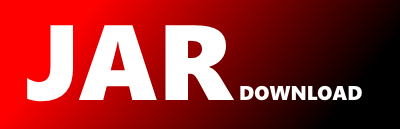
software.amazon.awssdk.services.cloudwatchlogs.model.LiveTailSessionStart Maven / Gradle / Ivy
Show all versions of cloudwatchlogs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudwatchlogs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* This object contains information about this Live Tail session, including the log groups included and the log stream
* filters, if any.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class LiveTailSessionStart implements SdkPojo, Serializable,
ToCopyableBuilder, StartLiveTailResponseStream {
private static final SdkField REQUEST_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("requestId").getter(getter(LiveTailSessionStart::requestId)).setter(setter(Builder::requestId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("requestId").build()).build();
private static final SdkField SESSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sessionId").getter(getter(LiveTailSessionStart::sessionId)).setter(setter(Builder::sessionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sessionId").build()).build();
private static final SdkField> LOG_GROUP_IDENTIFIERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("logGroupIdentifiers")
.getter(getter(LiveTailSessionStart::logGroupIdentifiers))
.setter(setter(Builder::logGroupIdentifiers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logGroupIdentifiers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LOG_STREAM_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("logStreamNames")
.getter(getter(LiveTailSessionStart::logStreamNames))
.setter(setter(Builder::logStreamNames))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logStreamNames").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LOG_STREAM_NAME_PREFIXES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("logStreamNamePrefixes")
.getter(getter(LiveTailSessionStart::logStreamNamePrefixes))
.setter(setter(Builder::logStreamNamePrefixes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logStreamNamePrefixes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LOG_EVENT_FILTER_PATTERN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logEventFilterPattern").getter(getter(LiveTailSessionStart::logEventFilterPattern))
.setter(setter(Builder::logEventFilterPattern))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logEventFilterPattern").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REQUEST_ID_FIELD,
SESSION_ID_FIELD, LOG_GROUP_IDENTIFIERS_FIELD, LOG_STREAM_NAMES_FIELD, LOG_STREAM_NAME_PREFIXES_FIELD,
LOG_EVENT_FILTER_PATTERN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String requestIdValue;
private final String sessionId;
private final List logGroupIdentifiers;
private final List logStreamNames;
private final List logStreamNamePrefixes;
private final String logEventFilterPattern;
protected LiveTailSessionStart(BuilderImpl builder) {
this.requestIdValue = builder.requestIdValue;
this.sessionId = builder.sessionId;
this.logGroupIdentifiers = builder.logGroupIdentifiers;
this.logStreamNames = builder.logStreamNames;
this.logStreamNamePrefixes = builder.logStreamNamePrefixes;
this.logEventFilterPattern = builder.logEventFilterPattern;
}
/**
*
* The unique ID generated by CloudWatch Logs to identify this Live Tail session request.
*
*
* @return The unique ID generated by CloudWatch Logs to identify this Live Tail session request.
*/
public final String requestId() {
return requestIdValue;
}
/**
*
* The unique ID generated by CloudWatch Logs to identify this Live Tail session.
*
*
* @return The unique ID generated by CloudWatch Logs to identify this Live Tail session.
*/
public final String sessionId() {
return sessionId;
}
/**
* For responses, this returns true if the service returned a value for the LogGroupIdentifiers property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLogGroupIdentifiers() {
return logGroupIdentifiers != null && !(logGroupIdentifiers instanceof SdkAutoConstructList);
}
/**
*
* An array of the names and ARNs of the log groups included in this Live Tail session.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogGroupIdentifiers} method.
*
*
* @return An array of the names and ARNs of the log groups included in this Live Tail session.
*/
public final List logGroupIdentifiers() {
return logGroupIdentifiers;
}
/**
* For responses, this returns true if the service returned a value for the LogStreamNames property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLogStreamNames() {
return logStreamNames != null && !(logStreamNames instanceof SdkAutoConstructList);
}
/**
*
* If your StartLiveTail operation request included a logStreamNames
parameter that filtered the
* session to only include certain log streams, these streams are listed here.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogStreamNames} method.
*
*
* @return If your StartLiveTail operation request included a logStreamNames
parameter that filtered
* the session to only include certain log streams, these streams are listed here.
*/
public final List logStreamNames() {
return logStreamNames;
}
/**
* For responses, this returns true if the service returned a value for the LogStreamNamePrefixes property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLogStreamNamePrefixes() {
return logStreamNamePrefixes != null && !(logStreamNamePrefixes instanceof SdkAutoConstructList);
}
/**
*
* If your StartLiveTail operation request included a logStreamNamePrefixes
parameter that filtered the
* session to only include log streams that have names that start with certain prefixes, these prefixes are listed
* here.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogStreamNamePrefixes} method.
*
*
* @return If your StartLiveTail operation request included a logStreamNamePrefixes
parameter that
* filtered the session to only include log streams that have names that start with certain prefixes, these
* prefixes are listed here.
*/
public final List logStreamNamePrefixes() {
return logStreamNamePrefixes;
}
/**
*
* An optional pattern to filter the results to include only log events that match the pattern. For example, a
* filter pattern of error 404
displays only log events that include both error
and
* 404
.
*
*
* For more information about filter pattern syntax, see Filter and Pattern
* Syntax.
*
*
* @return An optional pattern to filter the results to include only log events that match the pattern. For example,
* a filter pattern of error 404
displays only log events that include both error
* and 404
.
*
* For more information about filter pattern syntax, see Filter and
* Pattern Syntax.
*/
public final String logEventFilterPattern() {
return logEventFilterPattern;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(requestId());
hashCode = 31 * hashCode + Objects.hashCode(sessionId());
hashCode = 31 * hashCode + Objects.hashCode(hasLogGroupIdentifiers() ? logGroupIdentifiers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLogStreamNames() ? logStreamNames() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLogStreamNamePrefixes() ? logStreamNamePrefixes() : null);
hashCode = 31 * hashCode + Objects.hashCode(logEventFilterPattern());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LiveTailSessionStart)) {
return false;
}
LiveTailSessionStart other = (LiveTailSessionStart) obj;
return Objects.equals(requestId(), other.requestId()) && Objects.equals(sessionId(), other.sessionId())
&& hasLogGroupIdentifiers() == other.hasLogGroupIdentifiers()
&& Objects.equals(logGroupIdentifiers(), other.logGroupIdentifiers())
&& hasLogStreamNames() == other.hasLogStreamNames() && Objects.equals(logStreamNames(), other.logStreamNames())
&& hasLogStreamNamePrefixes() == other.hasLogStreamNamePrefixes()
&& Objects.equals(logStreamNamePrefixes(), other.logStreamNamePrefixes())
&& Objects.equals(logEventFilterPattern(), other.logEventFilterPattern());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LiveTailSessionStart").add("RequestId", requestId()).add("SessionId", sessionId())
.add("LogGroupIdentifiers", hasLogGroupIdentifiers() ? logGroupIdentifiers() : null)
.add("LogStreamNames", hasLogStreamNames() ? logStreamNames() : null)
.add("LogStreamNamePrefixes", hasLogStreamNamePrefixes() ? logStreamNamePrefixes() : null)
.add("LogEventFilterPattern", logEventFilterPattern()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "requestId":
return Optional.ofNullable(clazz.cast(requestId()));
case "sessionId":
return Optional.ofNullable(clazz.cast(sessionId()));
case "logGroupIdentifiers":
return Optional.ofNullable(clazz.cast(logGroupIdentifiers()));
case "logStreamNames":
return Optional.ofNullable(clazz.cast(logStreamNames()));
case "logStreamNamePrefixes":
return Optional.ofNullable(clazz.cast(logStreamNamePrefixes()));
case "logEventFilterPattern":
return Optional.ofNullable(clazz.cast(logEventFilterPattern()));
default:
return Optional.empty();
}
}
@Override
public final LiveTailSessionStart copy(Consumer super Builder> modifier) {
return ToCopyableBuilder.super.copy(modifier);
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("requestId", REQUEST_ID_FIELD);
map.put("sessionId", SESSION_ID_FIELD);
