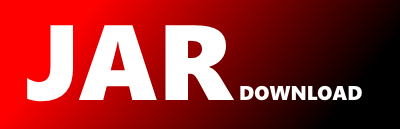
software.amazon.awssdk.services.codeartifact.DefaultCodeartifactAsyncClient Maven / Gradle / Ivy
Show all versions of codeartifact Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codeartifact;
import static software.amazon.awssdk.utils.FunctionalUtils.runAndLogError;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.core.async.AsyncResponseTransformerUtils;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.runtime.transform.AsyncStreamingRequestMarshaller;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.codeartifact.internal.CodeartifactServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.codeartifact.model.AccessDeniedException;
import software.amazon.awssdk.services.codeartifact.model.AssociateExternalConnectionRequest;
import software.amazon.awssdk.services.codeartifact.model.AssociateExternalConnectionResponse;
import software.amazon.awssdk.services.codeartifact.model.CodeartifactException;
import software.amazon.awssdk.services.codeartifact.model.ConflictException;
import software.amazon.awssdk.services.codeartifact.model.CopyPackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.CopyPackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.CreateDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.CreateDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.CreateRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.CreateRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageRequest;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageResponse;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribeDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribeDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageVersionRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageVersionResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribeRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribeRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DisassociateExternalConnectionRequest;
import software.amazon.awssdk.services.codeartifact.model.DisassociateExternalConnectionResponse;
import software.amazon.awssdk.services.codeartifact.model.DisposePackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.DisposePackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.GetAuthorizationTokenRequest;
import software.amazon.awssdk.services.codeartifact.model.GetAuthorizationTokenResponse;
import software.amazon.awssdk.services.codeartifact.model.GetDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.GetDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetRequest;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetResponse;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionReadmeRequest;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionReadmeResponse;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryEndpointRequest;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryEndpointResponse;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.InternalServerException;
import software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListDomainsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionDependenciesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionDependenciesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackagesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.PublishPackageVersionRequest;
import software.amazon.awssdk.services.codeartifact.model.PublishPackageVersionResponse;
import software.amazon.awssdk.services.codeartifact.model.PutDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.PutDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.PutPackageOriginConfigurationRequest;
import software.amazon.awssdk.services.codeartifact.model.PutPackageOriginConfigurationResponse;
import software.amazon.awssdk.services.codeartifact.model.PutRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.PutRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.ResourceNotFoundException;
import software.amazon.awssdk.services.codeartifact.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.codeartifact.model.TagResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.TagResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.ThrottlingException;
import software.amazon.awssdk.services.codeartifact.model.UntagResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.UntagResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageVersionsStatusRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageVersionsStatusResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdateRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdateRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.ValidationException;
import software.amazon.awssdk.services.codeartifact.transform.AssociateExternalConnectionRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.CopyPackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.CreateDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.CreateRepositoryRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteDomainPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeletePackageRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeletePackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteRepositoryPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DeleteRepositoryRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DescribeDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DescribePackageRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DescribePackageVersionRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DescribeRepositoryRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DisassociateExternalConnectionRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.DisposePackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetAuthorizationTokenRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetDomainPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetPackageVersionAssetRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetPackageVersionReadmeRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetRepositoryEndpointRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.GetRepositoryPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListDomainsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackageVersionAssetsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackageVersionDependenciesRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackageVersionsRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListPackagesRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListRepositoriesInDomainRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListRepositoriesRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.PublishPackageVersionRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.PutDomainPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.PutPackageOriginConfigurationRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.PutRepositoryPermissionsPolicyRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.UpdatePackageVersionsStatusRequestMarshaller;
import software.amazon.awssdk.services.codeartifact.transform.UpdateRepositoryRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
import software.amazon.awssdk.utils.Pair;
/**
* Internal implementation of {@link CodeartifactAsyncClient}.
*
* @see CodeartifactAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCodeartifactAsyncClient implements CodeartifactAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultCodeartifactAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCodeartifactAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Adds an existing external connection to a repository. One external connection is allowed per repository.
