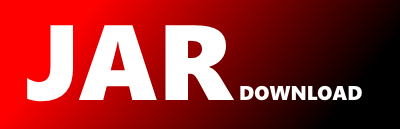
software.amazon.awssdk.services.codeartifact.model.PackageDependency Maven / Gradle / Ivy
Show all versions of codeartifact Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codeartifact.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about a package dependency.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PackageDependency implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("namespace").getter(getter(PackageDependency::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("namespace").build()).build();
private static final SdkField PACKAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("package")
.getter(getter(PackageDependency::packageValue)).setter(setter(Builder::packageValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("package").build()).build();
private static final SdkField DEPENDENCY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dependencyType").getter(getter(PackageDependency::dependencyType))
.setter(setter(Builder::dependencyType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dependencyType").build()).build();
private static final SdkField VERSION_REQUIREMENT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("versionRequirement").getter(getter(PackageDependency::versionRequirement))
.setter(setter(Builder::versionRequirement))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("versionRequirement").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAMESPACE_FIELD,
PACKAGE_FIELD, DEPENDENCY_TYPE_FIELD, VERSION_REQUIREMENT_FIELD));
private static final long serialVersionUID = 1L;
private final String namespace;
private final String packageValue;
private final String dependencyType;
private final String versionRequirement;
private PackageDependency(BuilderImpl builder) {
this.namespace = builder.namespace;
this.packageValue = builder.packageValue;
this.dependencyType = builder.dependencyType;
this.versionRequirement = builder.versionRequirement;
}
/**
*
* The namespace of the package. The package component that specifies its namespace depends on its type. For
* example:
*
*
* -
*
* The namespace of a Maven package is its groupId
.
*
*
* -
*
* The namespace of an npm package is its scope
.
*
*
* -
*
* A Python package does not contain a corresponding component, so Python packages do not have a namespace.
*
*
*
*
* @return The namespace of the package. The package component that specifies its namespace depends on its type. For
* example:
*
* -
*
* The namespace of a Maven package is its groupId
.
*
*
* -
*
* The namespace of an npm package is its scope
.
*
*
* -
*
* A Python package does not contain a corresponding component, so Python packages do not have a namespace.
*
*
*/
public String namespace() {
return namespace;
}
/**
*
* The name of the package that this package depends on.
*
*
* @return The name of the package that this package depends on.
*/
public String packageValue() {
return packageValue;
}
/**
*
* The type of a package dependency. The possible values depend on the package type. Example types are
* compile
, runtime
, and test
for Maven packages, and dev
,
* prod
, and optional
for npm packages.
*
*
* @return The type of a package dependency. The possible values depend on the package type. Example types are
* compile
, runtime
, and test
for Maven packages, and
* dev
, prod
, and optional
for npm packages.
*/
public String dependencyType() {
return dependencyType;
}
/**
*
* The required version, or version range, of the package that this package depends on. The version format is
* specific to the package type. For example, the following are possible valid required versions: 1.2.3
, ^2.3.4
, or 4.x
.
*
*
* @return The required version, or version range, of the package that this package depends on. The version format
* is specific to the package type. For example, the following are possible valid required versions:
* 1.2.3
, ^2.3.4
, or 4.x
.
*/
public String versionRequirement() {
return versionRequirement;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(packageValue());
hashCode = 31 * hashCode + Objects.hashCode(dependencyType());
hashCode = 31 * hashCode + Objects.hashCode(versionRequirement());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PackageDependency)) {
return false;
}
PackageDependency other = (PackageDependency) obj;
return Objects.equals(namespace(), other.namespace()) && Objects.equals(packageValue(), other.packageValue())
&& Objects.equals(dependencyType(), other.dependencyType())
&& Objects.equals(versionRequirement(), other.versionRequirement());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("PackageDependency").add("Namespace", namespace()).add("Package", packageValue())
.add("DependencyType", dependencyType()).add("VersionRequirement", versionRequirement()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "package":
return Optional.ofNullable(clazz.cast(packageValue()));
case "dependencyType":
return Optional.ofNullable(clazz.cast(dependencyType()));
case "versionRequirement":
return Optional.ofNullable(clazz.cast(versionRequirement()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function