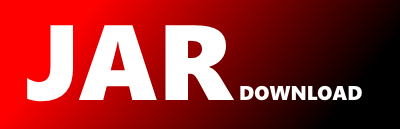
software.amazon.awssdk.services.codebuild.model.ProjectEnvironment Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the build environment of the build project.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProjectEnvironment implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ProjectEnvironment::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField IMAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ProjectEnvironment::image)).setter(setter(Builder::image))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("image").build()).build();
private static final SdkField COMPUTE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ProjectEnvironment::computeTypeAsString)).setter(setter(Builder::computeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("computeType").build()).build();
private static final SdkField> ENVIRONMENT_VARIABLES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ProjectEnvironment::environmentVariables))
.setter(setter(Builder::environmentVariables))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environmentVariables").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EnvironmentVariable::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PRIVILEGED_MODE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(ProjectEnvironment::privilegedMode)).setter(setter(Builder::privilegedMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("privilegedMode").build()).build();
private static final SdkField CERTIFICATE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ProjectEnvironment::certificate)).setter(setter(Builder::certificate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificate").build()).build();
private static final SdkField REGISTRY_CREDENTIAL_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(ProjectEnvironment::registryCredential))
.setter(setter(Builder::registryCredential)).constructor(RegistryCredential::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryCredential").build())
.build();
private static final SdkField IMAGE_PULL_CREDENTIALS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ProjectEnvironment::imagePullCredentialsTypeAsString))
.setter(setter(Builder::imagePullCredentialsType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imagePullCredentialsType").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD, IMAGE_FIELD,
COMPUTE_TYPE_FIELD, ENVIRONMENT_VARIABLES_FIELD, PRIVILEGED_MODE_FIELD, CERTIFICATE_FIELD, REGISTRY_CREDENTIAL_FIELD,
IMAGE_PULL_CREDENTIALS_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String type;
private final String image;
private final String computeType;
private final List environmentVariables;
private final Boolean privilegedMode;
private final String certificate;
private final RegistryCredential registryCredential;
private final String imagePullCredentialsType;
private ProjectEnvironment(BuilderImpl builder) {
this.type = builder.type;
this.image = builder.image;
this.computeType = builder.computeType;
this.environmentVariables = builder.environmentVariables;
this.privilegedMode = builder.privilegedMode;
this.certificate = builder.certificate;
this.registryCredential = builder.registryCredential;
this.imagePullCredentialsType = builder.imagePullCredentialsType;
}
/**
*
* The type of build environment to use for related builds.
*
*
* -
*
* The environment type ARM_CONTAINER
is available only in regions US East (N. Virginia), US East
* (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific (Sydney), and
* EU (Frankfurt).
*
*
* -
*
* The environment type LINUX_CONTAINER
with compute type build.general1.2xlarge
is
* available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), Canada (Central), EU
* (Ireland), EU (London), EU (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore),
* Asia Pacific (Sydney), China (Beijing), and China (Ningxia).
*
*
* -
*
* The environment type LINUX_GPU_CONTAINER
is available only in regions US East (N. Virginia), US East
* (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU (Frankfurt), Asia Pacific (Tokyo), Asia
* Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific (Sydney) , China (Beijing), and China (Ningxia).
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link EnvironmentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of build environment to use for related builds.
*
* -
*
* The environment type ARM_CONTAINER
is available only in regions US East (N. Virginia), US
* East (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific
* (Sydney), and EU (Frankfurt).
*
*
* -
*
* The environment type LINUX_CONTAINER
with compute type build.general1.2xlarge
* is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), Canada (Central),
* EU (Ireland), EU (London), EU (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific
* (Singapore), Asia Pacific (Sydney), China (Beijing), and China (Ningxia).
*
*
* -
*
* The environment type LINUX_GPU_CONTAINER
is available only in regions US East (N. Virginia),
* US East (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU (Frankfurt), Asia
* Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific (Sydney) , China (Beijing),
* and China (Ningxia).
*
*
* @see EnvironmentType
*/
public EnvironmentType type() {
return EnvironmentType.fromValue(type);
}
/**
*
* The type of build environment to use for related builds.
*
*
* -
*
* The environment type ARM_CONTAINER
is available only in regions US East (N. Virginia), US East
* (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific (Sydney), and
* EU (Frankfurt).
*
*
* -
*
* The environment type LINUX_CONTAINER
with compute type build.general1.2xlarge
is
* available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), Canada (Central), EU
* (Ireland), EU (London), EU (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore),
* Asia Pacific (Sydney), China (Beijing), and China (Ningxia).
*
*
* -
*
* The environment type LINUX_GPU_CONTAINER
is available only in regions US East (N. Virginia), US East
* (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU (Frankfurt), Asia Pacific (Tokyo), Asia
* Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific (Sydney) , China (Beijing), and China (Ningxia).
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link EnvironmentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of build environment to use for related builds.
*
* -
*
* The environment type ARM_CONTAINER
is available only in regions US East (N. Virginia), US
* East (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific
* (Sydney), and EU (Frankfurt).
*
*
* -
*
* The environment type LINUX_CONTAINER
with compute type build.general1.2xlarge
* is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), Canada (Central),
* EU (Ireland), EU (London), EU (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific
* (Singapore), Asia Pacific (Sydney), China (Beijing), and China (Ningxia).
*
*
* -
*
* The environment type LINUX_GPU_CONTAINER
is available only in regions US East (N. Virginia),
* US East (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU (Frankfurt), Asia
* Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific (Sydney) , China (Beijing),
* and China (Ningxia).
*
*
* @see EnvironmentType
*/
public String typeAsString() {
return type;
}
/**
*
* The image tag or image digest that identifies the Docker image to use for this build project. Use the following
* formats:
*
*
* -
*
* For an image tag: registry/repository:tag
. For example, to specify an image with the tag "latest,"
* use registry/repository:latest
.
*
*
* -
*
* For an image digest: registry/repository@digest
. For example, to specify an image with the digest
* "sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf," use
* registry/repository@sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf
.
*
*
*
*
* @return The image tag or image digest that identifies the Docker image to use for this build project. Use the
* following formats:
*
* -
*
* For an image tag: registry/repository:tag
. For example, to specify an image with the tag
* "latest," use registry/repository:latest
.
*
*
* -
*
* For an image digest: registry/repository@digest
. For example, to specify an image with the
* digest "sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf," use
* registry/repository@sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf
.
*
*
*/
public String image() {
return image;
}
/**
*
* Information about the compute resources the build project uses. Available values include:
*
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your environment
* type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for builds.
* This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and 4 NVIDIA
* Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on ARM-based
* processors for builds.
*
*
*
*
* For more information, see Build Environment
* Compute Types in the AWS CodeBuild User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #computeType} will
* return {@link ComputeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #computeTypeAsString}.
*
*
* @return Information about the compute resources the build project uses. Available values include:
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your
* environment type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for
* builds. This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and 4
* NVIDIA Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on ARM-based
* processors for builds.
*
*
*
*
* For more information, see Build
* Environment Compute Types in the AWS CodeBuild User Guide.
* @see ComputeType
*/
public ComputeType computeType() {
return ComputeType.fromValue(computeType);
}
/**
*
* Information about the compute resources the build project uses. Available values include:
*
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your environment
* type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for builds.
* This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and 4 NVIDIA
* Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on ARM-based
* processors for builds.
*
*
*
*
* For more information, see Build Environment
* Compute Types in the AWS CodeBuild User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #computeType} will
* return {@link ComputeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #computeTypeAsString}.
*
*
* @return Information about the compute resources the build project uses. Available values include:
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your
* environment type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for
* builds. This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and 4
* NVIDIA Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on ARM-based
* processors for builds.
