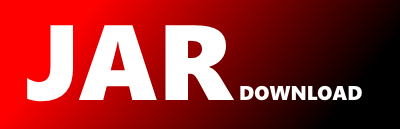
software.amazon.awssdk.services.codeartifact.CodeartifactAsyncClient Maven / Gradle / Ivy
Show all versions of codeartifact Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codeartifact;
import java.nio.file.Path;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.services.codeartifact.model.AssociateExternalConnectionRequest;
import software.amazon.awssdk.services.codeartifact.model.AssociateExternalConnectionResponse;
import software.amazon.awssdk.services.codeartifact.model.CopyPackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.CopyPackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.CreateDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.CreateDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.CreatePackageGroupRequest;
import software.amazon.awssdk.services.codeartifact.model.CreatePackageGroupResponse;
import software.amazon.awssdk.services.codeartifact.model.CreateRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.CreateRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageGroupRequest;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageGroupResponse;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageRequest;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageResponse;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.DeletePackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribeDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribeDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageGroupRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageGroupResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageVersionRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribePackageVersionResponse;
import software.amazon.awssdk.services.codeartifact.model.DescribeRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.DescribeRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.model.DisassociateExternalConnectionRequest;
import software.amazon.awssdk.services.codeartifact.model.DisassociateExternalConnectionResponse;
import software.amazon.awssdk.services.codeartifact.model.DisposePackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.DisposePackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.GetAssociatedPackageGroupRequest;
import software.amazon.awssdk.services.codeartifact.model.GetAssociatedPackageGroupResponse;
import software.amazon.awssdk.services.codeartifact.model.GetAuthorizationTokenRequest;
import software.amazon.awssdk.services.codeartifact.model.GetAuthorizationTokenResponse;
import software.amazon.awssdk.services.codeartifact.model.GetDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.GetDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetRequest;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetResponse;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionReadmeRequest;
import software.amazon.awssdk.services.codeartifact.model.GetPackageVersionReadmeResponse;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryEndpointRequest;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryEndpointResponse;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.GetRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupRequest;
import software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupResponse;
import software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListDomainsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionDependenciesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionDependenciesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListPackagesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainResponse;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest;
import software.amazon.awssdk.services.codeartifact.model.ListRepositoriesResponse;
import software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsRequest;
import software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsResponse;
import software.amazon.awssdk.services.codeartifact.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.PublishPackageVersionRequest;
import software.amazon.awssdk.services.codeartifact.model.PublishPackageVersionResponse;
import software.amazon.awssdk.services.codeartifact.model.PutDomainPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.PutDomainPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.PutPackageOriginConfigurationRequest;
import software.amazon.awssdk.services.codeartifact.model.PutPackageOriginConfigurationResponse;
import software.amazon.awssdk.services.codeartifact.model.PutRepositoryPermissionsPolicyRequest;
import software.amazon.awssdk.services.codeartifact.model.PutRepositoryPermissionsPolicyResponse;
import software.amazon.awssdk.services.codeartifact.model.TagResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.TagResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.UntagResourceRequest;
import software.amazon.awssdk.services.codeartifact.model.UntagResourceResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageGroupOriginConfigurationRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageGroupOriginConfigurationResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageGroupRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageGroupResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageVersionsStatusRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdatePackageVersionsStatusResponse;
import software.amazon.awssdk.services.codeartifact.model.UpdateRepositoryRequest;
import software.amazon.awssdk.services.codeartifact.model.UpdateRepositoryResponse;
import software.amazon.awssdk.services.codeartifact.paginators.ListAllowedRepositoriesForGroupPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListAssociatedPackagesPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListDomainsPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListPackageGroupsPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListPackagesPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesPublisher;
import software.amazon.awssdk.services.codeartifact.paginators.ListSubPackageGroupsPublisher;
/**
* Service client for accessing CodeArtifact asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
*
* CodeArtifact is a fully managed artifact repository compatible with language-native package managers and build tools
* such as npm, Apache Maven, pip, and dotnet. You can use CodeArtifact to share packages with development teams and
* pull packages. Packages can be pulled from both public and CodeArtifact repositories. You can also create an upstream
* relationship between a CodeArtifact repository and another repository, which effectively merges their contents from
* the point of view of a package manager client.
*
*
* CodeArtifact concepts
*
*
* -
*
* Repository: A CodeArtifact repository contains a set of package
* versions, each of which maps to a set of assets, or files. Repositories are polyglot, so a single repository can
* contain packages of any supported type. Each repository exposes endpoints for fetching and publishing packages using
* tools such as the npm
CLI or the Maven CLI ( mvn
). For a list of supported
* package managers, see the CodeArtifact User
* Guide.
*
*
* -
*
* Domain: Repositories are aggregated into a higher-level entity known as a domain. All package assets
* and metadata are stored in the domain, but are consumed through repositories. A given package asset, such as a Maven
* JAR file, is stored once per domain, no matter how many repositories it's present in. All of the assets and metadata
* in a domain are encrypted with the same customer master key (CMK) stored in Key Management Service (KMS).
*
*
* Each repository is a member of a single domain and can't be moved to a different domain.
*
*
* The domain allows organizational policy to be applied across multiple repositories, such as which accounts can access
* repositories in the domain, and which public repositories can be used as sources of packages.
*
*
* Although an organization can have multiple domains, we recommend a single production domain that contains all
* published artifacts so that teams can find and share packages across their organization.
*
*
* -
*
* Package: A package is a bundle of software and the metadata required to resolve dependencies and
* install the software. CodeArtifact supports npm, PyPI, Maven, NuGet, Swift, Ruby, Cargo, and generic package formats.
* For more information about the supported package formats and how to use CodeArtifact with them, see the CodeArtifact User Guide.
*
*
* In CodeArtifact, a package consists of:
*
*
* -
*
* A name (for example, webpack
is the name of a popular npm package)
*
*
* -
*
* An optional namespace (for example, @types
in @types/node
)
*
*
* -
*
* A set of versions (for example, 1.0.0
, 1.0.1
, 1.0.2
, etc.)
*
*
* -
*
* Package-level metadata (for example, npm tags)
*
*
*
*
* -
*
* Package group: A group of packages that match a specified definition. Package groups can be used to apply
* configuration to multiple packages that match a defined pattern using package format, package namespace, and package
* name. You can use package groups to more conveniently configure package origin controls for multiple packages.
* Package origin controls are used to block or allow ingestion or publishing of new package versions, which protects
* users from malicious actions known as dependency substitution attacks.
*
*
* -
*
* Package version: A version of a package, such as @types/node 12.6.9
. The version number format
* and semantics vary for different package formats. For example, npm package versions must conform to the Semantic Versioning specification. In CodeArtifact, a package version consists of the
* version identifier, metadata at the package version level, and a set of assets.
*
*
* -
*
* Upstream repository: One repository is upstream of another when the package versions in it can be
* accessed from the repository endpoint of the downstream repository, effectively merging the contents of the two
* repositories from the point of view of a client. CodeArtifact allows creating an upstream relationship between two
* repositories.
*
*
* -
*
* Asset: An individual file stored in CodeArtifact associated with a package version, such as an npm
* .tgz
file or Maven POM and JAR files.
*
*
*
*
* CodeArtifact supported API operations
*
*
* -
*
* AssociateExternalConnection
: Adds an existing external connection to a repository.
*
*
* -
*
* CopyPackageVersions
: Copies package versions from one repository to another repository in the same
* domain.
*
*
* -
*
* CreateDomain
: Creates a domain.
*
*
* -
*
* CreatePackageGroup
: Creates a package group.
*
*
* -
*
* CreateRepository
: Creates a CodeArtifact repository in a domain.
*
*
* -
*
* DeleteDomain
: Deletes a domain. You cannot delete a domain that contains repositories.
*
*
* -
*
* DeleteDomainPermissionsPolicy
: Deletes the resource policy that is set on a domain.
*
*
* -
*
* DeletePackage
: Deletes a package and all associated package versions.
*
*
* -
*
* DeletePackageGroup
: Deletes a package group. Does not delete packages or package versions that are
* associated with a package group.
*
*
* -
*
* DeletePackageVersions
: Deletes versions of a package. After a package has been deleted, it can be
* republished, but its assets and metadata cannot be restored because they have been permanently removed from storage.
*
*
* -
*
* DeleteRepository
: Deletes a repository.
*
*
* -
*
* DeleteRepositoryPermissionsPolicy
: Deletes the resource policy that is set on a repository.
*
*
* -
*
* DescribeDomain
: Returns a DomainDescription
object that contains information about the
* requested domain.
*
*
* -
*
* DescribePackage
: Returns a PackageDescription object that contains details about a package.
*
*
* -
*
* DescribePackageGroup
: Returns a PackageGroup object
* that contains details about a package group.
*
*
* -
*
* DescribePackageVersion
: Returns a PackageVersionDescription object that contains details about a package version.
*
*
* -
*
* DescribeRepository
: Returns a RepositoryDescription
object that contains detailed
* information about the requested repository.
*
*
* -
*
* DisposePackageVersions
: Disposes versions of a package. A package version with the status
* Disposed
cannot be restored because they have been permanently removed from storage.
*
*
* -
*
* DisassociateExternalConnection
: Removes an existing external connection from a repository.
*
*
* -
*
* GetAssociatedPackageGroup
: Returns the most closely associated package group to the specified package.
*
*
* -
*
* GetAuthorizationToken
: Generates a temporary authorization token for accessing repositories in the
* domain. The token expires the authorization period has passed. The default authorization period is 12 hours and can
* be customized to any length with a maximum of 12 hours.
*
*
* -
*
* GetDomainPermissionsPolicy
: Returns the policy of a resource that is attached to the specified domain.
*
*
* -
*
* GetPackageVersionAsset
: Returns the contents of an asset that is in a package version.
*
*
* -
*
* GetPackageVersionReadme
: Gets the readme file or descriptive text for a package version.
