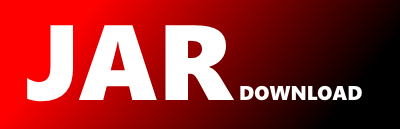
software.amazon.awssdk.services.cloudwatchlogs.model.LogGroup Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatchlogs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a log group.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LogGroup implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField LOG_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logGroupName").getter(getter(LogGroup::logGroupName)).setter(setter(Builder::logGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logGroupName").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("creationTime").getter(getter(LogGroup::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("creationTime").build()).build();
private static final SdkField RETENTION_IN_DAYS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("retentionInDays").getter(getter(LogGroup::retentionInDays)).setter(setter(Builder::retentionInDays))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("retentionInDays").build()).build();
private static final SdkField METRIC_FILTER_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("metricFilterCount").getter(getter(LogGroup::metricFilterCount))
.setter(setter(Builder::metricFilterCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metricFilterCount").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(LogGroup::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField STORED_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("storedBytes").getter(getter(LogGroup::storedBytes)).setter(setter(Builder::storedBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("storedBytes").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kmsKeyId").getter(getter(LogGroup::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyId").build()).build();
private static final SdkField DATA_PROTECTION_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dataProtectionStatus").getter(getter(LogGroup::dataProtectionStatusAsString))
.setter(setter(Builder::dataProtectionStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataProtectionStatus").build())
.build();
private static final SdkField> INHERITED_PROPERTIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("inheritedProperties")
.getter(getter(LogGroup::inheritedPropertiesAsStrings))
.setter(setter(Builder::inheritedPropertiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inheritedProperties").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LOG_GROUP_CLASS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logGroupClass").getter(getter(LogGroup::logGroupClassAsString)).setter(setter(Builder::logGroupClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logGroupClass").build()).build();
private static final SdkField LOG_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logGroupArn").getter(getter(LogGroup::logGroupArn)).setter(setter(Builder::logGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logGroupArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOG_GROUP_NAME_FIELD,
CREATION_TIME_FIELD, RETENTION_IN_DAYS_FIELD, METRIC_FILTER_COUNT_FIELD, ARN_FIELD, STORED_BYTES_FIELD,
KMS_KEY_ID_FIELD, DATA_PROTECTION_STATUS_FIELD, INHERITED_PROPERTIES_FIELD, LOG_GROUP_CLASS_FIELD,
LOG_GROUP_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String logGroupName;
private final Long creationTime;
private final Integer retentionInDays;
private final Integer metricFilterCount;
private final String arn;
private final Long storedBytes;
private final String kmsKeyId;
private final String dataProtectionStatus;
private final List inheritedProperties;
private final String logGroupClass;
private final String logGroupArn;
private LogGroup(BuilderImpl builder) {
this.logGroupName = builder.logGroupName;
this.creationTime = builder.creationTime;
this.retentionInDays = builder.retentionInDays;
this.metricFilterCount = builder.metricFilterCount;
this.arn = builder.arn;
this.storedBytes = builder.storedBytes;
this.kmsKeyId = builder.kmsKeyId;
this.dataProtectionStatus = builder.dataProtectionStatus;
this.inheritedProperties = builder.inheritedProperties;
this.logGroupClass = builder.logGroupClass;
this.logGroupArn = builder.logGroupArn;
}
/**
*
* The name of the log group.
*
*
* @return The name of the log group.
*/
public final String logGroupName() {
return logGroupName;
}
/**
*
* The creation time of the log group, expressed as the number of milliseconds after Jan 1, 1970 00:00:00 UTC.
*
*
* @return The creation time of the log group, expressed as the number of milliseconds after Jan 1, 1970 00:00:00
* UTC.
*/
public final Long creationTime() {
return creationTime;
}
/**
* Returns the value of the RetentionInDays property for this object.
*
* @return The value of the RetentionInDays property for this object.
*/
public final Integer retentionInDays() {
return retentionInDays;
}
/**
*
* The number of metric filters.
*
*
* @return The number of metric filters.
*/
public final Integer metricFilterCount() {
return metricFilterCount;
}
/**
*
* The Amazon Resource Name (ARN) of the log group. This version of the ARN includes a trailing :*
* after the log group name.
