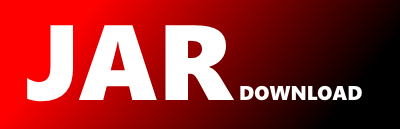
software.amazon.awssdk.services.codebuild.model.UpdateProjectRequest Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateProjectRequest extends CodeBuildRequest implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateProjectRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateProjectRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateProjectRequest::source)).setter(setter(Builder::source)).constructor(ProjectSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("source").build()).build();
private static final SdkField> SECONDARY_SOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateProjectRequest::secondarySources))
.setter(setter(Builder::secondarySources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySources").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SOURCE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateProjectRequest::sourceVersion)).setter(setter(Builder::sourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceVersion").build()).build();
private static final SdkField> SECONDARY_SOURCE_VERSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateProjectRequest::secondarySourceVersions))
.setter(setter(Builder::secondarySourceVersions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySourceVersions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSourceVersion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ARTIFACTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(UpdateProjectRequest::artifacts))
.setter(setter(Builder::artifacts)).constructor(ProjectArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("artifacts").build()).build();
private static final SdkField> SECONDARY_ARTIFACTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateProjectRequest::secondaryArtifacts))
.setter(setter(Builder::secondaryArtifacts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondaryArtifacts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CACHE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateProjectRequest::cache)).setter(setter(Builder::cache)).constructor(ProjectCache::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cache").build()).build();
private static final SdkField ENVIRONMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(UpdateProjectRequest::environment))
.setter(setter(Builder::environment)).constructor(ProjectEnvironment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environment").build()).build();
private static final SdkField SERVICE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateProjectRequest::serviceRole)).setter(setter(Builder::serviceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRole").build()).build();
private static final SdkField TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(UpdateProjectRequest::timeoutInMinutes)).setter(setter(Builder::timeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeoutInMinutes").build()).build();
private static final SdkField QUEUED_TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(UpdateProjectRequest::queuedTimeoutInMinutes)).setter(setter(Builder::queuedTimeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("queuedTimeoutInMinutes").build())
.build();
private static final SdkField ENCRYPTION_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateProjectRequest::encryptionKey)).setter(setter(Builder::encryptionKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionKey").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateProjectRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VPC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateProjectRequest::vpcConfig)).setter(setter(Builder::vpcConfig)).constructor(VpcConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpcConfig").build()).build();
private static final SdkField BADGE_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(UpdateProjectRequest::badgeEnabled)).setter(setter(Builder::badgeEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("badgeEnabled").build()).build();
private static final SdkField LOGS_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateProjectRequest::logsConfig)).setter(setter(Builder::logsConfig))
.constructor(LogsConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logsConfig").build()).build();
private static final SdkField> FILE_SYSTEM_LOCATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateProjectRequest::fileSystemLocations))
.setter(setter(Builder::fileSystemLocations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fileSystemLocations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectFileSystemLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField BUILD_BATCH_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(UpdateProjectRequest::buildBatchConfig))
.setter(setter(Builder::buildBatchConfig)).constructor(ProjectBuildBatchConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildBatchConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, DESCRIPTION_FIELD,
SOURCE_FIELD, SECONDARY_SOURCES_FIELD, SOURCE_VERSION_FIELD, SECONDARY_SOURCE_VERSIONS_FIELD, ARTIFACTS_FIELD,
SECONDARY_ARTIFACTS_FIELD, CACHE_FIELD, ENVIRONMENT_FIELD, SERVICE_ROLE_FIELD, TIMEOUT_IN_MINUTES_FIELD,
QUEUED_TIMEOUT_IN_MINUTES_FIELD, ENCRYPTION_KEY_FIELD, TAGS_FIELD, VPC_CONFIG_FIELD, BADGE_ENABLED_FIELD,
LOGS_CONFIG_FIELD, FILE_SYSTEM_LOCATIONS_FIELD, BUILD_BATCH_CONFIG_FIELD));
private final String name;
private final String description;
private final ProjectSource source;
private final List secondarySources;
private final String sourceVersion;
private final List secondarySourceVersions;
private final ProjectArtifacts artifacts;
private final List secondaryArtifacts;
private final ProjectCache cache;
private final ProjectEnvironment environment;
private final String serviceRole;
private final Integer timeoutInMinutes;
private final Integer queuedTimeoutInMinutes;
private final String encryptionKey;
private final List tags;
private final VpcConfig vpcConfig;
private final Boolean badgeEnabled;
private final LogsConfig logsConfig;
private final List fileSystemLocations;
private final ProjectBuildBatchConfig buildBatchConfig;
private UpdateProjectRequest(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.description = builder.description;
this.source = builder.source;
this.secondarySources = builder.secondarySources;
this.sourceVersion = builder.sourceVersion;
this.secondarySourceVersions = builder.secondarySourceVersions;
this.artifacts = builder.artifacts;
this.secondaryArtifacts = builder.secondaryArtifacts;
this.cache = builder.cache;
this.environment = builder.environment;
this.serviceRole = builder.serviceRole;
this.timeoutInMinutes = builder.timeoutInMinutes;
this.queuedTimeoutInMinutes = builder.queuedTimeoutInMinutes;
this.encryptionKey = builder.encryptionKey;
this.tags = builder.tags;
this.vpcConfig = builder.vpcConfig;
this.badgeEnabled = builder.badgeEnabled;
this.logsConfig = builder.logsConfig;
this.fileSystemLocations = builder.fileSystemLocations;
this.buildBatchConfig = builder.buildBatchConfig;
}
/**
*
* The name of the build project.