*
*
*
* A repository can have one or more upstream repositories, or an external connection.
*
*
*
* @param associateExternalConnectionRequest
* @return A Java Future containing the result of the AssociateExternalConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.AssociateExternalConnection
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateExternalConnection(
AssociateExternalConnectionRequest associateExternalConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateExternalConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateExternalConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateExternalConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateExternalConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateExternalConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateExternalConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateExternalConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Copies package versions from one repository to another repository in the same domain.
*
*
*
* You must specify versions
or versionRevisions
. You cannot specify both.
*
*
*
* @param copyPackageVersionsRequest
* @return A Java Future containing the result of the CopyPackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CopyPackageVersions
* @see AWS API Documentation
*/
@Override
public CompletableFuture copyPackageVersions(
CopyPackageVersionsRequest copyPackageVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(copyPackageVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, copyPackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CopyPackageVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CopyPackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CopyPackageVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CopyPackageVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(copyPackageVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a domain. CodeArtifact domains make it easier to manage multiple repositories across an
* organization. You can use a domain to apply permissions across many repositories owned by different Amazon Web
* Services accounts. An asset is stored only once in a domain, even if it's in multiple repositories.
*
*
* Although you can have multiple domains, we recommend a single production domain that contains all published
* artifacts so that your development teams can find and share packages. You can use a second pre-production domain
* to test changes to the production domain configuration.
*
*
* @param createDomainRequest
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreateDomain
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createDomain(CreateDomainRequest createDomainRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDomainRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDomain");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDomain").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDomainRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDomainRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a repository.
*
*
* @param createRepositoryRequest
* @return A Java Future containing the result of the CreateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreateRepository
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createRepository(CreateRepositoryRequest createRepositoryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createRepositoryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRepository");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateRepository").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateRepositoryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createRepositoryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a domain. You cannot delete a domain that contains repositories. If you want to delete a domain with
* repositories, first delete its repositories.
*
*
* @param deleteDomainRequest
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteDomain
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteDomain(DeleteDomainRequest deleteDomainRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDomainRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDomain");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDomain").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteDomainRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteDomainRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the resource policy set on a domain.
*
*
* @param deleteDomainPermissionsPolicyRequest
* @return A Java Future containing the result of the DeleteDomainPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteDomainPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteDomainPermissionsPolicy(
DeleteDomainPermissionsPolicyRequest deleteDomainPermissionsPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDomainPermissionsPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteDomainPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDomainPermissionsPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDomainPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDomainPermissionsPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteDomainPermissionsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteDomainPermissionsPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a package and all associated package versions. A deleted package cannot be restored. To delete one or
* more package versions, use the DeletePackageVersions API.
*
*
* @param deletePackageRequest
* @return A Java Future containing the result of the DeletePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackage
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deletePackage(DeletePackageRequest deletePackageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePackageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePackageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePackage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeletePackageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePackage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeletePackageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deletePackageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes one or more versions of a package. A deleted package version cannot be restored in your repository. If
* you want to remove a package version from your repository and be able to restore it later, set its status to
* Archived
. Archived packages cannot be downloaded from a repository and don't show up with list
* package APIs (for example, ListPackageVersions), but you can restore them using UpdatePackageVersionsStatus.
*
*
* @param deletePackageVersionsRequest
* @return A Java Future containing the result of the DeletePackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackageVersions
* @see AWS API Documentation
*/
@Override
public CompletableFuture deletePackageVersions(
DeletePackageVersionsRequest deletePackageVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePackageVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePackageVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeletePackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePackageVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeletePackageVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deletePackageVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a repository.
*
*
* @param deleteRepositoryRequest
* @return A Java Future containing the result of the DeleteRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteRepository
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteRepository(DeleteRepositoryRequest deleteRepositoryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteRepositoryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRepository");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRepository").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteRepositoryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRepositoryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the resource policy that is set on a repository. After a resource policy is deleted, the permissions
* allowed and denied by the deleted policy are removed. The effect of deleting a resource policy might not be
* immediate.
