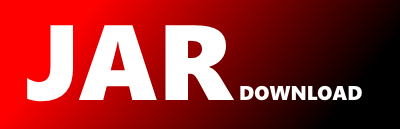
software.amazon.awssdk.services.cloudwatchlogs.model.PutAccountPolicyRequest Maven / Gradle / Ivy
Show all versions of cloudwatchlogs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatchlogs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutAccountPolicyRequest extends CloudWatchLogsRequest implements
ToCopyableBuilder {
private static final SdkField POLICY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("policyName").getter(getter(PutAccountPolicyRequest::policyName)).setter(setter(Builder::policyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("policyName").build()).build();
private static final SdkField POLICY_DOCUMENT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("policyDocument").getter(getter(PutAccountPolicyRequest::policyDocument))
.setter(setter(Builder::policyDocument))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("policyDocument").build()).build();
private static final SdkField POLICY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("policyType").getter(getter(PutAccountPolicyRequest::policyTypeAsString))
.setter(setter(Builder::policyType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("policyType").build()).build();
private static final SdkField SCOPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("scope")
.getter(getter(PutAccountPolicyRequest::scopeAsString)).setter(setter(Builder::scope))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scope").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(POLICY_NAME_FIELD,
POLICY_DOCUMENT_FIELD, POLICY_TYPE_FIELD, SCOPE_FIELD));
private final String policyName;
private final String policyDocument;
private final String policyType;
private final String scope;
private PutAccountPolicyRequest(BuilderImpl builder) {
super(builder);
this.policyName = builder.policyName;
this.policyDocument = builder.policyDocument;
this.policyType = builder.policyType;
this.scope = builder.scope;
}
/**
*
* A name for the policy. This must be unique within the account.
*
*
* @return A name for the policy. This must be unique within the account.
*/
public final String policyName() {
return policyName;
}
/**
*
* Specify the data protection policy, in JSON.
*
*
* This policy must include two JSON blocks:
*
*
* -
*
* The first block must include both a DataIdentifer
array and an Operation
property with
* an Audit
action. The DataIdentifer
array lists the types of sensitive data that you
* want to mask. For more information about the available options, see Types of data
* that you can mask.
*
*
* The Operation
property with an Audit
action is required to find the sensitive data
* terms. This Audit
action must contain a FindingsDestination
object. You can optionally
* use that FindingsDestination
object to list one or more destinations to send audit findings to. If
* you specify destinations such as log groups, Kinesis Data Firehose streams, and S3 buckets, they must already
* exist.
*
*
* -
*
* The second block must include both a DataIdentifer
array and an Operation
property with
* an Deidentify
action. The DataIdentifer
array must exactly match the
* DataIdentifer
array in the first block of the policy.
*
*
* The Operation
property with the Deidentify
action is what actually masks the data, and
* it must contain the "MaskConfig": {}
object. The "MaskConfig": {}
object must be
* empty.
*
*
*
*
* For an example data protection policy, see the Examples section on this page.
*
*
*
* The contents of the two DataIdentifer
arrays must match exactly.
*
*
*
* In addition to the two JSON blocks, the policyDocument
can also include Name
,
* Description
, and Version
fields. The Name
is different than the
* operation's policyName
parameter, and is used as a dimension when CloudWatch Logs reports audit
* findings metrics to CloudWatch.
*
*
* The JSON specified in policyDocument
can be up to 30,720 characters.
*
*
* @return Specify the data protection policy, in JSON.
*
* This policy must include two JSON blocks:
*
*
* -
*
* The first block must include both a DataIdentifer
array and an Operation
* property with an Audit
action. The DataIdentifer
array lists the types of
* sensitive data that you want to mask. For more information about the available options, see Types
* of data that you can mask.
*
*
* The Operation
property with an Audit
action is required to find the sensitive
* data terms. This Audit
action must contain a FindingsDestination
object. You
* can optionally use that FindingsDestination
object to list one or more destinations to send
* audit findings to. If you specify destinations such as log groups, Kinesis Data Firehose streams, and S3
* buckets, they must already exist.
