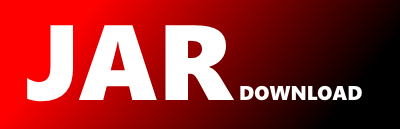
software.amazon.awssdk.services.codeartifact.model.PackageVersionDescription Maven / Gradle / Ivy
Show all versions of codeartifact Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codeartifact.model;
import java.beans.Transient;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about a package version.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PackageVersionDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField FORMAT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("format")
.getter(getter(PackageVersionDescription::formatAsString)).setter(setter(Builder::format))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("format").build()).build();
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("namespace").getter(getter(PackageVersionDescription::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("namespace").build()).build();
private static final SdkField PACKAGE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("packageName").getter(getter(PackageVersionDescription::packageName))
.setter(setter(Builder::packageName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("packageName").build()).build();
private static final SdkField DISPLAY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("displayName").getter(getter(PackageVersionDescription::displayName))
.setter(setter(Builder::displayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("displayName").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("version")
.getter(getter(PackageVersionDescription::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField SUMMARY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("summary")
.getter(getter(PackageVersionDescription::summary)).setter(setter(Builder::summary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("summary").build()).build();
private static final SdkField HOME_PAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("homePage").getter(getter(PackageVersionDescription::homePage)).setter(setter(Builder::homePage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("homePage").build()).build();
private static final SdkField SOURCE_CODE_REPOSITORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceCodeRepository").getter(getter(PackageVersionDescription::sourceCodeRepository))
.setter(setter(Builder::sourceCodeRepository))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceCodeRepository").build())
.build();
private static final SdkField PUBLISHED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("publishedTime").getter(getter(PackageVersionDescription::publishedTime))
.setter(setter(Builder::publishedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("publishedTime").build()).build();
private static final SdkField> LICENSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("licenses")
.getter(getter(PackageVersionDescription::licenses))
.setter(setter(Builder::licenses))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("licenses").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LicenseInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField REVISION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("revision").getter(getter(PackageVersionDescription::revision)).setter(setter(Builder::revision))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("revision").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(PackageVersionDescription::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FORMAT_FIELD, NAMESPACE_FIELD,
PACKAGE_NAME_FIELD, DISPLAY_NAME_FIELD, VERSION_FIELD, SUMMARY_FIELD, HOME_PAGE_FIELD, SOURCE_CODE_REPOSITORY_FIELD,
PUBLISHED_TIME_FIELD, LICENSES_FIELD, REVISION_FIELD, STATUS_FIELD));
private static final long serialVersionUID = 1L;
private final String format;
private final String namespace;
private final String packageName;
private final String displayName;
private final String version;
private final String summary;
private final String homePage;
private final String sourceCodeRepository;
private final Instant publishedTime;
private final List licenses;
private final String revision;
private final String status;
private PackageVersionDescription(BuilderImpl builder) {
this.format = builder.format;
this.namespace = builder.namespace;
this.packageName = builder.packageName;
this.displayName = builder.displayName;
this.version = builder.version;
this.summary = builder.summary;
this.homePage = builder.homePage;
this.sourceCodeRepository = builder.sourceCodeRepository;
this.publishedTime = builder.publishedTime;
this.licenses = builder.licenses;
this.revision = builder.revision;
this.status = builder.status;
}
/**
*
* The format of the package version. The valid package formats are:
*
*
* -
*
* npm
: A Node Package Manager (npm) package.
*
*
* -
*
* pypi
: A Python Package Index (PyPI) package.
*
*
* -
*
* maven
: A Maven package that contains compiled code in a distributable format, such as a JAR file.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link PackageFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formatAsString}.
*
*
* @return The format of the package version. The valid package formats are:
*
* -
*
* npm
: A Node Package Manager (npm) package.
*
*
* -
*
* pypi
: A Python Package Index (PyPI) package.
*
*
* -
*
* maven
: A Maven package that contains compiled code in a distributable format, such as a JAR
* file.
*
*
* @see PackageFormat
*/
public final PackageFormat format() {
return PackageFormat.fromValue(format);
}
/**
*
* The format of the package version. The valid package formats are:
*
*
* -
*
* npm
: A Node Package Manager (npm) package.
*
*
* -
*
* pypi
: A Python Package Index (PyPI) package.
*
*
* -
*
* maven
: A Maven package that contains compiled code in a distributable format, such as a JAR file.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link PackageFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formatAsString}.
*
*
* @return The format of the package version. The valid package formats are:
*
* -
*
* npm
: A Node Package Manager (npm) package.
*
*
* -
*
* pypi
: A Python Package Index (PyPI) package.
*
*
* -
*
* maven
: A Maven package that contains compiled code in a distributable format, such as a JAR
* file.
*
*
* @see PackageFormat
*/
public final String formatAsString() {
return format;
}
/**
*
* The namespace of the package. The package component that specifies its namespace depends on its type. For
* example:
*
*
* -
*
* The namespace of a Maven package is its groupId
.
*
*
* -
*
* The namespace of an npm package is its scope
.
*
*
* -
*
* A Python package does not contain a corresponding component, so Python packages do not have a namespace.
*
*
*
*
* @return The namespace of the package. The package component that specifies its namespace depends on its type. For
* example:
*
* -
*
* The namespace of a Maven package is its groupId
.
*
*
* -
*
* The namespace of an npm package is its scope
.
*
*
* -
*
* A Python package does not contain a corresponding component, so Python packages do not have a namespace.
*
*
*/
public final String namespace() {
return namespace;
}
/**
*
* The name of the requested package.
*
*
* @return The name of the requested package.
*/
public final String packageName() {
return packageName;
}
/**
*
* The name of the package that is displayed. The displayName
varies depending on the package version's
* format. For example, if an npm package is named ui
, is in the namespace vue
, and has
* the format npm
, then the displayName
is @vue/ui
.
*
*
* @return The name of the package that is displayed. The displayName
varies depending on the package
* version's format. For example, if an npm package is named ui
, is in the namespace
* vue
, and has the format npm
, then the displayName
is
* @vue/ui
.
*/
public final String displayName() {
return displayName;
}
/**
*
* The version of the package.
*
*
* @return The version of the package.
*/
public final String version() {
return version;
}
/**
*
* A summary of the package version. The summary is extracted from the package. The information in and detail level
* of the summary depends on the package version's format.
*
*
* @return A summary of the package version. The summary is extracted from the package. The information in and
* detail level of the summary depends on the package version's format.
*/
public final String summary() {
return summary;
}
/**
*
* The homepage associated with the package.
*
*
* @return The homepage associated with the package.
*/
public final String homePage() {
return homePage;
}
/**
*
* The repository for the source code in the package version, or the source code used to build it.
*
*
* @return The repository for the source code in the package version, or the source code used to build it.
*/
public final String sourceCodeRepository() {
return sourceCodeRepository;
}
/**
*
* A timestamp that contains the date and time the package version was published.
*
*
* @return A timestamp that contains the date and time the package version was published.
*/
public final Instant publishedTime() {
return publishedTime;
}
/**
* For responses, this returns true if the service returned a value for the Licenses property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasLicenses() {
return licenses != null && !(licenses instanceof SdkAutoConstructList);
}
/**
*
* Information about licenses associated with the package version.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLicenses} method.
*
*
* @return Information about licenses associated with the package version.
*/
public final List licenses() {
return licenses;
}
/**
*
* The revision of the package version.
*
*
* @return The revision of the package version.
*/
public final String revision() {
return revision;
}
/**
*
* A string that contains the status of the package version. It can be one of the following:
*
*
* -
*
* Published
*
*
* -
*
* Unfinished
*
*
* -
*
* Unlisted
*
*
* -
*
* Archived
*
*
* -
*
* Disposed
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link PackageVersionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return A string that contains the status of the package version. It can be one of the following:
*
* -
*
* Published
*
*
* -
*
* Unfinished
*
*
* -
*
* Unlisted
*
*
* -
*
* Archived
*
*
* -
*
* Disposed
*
*
* @see PackageVersionStatus
*/
public final PackageVersionStatus status() {
return PackageVersionStatus.fromValue(status);
}
/**
*
* A string that contains the status of the package version. It can be one of the following:
*
*
* -
*
* Published
*
*
* -
*
* Unfinished
*
*
* -
*
* Unlisted
*
*
* -
*
* Archived
*
*
* -
*
* Disposed
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link PackageVersionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return A string that contains the status of the package version. It can be one of the following:
*
* -
*
* Published
*
*
* -
*
* Unfinished
*
*
* -
*
* Unlisted
*
*
* -
*
* Archived
*
*
* -
*
* Disposed
*
*
* @see PackageVersionStatus
*/
public final String statusAsString() {
return status;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(formatAsString());
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(packageName());
hashCode = 31 * hashCode + Objects.hashCode(displayName());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(summary());
hashCode = 31 * hashCode + Objects.hashCode(homePage());
hashCode = 31 * hashCode + Objects.hashCode(sourceCodeRepository());
hashCode = 31 * hashCode + Objects.hashCode(publishedTime());
hashCode = 31 * hashCode + Objects.hashCode(hasLicenses() ? licenses() : null);
hashCode = 31 * hashCode + Objects.hashCode(revision());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PackageVersionDescription)) {
return false;
}
PackageVersionDescription other = (PackageVersionDescription) obj;
return Objects.equals(formatAsString(), other.formatAsString()) && Objects.equals(namespace(), other.namespace())
&& Objects.equals(packageName(), other.packageName()) && Objects.equals(displayName(), other.displayName())
&& Objects.equals(version(), other.version()) && Objects.equals(summary(), other.summary())
&& Objects.equals(homePage(), other.homePage())
&& Objects.equals(sourceCodeRepository(), other.sourceCodeRepository())
&& Objects.equals(publishedTime(), other.publishedTime()) && hasLicenses() == other.hasLicenses()
&& Objects.equals(licenses(), other.licenses()) && Objects.equals(revision(), other.revision())
&& Objects.equals(statusAsString(), other.statusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PackageVersionDescription").add("Format", formatAsString()).add("Namespace", namespace())
.add("PackageName", packageName()).add("DisplayName", displayName()).add("Version", version())
.add("Summary", summary()).add("HomePage", homePage()).add("SourceCodeRepository", sourceCodeRepository())
.add("PublishedTime", publishedTime()).add("Licenses", hasLicenses() ? licenses() : null)
.add("Revision", revision()).add("Status", statusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "format":
return Optional.ofNullable(clazz.cast(formatAsString()));
case "namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "packageName":
return Optional.ofNullable(clazz.cast(packageName()));
case "displayName":
return Optional.ofNullable(clazz.cast(displayName()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "summary":
return Optional.ofNullable(clazz.cast(summary()));
case "homePage":
return Optional.ofNullable(clazz.cast(homePage()));
case "sourceCodeRepository":
return Optional.ofNullable(clazz.cast(sourceCodeRepository()));
case "publishedTime":
return Optional.ofNullable(clazz.cast(publishedTime()));
case "licenses":
return Optional.ofNullable(clazz.cast(licenses()));
case "revision":
return Optional.ofNullable(clazz.cast(revision()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function