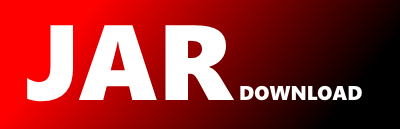
software.amazon.awssdk.services.codebuild.model.ProjectSource Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the build input source code for the build project.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProjectSource implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(ProjectSource::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField LOCATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("location").getter(getter(ProjectSource::location)).setter(setter(Builder::location))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("location").build()).build();
private static final SdkField GIT_CLONE_DEPTH_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("gitCloneDepth").getter(getter(ProjectSource::gitCloneDepth)).setter(setter(Builder::gitCloneDepth))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("gitCloneDepth").build()).build();
private static final SdkField GIT_SUBMODULES_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("gitSubmodulesConfig")
.getter(getter(ProjectSource::gitSubmodulesConfig)).setter(setter(Builder::gitSubmodulesConfig))
.constructor(GitSubmodulesConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("gitSubmodulesConfig").build())
.build();
private static final SdkField BUILDSPEC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildspec").getter(getter(ProjectSource::buildspec)).setter(setter(Builder::buildspec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildspec").build()).build();
private static final SdkField AUTH_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("auth").getter(getter(ProjectSource::auth)).setter(setter(Builder::auth))
.constructor(SourceAuth::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("auth").build()).build();
private static final SdkField REPORT_BUILD_STATUS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("reportBuildStatus").getter(getter(ProjectSource::reportBuildStatus))
.setter(setter(Builder::reportBuildStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reportBuildStatus").build()).build();
private static final SdkField BUILD_STATUS_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("buildStatusConfig")
.getter(getter(ProjectSource::buildStatusConfig)).setter(setter(Builder::buildStatusConfig))
.constructor(BuildStatusConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildStatusConfig").build()).build();
private static final SdkField INSECURE_SSL_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("insecureSsl").getter(getter(ProjectSource::insecureSsl)).setter(setter(Builder::insecureSsl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("insecureSsl").build()).build();
private static final SdkField SOURCE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceIdentifier").getter(getter(ProjectSource::sourceIdentifier))
.setter(setter(Builder::sourceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceIdentifier").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD, LOCATION_FIELD,
GIT_CLONE_DEPTH_FIELD, GIT_SUBMODULES_CONFIG_FIELD, BUILDSPEC_FIELD, AUTH_FIELD, REPORT_BUILD_STATUS_FIELD,
BUILD_STATUS_CONFIG_FIELD, INSECURE_SSL_FIELD, SOURCE_IDENTIFIER_FIELD));
private static final long serialVersionUID = 1L;
private final String type;
private final String location;
private final Integer gitCloneDepth;
private final GitSubmodulesConfig gitSubmodulesConfig;
private final String buildspec;
private final SourceAuth auth;
private final Boolean reportBuildStatus;
private final BuildStatusConfig buildStatusConfig;
private final Boolean insecureSsl;
private final String sourceIdentifier;
private ProjectSource(BuilderImpl builder) {
this.type = builder.type;
this.location = builder.location;
this.gitCloneDepth = builder.gitCloneDepth;
this.gitSubmodulesConfig = builder.gitSubmodulesConfig;
this.buildspec = builder.buildspec;
this.auth = builder.auth;
this.reportBuildStatus = builder.reportBuildStatus;
this.buildStatusConfig = builder.buildStatusConfig;
this.insecureSsl = builder.insecureSsl;
this.sourceIdentifier = builder.sourceIdentifier;
}
/**
*
* The type of repository that contains the source code to be built. Valid values include:
*
*
* -
*
* BITBUCKET
: The source code is in a Bitbucket repository.
*
*
* -
*
* CODECOMMIT
: The source code is in an CodeCommit repository.
*
*
* -
*
* CODEPIPELINE
: The source code settings are specified in the source action of a pipeline in
* CodePipeline.
*
*
* -
*
* GITHUB
: The source code is in a GitHub or GitHub Enterprise Cloud repository.
*
*
* -
*
* GITHUB_ENTERPRISE
: The source code is in a GitHub Enterprise Server repository.
*
*
* -
*
* NO_SOURCE
: The project does not have input source code.
*
*
* -
*
* S3
: The source code is in an Amazon S3 bucket.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of repository that contains the source code to be built. Valid values include:
*
* -
*
* BITBUCKET
: The source code is in a Bitbucket repository.
*
*
* -
*
* CODECOMMIT
: The source code is in an CodeCommit repository.
*
*
* -
*
* CODEPIPELINE
: The source code settings are specified in the source action of a pipeline in
* CodePipeline.
