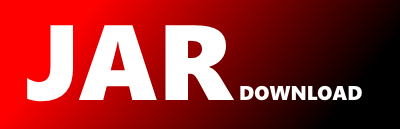
software.amazon.awssdk.services.cloudwatchlogs.model.GetLogEventsRequest Maven / Gradle / Ivy
Show all versions of cloudwatchlogs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatchlogs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetLogEventsRequest extends CloudWatchLogsRequest implements
ToCopyableBuilder {
private static final SdkField LOG_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logGroupName").getter(getter(GetLogEventsRequest::logGroupName)).setter(setter(Builder::logGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logGroupName").build()).build();
private static final SdkField LOG_GROUP_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logGroupIdentifier").getter(getter(GetLogEventsRequest::logGroupIdentifier))
.setter(setter(Builder::logGroupIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logGroupIdentifier").build())
.build();
private static final SdkField LOG_STREAM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logStreamName").getter(getter(GetLogEventsRequest::logStreamName))
.setter(setter(Builder::logStreamName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logStreamName").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.LONG).memberName("startTime")
.getter(getter(GetLogEventsRequest::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.LONG).memberName("endTime")
.getter(getter(GetLogEventsRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("nextToken").getter(getter(GetLogEventsRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextToken").build()).build();
private static final SdkField LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("limit")
.getter(getter(GetLogEventsRequest::limit)).setter(setter(Builder::limit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("limit").build()).build();
private static final SdkField START_FROM_HEAD_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("startFromHead").getter(getter(GetLogEventsRequest::startFromHead))
.setter(setter(Builder::startFromHead))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startFromHead").build()).build();
private static final SdkField UNMASK_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("unmask").getter(getter(GetLogEventsRequest::unmask)).setter(setter(Builder::unmask))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("unmask").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOG_GROUP_NAME_FIELD,
LOG_GROUP_IDENTIFIER_FIELD, LOG_STREAM_NAME_FIELD, START_TIME_FIELD, END_TIME_FIELD, NEXT_TOKEN_FIELD, LIMIT_FIELD,
START_FROM_HEAD_FIELD, UNMASK_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String logGroupName;
private final String logGroupIdentifier;
private final String logStreamName;
private final Long startTime;
private final Long endTime;
private final String nextToken;
private final Integer limit;
private final Boolean startFromHead;
private final Boolean unmask;
private GetLogEventsRequest(BuilderImpl builder) {
super(builder);
this.logGroupName = builder.logGroupName;
this.logGroupIdentifier = builder.logGroupIdentifier;
this.logStreamName = builder.logStreamName;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.nextToken = builder.nextToken;
this.limit = builder.limit;
this.startFromHead = builder.startFromHead;
this.unmask = builder.unmask;
}
/**
*
* The name of the log group.
*
*
*
* You must include either logGroupIdentifier
or logGroupName
, but not both.
*
*
*
* @return The name of the log group.
*
* You must include either logGroupIdentifier
or logGroupName
, but not both.
*
*/
public final String logGroupName() {
return logGroupName;
}
/**
*
* Specify either the name or ARN of the log group to view events from. If the log group is in a source account and
* you are using a monitoring account, you must use the log group ARN.
*
*
*
* You must include either logGroupIdentifier
or logGroupName
, but not both.
*
*
*
* @return Specify either the name or ARN of the log group to view events from. If the log group is in a source
* account and you are using a monitoring account, you must use the log group ARN.
*
* You must include either logGroupIdentifier
or logGroupName
, but not both.
*
*/
public final String logGroupIdentifier() {
return logGroupIdentifier;
}
/**
*
* The name of the log stream.
*
*
* @return The name of the log stream.
*/
public final String logStreamName() {
return logStreamName;
}
/**
*
* The start of the time range, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC
.
* Events with a timestamp equal to this time or later than this time are included. Events with a timestamp earlier
* than this time are not included.
*
*
* @return The start of the time range, expressed as the number of milliseconds after
* Jan 1, 1970 00:00:00 UTC
. Events with a timestamp equal to this time or later than this time
* are included. Events with a timestamp earlier than this time are not included.
*/
public final Long startTime() {
return startTime;
}
/**
*
* The end of the time range, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC
.
* Events with a timestamp equal to or later than this time are not included.
*
*
* @return The end of the time range, expressed as the number of milliseconds after
* Jan 1, 1970 00:00:00 UTC
. Events with a timestamp equal to or later than this time are not
* included.
*/
public final Long endTime() {
return endTime;
}
/**
*
* The token for the next set of items to return. (You received this token from a previous call.)
*
*
* @return The token for the next set of items to return. (You received this token from a previous call.)
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The maximum number of log events returned. If you don't specify a limit, the default is as many log events as can
* fit in a response size of 1 MB (up to 10,000 log events).