*
*
*
* Use DeleteRepositoryPermissionsPolicy
with caution. After a policy is deleted, Amazon Web Services
* users, roles, and accounts lose permissions to perform the repository actions granted by the deleted policy.
*
*
*
* @param deleteRepositoryPermissionsPolicyRequest
* @return A Java Future containing the result of the DeleteRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteRepositoryPermissionsPolicy(
DeleteRepositoryPermissionsPolicyRequest deleteRepositoryPermissionsPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteRepositoryPermissionsPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteRepositoryPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRepositoryPermissionsPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DeleteRepositoryPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRepositoryPermissionsPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteRepositoryPermissionsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRepositoryPermissionsPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a
* DomainDescription object that contains information about the requested domain.
*
*
* @param describeDomainRequest
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribeDomain
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeDomain(DescribeDomainRequest describeDomainRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomain");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomain").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a
* PackageDescription object that contains information about the requested package.
*
*
* @param describePackageRequest
* @return A Java Future containing the result of the DescribePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackage
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describePackage(DescribePackageRequest describePackageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describePackageRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePackageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePackage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePackageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePackage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribePackageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describePackageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a PackageVersionDescription object that contains information about the requested package version.
*
*
* @param describePackageVersionRequest
* @return A Java Future containing the result of the DescribePackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackageVersion
* @see AWS API Documentation
*/
@Override
public CompletableFuture describePackageVersion(
DescribePackageVersionRequest describePackageVersionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describePackageVersionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePackageVersionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePackageVersion");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePackageVersionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePackageVersion").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribePackageVersionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describePackageVersionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a RepositoryDescription
object that contains detailed information about the requested
* repository.
*
*
* @param describeRepositoryRequest
* @return A Java Future containing the result of the DescribeRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribeRepository
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeRepository(DescribeRepositoryRequest describeRepositoryRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeRepositoryRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRepository");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRepository").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeRepositoryRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeRepositoryRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes an existing external connection from a repository.
*
*
* @param disassociateExternalConnectionRequest
* @return A Java Future containing the result of the DisassociateExternalConnection operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DisassociateExternalConnection
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateExternalConnection(
DisassociateExternalConnectionRequest disassociateExternalConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateExternalConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateExternalConnectionRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateExternalConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateExternalConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateExternalConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateExternalConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateExternalConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the assets in package versions and sets the package versions' status to Disposed
. A disposed
* package version cannot be restored in your repository because its assets are deleted.
*
*
* To view all disposed package versions in a repository, use ListPackageVersions and set the status parameter to Disposed
.
*
*
* To view information about a disposed package version, use DescribePackageVersion.
*
*
* @param disposePackageVersionsRequest
* @return A Java Future containing the result of the DisposePackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DisposePackageVersions
* @see AWS API Documentation
*/
@Override
public CompletableFuture disposePackageVersions(
DisposePackageVersionsRequest disposePackageVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disposePackageVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disposePackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisposePackageVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisposePackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisposePackageVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisposePackageVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disposePackageVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Generates a temporary authorization token for accessing repositories in the domain. This API requires the
* codeartifact:GetAuthorizationToken
and sts:GetServiceBearerToken
permissions. For more
* information about authorization tokens, see CodeArtifact authentication
* and tokens.
*
*
*
* CodeArtifact authorization tokens are valid for a period of 12 hours when created with the login
* command. You can call login
periodically to refresh the token. When you create an authorization
* token with the GetAuthorizationToken
API, you can set a custom authorization period, up to a maximum
* of 12 hours, with the durationSeconds
parameter.
*
*
* The authorization period begins after login
or GetAuthorizationToken
is called. If
* login
or GetAuthorizationToken
is called while assuming a role, the token lifetime is
* independent of the maximum session duration of the role. For example, if you call sts assume-role
* and specify a session duration of 15 minutes, then generate a CodeArtifact authorization token, the token will be
* valid for the full authorization period even though this is longer than the 15-minute session duration.
