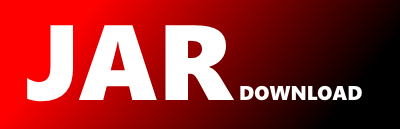
software.amazon.awssdk.services.codebuild.CodeBuildClient Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.codebuild.model.AccountLimitExceededException;
import software.amazon.awssdk.services.codebuild.model.BatchDeleteBuildsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchDeleteBuildsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildBatchesRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildBatchesResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetProjectsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetProjectsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportGroupsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportGroupsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportsResponse;
import software.amazon.awssdk.services.codebuild.model.CodeBuildException;
import software.amazon.awssdk.services.codebuild.model.CreateProjectRequest;
import software.amazon.awssdk.services.codebuild.model.CreateProjectResponse;
import software.amazon.awssdk.services.codebuild.model.CreateReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.CreateReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.CreateWebhookRequest;
import software.amazon.awssdk.services.codebuild.model.CreateWebhookResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteProjectRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteProjectResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteReportRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteReportResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteResourcePolicyRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteResourcePolicyResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteSourceCredentialsRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteSourceCredentialsResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteWebhookRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteWebhookResponse;
import software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest;
import software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesResponse;
import software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest;
import software.amazon.awssdk.services.codebuild.model.DescribeTestCasesResponse;
import software.amazon.awssdk.services.codebuild.model.GetReportGroupTrendRequest;
import software.amazon.awssdk.services.codebuild.model.GetReportGroupTrendResponse;
import software.amazon.awssdk.services.codebuild.model.GetResourcePolicyRequest;
import software.amazon.awssdk.services.codebuild.model.GetResourcePolicyResponse;
import software.amazon.awssdk.services.codebuild.model.ImportSourceCredentialsRequest;
import software.amazon.awssdk.services.codebuild.model.ImportSourceCredentialsResponse;
import software.amazon.awssdk.services.codebuild.model.InvalidInputException;
import software.amazon.awssdk.services.codebuild.model.InvalidateProjectCacheRequest;
import software.amazon.awssdk.services.codebuild.model.InvalidateProjectCacheResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildsRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildsResponse;
import software.amazon.awssdk.services.codebuild.model.ListCuratedEnvironmentImagesRequest;
import software.amazon.awssdk.services.codebuild.model.ListCuratedEnvironmentImagesResponse;
import software.amazon.awssdk.services.codebuild.model.ListProjectsRequest;
import software.amazon.awssdk.services.codebuild.model.ListProjectsResponse;
import software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest;
import software.amazon.awssdk.services.codebuild.model.ListReportGroupsResponse;
import software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.ListReportsRequest;
import software.amazon.awssdk.services.codebuild.model.ListReportsResponse;
import software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest;
import software.amazon.awssdk.services.codebuild.model.ListSharedProjectsResponse;
import software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest;
import software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsResponse;
import software.amazon.awssdk.services.codebuild.model.ListSourceCredentialsRequest;
import software.amazon.awssdk.services.codebuild.model.ListSourceCredentialsResponse;
import software.amazon.awssdk.services.codebuild.model.OAuthProviderException;
import software.amazon.awssdk.services.codebuild.model.PutResourcePolicyRequest;
import software.amazon.awssdk.services.codebuild.model.PutResourcePolicyResponse;
import software.amazon.awssdk.services.codebuild.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.codebuild.model.ResourceNotFoundException;
import software.amazon.awssdk.services.codebuild.model.RetryBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.RetryBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.RetryBuildRequest;
import software.amazon.awssdk.services.codebuild.model.RetryBuildResponse;
import software.amazon.awssdk.services.codebuild.model.StartBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.StartBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.StartBuildRequest;
import software.amazon.awssdk.services.codebuild.model.StartBuildResponse;
import software.amazon.awssdk.services.codebuild.model.StopBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.StopBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.StopBuildRequest;
import software.amazon.awssdk.services.codebuild.model.StopBuildResponse;
import software.amazon.awssdk.services.codebuild.model.UpdateProjectRequest;
import software.amazon.awssdk.services.codebuild.model.UpdateProjectResponse;
import software.amazon.awssdk.services.codebuild.model.UpdateReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.UpdateReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.UpdateWebhookRequest;
import software.amazon.awssdk.services.codebuild.model.UpdateWebhookResponse;
import software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesIterable;
import software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListReportsIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsIterable;
import software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsIterable;
/**
* Service client for accessing AWS CodeBuild. This can be created using the static {@link #builder()} method.
*
* AWS CodeBuild
*
* AWS CodeBuild is a fully managed build service in the cloud. AWS CodeBuild compiles your source code, runs unit
* tests, and produces artifacts that are ready to deploy. AWS CodeBuild eliminates the need to provision, manage, and
* scale your own build servers. It provides prepackaged build environments for the most popular programming languages
* and build tools, such as Apache Maven, Gradle, and more. You can also fully customize build environments in AWS
* CodeBuild to use your own build tools. AWS CodeBuild scales automatically to meet peak build requests. You pay only
* for the build time you consume. For more information about AWS CodeBuild, see the AWS CodeBuild User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface CodeBuildClient extends SdkClient {
String SERVICE_NAME = "codebuild";
/**
* Create a {@link CodeBuildClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CodeBuildClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CodeBuildClient}.
*/
static CodeBuildClientBuilder builder() {
return new DefaultCodeBuildClientBuilder();
}
/**
*
* Deletes one or more builds.
*
*
* @param batchDeleteBuildsRequest
* @return Result of the BatchDeleteBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchDeleteBuilds
* @see AWS
* API Documentation
*/
default BatchDeleteBuildsResponse batchDeleteBuilds(BatchDeleteBuildsRequest batchDeleteBuildsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes one or more builds.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDeleteBuildsRequest.Builder} avoiding the need
* to create one manually via {@link BatchDeleteBuildsRequest#builder()}
*
*
* @param batchDeleteBuildsRequest
* A {@link Consumer} that will call methods on {@link BatchDeleteBuildsInput.Builder} to create a request.
* @return Result of the BatchDeleteBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchDeleteBuilds
* @see AWS
* API Documentation
*/
default BatchDeleteBuildsResponse batchDeleteBuilds(Consumer batchDeleteBuildsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return batchDeleteBuilds(BatchDeleteBuildsRequest.builder().applyMutation(batchDeleteBuildsRequest).build());
}
/**
*
* Retrieves information about one or more batch builds.
*
*
* @param batchGetBuildBatchesRequest
* @return Result of the BatchGetBuildBatches operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetBuildBatches
* @see AWS
* API Documentation
*/
default BatchGetBuildBatchesResponse batchGetBuildBatches(BatchGetBuildBatchesRequest batchGetBuildBatchesRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about one or more batch builds.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetBuildBatchesRequest.Builder} avoiding the
* need to create one manually via {@link BatchGetBuildBatchesRequest#builder()}
*
*
* @param batchGetBuildBatchesRequest
* A {@link Consumer} that will call methods on {@link BatchGetBuildBatchesInput.Builder} to create a
* request.
* @return Result of the BatchGetBuildBatches operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetBuildBatches
* @see AWS
* API Documentation
*/
default BatchGetBuildBatchesResponse batchGetBuildBatches(
Consumer batchGetBuildBatchesRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return batchGetBuildBatches(BatchGetBuildBatchesRequest.builder().applyMutation(batchGetBuildBatchesRequest).build());
}
/**
*
* Gets information about one or more builds.
*
*
* @param batchGetBuildsRequest
* @return Result of the BatchGetBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetBuilds
* @see AWS API
* Documentation
*/
default BatchGetBuildsResponse batchGetBuilds(BatchGetBuildsRequest batchGetBuildsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more builds.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetBuildsRequest.Builder} avoiding the need to
* create one manually via {@link BatchGetBuildsRequest#builder()}
*
*
* @param batchGetBuildsRequest
* A {@link Consumer} that will call methods on {@link BatchGetBuildsInput.Builder} to create a request.
* @return Result of the BatchGetBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetBuilds
* @see AWS API
* Documentation
*/
default BatchGetBuildsResponse batchGetBuilds(Consumer batchGetBuildsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return batchGetBuilds(BatchGetBuildsRequest.builder().applyMutation(batchGetBuildsRequest).build());
}
/**
*
* Gets information about one or more build projects.