*
*
* Use this version to refer to the ARN in IAM policies when specifying permissions for most API actions. The
* exception is when specifying permissions for TagResource,
*
* UntagResource, and ListTagsForResource. The permissions for those three actions require the ARN version that doesn't include a
* trailing :*
.
*
*
* @return The Amazon Resource Name (ARN) of the log group. This version of the ARN includes a trailing
* :*
after the log group name.
*
* Use this version to refer to the ARN in IAM policies when specifying permissions for most API actions.
* The exception is when specifying permissions for TagResource, UntagResource, and ListTagsForResource. The permissions for those three actions require the ARN version that doesn't
* include a trailing :*
.
*/
public final String arn() {
return arn;
}
/**
*
* The number of bytes stored.
*
*
* @return The number of bytes stored.
*/
public final Long storedBytes() {
return storedBytes;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key to use when encrypting log data.
*
*
* @return The Amazon Resource Name (ARN) of the KMS key to use when encrypting log data.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* Displays whether this log group has a protection policy, or whether it had one in the past. For more information,
* see PutDataProtectionPolicy.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #dataProtectionStatus} will return {@link DataProtectionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #dataProtectionStatusAsString}.
*
*
* @return Displays whether this log group has a protection policy, or whether it had one in the past. For more
* information, see PutDataProtectionPolicy.
* @see DataProtectionStatus
*/
public final DataProtectionStatus dataProtectionStatus() {
return DataProtectionStatus.fromValue(dataProtectionStatus);
}
/**
*
* Displays whether this log group has a protection policy, or whether it had one in the past. For more information,
* see PutDataProtectionPolicy.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #dataProtectionStatus} will return {@link DataProtectionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #dataProtectionStatusAsString}.
*
*
* @return Displays whether this log group has a protection policy, or whether it had one in the past. For more
* information, see PutDataProtectionPolicy.
* @see DataProtectionStatus
*/
public final String dataProtectionStatusAsString() {
return dataProtectionStatus;
}
/**
*
* Displays all the properties that this log group has inherited from account-level settings.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInheritedProperties} method.
*
*
* @return Displays all the properties that this log group has inherited from account-level settings.
*/
public final List inheritedProperties() {
return InheritedPropertiesCopier.copyStringToEnum(inheritedProperties);
}
/**
* For responses, this returns true if the service returned a value for the InheritedProperties property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasInheritedProperties() {
return inheritedProperties != null && !(inheritedProperties instanceof SdkAutoConstructList);
}
/**
*
* Displays all the properties that this log group has inherited from account-level settings.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInheritedProperties} method.
*
*
* @return Displays all the properties that this log group has inherited from account-level settings.
*/
public final List inheritedPropertiesAsStrings() {
return inheritedProperties;
}
/**
*
* This specifies the log group class for this log group. There are two classes:
*
*
* -
*
* The Standard
log class supports all CloudWatch Logs features.
*
*
* -
*
* The Infrequent Access
log class supports a subset of CloudWatch Logs features and incurs lower
* costs.
*
*
*
*
* For details about the features supported by each class, see Log classes
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #logGroupClass}
* will return {@link LogGroupClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #logGroupClassAsString}.
*
*
* @return This specifies the log group class for this log group. There are two classes:
*
* -
*
* The Standard
log class supports all CloudWatch Logs features.
*
*
* -
*
* The Infrequent Access
log class supports a subset of CloudWatch Logs features and incurs
* lower costs.
*
*
*
*
* For details about the features supported by each class, see Log
* classes
* @see LogGroupClass
*/
public final LogGroupClass logGroupClass() {
return LogGroupClass.fromValue(logGroupClass);
}
/**
*
* This specifies the log group class for this log group. There are two classes:
*
*
* -
*
* The Standard
log class supports all CloudWatch Logs features.
*
*
* -
*
* The Infrequent Access
log class supports a subset of CloudWatch Logs features and incurs lower
* costs.
*
*
*
*
* For details about the features supported by each class, see Log classes
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #logGroupClass}
* will return {@link LogGroupClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #logGroupClassAsString}.
*
*
* @return This specifies the log group class for this log group. There are two classes:
*
* -
*
* The Standard
log class supports all CloudWatch Logs features.
*
*
* -
*
* The Infrequent Access
log class supports a subset of CloudWatch Logs features and incurs
* lower costs.
