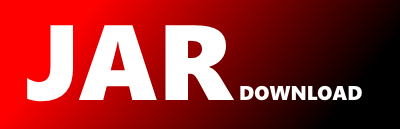
software.amazon.awssdk.services.codeartifact.DefaultCodeartifactClient Maven / Gradle / Ivy
Show all versions of codeartifact Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codeartifact;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.sync.ResponseTransformer;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.codeartifact.model.AccessDeniedException;
import software.amazon.awssdk.services.codeartifact.model.AssociateExternalConnectionRequest;
import software.amazon.awssdk.services.codeartifact.model.AssociateExternalConnectionResponse;
import software.amazon.awssdk.services.codeartifact.model.CodeartifactException;
import software.amazon.awssdk.services.codeartifact.model.CodeartifactRequest;
import software.amazon.awssdk.services.codeartifact.model.ConflictException;
import software.amazon.awssdk.services.codeartifact.model.CopyPackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.CopyPackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.CreateDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.CreateDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.CreateRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.CreateRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribeDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribeDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageVersionRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageVersionResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribeRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribeRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DisassociateExternalConnectionRequest;
import software.amazon.awssdk.services.codeartifact.model.DisassociateExternalConnectionResponse;
import software.amazon.awssdk.services.codeartifact.model.DisposePackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.DisposePackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.GetAuthorizationTokenRequest;
import software.amazon.awssdk.services.codeartifact.model.GetAuthorizationTokenResponse;
import software.amazon.awssdk.services.codeartifact.model.GetDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.GetDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetRequest;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetResponse;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionReadmeRequest;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionReadmeResponse;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryEndpointRequest;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryEndpointResponse;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.InternalServerException;
import software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListDomainsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionDependenciesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionDependenciesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackagesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.PutDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.PutDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.PutRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.PutRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.ResourceNotFoundException;
import software.amazon.awssdk.services.codeartifact.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.codeartifact.model.TagResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.TagResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.ThrottlingException;
import software.amazon.awssdk.services.codeartifact.model.UntagResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.UntagResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageVersionsStatusRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageVersionsStatusResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdateRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdateRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.ValidationException;
import software.amazon.awssdk.services.codeartifact.paginators.ListDomainsIterable;
import software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsIterable;
import software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsIterable;
import software.amazon.awssdk.services.codeartifact.paginators.ListPackagesIterable;
import software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainIterable;
import software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesIterable;
import software.amazon.awssdk.services.codeartifact.transform.AssociateExternalConnectionRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.CopyPackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.CreateDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.CreateRepositoryRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteDomainPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeletePackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteRepositoryPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteRepositoryRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DescribeDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DescribePackageVersionRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DescribeRepositoryRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DisassociateExternalConnectionRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DisposePackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetAuthorizationTokenRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetDomainPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetPackageVersionAssetRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetPackageVersionReadmeRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetRepositoryEndpointRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetRepositoryPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListDomainsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackageVersionAssetsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackageVersionDependenciesRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackagesRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListRepositoriesInDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListRepositoriesRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.PutDomainPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.PutRepositoryPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.UpdatePackageVersionsStatusRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.UpdateRepositoryRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link CodeartifactClient}.
*
* @see CodeartifactClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCodeartifactClient implements CodeartifactClient {
private static final Logger log = Logger.loggerFor(DefaultCodeartifactClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCodeartifactClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Adds an existing external connection to a repository. One external connection is allowed per repository.
*
*
*
* A repository can have one or more upstream repositories, or an external connection.
*
*
*
* @param associateExternalConnectionRequest
* @return Result of the AssociateExternalConnection operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.AssociateExternalConnection
* @see AWS API Documentation
*/
@Override
public AssociateExternalConnectionResponse associateExternalConnection(
AssociateExternalConnectionRequest associateExternalConnectionRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ResourceNotFoundException, ServiceQuotaExceededException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateExternalConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateExternalConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateExternalConnection");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateExternalConnection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateExternalConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateExternalConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Copies package versions from one repository to another repository in the same domain.
*
*
*
* You must specify versions
or versionRevisions
. You cannot specify both.