*
*
* @param batchGetProjectsRequest
* @return Result of the BatchGetProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetProjects
* @see AWS API
* Documentation
*/
default BatchGetProjectsResponse batchGetProjects(BatchGetProjectsRequest batchGetProjectsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more build projects.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetProjectsRequest.Builder} avoiding the need
* to create one manually via {@link BatchGetProjectsRequest#builder()}
*
*
* @param batchGetProjectsRequest
* A {@link Consumer} that will call methods on {@link BatchGetProjectsInput.Builder} to create a request.
* @return Result of the BatchGetProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetProjects
* @see AWS API
* Documentation
*/
default BatchGetProjectsResponse batchGetProjects(Consumer batchGetProjectsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return batchGetProjects(BatchGetProjectsRequest.builder().applyMutation(batchGetProjectsRequest).build());
}
/**
*
* Returns an array of report groups.
*
*
* @param batchGetReportGroupsRequest
* @return Result of the BatchGetReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetReportGroups
* @see AWS
* API Documentation
*/
default BatchGetReportGroupsResponse batchGetReportGroups(BatchGetReportGroupsRequest batchGetReportGroupsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of report groups.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetReportGroupsRequest.Builder} avoiding the
* need to create one manually via {@link BatchGetReportGroupsRequest#builder()}
*
*
* @param batchGetReportGroupsRequest
* A {@link Consumer} that will call methods on {@link BatchGetReportGroupsInput.Builder} to create a
* request.
* @return Result of the BatchGetReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetReportGroups
* @see AWS
* API Documentation
*/
default BatchGetReportGroupsResponse batchGetReportGroups(
Consumer batchGetReportGroupsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return batchGetReportGroups(BatchGetReportGroupsRequest.builder().applyMutation(batchGetReportGroupsRequest).build());
}
/**
*
* Returns an array of reports.
*
*
* @param batchGetReportsRequest
* @return Result of the BatchGetReports operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetReports
* @see AWS API
* Documentation
*/
default BatchGetReportsResponse batchGetReports(BatchGetReportsRequest batchGetReportsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of reports.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetReportsRequest.Builder} avoiding the need
* to create one manually via {@link BatchGetReportsRequest#builder()}
*
*
* @param batchGetReportsRequest
* A {@link Consumer} that will call methods on {@link BatchGetReportsInput.Builder} to create a request.
* @return Result of the BatchGetReports operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.BatchGetReports
* @see AWS API
* Documentation
*/
default BatchGetReportsResponse batchGetReports(Consumer batchGetReportsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return batchGetReports(BatchGetReportsRequest.builder().applyMutation(batchGetReportsRequest).build());
}
/**
*
* Creates a build project.
*
*
* @param createProjectRequest
* @return Result of the CreateProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.CreateProject
* @see AWS API
* Documentation
*/
default CreateProjectResponse createProject(CreateProjectRequest createProjectRequest) throws InvalidInputException,
ResourceAlreadyExistsException, AccountLimitExceededException, AwsServiceException, SdkClientException,
CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a build project.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProjectRequest.Builder} avoiding the need to
* create one manually via {@link CreateProjectRequest#builder()}
*
*
* @param createProjectRequest
* A {@link Consumer} that will call methods on {@link CreateProjectInput.Builder} to create a request.
* @return Result of the CreateProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.CreateProject
* @see AWS API
* Documentation
*/
default CreateProjectResponse createProject(Consumer createProjectRequest)
throws InvalidInputException, ResourceAlreadyExistsException, AccountLimitExceededException, AwsServiceException,
SdkClientException, CodeBuildException {
return createProject(CreateProjectRequest.builder().applyMutation(createProjectRequest).build());
}
/**
*
* Creates a report group. A report group contains a collection of reports.
*
*
* @param createReportGroupRequest
* @return Result of the CreateReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.CreateReportGroup
* @see AWS
* API Documentation
*/
default CreateReportGroupResponse createReportGroup(CreateReportGroupRequest createReportGroupRequest)
throws InvalidInputException, ResourceAlreadyExistsException, AccountLimitExceededException, AwsServiceException,
SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a report group. A report group contains a collection of reports.
*
*
*
* This is a convenience which creates an instance of the {@link CreateReportGroupRequest.Builder} avoiding the need
* to create one manually via {@link CreateReportGroupRequest#builder()}
*
*
* @param createReportGroupRequest
* A {@link Consumer} that will call methods on {@link CreateReportGroupInput.Builder} to create a request.
* @return Result of the CreateReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.CreateReportGroup
* @see AWS
* API Documentation
*/
default CreateReportGroupResponse createReportGroup(Consumer createReportGroupRequest)
throws InvalidInputException, ResourceAlreadyExistsException, AccountLimitExceededException, AwsServiceException,
SdkClientException, CodeBuildException {
return createReportGroup(CreateReportGroupRequest.builder().applyMutation(createReportGroupRequest).build());
}
/**
*
* For an existing AWS CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* enables AWS CodeBuild to start rebuilding the source code every time a code change is pushed to the repository.
*
*
*
* If you enable webhooks for an AWS CodeBuild project, and the project is used as a build step in AWS CodePipeline,
* then two identical builds are created for each commit. One build is triggered through webhooks, and one through
* AWS CodePipeline. Because billing is on a per-build basis, you are billed for both builds. Therefore, if you are
* using AWS CodePipeline, we recommend that you disable webhooks in AWS CodeBuild. In the AWS CodeBuild console,
* clear the Webhook box. For more information, see step 5 in Change a
* Build Project's Settings.
*
*
*
* @param createWebhookRequest
* @return Result of the CreateWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.CreateWebhook
* @see AWS API
* Documentation
*/
default CreateWebhookResponse createWebhook(CreateWebhookRequest createWebhookRequest) throws InvalidInputException,
OAuthProviderException, ResourceAlreadyExistsException, ResourceNotFoundException, AwsServiceException,
SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* For an existing AWS CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* enables AWS CodeBuild to start rebuilding the source code every time a code change is pushed to the repository.
*
*
*
* If you enable webhooks for an AWS CodeBuild project, and the project is used as a build step in AWS CodePipeline,
* then two identical builds are created for each commit. One build is triggered through webhooks, and one through
* AWS CodePipeline. Because billing is on a per-build basis, you are billed for both builds. Therefore, if you are
* using AWS CodePipeline, we recommend that you disable webhooks in AWS CodeBuild. In the AWS CodeBuild console,
* clear the Webhook box. For more information, see step 5 in Change a
* Build Project's Settings.
*
*
*
* This is a convenience which creates an instance of the {@link CreateWebhookRequest.Builder} avoiding the need to
* create one manually via {@link CreateWebhookRequest#builder()}
*
*
* @param createWebhookRequest
* A {@link Consumer} that will call methods on {@link CreateWebhookInput.Builder} to create a request.
* @return Result of the CreateWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.CreateWebhook
* @see AWS API
* Documentation
*/
default CreateWebhookResponse createWebhook(Consumer createWebhookRequest)
throws InvalidInputException, OAuthProviderException, ResourceAlreadyExistsException, ResourceNotFoundException,
AwsServiceException, SdkClientException, CodeBuildException {
return createWebhook(CreateWebhookRequest.builder().applyMutation(createWebhookRequest).build());
}
/**
*
* Deletes a batch build.
*
*
* @param deleteBuildBatchRequest
* @return Result of the DeleteBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteBuildBatch
* @see AWS API
* Documentation
*/
default DeleteBuildBatchResponse deleteBuildBatch(DeleteBuildBatchRequest deleteBuildBatchRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a batch build.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBuildBatchRequest.Builder} avoiding the need
* to create one manually via {@link DeleteBuildBatchRequest#builder()}
*
*
* @param deleteBuildBatchRequest
* A {@link Consumer} that will call methods on {@link DeleteBuildBatchInput.Builder} to create a request.
* @return Result of the DeleteBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteBuildBatch
* @see AWS API
* Documentation
*/
default DeleteBuildBatchResponse deleteBuildBatch(Consumer deleteBuildBatchRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return deleteBuildBatch(DeleteBuildBatchRequest.builder().applyMutation(deleteBuildBatchRequest).build());
}
/**
*
* Deletes a build project. When you delete a project, its builds are not deleted.
*
*
* @param deleteProjectRequest
* @return Result of the DeleteProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteProject
* @see AWS API
* Documentation
*/
default DeleteProjectResponse deleteProject(DeleteProjectRequest deleteProjectRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a build project. When you delete a project, its builds are not deleted.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProjectRequest.Builder} avoiding the need to
* create one manually via {@link DeleteProjectRequest#builder()}
*
*
* @param deleteProjectRequest
* A {@link Consumer} that will call methods on {@link DeleteProjectInput.Builder} to create a request.