*
*
*
*
* For more information, see Build
* Environment Compute Types in the AWS CodeBuild User Guide.
* @see ComputeType
*/
public String computeTypeAsString() {
return computeType;
}
/**
* Returns true if the EnvironmentVariables property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasEnvironmentVariables() {
return environmentVariables != null && !(environmentVariables instanceof SdkAutoConstructList);
}
/**
*
* A set of environment variables to make available to builds for this build project.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasEnvironmentVariables()} to see if a value was sent in this field.
*
*
* @return A set of environment variables to make available to builds for this build project.
*/
public List environmentVariables() {
return environmentVariables;
}
/**
*
* Enables running the Docker daemon inside a Docker container. Set to true only if the build project is used to
* build Docker images. Otherwise, a build that attempts to interact with the Docker daemon fails. The default
* setting is false
.
*
*
* You can initialize the Docker daemon during the install phase of your build by adding one of the following sets
* of commands to the install phase of your buildspec file:
*
*
* If the operating system's base image is Ubuntu Linux:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout 15 sh -c "until docker info; do echo .; sleep 1; done"
*
*
* If the operating system's base image is Alpine Linux and the previous command does not work, add the
* -t
argument to timeout
:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout -t 15 sh -c "until docker info; do echo .; sleep 1; done"
*
*
* @return Enables running the Docker daemon inside a Docker container. Set to true only if the build project is
* used to build Docker images. Otherwise, a build that attempts to interact with the Docker daemon fails.
* The default setting is false
.
*
* You can initialize the Docker daemon during the install phase of your build by adding one of the
* following sets of commands to the install phase of your buildspec file:
*
*
* If the operating system's base image is Ubuntu Linux:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout 15 sh -c "until docker info; do echo .; sleep 1; done"
*
*
* If the operating system's base image is Alpine Linux and the previous command does not work, add the
* -t
argument to timeout
:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout -t 15 sh -c "until docker info; do echo .; sleep 1; done"
*/
public Boolean privilegedMode() {
return privilegedMode;
}
/**
*
* The certificate to use with this build project.
*
*
* @return The certificate to use with this build project.
*/
public String certificate() {
return certificate;
}
/**
*
* The credentials for access to a private registry.
*
*
* @return The credentials for access to a private registry.
*/
public RegistryCredential registryCredential() {
return registryCredential;
}
/**
*
* The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you modify your
* ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When you use an
* AWS CodeBuild curated image, you must use CODEBUILD credentials.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imagePullCredentialsType} will return {@link ImagePullCredentialsType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #imagePullCredentialsTypeAsString}.
*
*
* @return The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you
* modify your ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When you
* use an AWS CodeBuild curated image, you must use CODEBUILD credentials.
* @see ImagePullCredentialsType
*/
public ImagePullCredentialsType imagePullCredentialsType() {
return ImagePullCredentialsType.fromValue(imagePullCredentialsType);
}
/**
*
* The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you modify your
* ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When you use an
* AWS CodeBuild curated image, you must use CODEBUILD credentials.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imagePullCredentialsType} will return {@link ImagePullCredentialsType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #imagePullCredentialsTypeAsString}.
*
*
* @return The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you
* modify your ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When you
* use an AWS CodeBuild curated image, you must use CODEBUILD credentials.
* @see ImagePullCredentialsType
*/
public String imagePullCredentialsTypeAsString() {
return imagePullCredentialsType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(image());
hashCode = 31 * hashCode + Objects.hashCode(computeTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(environmentVariables());
hashCode = 31 * hashCode + Objects.hashCode(privilegedMode());
hashCode = 31 * hashCode + Objects.hashCode(certificate());
hashCode = 31 * hashCode + Objects.hashCode(registryCredential());
hashCode = 31 * hashCode + Objects.hashCode(imagePullCredentialsTypeAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProjectEnvironment)) {
return false;
}
ProjectEnvironment other = (ProjectEnvironment) obj;
return Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(image(), other.image())
&& Objects.equals(computeTypeAsString(), other.computeTypeAsString())
&& Objects.equals(environmentVariables(), other.environmentVariables())
&& Objects.equals(privilegedMode(), other.privilegedMode()) && Objects.equals(certificate(), other.certificate())
&& Objects.equals(registryCredential(), other.registryCredential())
&& Objects.equals(imagePullCredentialsTypeAsString(), other.imagePullCredentialsTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ProjectEnvironment").add("Type", typeAsString()).add("Image", image())
.add("ComputeType", computeTypeAsString()).add("EnvironmentVariables", environmentVariables())
.add("PrivilegedMode", privilegedMode()).add("Certificate", certificate())
.add("RegistryCredential", registryCredential())
.add("ImagePullCredentialsType", imagePullCredentialsTypeAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "image":
return Optional.ofNullable(clazz.cast(image()));
case "computeType":
return Optional.ofNullable(clazz.cast(computeTypeAsString()));
case "environmentVariables":
return Optional.ofNullable(clazz.cast(environmentVariables()));
case "privilegedMode":
return Optional.ofNullable(clazz.cast(privilegedMode()));
case "certificate":
return Optional.ofNullable(clazz.cast(certificate()));
case "registryCredential":
return Optional.ofNullable(clazz.cast(registryCredential()));
case "imagePullCredentialsType":
return Optional.ofNullable(clazz.cast(imagePullCredentialsTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* The environment type ARM_CONTAINER
is available only in regions US East (N. Virginia), US
* East (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific
* (Sydney), and EU (Frankfurt).
*
*
* -
*
* The environment type LINUX_CONTAINER
with compute type
* build.general1.2xlarge
is available only in regions US East (N. Virginia), US East
* (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU (Frankfurt), Asia Pacific
* (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific (Sydney), China (Beijing), and
* China (Ningxia).
*
*
* -
*
* The environment type LINUX_GPU_CONTAINER
is available only in regions US East (N.
* Virginia), US East (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU
* (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific
* (Sydney) , China (Beijing), and China (Ningxia).
*
*
* @see EnvironmentType
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnvironmentType
*/
Builder type(String type);
/**
*
* The type of build environment to use for related builds.
*
*
* -
*
* The environment type ARM_CONTAINER
is available only in regions US East (N. Virginia), US East
* (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific (Sydney),
* and EU (Frankfurt).
*
*
* -
*
* The environment type LINUX_CONTAINER
with compute type build.general1.2xlarge
is
* available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), Canada (Central), EU
* (Ireland), EU (London), EU (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore),
* Asia Pacific (Sydney), China (Beijing), and China (Ningxia).
*
*
* -
*
* The environment type LINUX_GPU_CONTAINER
is available only in regions US East (N. Virginia), US
* East (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU (Frankfurt), Asia Pacific
* (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific (Sydney) , China (Beijing), and China
* (Ningxia).
*
*
*
*
* @param type
* The type of build environment to use for related builds.
*
* -
*
* The environment type ARM_CONTAINER
is available only in regions US East (N. Virginia), US
* East (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific
* (Sydney), and EU (Frankfurt).
*
*
* -
*
* The environment type LINUX_CONTAINER
with compute type
* build.general1.2xlarge
is available only in regions US East (N. Virginia), US East
* (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU (Frankfurt), Asia Pacific
* (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific (Sydney), China (Beijing), and
* China (Ningxia).
*
*
* -
*
* The environment type LINUX_GPU_CONTAINER
is available only in regions US East (N.
* Virginia), US East (Ohio), US West (Oregon), Canada (Central), EU (Ireland), EU (London), EU
* (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Seoul), Asia Pacific (Singapore), Asia Pacific
* (Sydney) , China (Beijing), and China (Ningxia).
*
*
* @see EnvironmentType
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnvironmentType
*/
Builder type(EnvironmentType type);
/**
*
* The image tag or image digest that identifies the Docker image to use for this build project. Use the
* following formats:
*
*
* -
*
* For an image tag: registry/repository:tag
. For example, to specify an image with the tag
* "latest," use registry/repository:latest
.
*
*
* -
*
* For an image digest: registry/repository@digest
. For example, to specify an image with the
* digest "sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf," use
* registry/repository@sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf
.
*
*
*
*
* @param image
* The image tag or image digest that identifies the Docker image to use for this build project. Use the
* following formats:
*
* -
*
* For an image tag: registry/repository:tag
. For example, to specify an image with the tag
* "latest," use registry/repository:latest
.
*
*
* -
*
* For an image digest: registry/repository@digest
. For example, to specify an image with
* the digest "sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf," use
* registry/repository@sha256:cbbf2f9a99b47fc460d422812b6a5adff7dfee951d8fa2e4a98caa0382cfbdbf
* .
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder image(String image);
/**
*
* Information about the compute resources the build project uses. Available values include:
*
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your
* environment type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for builds.
* This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and 4
* NVIDIA Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on ARM-based
* processors for builds.
*
*
*
*
* For more information, see Build
* Environment Compute Types in the AWS CodeBuild User Guide.
*
*
* @param computeType
* Information about the compute resources the build project uses. Available values include:
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your
* environment type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for
* builds. This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for
* builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and
* 4 NVIDIA Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on
* ARM-based processors for builds.
*
*
*
*
* For more information, see Build
* Environment Compute Types in the AWS CodeBuild User Guide.
* @see ComputeType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputeType
*/
Builder computeType(String computeType);
/**
*
* Information about the compute resources the build project uses. Available values include:
*
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your
* environment type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for builds.
* This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and 4
* NVIDIA Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on ARM-based
* processors for builds.
*
*
*
*
* For more information, see Build
* Environment Compute Types in the AWS CodeBuild User Guide.
*
*
* @param computeType
* Information about the compute resources the build project uses. Available values include:
*
* -
*
* BUILD_GENERAL1_SMALL
: Use up to 3 GB memory and 2 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_MEDIUM
: Use up to 7 GB memory and 4 vCPUs for builds.
*
*
* -
*
* BUILD_GENERAL1_LARGE
: Use up to 16 GB memory and 8 vCPUs for builds, depending on your
* environment type.
*
*
* -
*
* BUILD_GENERAL1_2XLARGE
: Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for
* builds. This compute type supports Docker images up to 100 GB uncompressed.
*
*
*
*
* If you use BUILD_GENERAL1_LARGE
:
*
*
* -
*
* For environment type LINUX_CONTAINER
, you can use up to 15 GB memory and 8 vCPUs for
* builds.
*
*
* -
*
* For environment type LINUX_GPU_CONTAINER
, you can use up to 255 GB memory, 32 vCPUs, and
* 4 NVIDIA Tesla V100 GPUs for builds.
*
*
* -
*
* For environment type ARM_CONTAINER
, you can use up to 16 GB memory and 8 vCPUs on
* ARM-based processors for builds.
*
*
*
*
* For more information, see Build
* Environment Compute Types in the AWS CodeBuild User Guide.
* @see ComputeType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputeType
*/
Builder computeType(ComputeType computeType);
/**
*
* A set of environment variables to make available to builds for this build project.
*
*
* @param environmentVariables
* A set of environment variables to make available to builds for this build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder environmentVariables(Collection environmentVariables);
/**
*
* A set of environment variables to make available to builds for this build project.
*
*
* @param environmentVariables
* A set of environment variables to make available to builds for this build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder environmentVariables(EnvironmentVariable... environmentVariables);
/**
*
* A set of environment variables to make available to builds for this build project.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately
* and its result is passed to {@link #environmentVariables(List)}.
*
* @param environmentVariables
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #environmentVariables(List)
*/
Builder environmentVariables(Consumer... environmentVariables);
/**
*
* Enables running the Docker daemon inside a Docker container. Set to true only if the build project is used to
* build Docker images. Otherwise, a build that attempts to interact with the Docker daemon fails. The default
* setting is false
.
*
*
* You can initialize the Docker daemon during the install phase of your build by adding one of the following
* sets of commands to the install phase of your buildspec file:
*
*
* If the operating system's base image is Ubuntu Linux:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout 15 sh -c "until docker info; do echo .; sleep 1; done"
*
*
* If the operating system's base image is Alpine Linux and the previous command does not work, add the
* -t
argument to timeout
:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout -t 15 sh -c "until docker info; do echo .; sleep 1; done"
*
*
* @param privilegedMode
* Enables running the Docker daemon inside a Docker container. Set to true only if the build project is
* used to build Docker images. Otherwise, a build that attempts to interact with the Docker daemon
* fails. The default setting is false
.
*
* You can initialize the Docker daemon during the install phase of your build by adding one of the
* following sets of commands to the install phase of your buildspec file:
*
*
* If the operating system's base image is Ubuntu Linux:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout 15 sh -c "until docker info; do echo .; sleep 1; done"
*
*
* If the operating system's base image is Alpine Linux and the previous command does not work, add the
* -t
argument to timeout
:
*
*
* - nohup /usr/local/bin/dockerd --host=unix:///var/run/docker.sock --host=tcp://0.0.0.0:2375 --storage-driver=overlay&
*
*
* - timeout -t 15 sh -c "until docker info; do echo .; sleep 1; done"
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder privilegedMode(Boolean privilegedMode);
/**
*
* The certificate to use with this build project.
*
*
* @param certificate
* The certificate to use with this build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder certificate(String certificate);
/**
*
* The credentials for access to a private registry.
*
*
* @param registryCredential
* The credentials for access to a private registry.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder registryCredential(RegistryCredential registryCredential);
/**
*
* The credentials for access to a private registry.
*
* This is a convenience that creates an instance of the {@link RegistryCredential.Builder} avoiding the need to
* create one manually via {@link RegistryCredential#builder()}.
*
* When the {@link Consumer} completes, {@link RegistryCredential.Builder#build()} is called immediately and its
* result is passed to {@link #registryCredential(RegistryCredential)}.
*
* @param registryCredential
* a consumer that will call methods on {@link RegistryCredential.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #registryCredential(RegistryCredential)
*/
default Builder registryCredential(Consumer registryCredential) {
return registryCredential(RegistryCredential.builder().applyMutation(registryCredential).build());
}
/**
*
* The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you modify
* your ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When you use
* an AWS CodeBuild curated image, you must use CODEBUILD credentials.
*
*
* @param imagePullCredentialsType
* The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you
* modify your ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When
* you use an AWS CodeBuild curated image, you must use CODEBUILD credentials.
* @see ImagePullCredentialsType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImagePullCredentialsType
*/
Builder imagePullCredentialsType(String imagePullCredentialsType);
/**
*
* The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you modify
* your ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When you use
* an AWS CodeBuild curated image, you must use CODEBUILD credentials.
*
*
* @param imagePullCredentialsType
* The type of credentials AWS CodeBuild uses to pull images in your build. There are two valid values:
*
*
* -
*
* CODEBUILD
specifies that AWS CodeBuild uses its own credentials. This requires that you
* modify your ECR repository policy to trust AWS CodeBuild's service principal.
*
*
* -
*
* SERVICE_ROLE
specifies that AWS CodeBuild uses your build project's service role.
*
*
*
*
* When you use a cross-account or private registry image, you must use SERVICE_ROLE credentials. When
* you use an AWS CodeBuild curated image, you must use CODEBUILD credentials.