*
*
*
* You cannot change a build project's name.
*
*
*
* @return The name of the build project.
*
* You cannot change a build project's name.
*
*/
public String name() {
return name;
}
/**
*
* A new or replacement description of the build project.
*
*
* @return A new or replacement description of the build project.
*/
public String description() {
return description;
}
/**
*
* Information to be changed about the build input source code for the build project.
*
*
* @return Information to be changed about the build input source code for the build project.
*/
public ProjectSource source() {
return source;
}
/**
* Returns true if the SecondarySources property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasSecondarySources() {
return secondarySources != null && !(secondarySources instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSource
objects.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSecondarySources()} to see if a value was sent in this field.
*
*
* @return An array of ProjectSource
objects.
*/
public List secondarySources() {
return secondarySources;
}
/**
*
* A version of the build input to be built for this project. If not specified, the latest version is used. If
* specified, it must be one of:
*
*
* -
*
* For AWS CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example pr/25
). If a branch name is specified, the branch's
* HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default
* branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon Simple Storage Service (Amazon S3): the version ID of the object that represents the build input ZIP
* file to use.
*
*
*
*
* If sourceVersion
is specified at the build level, then that version takes precedence over this
* sourceVersion
(at the project level).
*
*
* For more information, see Source Version Sample
* with CodeBuild in the AWS CodeBuild User Guide.
*
*
* @return A version of the build input to be built for this project. If not specified, the latest version is used.
* If specified, it must be one of:
*
* -
*
* For AWS CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of
* the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code
* you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified,
* the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon Simple Storage Service (Amazon S3): the version ID of the object that represents the build
* input ZIP file to use.
*
*
*
*
* If sourceVersion
is specified at the build level, then that version takes precedence over
* this sourceVersion
(at the project level).
*
*
* For more information, see Source Version
* Sample with CodeBuild in the AWS CodeBuild User Guide.
*/
public String sourceVersion() {
return sourceVersion;
}
/**
* Returns true if the SecondarySourceVersions property was specified by the sender (it may be empty), or false if
* the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasSecondarySourceVersions() {
return secondarySourceVersions != null && !(secondarySourceVersions instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSourceVersion
objects. If secondarySourceVersions
is specified at
* the build level, then they take over these secondarySourceVersions
(at the project level).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSecondarySourceVersions()} to see if a value was sent in this field.
*
*
* @return An array of ProjectSourceVersion
objects. If secondarySourceVersions
is
* specified at the build level, then they take over these secondarySourceVersions
(at the
* project level).
*/
public List secondarySourceVersions() {
return secondarySourceVersions;
}
/**
*
* Information to be changed about the build output artifacts for the build project.
*
*
* @return Information to be changed about the build output artifacts for the build project.
*/
public ProjectArtifacts artifacts() {
return artifacts;
}
/**
* Returns true if the SecondaryArtifacts property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasSecondaryArtifacts() {
return secondaryArtifacts != null && !(secondaryArtifacts instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSource
objects.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSecondaryArtifacts()} to see if a value was sent in this field.
*
*
* @return An array of ProjectSource
objects.
*/
public List secondaryArtifacts() {
return secondaryArtifacts;
}
/**
*
* Stores recently used information so that it can be quickly accessed at a later time.
*
*
* @return Stores recently used information so that it can be quickly accessed at a later time.
*/
public ProjectCache cache() {
return cache;
}
/**
*
* Information to be changed about the build environment for the build project.
*
*
* @return Information to be changed about the build environment for the build project.
*/
public ProjectEnvironment environment() {
return environment;
}
/**
*
* The replacement ARN of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to interact
* with dependent AWS services on behalf of the AWS account.
*
*
* @return The replacement ARN of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
*/
public String serviceRole() {
return serviceRole;
}
/**
*
* The replacement value in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait before timing out any
* related build that did not get marked as completed.
*
*
* @return The replacement value in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait before timing out
* any related build that did not get marked as completed.
*/
public Integer timeoutInMinutes() {
return timeoutInMinutes;
}
/**
*
* The number of minutes a build is allowed to be queued before it times out.
*
*
* @return The number of minutes a build is allowed to be queued before it times out.
*/
public Integer queuedTimeoutInMinutes() {
return queuedTimeoutInMinutes;
}
/**
*
* The AWS Key Management Service (AWS KMS) customer master key (CMK) to be used for encrypting the build output
* artifacts.
*
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission to
* that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the
* format alias/<alias-name>
).
*
*
* @return The AWS Key Management Service (AWS KMS) customer master key (CMK) to be used for encrypting the build
* output artifacts.
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has
* permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using
* the format alias/<alias-name>
).
*/
public String encryptionKey() {
return encryptionKey;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* An updated list of tag key and value pairs associated with this build project.
*
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return An updated list of tag key and value pairs associated with this build project.
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
*/
public List tags() {
return tags;
}
/**
*
* VpcConfig enables AWS CodeBuild to access resources in an Amazon VPC.
*
*
* @return VpcConfig enables AWS CodeBuild to access resources in an Amazon VPC.
*/
public VpcConfig vpcConfig() {
return vpcConfig;
}
/**
*
* Set this to true to generate a publicly accessible URL for your project's build badge.
*
*
* @return Set this to true to generate a publicly accessible URL for your project's build badge.
*/
public Boolean badgeEnabled() {
return badgeEnabled;
}
/**
*
* Information about logs for the build project. A project can create logs in Amazon CloudWatch Logs, logs in an S3
* bucket, or both.
*
*
* @return Information about logs for the build project. A project can create logs in Amazon CloudWatch Logs, logs
* in an S3 bucket, or both.
*/
public LogsConfig logsConfig() {
return logsConfig;
}
/**
* Returns true if the FileSystemLocations property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasFileSystemLocations() {
return fileSystemLocations != null && !(fileSystemLocations instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using Amazon
* Elastic File System.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasFileSystemLocations()} to see if a value was sent in this field.
*
*
* @return An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
,
* location
, mountOptions
, mountPoint
, and type
of a
* file system created using Amazon Elastic File System.
*/
public List fileSystemLocations() {
return fileSystemLocations;
}
/**
* Returns the value of the BuildBatchConfig property for this object.
*
* @return The value of the BuildBatchConfig property for this object.
*/
public ProjectBuildBatchConfig buildBatchConfig() {
return buildBatchConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(secondarySources());
hashCode = 31 * hashCode + Objects.hashCode(sourceVersion());
hashCode = 31 * hashCode + Objects.hashCode(secondarySourceVersions());
hashCode = 31 * hashCode + Objects.hashCode(artifacts());
hashCode = 31 * hashCode + Objects.hashCode(secondaryArtifacts());
hashCode = 31 * hashCode + Objects.hashCode(cache());
hashCode = 31 * hashCode + Objects.hashCode(environment());
hashCode = 31 * hashCode + Objects.hashCode(serviceRole());
hashCode = 31 * hashCode + Objects.hashCode(timeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(queuedTimeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(encryptionKey());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(vpcConfig());
hashCode = 31 * hashCode + Objects.hashCode(badgeEnabled());
hashCode = 31 * hashCode + Objects.hashCode(logsConfig());
hashCode = 31 * hashCode + Objects.hashCode(fileSystemLocations());
hashCode = 31 * hashCode + Objects.hashCode(buildBatchConfig());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateProjectRequest)) {
return false;
}
UpdateProjectRequest other = (UpdateProjectRequest) obj;
return Objects.equals(name(), other.name()) && Objects.equals(description(), other.description())
&& Objects.equals(source(), other.source()) && Objects.equals(secondarySources(), other.secondarySources())
&& Objects.equals(sourceVersion(), other.sourceVersion())
&& Objects.equals(secondarySourceVersions(), other.secondarySourceVersions())
&& Objects.equals(artifacts(), other.artifacts())
&& Objects.equals(secondaryArtifacts(), other.secondaryArtifacts()) && Objects.equals(cache(), other.cache())
&& Objects.equals(environment(), other.environment()) && Objects.equals(serviceRole(), other.serviceRole())
&& Objects.equals(timeoutInMinutes(), other.timeoutInMinutes())
&& Objects.equals(queuedTimeoutInMinutes(), other.queuedTimeoutInMinutes())
&& Objects.equals(encryptionKey(), other.encryptionKey()) && Objects.equals(tags(), other.tags())
&& Objects.equals(vpcConfig(), other.vpcConfig()) && Objects.equals(badgeEnabled(), other.badgeEnabled())
&& Objects.equals(logsConfig(), other.logsConfig())
&& Objects.equals(fileSystemLocations(), other.fileSystemLocations())
&& Objects.equals(buildBatchConfig(), other.buildBatchConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("UpdateProjectRequest").add("Name", name()).add("Description", description())
.add("Source", source()).add("SecondarySources", secondarySources()).add("SourceVersion", sourceVersion())
.add("SecondarySourceVersions", secondarySourceVersions()).add("Artifacts", artifacts())
.add("SecondaryArtifacts", secondaryArtifacts()).add("Cache", cache()).add("Environment", environment())
.add("ServiceRole", serviceRole()).add("TimeoutInMinutes", timeoutInMinutes())