*
*
* -
*
* GITHUB
: The source code is in a GitHub or GitHub Enterprise Cloud repository.
*
*
* -
*
* GITHUB_ENTERPRISE
: The source code is in a GitHub Enterprise Server repository.
*
*
* -
*
* NO_SOURCE
: The project does not have input source code.
*
*
* -
*
* S3
: The source code is in an Amazon S3 bucket.
*
*
* @see SourceType
*/
public final SourceType type() {
return SourceType.fromValue(type);
}
/**
*
* The type of repository that contains the source code to be built. Valid values include:
*
*
* -
*
* BITBUCKET
: The source code is in a Bitbucket repository.
*
*
* -
*
* CODECOMMIT
: The source code is in an CodeCommit repository.
*
*
* -
*
* CODEPIPELINE
: The source code settings are specified in the source action of a pipeline in
* CodePipeline.
*
*
* -
*
* GITHUB
: The source code is in a GitHub or GitHub Enterprise Cloud repository.
*
*
* -
*
* GITHUB_ENTERPRISE
: The source code is in a GitHub Enterprise Server repository.
*
*
* -
*
* NO_SOURCE
: The project does not have input source code.
*
*
* -
*
* S3
: The source code is in an Amazon S3 bucket.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of repository that contains the source code to be built. Valid values include:
*
* -
*
* BITBUCKET
: The source code is in a Bitbucket repository.
*
*
* -
*
* CODECOMMIT
: The source code is in an CodeCommit repository.
*
*
* -
*
* CODEPIPELINE
: The source code settings are specified in the source action of a pipeline in
* CodePipeline.
*
*
* -
*
* GITHUB
: The source code is in a GitHub or GitHub Enterprise Cloud repository.
*
*
* -
*
* GITHUB_ENTERPRISE
: The source code is in a GitHub Enterprise Server repository.
*
*
* -
*
* NO_SOURCE
: The project does not have input source code.
*
*
* -
*
* S3
: The source code is in an Amazon S3 bucket.
*
*
* @see SourceType
*/
public final String typeAsString() {
return type;
}
/**
*
* Information about the location of the source code to be built. Valid values include:
*
*
* -
*
* For source code settings that are specified in the source action of a pipeline in CodePipeline,
* location
should not be specified. If it is specified, CodePipeline ignores it. This is because
* CodePipeline uses the settings in a pipeline's source action instead of this value.
*
*
* -
*
* For source code in an CodeCommit repository, the HTTPS clone URL to the repository that contains the source code
* and the buildspec file (for example,
* https://git-codecommit.<region-ID>.amazonaws.com/v1/repos/<repo-name>
).
*
*
* -
*
* For source code in an Amazon S3 input bucket, one of the following.
*
*
* -
*
* The path to the ZIP file that contains the source code (for example,
* <bucket-name>/<path>/<object-name>.zip
).
*
*
* -
*
* The path to the folder that contains the source code (for example,
* <bucket-name>/<path-to-source-code>/<folder>/
).
*
*
*
*
* -
*
* For source code in a GitHub repository, the HTTPS clone URL to the repository that contains the source and the
* buildspec file. You must connect your Amazon Web Services account to your GitHub account. Use the CodeBuild
* console to start creating a build project. When you use the console to connect (or reconnect) with GitHub, on the
* GitHub Authorize application page, for Organization access, choose Request access next to
* each repository you want to allow CodeBuild to have access to, and then choose Authorize application.
* (After you have connected to your GitHub account, you do not need to finish creating the build project. You can
* leave the CodeBuild console.) To instruct CodeBuild to use this connection, in the source
object,
* set the auth
object's type
value to OAUTH
.
*
*
* -
*
* For source code in a Bitbucket repository, the HTTPS clone URL to the repository that contains the source and the
* buildspec file. You must connect your Amazon Web Services account to your Bitbucket account. Use the CodeBuild
* console to start creating a build project. When you use the console to connect (or reconnect) with Bitbucket, on
* the Bitbucket Confirm access to your account page, choose Grant access. (After you have connected
* to your Bitbucket account, you do not need to finish creating the build project. You can leave the CodeBuild
* console.) To instruct CodeBuild to use this connection, in the source
object, set the
* auth
object's type
value to OAUTH
.
*
*
*
*
* If you specify CODEPIPELINE
for the Type
property, don't specify this property. For all
* of the other types, you must specify Location
.
*
*
* @return Information about the location of the source code to be built. Valid values include:
*
* -
*
* For source code settings that are specified in the source action of a pipeline in CodePipeline,
* location
should not be specified. If it is specified, CodePipeline ignores it. This is
* because CodePipeline uses the settings in a pipeline's source action instead of this value.
*
*
* -
*
* For source code in an CodeCommit repository, the HTTPS clone URL to the repository that contains the
* source code and the buildspec file (for example,
* https://git-codecommit.<region-ID>.amazonaws.com/v1/repos/<repo-name>
).
*
*
* -
*
* For source code in an Amazon S3 input bucket, one of the following.
*
*
* -
*
* The path to the ZIP file that contains the source code (for example,
* <bucket-name>/<path>/<object-name>.zip
).
*
*
* -
*
* The path to the folder that contains the source code (for example,
* <bucket-name>/<path-to-source-code>/<folder>/
).
*
*
*
*
* -
*
* For source code in a GitHub repository, the HTTPS clone URL to the repository that contains the source
* and the buildspec file. You must connect your Amazon Web Services account to your GitHub account. Use the
* CodeBuild console to start creating a build project. When you use the console to connect (or reconnect)
* with GitHub, on the GitHub Authorize application page, for Organization access, choose
* Request access next to each repository you want to allow CodeBuild to have access to, and then
* choose Authorize application. (After you have connected to your GitHub account, you do not need to
* finish creating the build project. You can leave the CodeBuild console.) To instruct CodeBuild to use
* this connection, in the source
object, set the auth
object's type
* value to OAUTH
.
*
*
* -
*
* For source code in a Bitbucket repository, the HTTPS clone URL to the repository that contains the source
* and the buildspec file. You must connect your Amazon Web Services account to your Bitbucket account. Use
* the CodeBuild console to start creating a build project. When you use the console to connect (or
* reconnect) with Bitbucket, on the Bitbucket Confirm access to your account page, choose Grant
* access. (After you have connected to your Bitbucket account, you do not need to finish creating the
* build project. You can leave the CodeBuild console.) To instruct CodeBuild to use this connection, in the
* source
object, set the auth
object's type
value to
* OAUTH
.
*
*
*
*
* If you specify CODEPIPELINE
for the Type
property, don't specify this property.
* For all of the other types, you must specify Location
.
*/
public final String location() {
return location;
}
/**
*
* Information about the Git clone depth for the build project.
*
*
* @return Information about the Git clone depth for the build project.
*/
public final Integer gitCloneDepth() {
return gitCloneDepth;
}
/**
*
* Information about the Git submodules configuration for the build project.
*
*
* @return Information about the Git submodules configuration for the build project.
*/
public final GitSubmodulesConfig gitSubmodulesConfig() {
return gitSubmodulesConfig;
}
/**
*
* The buildspec file declaration to use for the builds in this build project.
*
*
* If this value is set, it can be either an inline buildspec definition, the path to an alternate buildspec file
* relative to the value of the built-in CODEBUILD_SRC_DIR
environment variable, or the path to an S3
* bucket. The bucket must be in the same Amazon Web Services Region as the build project. Specify the buildspec
* file using its ARN (for example, arn:aws:s3:::my-codebuild-sample2/buildspec.yml
). If this value is
* not provided or is set to an empty string, the source code must contain a buildspec file in its root directory.
* For more information, see Buildspec File Name and Storage Location.
*
*
* @return The buildspec file declaration to use for the builds in this build project.
*
* If this value is set, it can be either an inline buildspec definition, the path to an alternate buildspec
* file relative to the value of the built-in CODEBUILD_SRC_DIR
environment variable, or the
* path to an S3 bucket. The bucket must be in the same Amazon Web Services Region as the build project.
* Specify the buildspec file using its ARN (for example,
* arn:aws:s3:::my-codebuild-sample2/buildspec.yml
). If this value is not provided or is set to
* an empty string, the source code must contain a buildspec file in its root directory. For more
* information, see Buildspec File Name and Storage Location.
*/
public final String buildspec() {
return buildspec;
}
/**
*
* Information about the authorization settings for CodeBuild to access the source code to be built.
*
*
* This information is for the CodeBuild console's use only. Your code should not get or set this information
* directly.
*
*
* @return Information about the authorization settings for CodeBuild to access the source code to be built.
*
* This information is for the CodeBuild console's use only. Your code should not get or set this
* information directly.
*/
public final SourceAuth auth() {
return auth;
}
/**
*
* Set to true to report the status of a build's start and finish to your source provider. This option is valid only
* when your source provider is GitHub, GitHub Enterprise, or Bitbucket. If this is set and you use a different
* source provider, an invalidInputException
is thrown.
*
*
* To be able to report the build status to the source provider, the user associated with the source provider must
* have write access to the repo. If the user does not have write access, the build status cannot be updated. For
* more information, see Source
* provider access in the CodeBuild User Guide.
*
*
* The status of a build triggered by a webhook is always reported to your source provider.
*
*
* If your project's builds are triggered by a webhook, you must push a new commit to the repo for a change to this
* property to take effect.
*
*
* @return Set to true to report the status of a build's start and finish to your source provider. This option is
* valid only when your source provider is GitHub, GitHub Enterprise, or Bitbucket. If this is set and you
* use a different source provider, an invalidInputException
is thrown.
*
* To be able to report the build status to the source provider, the user associated with the source
* provider must have write access to the repo. If the user does not have write access, the build status
* cannot be updated. For more information, see Source provider
* access in the CodeBuild User Guide.
*
*
* The status of a build triggered by a webhook is always reported to your source provider.
*
*
* If your project's builds are triggered by a webhook, you must push a new commit to the repo for a change
* to this property to take effect.
*/
public final Boolean reportBuildStatus() {
return reportBuildStatus;
}
/**
*
* Contains information that defines how the build project reports the build status to the source provider. This
* option is only used when the source provider is GITHUB
, GITHUB_ENTERPRISE
, or
* BITBUCKET
.
*
*
* @return Contains information that defines how the build project reports the build status to the source provider.
* This option is only used when the source provider is GITHUB
, GITHUB_ENTERPRISE
,
* or BITBUCKET
.
*/
public final BuildStatusConfig buildStatusConfig() {
return buildStatusConfig;
}
/**
*
* Enable this flag to ignore SSL warnings while connecting to the project source code.
*
*
* @return Enable this flag to ignore SSL warnings while connecting to the project source code.
*/
public final Boolean insecureSsl() {
return insecureSsl;
}
/**
*
* An identifier for this project source. The identifier can only contain alphanumeric characters and underscores,
* and must be less than 128 characters in length.
*
*
* @return An identifier for this project source. The identifier can only contain alphanumeric characters and
* underscores, and must be less than 128 characters in length.
*/
public final String sourceIdentifier() {
return sourceIdentifier;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(location());
hashCode = 31 * hashCode + Objects.hashCode(gitCloneDepth());
hashCode = 31 * hashCode + Objects.hashCode(gitSubmodulesConfig());
hashCode = 31 * hashCode + Objects.hashCode(buildspec());
hashCode = 31 * hashCode + Objects.hashCode(auth());
hashCode = 31 * hashCode + Objects.hashCode(reportBuildStatus());
hashCode = 31 * hashCode + Objects.hashCode(buildStatusConfig());
hashCode = 31 * hashCode + Objects.hashCode(insecureSsl());
hashCode = 31 * hashCode + Objects.hashCode(sourceIdentifier());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProjectSource)) {
return false;
}
ProjectSource other = (ProjectSource) obj;
return Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(location(), other.location())
&& Objects.equals(gitCloneDepth(), other.gitCloneDepth())
&& Objects.equals(gitSubmodulesConfig(), other.gitSubmodulesConfig())
&& Objects.equals(buildspec(), other.buildspec()) && Objects.equals(auth(), other.auth())
&& Objects.equals(reportBuildStatus(), other.reportBuildStatus())
&& Objects.equals(buildStatusConfig(), other.buildStatusConfig())
&& Objects.equals(insecureSsl(), other.insecureSsl())
&& Objects.equals(sourceIdentifier(), other.sourceIdentifier());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProjectSource").add("Type", typeAsString()).add("Location", location())
.add("GitCloneDepth", gitCloneDepth()).add("GitSubmodulesConfig", gitSubmodulesConfig())
.add("Buildspec", buildspec()).add("Auth", auth()).add("ReportBuildStatus", reportBuildStatus())
.add("BuildStatusConfig", buildStatusConfig()).add("InsecureSsl", insecureSsl())
.add("SourceIdentifier", sourceIdentifier()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "location":
return Optional.ofNullable(clazz.cast(location()));
case "gitCloneDepth":
return Optional.ofNullable(clazz.cast(gitCloneDepth()));
case "gitSubmodulesConfig":
return Optional.ofNullable(clazz.cast(gitSubmodulesConfig()));
case "buildspec":
return Optional.ofNullable(clazz.cast(buildspec()));
case "auth":
return Optional.ofNullable(clazz.cast(auth()));
case "reportBuildStatus":
return Optional.ofNullable(clazz.cast(reportBuildStatus()));
case "buildStatusConfig":
return Optional.ofNullable(clazz.cast(buildStatusConfig()));
case "insecureSsl":
return Optional.ofNullable(clazz.cast(insecureSsl()));
case "sourceIdentifier":
return Optional.ofNullable(clazz.cast(sourceIdentifier()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function