*
*
* -
*
* GetRepositoryEndpoint
: Returns the endpoint of a repository for a specific package format. A repository
* has one endpoint for each package format:
*
*
* -
*
* cargo
*
*
* -
*
* generic
*
*
* -
*
* maven
*
*
* -
*
* npm
*
*
* -
*
* nuget
*
*
* -
*
* pypi
*
*
* -
*
* ruby
*
*
* -
*
* swift
*
*
*
*
* -
*
* GetRepositoryPermissionsPolicy
: Returns the resource policy that is set on a repository.
*
*
* -
*
* ListAllowedRepositoriesForGroup
: Lists the allowed repositories for a package group that has origin
* configuration set to ALLOW_SPECIFIC_REPOSITORIES
.
*
*
* -
*
* ListAssociatedPackages
: Returns a list of packages associated with the requested package group.
*
*
* -
*
* ListDomains
: Returns a list of DomainSummary
objects. Each returned
* DomainSummary
object contains information about a domain.
*
*
* -
*
* ListPackages
: Lists the packages in a repository.
*
*
* -
*
* ListPackageGroups
: Returns a list of package groups in the requested domain.
*
*
* -
*
* ListPackageVersionAssets
: Lists the assets for a given package version.
*
*
* -
*
* ListPackageVersionDependencies
: Returns a list of the direct dependencies for a package version.
*
*
* -
*
* ListPackageVersions
: Returns a list of package versions for a specified package in a repository.
*
*
* -
*
* ListRepositories
: Returns a list of repositories owned by the Amazon Web Services account that called
* this method.
*
*
* -
*
* ListRepositoriesInDomain
: Returns a list of the repositories in a domain.
*
*
* -
*
* ListSubPackageGroups
: Returns a list of direct children of the specified package group.
*
*
* -
*
* PublishPackageVersion
: Creates a new package version containing one or more assets.
*
*
* -
*
* PutDomainPermissionsPolicy
: Attaches a resource policy to a domain.
*
*
* -
*
* PutPackageOriginConfiguration
: Sets the package origin configuration for a package, which determine how
* new versions of the package can be added to a specific repository.
*
*
* -
*
* PutRepositoryPermissionsPolicy
: Sets the resource policy on a repository that specifies permissions to
* access it.
*
*
* -
*
* UpdatePackageGroup
: Updates a package group. This API cannot be used to update a package group's origin
* configuration or pattern.
*
*
* -
*
* UpdatePackageGroupOriginConfiguration
: Updates the package origin configuration for a package group.
*
*
* -
*
* UpdatePackageVersionsStatus
: Updates the status of one or more versions of a package.
*
*
* -
*
* UpdateRepository
: Updates the properties of a repository.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface CodeartifactAsyncClient extends AwsClient {
String SERVICE_NAME = "codeartifact";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "codeartifact";
/**
*
* Adds an existing external connection to a repository. One external connection is allowed per repository.
*
*
*
* A repository can have one or more upstream repositories, or an external connection.
*
*
*
* @param associateExternalConnectionRequest
* @return A Java Future containing the result of the AssociateExternalConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.AssociateExternalConnection
* @see AWS API Documentation
*/
default CompletableFuture associateExternalConnection(
AssociateExternalConnectionRequest associateExternalConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds an existing external connection to a repository. One external connection is allowed per repository.
*
*
*
* A repository can have one or more upstream repositories, or an external connection.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateExternalConnectionRequest.Builder}
* avoiding the need to create one manually via {@link AssociateExternalConnectionRequest#builder()}
*
*
* @param associateExternalConnectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.AssociateExternalConnectionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateExternalConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.AssociateExternalConnection
* @see AWS API Documentation
*/
default CompletableFuture associateExternalConnection(
Consumer associateExternalConnectionRequest) {
return associateExternalConnection(AssociateExternalConnectionRequest.builder()
.applyMutation(associateExternalConnectionRequest).build());
}
/**
*
* Copies package versions from one repository to another repository in the same domain.
*
*
*
* You must specify versions
or versionRevisions
. You cannot specify both.
*
*
*
* @param copyPackageVersionsRequest
* @return A Java Future containing the result of the CopyPackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CopyPackageVersions
* @see AWS API Documentation
*/
default CompletableFuture copyPackageVersions(
CopyPackageVersionsRequest copyPackageVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copies package versions from one repository to another repository in the same domain.
*
*
*
* You must specify versions
or versionRevisions
. You cannot specify both.
*
*
*
* This is a convenience which creates an instance of the {@link CopyPackageVersionsRequest.Builder} avoiding the
* need to create one manually via {@link CopyPackageVersionsRequest#builder()}
*
*
* @param copyPackageVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.CopyPackageVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CopyPackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CopyPackageVersions
* @see AWS API Documentation
*/
default CompletableFuture copyPackageVersions(
Consumer copyPackageVersionsRequest) {
return copyPackageVersions(CopyPackageVersionsRequest.builder().applyMutation(copyPackageVersionsRequest).build());
}
/**
*
* Creates a domain. CodeArtifact domains make it easier to manage multiple repositories across an
* organization. You can use a domain to apply permissions across many repositories owned by different Amazon Web
* Services accounts. An asset is stored only once in a domain, even if it's in multiple repositories.
*
*
* Although you can have multiple domains, we recommend a single production domain that contains all published
* artifacts so that your development teams can find and share packages. You can use a second pre-production domain
* to test changes to the production domain configuration.
*
*
* @param createDomainRequest
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreateDomain
* @see AWS API
* Documentation
*/
default CompletableFuture createDomain(CreateDomainRequest createDomainRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a domain. CodeArtifact domains make it easier to manage multiple repositories across an
* organization. You can use a domain to apply permissions across many repositories owned by different Amazon Web
* Services accounts. An asset is stored only once in a domain, even if it's in multiple repositories.
*
*
* Although you can have multiple domains, we recommend a single production domain that contains all published
* artifacts so that your development teams can find and share packages. You can use a second pre-production domain
* to test changes to the production domain configuration.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDomainRequest.Builder} avoiding the need to
* create one manually via {@link CreateDomainRequest#builder()}
*
*
* @param createDomainRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.CreateDomainRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreateDomain
* @see AWS API
* Documentation
*/
default CompletableFuture createDomain(Consumer createDomainRequest) {
return createDomain(CreateDomainRequest.builder().applyMutation(createDomainRequest).build());
}
/**
*
* Creates a package group. For more information about creating package groups, including example CLI commands, see
* Create a package group
* in the CodeArtifact User Guide.
*
*
* @param createPackageGroupRequest
* @return A Java Future containing the result of the CreatePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreatePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture createPackageGroup(CreatePackageGroupRequest createPackageGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a package group. For more information about creating package groups, including example CLI commands, see
* Create a package group
* in the CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePackageGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreatePackageGroupRequest#builder()}
*
*
* @param createPackageGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.CreatePackageGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreatePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreatePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture createPackageGroup(
Consumer createPackageGroupRequest) {
return createPackageGroup(CreatePackageGroupRequest.builder().applyMutation(createPackageGroupRequest).build());
}
/**
*
* Creates a repository.
*
*
* @param createRepositoryRequest
* @return A Java Future containing the result of the CreateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreateRepository
* @see AWS
* API Documentation
*/
default CompletableFuture createRepository(CreateRepositoryRequest createRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a repository.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link CreateRepositoryRequest#builder()}
*
*
* @param createRepositoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.CreateRepositoryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.CreateRepository
* @see AWS
* API Documentation
*/
default CompletableFuture createRepository(
Consumer createRepositoryRequest) {
return createRepository(CreateRepositoryRequest.builder().applyMutation(createRepositoryRequest).build());
}
/**
*
* Deletes a domain. You cannot delete a domain that contains repositories. If you want to delete a domain with
* repositories, first delete its repositories.
*
*
* @param deleteDomainRequest
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteDomain
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDomain(DeleteDomainRequest deleteDomainRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a domain. You cannot delete a domain that contains repositories. If you want to delete a domain with
* repositories, first delete its repositories.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDomainRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDomainRequest#builder()}
*
*
* @param deleteDomainRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DeleteDomainRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteDomain
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDomain(Consumer deleteDomainRequest) {
return deleteDomain(DeleteDomainRequest.builder().applyMutation(deleteDomainRequest).build());
}
/**
*
* Deletes the resource policy set on a domain.
*
*
* @param deleteDomainPermissionsPolicyRequest
* @return A Java Future containing the result of the DeleteDomainPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteDomainPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteDomainPermissionsPolicy(
DeleteDomainPermissionsPolicyRequest deleteDomainPermissionsPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the resource policy set on a domain.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDomainPermissionsPolicyRequest.Builder}
* avoiding the need to create one manually via {@link DeleteDomainPermissionsPolicyRequest#builder()}
*
*
* @param deleteDomainPermissionsPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DeleteDomainPermissionsPolicyRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteDomainPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteDomainPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteDomainPermissionsPolicy(
Consumer deleteDomainPermissionsPolicyRequest) {
return deleteDomainPermissionsPolicy(DeleteDomainPermissionsPolicyRequest.builder()
.applyMutation(deleteDomainPermissionsPolicyRequest).build());
}
/**
*
* Deletes a package and all associated package versions. A deleted package cannot be restored. To delete one or
* more package versions, use the DeletePackageVersions API.
*
*
* @param deletePackageRequest
* @return A Java Future containing the result of the DeletePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackage
* @see AWS
* API Documentation
*/
default CompletableFuture deletePackage(DeletePackageRequest deletePackageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a package and all associated package versions. A deleted package cannot be restored. To delete one or
* more package versions, use the DeletePackageVersions API.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePackageRequest.Builder} avoiding the need to
* create one manually via {@link DeletePackageRequest#builder()}
*
*
* @param deletePackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DeletePackageRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeletePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackage
* @see AWS
* API Documentation
*/
default CompletableFuture deletePackage(Consumer deletePackageRequest) {
return deletePackage(DeletePackageRequest.builder().applyMutation(deletePackageRequest).build());
}
/**
*
* Deletes a package group. Deleting a package group does not delete packages or package versions associated with
* the package group. When a package group is deleted, the direct child package groups will become children of the
* package group's direct parent package group. Therefore, if any of the child groups are inheriting any settings
* from the parent, those settings could change.