*
*
* @return The maximum number of log events returned. If you don't specify a limit, the default is as many log
* events as can fit in a response size of 1 MB (up to 10,000 log events).
*/
public final Integer limit() {
return limit;
}
/**
*
* If the value is true, the earliest log events are returned first. If the value is false, the latest log events
* are returned first. The default value is false.
*
*
* If you are using a previous nextForwardToken
value as the nextToken
in this operation,
* you must specify true
for startFromHead
.
*
*
* @return If the value is true, the earliest log events are returned first. If the value is false, the latest log
* events are returned first. The default value is false.
*
* If you are using a previous nextForwardToken
value as the nextToken
in this
* operation, you must specify true
for startFromHead
.
*/
public final Boolean startFromHead() {
return startFromHead;
}
/**
*
* Specify true
to display the log event fields with all sensitive data unmasked and visible. The
* default is false
.
*
*
* To use this operation with this parameter, you must be signed into an account with the logs:Unmask
* permission.
*
*
* @return Specify true
to display the log event fields with all sensitive data unmasked and visible.
* The default is false
.
*
* To use this operation with this parameter, you must be signed into an account with the
* logs:Unmask
permission.
*/
public final Boolean unmask() {
return unmask;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(logGroupName());
hashCode = 31 * hashCode + Objects.hashCode(logGroupIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(logStreamName());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(limit());
hashCode = 31 * hashCode + Objects.hashCode(startFromHead());
hashCode = 31 * hashCode + Objects.hashCode(unmask());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetLogEventsRequest)) {
return false;
}
GetLogEventsRequest other = (GetLogEventsRequest) obj;
return Objects.equals(logGroupName(), other.logGroupName())
&& Objects.equals(logGroupIdentifier(), other.logGroupIdentifier())
&& Objects.equals(logStreamName(), other.logStreamName()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(nextToken(), other.nextToken())
&& Objects.equals(limit(), other.limit()) && Objects.equals(startFromHead(), other.startFromHead())
&& Objects.equals(unmask(), other.unmask());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetLogEventsRequest").add("LogGroupName", logGroupName())
.add("LogGroupIdentifier", logGroupIdentifier()).add("LogStreamName", logStreamName())
.add("StartTime", startTime()).add("EndTime", endTime()).add("NextToken", nextToken()).add("Limit", limit())
.add("StartFromHead", startFromHead()).add("Unmask", unmask()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "logGroupName":
return Optional.ofNullable(clazz.cast(logGroupName()));
case "logGroupIdentifier":
return Optional.ofNullable(clazz.cast(logGroupIdentifier()));
case "logStreamName":
return Optional.ofNullable(clazz.cast(logStreamName()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "endTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "limit":
return Optional.ofNullable(clazz.cast(limit()));
case "startFromHead":
return Optional.ofNullable(clazz.cast(startFromHead()));
case "unmask":
return Optional.ofNullable(clazz.cast(unmask()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("logGroupName", LOG_GROUP_NAME_FIELD);
map.put("logGroupIdentifier", LOG_GROUP_IDENTIFIER_FIELD);
map.put("logStreamName", LOG_STREAM_NAME_FIELD);
map.put("startTime", START_TIME_FIELD);
map.put("endTime", END_TIME_FIELD);
map.put("nextToken", NEXT_TOKEN_FIELD);
map.put("limit", LIMIT_FIELD);
map.put("startFromHead", START_FROM_HEAD_FIELD);
map.put("unmask", UNMASK_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* You must include either logGroupIdentifier
or logGroupName
, but not both.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder logGroupName(String logGroupName);
/**
*
* Specify either the name or ARN of the log group to view events from. If the log group is in a source account
* and you are using a monitoring account, you must use the log group ARN.
*
*
*
* You must include either logGroupIdentifier
or logGroupName
, but not both.
*
*
*
* @param logGroupIdentifier
* Specify either the name or ARN of the log group to view events from. If the log group is in a source
* account and you are using a monitoring account, you must use the log group ARN.
*
* You must include either logGroupIdentifier
or logGroupName
, but not both.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder logGroupIdentifier(String logGroupIdentifier);
/**
*
* The name of the log stream.
*
*
* @param logStreamName
* The name of the log stream.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder logStreamName(String logStreamName);
/**
*
* The start of the time range, expressed as the number of milliseconds after
* Jan 1, 1970 00:00:00 UTC
. Events with a timestamp equal to this time or later than this time are
* included. Events with a timestamp earlier than this time are not included.
*
*
* @param startTime
* The start of the time range, expressed as the number of milliseconds after
* Jan 1, 1970 00:00:00 UTC
. Events with a timestamp equal to this time or later than this
* time are included. Events with a timestamp earlier than this time are not included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder startTime(Long startTime);
/**
*
* The end of the time range, expressed as the number of milliseconds after
* Jan 1, 1970 00:00:00 UTC
. Events with a timestamp equal to or later than this time are not
* included.