*
*
* -
*
* The second block must include both a DataIdentifer
array and an Operation
* property with an Deidentify
action. The DataIdentifer
array must exactly match
* the DataIdentifer
array in the first block of the policy.
*
*
* The Operation
property with the Deidentify
action is what actually masks the
* data, and it must contain the "MaskConfig": {}
object. The "MaskConfig": {}
* object must be empty.
*
*
*
*
* For an example data protection policy, see the Examples section on this page.
*
*
*
* The contents of the two DataIdentifer
arrays must match exactly.
*
*
*
* In addition to the two JSON blocks, the policyDocument
can also include Name
,
* Description
, and Version
fields. The Name
is different than the
* operation's policyName
parameter, and is used as a dimension when CloudWatch Logs reports
* audit findings metrics to CloudWatch.
*
*
* The JSON specified in policyDocument
can be up to 30,720 characters.
*/
public final String policyDocument() {
return policyDocument;
}
/**
*
* Currently the only valid value for this parameter is DATA_PROTECTION_POLICY
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #policyType} will
* return {@link PolicyType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #policyTypeAsString}.
*
*
* @return Currently the only valid value for this parameter is DATA_PROTECTION_POLICY
.
* @see PolicyType
*/
public final PolicyType policyType() {
return PolicyType.fromValue(policyType);
}
/**
*
* Currently the only valid value for this parameter is DATA_PROTECTION_POLICY
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #policyType} will
* return {@link PolicyType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #policyTypeAsString}.
*
*
* @return Currently the only valid value for this parameter is DATA_PROTECTION_POLICY
.
* @see PolicyType
*/
public final String policyTypeAsString() {
return policyType;
}
/**
*
* Currently the only valid value for this parameter is ALL
, which specifies that the data protection
* policy applies to all log groups in the account. If you omit this parameter, the default of ALL
is
* used.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scope} will return
* {@link Scope#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scopeAsString}.
*
*
* @return Currently the only valid value for this parameter is ALL
, which specifies that the data
* protection policy applies to all log groups in the account. If you omit this parameter, the default of
* ALL
is used.
* @see Scope
*/
public final Scope scope() {
return Scope.fromValue(scope);
}
/**
*
* Currently the only valid value for this parameter is ALL
, which specifies that the data protection
* policy applies to all log groups in the account. If you omit this parameter, the default of ALL
is
* used.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scope} will return
* {@link Scope#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #scopeAsString}.
*
*
* @return Currently the only valid value for this parameter is ALL
, which specifies that the data
* protection policy applies to all log groups in the account. If you omit this parameter, the default of
* ALL
is used.
* @see Scope
*/
public final String scopeAsString() {
return scope;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(policyName());
hashCode = 31 * hashCode + Objects.hashCode(policyDocument());
hashCode = 31 * hashCode + Objects.hashCode(policyTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(scopeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutAccountPolicyRequest)) {
return false;
}
PutAccountPolicyRequest other = (PutAccountPolicyRequest) obj;
return Objects.equals(policyName(), other.policyName()) && Objects.equals(policyDocument(), other.policyDocument())
&& Objects.equals(policyTypeAsString(), other.policyTypeAsString())
&& Objects.equals(scopeAsString(), other.scopeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PutAccountPolicyRequest").add("PolicyName", policyName())
.add("PolicyDocument", policyDocument()).add("PolicyType", policyTypeAsString()).add("Scope", scopeAsString())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "policyName":
return Optional.ofNullable(clazz.cast(policyName()));
case "policyDocument":
return Optional.ofNullable(clazz.cast(policyDocument()));
case "policyType":
return Optional.ofNullable(clazz.cast(policyTypeAsString()));
case "scope":
return Optional.ofNullable(clazz.cast(scopeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function