*
*
*
* @param copyPackageVersionsRequest
* @return Result of the CopyPackageVersions operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.CopyPackageVersions
* @see AWS API Documentation
*/
@Override
public CopyPackageVersionsResponse copyPackageVersions(CopyPackageVersionsRequest copyPackageVersionsRequest)
throws AccessDeniedException, ConflictException, InternalServerException, ResourceNotFoundException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, AwsServiceException, SdkClientException,
CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CopyPackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, copyPackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CopyPackageVersions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CopyPackageVersions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(copyPackageVersionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CopyPackageVersionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a domain. CodeArtifact domains make it easier to manage multiple repositories across an
* organization. You can use a domain to apply permissions across many repositories owned by different AWS accounts.
* An asset is stored only once in a domain, even if it's in multiple repositories.
*
*
* Although you can have multiple domains, we recommend a single production domain that contains all published
* artifacts so that your development teams can find and share packages. You can use a second pre-production domain
* to test changes to the production domain configuration.
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.CreateDomain
* @see AWS API
* Documentation
*/
@Override
public CreateDomainResponse createDomain(CreateDomainRequest createDomainRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ResourceNotFoundException, ServiceQuotaExceededException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDomain");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateDomain").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createDomainRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateDomainRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a repository.
*
*
* @param createRepositoryRequest
* @return Result of the CreateRepository operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.CreateRepository
* @see AWS
* API Documentation
*/
@Override
public CreateRepositoryResponse createRepository(CreateRepositoryRequest createRepositoryRequest)
throws AccessDeniedException, ConflictException, InternalServerException, ResourceNotFoundException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, AwsServiceException, SdkClientException,
CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRepository");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateRepository").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createRepositoryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateRepositoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a domain. You cannot delete a domain that contains repositories. If you want to delete a domain with
* repositories, first delete its repositories.
*
*
* @param deleteDomainRequest
* @return Result of the DeleteDomain operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DeleteDomain
* @see AWS API
* Documentation
*/
@Override
public DeleteDomainResponse deleteDomain(DeleteDomainRequest deleteDomainRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDomain");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteDomain").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteDomainRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteDomainRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the resource policy set on a domain.
*
*
* @param deleteDomainPermissionsPolicyRequest
* @return Result of the DeleteDomainPermissionsPolicy operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DeleteDomainPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public DeleteDomainPermissionsPolicyResponse deleteDomainPermissionsPolicy(
DeleteDomainPermissionsPolicyRequest deleteDomainPermissionsPolicyRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException,
AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDomainPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteDomainPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDomainPermissionsPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDomainPermissionsPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteDomainPermissionsPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteDomainPermissionsPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes one or more versions of a package. A deleted package version cannot be restored in your repository. If
* you want to remove a package version from your repository and be able to restore it later, set its status to
* Archived
. Archived packages cannot be downloaded from a repository and don't show up with list
* package APIs (for example, ListackageVersions), but you can restore them using UpdatePackageVersionsStatus.
*
*
* @param deletePackageVersionsRequest
* @return Result of the DeletePackageVersions operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DeletePackageVersions
* @see AWS API Documentation
*/
@Override
public DeletePackageVersionsResponse deletePackageVersions(DeletePackageVersionsRequest deletePackageVersionsRequest)
throws AccessDeniedException, ConflictException, InternalServerException, ResourceNotFoundException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeletePackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePackageVersions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeletePackageVersions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deletePackageVersionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeletePackageVersionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a repository.
*
*
* @param deleteRepositoryRequest
* @return Result of the DeleteRepository operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DeleteRepository
* @see AWS
* API Documentation
*/
@Override
public DeleteRepositoryResponse deleteRepository(DeleteRepositoryRequest deleteRepositoryRequest)
throws AccessDeniedException, ConflictException, InternalServerException, ResourceNotFoundException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRepository");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteRepository").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteRepositoryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteRepositoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the resource policy that is set on a repository. After a resource policy is deleted, the permissions
* allowed and denied by the deleted policy are removed. The effect of deleting a resource policy might not be
* immediate.
*
*
*
* Use DeleteRepositoryPermissionsPolicy
with caution. After a policy is deleted, AWS users, roles, and
* accounts lose permissions to perform the repository actions granted by the deleted policy.