* @return Result of the DeleteProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteProject
* @see AWS API
* Documentation
*/
default DeleteProjectResponse deleteProject(Consumer deleteProjectRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return deleteProject(DeleteProjectRequest.builder().applyMutation(deleteProjectRequest).build());
}
/**
*
* Deletes a report.
*
*
* @param deleteReportRequest
* @return Result of the DeleteReport operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteReport
* @see AWS API
* Documentation
*/
default DeleteReportResponse deleteReport(DeleteReportRequest deleteReportRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a report.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteReportRequest.Builder} avoiding the need to
* create one manually via {@link DeleteReportRequest#builder()}
*
*
* @param deleteReportRequest
* A {@link Consumer} that will call methods on {@link DeleteReportInput.Builder} to create a request.
* @return Result of the DeleteReport operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteReport
* @see AWS API
* Documentation
*/
default DeleteReportResponse deleteReport(Consumer deleteReportRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return deleteReport(DeleteReportRequest.builder().applyMutation(deleteReportRequest).build());
}
/**
*
* Deletes a report group. Before you delete a report group, you must delete its reports.
*
*
* @param deleteReportGroupRequest
* @return Result of the DeleteReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteReportGroup
* @see AWS
* API Documentation
*/
default DeleteReportGroupResponse deleteReportGroup(DeleteReportGroupRequest deleteReportGroupRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a report group. Before you delete a report group, you must delete its reports.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteReportGroupRequest.Builder} avoiding the need
* to create one manually via {@link DeleteReportGroupRequest#builder()}
*
*
* @param deleteReportGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteReportGroupInput.Builder} to create a request.
* @return Result of the DeleteReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteReportGroup
* @see AWS
* API Documentation
*/
default DeleteReportGroupResponse deleteReportGroup(Consumer deleteReportGroupRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return deleteReportGroup(DeleteReportGroupRequest.builder().applyMutation(deleteReportGroupRequest).build());
}
/**
*
* Deletes a resource policy that is identified by its resource ARN.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
default DeleteResourcePolicyResponse deleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a resource policy that is identified by its resource ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteResourcePolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteResourcePolicyRequest#builder()}
*
*
* @param deleteResourcePolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteResourcePolicyInput.Builder} to create a
* request.
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
default DeleteResourcePolicyResponse deleteResourcePolicy(
Consumer deleteResourcePolicyRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return deleteResourcePolicy(DeleteResourcePolicyRequest.builder().applyMutation(deleteResourcePolicyRequest).build());
}
/**
*
* Deletes a set of GitHub, GitHub Enterprise, or Bitbucket source credentials.
*
*
* @param deleteSourceCredentialsRequest
* @return Result of the DeleteSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteSourceCredentials
* @see AWS API Documentation
*/
default DeleteSourceCredentialsResponse deleteSourceCredentials(DeleteSourceCredentialsRequest deleteSourceCredentialsRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a set of GitHub, GitHub Enterprise, or Bitbucket source credentials.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSourceCredentialsRequest.Builder} avoiding
* the need to create one manually via {@link DeleteSourceCredentialsRequest#builder()}
*
*
* @param deleteSourceCredentialsRequest
* A {@link Consumer} that will call methods on {@link DeleteSourceCredentialsInput.Builder} to create a
* request.
* @return Result of the DeleteSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteSourceCredentials
* @see AWS API Documentation
*/
default DeleteSourceCredentialsResponse deleteSourceCredentials(
Consumer deleteSourceCredentialsRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return deleteSourceCredentials(DeleteSourceCredentialsRequest.builder().applyMutation(deleteSourceCredentialsRequest)
.build());
}
/**
*
* For an existing AWS CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* stops AWS CodeBuild from rebuilding the source code every time a code change is pushed to the repository.
*
*
* @param deleteWebhookRequest
* @return Result of the DeleteWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteWebhook
* @see AWS API
* Documentation
*/
default DeleteWebhookResponse deleteWebhook(DeleteWebhookRequest deleteWebhookRequest) throws InvalidInputException,
ResourceNotFoundException, OAuthProviderException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* For an existing AWS CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* stops AWS CodeBuild from rebuilding the source code every time a code change is pushed to the repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteWebhookRequest.Builder} avoiding the need to
* create one manually via {@link DeleteWebhookRequest#builder()}
*
*
* @param deleteWebhookRequest
* A {@link Consumer} that will call methods on {@link DeleteWebhookInput.Builder} to create a request.
* @return Result of the DeleteWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DeleteWebhook
* @see AWS API
* Documentation
*/
default DeleteWebhookResponse deleteWebhook(Consumer deleteWebhookRequest)
throws InvalidInputException, ResourceNotFoundException, OAuthProviderException, AwsServiceException,
SdkClientException, CodeBuildException {
return deleteWebhook(DeleteWebhookRequest.builder().applyMutation(deleteWebhookRequest).build());
}
/**
*
* Retrieves one or more code coverage reports.
*
*
* @param describeCodeCoveragesRequest
* @return Result of the DescribeCodeCoverages operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeCodeCoverages
* @see AWS API Documentation
*/
default DescribeCodeCoveragesResponse describeCodeCoverages(DescribeCodeCoveragesRequest describeCodeCoveragesRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves one or more code coverage reports.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCodeCoveragesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeCodeCoveragesRequest#builder()}
*
*
* @param describeCodeCoveragesRequest
* A {@link Consumer} that will call methods on {@link DescribeCodeCoveragesInput.Builder} to create a
* request.
* @return Result of the DescribeCodeCoverages operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeCodeCoverages
* @see AWS API Documentation
*/
default DescribeCodeCoveragesResponse describeCodeCoverages(
Consumer describeCodeCoveragesRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return describeCodeCoverages(DescribeCodeCoveragesRequest.builder().applyMutation(describeCodeCoveragesRequest).build());
}
/**
*
* Retrieves one or more code coverage reports.
*
*
*
* This is a variant of
* {@link #describeCodeCoverages(software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesIterable responses = client.describeCodeCoveragesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesIterable responses = client
* .describeCodeCoveragesPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesIterable responses = client.describeCodeCoveragesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCodeCoverages(software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest)}
* operation.
*
*
* @param describeCodeCoveragesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeCodeCoverages
* @see AWS API Documentation
*/
default DescribeCodeCoveragesIterable describeCodeCoveragesPaginator(DescribeCodeCoveragesRequest describeCodeCoveragesRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves one or more code coverage reports.
*
*
*
* This is a variant of
* {@link #describeCodeCoverages(software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesIterable responses = client.describeCodeCoveragesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesIterable responses = client
* .describeCodeCoveragesPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesIterable responses = client.describeCodeCoveragesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCodeCoverages(software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeCodeCoveragesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeCodeCoveragesRequest#builder()}
*
*
* @param describeCodeCoveragesRequest
* A {@link Consumer} that will call methods on {@link DescribeCodeCoveragesInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeCodeCoverages
* @see AWS API Documentation
*/
default DescribeCodeCoveragesIterable describeCodeCoveragesPaginator(
Consumer describeCodeCoveragesRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return describeCodeCoveragesPaginator(DescribeCodeCoveragesRequest.builder().applyMutation(describeCodeCoveragesRequest)
.build());
}
/**
*
* Returns a list of details about test cases for a report.
*
*
* @param describeTestCasesRequest
* @return Result of the DescribeTestCases operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeTestCases
* @see AWS
* API Documentation
*/
default DescribeTestCasesResponse describeTestCases(DescribeTestCasesRequest describeTestCasesRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of details about test cases for a report.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTestCasesRequest.Builder} avoiding the need
* to create one manually via {@link DescribeTestCasesRequest#builder()}
*
*
* @param describeTestCasesRequest
* A {@link Consumer} that will call methods on {@link DescribeTestCasesInput.Builder} to create a request.
* @return Result of the DescribeTestCases operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeTestCases
* @see AWS
* API Documentation
*/
default DescribeTestCasesResponse describeTestCases(Consumer describeTestCasesRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return describeTestCases(DescribeTestCasesRequest.builder().applyMutation(describeTestCasesRequest).build());
}
/**
*
* Returns a list of details about test cases for a report.
*
*
*
* This is a variant of
* {@link #describeTestCases(software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesIterable responses = client.describeTestCasesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesIterable responses = client
* .describeTestCasesPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.DescribeTestCasesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesIterable responses = client.describeTestCasesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTestCases(software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest)}
* operation.
*
*
* @param describeTestCasesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeTestCases
* @see AWS
* API Documentation
*/
default DescribeTestCasesIterable describeTestCasesPaginator(DescribeTestCasesRequest describeTestCasesRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of details about test cases for a report.
*
*
*
* This is a variant of
* {@link #describeTestCases(software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesIterable responses = client.describeTestCasesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesIterable responses = client
* .describeTestCasesPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.DescribeTestCasesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesIterable responses = client.describeTestCasesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTestCases(software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeTestCasesRequest.Builder} avoiding the need
* to create one manually via {@link DescribeTestCasesRequest#builder()}
*
*
* @param describeTestCasesRequest
* A {@link Consumer} that will call methods on {@link DescribeTestCasesInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.DescribeTestCases
* @see AWS
* API Documentation
*/
default DescribeTestCasesIterable describeTestCasesPaginator(
Consumer describeTestCasesRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return describeTestCasesPaginator(DescribeTestCasesRequest.builder().applyMutation(describeTestCasesRequest).build());
}
/**
*
* Analyzes and accumulates test report values for the specified test reports.
*
*
* @param getReportGroupTrendRequest
* @return Result of the GetReportGroupTrend operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.GetReportGroupTrend
* @see AWS
* API Documentation
*/
default GetReportGroupTrendResponse getReportGroupTrend(GetReportGroupTrendRequest getReportGroupTrendRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Analyzes and accumulates test report values for the specified test reports.
*
*
*
* This is a convenience which creates an instance of the {@link GetReportGroupTrendRequest.Builder} avoiding the
* need to create one manually via {@link GetReportGroupTrendRequest#builder()}
*
*
* @param getReportGroupTrendRequest
* A {@link Consumer} that will call methods on {@link GetReportGroupTrendInput.Builder} to create a request.
* @return Result of the GetReportGroupTrend operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.GetReportGroupTrend
* @see AWS
* API Documentation
*/
default GetReportGroupTrendResponse getReportGroupTrend(
Consumer getReportGroupTrendRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return getReportGroupTrend(GetReportGroupTrendRequest.builder().applyMutation(getReportGroupTrendRequest).build());
}
/**
*
* Gets a resource policy that is identified by its resource ARN.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.GetResourcePolicy
* @see AWS
* API Documentation
*/
default GetResourcePolicyResponse getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest)
throws ResourceNotFoundException, InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a resource policy that is identified by its resource ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourcePolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetResourcePolicyRequest#builder()}
*
*
* @param getResourcePolicyRequest
* A {@link Consumer} that will call methods on {@link GetResourcePolicyInput.Builder} to create a request.
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.GetResourcePolicy
* @see AWS
* API Documentation
*/
default GetResourcePolicyResponse getResourcePolicy(Consumer getResourcePolicyRequest)
throws ResourceNotFoundException, InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return getResourcePolicy(GetResourcePolicyRequest.builder().applyMutation(getResourcePolicyRequest).build());
}
/**
*
* Imports the source repository credentials for an AWS CodeBuild project that has its source code stored in a
* GitHub, GitHub Enterprise, or Bitbucket repository.
*
*
* @param importSourceCredentialsRequest
* @return Result of the ImportSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ImportSourceCredentials
* @see AWS API Documentation
*/
default ImportSourceCredentialsResponse importSourceCredentials(ImportSourceCredentialsRequest importSourceCredentialsRequest)
throws InvalidInputException, AccountLimitExceededException, ResourceAlreadyExistsException, AwsServiceException,
SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Imports the source repository credentials for an AWS CodeBuild project that has its source code stored in a
* GitHub, GitHub Enterprise, or Bitbucket repository.
*
*
*
* This is a convenience which creates an instance of the {@link ImportSourceCredentialsRequest.Builder} avoiding
* the need to create one manually via {@link ImportSourceCredentialsRequest#builder()}
*
*
* @param importSourceCredentialsRequest
* A {@link Consumer} that will call methods on {@link ImportSourceCredentialsInput.Builder} to create a
* request.
* @return Result of the ImportSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws ResourceAlreadyExistsException
* The specified AWS resource cannot be created, because an AWS resource with the same settings already
* exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ImportSourceCredentials
* @see AWS API Documentation
*/
default ImportSourceCredentialsResponse importSourceCredentials(
Consumer importSourceCredentialsRequest) throws InvalidInputException,
AccountLimitExceededException, ResourceAlreadyExistsException, AwsServiceException, SdkClientException,
CodeBuildException {
return importSourceCredentials(ImportSourceCredentialsRequest.builder().applyMutation(importSourceCredentialsRequest)
.build());
}
/**
*
* Resets the cache for a project.
*
*
* @param invalidateProjectCacheRequest
* @return Result of the InvalidateProjectCache operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.InvalidateProjectCache
* @see AWS API Documentation
*/
default InvalidateProjectCacheResponse invalidateProjectCache(InvalidateProjectCacheRequest invalidateProjectCacheRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Resets the cache for a project.
*
*
*
* This is a convenience which creates an instance of the {@link InvalidateProjectCacheRequest.Builder} avoiding the
* need to create one manually via {@link InvalidateProjectCacheRequest#builder()}
*
*
* @param invalidateProjectCacheRequest
* A {@link Consumer} that will call methods on {@link InvalidateProjectCacheInput.Builder} to create a
* request.
* @return Result of the InvalidateProjectCache operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.InvalidateProjectCache
* @see AWS API Documentation
*/
default InvalidateProjectCacheResponse invalidateProjectCache(
Consumer invalidateProjectCacheRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return invalidateProjectCache(InvalidateProjectCacheRequest.builder().applyMutation(invalidateProjectCacheRequest)
.build());
}
/**
*
* Retrieves the identifiers of your build batches in the current region.
*
*
* @param listBuildBatchesRequest
* @return Result of the ListBuildBatches operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatches
* @see AWS API
* Documentation
*/
default ListBuildBatchesResponse listBuildBatches(ListBuildBatchesRequest listBuildBatchesRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the identifiers of your build batches in the current region.
*
*
*
* This is a convenience which creates an instance of the {@link ListBuildBatchesRequest.Builder} avoiding the need
* to create one manually via {@link ListBuildBatchesRequest#builder()}
*
*
* @param listBuildBatchesRequest
* A {@link Consumer} that will call methods on {@link ListBuildBatchesInput.Builder} to create a request.
* @return Result of the ListBuildBatches operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatches
* @see AWS API
* Documentation
*/
default ListBuildBatchesResponse listBuildBatches(Consumer listBuildBatchesRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listBuildBatches(ListBuildBatchesRequest.builder().applyMutation(listBuildBatchesRequest).build());
}
/**
*
* Retrieves the identifiers of your build batches in the current region.
*
*
*
* This is a variant of
* {@link #listBuildBatches(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesIterable responses = client.listBuildBatchesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesIterable responses = client
* .listBuildBatchesPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildBatchesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesIterable responses = client.listBuildBatchesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildBatches(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest)} operation.
*
*
* @param listBuildBatchesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatches
* @see AWS API
* Documentation
*/
default ListBuildBatchesIterable listBuildBatchesPaginator(ListBuildBatchesRequest listBuildBatchesRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the identifiers of your build batches in the current region.
*
*
*
* This is a variant of
* {@link #listBuildBatches(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesIterable responses = client.listBuildBatchesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesIterable responses = client
* .listBuildBatchesPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildBatchesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesIterable responses = client.listBuildBatchesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildBatches(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListBuildBatchesRequest.Builder} avoiding the need
* to create one manually via {@link ListBuildBatchesRequest#builder()}
*
*
* @param listBuildBatchesRequest
* A {@link Consumer} that will call methods on {@link ListBuildBatchesInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatches
* @see AWS API
* Documentation
*/
default ListBuildBatchesIterable listBuildBatchesPaginator(Consumer listBuildBatchesRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listBuildBatchesPaginator(ListBuildBatchesRequest.builder().applyMutation(listBuildBatchesRequest).build());
}
/**
*
* Retrieves the identifiers of the build batches for a specific project.
*
*
* @param listBuildBatchesForProjectRequest
* @return Result of the ListBuildBatchesForProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatchesForProject
* @see AWS API Documentation
*/
default ListBuildBatchesForProjectResponse listBuildBatchesForProject(
ListBuildBatchesForProjectRequest listBuildBatchesForProjectRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the identifiers of the build batches for a specific project.
*
*
*
* This is a convenience which creates an instance of the {@link ListBuildBatchesForProjectRequest.Builder} avoiding
* the need to create one manually via {@link ListBuildBatchesForProjectRequest#builder()}
*
*
* @param listBuildBatchesForProjectRequest
* A {@link Consumer} that will call methods on {@link ListBuildBatchesForProjectInput.Builder} to create a
* request.
* @return Result of the ListBuildBatchesForProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatchesForProject
* @see AWS API Documentation
*/
default ListBuildBatchesForProjectResponse listBuildBatchesForProject(
Consumer listBuildBatchesForProjectRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return listBuildBatchesForProject(ListBuildBatchesForProjectRequest.builder()
.applyMutation(listBuildBatchesForProjectRequest).build());
}
/**
*
* Retrieves the identifiers of the build batches for a specific project.
*
*
*
* This is a variant of
* {@link #listBuildBatchesForProject(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectIterable responses = client.listBuildBatchesForProjectPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectIterable responses = client
* .listBuildBatchesForProjectPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectIterable responses = client.listBuildBatchesForProjectPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildBatchesForProject(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest)}
* operation.
*
*
* @param listBuildBatchesForProjectRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatchesForProject
* @see AWS API Documentation
*/
default ListBuildBatchesForProjectIterable listBuildBatchesForProjectPaginator(
ListBuildBatchesForProjectRequest listBuildBatchesForProjectRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the identifiers of the build batches for a specific project.
*
*
*
* This is a variant of
* {@link #listBuildBatchesForProject(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectIterable responses = client.listBuildBatchesForProjectPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectIterable responses = client
* .listBuildBatchesForProjectPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectIterable responses = client.listBuildBatchesForProjectPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildBatchesForProject(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListBuildBatchesForProjectRequest.Builder} avoiding
* the need to create one manually via {@link ListBuildBatchesForProjectRequest#builder()}
*
*
* @param listBuildBatchesForProjectRequest
* A {@link Consumer} that will call methods on {@link ListBuildBatchesForProjectInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildBatchesForProject
* @see AWS API Documentation
*/
default ListBuildBatchesForProjectIterable listBuildBatchesForProjectPaginator(
Consumer listBuildBatchesForProjectRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return listBuildBatchesForProjectPaginator(ListBuildBatchesForProjectRequest.builder()
.applyMutation(listBuildBatchesForProjectRequest).build());
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
* @return Result of the ListBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuilds
* @see #listBuilds(ListBuildsRequest)
* @see AWS API
* Documentation
*/
default ListBuildsResponse listBuilds() throws InvalidInputException, AwsServiceException, SdkClientException,
CodeBuildException {
return listBuilds(ListBuildsRequest.builder().build());
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
* @param listBuildsRequest
* @return Result of the ListBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuilds
* @see AWS API
* Documentation
*/
default ListBuildsResponse listBuilds(ListBuildsRequest listBuildsRequest) throws InvalidInputException, AwsServiceException,
SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
*
* This is a convenience which creates an instance of the {@link ListBuildsRequest.Builder} avoiding the need to
* create one manually via {@link ListBuildsRequest#builder()}
*
*
* @param listBuildsRequest
* A {@link Consumer} that will call methods on {@link ListBuildsInput.Builder} to create a request.
* @return Result of the ListBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuilds
* @see AWS API
* Documentation
*/
default ListBuildsResponse listBuilds(Consumer listBuildsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return listBuilds(ListBuildsRequest.builder().applyMutation(listBuildsRequest).build());
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
*
* This is a variant of {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuilds
* @see #listBuildsPaginator(ListBuildsRequest)
* @see AWS API
* Documentation
*/
default ListBuildsIterable listBuildsPaginator() throws InvalidInputException, AwsServiceException, SdkClientException,
CodeBuildException {
return listBuildsPaginator(ListBuildsRequest.builder().build());
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
*
* This is a variant of {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)} operation.
*
*
* @param listBuildsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuilds
* @see AWS API
* Documentation
*/
default ListBuildsIterable listBuildsPaginator(ListBuildsRequest listBuildsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
*
* This is a variant of {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsIterable responses = client.listBuildsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListBuildsRequest.Builder} avoiding the need to
* create one manually via {@link ListBuildsRequest#builder()}
*
*
* @param listBuildsRequest
* A {@link Consumer} that will call methods on {@link ListBuildsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuilds
* @see AWS API
* Documentation
*/
default ListBuildsIterable listBuildsPaginator(Consumer listBuildsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listBuildsPaginator(ListBuildsRequest.builder().applyMutation(listBuildsRequest).build());
}
/**
*
* Gets a list of build identifiers for the specified build project, with each build identifier representing a
* single build.
*
*
* @param listBuildsForProjectRequest
* @return Result of the ListBuildsForProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildsForProject
* @see AWS
* API Documentation
*/
default ListBuildsForProjectResponse listBuildsForProject(ListBuildsForProjectRequest listBuildsForProjectRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of build identifiers for the specified build project, with each build identifier representing a
* single build.
*
*
*
* This is a convenience which creates an instance of the {@link ListBuildsForProjectRequest.Builder} avoiding the
* need to create one manually via {@link ListBuildsForProjectRequest#builder()}
*
*
* @param listBuildsForProjectRequest
* A {@link Consumer} that will call methods on {@link ListBuildsForProjectInput.Builder} to create a
* request.
* @return Result of the ListBuildsForProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildsForProject
* @see AWS
* API Documentation
*/
default ListBuildsForProjectResponse listBuildsForProject(
Consumer listBuildsForProjectRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return listBuildsForProject(ListBuildsForProjectRequest.builder().applyMutation(listBuildsForProjectRequest).build());
}
/**
*
* Gets a list of build identifiers for the specified build project, with each build identifier representing a
* single build.
*
*
*
* This is a variant of
* {@link #listBuildsForProject(software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectIterable responses = client.listBuildsForProjectPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectIterable responses = client
* .listBuildsForProjectPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectIterable responses = client.listBuildsForProjectPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildsForProject(software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest)}
* operation.
*
*
* @param listBuildsForProjectRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildsForProject
* @see AWS
* API Documentation
*/
default ListBuildsForProjectIterable listBuildsForProjectPaginator(ListBuildsForProjectRequest listBuildsForProjectRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of build identifiers for the specified build project, with each build identifier representing a
* single build.
*
*
*
* This is a variant of
* {@link #listBuildsForProject(software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectIterable responses = client.listBuildsForProjectPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectIterable responses = client
* .listBuildsForProjectPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectIterable responses = client.listBuildsForProjectPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildsForProject(software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListBuildsForProjectRequest.Builder} avoiding the
* need to create one manually via {@link ListBuildsForProjectRequest#builder()}
*
*
* @param listBuildsForProjectRequest
* A {@link Consumer} that will call methods on {@link ListBuildsForProjectInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListBuildsForProject
* @see AWS
* API Documentation
*/
default ListBuildsForProjectIterable listBuildsForProjectPaginator(
Consumer listBuildsForProjectRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return listBuildsForProjectPaginator(ListBuildsForProjectRequest.builder().applyMutation(listBuildsForProjectRequest)
.build());
}
/**
*
* Gets information about Docker images that are managed by AWS CodeBuild.
*
*
* @return Result of the ListCuratedEnvironmentImages operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListCuratedEnvironmentImages
* @see #listCuratedEnvironmentImages(ListCuratedEnvironmentImagesRequest)
* @see AWS API Documentation
*/
default ListCuratedEnvironmentImagesResponse listCuratedEnvironmentImages() throws AwsServiceException, SdkClientException,
CodeBuildException {
return listCuratedEnvironmentImages(ListCuratedEnvironmentImagesRequest.builder().build());
}
/**
*
* Gets information about Docker images that are managed by AWS CodeBuild.
*
*
* @param listCuratedEnvironmentImagesRequest
* @return Result of the ListCuratedEnvironmentImages operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListCuratedEnvironmentImages
* @see AWS API Documentation
*/
default ListCuratedEnvironmentImagesResponse listCuratedEnvironmentImages(
ListCuratedEnvironmentImagesRequest listCuratedEnvironmentImagesRequest) throws AwsServiceException,
SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about Docker images that are managed by AWS CodeBuild.
*
*
*
* This is a convenience which creates an instance of the {@link ListCuratedEnvironmentImagesRequest.Builder}
* avoiding the need to create one manually via {@link ListCuratedEnvironmentImagesRequest#builder()}
*
*
* @param listCuratedEnvironmentImagesRequest
* A {@link Consumer} that will call methods on {@link ListCuratedEnvironmentImagesInput.Builder} to create a
* request.
* @return Result of the ListCuratedEnvironmentImages operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListCuratedEnvironmentImages
* @see AWS API Documentation
*/
default ListCuratedEnvironmentImagesResponse listCuratedEnvironmentImages(
Consumer listCuratedEnvironmentImagesRequest)
throws AwsServiceException, SdkClientException, CodeBuildException {
return listCuratedEnvironmentImages(ListCuratedEnvironmentImagesRequest.builder()
.applyMutation(listCuratedEnvironmentImagesRequest).build());
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
* @return Result of the ListProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListProjects
* @see #listProjects(ListProjectsRequest)
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects() throws InvalidInputException, AwsServiceException, SdkClientException,
CodeBuildException {
return listProjects(ListProjectsRequest.builder().build());
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
* @param listProjectsRequest
* @return Result of the ListProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects(ListProjectsRequest listProjectsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
*
* This is a convenience which creates an instance of the {@link ListProjectsRequest.Builder} avoiding the need to
* create one manually via {@link ListProjectsRequest#builder()}
*
*
* @param listProjectsRequest
* A {@link Consumer} that will call methods on {@link ListProjectsInput.Builder} to create a request.
* @return Result of the ListProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects(Consumer listProjectsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listProjects(ListProjectsRequest.builder().applyMutation(listProjectsRequest).build());
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
*
* This is a variant of {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListProjects
* @see #listProjectsPaginator(ListProjectsRequest)
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator() throws InvalidInputException, AwsServiceException, SdkClientException,
CodeBuildException {
return listProjectsPaginator(ListProjectsRequest.builder().build());
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
*
* This is a variant of {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)} operation.
*
*
* @param listProjectsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator(ListProjectsRequest listProjectsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
*
* This is a variant of {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListProjectsRequest.Builder} avoiding the need to
* create one manually via {@link ListProjectsRequest#builder()}
*
*
* @param listProjectsRequest
* A {@link Consumer} that will call methods on {@link ListProjectsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator(Consumer listProjectsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listProjectsPaginator(ListProjectsRequest.builder().applyMutation(listProjectsRequest).build());
}
/**
*
* Gets a list ARNs for the report groups in the current AWS account.
*
*
* @param listReportGroupsRequest
* @return Result of the ListReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportGroups
* @see AWS API
* Documentation
*/
default ListReportGroupsResponse listReportGroups(ListReportGroupsRequest listReportGroupsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list ARNs for the report groups in the current AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListReportGroupsRequest.Builder} avoiding the need
* to create one manually via {@link ListReportGroupsRequest#builder()}
*
*
* @param listReportGroupsRequest
* A {@link Consumer} that will call methods on {@link ListReportGroupsInput.Builder} to create a request.
* @return Result of the ListReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportGroups
* @see AWS API
* Documentation
*/
default ListReportGroupsResponse listReportGroups(Consumer listReportGroupsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listReportGroups(ListReportGroupsRequest.builder().applyMutation(listReportGroupsRequest).build());
}
/**
*
* Gets a list ARNs for the report groups in the current AWS account.
*
*
*
* This is a variant of
* {@link #listReportGroups(software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsIterable responses = client.listReportGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsIterable responses = client
* .listReportGroupsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListReportGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsIterable responses = client.listReportGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReportGroups(software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest)} operation.
*
*
* @param listReportGroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportGroups
* @see AWS API
* Documentation
*/
default ListReportGroupsIterable listReportGroupsPaginator(ListReportGroupsRequest listReportGroupsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list ARNs for the report groups in the current AWS account.
*
*
*
* This is a variant of
* {@link #listReportGroups(software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsIterable responses = client.listReportGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsIterable responses = client
* .listReportGroupsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListReportGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsIterable responses = client.listReportGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReportGroups(software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListReportGroupsRequest.Builder} avoiding the need
* to create one manually via {@link ListReportGroupsRequest#builder()}
*
*
* @param listReportGroupsRequest
* A {@link Consumer} that will call methods on {@link ListReportGroupsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportGroups
* @see AWS API
* Documentation
*/
default ListReportGroupsIterable listReportGroupsPaginator(Consumer listReportGroupsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listReportGroupsPaginator(ListReportGroupsRequest.builder().applyMutation(listReportGroupsRequest).build());
}
/**
*
* Returns a list of ARNs for the reports in the current AWS account.
*
*
* @param listReportsRequest
* @return Result of the ListReports operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReports
* @see AWS API
* Documentation
*/
default ListReportsResponse listReports(ListReportsRequest listReportsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of ARNs for the reports in the current AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListReportsRequest.Builder} avoiding the need to
* create one manually via {@link ListReportsRequest#builder()}
*
*
* @param listReportsRequest
* A {@link Consumer} that will call methods on {@link ListReportsInput.Builder} to create a request.
* @return Result of the ListReports operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReports
* @see AWS API
* Documentation
*/
default ListReportsResponse listReports(Consumer listReportsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listReports(ListReportsRequest.builder().applyMutation(listReportsRequest).build());
}
/**
*
* Returns a list of ARNs for the reports in the current AWS account.
*
*
*
* This is a variant of {@link #listReports(software.amazon.awssdk.services.codebuild.model.ListReportsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsIterable responses = client.listReportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsIterable responses = client.listReportsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListReportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsIterable responses = client.listReportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReports(software.amazon.awssdk.services.codebuild.model.ListReportsRequest)} operation.
*
*
* @param listReportsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReports
* @see AWS API
* Documentation
*/
default ListReportsIterable listReportsPaginator(ListReportsRequest listReportsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of ARNs for the reports in the current AWS account.
*
*
*
* This is a variant of {@link #listReports(software.amazon.awssdk.services.codebuild.model.ListReportsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsIterable responses = client.listReportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsIterable responses = client.listReportsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListReportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsIterable responses = client.listReportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReports(software.amazon.awssdk.services.codebuild.model.ListReportsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListReportsRequest.Builder} avoiding the need to
* create one manually via {@link ListReportsRequest#builder()}
*
*
* @param listReportsRequest
* A {@link Consumer} that will call methods on {@link ListReportsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReports
* @see AWS API
* Documentation
*/
default ListReportsIterable listReportsPaginator(Consumer listReportsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listReportsPaginator(ListReportsRequest.builder().applyMutation(listReportsRequest).build());
}
/**
*
* Returns a list of ARNs for the reports that belong to a ReportGroup
.
*
*
* @param listReportsForReportGroupRequest
* @return Result of the ListReportsForReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportsForReportGroup
* @see AWS API Documentation
*/
default ListReportsForReportGroupResponse listReportsForReportGroup(
ListReportsForReportGroupRequest listReportsForReportGroupRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of ARNs for the reports that belong to a ReportGroup
.
*
*
*
* This is a convenience which creates an instance of the {@link ListReportsForReportGroupRequest.Builder} avoiding
* the need to create one manually via {@link ListReportsForReportGroupRequest#builder()}
*
*
* @param listReportsForReportGroupRequest
* A {@link Consumer} that will call methods on {@link ListReportsForReportGroupInput.Builder} to create a
* request.
* @return Result of the ListReportsForReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportsForReportGroup
* @see AWS API Documentation
*/
default ListReportsForReportGroupResponse listReportsForReportGroup(
Consumer listReportsForReportGroupRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return listReportsForReportGroup(ListReportsForReportGroupRequest.builder()
.applyMutation(listReportsForReportGroupRequest).build());
}
/**
*
* Returns a list of ARNs for the reports that belong to a ReportGroup
.
*
*
*
* This is a variant of
* {@link #listReportsForReportGroup(software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupIterable responses = client.listReportsForReportGroupPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupIterable responses = client
* .listReportsForReportGroupPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupIterable responses = client.listReportsForReportGroupPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReportsForReportGroup(software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest)}
* operation.
*
*
* @param listReportsForReportGroupRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportsForReportGroup
* @see AWS API Documentation
*/
default ListReportsForReportGroupIterable listReportsForReportGroupPaginator(
ListReportsForReportGroupRequest listReportsForReportGroupRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of ARNs for the reports that belong to a ReportGroup
.
*
*
*
* This is a variant of
* {@link #listReportsForReportGroup(software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupIterable responses = client.listReportsForReportGroupPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupIterable responses = client
* .listReportsForReportGroupPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupIterable responses = client.listReportsForReportGroupPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReportsForReportGroup(software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListReportsForReportGroupRequest.Builder} avoiding
* the need to create one manually via {@link ListReportsForReportGroupRequest#builder()}
*
*
* @param listReportsForReportGroupRequest
* A {@link Consumer} that will call methods on {@link ListReportsForReportGroupInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListReportsForReportGroup
* @see AWS API Documentation
*/
default ListReportsForReportGroupIterable listReportsForReportGroupPaginator(
Consumer listReportsForReportGroupRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return listReportsForReportGroupPaginator(ListReportsForReportGroupRequest.builder()
.applyMutation(listReportsForReportGroupRequest).build());
}
/**
*
* Gets a list of projects that are shared with other AWS accounts or users.
*
*
* @param listSharedProjectsRequest
* @return Result of the ListSharedProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedProjects
* @see AWS
* API Documentation
*/
default ListSharedProjectsResponse listSharedProjects(ListSharedProjectsRequest listSharedProjectsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of projects that are shared with other AWS accounts or users.
*
*
*
* This is a convenience which creates an instance of the {@link ListSharedProjectsRequest.Builder} avoiding the
* need to create one manually via {@link ListSharedProjectsRequest#builder()}
*
*
* @param listSharedProjectsRequest
* A {@link Consumer} that will call methods on {@link ListSharedProjectsInput.Builder} to create a request.
* @return Result of the ListSharedProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedProjects
* @see AWS
* API Documentation
*/
default ListSharedProjectsResponse listSharedProjects(Consumer listSharedProjectsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return listSharedProjects(ListSharedProjectsRequest.builder().applyMutation(listSharedProjectsRequest).build());
}
/**
*
* Gets a list of projects that are shared with other AWS accounts or users.
*
*
*
* This is a variant of
* {@link #listSharedProjects(software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsIterable responses = client.listSharedProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsIterable responses = client
* .listSharedProjectsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListSharedProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsIterable responses = client.listSharedProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSharedProjects(software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest)}
* operation.
*
*
* @param listSharedProjectsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedProjects
* @see AWS
* API Documentation
*/
default ListSharedProjectsIterable listSharedProjectsPaginator(ListSharedProjectsRequest listSharedProjectsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of projects that are shared with other AWS accounts or users.
*
*
*
* This is a variant of
* {@link #listSharedProjects(software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsIterable responses = client.listSharedProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsIterable responses = client
* .listSharedProjectsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListSharedProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsIterable responses = client.listSharedProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSharedProjects(software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListSharedProjectsRequest.Builder} avoiding the
* need to create one manually via {@link ListSharedProjectsRequest#builder()}
*
*
* @param listSharedProjectsRequest
* A {@link Consumer} that will call methods on {@link ListSharedProjectsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedProjects
* @see AWS
* API Documentation
*/
default ListSharedProjectsIterable listSharedProjectsPaginator(
Consumer listSharedProjectsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return listSharedProjectsPaginator(ListSharedProjectsRequest.builder().applyMutation(listSharedProjectsRequest).build());
}
/**
*
* Gets a list of report groups that are shared with other AWS accounts or users.
*
*
* @param listSharedReportGroupsRequest
* @return Result of the ListSharedReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedReportGroups
* @see AWS API Documentation
*/
default ListSharedReportGroupsResponse listSharedReportGroups(ListSharedReportGroupsRequest listSharedReportGroupsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of report groups that are shared with other AWS accounts or users.
*
*
*
* This is a convenience which creates an instance of the {@link ListSharedReportGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListSharedReportGroupsRequest#builder()}
*
*
* @param listSharedReportGroupsRequest
* A {@link Consumer} that will call methods on {@link ListSharedReportGroupsInput.Builder} to create a
* request.
* @return Result of the ListSharedReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedReportGroups
* @see AWS API Documentation
*/
default ListSharedReportGroupsResponse listSharedReportGroups(
Consumer listSharedReportGroupsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return listSharedReportGroups(ListSharedReportGroupsRequest.builder().applyMutation(listSharedReportGroupsRequest)
.build());
}
/**
*
* Gets a list of report groups that are shared with other AWS accounts or users.
*
*
*
* This is a variant of
* {@link #listSharedReportGroups(software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsIterable responses = client.listSharedReportGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsIterable responses = client
* .listSharedReportGroupsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsIterable responses = client.listSharedReportGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSharedReportGroups(software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest)}
* operation.
*
*
* @param listSharedReportGroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedReportGroups
* @see AWS API Documentation
*/
default ListSharedReportGroupsIterable listSharedReportGroupsPaginator(
ListSharedReportGroupsRequest listSharedReportGroupsRequest) throws InvalidInputException, AwsServiceException,
SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of report groups that are shared with other AWS accounts or users.
*
*
*
* This is a variant of
* {@link #listSharedReportGroups(software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsIterable responses = client.listSharedReportGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsIterable responses = client
* .listSharedReportGroupsPaginator(request);
* for (software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsIterable responses = client.listSharedReportGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSharedReportGroups(software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListSharedReportGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListSharedReportGroupsRequest#builder()}
*
*
* @param listSharedReportGroupsRequest
* A {@link Consumer} that will call methods on {@link ListSharedReportGroupsInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSharedReportGroups
* @see AWS API Documentation
*/
default ListSharedReportGroupsIterable listSharedReportGroupsPaginator(
Consumer listSharedReportGroupsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return listSharedReportGroupsPaginator(ListSharedReportGroupsRequest.builder()
.applyMutation(listSharedReportGroupsRequest).build());
}
/**
*
* Returns a list of SourceCredentialsInfo
objects.
*
*
* @return Result of the ListSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSourceCredentials
* @see #listSourceCredentials(ListSourceCredentialsRequest)
* @see AWS API Documentation
*/
default ListSourceCredentialsResponse listSourceCredentials() throws InvalidInputException, AwsServiceException,
SdkClientException, CodeBuildException {
return listSourceCredentials(ListSourceCredentialsRequest.builder().build());
}
/**
*
* Returns a list of SourceCredentialsInfo
objects.
*
*
* @param listSourceCredentialsRequest
* @return Result of the ListSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSourceCredentials
* @see AWS API Documentation
*/
default ListSourceCredentialsResponse listSourceCredentials(ListSourceCredentialsRequest listSourceCredentialsRequest)
throws InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of SourceCredentialsInfo
objects.
*
*
*
* This is a convenience which creates an instance of the {@link ListSourceCredentialsRequest.Builder} avoiding the
* need to create one manually via {@link ListSourceCredentialsRequest#builder()}
*
*
* @param listSourceCredentialsRequest
* A {@link Consumer} that will call methods on {@link ListSourceCredentialsInput.Builder} to create a
* request.
* @return Result of the ListSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.ListSourceCredentials
* @see AWS API Documentation
*/
default ListSourceCredentialsResponse listSourceCredentials(
Consumer listSourceCredentialsRequest) throws InvalidInputException,
AwsServiceException, SdkClientException, CodeBuildException {
return listSourceCredentials(ListSourceCredentialsRequest.builder().applyMutation(listSourceCredentialsRequest).build());
}
/**
*
* Stores a resource policy for the ARN of a Project
or ReportGroup
object.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.PutResourcePolicy
* @see AWS
* API Documentation
*/
default PutResourcePolicyResponse putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest)
throws ResourceNotFoundException, InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Stores a resource policy for the ARN of a Project
or ReportGroup
object.
*
*
*
* This is a convenience which creates an instance of the {@link PutResourcePolicyRequest.Builder} avoiding the need
* to create one manually via {@link PutResourcePolicyRequest#builder()}
*
*
* @param putResourcePolicyRequest
* A {@link Consumer} that will call methods on {@link PutResourcePolicyInput.Builder} to create a request.
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.PutResourcePolicy
* @see AWS
* API Documentation
*/
default PutResourcePolicyResponse putResourcePolicy(Consumer putResourcePolicyRequest)
throws ResourceNotFoundException, InvalidInputException, AwsServiceException, SdkClientException, CodeBuildException {
return putResourcePolicy(PutResourcePolicyRequest.builder().applyMutation(putResourcePolicyRequest).build());
}
/**
*
* Restarts a build.
*
*
* @param retryBuildRequest
* @return Result of the RetryBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.RetryBuild
* @see AWS API
* Documentation
*/
default RetryBuildResponse retryBuild(RetryBuildRequest retryBuildRequest) throws InvalidInputException,
ResourceNotFoundException, AccountLimitExceededException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Restarts a build.
*
*
*
* This is a convenience which creates an instance of the {@link RetryBuildRequest.Builder} avoiding the need to
* create one manually via {@link RetryBuildRequest#builder()}
*
*
* @param retryBuildRequest
* A {@link Consumer} that will call methods on {@link RetryBuildInput.Builder} to create a request.
* @return Result of the RetryBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.RetryBuild
* @see AWS API
* Documentation
*/
default RetryBuildResponse retryBuild(Consumer retryBuildRequest) throws InvalidInputException,
ResourceNotFoundException, AccountLimitExceededException, AwsServiceException, SdkClientException, CodeBuildException {
return retryBuild(RetryBuildRequest.builder().applyMutation(retryBuildRequest).build());
}
/**
*
* Restarts a failed batch build. Only batch builds that have failed can be retried.
*
*
* @param retryBuildBatchRequest
* @return Result of the RetryBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.RetryBuildBatch
* @see AWS API
* Documentation
*/
default RetryBuildBatchResponse retryBuildBatch(RetryBuildBatchRequest retryBuildBatchRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Restarts a failed batch build. Only batch builds that have failed can be retried.
*
*
*
* This is a convenience which creates an instance of the {@link RetryBuildBatchRequest.Builder} avoiding the need
* to create one manually via {@link RetryBuildBatchRequest#builder()}
*
*
* @param retryBuildBatchRequest
* A {@link Consumer} that will call methods on {@link RetryBuildBatchInput.Builder} to create a request.
* @return Result of the RetryBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.RetryBuildBatch
* @see AWS API
* Documentation
*/
default RetryBuildBatchResponse retryBuildBatch(Consumer retryBuildBatchRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return retryBuildBatch(RetryBuildBatchRequest.builder().applyMutation(retryBuildBatchRequest).build());
}
/**
*
* Starts running a build.
*
*
* @param startBuildRequest
* @return Result of the StartBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StartBuild
* @see AWS API
* Documentation
*/
default StartBuildResponse startBuild(StartBuildRequest startBuildRequest) throws InvalidInputException,
ResourceNotFoundException, AccountLimitExceededException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Starts running a build.
*
*
*
* This is a convenience which creates an instance of the {@link StartBuildRequest.Builder} avoiding the need to
* create one manually via {@link StartBuildRequest#builder()}
*
*
* @param startBuildRequest
* A {@link Consumer} that will call methods on {@link StartBuildInput.Builder} to create a request.
* @return Result of the StartBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws AccountLimitExceededException
* An AWS service limit was exceeded for the calling AWS account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StartBuild
* @see AWS API
* Documentation
*/
default StartBuildResponse startBuild(Consumer startBuildRequest) throws InvalidInputException,
ResourceNotFoundException, AccountLimitExceededException, AwsServiceException, SdkClientException, CodeBuildException {
return startBuild(StartBuildRequest.builder().applyMutation(startBuildRequest).build());
}
/**
*
* Starts a batch build for a project.
*
*
* @param startBuildBatchRequest
* @return Result of the StartBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StartBuildBatch
* @see AWS API
* Documentation
*/
default StartBuildBatchResponse startBuildBatch(StartBuildBatchRequest startBuildBatchRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Starts a batch build for a project.
*
*
*
* This is a convenience which creates an instance of the {@link StartBuildBatchRequest.Builder} avoiding the need
* to create one manually via {@link StartBuildBatchRequest#builder()}
*
*
* @param startBuildBatchRequest
* A {@link Consumer} that will call methods on {@link StartBuildBatchInput.Builder} to create a request.
* @return Result of the StartBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StartBuildBatch
* @see AWS API
* Documentation
*/
default StartBuildBatchResponse startBuildBatch(Consumer startBuildBatchRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return startBuildBatch(StartBuildBatchRequest.builder().applyMutation(startBuildBatchRequest).build());
}
/**
*
* Attempts to stop running a build.
*
*
* @param stopBuildRequest
* @return Result of the StopBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StopBuild
* @see AWS API
* Documentation
*/
default StopBuildResponse stopBuild(StopBuildRequest stopBuildRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Attempts to stop running a build.
*
*
*
* This is a convenience which creates an instance of the {@link StopBuildRequest.Builder} avoiding the need to
* create one manually via {@link StopBuildRequest#builder()}
*
*
* @param stopBuildRequest
* A {@link Consumer} that will call methods on {@link StopBuildInput.Builder} to create a request.
* @return Result of the StopBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StopBuild
* @see AWS API
* Documentation
*/
default StopBuildResponse stopBuild(Consumer stopBuildRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return stopBuild(StopBuildRequest.builder().applyMutation(stopBuildRequest).build());
}
/**
*
* Stops a running batch build.
*
*
* @param stopBuildBatchRequest
* @return Result of the StopBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StopBuildBatch
* @see AWS API
* Documentation
*/
default StopBuildBatchResponse stopBuildBatch(StopBuildBatchRequest stopBuildBatchRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Stops a running batch build.
*
*
*
* This is a convenience which creates an instance of the {@link StopBuildBatchRequest.Builder} avoiding the need to
* create one manually via {@link StopBuildBatchRequest#builder()}
*
*
* @param stopBuildBatchRequest
* A {@link Consumer} that will call methods on {@link StopBuildBatchInput.Builder} to create a request.
* @return Result of the StopBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.StopBuildBatch
* @see AWS API
* Documentation
*/
default StopBuildBatchResponse stopBuildBatch(Consumer stopBuildBatchRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return stopBuildBatch(StopBuildBatchRequest.builder().applyMutation(stopBuildBatchRequest).build());
}
/**
*
* Changes the settings of a build project.
*
*
* @param updateProjectRequest
* @return Result of the UpdateProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.UpdateProject
* @see AWS API
* Documentation
*/
default UpdateProjectResponse updateProject(UpdateProjectRequest updateProjectRequest) throws InvalidInputException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Changes the settings of a build project.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateProjectRequest.Builder} avoiding the need to
* create one manually via {@link UpdateProjectRequest#builder()}
*
*
* @param updateProjectRequest
* A {@link Consumer} that will call methods on {@link UpdateProjectInput.Builder} to create a request.
* @return Result of the UpdateProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.UpdateProject
* @see AWS API
* Documentation
*/
default UpdateProjectResponse updateProject(Consumer updateProjectRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return updateProject(UpdateProjectRequest.builder().applyMutation(updateProjectRequest).build());
}
/**
*
* Updates a report group.
*
*
* @param updateReportGroupRequest
* @return Result of the UpdateReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.UpdateReportGroup
* @see AWS
* API Documentation
*/
default UpdateReportGroupResponse updateReportGroup(UpdateReportGroupRequest updateReportGroupRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a report group.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateReportGroupRequest.Builder} avoiding the need
* to create one manually via {@link UpdateReportGroupRequest#builder()}
*
*
* @param updateReportGroupRequest
* A {@link Consumer} that will call methods on {@link UpdateReportGroupInput.Builder} to create a request.
* @return Result of the UpdateReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.UpdateReportGroup
* @see AWS
* API Documentation
*/
default UpdateReportGroupResponse updateReportGroup(Consumer updateReportGroupRequest)
throws InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, CodeBuildException {
return updateReportGroup(UpdateReportGroupRequest.builder().applyMutation(updateReportGroupRequest).build());
}
/**
*
* Updates the webhook associated with an AWS CodeBuild build project.
*
*
*
* If you use Bitbucket for your repository, rotateSecret
is ignored.
*
*
*
* @param updateWebhookRequest
* @return Result of the UpdateWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.UpdateWebhook
* @see AWS API
* Documentation
*/
default UpdateWebhookResponse updateWebhook(UpdateWebhookRequest updateWebhookRequest) throws InvalidInputException,
ResourceNotFoundException, OAuthProviderException, AwsServiceException, SdkClientException, CodeBuildException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the webhook associated with an AWS CodeBuild build project.
*
*
*
* If you use Bitbucket for your repository, rotateSecret
is ignored.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateWebhookRequest.Builder} avoiding the need to
* create one manually via {@link UpdateWebhookRequest#builder()}
*
*
* @param updateWebhookRequest
* A {@link Consumer} that will call methods on {@link UpdateWebhookInput.Builder} to create a request.
* @return Result of the UpdateWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified AWS resource cannot be found.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeBuildException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeBuildClient.UpdateWebhook
* @see AWS API
* Documentation
*/
default UpdateWebhookResponse updateWebhook(Consumer updateWebhookRequest)
throws InvalidInputException, ResourceNotFoundException, OAuthProviderException, AwsServiceException,
SdkClientException, CodeBuildException {
return updateWebhook(UpdateWebhookRequest.builder().applyMutation(updateWebhookRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("codebuild");
}
}