*
*
*
*
* For details about the features supported by each class, see Log
* classes
* @see LogGroupClass
*/
public final String logGroupClassAsString() {
return logGroupClass;
}
/**
*
* The Amazon Resource Name (ARN) of the log group. This version of the ARN doesn't include a trailing
* :*
after the log group name.
*
*
* Use this version to refer to the ARN in the following situations:
*
*
* -
*
* In the logGroupIdentifier
input field in many CloudWatch Logs APIs.
*
*
* -
*
* In the resourceArn
field in tagging APIs
*
*
* -
*
* In IAM policies, when specifying permissions for TagResource,
*
* UntagResource, and ListTagsForResource.
*
*
*
*
* @return The Amazon Resource Name (ARN) of the log group. This version of the ARN doesn't include a trailing
* :*
after the log group name.
*
* Use this version to refer to the ARN in the following situations:
*
*
* -
*
* In the logGroupIdentifier
input field in many CloudWatch Logs APIs.
*
*
* -
*
* In the resourceArn
field in tagging APIs
*
*
* -
*
* In IAM policies, when specifying permissions for TagResource, UntagResource, and ListTagsForResource.
*
*
*/
public final String logGroupArn() {
return logGroupArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(logGroupName());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(retentionInDays());
hashCode = 31 * hashCode + Objects.hashCode(metricFilterCount());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(storedBytes());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(dataProtectionStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasInheritedProperties() ? inheritedPropertiesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(logGroupClassAsString());
hashCode = 31 * hashCode + Objects.hashCode(logGroupArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LogGroup)) {
return false;
}
LogGroup other = (LogGroup) obj;
return Objects.equals(logGroupName(), other.logGroupName()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(retentionInDays(), other.retentionInDays())
&& Objects.equals(metricFilterCount(), other.metricFilterCount()) && Objects.equals(arn(), other.arn())
&& Objects.equals(storedBytes(), other.storedBytes()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(dataProtectionStatusAsString(), other.dataProtectionStatusAsString())
&& hasInheritedProperties() == other.hasInheritedProperties()
&& Objects.equals(inheritedPropertiesAsStrings(), other.inheritedPropertiesAsStrings())
&& Objects.equals(logGroupClassAsString(), other.logGroupClassAsString())
&& Objects.equals(logGroupArn(), other.logGroupArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LogGroup").add("LogGroupName", logGroupName()).add("CreationTime", creationTime())
.add("RetentionInDays", retentionInDays()).add("MetricFilterCount", metricFilterCount()).add("Arn", arn())
.add("StoredBytes", storedBytes()).add("KmsKeyId", kmsKeyId())
.add("DataProtectionStatus", dataProtectionStatusAsString())
.add("InheritedProperties", hasInheritedProperties() ? inheritedPropertiesAsStrings() : null)
.add("LogGroupClass", logGroupClassAsString()).add("LogGroupArn", logGroupArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "logGroupName":
return Optional.ofNullable(clazz.cast(logGroupName()));
case "creationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "retentionInDays":
return Optional.ofNullable(clazz.cast(retentionInDays()));
case "metricFilterCount":
return Optional.ofNullable(clazz.cast(metricFilterCount()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "storedBytes":
return Optional.ofNullable(clazz.cast(storedBytes()));
case "kmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "dataProtectionStatus":
return Optional.ofNullable(clazz.cast(dataProtectionStatusAsString()));
case "inheritedProperties":
return Optional.ofNullable(clazz.cast(inheritedPropertiesAsStrings()));
case "logGroupClass":
return Optional.ofNullable(clazz.cast(logGroupClassAsString()));
case "logGroupArn":
return Optional.ofNullable(clazz.cast(logGroupArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("logGroupName", LOG_GROUP_NAME_FIELD);
map.put("creationTime", CREATION_TIME_FIELD);
map.put("retentionInDays", RETENTION_IN_DAYS_FIELD);
map.put("metricFilterCount", METRIC_FILTER_COUNT_FIELD);
map.put("arn", ARN_FIELD);
map.put("storedBytes", STORED_BYTES_FIELD);
map.put("kmsKeyId", KMS_KEY_ID_FIELD);
map.put("dataProtectionStatus", DATA_PROTECTION_STATUS_FIELD);
map.put("inheritedProperties", INHERITED_PROPERTIES_FIELD);
map.put("logGroupClass", LOG_GROUP_CLASS_FIELD);
map.put("logGroupArn", LOG_GROUP_ARN_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function