map.put("logGroupIdentifiers", LOG_GROUP_IDENTIFIERS_FIELD);
map.put("logStreamNames", LOG_STREAM_NAMES_FIELD);
map.put("logStreamNamePrefixes", LOG_STREAM_NAME_PREFIXES_FIELD);
map.put("logEventFilterPattern", LOG_EVENT_FILTER_PATTERN_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* For more information about filter pattern syntax, see Filter and
* Pattern Syntax.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder logEventFilterPattern(String logEventFilterPattern);
}
protected static class BuilderImpl implements Builder {
private String requestIdValue;
private String sessionId;
private List logGroupIdentifiers = DefaultSdkAutoConstructList.getInstance();
private List logStreamNames = DefaultSdkAutoConstructList.getInstance();
private List logStreamNamePrefixes = DefaultSdkAutoConstructList.getInstance();
private String logEventFilterPattern;
protected BuilderImpl() {
}
protected BuilderImpl(LiveTailSessionStart model) {
requestId(model.requestIdValue);
sessionId(model.sessionId);
logGroupIdentifiers(model.logGroupIdentifiers);
logStreamNames(model.logStreamNames);
logStreamNamePrefixes(model.logStreamNamePrefixes);
logEventFilterPattern(model.logEventFilterPattern);
}
public final String getRequestId() {
return requestIdValue;
}
public final void setRequestId(String requestIdValue) {
this.requestIdValue = requestIdValue;
}
@Override
public final Builder requestId(String requestIdValue) {
this.requestIdValue = requestIdValue;
return this;
}
public final String getSessionId() {
return sessionId;
}
public final void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
@Override
public final Builder sessionId(String sessionId) {
this.sessionId = sessionId;
return this;
}
public final Collection getLogGroupIdentifiers() {
if (logGroupIdentifiers instanceof SdkAutoConstructList) {
return null;
}
return logGroupIdentifiers;
}
public final void setLogGroupIdentifiers(Collection logGroupIdentifiers) {
this.logGroupIdentifiers = StartLiveTailLogGroupIdentifiersCopier.copy(logGroupIdentifiers);
}
@Override
public final Builder logGroupIdentifiers(Collection logGroupIdentifiers) {
this.logGroupIdentifiers = StartLiveTailLogGroupIdentifiersCopier.copy(logGroupIdentifiers);
return this;
}
@Override
@SafeVarargs
public final Builder logGroupIdentifiers(String... logGroupIdentifiers) {
logGroupIdentifiers(Arrays.asList(logGroupIdentifiers));
return this;
}
public final Collection getLogStreamNames() {
if (logStreamNames instanceof SdkAutoConstructList) {
return null;
}
return logStreamNames;
}
public final void setLogStreamNames(Collection logStreamNames) {
this.logStreamNames = InputLogStreamNamesCopier.copy(logStreamNames);
}
@Override
public final Builder logStreamNames(Collection logStreamNames) {
this.logStreamNames = InputLogStreamNamesCopier.copy(logStreamNames);
return this;
}
@Override
@SafeVarargs
public final Builder logStreamNames(String... logStreamNames) {
logStreamNames(Arrays.asList(logStreamNames));
return this;
}
public final Collection getLogStreamNamePrefixes() {
if (logStreamNamePrefixes instanceof SdkAutoConstructList) {
return null;
}
return logStreamNamePrefixes;
}
public final void setLogStreamNamePrefixes(Collection logStreamNamePrefixes) {
this.logStreamNamePrefixes = InputLogStreamNamesCopier.copy(logStreamNamePrefixes);
}
@Override
public final Builder logStreamNamePrefixes(Collection logStreamNamePrefixes) {
this.logStreamNamePrefixes = InputLogStreamNamesCopier.copy(logStreamNamePrefixes);
return this;
}
@Override
@SafeVarargs
public final Builder logStreamNamePrefixes(String... logStreamNamePrefixes) {
logStreamNamePrefixes(Arrays.asList(logStreamNamePrefixes));
return this;
}
public final String getLogEventFilterPattern() {
return logEventFilterPattern;
}
public final void setLogEventFilterPattern(String logEventFilterPattern) {
this.logEventFilterPattern = logEventFilterPattern;
}
@Override
public final Builder logEventFilterPattern(String logEventFilterPattern) {
this.logEventFilterPattern = logEventFilterPattern;
return this;
}
@Override
public LiveTailSessionStart build() {
return new LiveTailSessionStart(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}