*
*
*
* @param deleteRepositoryPermissionsPolicyRequest
* @return Result of the DeleteRepositoryPermissionsPolicy operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DeleteRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public DeleteRepositoryPermissionsPolicyResponse deleteRepositoryPermissionsPolicy(
DeleteRepositoryPermissionsPolicyRequest deleteRepositoryPermissionsPolicyRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException,
AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteRepositoryPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteRepositoryPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRepositoryPermissionsPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRepositoryPermissionsPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteRepositoryPermissionsPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteRepositoryPermissionsPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a
* DomainDescription object that contains information about the requested domain.
*
*
* @param describeDomainRequest
* @return Result of the DescribeDomain operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DescribeDomain
* @see AWS
* API Documentation
*/
@Override
public DescribeDomainResponse describeDomain(DescribeDomainRequest describeDomainRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomain");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeDomain").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeDomainRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeDomainRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a PackageVersionDescription object that contains information about the requested package version.
*
*
* @param describePackageVersionRequest
* @return Result of the DescribePackageVersion operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DescribePackageVersion
* @see AWS API Documentation
*/
@Override
public DescribePackageVersionResponse describePackageVersion(DescribePackageVersionRequest describePackageVersionRequest)
throws AccessDeniedException, ConflictException, InternalServerException, ResourceNotFoundException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePackageVersionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePackageVersionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePackageVersion");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePackageVersion").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describePackageVersionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribePackageVersionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a RepositoryDescription
object that contains detailed information about the requested
* repository.
*
*
* @param describeRepositoryRequest
* @return Result of the DescribeRepository operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DescribeRepository
* @see AWS API Documentation
*/
@Override
public DescribeRepositoryResponse describeRepository(DescribeRepositoryRequest describeRepositoryRequest)
throws AccessDeniedException, InternalServerException, ResourceNotFoundException, ThrottlingException,
ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRepository");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeRepository").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeRepositoryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeRepositoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes an existing external connection from a repository.
*
*
* @param disassociateExternalConnectionRequest
* @return Result of the DisassociateExternalConnection operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DisassociateExternalConnection
* @see AWS API Documentation
*/
@Override
public DisassociateExternalConnectionResponse disassociateExternalConnection(
DisassociateExternalConnectionRequest disassociateExternalConnectionRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ResourceNotFoundException, ServiceQuotaExceededException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateExternalConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateExternalConnectionRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateExternalConnection");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateExternalConnection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateExternalConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateExternalConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the assets in package versions and sets the package versions' status to Disposed
. A disposed
* package version cannot be restored in your repository because its assets are deleted.
*
*
* To view all disposed package versions in a repository, use ListPackageVersions and set the status parameter to Disposed
.
*
*
* To view information about a disposed package version, use DescribePackageVersion.
*
*
* @param disposePackageVersionsRequest
* @return Result of the DisposePackageVersions operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.DisposePackageVersions
* @see AWS API Documentation
*/
@Override
public DisposePackageVersionsResponse disposePackageVersions(DisposePackageVersionsRequest disposePackageVersionsRequest)
throws AccessDeniedException, ConflictException, InternalServerException, ResourceNotFoundException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisposePackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disposePackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisposePackageVersions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisposePackageVersions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disposePackageVersionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisposePackageVersionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Generates a temporary authorization token for accessing repositories in the domain. This API requires the
* codeartifact:GetAuthorizationToken
and sts:GetServiceBearerToken
permissions. For more
* information about authorization tokens, see AWS CodeArtifact
* authentication and tokens.
*
*
*
* CodeArtifact authorization tokens are valid for a period of 12 hours when created with the login
* command. You can call login
periodically to refresh the token. When you create an authorization
* token with the GetAuthorizationToken
API, you can set a custom authorization period, up to a maximum
* of 12 hours, with the durationSeconds
parameter.
*
*
* The authorization period begins after login
or GetAuthorizationToken
is called. If
* login
or GetAuthorizationToken
is called while assuming a role, the token lifetime is
* independent of the maximum session duration of the role. For example, if you call sts assume-role
* and specify a session duration of 15 minutes, then generate a CodeArtifact authorization token, the token will be
* valid for the full authorization period even though this is longer than the 15-minute session duration.
*
*
* See Using IAM Roles for more
* information on controlling session duration.