*
*
* @param deletePackageGroupRequest
* @return A Java Future containing the result of the DeletePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture deletePackageGroup(DeletePackageGroupRequest deletePackageGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a package group. Deleting a package group does not delete packages or package versions associated with
* the package group. When a package group is deleted, the direct child package groups will become children of the
* package group's direct parent package group. Therefore, if any of the child groups are inheriting any settings
* from the parent, those settings could change.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePackageGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeletePackageGroupRequest#builder()}
*
*
* @param deletePackageGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DeletePackageGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeletePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture deletePackageGroup(
Consumer deletePackageGroupRequest) {
return deletePackageGroup(DeletePackageGroupRequest.builder().applyMutation(deletePackageGroupRequest).build());
}
/**
*
* Deletes one or more versions of a package. A deleted package version cannot be restored in your repository. If
* you want to remove a package version from your repository and be able to restore it later, set its status to
* Archived
. Archived packages cannot be downloaded from a repository and don't show up with list
* package APIs (for example, ListPackageVersions), but you can restore them using UpdatePackageVersionsStatus.
*
*
* @param deletePackageVersionsRequest
* @return A Java Future containing the result of the DeletePackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackageVersions
* @see AWS API Documentation
*/
default CompletableFuture deletePackageVersions(
DeletePackageVersionsRequest deletePackageVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes one or more versions of a package. A deleted package version cannot be restored in your repository. If
* you want to remove a package version from your repository and be able to restore it later, set its status to
* Archived
. Archived packages cannot be downloaded from a repository and don't show up with list
* package APIs (for example, ListPackageVersions), but you can restore them using UpdatePackageVersionsStatus.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePackageVersionsRequest.Builder} avoiding the
* need to create one manually via {@link DeletePackageVersionsRequest#builder()}
*
*
* @param deletePackageVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DeletePackageVersionsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeletePackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeletePackageVersions
* @see AWS API Documentation
*/
default CompletableFuture deletePackageVersions(
Consumer deletePackageVersionsRequest) {
return deletePackageVersions(DeletePackageVersionsRequest.builder().applyMutation(deletePackageVersionsRequest).build());
}
/**
*
* Deletes a repository.
*
*
* @param deleteRepositoryRequest
* @return A Java Future containing the result of the DeleteRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteRepository
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRepository(DeleteRepositoryRequest deleteRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link DeleteRepositoryRequest#builder()}
*
*
* @param deleteRepositoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteRepository
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRepository(
Consumer deleteRepositoryRequest) {
return deleteRepository(DeleteRepositoryRequest.builder().applyMutation(deleteRepositoryRequest).build());
}
/**
*
* Deletes the resource policy that is set on a repository. After a resource policy is deleted, the permissions
* allowed and denied by the deleted policy are removed. The effect of deleting a resource policy might not be
* immediate.
*
*
*
* Use DeleteRepositoryPermissionsPolicy
with caution. After a policy is deleted, Amazon Web Services
* users, roles, and accounts lose permissions to perform the repository actions granted by the deleted policy.
*
*
*
* @param deleteRepositoryPermissionsPolicyRequest
* @return A Java Future containing the result of the DeleteRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteRepositoryPermissionsPolicy(
DeleteRepositoryPermissionsPolicyRequest deleteRepositoryPermissionsPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the resource policy that is set on a repository. After a resource policy is deleted, the permissions
* allowed and denied by the deleted policy are removed. The effect of deleting a resource policy might not be
* immediate.
*
*
*
* Use DeleteRepositoryPermissionsPolicy
with caution. After a policy is deleted, Amazon Web Services
* users, roles, and accounts lose permissions to perform the repository actions granted by the deleted policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryPermissionsPolicyRequest.Builder}
* avoiding the need to create one manually via {@link DeleteRepositoryPermissionsPolicyRequest#builder()}
*
*
* @param deleteRepositoryPermissionsPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DeleteRepositoryPermissionsPolicyRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DeleteRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DeleteRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteRepositoryPermissionsPolicy(
Consumer deleteRepositoryPermissionsPolicyRequest) {
return deleteRepositoryPermissionsPolicy(DeleteRepositoryPermissionsPolicyRequest.builder()
.applyMutation(deleteRepositoryPermissionsPolicyRequest).build());
}
/**
*
* Returns a
* DomainDescription object that contains information about the requested domain.
*
*
* @param describeDomainRequest
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribeDomain
* @see AWS
* API Documentation
*/
default CompletableFuture describeDomain(DescribeDomainRequest describeDomainRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a
* DomainDescription object that contains information about the requested domain.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDomainRequest.Builder} avoiding the need to
* create one manually via {@link DescribeDomainRequest#builder()}
*
*
* @param describeDomainRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DescribeDomainRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribeDomain
* @see AWS
* API Documentation
*/
default CompletableFuture describeDomain(Consumer describeDomainRequest) {
return describeDomain(DescribeDomainRequest.builder().applyMutation(describeDomainRequest).build());
}
/**
*
* Returns a
* PackageDescription object that contains information about the requested package.
*
*
* @param describePackageRequest
* @return A Java Future containing the result of the DescribePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackage
* @see AWS
* API Documentation
*/
default CompletableFuture describePackage(DescribePackageRequest describePackageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a
* PackageDescription object that contains information about the requested package.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePackageRequest.Builder} avoiding the need
* to create one manually via {@link DescribePackageRequest#builder()}
*
*
* @param describePackageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DescribePackageRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackage
* @see AWS
* API Documentation
*/
default CompletableFuture describePackage(
Consumer describePackageRequest) {
return describePackage(DescribePackageRequest.builder().applyMutation(describePackageRequest).build());
}
/**
*
* Returns a
* PackageGroupDescription object that contains information about the requested package group.
*
*
* @param describePackageGroupRequest
* @return A Java Future containing the result of the DescribePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture describePackageGroup(
DescribePackageGroupRequest describePackageGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a
* PackageGroupDescription object that contains information about the requested package group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePackageGroupRequest.Builder} avoiding the
* need to create one manually via {@link DescribePackageGroupRequest#builder()}
*
*
* @param describePackageGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DescribePackageGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture describePackageGroup(
Consumer describePackageGroupRequest) {
return describePackageGroup(DescribePackageGroupRequest.builder().applyMutation(describePackageGroupRequest).build());
}
/**
*
* Returns a PackageVersionDescription object that contains information about the requested package version.
*
*
* @param describePackageVersionRequest
* @return A Java Future containing the result of the DescribePackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackageVersion
* @see AWS API Documentation
*/
default CompletableFuture describePackageVersion(
DescribePackageVersionRequest describePackageVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a PackageVersionDescription object that contains information about the requested package version.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePackageVersionRequest.Builder} avoiding the
* need to create one manually via {@link DescribePackageVersionRequest#builder()}
*
*
* @param describePackageVersionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DescribePackageVersionRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribePackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribePackageVersion
* @see AWS API Documentation
*/
default CompletableFuture describePackageVersion(
Consumer describePackageVersionRequest) {
return describePackageVersion(DescribePackageVersionRequest.builder().applyMutation(describePackageVersionRequest)
.build());
}
/**
*
* Returns a RepositoryDescription
object that contains detailed information about the requested
* repository.
*
*
* @param describeRepositoryRequest
* @return A Java Future containing the result of the DescribeRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribeRepository
* @see AWS API Documentation
*/
default CompletableFuture describeRepository(DescribeRepositoryRequest describeRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a RepositoryDescription
object that contains detailed information about the requested
* repository.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoryRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRepositoryRequest#builder()}
*
*
* @param describeRepositoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DescribeRepositoryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DescribeRepository
* @see AWS API Documentation
*/
default CompletableFuture describeRepository(
Consumer describeRepositoryRequest) {
return describeRepository(DescribeRepositoryRequest.builder().applyMutation(describeRepositoryRequest).build());
}
/**
*
* Removes an existing external connection from a repository.
*
*
* @param disassociateExternalConnectionRequest
* @return A Java Future containing the result of the DisassociateExternalConnection operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DisassociateExternalConnection
* @see AWS API Documentation
*/
default CompletableFuture disassociateExternalConnection(
DisassociateExternalConnectionRequest disassociateExternalConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes an existing external connection from a repository.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateExternalConnectionRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateExternalConnectionRequest#builder()}
*
*
* @param disassociateExternalConnectionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DisassociateExternalConnectionRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DisassociateExternalConnection operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DisassociateExternalConnection
* @see AWS API Documentation
*/
default CompletableFuture disassociateExternalConnection(
Consumer disassociateExternalConnectionRequest) {
return disassociateExternalConnection(DisassociateExternalConnectionRequest.builder()
.applyMutation(disassociateExternalConnectionRequest).build());
}
/**
*
* Deletes the assets in package versions and sets the package versions' status to Disposed
. A disposed
* package version cannot be restored in your repository because its assets are deleted.
*
*
* To view all disposed package versions in a repository, use ListPackageVersions and set the status parameter to Disposed
.
*
*
* To view information about a disposed package version, use DescribePackageVersion.
*
*
* @param disposePackageVersionsRequest
* @return A Java Future containing the result of the DisposePackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DisposePackageVersions
* @see AWS API Documentation
*/
default CompletableFuture disposePackageVersions(
DisposePackageVersionsRequest disposePackageVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the assets in package versions and sets the package versions' status to Disposed
. A disposed
* package version cannot be restored in your repository because its assets are deleted.
*
*
* To view all disposed package versions in a repository, use ListPackageVersions and set the status parameter to Disposed
.
*
*
* To view information about a disposed package version, use DescribePackageVersion.
*
*
*
* This is a convenience which creates an instance of the {@link DisposePackageVersionsRequest.Builder} avoiding the
* need to create one manually via {@link DisposePackageVersionsRequest#builder()}
*
*
* @param disposePackageVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.DisposePackageVersionsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DisposePackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.DisposePackageVersions
* @see AWS API Documentation
*/
default CompletableFuture disposePackageVersions(
Consumer disposePackageVersionsRequest) {
return disposePackageVersions(DisposePackageVersionsRequest.builder().applyMutation(disposePackageVersionsRequest)
.build());
}
/**
*
* Returns the most closely associated package group to the specified package. This API does not require that the
* package exist in any repository in the domain. As such, GetAssociatedPackageGroup
can be used to see
* which package group's origin configuration applies to a package before that package is in a repository. This can
* be helpful to check if public packages are blocked without ingesting them.
*
*
* For information package group association and matching, see Package group definition syntax and matching behavior in the CodeArtifact User Guide.
*
*
* @param getAssociatedPackageGroupRequest
* @return A Java Future containing the result of the GetAssociatedPackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetAssociatedPackageGroup
* @see AWS API Documentation
*/
default CompletableFuture getAssociatedPackageGroup(
GetAssociatedPackageGroupRequest getAssociatedPackageGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the most closely associated package group to the specified package. This API does not require that the
* package exist in any repository in the domain. As such, GetAssociatedPackageGroup
can be used to see
* which package group's origin configuration applies to a package before that package is in a repository. This can
* be helpful to check if public packages are blocked without ingesting them.
*
*
* For information package group association and matching, see Package group definition syntax and matching behavior in the CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetAssociatedPackageGroupRequest.Builder} avoiding
* the need to create one manually via {@link GetAssociatedPackageGroupRequest#builder()}
*
*
* @param getAssociatedPackageGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetAssociatedPackageGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetAssociatedPackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetAssociatedPackageGroup
* @see AWS API Documentation
*/
default CompletableFuture getAssociatedPackageGroup(
Consumer getAssociatedPackageGroupRequest) {
return getAssociatedPackageGroup(GetAssociatedPackageGroupRequest.builder()
.applyMutation(getAssociatedPackageGroupRequest).build());
}
/**
*
* Generates a temporary authorization token for accessing repositories in the domain. This API requires the
* codeartifact:GetAuthorizationToken
and sts:GetServiceBearerToken
permissions. For more
* information about authorization tokens, see CodeArtifact authentication
* and tokens.
*
*
*
* CodeArtifact authorization tokens are valid for a period of 12 hours when created with the login
* command. You can call login
periodically to refresh the token. When you create an authorization
* token with the GetAuthorizationToken
API, you can set a custom authorization period, up to a maximum
* of 12 hours, with the durationSeconds
parameter.
*
*
* The authorization period begins after login
or GetAuthorizationToken
is called. If
* login
or GetAuthorizationToken
is called while assuming a role, the token lifetime is
* independent of the maximum session duration of the role. For example, if you call sts assume-role
* and specify a session duration of 15 minutes, then generate a CodeArtifact authorization token, the token will be
* valid for the full authorization period even though this is longer than the 15-minute session duration.
*
*
* See Using IAM Roles for more
* information on controlling session duration.
*
*
*
* @param getAuthorizationTokenRequest
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetAuthorizationToken
* @see AWS API Documentation
*/
default CompletableFuture getAuthorizationToken(
GetAuthorizationTokenRequest getAuthorizationTokenRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Generates a temporary authorization token for accessing repositories in the domain. This API requires the
* codeartifact:GetAuthorizationToken
and sts:GetServiceBearerToken
permissions. For more
* information about authorization tokens, see CodeArtifact authentication
* and tokens.
*
*
*
* CodeArtifact authorization tokens are valid for a period of 12 hours when created with the login
* command. You can call login
periodically to refresh the token. When you create an authorization
* token with the GetAuthorizationToken
API, you can set a custom authorization period, up to a maximum
* of 12 hours, with the durationSeconds
parameter.
*
*
* The authorization period begins after login
or GetAuthorizationToken
is called. If
* login
or GetAuthorizationToken
is called while assuming a role, the token lifetime is
* independent of the maximum session duration of the role. For example, if you call sts assume-role
* and specify a session duration of 15 minutes, then generate a CodeArtifact authorization token, the token will be
* valid for the full authorization period even though this is longer than the 15-minute session duration.
*
*
* See Using IAM Roles for more
* information on controlling session duration.
*
*
*
* This is a convenience which creates an instance of the {@link GetAuthorizationTokenRequest.Builder} avoiding the
* need to create one manually via {@link GetAuthorizationTokenRequest#builder()}
*
*
* @param getAuthorizationTokenRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetAuthorizationTokenRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetAuthorizationToken
* @see AWS API Documentation
*/
default CompletableFuture getAuthorizationToken(
Consumer getAuthorizationTokenRequest) {
return getAuthorizationToken(GetAuthorizationTokenRequest.builder().applyMutation(getAuthorizationTokenRequest).build());
}
/**
*
* Returns the resource policy attached to the specified domain.
*
*
*
* The policy is a resource-based policy, not an identity-based policy. For more information, see Identity-based
* policies and resource-based policies in the IAM User Guide.
*
*
*
* @param getDomainPermissionsPolicyRequest
* @return A Java Future containing the result of the GetDomainPermissionsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetDomainPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture getDomainPermissionsPolicy(
GetDomainPermissionsPolicyRequest getDomainPermissionsPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the resource policy attached to the specified domain.
*
*
*
* The policy is a resource-based policy, not an identity-based policy. For more information, see Identity-based
* policies and resource-based policies in the IAM User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetDomainPermissionsPolicyRequest.Builder} avoiding
* the need to create one manually via {@link GetDomainPermissionsPolicyRequest#builder()}
*
*
* @param getDomainPermissionsPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetDomainPermissionsPolicyRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetDomainPermissionsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetDomainPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture getDomainPermissionsPolicy(
Consumer getDomainPermissionsPolicyRequest) {
return getDomainPermissionsPolicy(GetDomainPermissionsPolicyRequest.builder()
.applyMutation(getDomainPermissionsPolicyRequest).build());
}
/**
*
* Returns an asset (or file) that is in a package. For example, for a Maven package version, use
* GetPackageVersionAsset
to download a JAR
file, a POM
file, or any other
* assets in the package version.
*
*
* @param getPackageVersionAssetRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The binary file, or asset, that is downloaded.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionAsset
* @see AWS API Documentation
*/
default CompletableFuture getPackageVersionAsset(
GetPackageVersionAssetRequest getPackageVersionAssetRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Returns an asset (or file) that is in a package. For example, for a Maven package version, use
* GetPackageVersionAsset
to download a JAR
file, a POM
file, or any other
* assets in the package version.
*
*
*
* This is a convenience which creates an instance of the {@link GetPackageVersionAssetRequest.Builder} avoiding the
* need to create one manually via {@link GetPackageVersionAssetRequest#builder()}
*
*
* @param getPackageVersionAssetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetRequest.Builder} to create
* a request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The binary file, or asset, that is downloaded.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionAsset
* @see AWS API Documentation
*/
default CompletableFuture getPackageVersionAsset(
Consumer getPackageVersionAssetRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return getPackageVersionAsset(GetPackageVersionAssetRequest.builder().applyMutation(getPackageVersionAssetRequest)
.build(), asyncResponseTransformer);
}
/**
*
* Returns an asset (or file) that is in a package. For example, for a Maven package version, use
* GetPackageVersionAsset
to download a JAR
file, a POM
file, or any other
* assets in the package version.
*
*
* @param getPackageVersionAssetRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The binary file, or asset, that is downloaded.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionAsset
* @see AWS API Documentation
*/
default CompletableFuture getPackageVersionAsset(
GetPackageVersionAssetRequest getPackageVersionAssetRequest, Path destinationPath) {
return getPackageVersionAsset(getPackageVersionAssetRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Returns an asset (or file) that is in a package. For example, for a Maven package version, use
* GetPackageVersionAsset
to download a JAR
file, a POM
file, or any other
* assets in the package version.
*
*
*
* This is a convenience which creates an instance of the {@link GetPackageVersionAssetRequest.Builder} avoiding the
* need to create one manually via {@link GetPackageVersionAssetRequest#builder()}
*
*
* @param getPackageVersionAssetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetPackageVersionAssetRequest.Builder} to create
* a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The binary file, or asset, that is downloaded.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionAsset
* @see AWS API Documentation
*/
default CompletableFuture getPackageVersionAsset(
Consumer getPackageVersionAssetRequest, Path destinationPath) {
return getPackageVersionAsset(GetPackageVersionAssetRequest.builder().applyMutation(getPackageVersionAssetRequest)
.build(), destinationPath);
}
/**
*
* Gets the readme file or descriptive text for a package version.
*
*
* The returned text might contain formatting. For example, it might contain formatting for Markdown or
* reStructuredText.
*
*
* @param getPackageVersionReadmeRequest
* @return A Java Future containing the result of the GetPackageVersionReadme operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionReadme
* @see AWS API Documentation
*/
default CompletableFuture getPackageVersionReadme(
GetPackageVersionReadmeRequest getPackageVersionReadmeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the readme file or descriptive text for a package version.
*
*
* The returned text might contain formatting. For example, it might contain formatting for Markdown or
* reStructuredText.
*
*
*
* This is a convenience which creates an instance of the {@link GetPackageVersionReadmeRequest.Builder} avoiding
* the need to create one manually via {@link GetPackageVersionReadmeRequest#builder()}
*
*
* @param getPackageVersionReadmeRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetPackageVersionReadmeRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetPackageVersionReadme operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetPackageVersionReadme
* @see AWS API Documentation
*/
default CompletableFuture getPackageVersionReadme(
Consumer getPackageVersionReadmeRequest) {
return getPackageVersionReadme(GetPackageVersionReadmeRequest.builder().applyMutation(getPackageVersionReadmeRequest)
.build());
}
/**
*
* Returns the endpoint of a repository for a specific package format. A repository has one endpoint for each
* package format:
*
*
* -
*
* cargo
*
*
* -
*
* generic
*
*
* -
*
* maven
*
*
* -
*
* npm
*
*
* -
*
* nuget
*
*
* -
*
* pypi
*
*
* -
*
* ruby
*
*
* -
*
* swift
*
*
*
*
* @param getRepositoryEndpointRequest
* @return A Java Future containing the result of the GetRepositoryEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetRepositoryEndpoint
* @see AWS API Documentation
*/
default CompletableFuture getRepositoryEndpoint(
GetRepositoryEndpointRequest getRepositoryEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the endpoint of a repository for a specific package format. A repository has one endpoint for each
* package format:
*
*
* -
*
* cargo
*
*
* -
*
* generic
*
*
* -
*
* maven
*
*
* -
*
* npm
*
*
* -
*
* nuget
*
*
* -
*
* pypi
*
*
* -
*
* ruby
*
*
* -
*
* swift
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetRepositoryEndpointRequest.Builder} avoiding the
* need to create one manually via {@link GetRepositoryEndpointRequest#builder()}
*
*
* @param getRepositoryEndpointRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetRepositoryEndpointRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetRepositoryEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetRepositoryEndpoint
* @see AWS API Documentation
*/
default CompletableFuture getRepositoryEndpoint(
Consumer getRepositoryEndpointRequest) {
return getRepositoryEndpoint(GetRepositoryEndpointRequest.builder().applyMutation(getRepositoryEndpointRequest).build());
}
/**
*
* Returns the resource policy that is set on a repository.