* @see ImagePullCredentialsType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImagePullCredentialsType
*/
Builder imagePullCredentialsType(ImagePullCredentialsType imagePullCredentialsType);
}
static final class BuilderImpl implements Builder {
private String type;
private String image;
private String computeType;
private List environmentVariables = DefaultSdkAutoConstructList.getInstance();
private Boolean privilegedMode;
private String certificate;
private RegistryCredential registryCredential;
private String imagePullCredentialsType;
private BuilderImpl() {
}
private BuilderImpl(ProjectEnvironment model) {
type(model.type);
image(model.image);
computeType(model.computeType);
environmentVariables(model.environmentVariables);
privilegedMode(model.privilegedMode);
certificate(model.certificate);
registryCredential(model.registryCredential);
imagePullCredentialsType(model.imagePullCredentialsType);
}
public final String getType() {
return type;
}
@Override
public final Builder type(String type) {
this.type = type;
return this;
}
@Override
public final Builder type(EnvironmentType type) {
this.type(type == null ? null : type.toString());
return this;
}
public final void setType(String type) {
this.type = type;
}
public final String getImage() {
return image;
}
@Override
public final Builder image(String image) {
this.image = image;
return this;
}
public final void setImage(String image) {
this.image = image;
}
public final String getComputeType() {
return computeType;
}
@Override
public final Builder computeType(String computeType) {
this.computeType = computeType;
return this;
}
@Override
public final Builder computeType(ComputeType computeType) {
this.computeType(computeType == null ? null : computeType.toString());
return this;
}
public final void setComputeType(String computeType) {
this.computeType = computeType;
}
public final Collection getEnvironmentVariables() {
return environmentVariables != null ? environmentVariables.stream().map(EnvironmentVariable::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder environmentVariables(Collection environmentVariables) {
this.environmentVariables = EnvironmentVariablesCopier.copy(environmentVariables);
return this;
}
@Override
@SafeVarargs
public final Builder environmentVariables(EnvironmentVariable... environmentVariables) {
environmentVariables(Arrays.asList(environmentVariables));
return this;
}
@Override
@SafeVarargs
public final Builder environmentVariables(Consumer... environmentVariables) {
environmentVariables(Stream.of(environmentVariables).map(c -> EnvironmentVariable.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setEnvironmentVariables(Collection environmentVariables) {
this.environmentVariables = EnvironmentVariablesCopier.copyFromBuilder(environmentVariables);
}
public final Boolean getPrivilegedMode() {
return privilegedMode;
}
@Override
public final Builder privilegedMode(Boolean privilegedMode) {
this.privilegedMode = privilegedMode;
return this;
}
public final void setPrivilegedMode(Boolean privilegedMode) {
this.privilegedMode = privilegedMode;
}
public final String getCertificate() {
return certificate;
}
@Override
public final Builder certificate(String certificate) {
this.certificate = certificate;
return this;
}
public final void setCertificate(String certificate) {
this.certificate = certificate;
}
public final RegistryCredential.Builder getRegistryCredential() {
return registryCredential != null ? registryCredential.toBuilder() : null;
}
@Override
public final Builder registryCredential(RegistryCredential registryCredential) {
this.registryCredential = registryCredential;
return this;
}
public final void setRegistryCredential(RegistryCredential.BuilderImpl registryCredential) {
this.registryCredential = registryCredential != null ? registryCredential.build() : null;
}
public final String getImagePullCredentialsType() {
return imagePullCredentialsType;
}
@Override
public final Builder imagePullCredentialsType(String imagePullCredentialsType) {
this.imagePullCredentialsType = imagePullCredentialsType;
return this;
}
@Override
public final Builder imagePullCredentialsType(ImagePullCredentialsType imagePullCredentialsType) {
this.imagePullCredentialsType(imagePullCredentialsType == null ? null : imagePullCredentialsType.toString());
return this;
}
public final void setImagePullCredentialsType(String imagePullCredentialsType) {
this.imagePullCredentialsType = imagePullCredentialsType;
}
@Override
public ProjectEnvironment build() {
return new ProjectEnvironment(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}