*
*
* @param endTime
* The end of the time range, expressed as the number of milliseconds after
* Jan 1, 1970 00:00:00 UTC
. Events with a timestamp equal to or later than this time are
* not included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder endTime(Long endTime);
/**
*
* The token for the next set of items to return. (You received this token from a previous call.)
*
*
* @param nextToken
* The token for the next set of items to return. (You received this token from a previous call.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nextToken(String nextToken);
/**
*
* The maximum number of log events returned. If you don't specify a limit, the default is as many log events as
* can fit in a response size of 1 MB (up to 10,000 log events).
*
*
* @param limit
* The maximum number of log events returned. If you don't specify a limit, the default is as many log
* events as can fit in a response size of 1 MB (up to 10,000 log events).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder limit(Integer limit);
/**
*
* If the value is true, the earliest log events are returned first. If the value is false, the latest log
* events are returned first. The default value is false.
*
*
* If you are using a previous nextForwardToken
value as the nextToken
in this
* operation, you must specify true
for startFromHead
.
*
*
* @param startFromHead
* If the value is true, the earliest log events are returned first. If the value is false, the latest
* log events are returned first. The default value is false.
*
* If you are using a previous nextForwardToken
value as the nextToken
in this
* operation, you must specify true
for startFromHead
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder startFromHead(Boolean startFromHead);
/**
*
* Specify true
to display the log event fields with all sensitive data unmasked and visible. The
* default is false
.
*
*
* To use this operation with this parameter, you must be signed into an account with the
* logs:Unmask
permission.
*
*
* @param unmask
* Specify true
to display the log event fields with all sensitive data unmasked and
* visible. The default is false
.
*
* To use this operation with this parameter, you must be signed into an account with the
* logs:Unmask
permission.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder unmask(Boolean unmask);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudWatchLogsRequest.BuilderImpl implements Builder {
private String logGroupName;
private String logGroupIdentifier;
private String logStreamName;
private Long startTime;
private Long endTime;
private String nextToken;
private Integer limit;
private Boolean startFromHead;
private Boolean unmask;
private BuilderImpl() {
}
private BuilderImpl(GetLogEventsRequest model) {
super(model);
logGroupName(model.logGroupName);
logGroupIdentifier(model.logGroupIdentifier);
logStreamName(model.logStreamName);
startTime(model.startTime);
endTime(model.endTime);
nextToken(model.nextToken);
limit(model.limit);
startFromHead(model.startFromHead);
unmask(model.unmask);
}
public final String getLogGroupName() {
return logGroupName;
}
public final void setLogGroupName(String logGroupName) {
this.logGroupName = logGroupName;
}
@Override
public final Builder logGroupName(String logGroupName) {
this.logGroupName = logGroupName;
return this;
}
public final String getLogGroupIdentifier() {
return logGroupIdentifier;
}
public final void setLogGroupIdentifier(String logGroupIdentifier) {
this.logGroupIdentifier = logGroupIdentifier;
}
@Override
public final Builder logGroupIdentifier(String logGroupIdentifier) {
this.logGroupIdentifier = logGroupIdentifier;
return this;
}
public final String getLogStreamName() {
return logStreamName;
}
public final void setLogStreamName(String logStreamName) {
this.logStreamName = logStreamName;
}
@Override
public final Builder logStreamName(String logStreamName) {
this.logStreamName = logStreamName;
return this;
}
public final Long getStartTime() {
return startTime;
}
public final void setStartTime(Long startTime) {
this.startTime = startTime;
}
@Override
public final Builder startTime(Long startTime) {
this.startTime = startTime;
return this;
}
public final Long getEndTime() {
return endTime;
}
public final void setEndTime(Long endTime) {
this.endTime = endTime;
}
@Override
public final Builder endTime(Long endTime) {
this.endTime = endTime;
return this;
}
public final String getNextToken() {
return nextToken;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
public final Integer getLimit() {
return limit;
}
public final void setLimit(Integer limit) {
this.limit = limit;
}
@Override
public final Builder limit(Integer limit) {
this.limit = limit;
return this;
}
public final Boolean getStartFromHead() {
return startFromHead;
}
public final void setStartFromHead(Boolean startFromHead) {
this.startFromHead = startFromHead;
}
@Override
public final Builder startFromHead(Boolean startFromHead) {
this.startFromHead = startFromHead;
return this;
}
public final Boolean getUnmask() {
return unmask;
}
public final void setUnmask(Boolean unmask) {
this.unmask = unmask;
}
@Override
public final Builder unmask(Boolean unmask) {
this.unmask = unmask;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public GetLogEventsRequest build() {
return new GetLogEventsRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}