.add("QueuedTimeoutInMinutes", queuedTimeoutInMinutes()).add("EncryptionKey", encryptionKey())
.add("Tags", tags()).add("VpcConfig", vpcConfig()).add("BadgeEnabled", badgeEnabled())
.add("LogsConfig", logsConfig()).add("FileSystemLocations", fileSystemLocations())
.add("BuildBatchConfig", buildBatchConfig()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "source":
return Optional.ofNullable(clazz.cast(source()));
case "secondarySources":
return Optional.ofNullable(clazz.cast(secondarySources()));
case "sourceVersion":
return Optional.ofNullable(clazz.cast(sourceVersion()));
case "secondarySourceVersions":
return Optional.ofNullable(clazz.cast(secondarySourceVersions()));
case "artifacts":
return Optional.ofNullable(clazz.cast(artifacts()));
case "secondaryArtifacts":
return Optional.ofNullable(clazz.cast(secondaryArtifacts()));
case "cache":
return Optional.ofNullable(clazz.cast(cache()));
case "environment":
return Optional.ofNullable(clazz.cast(environment()));
case "serviceRole":
return Optional.ofNullable(clazz.cast(serviceRole()));
case "timeoutInMinutes":
return Optional.ofNullable(clazz.cast(timeoutInMinutes()));
case "queuedTimeoutInMinutes":
return Optional.ofNullable(clazz.cast(queuedTimeoutInMinutes()));
case "encryptionKey":
return Optional.ofNullable(clazz.cast(encryptionKey()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "vpcConfig":
return Optional.ofNullable(clazz.cast(vpcConfig()));
case "badgeEnabled":
return Optional.ofNullable(clazz.cast(badgeEnabled()));
case "logsConfig":
return Optional.ofNullable(clazz.cast(logsConfig()));
case "fileSystemLocations":
return Optional.ofNullable(clazz.cast(fileSystemLocations()));
case "buildBatchConfig":
return Optional.ofNullable(clazz.cast(buildBatchConfig()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* You cannot change a build project's name.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
*
* A new or replacement description of the build project.
*
*
* @param description
* A new or replacement description of the build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
*
* Information to be changed about the build input source code for the build project.
*
*
* @param source
* Information to be changed about the build input source code for the build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder source(ProjectSource source);
/**
*
* Information to be changed about the build input source code for the build project.
*
* This is a convenience that creates an instance of the {@link ProjectSource.Builder} avoiding the need to
* create one manually via {@link ProjectSource#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectSource.Builder#build()} is called immediately and its
* result is passed to {@link #source(ProjectSource)}.
*
* @param source
* a consumer that will call methods on {@link ProjectSource.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #source(ProjectSource)
*/
default Builder source(Consumer source) {
return source(ProjectSource.builder().applyMutation(source).build());
}
/**
*
* An array of ProjectSource
objects.
*
*
* @param secondarySources
* An array of ProjectSource
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySources(Collection secondarySources);
/**
*
* An array of ProjectSource
objects.
*
*
* @param secondarySources
* An array of ProjectSource
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySources(ProjectSource... secondarySources);
/**
*
* An array of ProjectSource
objects.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need
* to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and
* its result is passed to {@link #secondarySources(List)}.
*
* @param secondarySources
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #secondarySources(List)
*/
Builder secondarySources(Consumer... secondarySources);
/**
*
* A version of the build input to be built for this project. If not specified, the latest version is used. If
* specified, it must be one of:
*
*
* -
*
* For AWS CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example pr/25
). If a branch name is specified, the branch's
* HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the
* default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon Simple Storage Service (Amazon S3): the version ID of the object that represents the build input
* ZIP file to use.
*
*
*
*
* If sourceVersion
is specified at the build level, then that version takes precedence over this
* sourceVersion
(at the project level).
*
*
* For more information, see Source Version
* Sample with CodeBuild in the AWS CodeBuild User Guide.
*
*
* @param sourceVersion
* A version of the build input to be built for this project. If not specified, the latest version is
* used. If specified, it must be one of:
*
* -
*
* For AWS CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version
* of the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source
* code you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not
* specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon Simple Storage Service (Amazon S3): the version ID of the object that represents the build
* input ZIP file to use.
*
*
*
*
* If sourceVersion
is specified at the build level, then that version takes precedence over
* this sourceVersion
(at the project level).
*
*
* For more information, see Source
* Version Sample with CodeBuild in the AWS CodeBuild User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceVersion(String sourceVersion);
/**
*
* An array of ProjectSourceVersion
objects. If secondarySourceVersions
is specified
* at the build level, then they take over these secondarySourceVersions
(at the project level).
*
*
* @param secondarySourceVersions
* An array of ProjectSourceVersion
objects. If secondarySourceVersions
is
* specified at the build level, then they take over these secondarySourceVersions
(at the
* project level).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySourceVersions(Collection secondarySourceVersions);
/**
*
* An array of ProjectSourceVersion
objects. If secondarySourceVersions
is specified
* at the build level, then they take over these secondarySourceVersions
(at the project level).
*
*
* @param secondarySourceVersions
* An array of ProjectSourceVersion
objects. If secondarySourceVersions
is
* specified at the build level, then they take over these secondarySourceVersions
(at the
* project level).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySourceVersions(ProjectSourceVersion... secondarySourceVersions);
/**
*
* An array of ProjectSourceVersion
objects. If secondarySourceVersions
is specified
* at the build level, then they take over these secondarySourceVersions
(at the project level).
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately
* and its result is passed to {@link #secondarySourceVersions(List)}.
*
* @param secondarySourceVersions
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #secondarySourceVersions(List)
*/
Builder secondarySourceVersions(Consumer... secondarySourceVersions);
/**
*
* Information to be changed about the build output artifacts for the build project.
*
*
* @param artifacts
* Information to be changed about the build output artifacts for the build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder artifacts(ProjectArtifacts artifacts);
/**
*
* Information to be changed about the build output artifacts for the build project.
*
* This is a convenience that creates an instance of the {@link ProjectArtifacts.Builder} avoiding the need to
* create one manually via {@link ProjectArtifacts#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectArtifacts.Builder#build()} is called immediately and its
* result is passed to {@link #artifacts(ProjectArtifacts)}.
*
* @param artifacts
* a consumer that will call methods on {@link ProjectArtifacts.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #artifacts(ProjectArtifacts)
*/
default Builder artifacts(Consumer artifacts) {
return artifacts(ProjectArtifacts.builder().applyMutation(artifacts).build());
}
/**
*
* An array of ProjectSource
objects.
*
*
* @param secondaryArtifacts
* An array of ProjectSource
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondaryArtifacts(Collection secondaryArtifacts);
/**
*
* An array of ProjectSource
objects.
*
*
* @param secondaryArtifacts
* An array of ProjectSource
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondaryArtifacts(ProjectArtifacts... secondaryArtifacts);
/**
*
* An array of ProjectSource
objects.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and
* its result is passed to {@link #secondaryArtifacts(List)}.
*
* @param secondaryArtifacts
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #secondaryArtifacts(List)
*/
Builder secondaryArtifacts(Consumer... secondaryArtifacts);
/**
*
* Stores recently used information so that it can be quickly accessed at a later time.
*
*
* @param cache
* Stores recently used information so that it can be quickly accessed at a later time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cache(ProjectCache cache);
/**
*
* Stores recently used information so that it can be quickly accessed at a later time.
*
* This is a convenience that creates an instance of the {@link ProjectCache.Builder} avoiding the need to
* create one manually via {@link ProjectCache#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectCache.Builder#build()} is called immediately and its
* result is passed to {@link #cache(ProjectCache)}.
*
* @param cache
* a consumer that will call methods on {@link ProjectCache.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #cache(ProjectCache)
*/
default Builder cache(Consumer cache) {
return cache(ProjectCache.builder().applyMutation(cache).build());
}
/**
*
* Information to be changed about the build environment for the build project.
*
*
* @param environment
* Information to be changed about the build environment for the build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder environment(ProjectEnvironment environment);
/**
*
* Information to be changed about the build environment for the build project.
*
* This is a convenience that creates an instance of the {@link ProjectEnvironment.Builder} avoiding the need to
* create one manually via {@link ProjectEnvironment#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectEnvironment.Builder#build()} is called immediately and its
* result is passed to {@link #environment(ProjectEnvironment)}.
*
* @param environment
* a consumer that will call methods on {@link ProjectEnvironment.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #environment(ProjectEnvironment)
*/
default Builder environment(Consumer environment) {
return environment(ProjectEnvironment.builder().applyMutation(environment).build());
}
/**
*
* The replacement ARN of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
*
*
* @param serviceRole
* The replacement ARN of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder serviceRole(String serviceRole);
/**
*
* The replacement value in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait before timing out any
* related build that did not get marked as completed.
*
*
* @param timeoutInMinutes
* The replacement value in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait before timing out
* any related build that did not get marked as completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timeoutInMinutes(Integer timeoutInMinutes);
/**
*
* The number of minutes a build is allowed to be queued before it times out.
*
*
* @param queuedTimeoutInMinutes
* The number of minutes a build is allowed to be queued before it times out.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queuedTimeoutInMinutes(Integer queuedTimeoutInMinutes);
/**
*
* The AWS Key Management Service (AWS KMS) customer master key (CMK) to be used for encrypting the build output
* artifacts.
*
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission
* to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the
* format alias/<alias-name>
).
*
*
* @param encryptionKey
* The AWS Key Management Service (AWS KMS) customer master key (CMK) to be used for encrypting the build
* output artifacts.
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has
* permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias
* (using the format alias/<alias-name>
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder encryptionKey(String encryptionKey);
/**
*
* An updated list of tag key and value pairs associated with this build project.
*
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
*
*
* @param tags
* An updated list of tag key and value pairs associated with this build project.
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* An updated list of tag key and value pairs associated with this build project.
*
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
*
*
* @param tags
* An updated list of tag key and value pairs associated with this build project.
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* An updated list of tag key and value pairs associated with this build project.
*
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to create
* one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its result
* is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(List)
*/
Builder tags(Consumer... tags);
/**
*
* VpcConfig enables AWS CodeBuild to access resources in an Amazon VPC.
*
*
* @param vpcConfig
* VpcConfig enables AWS CodeBuild to access resources in an Amazon VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vpcConfig(VpcConfig vpcConfig);
/**
*
* VpcConfig enables AWS CodeBuild to access resources in an Amazon VPC.
*
* This is a convenience that creates an instance of the {@link VpcConfig.Builder} avoiding the need to create
* one manually via {@link VpcConfig#builder()}.
*
* When the {@link Consumer} completes, {@link VpcConfig.Builder#build()} is called immediately and its result
* is passed to {@link #vpcConfig(VpcConfig)}.
*
* @param vpcConfig
* a consumer that will call methods on {@link VpcConfig.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #vpcConfig(VpcConfig)
*/
default Builder vpcConfig(Consumer vpcConfig) {
return vpcConfig(VpcConfig.builder().applyMutation(vpcConfig).build());
}
/**
*
* Set this to true to generate a publicly accessible URL for your project's build badge.
*
*
* @param badgeEnabled
* Set this to true to generate a publicly accessible URL for your project's build badge.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder badgeEnabled(Boolean badgeEnabled);
/**
*
* Information about logs for the build project. A project can create logs in Amazon CloudWatch Logs, logs in an
* S3 bucket, or both.
*
*
* @param logsConfig
* Information about logs for the build project. A project can create logs in Amazon CloudWatch Logs,
* logs in an S3 bucket, or both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder logsConfig(LogsConfig logsConfig);
/**
*
* Information about logs for the build project. A project can create logs in Amazon CloudWatch Logs, logs in an
* S3 bucket, or both.
*
* This is a convenience that creates an instance of the {@link LogsConfig.Builder} avoiding the need to create
* one manually via {@link LogsConfig#builder()}.
*
* When the {@link Consumer} completes, {@link LogsConfig.Builder#build()} is called immediately and its result
* is passed to {@link #logsConfig(LogsConfig)}.
*
* @param logsConfig
* a consumer that will call methods on {@link LogsConfig.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #logsConfig(LogsConfig)
*/
default Builder logsConfig(Consumer logsConfig) {
return logsConfig(LogsConfig.builder().applyMutation(logsConfig).build());
}
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using
* Amazon Elastic File System.
*
*
* @param fileSystemLocations
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
,
* location
, mountOptions
, mountPoint
, and type
of a
* file system created using Amazon Elastic File System.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder fileSystemLocations(Collection fileSystemLocations);
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using
* Amazon Elastic File System.
*
*
* @param fileSystemLocations
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
,
* location
, mountOptions
, mountPoint
, and type
of a
* file system created using Amazon Elastic File System.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder fileSystemLocations(ProjectFileSystemLocation... fileSystemLocations);
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using
* Amazon Elastic File System.
*
* This is a convenience that creates an instance of the {@link List.Builder}
* avoiding the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called
* immediately and its result is passed to {@link #fileSystemLocations(List)}.
*
* @param fileSystemLocations
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #fileSystemLocations(List)
*/
Builder fileSystemLocations(Consumer... fileSystemLocations);
/**
* Sets the value of the BuildBatchConfig property for this object.
*
* @param buildBatchConfig
* The new value for the BuildBatchConfig property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder buildBatchConfig(ProjectBuildBatchConfig buildBatchConfig);
/**
* Sets the value of the BuildBatchConfig property for this object.
*
* This is a convenience that creates an instance of the {@link ProjectBuildBatchConfig.Builder} avoiding the
* need to create one manually via {@link ProjectBuildBatchConfig#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectBuildBatchConfig.Builder#build()} is called immediately
* and its result is passed to {@link #buildBatchConfig(ProjectBuildBatchConfig)}.
*
* @param buildBatchConfig
* a consumer that will call methods on {@link ProjectBuildBatchConfig.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #buildBatchConfig(ProjectBuildBatchConfig)
*/
default Builder buildBatchConfig(Consumer buildBatchConfig) {
return buildBatchConfig(ProjectBuildBatchConfig.builder().applyMutation(buildBatchConfig).build());
}
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CodeBuildRequest.BuilderImpl implements Builder {
private String name;
private String description;
private ProjectSource source;
private List secondarySources = DefaultSdkAutoConstructList.getInstance();
private String sourceVersion;
private List secondarySourceVersions = DefaultSdkAutoConstructList.getInstance();
private ProjectArtifacts artifacts;
private List secondaryArtifacts = DefaultSdkAutoConstructList.getInstance();
private ProjectCache cache;
private ProjectEnvironment environment;
private String serviceRole;
private Integer timeoutInMinutes;
private Integer queuedTimeoutInMinutes;
private String encryptionKey;
private List tags = DefaultSdkAutoConstructList.getInstance();
private VpcConfig vpcConfig;
private Boolean badgeEnabled;
private LogsConfig logsConfig;
private List fileSystemLocations = DefaultSdkAutoConstructList.getInstance();
private ProjectBuildBatchConfig buildBatchConfig;
private BuilderImpl() {
}
private BuilderImpl(UpdateProjectRequest model) {
super(model);
name(model.name);
description(model.description);
source(model.source);
secondarySources(model.secondarySources);
sourceVersion(model.sourceVersion);
secondarySourceVersions(model.secondarySourceVersions);
artifacts(model.artifacts);
secondaryArtifacts(model.secondaryArtifacts);
cache(model.cache);
environment(model.environment);
serviceRole(model.serviceRole);
timeoutInMinutes(model.timeoutInMinutes);
queuedTimeoutInMinutes(model.queuedTimeoutInMinutes);
encryptionKey(model.encryptionKey);
tags(model.tags);
vpcConfig(model.vpcConfig);
badgeEnabled(model.badgeEnabled);
logsConfig(model.logsConfig);
fileSystemLocations(model.fileSystemLocations);
buildBatchConfig(model.buildBatchConfig);
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final ProjectSource.Builder getSource() {
return source != null ? source.toBuilder() : null;
}
@Override
public final Builder source(ProjectSource source) {
this.source = source;
return this;
}
public final void setSource(ProjectSource.BuilderImpl source) {
this.source = source != null ? source.build() : null;
}
public final Collection getSecondarySources() {
return secondarySources != null ? secondarySources.stream().map(ProjectSource::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder secondarySources(Collection secondarySources) {
this.secondarySources = ProjectSourcesCopier.copy(secondarySources);
return this;
}
@Override
@SafeVarargs
public final Builder secondarySources(ProjectSource... secondarySources) {
secondarySources(Arrays.asList(secondarySources));
return this;
}
@Override
@SafeVarargs
public final Builder secondarySources(Consumer... secondarySources) {
secondarySources(Stream.of(secondarySources).map(c -> ProjectSource.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setSecondarySources(Collection secondarySources) {
this.secondarySources = ProjectSourcesCopier.copyFromBuilder(secondarySources);
}
public final String getSourceVersion() {
return sourceVersion;
}
@Override
public final Builder sourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
return this;
}
public final void setSourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
}
public final Collection getSecondarySourceVersions() {
return secondarySourceVersions != null ? secondarySourceVersions.stream().map(ProjectSourceVersion::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder secondarySourceVersions(Collection secondarySourceVersions) {
this.secondarySourceVersions = ProjectSecondarySourceVersionsCopier.copy(secondarySourceVersions);
return this;
}
@Override
@SafeVarargs
public final Builder secondarySourceVersions(ProjectSourceVersion... secondarySourceVersions) {
secondarySourceVersions(Arrays.asList(secondarySourceVersions));
return this;
}
@Override
@SafeVarargs
public final Builder secondarySourceVersions(Consumer... secondarySourceVersions) {
secondarySourceVersions(Stream.of(secondarySourceVersions)
.map(c -> ProjectSourceVersion.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setSecondarySourceVersions(Collection secondarySourceVersions) {
this.secondarySourceVersions = ProjectSecondarySourceVersionsCopier.copyFromBuilder(secondarySourceVersions);
}
public final ProjectArtifacts.Builder getArtifacts() {
return artifacts != null ? artifacts.toBuilder() : null;
}
@Override
public final Builder artifacts(ProjectArtifacts artifacts) {
this.artifacts = artifacts;
return this;
}
public final void setArtifacts(ProjectArtifacts.BuilderImpl artifacts) {
this.artifacts = artifacts != null ? artifacts.build() : null;
}
public final Collection getSecondaryArtifacts() {
return secondaryArtifacts != null ? secondaryArtifacts.stream().map(ProjectArtifacts::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder secondaryArtifacts(Collection secondaryArtifacts) {
this.secondaryArtifacts = ProjectArtifactsListCopier.copy(secondaryArtifacts);
return this;
}
@Override
@SafeVarargs
public final Builder secondaryArtifacts(ProjectArtifacts... secondaryArtifacts) {
secondaryArtifacts(Arrays.asList(secondaryArtifacts));
return this;
}
@Override
@SafeVarargs
public final Builder secondaryArtifacts(Consumer... secondaryArtifacts) {
secondaryArtifacts(Stream.of(secondaryArtifacts).map(c -> ProjectArtifacts.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setSecondaryArtifacts(Collection secondaryArtifacts) {
this.secondaryArtifacts = ProjectArtifactsListCopier.copyFromBuilder(secondaryArtifacts);
}
public final ProjectCache.Builder getCache() {
return cache != null ? cache.toBuilder() : null;
}
@Override
public final Builder cache(ProjectCache cache) {
this.cache = cache;
return this;
}
public final void setCache(ProjectCache.BuilderImpl cache) {
this.cache = cache != null ? cache.build() : null;
}
public final ProjectEnvironment.Builder getEnvironment() {
return environment != null ? environment.toBuilder() : null;
}
@Override
public final Builder environment(ProjectEnvironment environment) {
this.environment = environment;
return this;
}
public final void setEnvironment(ProjectEnvironment.BuilderImpl environment) {
this.environment = environment != null ? environment.build() : null;
}
public final String getServiceRole() {
return serviceRole;
}
@Override
public final Builder serviceRole(String serviceRole) {
this.serviceRole = serviceRole;
return this;
}
public final void setServiceRole(String serviceRole) {
this.serviceRole = serviceRole;
}
public final Integer getTimeoutInMinutes() {
return timeoutInMinutes;
}
@Override
public final Builder timeoutInMinutes(Integer timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
return this;
}
public final void setTimeoutInMinutes(Integer timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
}
public final Integer getQueuedTimeoutInMinutes() {
return queuedTimeoutInMinutes;
}
@Override
public final Builder queuedTimeoutInMinutes(Integer queuedTimeoutInMinutes) {
this.queuedTimeoutInMinutes = queuedTimeoutInMinutes;
return this;
}
public final void setQueuedTimeoutInMinutes(Integer queuedTimeoutInMinutes) {
this.queuedTimeoutInMinutes = queuedTimeoutInMinutes;
}
public final String getEncryptionKey() {
return encryptionKey;
}
@Override
public final Builder encryptionKey(String encryptionKey) {
this.encryptionKey = encryptionKey;
return this;
}
public final void setEncryptionKey(String encryptionKey) {
this.encryptionKey = encryptionKey;
}
public final Collection getTags() {
return tags != null ? tags.stream().map(Tag::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
public final VpcConfig.Builder getVpcConfig() {
return vpcConfig != null ? vpcConfig.toBuilder() : null;
}
@Override
public final Builder vpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
public final void setVpcConfig(VpcConfig.BuilderImpl vpcConfig) {
this.vpcConfig = vpcConfig != null ? vpcConfig.build() : null;
}
public final Boolean getBadgeEnabled() {
return badgeEnabled;
}
@Override
public final Builder badgeEnabled(Boolean badgeEnabled) {
this.badgeEnabled = badgeEnabled;
return this;
}
public final void setBadgeEnabled(Boolean badgeEnabled) {
this.badgeEnabled = badgeEnabled;
}
public final LogsConfig.Builder getLogsConfig() {
return logsConfig != null ? logsConfig.toBuilder() : null;
}
@Override
public final Builder logsConfig(LogsConfig logsConfig) {
this.logsConfig = logsConfig;
return this;
}
public final void setLogsConfig(LogsConfig.BuilderImpl logsConfig) {
this.logsConfig = logsConfig != null ? logsConfig.build() : null;
}
public final Collection getFileSystemLocations() {
return fileSystemLocations != null ? fileSystemLocations.stream().map(ProjectFileSystemLocation::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder fileSystemLocations(Collection fileSystemLocations) {
this.fileSystemLocations = ProjectFileSystemLocationsCopier.copy(fileSystemLocations);
return this;
}
@Override
@SafeVarargs
public final Builder fileSystemLocations(ProjectFileSystemLocation... fileSystemLocations) {
fileSystemLocations(Arrays.asList(fileSystemLocations));
return this;
}
@Override
@SafeVarargs
public final Builder fileSystemLocations(Consumer... fileSystemLocations) {
fileSystemLocations(Stream.of(fileSystemLocations)
.map(c -> ProjectFileSystemLocation.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setFileSystemLocations(Collection fileSystemLocations) {
this.fileSystemLocations = ProjectFileSystemLocationsCopier.copyFromBuilder(fileSystemLocations);
}
public final ProjectBuildBatchConfig.Builder getBuildBatchConfig() {
return buildBatchConfig != null ? buildBatchConfig.toBuilder() : null;
}
@Override
public final Builder buildBatchConfig(ProjectBuildBatchConfig buildBatchConfig) {
this.buildBatchConfig = buildBatchConfig;
return this;
}
public final void setBuildBatchConfig(ProjectBuildBatchConfig.BuilderImpl buildBatchConfig) {
this.buildBatchConfig = buildBatchConfig != null ? buildBatchConfig.build() : null;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateProjectRequest build() {
return new UpdateProjectRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}