*
*
* @param getRepositoryPermissionsPolicyRequest
* @return A Java Future containing the result of the GetRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture getRepositoryPermissionsPolicy(
GetRepositoryPermissionsPolicyRequest getRepositoryPermissionsPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the resource policy that is set on a repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetRepositoryPermissionsPolicyRequest.Builder}
* avoiding the need to create one manually via {@link GetRepositoryPermissionsPolicyRequest#builder()}
*
*
* @param getRepositoryPermissionsPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.GetRepositoryPermissionsPolicyRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.GetRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture getRepositoryPermissionsPolicy(
Consumer getRepositoryPermissionsPolicyRequest) {
return getRepositoryPermissionsPolicy(GetRepositoryPermissionsPolicyRequest.builder()
.applyMutation(getRepositoryPermissionsPolicyRequest).build());
}
/**
*
* Lists the repositories in the added repositories list of the specified restriction type for a package group. For
* more information about restriction types and added repository lists, see Package group origin
* controls in the CodeArtifact User Guide.
*
*
* @param listAllowedRepositoriesForGroupRequest
* @return A Java Future containing the result of the ListAllowedRepositoriesForGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAllowedRepositoriesForGroup
* @see AWS API Documentation
*/
default CompletableFuture listAllowedRepositoriesForGroup(
ListAllowedRepositoriesForGroupRequest listAllowedRepositoriesForGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the repositories in the added repositories list of the specified restriction type for a package group. For
* more information about restriction types and added repository lists, see Package group origin
* controls in the CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListAllowedRepositoriesForGroupRequest.Builder}
* avoiding the need to create one manually via {@link ListAllowedRepositoriesForGroupRequest#builder()}
*
*
* @param listAllowedRepositoriesForGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ListAllowedRepositoriesForGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAllowedRepositoriesForGroup
* @see AWS API Documentation
*/
default CompletableFuture listAllowedRepositoriesForGroup(
Consumer listAllowedRepositoriesForGroupRequest) {
return listAllowedRepositoriesForGroup(ListAllowedRepositoriesForGroupRequest.builder()
.applyMutation(listAllowedRepositoriesForGroupRequest).build());
}
/**
*
* This is a variant of
* {@link #listAllowedRepositoriesForGroup(software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAllowedRepositoriesForGroupPublisher publisher = client.listAllowedRepositoriesForGroupPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAllowedRepositoriesForGroupPublisher publisher = client.listAllowedRepositoriesForGroupPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAllowedRepositoriesForGroup(software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupRequest)}
* operation.
*
*
* @param listAllowedRepositoriesForGroupRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAllowedRepositoriesForGroup
* @see AWS API Documentation
*/
default ListAllowedRepositoriesForGroupPublisher listAllowedRepositoriesForGroupPaginator(
ListAllowedRepositoriesForGroupRequest listAllowedRepositoriesForGroupRequest) {
return new ListAllowedRepositoriesForGroupPublisher(this, listAllowedRepositoriesForGroupRequest);
}
/**
*
* This is a variant of
* {@link #listAllowedRepositoriesForGroup(software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAllowedRepositoriesForGroupPublisher publisher = client.listAllowedRepositoriesForGroupPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAllowedRepositoriesForGroupPublisher publisher = client.listAllowedRepositoriesForGroupPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAllowedRepositoriesForGroup(software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAllowedRepositoriesForGroupRequest.Builder}
* avoiding the need to create one manually via {@link ListAllowedRepositoriesForGroupRequest#builder()}
*
*
* @param listAllowedRepositoriesForGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListAllowedRepositoriesForGroupRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAllowedRepositoriesForGroup
* @see AWS API Documentation
*/
default ListAllowedRepositoriesForGroupPublisher listAllowedRepositoriesForGroupPaginator(
Consumer listAllowedRepositoriesForGroupRequest) {
return listAllowedRepositoriesForGroupPaginator(ListAllowedRepositoriesForGroupRequest.builder()
.applyMutation(listAllowedRepositoriesForGroupRequest).build());
}
/**
*
* Returns a list of packages associated with the requested package group. For information package group association
* and matching, see Package group definition syntax and matching behavior in the CodeArtifact User Guide.
*
*
* @param listAssociatedPackagesRequest
* @return A Java Future containing the result of the ListAssociatedPackages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAssociatedPackages
* @see AWS API Documentation
*/
default CompletableFuture listAssociatedPackages(
ListAssociatedPackagesRequest listAssociatedPackagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of packages associated with the requested package group. For information package group association
* and matching, see Package group definition syntax and matching behavior in the CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListAssociatedPackagesRequest.Builder} avoiding the
* need to create one manually via {@link ListAssociatedPackagesRequest#builder()}
*
*
* @param listAssociatedPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListAssociatedPackages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAssociatedPackages
* @see AWS API Documentation
*/
default CompletableFuture listAssociatedPackages(
Consumer listAssociatedPackagesRequest) {
return listAssociatedPackages(ListAssociatedPackagesRequest.builder().applyMutation(listAssociatedPackagesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listAssociatedPackages(software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAssociatedPackagesPublisher publisher = client.listAssociatedPackagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAssociatedPackagesPublisher publisher = client.listAssociatedPackagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAssociatedPackages(software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesRequest)}
* operation.
*
*
* @param listAssociatedPackagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAssociatedPackages
* @see AWS API Documentation
*/
default ListAssociatedPackagesPublisher listAssociatedPackagesPaginator(
ListAssociatedPackagesRequest listAssociatedPackagesRequest) {
return new ListAssociatedPackagesPublisher(this, listAssociatedPackagesRequest);
}
/**
*
* This is a variant of
* {@link #listAssociatedPackages(software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAssociatedPackagesPublisher publisher = client.listAssociatedPackagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListAssociatedPackagesPublisher publisher = client.listAssociatedPackagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAssociatedPackages(software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAssociatedPackagesRequest.Builder} avoiding the
* need to create one manually via {@link ListAssociatedPackagesRequest#builder()}
*
*
* @param listAssociatedPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListAssociatedPackagesRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListAssociatedPackages
* @see AWS API Documentation
*/
default ListAssociatedPackagesPublisher listAssociatedPackagesPaginator(
Consumer listAssociatedPackagesRequest) {
return listAssociatedPackagesPaginator(ListAssociatedPackagesRequest.builder()
.applyMutation(listAssociatedPackagesRequest).build());
}
/**
*
* Returns a list of DomainSummary objects for all domains owned by the Amazon Web Services account that makes this call. Each
* returned DomainSummary
object contains information about a domain.
*
*
* @param listDomainsRequest
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListDomains
* @see AWS API
* Documentation
*/
default CompletableFuture listDomains(ListDomainsRequest listDomainsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DomainSummary objects for all domains owned by the Amazon Web Services account that makes this call. Each
* returned DomainSummary
object contains information about a domain.
*
*
*
* This is a convenience which creates an instance of the {@link ListDomainsRequest.Builder} avoiding the need to
* create one manually via {@link ListDomainsRequest#builder()}
*
*
* @param listDomainsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListDomains
* @see AWS API
* Documentation
*/
default CompletableFuture listDomains(Consumer listDomainsRequest) {
return listDomains(ListDomainsRequest.builder().applyMutation(listDomainsRequest).build());
}
/**
*
* This is a variant of {@link #listDomains(software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListDomainsPublisher publisher = client.listDomainsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListDomainsPublisher publisher = client.listDomainsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListDomainsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDomains(software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest)} operation.
*
*
* @param listDomainsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListDomains
* @see AWS API
* Documentation
*/
default ListDomainsPublisher listDomainsPaginator(ListDomainsRequest listDomainsRequest) {
return new ListDomainsPublisher(this, listDomainsRequest);
}
/**
*
* This is a variant of {@link #listDomains(software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListDomainsPublisher publisher = client.listDomainsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListDomainsPublisher publisher = client.listDomainsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListDomainsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDomains(software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListDomainsRequest.Builder} avoiding the need to
* create one manually via {@link ListDomainsRequest#builder()}
*
*
* @param listDomainsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListDomainsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListDomains
* @see AWS API
* Documentation
*/
default ListDomainsPublisher listDomainsPaginator(Consumer listDomainsRequest) {
return listDomainsPaginator(ListDomainsRequest.builder().applyMutation(listDomainsRequest).build());
}
/**
*
* Returns a list of package groups in the requested domain.
*
*
* @param listPackageGroupsRequest
* @return A Java Future containing the result of the ListPackageGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageGroups
* @see AWS API Documentation
*/
default CompletableFuture listPackageGroups(ListPackageGroupsRequest listPackageGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of package groups in the requested domain.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackageGroupsRequest.Builder} avoiding the need
* to create one manually via {@link ListPackageGroupsRequest#builder()}
*
*
* @param listPackageGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPackageGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageGroups
* @see AWS API Documentation
*/
default CompletableFuture listPackageGroups(
Consumer listPackageGroupsRequest) {
return listPackageGroups(ListPackageGroupsRequest.builder().applyMutation(listPackageGroupsRequest).build());
}
/**
*
* This is a variant of
* {@link #listPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageGroupsPublisher publisher = client.listPackageGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageGroupsPublisher publisher = client.listPackageGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsRequest)}
* operation.