*
*
* See Using IAM Roles for more
* information on controlling session duration.
*
*
*
* @param getAuthorizationTokenRequest
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetAuthorizationToken
* @see AWS API Documentation
*/
@Override
public CompletableFuture getAuthorizationToken(
GetAuthorizationTokenRequest getAuthorizationTokenRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAuthorizationTokenRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAuthorizationTokenRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAuthorizationToken");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAuthorizationTokenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAuthorizationToken").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAuthorizationTokenRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAuthorizationTokenRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the resource policy attached to the specified domain.
*
*
*
* The policy is a resource-based policy, not an identity-based policy. For more information, see Identity-based
* policies and resource-based policies in the IAM User Guide.
*
*
*
* @param getDomainPermissionsPolicyRequest
* @return A Java Future containing the result of the GetDomainPermissionsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetDomainPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture getDomainPermissionsPolicy(
GetDomainPermissionsPolicyRequest getDomainPermissionsPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDomainPermissionsPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDomainPermissionsPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDomainPermissionsPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDomainPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDomainPermissionsPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDomainPermissionsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDomainPermissionsPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an asset (or file) that is in a package. For example, for a Maven package version, use
* GetPackageVersionAsset
to download a JAR
file, a POM
file, or any other
* assets in the package version.
*
*
* @param getPackageVersionAssetRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The binary file, or asset, that is downloaded.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionAsset
* @see AWS API Documentation
*/
@Override
public CompletableFuture getPackageVersionAsset(
GetPackageVersionAssetRequest getPackageVersionAssetRequest,
AsyncResponseTransformer asyncResponseTransformer) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getPackageVersionAssetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPackageVersionAssetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPackageVersionAsset");
Pair, CompletableFuture> pair = AsyncResponseTransformerUtils
.wrapWithEndOfStreamFuture(asyncResponseTransformer);
asyncResponseTransformer = pair.left();
CompletableFuture endOfStreamFuture = pair.right();
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(true)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetPackageVersionAssetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler.execute(
new ClientExecutionParams()
.withOperationName("GetPackageVersionAsset").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetPackageVersionAssetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getPackageVersionAssetRequest), asyncResponseTransformer);
AsyncResponseTransformer finalAsyncResponseTransformer = asyncResponseTransformer;
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
if (e != null) {
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> finalAsyncResponseTransformer.exceptionOccurred(e));
}
endOfStreamFuture.whenComplete((r2, e2) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
AsyncResponseTransformer finalAsyncResponseTransformer = asyncResponseTransformer;
runAndLogError(log, "Exception thrown in exceptionOccurred callback, ignoring",
() -> finalAsyncResponseTransformer.exceptionOccurred(t));
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the readme file or descriptive text for a package version.
*
*
* The returned text might contain formatting. For example, it might contain formatting for Markdown or
* reStructuredText.
*
*
* @param getPackageVersionReadmeRequest
* @return A Java Future containing the result of the GetPackageVersionReadme operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionReadme
* @see AWS API Documentation
*/
@Override
public CompletableFuture getPackageVersionReadme(
GetPackageVersionReadmeRequest getPackageVersionReadmeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getPackageVersionReadmeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPackageVersionReadmeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPackageVersionReadme");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetPackageVersionReadmeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetPackageVersionReadme").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetPackageVersionReadmeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getPackageVersionReadmeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the endpoint of a repository for a specific package format. A repository has one endpoint for each
* package format:
*
*
* -
*
* maven
*
*
* -
*
* npm
*
*
* -
*
* nuget
*
*
* -
*
* pypi
*
*
*
*
* @param getRepositoryEndpointRequest
* @return A Java Future containing the result of the GetRepositoryEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetRepositoryEndpoint
* @see AWS API Documentation
*/
@Override
public CompletableFuture getRepositoryEndpoint(
GetRepositoryEndpointRequest getRepositoryEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getRepositoryEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRepositoryEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRepositoryEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRepositoryEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRepositoryEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetRepositoryEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getRepositoryEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the resource policy that is set on a repository.