*
*
*
* @param getAuthorizationTokenRequest
* @return Result of the GetAuthorizationToken operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.GetAuthorizationToken
* @see AWS API Documentation
*/
@Override
public GetAuthorizationTokenResponse getAuthorizationToken(GetAuthorizationTokenRequest getAuthorizationTokenRequest)
throws AccessDeniedException, InternalServerException, ResourceNotFoundException, ThrottlingException,
ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAuthorizationTokenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAuthorizationTokenRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAuthorizationToken");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetAuthorizationToken").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAuthorizationTokenRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAuthorizationTokenRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the resource policy attached to the specified domain.
*
*
*
* The policy is a resource-based policy, not an identity-based policy. For more information, see Identity-based
* policies and resource-based policies in the AWS Identity and Access Management User Guide.
*
*
*
* @param getDomainPermissionsPolicyRequest
* @return Result of the GetDomainPermissionsPolicy operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.GetDomainPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public GetDomainPermissionsPolicyResponse getDomainPermissionsPolicy(
GetDomainPermissionsPolicyRequest getDomainPermissionsPolicyRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDomainPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDomainPermissionsPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDomainPermissionsPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDomainPermissionsPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getDomainPermissionsPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDomainPermissionsPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns an asset (or file) that is in a package. For example, for a Maven package version, use
* GetPackageVersionAsset
to download a JAR
file, a POM
file, or any other
* assets in the package version.
*
*
* @param getPackageVersionAssetRequest
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled
* GetPackageVersionAssetResponse and an InputStream to the response content are provided as parameters to
* the callback. The callback may return a transformed type which will be the return value of this method.
* See {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this
* interface and for links to pre-canned implementations for common scenarios like downloading to a file. The
* service documentation for the response content is as follows '
*
* The binary file, or asset, that is downloaded.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.GetPackageVersionAsset
* @see AWS API Documentation
*/
@Override
public ReturnT getPackageVersionAsset(GetPackageVersionAssetRequest getPackageVersionAssetRequest,
ResponseTransformer responseTransformer) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, ConflictException,
AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(true)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetPackageVersionAssetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPackageVersionAssetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPackageVersionAsset");
return clientHandler.execute(
new ClientExecutionParams()
.withOperationName("GetPackageVersionAsset").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getPackageVersionAssetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetPackageVersionAssetRequestMarshaller(protocolFactory)), responseTransformer);
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the readme file or descriptive text for a package version. For packages that do not contain a readme file,
* CodeArtifact extracts a description from a metadata file. For example, from the <description>
* element in the pom.xml
file of a Maven package.
*
*
* The returned text might contain formatting. For example, it might contain formatting for Markdown or
* reStructuredText.
*
*
* @param getPackageVersionReadmeRequest
* @return Result of the GetPackageVersionReadme operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.GetPackageVersionReadme
* @see AWS API Documentation
*/
@Override
public GetPackageVersionReadmeResponse getPackageVersionReadme(GetPackageVersionReadmeRequest getPackageVersionReadmeRequest)
throws AccessDeniedException, InternalServerException, ResourceNotFoundException, ThrottlingException,
ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetPackageVersionReadmeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPackageVersionReadmeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPackageVersionReadme");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetPackageVersionReadme").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getPackageVersionReadmeRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetPackageVersionReadmeRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the endpoint of a repository for a specific package format. A repository has one endpoint for each
* package format:
*
*
* -
*
* npm
*
*
* -
*
* pypi
*
*
* -
*
* maven
*
*
*
*
* @param getRepositoryEndpointRequest
* @return Result of the GetRepositoryEndpoint operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.GetRepositoryEndpoint
* @see AWS API Documentation
*/
@Override
public GetRepositoryEndpointResponse getRepositoryEndpoint(GetRepositoryEndpointRequest getRepositoryEndpointRequest)
throws AccessDeniedException, InternalServerException, ResourceNotFoundException, ThrottlingException,
ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRepositoryEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRepositoryEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRepositoryEndpoint");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetRepositoryEndpoint").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getRepositoryEndpointRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetRepositoryEndpointRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the resource policy that is set on a repository.
*
*
* @param getRepositoryPermissionsPolicyRequest
* @return Result of the GetRepositoryPermissionsPolicy operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.GetRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public GetRepositoryPermissionsPolicyResponse getRepositoryPermissionsPolicy(
GetRepositoryPermissionsPolicyRequest getRepositoryPermissionsPolicyRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRepositoryPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getRepositoryPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRepositoryPermissionsPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRepositoryPermissionsPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getRepositoryPermissionsPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetRepositoryPermissionsPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of DomainSummary objects for all domains owned by the AWS account that makes this call. Each returned
* DomainSummary
object contains information about a domain.
