogStream)) {
return false;
}
LogStream other = (LogStream) obj;
return Objects.equals(logStreamName(), other.logStreamName()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(firstEventTimestamp(), other.firstEventTimestamp())
&& Objects.equals(lastEventTimestamp(), other.lastEventTimestamp())
&& Objects.equals(lastIngestionTime(), other.lastIngestionTime())
&& Objects.equals(uploadSequenceToken(), other.uploadSequenceToken()) && Objects.equals(arn(), other.arn())
&& Objects.equals(storedBytes(), other.storedBytes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LogStream").add("LogStreamName", logStreamName()).add("CreationTime", creationTime())
.add("FirstEventTimestamp", firstEventTimestamp()).add("LastEventTimestamp", lastEventTimestamp())
.add("LastIngestionTime", lastIngestionTime()).add("UploadSequenceToken", uploadSequenceToken())
.add("Arn", arn()).add("StoredBytes", storedBytes()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "logStreamName":
return Optional.ofNullable(clazz.cast(logStreamName()));
case "creationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "firstEventTimestamp":
return Optional.ofNullable(clazz.cast(firstEventTimestamp()));
case "lastEventTimestamp":
return Optional.ofNullable(clazz.cast(lastEventTimestamp()));
case "lastIngestionTime":
return Optional.ofNullable(clazz.cast(lastIngestionTime()));
case "uploadSequenceToken":
return Optional.ofNullable(clazz.cast(uploadSequenceToken()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "storedBytes":
return Optional.ofNullable(clazz.cast(storedBytes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Important: On June 17, 2019, this parameter was deprecated for log streams, and is always
* reported as zero. This change applies only to log streams. The storedBytes
parameter for
* log groups is not affected.
* @return Returns a reference to this object so that method calls can be chained together.
* @deprecated Starting on June 17, 2019, this parameter will be deprecated for log streams, and will be
* reported as zero. This change applies only to log streams. The storedBytes parameter for log
* groups is not affected.
*/
@Deprecated
Builder storedBytes(Long storedBytes);
}
static final class BuilderImpl implements Builder {
private String logStreamName;
private Long creationTime;
private Long firstEventTimestamp;
private Long lastEventTimestamp;
private Long lastIngestionTime;
private String uploadSequenceToken;
private String arn;
private Long storedBytes;
private BuilderImpl() {
}
private BuilderImpl(LogStream model) {
logStreamName(model.logStreamName);
creationTime(model.creationTime);
firstEventTimestamp(model.firstEventTimestamp);
lastEventTimestamp(model.lastEventTimestamp);
lastIngestionTime(model.lastIngestionTime);
uploadSequenceToken(model.uploadSequenceToken);
arn(model.arn);
storedBytes(model.storedBytes);
}
public final String getLogStreamName() {
return logStreamName;
}
public final void setLogStreamName(String logStreamName) {
this.logStreamName = logStreamName;
}
@Override
@Transient
public final Builder logStreamName(String logStreamName) {
this.logStreamName = logStreamName;
return this;
}
public final Long getCreationTime() {
return creationTime;
}
public final void setCreationTime(Long creationTime) {
this.creationTime = creationTime;
}
@Override
@Transient
public final Builder creationTime(Long creationTime) {
this.creationTime = creationTime;
return this;
}
public final Long getFirstEventTimestamp() {
return firstEventTimestamp;
}
public final void setFirstEventTimestamp(Long firstEventTimestamp) {
this.firstEventTimestamp = firstEventTimestamp;
}
@Override
@Transient
public final Builder firstEventTimestamp(Long firstEventTimestamp) {
this.firstEventTimestamp = firstEventTimestamp;
return this;
}
public final Long getLastEventTimestamp() {
return lastEventTimestamp;
}
public final void setLastEventTimestamp(Long lastEventTimestamp) {
this.lastEventTimestamp = lastEventTimestamp;
}
@Override
@Transient
public final Builder lastEventTimestamp(Long lastEventTimestamp) {
this.lastEventTimestamp = lastEventTimestamp;
return this;
}
public final Long getLastIngestionTime() {
return lastIngestionTime;
}
public final void setLastIngestionTime(Long lastIngestionTime) {
this.lastIngestionTime = lastIngestionTime;
}
@Override
@Transient
public final Builder lastIngestionTime(Long lastIngestionTime) {
this.lastIngestionTime = lastIngestionTime;
return this;
}
public final String getUploadSequenceToken() {
return uploadSequenceToken;
}
public final void setUploadSequenceToken(String uploadSequenceToken) {
this.uploadSequenceToken = uploadSequenceToken;
}
@Override
@Transient
public final Builder uploadSequenceToken(String uploadSequenceToken) {
this.uploadSequenceToken = uploadSequenceToken;
return this;
}
public final String getArn() {
return arn;
}
public final void setArn(String arn) {
this.arn = arn;
}
@Override
@Transient
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
@Deprecated
public final Long getStoredBytes() {
return storedBytes;
}
@Deprecated
public final void setStoredBytes(Long storedBytes) {
this.storedBytes = storedBytes;
}
@Override
@Transient
@Deprecated
public final Builder storedBytes(Long storedBytes) {
this.storedBytes = storedBytes;
return this;
}
@Override
public LogStream build() {
return new LogStream(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}