*
*
* @param getRepositoryPermissionsPolicyRequest
* @return A Java Future containing the result of the GetRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture getRepositoryPermissionsPolicy(
GetRepositoryPermissionsPolicyRequest getRepositoryPermissionsPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getRepositoryPermissionsPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getRepositoryPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRepositoryPermissionsPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRepositoryPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRepositoryPermissionsPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetRepositoryPermissionsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getRepositoryPermissionsPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of DomainSummary objects for all domains owned by the Amazon Web Services account that makes this call. Each
* returned DomainSummary
object contains information about a domain.
*
*
* @param listDomainsRequest
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListDomains
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listDomains(ListDomainsRequest listDomainsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDomainsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDomainsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDomains");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListDomainsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDomains").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDomainsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDomainsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of AssetSummary
* objects for assets in a package version.
*
*
* @param listPackageVersionAssetsRequest
* @return A Java Future containing the result of the ListPackageVersionAssets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionAssets
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPackageVersionAssets(
ListPackageVersionAssetsRequest listPackageVersionAssetsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listPackageVersionAssetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPackageVersionAssetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackageVersionAssets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPackageVersionAssetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPackageVersionAssets").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListPackageVersionAssetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listPackageVersionAssetsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the direct dependencies for a package version. The dependencies are returned as PackageDependency objects. CodeArtifact extracts the dependencies for a package version from the metadata
* file for the package format (for example, the package.json
file for npm packages and the
* pom.xml
file for Maven). Any package version dependencies that are not listed in the configuration
* file are not returned.
*
*
* @param listPackageVersionDependenciesRequest
* @return A Java Future containing the result of the ListPackageVersionDependencies operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionDependencies
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPackageVersionDependencies(
ListPackageVersionDependenciesRequest listPackageVersionDependenciesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listPackageVersionDependenciesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listPackageVersionDependenciesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackageVersionDependencies");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPackageVersionDependenciesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPackageVersionDependencies").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListPackageVersionDependenciesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listPackageVersionDependenciesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of PackageVersionSummary objects for package versions in a repository that match the request parameters.
* Package versions of all statuses will be returned by default when calling list-package-versions
with
* no --status
parameter.
*
*
* @param listPackageVersionsRequest
* @return A Java Future containing the result of the ListPackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersions
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPackageVersions(
ListPackageVersionsRequest listPackageVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listPackageVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPackageVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackageVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPackageVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPackageVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListPackageVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listPackageVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of PackageSummary
* objects for packages in a repository that match the request parameters.
*
*
* @param listPackagesRequest
* @return A Java Future containing the result of the ListPackages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackages
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listPackages(ListPackagesRequest listPackagesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listPackagesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPackagesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPackages");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListPackagesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPackages").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListPackagesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listPackagesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified Amazon Web Services account and that matches the input parameters.
*
*
* @param listRepositoriesRequest
* @return A Java Future containing the result of the ListRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositories
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listRepositories(ListRepositoriesRequest listRepositoriesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listRepositoriesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRepositoriesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRepositories");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListRepositoriesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListRepositories").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListRepositoriesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listRepositoriesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified domain and that matches the input parameters.
*
*
* @param listRepositoriesInDomainRequest
* @return A Java Future containing the result of the ListRepositoriesInDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositoriesInDomain
* @see AWS API Documentation
*/
@Override
public CompletableFuture listRepositoriesInDomain(
ListRepositoriesInDomainRequest listRepositoriesInDomainRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listRepositoriesInDomainRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRepositoriesInDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRepositoriesInDomain");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListRepositoriesInDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListRepositoriesInDomain").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListRepositoriesInDomainRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listRepositoriesInDomainRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about Amazon Web Services tags for a specified Amazon Resource Name (ARN) in CodeArtifact.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new package version containing one or more assets (or files).