*
*
* @param listDomainsRequest
* @return Result of the ListDomains operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListDomains
* @see AWS API
* Documentation
*/
@Override
public ListDomainsResponse listDomains(ListDomainsRequest listDomainsRequest) throws AccessDeniedException,
InternalServerException, ThrottlingException, ValidationException, AwsServiceException, SdkClientException,
CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListDomainsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDomainsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDomains");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListDomains").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listDomainsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDomainsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of DomainSummary objects for all domains owned by the AWS account that makes this call. Each returned
* DomainSummary
object contains information about a domain.
*
*
*
* This is a variant of {@link #listDomains(software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListDomainsIterable responses = client.listDomainsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codeartifact.paginators.ListDomainsIterable responses = client.listDomainsPaginator(request);
* for (software.amazon.awssdk.services.codeartifact.model.ListDomainsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListDomainsIterable responses = client.listDomainsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDomains(software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest)} operation.
*
*
* @param listDomainsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListDomains
* @see AWS API
* Documentation
*/
@Override
public ListDomainsIterable listDomainsPaginator(ListDomainsRequest listDomainsRequest) throws AccessDeniedException,
InternalServerException, ThrottlingException, ValidationException, AwsServiceException, SdkClientException,
CodeartifactException {
return new ListDomainsIterable(this, applyPaginatorUserAgent(listDomainsRequest));
}
/**
*
* Returns a list of AssetSummary
* objects for assets in a package version.
*
*
* @param listPackageVersionAssetsRequest
* @return Result of the ListPackageVersionAssets operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListPackageVersionAssets
* @see AWS API Documentation
*/
@Override
public ListPackageVersionAssetsResponse listPackageVersionAssets(
ListPackageVersionAssetsRequest listPackageVersionAssetsRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPackageVersionAssetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPackageVersionAssetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackageVersionAssets");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPackageVersionAssets").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listPackageVersionAssetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListPackageVersionAssetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of AssetSummary
* objects for assets in a package version.
*
*
*
* This is a variant of
* {@link #listPackageVersionAssets(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsIterable responses = client.listPackageVersionAssetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsIterable responses = client
* .listPackageVersionAssetsPaginator(request);
* for (software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsIterable responses = client.listPackageVersionAssetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageVersionAssets(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest)}
* operation.
*
*
* @param listPackageVersionAssetsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListPackageVersionAssets
* @see AWS API Documentation
*/
@Override
public ListPackageVersionAssetsIterable listPackageVersionAssetsPaginator(
ListPackageVersionAssetsRequest listPackageVersionAssetsRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
return new ListPackageVersionAssetsIterable(this, applyPaginatorUserAgent(listPackageVersionAssetsRequest));
}
/**
*
* Returns the direct dependencies for a package version. The dependencies are returned as PackageDependency objects. CodeArtifact extracts the dependencies for a package version from the metadata
* file for the package format (for example, the package.json
file for npm packages and the
* pom.xml
file for Maven). Any package version dependencies that are not listed in the configuration
* file are not returned.
*
*
* @param listPackageVersionDependenciesRequest
* @return Result of the ListPackageVersionDependencies operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListPackageVersionDependencies
* @see AWS API Documentation
*/
@Override
public ListPackageVersionDependenciesResponse listPackageVersionDependencies(
ListPackageVersionDependenciesRequest listPackageVersionDependenciesRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPackageVersionDependenciesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listPackageVersionDependenciesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackageVersionDependencies");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPackageVersionDependencies").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listPackageVersionDependenciesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListPackageVersionDependenciesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of PackageVersionSummary objects for package versions in a repository that match the request parameters.
*
*
* @param listPackageVersionsRequest
* @return Result of the ListPackageVersions operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListPackageVersions
* @see AWS API Documentation
*/
@Override
public ListPackageVersionsResponse listPackageVersions(ListPackageVersionsRequest listPackageVersionsRequest)
throws AccessDeniedException, InternalServerException, ResourceNotFoundException, ThrottlingException,
ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackageVersions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListPackageVersions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listPackageVersionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListPackageVersionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of PackageVersionSummary objects for package versions in a repository that match the request parameters.
*
*
*
* This is a variant of
* {@link #listPackageVersions(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsIterable responses = client.listPackageVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsIterable responses = client
* .listPackageVersionsPaginator(request);
* for (software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsIterable responses = client.listPackageVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageVersions(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest)}
* operation.
*
*
* @param listPackageVersionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListPackageVersions
* @see AWS API Documentation
*/
@Override
public ListPackageVersionsIterable listPackageVersionsPaginator(ListPackageVersionsRequest listPackageVersionsRequest)
throws AccessDeniedException, InternalServerException, ResourceNotFoundException, ThrottlingException,
ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
return new ListPackageVersionsIterable(this, applyPaginatorUserAgent(listPackageVersionsRequest));
}
/**
*
* Returns a list of PackageSummary
* objects for packages in a repository that match the request parameters.
*
*
* @param listPackagesRequest
* @return Result of the ListPackages operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListPackages
* @see AWS API
* Documentation
*/
@Override
public ListPackagesResponse listPackages(ListPackagesRequest listPackagesRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListPackagesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPackagesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackages");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListPackages").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listPackagesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListPackagesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of PackageSummary
* objects for packages in a repository that match the request parameters.
*
*
*
* This is a variant of
* {@link #listPackages(software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackagesIterable responses = client.listPackagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackagesIterable responses = client
* .listPackagesPaginator(request);
* for (software.amazon.awssdk.services.codeartifact.model.ListPackagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackagesIterable responses = client.listPackagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackages(software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest)} operation.
*
*
* @param listPackagesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListPackages
* @see AWS API
* Documentation
*/
@Override
public ListPackagesIterable listPackagesPaginator(ListPackagesRequest listPackagesRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
return new ListPackagesIterable(this, applyPaginatorUserAgent(listPackagesRequest));
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified AWS account and that matches the input parameters.
*
*
* @param listRepositoriesRequest
* @return Result of the ListRepositories operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListRepositories
* @see AWS
* API Documentation
*/
@Override
public ListRepositoriesResponse listRepositories(ListRepositoriesRequest listRepositoriesRequest)
throws AccessDeniedException, InternalServerException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListRepositoriesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRepositoriesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRepositories");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListRepositories").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listRepositoriesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListRepositoriesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified AWS account and that matches the input parameters.
*
*
*
* This is a variant of
* {@link #listRepositories(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesIterable responses = client.listRepositoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesIterable responses = client
* .listRepositoriesPaginator(request);
* for (software.amazon.awssdk.services.codeartifact.model.ListRepositoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesIterable responses = client.listRepositoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositories(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest)}
* operation.
*
*
* @param listRepositoriesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListRepositories
* @see AWS
* API Documentation
*/
@Override
public ListRepositoriesIterable listRepositoriesPaginator(ListRepositoriesRequest listRepositoriesRequest)
throws AccessDeniedException, InternalServerException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
return new ListRepositoriesIterable(this, applyPaginatorUserAgent(listRepositoriesRequest));
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified domain and that matches the input parameters.
*
*
* @param listRepositoriesInDomainRequest
* @return Result of the ListRepositoriesInDomain operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListRepositoriesInDomain
* @see AWS API Documentation
*/
@Override
public ListRepositoriesInDomainResponse listRepositoriesInDomain(
ListRepositoriesInDomainRequest listRepositoriesInDomainRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListRepositoriesInDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRepositoriesInDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRepositoriesInDomain");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListRepositoriesInDomain").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listRepositoriesInDomainRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListRepositoriesInDomainRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified domain and that matches the input parameters.
*
*
*
* This is a variant of
* {@link #listRepositoriesInDomain(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainIterable responses = client.listRepositoriesInDomainPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainIterable responses = client
* .listRepositoriesInDomainPaginator(request);
* for (software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainIterable responses = client.listRepositoriesInDomainPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositoriesInDomain(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest)}
* operation.
*
*
* @param listRepositoriesInDomainRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListRepositoriesInDomain
* @see AWS API Documentation
*/
@Override
public ListRepositoriesInDomainIterable listRepositoriesInDomainPaginator(
ListRepositoriesInDomainRequest listRepositoriesInDomainRequest) throws AccessDeniedException,
InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException,
SdkClientException, CodeartifactException {
return new ListRepositoriesInDomainIterable(this, applyPaginatorUserAgent(listRepositoriesInDomainRequest));
}
/**
*
* Gets information about AWS tags for a specified Amazon Resource Name (ARN) in AWS CodeArtifact.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws AccessDeniedException, ResourceNotFoundException, ThrottlingException, ValidationException,
AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sets a resource policy on a domain that specifies permissions to access it.
*
*
* When you call PutDomainPermissionsPolicy
, the resource policy on the domain is ignored when
* evaluting permissions. This ensures that the owner of a domain cannot lock themselves out of the domain, which
* would prevent them from being able to update the resource policy.
*
*
* @param putDomainPermissionsPolicyRequest
* @return Result of the PutDomainPermissionsPolicy operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.PutDomainPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public PutDomainPermissionsPolicyResponse putDomainPermissionsPolicy(
PutDomainPermissionsPolicyRequest putDomainPermissionsPolicyRequest) throws AccessDeniedException, ConflictException,
InternalServerException, ResourceNotFoundException, ServiceQuotaExceededException, ThrottlingException,
ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutDomainPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putDomainPermissionsPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutDomainPermissionsPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutDomainPermissionsPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putDomainPermissionsPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutDomainPermissionsPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sets the resource policy on a repository that specifies permissions to access it.
*
*
* When you call PutRepositoryPermissionsPolicy
, the resource policy on the repository is ignored when
* evaluting permissions. This ensures that the owner of a repository cannot lock themselves out of the repository,
* which would prevent them from being able to update the resource policy.
*
*
* @param putRepositoryPermissionsPolicyRequest
* @return Result of the PutRepositoryPermissionsPolicy operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.PutRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public PutRepositoryPermissionsPolicyResponse putRepositoryPermissionsPolicy(
PutRepositoryPermissionsPolicyRequest putRepositoryPermissionsPolicyRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ResourceNotFoundException, ServiceQuotaExceededException,
ThrottlingException, ValidationException, AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutRepositoryPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putRepositoryPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutRepositoryPermissionsPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutRepositoryPermissionsPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putRepositoryPermissionsPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutRepositoryPermissionsPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds or updates tags for a resource in AWS CodeArtifact.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws AccessDeniedException,
ResourceNotFoundException, ServiceQuotaExceededException, ThrottlingException, ValidationException,
AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes tags from a resource in AWS CodeArtifact.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws AccessDeniedException,
ResourceNotFoundException, ThrottlingException, ValidationException, AwsServiceException, SdkClientException,
CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the status of one or more versions of a package.
*
*
* @param updatePackageVersionsStatusRequest
* @return Result of the UpdatePackageVersionsStatus operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.UpdatePackageVersionsStatus
* @see AWS API Documentation
*/
@Override
public UpdatePackageVersionsStatusResponse updatePackageVersionsStatus(
UpdatePackageVersionsStatusRequest updatePackageVersionsStatusRequest) throws AccessDeniedException,
ConflictException, InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException,
AwsServiceException, SdkClientException, CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdatePackageVersionsStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updatePackageVersionsStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdatePackageVersionsStatus");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdatePackageVersionsStatus").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updatePackageVersionsStatusRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdatePackageVersionsStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Update the properties of a repository.
*
*
* @param updateRepositoryRequest
* @return Result of the UpdateRepository operation returned by the service.
* @throws AccessDeniedException
* The operation did not succeed because of an unauthorized access attempt.
* @throws ConflictException
* The operation did not succeed because prerequisites are not met.
* @throws InternalServerException
* The operation did not succeed because of an error that occurred inside AWS CodeArtifact.
* @throws ResourceNotFoundException
* The operation did not succeed because the resource requested is not found in the service.
* @throws ServiceQuotaExceededException
* The operation did not succeed because it would have exceeded a service limit for your account.
* @throws ThrottlingException
* The operation did not succeed because too many requests are sent to the service.
* @throws ValidationException
* The operation did not succeed because a parameter in the request was sent with an invalid value.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeartifactException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeartifactClient.UpdateRepository
* @see AWS
* API Documentation
*/
@Override
public UpdateRepositoryResponse updateRepository(UpdateRepositoryRequest updateRepositoryRequest)
throws AccessDeniedException, ConflictException, InternalServerException, ResourceNotFoundException,
ServiceQuotaExceededException, ThrottlingException, ValidationException, AwsServiceException, SdkClientException,
CodeartifactException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateRepository");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateRepository").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateRepositoryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateRepositoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(CodeartifactException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}