*
*
* @param listPackageGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageGroups
* @see AWS API Documentation
*/
default ListPackageGroupsPublisher listPackageGroupsPaginator(ListPackageGroupsRequest listPackageGroupsRequest) {
return new ListPackageGroupsPublisher(this, listPackageGroupsRequest);
}
/**
*
* This is a variant of
* {@link #listPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageGroupsPublisher publisher = client.listPackageGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageGroupsPublisher publisher = client.listPackageGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackageGroupsRequest.Builder} avoiding the need
* to create one manually via {@link ListPackageGroupsRequest#builder()}
*
*
* @param listPackageGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackageGroupsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageGroups
* @see AWS API Documentation
*/
default ListPackageGroupsPublisher listPackageGroupsPaginator(
Consumer listPackageGroupsRequest) {
return listPackageGroupsPaginator(ListPackageGroupsRequest.builder().applyMutation(listPackageGroupsRequest).build());
}
/**
*
* Returns a list of AssetSummary
* objects for assets in a package version.
*
*
* @param listPackageVersionAssetsRequest
* @return A Java Future containing the result of the ListPackageVersionAssets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionAssets
* @see AWS API Documentation
*/
default CompletableFuture listPackageVersionAssets(
ListPackageVersionAssetsRequest listPackageVersionAssetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of AssetSummary
* objects for assets in a package version.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackageVersionAssetsRequest.Builder} avoiding
* the need to create one manually via {@link ListPackageVersionAssetsRequest#builder()}
*
*
* @param listPackageVersionAssetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListPackageVersionAssets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionAssets
* @see AWS API Documentation
*/
default CompletableFuture listPackageVersionAssets(
Consumer listPackageVersionAssetsRequest) {
return listPackageVersionAssets(ListPackageVersionAssetsRequest.builder().applyMutation(listPackageVersionAssetsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listPackageVersionAssets(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsPublisher publisher = client.listPackageVersionAssetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsPublisher publisher = client.listPackageVersionAssetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageVersionAssets(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest)}
* operation.
*
*
* @param listPackageVersionAssetsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionAssets
* @see AWS API Documentation
*/
default ListPackageVersionAssetsPublisher listPackageVersionAssetsPaginator(
ListPackageVersionAssetsRequest listPackageVersionAssetsRequest) {
return new ListPackageVersionAssetsPublisher(this, listPackageVersionAssetsRequest);
}
/**
*
* This is a variant of
* {@link #listPackageVersionAssets(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsPublisher publisher = client.listPackageVersionAssetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionAssetsPublisher publisher = client.listPackageVersionAssetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageVersionAssets(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackageVersionAssetsRequest.Builder} avoiding
* the need to create one manually via {@link ListPackageVersionAssetsRequest#builder()}
*
*
* @param listPackageVersionAssetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackageVersionAssetsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionAssets
* @see AWS API Documentation
*/
default ListPackageVersionAssetsPublisher listPackageVersionAssetsPaginator(
Consumer listPackageVersionAssetsRequest) {
return listPackageVersionAssetsPaginator(ListPackageVersionAssetsRequest.builder()
.applyMutation(listPackageVersionAssetsRequest).build());
}
/**
*
* Returns the direct dependencies for a package version. The dependencies are returned as PackageDependency objects. CodeArtifact extracts the dependencies for a package version from the metadata
* file for the package format (for example, the package.json
file for npm packages and the
* pom.xml
file for Maven). Any package version dependencies that are not listed in the configuration
* file are not returned.
*
*
* @param listPackageVersionDependenciesRequest
* @return A Java Future containing the result of the ListPackageVersionDependencies operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionDependencies
* @see AWS API Documentation
*/
default CompletableFuture listPackageVersionDependencies(
ListPackageVersionDependenciesRequest listPackageVersionDependenciesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the direct dependencies for a package version. The dependencies are returned as PackageDependency objects. CodeArtifact extracts the dependencies for a package version from the metadata
* file for the package format (for example, the package.json
file for npm packages and the
* pom.xml
file for Maven). Any package version dependencies that are not listed in the configuration
* file are not returned.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackageVersionDependenciesRequest.Builder}
* avoiding the need to create one manually via {@link ListPackageVersionDependenciesRequest#builder()}
*
*
* @param listPackageVersionDependenciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackageVersionDependenciesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ListPackageVersionDependencies operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersionDependencies
* @see AWS API Documentation
*/
default CompletableFuture listPackageVersionDependencies(
Consumer listPackageVersionDependenciesRequest) {
return listPackageVersionDependencies(ListPackageVersionDependenciesRequest.builder()
.applyMutation(listPackageVersionDependenciesRequest).build());
}
/**
*
* Returns a list of PackageVersionSummary objects for package versions in a repository that match the request parameters.
* Package versions of all statuses will be returned by default when calling list-package-versions
with
* no --status
parameter.
*
*
* @param listPackageVersionsRequest
* @return A Java Future containing the result of the ListPackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersions
* @see AWS API Documentation
*/
default CompletableFuture listPackageVersions(
ListPackageVersionsRequest listPackageVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of PackageVersionSummary objects for package versions in a repository that match the request parameters.
* Package versions of all statuses will be returned by default when calling list-package-versions
with
* no --status
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackageVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPackageVersionsRequest#builder()}
*
*
* @param listPackageVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPackageVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersions
* @see AWS API Documentation
*/
default CompletableFuture listPackageVersions(
Consumer listPackageVersionsRequest) {
return listPackageVersions(ListPackageVersionsRequest.builder().applyMutation(listPackageVersionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listPackageVersions(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsPublisher publisher = client.listPackageVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsPublisher publisher = client.listPackageVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageVersions(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest)}
* operation.
*
*
* @param listPackageVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersions
* @see AWS API Documentation
*/
default ListPackageVersionsPublisher listPackageVersionsPaginator(ListPackageVersionsRequest listPackageVersionsRequest) {
return new ListPackageVersionsPublisher(this, listPackageVersionsRequest);
}
/**
*
* This is a variant of
* {@link #listPackageVersions(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsPublisher publisher = client.listPackageVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackageVersionsPublisher publisher = client.listPackageVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackageVersions(software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackageVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPackageVersionsRequest#builder()}
*
*
* @param listPackageVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackageVersionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackageVersions
* @see AWS API Documentation
*/
default ListPackageVersionsPublisher listPackageVersionsPaginator(
Consumer listPackageVersionsRequest) {
return listPackageVersionsPaginator(ListPackageVersionsRequest.builder().applyMutation(listPackageVersionsRequest)
.build());
}
/**
*
* Returns a list of PackageSummary
* objects for packages in a repository that match the request parameters.
*
*
* @param listPackagesRequest
* @return A Java Future containing the result of the ListPackages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackages
* @see AWS API
* Documentation
*/
default CompletableFuture listPackages(ListPackagesRequest listPackagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of PackageSummary
* objects for packages in a repository that match the request parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackagesRequest.Builder} avoiding the need to
* create one manually via {@link ListPackagesRequest#builder()}
*
*
* @param listPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPackages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackages
* @see AWS API
* Documentation
*/
default CompletableFuture listPackages(Consumer listPackagesRequest) {
return listPackages(ListPackagesRequest.builder().applyMutation(listPackagesRequest).build());
}
/**
*
* This is a variant of
* {@link #listPackages(software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackagesPublisher publisher = client.listPackagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackagesPublisher publisher = client.listPackagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackages(software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest)} operation.
*
*
* @param listPackagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackages
* @see AWS API
* Documentation
*/
default ListPackagesPublisher listPackagesPaginator(ListPackagesRequest listPackagesRequest) {
return new ListPackagesPublisher(this, listPackagesRequest);
}
/**
*
* This is a variant of
* {@link #listPackages(software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackagesPublisher publisher = client.listPackagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListPackagesPublisher publisher = client.listPackagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListPackagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackages(software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackagesRequest.Builder} avoiding the need to
* create one manually via {@link ListPackagesRequest#builder()}
*
*
* @param listPackagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListPackagesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListPackages
* @see AWS API
* Documentation
*/
default ListPackagesPublisher listPackagesPaginator(Consumer listPackagesRequest) {
return listPackagesPaginator(ListPackagesRequest.builder().applyMutation(listPackagesRequest).build());
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified Amazon Web Services account and that matches the input parameters.
*
*
* @param listRepositoriesRequest
* @return A Java Future containing the result of the ListRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositories
* @see AWS
* API Documentation
*/
default CompletableFuture listRepositories(ListRepositoriesRequest listRepositoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified Amazon Web Services account and that matches the input parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListRepositoriesRequest.Builder} avoiding the need
* to create one manually via {@link ListRepositoriesRequest#builder()}
*
*
* @param listRepositoriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositories
* @see AWS
* API Documentation
*/
default CompletableFuture listRepositories(
Consumer listRepositoriesRequest) {
return listRepositories(ListRepositoriesRequest.builder().applyMutation(listRepositoriesRequest).build());
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified domain and that matches the input parameters.
*
*
* @param listRepositoriesInDomainRequest
* @return A Java Future containing the result of the ListRepositoriesInDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositoriesInDomain
* @see AWS API Documentation
*/
default CompletableFuture listRepositoriesInDomain(
ListRepositoriesInDomainRequest listRepositoriesInDomainRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of RepositorySummary objects. Each RepositorySummary
contains information about a repository in
* the specified domain and that matches the input parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListRepositoriesInDomainRequest.Builder} avoiding
* the need to create one manually via {@link ListRepositoriesInDomainRequest#builder()}
*
*
* @param listRepositoriesInDomainRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListRepositoriesInDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositoriesInDomain
* @see AWS API Documentation
*/
default CompletableFuture listRepositoriesInDomain(
Consumer listRepositoriesInDomainRequest) {
return listRepositoriesInDomain(ListRepositoriesInDomainRequest.builder().applyMutation(listRepositoriesInDomainRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listRepositoriesInDomain(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainPublisher publisher = client.listRepositoriesInDomainPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainPublisher publisher = client.listRepositoriesInDomainPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositoriesInDomain(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest)}
* operation.
*
*
* @param listRepositoriesInDomainRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositoriesInDomain
* @see AWS API Documentation
*/
default ListRepositoriesInDomainPublisher listRepositoriesInDomainPaginator(
ListRepositoriesInDomainRequest listRepositoriesInDomainRequest) {
return new ListRepositoriesInDomainPublisher(this, listRepositoriesInDomainRequest);
}
/**
*
* This is a variant of
* {@link #listRepositoriesInDomain(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainPublisher publisher = client.listRepositoriesInDomainPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesInDomainPublisher publisher = client.listRepositoriesInDomainPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositoriesInDomain(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListRepositoriesInDomainRequest.Builder} avoiding
* the need to create one manually via {@link ListRepositoriesInDomainRequest#builder()}
*
*
* @param listRepositoriesInDomainRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListRepositoriesInDomainRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositoriesInDomain
* @see AWS API Documentation
*/
default ListRepositoriesInDomainPublisher listRepositoriesInDomainPaginator(
Consumer listRepositoriesInDomainRequest) {
return listRepositoriesInDomainPaginator(ListRepositoriesInDomainRequest.builder()
.applyMutation(listRepositoriesInDomainRequest).build());
}
/**
*
* This is a variant of
* {@link #listRepositories(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesPublisher publisher = client.listRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesPublisher publisher = client.listRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositories(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest)}
* operation.
*
*
* @param listRepositoriesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositories
* @see AWS
* API Documentation
*/
default ListRepositoriesPublisher listRepositoriesPaginator(ListRepositoriesRequest listRepositoriesRequest) {
return new ListRepositoriesPublisher(this, listRepositoriesRequest);
}
/**
*
* This is a variant of
* {@link #listRepositories(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesPublisher publisher = client.listRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListRepositoriesPublisher publisher = client.listRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositories(software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListRepositoriesRequest.Builder} avoiding the need
* to create one manually via {@link ListRepositoriesRequest#builder()}
*
*
* @param listRepositoriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListRepositoriesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListRepositories
* @see AWS
* API Documentation
*/
default ListRepositoriesPublisher listRepositoriesPaginator(Consumer listRepositoriesRequest) {
return listRepositoriesPaginator(ListRepositoriesRequest.builder().applyMutation(listRepositoriesRequest).build());
}
/**
*
* Returns a list of direct children of the specified package group.
*
*
* For information package group hierarchy, see Package group definition syntax and matching behavior in the CodeArtifact User Guide.
*
*
* @param listSubPackageGroupsRequest
* @return A Java Future containing the result of the ListSubPackageGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListSubPackageGroups
* @see AWS API Documentation
*/
default CompletableFuture listSubPackageGroups(
ListSubPackageGroupsRequest listSubPackageGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of direct children of the specified package group.
*
*
* For information package group hierarchy, see Package group definition syntax and matching behavior in the CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListSubPackageGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListSubPackageGroupsRequest#builder()}
*
*
* @param listSubPackageGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListSubPackageGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListSubPackageGroups
* @see AWS API Documentation
*/
default CompletableFuture listSubPackageGroups(
Consumer listSubPackageGroupsRequest) {
return listSubPackageGroups(ListSubPackageGroupsRequest.builder().applyMutation(listSubPackageGroupsRequest).build());
}
/**
*
* This is a variant of
* {@link #listSubPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListSubPackageGroupsPublisher publisher = client.listSubPackageGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListSubPackageGroupsPublisher publisher = client.listSubPackageGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSubPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsRequest)}
* operation.
*
*
* @param listSubPackageGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListSubPackageGroups
* @see AWS API Documentation
*/
default ListSubPackageGroupsPublisher listSubPackageGroupsPaginator(ListSubPackageGroupsRequest listSubPackageGroupsRequest) {
return new ListSubPackageGroupsPublisher(this, listSubPackageGroupsRequest);
}
/**
*
* This is a variant of
* {@link #listSubPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListSubPackageGroupsPublisher publisher = client.listSubPackageGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codeartifact.paginators.ListSubPackageGroupsPublisher publisher = client.listSubPackageGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSubPackageGroups(software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSubPackageGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListSubPackageGroupsRequest#builder()}
*
*
* @param listSubPackageGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListSubPackageGroupsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListSubPackageGroups
* @see AWS API Documentation
*/
default ListSubPackageGroupsPublisher listSubPackageGroupsPaginator(
Consumer listSubPackageGroupsRequest) {
return listSubPackageGroupsPaginator(ListSubPackageGroupsRequest.builder().applyMutation(listSubPackageGroupsRequest)
.build());
}
/**
*
* Gets information about Amazon Web Services tags for a specified Amazon Resource Name (ARN) in CodeArtifact.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about Amazon Web Services tags for a specified Amazon Resource Name (ARN) in CodeArtifact.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Creates a new package version containing one or more assets (or files).
*
*
* The unfinished
flag can be used to keep the package version in the Unfinished
state
* until all of its assets have been uploaded (see Package version status in the CodeArtifact user guide). To set the package version’s status to
* Published
, omit the unfinished
flag when uploading the final asset, or set the status
* using
* UpdatePackageVersionStatus. Once a package version’s status is set to Published
, it cannot
* change back to Unfinished
.
*
*
*
* Only generic packages can be published using this API. For more information, see Using generic packages in the
* CodeArtifact User Guide.
*
*
*
* @param publishPackageVersionRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The content of the asset to publish.
*
* '
* @return A Java Future containing the result of the PublishPackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PublishPackageVersion
* @see AWS API Documentation
*/
default CompletableFuture publishPackageVersion(
PublishPackageVersionRequest publishPackageVersionRequest, AsyncRequestBody requestBody) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new package version containing one or more assets (or files).
*
*
* The unfinished
flag can be used to keep the package version in the Unfinished
state
* until all of its assets have been uploaded (see Package version status in the CodeArtifact user guide). To set the package version’s status to
* Published
, omit the unfinished
flag when uploading the final asset, or set the status
* using
* UpdatePackageVersionStatus. Once a package version’s status is set to Published
, it cannot
* change back to Unfinished
.
*
*
*
* Only generic packages can be published using this API. For more information, see Using generic packages in the
* CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PublishPackageVersionRequest.Builder} avoiding the
* need to create one manually via {@link PublishPackageVersionRequest#builder()}
*
*
* @param publishPackageVersionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.PublishPackageVersionRequest.Builder} to create
* a request.
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The content of the asset to publish.
*
* '
* @return A Java Future containing the result of the PublishPackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PublishPackageVersion
* @see AWS API Documentation
*/
default CompletableFuture publishPackageVersion(
Consumer publishPackageVersionRequest, AsyncRequestBody requestBody) {
return publishPackageVersion(PublishPackageVersionRequest.builder().applyMutation(publishPackageVersionRequest).build(),
requestBody);
}
/**
*
* Creates a new package version containing one or more assets (or files).
*
*
* The unfinished
flag can be used to keep the package version in the Unfinished
state
* until all of its assets have been uploaded (see Package version status in the CodeArtifact user guide). To set the package version’s status to
* Published
, omit the unfinished
flag when uploading the final asset, or set the status
* using
* UpdatePackageVersionStatus. Once a package version’s status is set to Published
, it cannot
* change back to Unfinished
.
*
*
*
* Only generic packages can be published using this API. For more information, see Using generic packages in the
* CodeArtifact User Guide.
*
*
*
* @param publishPackageVersionRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The content of the asset to publish.
*
* '
* @return A Java Future containing the result of the PublishPackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PublishPackageVersion
* @see AWS API Documentation
*/
default CompletableFuture publishPackageVersion(
PublishPackageVersionRequest publishPackageVersionRequest, Path sourcePath) {
return publishPackageVersion(publishPackageVersionRequest, AsyncRequestBody.fromFile(sourcePath));
}
/**
*
* Creates a new package version containing one or more assets (or files).
*
*
* The unfinished
flag can be used to keep the package version in the Unfinished
state
* until all of its assets have been uploaded (see Package version status in the CodeArtifact user guide). To set the package version’s status to
* Published
, omit the unfinished
flag when uploading the final asset, or set the status
* using
* UpdatePackageVersionStatus. Once a package version’s status is set to Published
, it cannot
* change back to Unfinished
.
*
*
*
* Only generic packages can be published using this API. For more information, see Using generic packages in the
* CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PublishPackageVersionRequest.Builder} avoiding the
* need to create one manually via {@link PublishPackageVersionRequest#builder()}
*
*
* @param publishPackageVersionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.PublishPackageVersionRequest.Builder} to create
* a request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The content of the asset to publish.
*
* '
* @return A Java Future containing the result of the PublishPackageVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PublishPackageVersion
* @see AWS API Documentation
*/
default CompletableFuture publishPackageVersion(
Consumer publishPackageVersionRequest, Path sourcePath) {
return publishPackageVersion(PublishPackageVersionRequest.builder().applyMutation(publishPackageVersionRequest).build(),
sourcePath);
}
/**
*
* Sets a resource policy on a domain that specifies permissions to access it.
*
*
* When you call PutDomainPermissionsPolicy
, the resource policy on the domain is ignored when
* evaluting permissions. This ensures that the owner of a domain cannot lock themselves out of the domain, which
* would prevent them from being able to update the resource policy.
*
*
* @param putDomainPermissionsPolicyRequest
* @return A Java Future containing the result of the PutDomainPermissionsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutDomainPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture putDomainPermissionsPolicy(
PutDomainPermissionsPolicyRequest putDomainPermissionsPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets a resource policy on a domain that specifies permissions to access it.
*
*
* When you call PutDomainPermissionsPolicy
, the resource policy on the domain is ignored when
* evaluting permissions. This ensures that the owner of a domain cannot lock themselves out of the domain, which
* would prevent them from being able to update the resource policy.
*
*
*
* This is a convenience which creates an instance of the {@link PutDomainPermissionsPolicyRequest.Builder} avoiding
* the need to create one manually via {@link PutDomainPermissionsPolicyRequest#builder()}
*
*
* @param putDomainPermissionsPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.PutDomainPermissionsPolicyRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the PutDomainPermissionsPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutDomainPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture putDomainPermissionsPolicy(
Consumer putDomainPermissionsPolicyRequest) {
return putDomainPermissionsPolicy(PutDomainPermissionsPolicyRequest.builder()
.applyMutation(putDomainPermissionsPolicyRequest).build());
}
/**
*
* Sets the package origin configuration for a package.
*
*
* The package origin configuration determines how new versions of a package can be added to a repository. You can
* allow or block direct publishing of new package versions, or ingestion and retaining of new package versions from
* an external connection or upstream source. For more information about package origin controls and configuration,
* see Editing package
* origin controls in the CodeArtifact User Guide.
*
*
* PutPackageOriginConfiguration
can be called on a package that doesn't yet exist in the repository.
* When called on a package that does not exist, a package is created in the repository with no versions and the
* requested restrictions are set on the package. This can be used to preemptively block ingesting or retaining any
* versions from external connections or upstream repositories, or to block publishing any versions of the package
* into the repository before connecting any package managers or publishers to the repository.
*
*
* @param putPackageOriginConfigurationRequest
* @return A Java Future containing the result of the PutPackageOriginConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutPackageOriginConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putPackageOriginConfiguration(
PutPackageOriginConfigurationRequest putPackageOriginConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets the package origin configuration for a package.
*
*
* The package origin configuration determines how new versions of a package can be added to a repository. You can
* allow or block direct publishing of new package versions, or ingestion and retaining of new package versions from
* an external connection or upstream source. For more information about package origin controls and configuration,
* see Editing package
* origin controls in the CodeArtifact User Guide.
*
*
* PutPackageOriginConfiguration
can be called on a package that doesn't yet exist in the repository.
* When called on a package that does not exist, a package is created in the repository with no versions and the
* requested restrictions are set on the package. This can be used to preemptively block ingesting or retaining any
* versions from external connections or upstream repositories, or to block publishing any versions of the package
* into the repository before connecting any package managers or publishers to the repository.
*
*
*
* This is a convenience which creates an instance of the {@link PutPackageOriginConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutPackageOriginConfigurationRequest#builder()}
*
*
* @param putPackageOriginConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.PutPackageOriginConfigurationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the PutPackageOriginConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutPackageOriginConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putPackageOriginConfiguration(
Consumer putPackageOriginConfigurationRequest) {
return putPackageOriginConfiguration(PutPackageOriginConfigurationRequest.builder()
.applyMutation(putPackageOriginConfigurationRequest).build());
}
/**
*
* Sets the resource policy on a repository that specifies permissions to access it.
*
*
* When you call PutRepositoryPermissionsPolicy
, the resource policy on the repository is ignored when
* evaluting permissions. This ensures that the owner of a repository cannot lock themselves out of the repository,
* which would prevent them from being able to update the resource policy.
*
*
* @param putRepositoryPermissionsPolicyRequest
* @return A Java Future containing the result of the PutRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture putRepositoryPermissionsPolicy(
PutRepositoryPermissionsPolicyRequest putRepositoryPermissionsPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets the resource policy on a repository that specifies permissions to access it.
*
*
* When you call PutRepositoryPermissionsPolicy
, the resource policy on the repository is ignored when
* evaluting permissions. This ensures that the owner of a repository cannot lock themselves out of the repository,
* which would prevent them from being able to update the resource policy.
*
*
*
* This is a convenience which creates an instance of the {@link PutRepositoryPermissionsPolicyRequest.Builder}
* avoiding the need to create one manually via {@link PutRepositoryPermissionsPolicyRequest#builder()}
*
*
* @param putRepositoryPermissionsPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.PutRepositoryPermissionsPolicyRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the PutRepositoryPermissionsPolicy operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.PutRepositoryPermissionsPolicy
* @see AWS API Documentation
*/
default CompletableFuture putRepositoryPermissionsPolicy(
Consumer putRepositoryPermissionsPolicyRequest) {
return putRepositoryPermissionsPolicy(PutRepositoryPermissionsPolicyRequest.builder()
.applyMutation(putRepositoryPermissionsPolicyRequest).build());
}
/**
*
* Adds or updates tags for a resource in CodeArtifact.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates tags for a resource in CodeArtifact.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from a resource in CodeArtifact.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a resource in CodeArtifact.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a package group. This API cannot be used to update a package group's origin configuration or pattern. To
* update a package group's origin configuration, use UpdatePackageGroupOriginConfiguration.
*
*
* @param updatePackageGroupRequest
* @return A Java Future containing the result of the UpdatePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdatePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture updatePackageGroup(UpdatePackageGroupRequest updatePackageGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a package group. This API cannot be used to update a package group's origin configuration or pattern. To
* update a package group's origin configuration, use UpdatePackageGroupOriginConfiguration.
*
*
*
* This is a convenience which creates an instance of the {@link UpdatePackageGroupRequest.Builder} avoiding the
* need to create one manually via {@link UpdatePackageGroupRequest#builder()}
*
*
* @param updatePackageGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.UpdatePackageGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdatePackageGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdatePackageGroup
* @see AWS API Documentation
*/
default CompletableFuture updatePackageGroup(
Consumer updatePackageGroupRequest) {
return updatePackageGroup(UpdatePackageGroupRequest.builder().applyMutation(updatePackageGroupRequest).build());
}
/**
*
* Updates the package origin configuration for a package group.
*
*
* The package origin configuration determines how new versions of a package can be added to a repository. You can
* allow or block direct publishing of new package versions, or ingestion and retaining of new package versions from
* an external connection or upstream source. For more information about package group origin controls and
* configuration, see Package group origin
* controls in the CodeArtifact User Guide.
*
*
* @param updatePackageGroupOriginConfigurationRequest
* @return A Java Future containing the result of the UpdatePackageGroupOriginConfiguration operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdatePackageGroupOriginConfiguration
* @see AWS API Documentation
*/
default CompletableFuture updatePackageGroupOriginConfiguration(
UpdatePackageGroupOriginConfigurationRequest updatePackageGroupOriginConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the package origin configuration for a package group.
*
*
* The package origin configuration determines how new versions of a package can be added to a repository. You can
* allow or block direct publishing of new package versions, or ingestion and retaining of new package versions from
* an external connection or upstream source. For more information about package group origin controls and
* configuration, see Package group origin
* controls in the CodeArtifact User Guide.
*
*
*
* This is a convenience which creates an instance of the
* {@link UpdatePackageGroupOriginConfigurationRequest.Builder} avoiding the need to create one manually via
* {@link UpdatePackageGroupOriginConfigurationRequest#builder()}
*
*
* @param updatePackageGroupOriginConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.UpdatePackageGroupOriginConfigurationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the UpdatePackageGroupOriginConfiguration operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdatePackageGroupOriginConfiguration
* @see AWS API Documentation
*/
default CompletableFuture updatePackageGroupOriginConfiguration(
Consumer updatePackageGroupOriginConfigurationRequest) {
return updatePackageGroupOriginConfiguration(UpdatePackageGroupOriginConfigurationRequest.builder()
.applyMutation(updatePackageGroupOriginConfigurationRequest).build());
}
/**
*
* Updates the status of one or more versions of a package. Using UpdatePackageVersionsStatus
, you can
* update the status of package versions to Archived
, Published
, or Unlisted
.
* To set the status of a package version to Disposed
, use DisposePackageVersions.
*
*
* @param updatePackageVersionsStatusRequest
* @return A Java Future containing the result of the UpdatePackageVersionsStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdatePackageVersionsStatus
* @see AWS API Documentation
*/
default CompletableFuture updatePackageVersionsStatus(
UpdatePackageVersionsStatusRequest updatePackageVersionsStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the status of one or more versions of a package. Using UpdatePackageVersionsStatus
, you can
* update the status of package versions to Archived
, Published
, or Unlisted
.
* To set the status of a package version to Disposed
, use DisposePackageVersions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdatePackageVersionsStatusRequest.Builder}
* avoiding the need to create one manually via {@link UpdatePackageVersionsStatusRequest#builder()}
*
*
* @param updatePackageVersionsStatusRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.UpdatePackageVersionsStatusRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdatePackageVersionsStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdatePackageVersionsStatus
* @see AWS API Documentation
*/
default CompletableFuture updatePackageVersionsStatus(
Consumer updatePackageVersionsStatusRequest) {
return updatePackageVersionsStatus(UpdatePackageVersionsStatusRequest.builder()
.applyMutation(updatePackageVersionsStatusRequest).build());
}
/**
*
* Update the properties of a repository.
*
*
* @param updateRepositoryRequest
* @return A Java Future containing the result of the UpdateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdateRepository
* @see AWS
* API Documentation
*/
default CompletableFuture updateRepository(UpdateRepositoryRequest updateRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Update the properties of a repository.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link UpdateRepositoryRequest#builder()}
*
*
* @param updateRepositoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.codeartifact.model.UpdateRepositoryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException The operation did not succeed because of an unauthorized access attempt.
* - ConflictException The operation did not succeed because prerequisites are not met.
* - InternalServerException The operation did not succeed because of an error that occurred inside
* CodeArtifact.
* - ResourceNotFoundException The operation did not succeed because the resource requested is not found
* in the service.
* - ServiceQuotaExceededException The operation did not succeed because it would have exceeded a service
* limit for your account.
* - ThrottlingException The operation did not succeed because too many requests are sent to the service.
* - ValidationException The operation did not succeed because a parameter in the request was sent with an
* invalid value.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeartifactException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeartifactAsyncClient.UpdateRepository
* @see AWS
* API Documentation
*/
default CompletableFuture updateRepository(
Consumer updateRepositoryRequest) {
return updateRepository(UpdateRepositoryRequest.builder().applyMutation(updateRepositoryRequest).build());
}
@Override
default CodeartifactServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link CodeartifactAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CodeartifactAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CodeartifactAsyncClient}.
*/
static CodeartifactAsyncClientBuilder builder() {
return new DefaultCodeartifactAsyncClientBuilder();
}
}