*
*
* The unfinished
flag can be used to keep the package version in the Unfinished
state
* until all of its assets have been uploaded (see Package version status in the CodeArtifact user guide). To set the package version’s status to
* Published
, omit the unfinished
flag when uploading the final asset, or set the status
* using
* UpdatePackageVersionStatus. Once a package version’s status is set to Published
, it cannot
* change back to Unfinished
.
*
*
*
* Only generic packages can be published using this API. For more information, see Using generic packages in the
* CodeArtifact User Guide.
*
*
*
* @param publishPackageVersionRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The content of the asset to publish.
*
* '
* @return A Java Future containing the result of the PublishPackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PublishPackageVersion
* @see AWS API Documentation
*/
@Override
public CompletableFuture publishPackageVersion(
PublishPackageVersionRequest publishPackageVersionRequest, AsyncRequestBody requestBody) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(publishPackageVersionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, publishPackageVersionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PublishPackageVersion");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PublishPackageVersionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PublishPackageVersion")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(
AsyncStreamingRequestMarshaller.builder()
.delegateMarshaller(new PublishPackageVersionRequestMarshaller(protocolFactory))
.asyncRequestBody(requestBody).build()).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withAsyncRequestBody(requestBody)
.withInput(publishPackageVersionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets a resource policy on a domain that specifies permissions to access it.
*
*
* When you call PutDomainPermissionsPolicy
, the resource policy on the domain is ignored when
* evaluting permissions. This ensures that the owner of a domain cannot lock themselves out of the domain, which
* would prevent them from being able to update the resource policy.
*
*
* @param putDomainPermissionsPolicyRequest
* @return A Java Future containing the result of the PutDomainPermissionsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutDomainPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture putDomainPermissionsPolicy(
PutDomainPermissionsPolicyRequest putDomainPermissionsPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putDomainPermissionsPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putDomainPermissionsPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutDomainPermissionsPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutDomainPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutDomainPermissionsPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutDomainPermissionsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putDomainPermissionsPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the package origin configuration for a package.
*
*
* The package origin configuration determines how new versions of a package can be added to a repository. You can
* allow or block direct publishing of new package versions, or ingestion and retaining of new package versions from
* an external connection or upstream source. For more information about package origin controls and configuration,
* see Editing package
* origin controls in the CodeArtifact User Guide.
*
*
* PutPackageOriginConfiguration
can be called on a package that doesn't yet exist in the repository.
* When called on a package that does not exist, a package is created in the repository with no versions and the
* requested restrictions are set on the package. This can be used to preemptively block ingesting or retaining any
* versions from external connections or upstream repositories, or to block publishing any versions of the package
* into the repository before connecting any package managers or publishers to the repository.
*
*
* @param putPackageOriginConfigurationRequest
* @return A Java Future containing the result of the PutPackageOriginConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutPackageOriginConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture putPackageOriginConfiguration(
PutPackageOriginConfigurationRequest putPackageOriginConfigurationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putPackageOriginConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putPackageOriginConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutPackageOriginConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutPackageOriginConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutPackageOriginConfiguration").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutPackageOriginConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putPackageOriginConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the resource policy on a repository that specifies permissions to access it.
*
*
* When you call PutRepositoryPermissionsPolicy
, the resource policy on the repository is ignored when
* evaluting permissions. This ensures that the owner of a repository cannot lock themselves out of the repository,
* which would prevent them from being able to update the resource policy.
*
*
* @param putRepositoryPermissionsPolicyRequest
* @return A Java Future containing the result of the PutRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture putRepositoryPermissionsPolicy(
PutRepositoryPermissionsPolicyRequest putRepositoryPermissionsPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putRepositoryPermissionsPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putRepositoryPermissionsPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutRepositoryPermissionsPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutRepositoryPermissionsPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutRepositoryPermissionsPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutRepositoryPermissionsPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putRepositoryPermissionsPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds or updates tags for a resource in CodeArtifact.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "codeartifact");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams