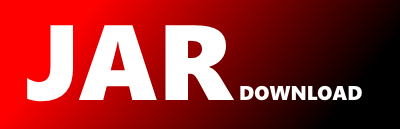
software.amazon.awssdk.services.codebuild.DefaultCodeBuildAsyncClient Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.codebuild.model.AccountLimitExceededException;
import software.amazon.awssdk.services.codebuild.model.BatchDeleteBuildsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchDeleteBuildsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildBatchesRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildBatchesResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetBuildsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetProjectsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetProjectsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportGroupsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportGroupsResponse;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportsRequest;
import software.amazon.awssdk.services.codebuild.model.BatchGetReportsResponse;
import software.amazon.awssdk.services.codebuild.model.CodeBuildException;
import software.amazon.awssdk.services.codebuild.model.CodeBuildRequest;
import software.amazon.awssdk.services.codebuild.model.CreateProjectRequest;
import software.amazon.awssdk.services.codebuild.model.CreateProjectResponse;
import software.amazon.awssdk.services.codebuild.model.CreateReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.CreateReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.CreateWebhookRequest;
import software.amazon.awssdk.services.codebuild.model.CreateWebhookResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteProjectRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteProjectResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteReportRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteReportResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteResourcePolicyRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteResourcePolicyResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteSourceCredentialsRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteSourceCredentialsResponse;
import software.amazon.awssdk.services.codebuild.model.DeleteWebhookRequest;
import software.amazon.awssdk.services.codebuild.model.DeleteWebhookResponse;
import software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest;
import software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesResponse;
import software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest;
import software.amazon.awssdk.services.codebuild.model.DescribeTestCasesResponse;
import software.amazon.awssdk.services.codebuild.model.GetReportGroupTrendRequest;
import software.amazon.awssdk.services.codebuild.model.GetReportGroupTrendResponse;
import software.amazon.awssdk.services.codebuild.model.GetResourcePolicyRequest;
import software.amazon.awssdk.services.codebuild.model.GetResourcePolicyResponse;
import software.amazon.awssdk.services.codebuild.model.ImportSourceCredentialsRequest;
import software.amazon.awssdk.services.codebuild.model.ImportSourceCredentialsResponse;
import software.amazon.awssdk.services.codebuild.model.InvalidInputException;
import software.amazon.awssdk.services.codebuild.model.InvalidateProjectCacheRequest;
import software.amazon.awssdk.services.codebuild.model.InvalidateProjectCacheResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildBatchesResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectResponse;
import software.amazon.awssdk.services.codebuild.model.ListBuildsRequest;
import software.amazon.awssdk.services.codebuild.model.ListBuildsResponse;
import software.amazon.awssdk.services.codebuild.model.ListCuratedEnvironmentImagesRequest;
import software.amazon.awssdk.services.codebuild.model.ListCuratedEnvironmentImagesResponse;
import software.amazon.awssdk.services.codebuild.model.ListProjectsRequest;
import software.amazon.awssdk.services.codebuild.model.ListProjectsResponse;
import software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest;
import software.amazon.awssdk.services.codebuild.model.ListReportGroupsResponse;
import software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.ListReportsRequest;
import software.amazon.awssdk.services.codebuild.model.ListReportsResponse;
import software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest;
import software.amazon.awssdk.services.codebuild.model.ListSharedProjectsResponse;
import software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest;
import software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsResponse;
import software.amazon.awssdk.services.codebuild.model.ListSourceCredentialsRequest;
import software.amazon.awssdk.services.codebuild.model.ListSourceCredentialsResponse;
import software.amazon.awssdk.services.codebuild.model.OAuthProviderException;
import software.amazon.awssdk.services.codebuild.model.PutResourcePolicyRequest;
import software.amazon.awssdk.services.codebuild.model.PutResourcePolicyResponse;
import software.amazon.awssdk.services.codebuild.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.codebuild.model.ResourceNotFoundException;
import software.amazon.awssdk.services.codebuild.model.RetryBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.RetryBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.RetryBuildRequest;
import software.amazon.awssdk.services.codebuild.model.RetryBuildResponse;
import software.amazon.awssdk.services.codebuild.model.StartBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.StartBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.StartBuildRequest;
import software.amazon.awssdk.services.codebuild.model.StartBuildResponse;
import software.amazon.awssdk.services.codebuild.model.StopBuildBatchRequest;
import software.amazon.awssdk.services.codebuild.model.StopBuildBatchResponse;
import software.amazon.awssdk.services.codebuild.model.StopBuildRequest;
import software.amazon.awssdk.services.codebuild.model.StopBuildResponse;
import software.amazon.awssdk.services.codebuild.model.UpdateProjectRequest;
import software.amazon.awssdk.services.codebuild.model.UpdateProjectResponse;
import software.amazon.awssdk.services.codebuild.model.UpdateReportGroupRequest;
import software.amazon.awssdk.services.codebuild.model.UpdateReportGroupResponse;
import software.amazon.awssdk.services.codebuild.model.UpdateWebhookRequest;
import software.amazon.awssdk.services.codebuild.model.UpdateWebhookResponse;
import software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesPublisher;
import software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListBuildsPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListProjectsPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListReportsPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsPublisher;
import software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsPublisher;
import software.amazon.awssdk.services.codebuild.transform.BatchDeleteBuildsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.BatchGetBuildBatchesRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.BatchGetBuildsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.BatchGetProjectsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.BatchGetReportGroupsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.BatchGetReportsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.CreateProjectRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.CreateReportGroupRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.CreateWebhookRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DeleteBuildBatchRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DeleteProjectRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DeleteReportGroupRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DeleteReportRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DeleteResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DeleteSourceCredentialsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DeleteWebhookRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DescribeCodeCoveragesRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.DescribeTestCasesRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.GetReportGroupTrendRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.GetResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ImportSourceCredentialsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.InvalidateProjectCacheRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListBuildBatchesForProjectRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListBuildBatchesRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListBuildsForProjectRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListBuildsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListCuratedEnvironmentImagesRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListProjectsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListReportGroupsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListReportsForReportGroupRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListReportsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListSharedProjectsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListSharedReportGroupsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.ListSourceCredentialsRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.PutResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.RetryBuildBatchRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.RetryBuildRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.StartBuildBatchRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.StartBuildRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.StopBuildBatchRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.StopBuildRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.UpdateProjectRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.UpdateReportGroupRequestMarshaller;
import software.amazon.awssdk.services.codebuild.transform.UpdateWebhookRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link CodeBuildAsyncClient}.
*
* @see CodeBuildAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCodeBuildAsyncClient implements CodeBuildAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultCodeBuildAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCodeBuildAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Deletes one or more builds.
*
*
* @param batchDeleteBuildsRequest
* @return A Java Future containing the result of the BatchDeleteBuilds operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.BatchDeleteBuilds
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchDeleteBuilds(BatchDeleteBuildsRequest batchDeleteBuildsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteBuildsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteBuilds");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteBuildsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteBuilds")
.withMarshaller(new BatchDeleteBuildsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(batchDeleteBuildsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = batchDeleteBuildsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about one or more batch builds.
*
*
* @param batchGetBuildBatchesRequest
* @return A Java Future containing the result of the BatchGetBuildBatches operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.BatchGetBuildBatches
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchGetBuildBatches(
BatchGetBuildBatchesRequest batchGetBuildBatchesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetBuildBatchesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetBuildBatches");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetBuildBatchesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetBuildBatches")
.withMarshaller(new BatchGetBuildBatchesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(batchGetBuildBatchesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = batchGetBuildBatchesRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about one or more builds.
*
*
* @param batchGetBuildsRequest
* @return A Java Future containing the result of the BatchGetBuilds operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.BatchGetBuilds
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetBuilds(BatchGetBuildsRequest batchGetBuildsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetBuildsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetBuilds");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetBuildsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetBuilds")
.withMarshaller(new BatchGetBuildsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(batchGetBuildsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = batchGetBuildsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about one or more build projects.
*
*
* @param batchGetProjectsRequest
* @return A Java Future containing the result of the BatchGetProjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.BatchGetProjects
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetProjects(BatchGetProjectsRequest batchGetProjectsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetProjectsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetProjects");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetProjectsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetProjects")
.withMarshaller(new BatchGetProjectsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(batchGetProjectsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = batchGetProjectsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of report groups.
*
*
* @param batchGetReportGroupsRequest
* @return A Java Future containing the result of the BatchGetReportGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.BatchGetReportGroups
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture batchGetReportGroups(
BatchGetReportGroupsRequest batchGetReportGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetReportGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetReportGroups");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetReportGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetReportGroups")
.withMarshaller(new BatchGetReportGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(batchGetReportGroupsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = batchGetReportGroupsRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns an array of reports.
*
*
* @param batchGetReportsRequest
* @return A Java Future containing the result of the BatchGetReports operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.BatchGetReports
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture batchGetReports(BatchGetReportsRequest batchGetReportsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetReportsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetReports");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetReportsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetReports")
.withMarshaller(new BatchGetReportsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(batchGetReportsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = batchGetReportsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a build project.
*
*
* @param createProjectRequest
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceAlreadyExistsException The specified AWS resource cannot be created, because an AWS resource
* with the same settings already exists.
* - AccountLimitExceededException An AWS service limit was exceeded for the calling AWS account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.CreateProject
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createProject(CreateProjectRequest createProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateProject")
.withMarshaller(new CreateProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createProjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createProjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a report group. A report group contains a collection of reports.
*
*
* @param createReportGroupRequest
* @return A Java Future containing the result of the CreateReportGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceAlreadyExistsException The specified AWS resource cannot be created, because an AWS resource
* with the same settings already exists.
* - AccountLimitExceededException An AWS service limit was exceeded for the calling AWS account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.CreateReportGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createReportGroup(CreateReportGroupRequest createReportGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createReportGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateReportGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateReportGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateReportGroup")
.withMarshaller(new CreateReportGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createReportGroupRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createReportGroupRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* For an existing AWS CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* enables AWS CodeBuild to start rebuilding the source code every time a code change is pushed to the repository.
*
*
*
* If you enable webhooks for an AWS CodeBuild project, and the project is used as a build step in AWS CodePipeline,
* then two identical builds are created for each commit. One build is triggered through webhooks, and one through
* AWS CodePipeline. Because billing is on a per-build basis, you are billed for both builds. Therefore, if you are
* using AWS CodePipeline, we recommend that you disable webhooks in AWS CodeBuild. In the AWS CodeBuild console,
* clear the Webhook box. For more information, see step 5 in Change a
* Build Project's Settings.
*
*
*
* @param createWebhookRequest
* @return A Java Future containing the result of the CreateWebhook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - OAuthProviderException There was a problem with the underlying OAuth provider.
* - ResourceAlreadyExistsException The specified AWS resource cannot be created, because an AWS resource
* with the same settings already exists.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.CreateWebhook
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createWebhook(CreateWebhookRequest createWebhookRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createWebhookRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateWebhook");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateWebhookResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateWebhook")
.withMarshaller(new CreateWebhookRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createWebhookRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createWebhookRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a batch build.
*
*
* @param deleteBuildBatchRequest
* @return A Java Future containing the result of the DeleteBuildBatch operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DeleteBuildBatch
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteBuildBatch(DeleteBuildBatchRequest deleteBuildBatchRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBuildBatchRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBuildBatch");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteBuildBatchResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBuildBatch")
.withMarshaller(new DeleteBuildBatchRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteBuildBatchRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBuildBatchRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a build project. When you delete a project, its builds are not deleted.
*
*
* @param deleteProjectRequest
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DeleteProject
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteProject(DeleteProjectRequest deleteProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProject")
.withMarshaller(new DeleteProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteProjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteProjectRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a report.
*
*
* @param deleteReportRequest
* @return A Java Future containing the result of the DeleteReport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DeleteReport
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteReport(DeleteReportRequest deleteReportRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteReportRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteReport");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteReportResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteReport").withMarshaller(new DeleteReportRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteReportRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteReportRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a report group. Before you delete a report group, you must delete its reports.
*
*
* @param deleteReportGroupRequest
* @return A Java Future containing the result of the DeleteReportGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DeleteReportGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteReportGroup(DeleteReportGroupRequest deleteReportGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteReportGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteReportGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteReportGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteReportGroup")
.withMarshaller(new DeleteReportGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteReportGroupRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteReportGroupRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a resource policy that is identified by its resource ARN.
*
*
* @param deleteResourcePolicyRequest
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteResourcePolicy(
DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteResourcePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteResourcePolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteResourcePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteResourcePolicy")
.withMarshaller(new DeleteResourcePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteResourcePolicyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteResourcePolicyRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a set of GitHub, GitHub Enterprise, or Bitbucket source credentials.
*
*
* @param deleteSourceCredentialsRequest
* @return A Java Future containing the result of the DeleteSourceCredentials operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DeleteSourceCredentials
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteSourceCredentials(
DeleteSourceCredentialsRequest deleteSourceCredentialsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSourceCredentialsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSourceCredentials");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSourceCredentialsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSourceCredentials")
.withMarshaller(new DeleteSourceCredentialsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteSourceCredentialsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteSourceCredentialsRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* For an existing AWS CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* stops AWS CodeBuild from rebuilding the source code every time a code change is pushed to the repository.
*
*
* @param deleteWebhookRequest
* @return A Java Future containing the result of the DeleteWebhook operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - OAuthProviderException There was a problem with the underlying OAuth provider.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DeleteWebhook
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteWebhook(DeleteWebhookRequest deleteWebhookRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteWebhookRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteWebhook");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteWebhookResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteWebhook")
.withMarshaller(new DeleteWebhookRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteWebhookRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteWebhookRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves one or more code coverage reports.
*
*
* @param describeCodeCoveragesRequest
* @return A Java Future containing the result of the DescribeCodeCoverages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DescribeCodeCoverages
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeCodeCoverages(
DescribeCodeCoveragesRequest describeCodeCoveragesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCodeCoveragesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCodeCoverages");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeCodeCoveragesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCodeCoverages")
.withMarshaller(new DescribeCodeCoveragesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeCodeCoveragesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeCodeCoveragesRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves one or more code coverage reports.
*
*
*
* This is a variant of
* {@link #describeCodeCoverages(software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesPublisher publisher = client.describeCodeCoveragesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeCodeCoveragesPublisher publisher = client.describeCodeCoveragesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCodeCoverages(software.amazon.awssdk.services.codebuild.model.DescribeCodeCoveragesRequest)}
* operation.
*
*
* @param describeCodeCoveragesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DescribeCodeCoverages
* @see AWS API Documentation
*/
public DescribeCodeCoveragesPublisher describeCodeCoveragesPaginator(DescribeCodeCoveragesRequest describeCodeCoveragesRequest) {
return new DescribeCodeCoveragesPublisher(this, applyPaginatorUserAgent(describeCodeCoveragesRequest));
}
/**
*
* Returns a list of details about test cases for a report.
*
*
* @param describeTestCasesRequest
* @return A Java Future containing the result of the DescribeTestCases operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DescribeTestCases
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeTestCases(DescribeTestCasesRequest describeTestCasesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTestCasesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTestCases");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeTestCasesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTestCases")
.withMarshaller(new DescribeTestCasesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeTestCasesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeTestCasesRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of details about test cases for a report.
*
*
*
* This is a variant of
* {@link #describeTestCases(software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesPublisher publisher = client.describeTestCasesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.DescribeTestCasesPublisher publisher = client.describeTestCasesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.DescribeTestCasesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTestCases(software.amazon.awssdk.services.codebuild.model.DescribeTestCasesRequest)}
* operation.
*
*
* @param describeTestCasesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.DescribeTestCases
* @see AWS
* API Documentation
*/
public DescribeTestCasesPublisher describeTestCasesPaginator(DescribeTestCasesRequest describeTestCasesRequest) {
return new DescribeTestCasesPublisher(this, applyPaginatorUserAgent(describeTestCasesRequest));
}
/**
*
* Analyzes and accumulates test report values for the specified test reports.
*
*
* @param getReportGroupTrendRequest
* @return A Java Future containing the result of the GetReportGroupTrend operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.GetReportGroupTrend
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getReportGroupTrend(
GetReportGroupTrendRequest getReportGroupTrendRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getReportGroupTrendRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetReportGroupTrend");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetReportGroupTrendResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetReportGroupTrend")
.withMarshaller(new GetReportGroupTrendRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getReportGroupTrendRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getReportGroupTrendRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a resource policy that is identified by its resource ARN.
*
*
* @param getResourcePolicyRequest
* @return A Java Future containing the result of the GetResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.GetResourcePolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getResourcePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetResourcePolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetResourcePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetResourcePolicy")
.withMarshaller(new GetResourcePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getResourcePolicyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = getResourcePolicyRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Imports the source repository credentials for an AWS CodeBuild project that has its source code stored in a
* GitHub, GitHub Enterprise, or Bitbucket repository.
*
*
* @param importSourceCredentialsRequest
* @return A Java Future containing the result of the ImportSourceCredentials operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - AccountLimitExceededException An AWS service limit was exceeded for the calling AWS account.
* - ResourceAlreadyExistsException The specified AWS resource cannot be created, because an AWS resource
* with the same settings already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ImportSourceCredentials
* @see AWS API Documentation
*/
@Override
public CompletableFuture importSourceCredentials(
ImportSourceCredentialsRequest importSourceCredentialsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, importSourceCredentialsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportSourceCredentials");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportSourceCredentialsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ImportSourceCredentials")
.withMarshaller(new ImportSourceCredentialsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(importSourceCredentialsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = importSourceCredentialsRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Resets the cache for a project.
*
*
* @param invalidateProjectCacheRequest
* @return A Java Future containing the result of the InvalidateProjectCache operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.InvalidateProjectCache
* @see AWS API Documentation
*/
@Override
public CompletableFuture invalidateProjectCache(
InvalidateProjectCacheRequest invalidateProjectCacheRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, invalidateProjectCacheRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "InvalidateProjectCache");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, InvalidateProjectCacheResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("InvalidateProjectCache")
.withMarshaller(new InvalidateProjectCacheRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(invalidateProjectCacheRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = invalidateProjectCacheRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the identifiers of your build batches in the current region.
*
*
* @param listBuildBatchesRequest
* @return A Java Future containing the result of the ListBuildBatches operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuildBatches
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listBuildBatches(ListBuildBatchesRequest listBuildBatchesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBuildBatchesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBuildBatches");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBuildBatchesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBuildBatches")
.withMarshaller(new ListBuildBatchesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listBuildBatchesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listBuildBatchesRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the identifiers of the build batches for a specific project.
*
*
* @param listBuildBatchesForProjectRequest
* @return A Java Future containing the result of the ListBuildBatchesForProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuildBatchesForProject
* @see AWS API Documentation
*/
@Override
public CompletableFuture listBuildBatchesForProject(
ListBuildBatchesForProjectRequest listBuildBatchesForProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBuildBatchesForProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBuildBatchesForProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBuildBatchesForProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBuildBatchesForProject")
.withMarshaller(new ListBuildBatchesForProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listBuildBatchesForProjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listBuildBatchesForProjectRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the identifiers of the build batches for a specific project.
*
*
*
* This is a variant of
* {@link #listBuildBatchesForProject(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectPublisher publisher = client.listBuildBatchesForProjectPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesForProjectPublisher publisher = client.listBuildBatchesForProjectPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildBatchesForProject(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesForProjectRequest)}
* operation.
*
*
* @param listBuildBatchesForProjectRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuildBatchesForProject
* @see AWS API Documentation
*/
public ListBuildBatchesForProjectPublisher listBuildBatchesForProjectPaginator(
ListBuildBatchesForProjectRequest listBuildBatchesForProjectRequest) {
return new ListBuildBatchesForProjectPublisher(this, applyPaginatorUserAgent(listBuildBatchesForProjectRequest));
}
/**
*
* Retrieves the identifiers of your build batches in the current region.
*
*
*
* This is a variant of
* {@link #listBuildBatches(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesPublisher publisher = client.listBuildBatchesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildBatchesPublisher publisher = client.listBuildBatchesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildBatches(software.amazon.awssdk.services.codebuild.model.ListBuildBatchesRequest)} operation.
*
*
* @param listBuildBatchesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuildBatches
* @see AWS API
* Documentation
*/
public ListBuildBatchesPublisher listBuildBatchesPaginator(ListBuildBatchesRequest listBuildBatchesRequest) {
return new ListBuildBatchesPublisher(this, applyPaginatorUserAgent(listBuildBatchesRequest));
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
* @param listBuildsRequest
* @return A Java Future containing the result of the ListBuilds operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuilds
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listBuilds(ListBuildsRequest listBuildsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBuildsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBuilds");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListBuildsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListBuilds")
.withMarshaller(new ListBuildsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listBuildsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listBuildsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a list of build identifiers for the specified build project, with each build identifier representing a
* single build.
*
*
* @param listBuildsForProjectRequest
* @return A Java Future containing the result of the ListBuildsForProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuildsForProject
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listBuildsForProject(
ListBuildsForProjectRequest listBuildsForProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBuildsForProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBuildsForProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBuildsForProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBuildsForProject")
.withMarshaller(new ListBuildsForProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listBuildsForProjectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listBuildsForProjectRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a list of build identifiers for the specified build project, with each build identifier representing a
* single build.
*
*
*
* This is a variant of
* {@link #listBuildsForProject(software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectPublisher publisher = client.listBuildsForProjectPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsForProjectPublisher publisher = client.listBuildsForProjectPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuildsForProject(software.amazon.awssdk.services.codebuild.model.ListBuildsForProjectRequest)}
* operation.
*
*
* @param listBuildsForProjectRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuildsForProject
* @see AWS
* API Documentation
*/
public ListBuildsForProjectPublisher listBuildsForProjectPaginator(ListBuildsForProjectRequest listBuildsForProjectRequest) {
return new ListBuildsForProjectPublisher(this, applyPaginatorUserAgent(listBuildsForProjectRequest));
}
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
*
* This is a variant of {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsPublisher publisher = client.listBuildsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListBuildsPublisher publisher = client.listBuildsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListBuildsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBuilds(software.amazon.awssdk.services.codebuild.model.ListBuildsRequest)} operation.
*
*
* @param listBuildsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListBuilds
* @see AWS API
* Documentation
*/
public ListBuildsPublisher listBuildsPaginator(ListBuildsRequest listBuildsRequest) {
return new ListBuildsPublisher(this, applyPaginatorUserAgent(listBuildsRequest));
}
/**
*
* Gets information about Docker images that are managed by AWS CodeBuild.
*
*
* @param listCuratedEnvironmentImagesRequest
* @return A Java Future containing the result of the ListCuratedEnvironmentImages operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListCuratedEnvironmentImages
* @see AWS API Documentation
*/
@Override
public CompletableFuture listCuratedEnvironmentImages(
ListCuratedEnvironmentImagesRequest listCuratedEnvironmentImagesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCuratedEnvironmentImagesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCuratedEnvironmentImages");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCuratedEnvironmentImagesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCuratedEnvironmentImages")
.withMarshaller(new ListCuratedEnvironmentImagesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listCuratedEnvironmentImagesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listCuratedEnvironmentImagesRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
* @param listProjectsRequest
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListProjects
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listProjects(ListProjectsRequest listProjectsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProjectsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProjects");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListProjectsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProjects").withMarshaller(new ListProjectsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listProjectsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listProjectsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
*
* This is a variant of {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsPublisher publisher = client.listProjectsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListProjectsPublisher publisher = client.listProjectsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListProjectsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.codebuild.model.ListProjectsRequest)} operation.
*
*
* @param listProjectsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListProjects
* @see AWS API
* Documentation
*/
public ListProjectsPublisher listProjectsPaginator(ListProjectsRequest listProjectsRequest) {
return new ListProjectsPublisher(this, applyPaginatorUserAgent(listProjectsRequest));
}
/**
*
* Gets a list ARNs for the report groups in the current AWS account.
*
*
* @param listReportGroupsRequest
* @return A Java Future containing the result of the ListReportGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListReportGroups
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listReportGroups(ListReportGroupsRequest listReportGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listReportGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReportGroups");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListReportGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListReportGroups")
.withMarshaller(new ListReportGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listReportGroupsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listReportGroupsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a list ARNs for the report groups in the current AWS account.
*
*
*
* This is a variant of
* {@link #listReportGroups(software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsPublisher publisher = client.listReportGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportGroupsPublisher publisher = client.listReportGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListReportGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReportGroups(software.amazon.awssdk.services.codebuild.model.ListReportGroupsRequest)} operation.
*
*
* @param listReportGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListReportGroups
* @see AWS API
* Documentation
*/
public ListReportGroupsPublisher listReportGroupsPaginator(ListReportGroupsRequest listReportGroupsRequest) {
return new ListReportGroupsPublisher(this, applyPaginatorUserAgent(listReportGroupsRequest));
}
/**
*
* Returns a list of ARNs for the reports in the current AWS account.
*
*
* @param listReportsRequest
* @return A Java Future containing the result of the ListReports operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListReports
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listReports(ListReportsRequest listReportsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listReportsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReports");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListReportsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListReports").withMarshaller(new ListReportsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listReportsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listReportsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of ARNs for the reports that belong to a ReportGroup
.
*
*
* @param listReportsForReportGroupRequest
* @return A Java Future containing the result of the ListReportsForReportGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListReportsForReportGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture listReportsForReportGroup(
ListReportsForReportGroupRequest listReportsForReportGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listReportsForReportGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReportsForReportGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListReportsForReportGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListReportsForReportGroup")
.withMarshaller(new ListReportsForReportGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listReportsForReportGroupRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listReportsForReportGroupRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of ARNs for the reports that belong to a ReportGroup
.
*
*
*
* This is a variant of
* {@link #listReportsForReportGroup(software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupPublisher publisher = client.listReportsForReportGroupPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsForReportGroupPublisher publisher = client.listReportsForReportGroupPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReportsForReportGroup(software.amazon.awssdk.services.codebuild.model.ListReportsForReportGroupRequest)}
* operation.
*
*
* @param listReportsForReportGroupRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListReportsForReportGroup
* @see AWS API Documentation
*/
public ListReportsForReportGroupPublisher listReportsForReportGroupPaginator(
ListReportsForReportGroupRequest listReportsForReportGroupRequest) {
return new ListReportsForReportGroupPublisher(this, applyPaginatorUserAgent(listReportsForReportGroupRequest));
}
/**
*
* Returns a list of ARNs for the reports in the current AWS account.
*
*
*
* This is a variant of {@link #listReports(software.amazon.awssdk.services.codebuild.model.ListReportsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsPublisher publisher = client.listReportsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListReportsPublisher publisher = client.listReportsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListReportsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReports(software.amazon.awssdk.services.codebuild.model.ListReportsRequest)} operation.
*
*
* @param listReportsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListReports
* @see AWS API
* Documentation
*/
public ListReportsPublisher listReportsPaginator(ListReportsRequest listReportsRequest) {
return new ListReportsPublisher(this, applyPaginatorUserAgent(listReportsRequest));
}
/**
*
* Gets a list of projects that are shared with other AWS accounts or users.
*
*
* @param listSharedProjectsRequest
* @return A Java Future containing the result of the ListSharedProjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListSharedProjects
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listSharedProjects(ListSharedProjectsRequest listSharedProjectsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSharedProjectsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSharedProjects");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSharedProjectsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSharedProjects")
.withMarshaller(new ListSharedProjectsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listSharedProjectsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listSharedProjectsRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a list of projects that are shared with other AWS accounts or users.
*
*
*
* This is a variant of
* {@link #listSharedProjects(software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsPublisher publisher = client.listSharedProjectsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedProjectsPublisher publisher = client.listSharedProjectsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListSharedProjectsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSharedProjects(software.amazon.awssdk.services.codebuild.model.ListSharedProjectsRequest)}
* operation.
*
*
* @param listSharedProjectsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListSharedProjects
* @see AWS
* API Documentation
*/
public ListSharedProjectsPublisher listSharedProjectsPaginator(ListSharedProjectsRequest listSharedProjectsRequest) {
return new ListSharedProjectsPublisher(this, applyPaginatorUserAgent(listSharedProjectsRequest));
}
/**
*
* Gets a list of report groups that are shared with other AWS accounts or users.
*
*
* @param listSharedReportGroupsRequest
* @return A Java Future containing the result of the ListSharedReportGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListSharedReportGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture listSharedReportGroups(
ListSharedReportGroupsRequest listSharedReportGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSharedReportGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSharedReportGroups");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSharedReportGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSharedReportGroups")
.withMarshaller(new ListSharedReportGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listSharedReportGroupsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listSharedReportGroupsRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets a list of report groups that are shared with other AWS accounts or users.
*
*
*
* This is a variant of
* {@link #listSharedReportGroups(software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsPublisher publisher = client.listSharedReportGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codebuild.paginators.ListSharedReportGroupsPublisher publisher = client.listSharedReportGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSharedReportGroups(software.amazon.awssdk.services.codebuild.model.ListSharedReportGroupsRequest)}
* operation.
*
*
* @param listSharedReportGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListSharedReportGroups
* @see AWS API Documentation
*/
public ListSharedReportGroupsPublisher listSharedReportGroupsPaginator(
ListSharedReportGroupsRequest listSharedReportGroupsRequest) {
return new ListSharedReportGroupsPublisher(this, applyPaginatorUserAgent(listSharedReportGroupsRequest));
}
/**
*
* Returns a list of SourceCredentialsInfo
objects.
*
*
* @param listSourceCredentialsRequest
* @return A Java Future containing the result of the ListSourceCredentials operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.ListSourceCredentials
* @see AWS API Documentation
*/
@Override
public CompletableFuture listSourceCredentials(
ListSourceCredentialsRequest listSourceCredentialsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSourceCredentialsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSourceCredentials");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSourceCredentialsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSourceCredentials")
.withMarshaller(new ListSourceCredentialsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listSourceCredentialsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listSourceCredentialsRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Stores a resource policy for the ARN of a Project
or ReportGroup
object.
*
*
* @param putResourcePolicyRequest
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - InvalidInputException The input value that was provided is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.PutResourcePolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putResourcePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutResourcePolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutResourcePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutResourcePolicy")
.withMarshaller(new PutResourcePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putResourcePolicyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = putResourcePolicyRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Restarts a build.
*
*
* @param retryBuildRequest
* @return A Java Future containing the result of the RetryBuild operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The input value that was provided is not valid.
* - ResourceNotFoundException The specified AWS resource cannot be found.
* - AccountLimitExceededException An AWS service limit was exceeded for the calling AWS account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeBuildException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeBuildAsyncClient.RetryBuild
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture retryBuild(RetryBuildRequest retryBuildRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, retryBuildRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeBuild");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RetryBuild");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RetryBuildResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams