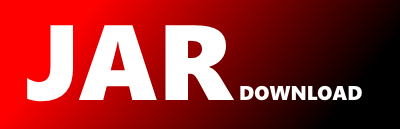
software.amazon.awssdk.services.codebuild.model.Build Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a build.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Build implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(Build::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(Build::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField BUILD_NUMBER_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("buildNumber").getter(getter(Build::buildNumber)).setter(setter(Builder::buildNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildNumber").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startTime").getter(getter(Build::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("endTime").getter(getter(Build::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build()).build();
private static final SdkField CURRENT_PHASE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("currentPhase").getter(getter(Build::currentPhase)).setter(setter(Builder::currentPhase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("currentPhase").build()).build();
private static final SdkField BUILD_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildStatus").getter(getter(Build::buildStatusAsString)).setter(setter(Builder::buildStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildStatus").build()).build();
private static final SdkField SOURCE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceVersion").getter(getter(Build::sourceVersion)).setter(setter(Builder::sourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceVersion").build()).build();
private static final SdkField RESOLVED_SOURCE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resolvedSourceVersion").getter(getter(Build::resolvedSourceVersion))
.setter(setter(Builder::resolvedSourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resolvedSourceVersion").build())
.build();
private static final SdkField PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("projectName").getter(getter(Build::projectName)).setter(setter(Builder::projectName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("projectName").build()).build();
private static final SdkField> PHASES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("phases")
.getter(getter(Build::phases))
.setter(setter(Builder::phases))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("phases").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BuildPhase::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("source").getter(getter(Build::source)).setter(setter(Builder::source))
.constructor(ProjectSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("source").build()).build();
private static final SdkField> SECONDARY_SOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondarySources")
.getter(getter(Build::secondarySources))
.setter(setter(Builder::secondarySources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySources").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SECONDARY_SOURCE_VERSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondarySourceVersions")
.getter(getter(Build::secondarySourceVersions))
.setter(setter(Builder::secondarySourceVersions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySourceVersions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSourceVersion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ARTIFACTS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("artifacts").getter(getter(Build::artifacts)).setter(setter(Builder::artifacts))
.constructor(BuildArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("artifacts").build()).build();
private static final SdkField> SECONDARY_ARTIFACTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondaryArtifacts")
.getter(getter(Build::secondaryArtifacts))
.setter(setter(Builder::secondaryArtifacts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondaryArtifacts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BuildArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CACHE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("cache").getter(getter(Build::cache)).setter(setter(Builder::cache)).constructor(ProjectCache::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cache").build()).build();
private static final SdkField ENVIRONMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("environment").getter(getter(Build::environment))
.setter(setter(Builder::environment)).constructor(ProjectEnvironment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environment").build()).build();
private static final SdkField SERVICE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceRole").getter(getter(Build::serviceRole)).setter(setter(Builder::serviceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRole").build()).build();
private static final SdkField LOGS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("logs").getter(getter(Build::logs)).setter(setter(Builder::logs)).constructor(LogsLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logs").build()).build();
private static final SdkField TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("timeoutInMinutes").getter(getter(Build::timeoutInMinutes)).setter(setter(Builder::timeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeoutInMinutes").build()).build();
private static final SdkField QUEUED_TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("queuedTimeoutInMinutes").getter(getter(Build::queuedTimeoutInMinutes))
.setter(setter(Builder::queuedTimeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("queuedTimeoutInMinutes").build())
.build();
private static final SdkField BUILD_COMPLETE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("buildComplete").getter(getter(Build::buildComplete)).setter(setter(Builder::buildComplete))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildComplete").build()).build();
private static final SdkField INITIATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("initiator").getter(getter(Build::initiator)).setter(setter(Builder::initiator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("initiator").build()).build();
private static final SdkField VPC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("vpcConfig").getter(getter(Build::vpcConfig)).setter(setter(Builder::vpcConfig))
.constructor(VpcConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpcConfig").build()).build();
private static final SdkField NETWORK_INTERFACE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkInterface")
.getter(getter(Build::networkInterface)).setter(setter(Builder::networkInterface))
.constructor(NetworkInterface::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkInterface").build()).build();
private static final SdkField ENCRYPTION_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("encryptionKey").getter(getter(Build::encryptionKey)).setter(setter(Builder::encryptionKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionKey").build()).build();
private static final SdkField> EXPORTED_ENVIRONMENT_VARIABLES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("exportedEnvironmentVariables")
.getter(getter(Build::exportedEnvironmentVariables))
.setter(setter(Builder::exportedEnvironmentVariables))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("exportedEnvironmentVariables")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExportedEnvironmentVariable::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> REPORT_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("reportArns")
.getter(getter(Build::reportArns))
.setter(setter(Builder::reportArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reportArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> FILE_SYSTEM_LOCATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("fileSystemLocations")
.getter(getter(Build::fileSystemLocations))
.setter(setter(Builder::fileSystemLocations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fileSystemLocations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectFileSystemLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DEBUG_SESSION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("debugSession").getter(getter(Build::debugSession)).setter(setter(Builder::debugSession))
.constructor(DebugSession::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("debugSession").build()).build();
private static final SdkField BUILD_BATCH_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildBatchArn").getter(getter(Build::buildBatchArn)).setter(setter(Builder::buildBatchArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildBatchArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ARN_FIELD,
BUILD_NUMBER_FIELD, START_TIME_FIELD, END_TIME_FIELD, CURRENT_PHASE_FIELD, BUILD_STATUS_FIELD, SOURCE_VERSION_FIELD,
RESOLVED_SOURCE_VERSION_FIELD, PROJECT_NAME_FIELD, PHASES_FIELD, SOURCE_FIELD, SECONDARY_SOURCES_FIELD,
SECONDARY_SOURCE_VERSIONS_FIELD, ARTIFACTS_FIELD, SECONDARY_ARTIFACTS_FIELD, CACHE_FIELD, ENVIRONMENT_FIELD,
SERVICE_ROLE_FIELD, LOGS_FIELD, TIMEOUT_IN_MINUTES_FIELD, QUEUED_TIMEOUT_IN_MINUTES_FIELD, BUILD_COMPLETE_FIELD,
INITIATOR_FIELD, VPC_CONFIG_FIELD, NETWORK_INTERFACE_FIELD, ENCRYPTION_KEY_FIELD,
EXPORTED_ENVIRONMENT_VARIABLES_FIELD, REPORT_ARNS_FIELD, FILE_SYSTEM_LOCATIONS_FIELD, DEBUG_SESSION_FIELD,
BUILD_BATCH_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String arn;
private final Long buildNumber;
private final Instant startTime;
private final Instant endTime;
private final String currentPhase;
private final String buildStatus;
private final String sourceVersion;
private final String resolvedSourceVersion;
private final String projectName;
private final List phases;
private final ProjectSource source;
private final List secondarySources;
private final List secondarySourceVersions;
private final BuildArtifacts artifacts;
private final List secondaryArtifacts;
private final ProjectCache cache;
private final ProjectEnvironment environment;
private final String serviceRole;
private final LogsLocation logs;
private final Integer timeoutInMinutes;
private final Integer queuedTimeoutInMinutes;
private final Boolean buildComplete;
private final String initiator;
private final VpcConfig vpcConfig;
private final NetworkInterface networkInterface;
private final String encryptionKey;
private final List exportedEnvironmentVariables;
private final List reportArns;
private final List fileSystemLocations;
private final DebugSession debugSession;
private final String buildBatchArn;
private Build(BuilderImpl builder) {
this.id = builder.id;
this.arn = builder.arn;
this.buildNumber = builder.buildNumber;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.currentPhase = builder.currentPhase;
this.buildStatus = builder.buildStatus;
this.sourceVersion = builder.sourceVersion;
this.resolvedSourceVersion = builder.resolvedSourceVersion;
this.projectName = builder.projectName;
this.phases = builder.phases;
this.source = builder.source;
this.secondarySources = builder.secondarySources;
this.secondarySourceVersions = builder.secondarySourceVersions;
this.artifacts = builder.artifacts;
this.secondaryArtifacts = builder.secondaryArtifacts;
this.cache = builder.cache;
this.environment = builder.environment;
this.serviceRole = builder.serviceRole;
this.logs = builder.logs;
this.timeoutInMinutes = builder.timeoutInMinutes;
this.queuedTimeoutInMinutes = builder.queuedTimeoutInMinutes;
this.buildComplete = builder.buildComplete;
this.initiator = builder.initiator;
this.vpcConfig = builder.vpcConfig;
this.networkInterface = builder.networkInterface;
this.encryptionKey = builder.encryptionKey;
this.exportedEnvironmentVariables = builder.exportedEnvironmentVariables;
this.reportArns = builder.reportArns;
this.fileSystemLocations = builder.fileSystemLocations;
this.debugSession = builder.debugSession;
this.buildBatchArn = builder.buildBatchArn;
}
/**
*
* The unique ID for the build.
*
*
* @return The unique ID for the build.
*/
public final String id() {
return id;
}
/**
*
* The Amazon Resource Name (ARN) of the build.
*
*
* @return The Amazon Resource Name (ARN) of the build.
*/
public final String arn() {
return arn;
}
/**
*
* The number of the build. For each project, the buildNumber
of its first build is 1
. The
* buildNumber
of each subsequent build is incremented by 1
. If a build is deleted, the
* buildNumber
of other builds does not change.
*
*
* @return The number of the build. For each project, the buildNumber
of its first build is
* 1
. The buildNumber
of each subsequent build is incremented by 1
.
* If a build is deleted, the buildNumber
of other builds does not change.
*/
public final Long buildNumber() {
return buildNumber;
}
/**
*
* When the build process started, expressed in Unix time format.
*
*
* @return When the build process started, expressed in Unix time format.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* When the build process ended, expressed in Unix time format.
*
*
* @return When the build process ended, expressed in Unix time format.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* The current build phase.
*
*
* @return The current build phase.
*/
public final String currentPhase() {
return currentPhase;
}
/**
*
* The current status of the build. Valid values include:
*
*
* -
*
* FAILED
: The build failed.
*
*
* -
*
* FAULT
: The build faulted.
*
*
* -
*
* IN_PROGRESS
: The build is still in progress.
*
*
* -
*
* STOPPED
: The build stopped.
*
*
* -
*
* SUCCEEDED
: The build succeeded.
*
*
* -
*
* TIMED_OUT
: The build timed out.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildStatus} will
* return {@link StatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildStatusAsString}.
*
*
* @return The current status of the build. Valid values include:
*
* -
*
* FAILED
: The build failed.
*
*
* -
*
* FAULT
: The build faulted.
*
*
* -
*
* IN_PROGRESS
: The build is still in progress.
*
*
* -
*
* STOPPED
: The build stopped.
*
*
* -
*
* SUCCEEDED
: The build succeeded.
*
*
* -
*
* TIMED_OUT
: The build timed out.
*
*
* @see StatusType
*/
public final StatusType buildStatus() {
return StatusType.fromValue(buildStatus);
}
/**
*
* The current status of the build. Valid values include:
*
*
* -
*
* FAILED
: The build failed.
*
*
* -
*
* FAULT
: The build faulted.
*
*
* -
*
* IN_PROGRESS
: The build is still in progress.
*
*
* -
*
* STOPPED
: The build stopped.
*
*
* -
*
* SUCCEEDED
: The build succeeded.
*
*
* -
*
* TIMED_OUT
: The build timed out.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildStatus} will
* return {@link StatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildStatusAsString}.
*
*
* @return The current status of the build. Valid values include:
*
* -
*
* FAILED
: The build failed.
*
*
* -
*
* FAULT
: The build faulted.
*
*
* -
*
* IN_PROGRESS
: The build is still in progress.
*
*
* -
*
* STOPPED
: The build stopped.
*
*
* -
*
* SUCCEEDED
: The build succeeded.
*
*
* -
*
* TIMED_OUT
: The build timed out.
*
*
* @see StatusType
*/
public final String buildStatusAsString() {
return buildStatus;
}
/**
*
* Any version identifier for the version of the source code to be built. If sourceVersion
is specified
* at the project level, then this sourceVersion
(at the build level) takes precedence.
*
*
* For more information, see Source Version Sample
* with CodeBuild in the CodeBuild User Guide.
*
*
* @return Any version identifier for the version of the source code to be built. If sourceVersion
is
* specified at the project level, then this sourceVersion
(at the build level) takes
* precedence.
*
* For more information, see Source Version
* Sample with CodeBuild in the CodeBuild User Guide.
*/
public final String sourceVersion() {
return sourceVersion;
}
/**
*
* An identifier for the version of this build's source code.
*
*
* -
*
* For CodeCommit, GitHub, GitHub Enterprise, and BitBucket, the commit ID.
*
*
* -
*
* For CodePipeline, the source revision provided by CodePipeline.
*
*
* -
*
* For Amazon S3, this does not apply.
*
*
*
*
* @return An identifier for the version of this build's source code.
*
* -
*
* For CodeCommit, GitHub, GitHub Enterprise, and BitBucket, the commit ID.
*
*
* -
*
* For CodePipeline, the source revision provided by CodePipeline.
*
*
* -
*
* For Amazon S3, this does not apply.
*
*
*/
public final String resolvedSourceVersion() {
return resolvedSourceVersion;
}
/**
*
* The name of the CodeBuild project.
*
*
* @return The name of the CodeBuild project.
*/
public final String projectName() {
return projectName;
}
/**
* For responses, this returns true if the service returned a value for the Phases property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasPhases() {
return phases != null && !(phases instanceof SdkAutoConstructList);
}
/**
*
* Information about all previous build phases that are complete and information about any current build phase that
* is not yet complete.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPhases} method.
*
*
* @return Information about all previous build phases that are complete and information about any current build
* phase that is not yet complete.
*/
public final List phases() {
return phases;
}
/**
*
* Information about the source code to be built.
*
*
* @return Information about the source code to be built.
*/
public final ProjectSource source() {
return source;
}
/**
* For responses, this returns true if the service returned a value for the SecondarySources property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecondarySources() {
return secondarySources != null && !(secondarySources instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSource
objects.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondarySources} method.
*
*
* @return An array of ProjectSource
objects.
*/
public final List secondarySources() {
return secondarySources;
}
/**
* For responses, this returns true if the service returned a value for the SecondarySourceVersions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasSecondarySourceVersions() {
return secondarySourceVersions != null && !(secondarySourceVersions instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be one of:
*
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the branch's
* HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default
* branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondarySourceVersions} method.
*
*
* @return An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be one
* of:
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of
* the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code
* you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified,
* the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*/
public final List secondarySourceVersions() {
return secondarySourceVersions;
}
/**
*
* Information about the output artifacts for the build.
*
*
* @return Information about the output artifacts for the build.
*/
public final BuildArtifacts artifacts() {
return artifacts;
}
/**
* For responses, this returns true if the service returned a value for the SecondaryArtifacts property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecondaryArtifacts() {
return secondaryArtifacts != null && !(secondaryArtifacts instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectArtifacts
objects.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondaryArtifacts} method.
*
*
* @return An array of ProjectArtifacts
objects.
*/
public final List secondaryArtifacts() {
return secondaryArtifacts;
}
/**
*
* Information about the cache for the build.
*
*
* @return Information about the cache for the build.
*/
public final ProjectCache cache() {
return cache;
}
/**
*
* Information about the build environment for this build.
*
*
* @return Information about the build environment for this build.
*/
public final ProjectEnvironment environment() {
return environment;
}
/**
*
* The name of a service role used for this build.
*
*
* @return The name of a service role used for this build.
*/
public final String serviceRole() {
return serviceRole;
}
/**
*
* Information about the build's logs in CloudWatch Logs.
*
*
* @return Information about the build's logs in CloudWatch Logs.
*/
public final LogsLocation logs() {
return logs;
}
/**
*
* How long, in minutes, for CodeBuild to wait before timing out this build if it does not get marked as completed.
*
*
* @return How long, in minutes, for CodeBuild to wait before timing out this build if it does not get marked as
* completed.
*/
public final Integer timeoutInMinutes() {
return timeoutInMinutes;
}
/**
*
* The number of minutes a build is allowed to be queued before it times out.
*
*
* @return The number of minutes a build is allowed to be queued before it times out.
*/
public final Integer queuedTimeoutInMinutes() {
return queuedTimeoutInMinutes;
}
/**
*
* Whether the build is complete. True if complete; otherwise, false.
*
*
* @return Whether the build is complete. True if complete; otherwise, false.
*/
public final Boolean buildComplete() {
return buildComplete;
}
/**
*
* The entity that started the build. Valid values include:
*
*
* -
*
* If CodePipeline started the build, the pipeline's name (for example, codepipeline/my-demo-pipeline
).
*
*
* -
*
* If an IAM user started the build, the user's name (for example, MyUserName
).
*
*
* -
*
* If the Jenkins plugin for CodeBuild started the build, the string CodeBuild-Jenkins-Plugin
.
*
*
*
*
* @return The entity that started the build. Valid values include:
*
* -
*
* If CodePipeline started the build, the pipeline's name (for example,
* codepipeline/my-demo-pipeline
).
*
*
* -
*
* If an IAM user started the build, the user's name (for example, MyUserName
).
*
*
* -
*
* If the Jenkins plugin for CodeBuild started the build, the string CodeBuild-Jenkins-Plugin
.
*
*
*/
public final String initiator() {
return initiator;
}
/**
*
* If your CodeBuild project accesses resources in an Amazon VPC, you provide this parameter that identifies the VPC
* ID and the list of security group IDs and subnet IDs. The security groups and subnets must belong to the same
* VPC. You must provide at least one security group and one subnet ID.
*
*
* @return If your CodeBuild project accesses resources in an Amazon VPC, you provide this parameter that identifies
* the VPC ID and the list of security group IDs and subnet IDs. The security groups and subnets must belong
* to the same VPC. You must provide at least one security group and one subnet ID.
*/
public final VpcConfig vpcConfig() {
return vpcConfig;
}
/**
*
* Describes a network interface.
*
*
* @return Describes a network interface.
*/
public final NetworkInterface networkInterface() {
return networkInterface;
}
/**
*
* The Key Management Service customer master key (CMK) to be used for encrypting the build output artifacts.
*
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission to
* that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the
* format alias/<alias-name>
).
*
*
* @return The Key Management Service customer master key (CMK) to be used for encrypting the build output
* artifacts.
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has
* permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using
* the format alias/<alias-name>
).
*/
public final String encryptionKey() {
return encryptionKey;
}
/**
* For responses, this returns true if the service returned a value for the ExportedEnvironmentVariables property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasExportedEnvironmentVariables() {
return exportedEnvironmentVariables != null && !(exportedEnvironmentVariables instanceof SdkAutoConstructList);
}
/**
*
* A list of exported environment variables for this build.
*
*
* Exported environment variables are used in conjunction with CodePipeline to export environment variables from the
* current build stage to subsequent stages in the pipeline. For more information, see Working with
* variables in the CodePipeline User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExportedEnvironmentVariables} method.
*
*
* @return A list of exported environment variables for this build.
*
* Exported environment variables are used in conjunction with CodePipeline to export environment variables
* from the current build stage to subsequent stages in the pipeline. For more information, see Working with
* variables in the CodePipeline User Guide.
*/
public final List exportedEnvironmentVariables() {
return exportedEnvironmentVariables;
}
/**
* For responses, this returns true if the service returned a value for the ReportArns property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasReportArns() {
return reportArns != null && !(reportArns instanceof SdkAutoConstructList);
}
/**
*
* An array of the ARNs associated with this build's reports.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReportArns} method.
*
*
* @return An array of the ARNs associated with this build's reports.
*/
public final List reportArns() {
return reportArns;
}
/**
* For responses, this returns true if the service returned a value for the FileSystemLocations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasFileSystemLocations() {
return fileSystemLocations != null && !(fileSystemLocations instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using Amazon
* Elastic File System.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFileSystemLocations} method.
*
*
* @return An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
,
* location
, mountOptions
, mountPoint
, and type
of a
* file system created using Amazon Elastic File System.
*/
public final List fileSystemLocations() {
return fileSystemLocations;
}
/**
*
* Contains information about the debug session for this build.
*
*
* @return Contains information about the debug session for this build.
*/
public final DebugSession debugSession() {
return debugSession;
}
/**
*
* The ARN of the batch build that this build is a member of, if applicable.
*
*
* @return The ARN of the batch build that this build is a member of, if applicable.
*/
public final String buildBatchArn() {
return buildBatchArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(buildNumber());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(currentPhase());
hashCode = 31 * hashCode + Objects.hashCode(buildStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(sourceVersion());
hashCode = 31 * hashCode + Objects.hashCode(resolvedSourceVersion());
hashCode = 31 * hashCode + Objects.hashCode(projectName());
hashCode = 31 * hashCode + Objects.hashCode(hasPhases() ? phases() : null);
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(hasSecondarySources() ? secondarySources() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSecondarySourceVersions() ? secondarySourceVersions() : null);
hashCode = 31 * hashCode + Objects.hashCode(artifacts());
hashCode = 31 * hashCode + Objects.hashCode(hasSecondaryArtifacts() ? secondaryArtifacts() : null);
hashCode = 31 * hashCode + Objects.hashCode(cache());
hashCode = 31 * hashCode + Objects.hashCode(environment());
hashCode = 31 * hashCode + Objects.hashCode(serviceRole());
hashCode = 31 * hashCode + Objects.hashCode(logs());
hashCode = 31 * hashCode + Objects.hashCode(timeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(queuedTimeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(buildComplete());
hashCode = 31 * hashCode + Objects.hashCode(initiator());
hashCode = 31 * hashCode + Objects.hashCode(vpcConfig());
hashCode = 31 * hashCode + Objects.hashCode(networkInterface());
hashCode = 31 * hashCode + Objects.hashCode(encryptionKey());
hashCode = 31 * hashCode + Objects.hashCode(hasExportedEnvironmentVariables() ? exportedEnvironmentVariables() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasReportArns() ? reportArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasFileSystemLocations() ? fileSystemLocations() : null);
hashCode = 31 * hashCode + Objects.hashCode(debugSession());
hashCode = 31 * hashCode + Objects.hashCode(buildBatchArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Build)) {
return false;
}
Build other = (Build) obj;
return Objects.equals(id(), other.id()) && Objects.equals(arn(), other.arn())
&& Objects.equals(buildNumber(), other.buildNumber()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(currentPhase(), other.currentPhase())
&& Objects.equals(buildStatusAsString(), other.buildStatusAsString())
&& Objects.equals(sourceVersion(), other.sourceVersion())
&& Objects.equals(resolvedSourceVersion(), other.resolvedSourceVersion())
&& Objects.equals(projectName(), other.projectName()) && hasPhases() == other.hasPhases()
&& Objects.equals(phases(), other.phases()) && Objects.equals(source(), other.source())
&& hasSecondarySources() == other.hasSecondarySources()
&& Objects.equals(secondarySources(), other.secondarySources())
&& hasSecondarySourceVersions() == other.hasSecondarySourceVersions()
&& Objects.equals(secondarySourceVersions(), other.secondarySourceVersions())
&& Objects.equals(artifacts(), other.artifacts()) && hasSecondaryArtifacts() == other.hasSecondaryArtifacts()
&& Objects.equals(secondaryArtifacts(), other.secondaryArtifacts()) && Objects.equals(cache(), other.cache())
&& Objects.equals(environment(), other.environment()) && Objects.equals(serviceRole(), other.serviceRole())
&& Objects.equals(logs(), other.logs()) && Objects.equals(timeoutInMinutes(), other.timeoutInMinutes())
&& Objects.equals(queuedTimeoutInMinutes(), other.queuedTimeoutInMinutes())
&& Objects.equals(buildComplete(), other.buildComplete()) && Objects.equals(initiator(), other.initiator())
&& Objects.equals(vpcConfig(), other.vpcConfig()) && Objects.equals(networkInterface(), other.networkInterface())
&& Objects.equals(encryptionKey(), other.encryptionKey())
&& hasExportedEnvironmentVariables() == other.hasExportedEnvironmentVariables()
&& Objects.equals(exportedEnvironmentVariables(), other.exportedEnvironmentVariables())
&& hasReportArns() == other.hasReportArns() && Objects.equals(reportArns(), other.reportArns())
&& hasFileSystemLocations() == other.hasFileSystemLocations()
&& Objects.equals(fileSystemLocations(), other.fileSystemLocations())
&& Objects.equals(debugSession(), other.debugSession()) && Objects.equals(buildBatchArn(), other.buildBatchArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Build").add("Id", id()).add("Arn", arn()).add("BuildNumber", buildNumber())
.add("StartTime", startTime()).add("EndTime", endTime()).add("CurrentPhase", currentPhase())
.add("BuildStatus", buildStatusAsString()).add("SourceVersion", sourceVersion())
.add("ResolvedSourceVersion", resolvedSourceVersion()).add("ProjectName", projectName())
.add("Phases", hasPhases() ? phases() : null).add("Source", source())
.add("SecondarySources", hasSecondarySources() ? secondarySources() : null)
.add("SecondarySourceVersions", hasSecondarySourceVersions() ? secondarySourceVersions() : null)
.add("Artifacts", artifacts()).add("SecondaryArtifacts", hasSecondaryArtifacts() ? secondaryArtifacts() : null)
.add("Cache", cache()).add("Environment", environment()).add("ServiceRole", serviceRole()).add("Logs", logs())
.add("TimeoutInMinutes", timeoutInMinutes()).add("QueuedTimeoutInMinutes", queuedTimeoutInMinutes())
.add("BuildComplete", buildComplete()).add("Initiator", initiator()).add("VpcConfig", vpcConfig())
.add("NetworkInterface", networkInterface()).add("EncryptionKey", encryptionKey())
.add("ExportedEnvironmentVariables", hasExportedEnvironmentVariables() ? exportedEnvironmentVariables() : null)
.add("ReportArns", hasReportArns() ? reportArns() : null)
.add("FileSystemLocations", hasFileSystemLocations() ? fileSystemLocations() : null)
.add("DebugSession", debugSession()).add("BuildBatchArn", buildBatchArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "buildNumber":
return Optional.ofNullable(clazz.cast(buildNumber()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "endTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "currentPhase":
return Optional.ofNullable(clazz.cast(currentPhase()));
case "buildStatus":
return Optional.ofNullable(clazz.cast(buildStatusAsString()));
case "sourceVersion":
return Optional.ofNullable(clazz.cast(sourceVersion()));
case "resolvedSourceVersion":
return Optional.ofNullable(clazz.cast(resolvedSourceVersion()));
case "projectName":
return Optional.ofNullable(clazz.cast(projectName()));
case "phases":
return Optional.ofNullable(clazz.cast(phases()));
case "source":
return Optional.ofNullable(clazz.cast(source()));
case "secondarySources":
return Optional.ofNullable(clazz.cast(secondarySources()));
case "secondarySourceVersions":
return Optional.ofNullable(clazz.cast(secondarySourceVersions()));
case "artifacts":
return Optional.ofNullable(clazz.cast(artifacts()));
case "secondaryArtifacts":
return Optional.ofNullable(clazz.cast(secondaryArtifacts()));
case "cache":
return Optional.ofNullable(clazz.cast(cache()));
case "environment":
return Optional.ofNullable(clazz.cast(environment()));
case "serviceRole":
return Optional.ofNullable(clazz.cast(serviceRole()));
case "logs":
return Optional.ofNullable(clazz.cast(logs()));
case "timeoutInMinutes":
return Optional.ofNullable(clazz.cast(timeoutInMinutes()));
case "queuedTimeoutInMinutes":
return Optional.ofNullable(clazz.cast(queuedTimeoutInMinutes()));
case "buildComplete":
return Optional.ofNullable(clazz.cast(buildComplete()));
case "initiator":
return Optional.ofNullable(clazz.cast(initiator()));
case "vpcConfig":
return Optional.ofNullable(clazz.cast(vpcConfig()));
case "networkInterface":
return Optional.ofNullable(clazz.cast(networkInterface()));
case "encryptionKey":
return Optional.ofNullable(clazz.cast(encryptionKey()));
case "exportedEnvironmentVariables":
return Optional.ofNullable(clazz.cast(exportedEnvironmentVariables()));
case "reportArns":
return Optional.ofNullable(clazz.cast(reportArns()));
case "fileSystemLocations":
return Optional.ofNullable(clazz.cast(fileSystemLocations()));
case "debugSession":
return Optional.ofNullable(clazz.cast(debugSession()));
case "buildBatchArn":
return Optional.ofNullable(clazz.cast(buildBatchArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* FAILED
: The build failed.
*
*
* -
*
* FAULT
: The build faulted.
*
*
* -
*
* IN_PROGRESS
: The build is still in progress.
*
*
* -
*
* STOPPED
: The build stopped.
*
*
* -
*
* SUCCEEDED
: The build succeeded.
*
*
* -
*
* TIMED_OUT
: The build timed out.
*
*
* @see StatusType
* @return Returns a reference to this object so that method calls can be chained together.
* @see StatusType
*/
Builder buildStatus(String buildStatus);
/**
*
* The current status of the build. Valid values include:
*
*
* -
*
* FAILED
: The build failed.
*
*
* -
*
* FAULT
: The build faulted.
*
*
* -
*
* IN_PROGRESS
: The build is still in progress.
*
*
* -
*
* STOPPED
: The build stopped.
*
*
* -
*
* SUCCEEDED
: The build succeeded.
*
*
* -
*
* TIMED_OUT
: The build timed out.
*
*
*
*
* @param buildStatus
* The current status of the build. Valid values include:
*
* -
*
* FAILED
: The build failed.
*
*
* -
*
* FAULT
: The build faulted.
*
*
* -
*
* IN_PROGRESS
: The build is still in progress.
*
*
* -
*
* STOPPED
: The build stopped.
*
*
* -
*
* SUCCEEDED
: The build succeeded.
*
*
* -
*
* TIMED_OUT
: The build timed out.
*
*
* @see StatusType
* @return Returns a reference to this object so that method calls can be chained together.
* @see StatusType
*/
Builder buildStatus(StatusType buildStatus);
/**
*
* Any version identifier for the version of the source code to be built. If sourceVersion
is
* specified at the project level, then this sourceVersion
(at the build level) takes precedence.
*
*
* For more information, see Source Version
* Sample with CodeBuild in the CodeBuild User Guide.
*
*
* @param sourceVersion
* Any version identifier for the version of the source code to be built. If sourceVersion
* is specified at the project level, then this sourceVersion
(at the build level) takes
* precedence.
*
* For more information, see Source
* Version Sample with CodeBuild in the CodeBuild User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceVersion(String sourceVersion);
/**
*
* An identifier for the version of this build's source code.
*
*
* -
*
* For CodeCommit, GitHub, GitHub Enterprise, and BitBucket, the commit ID.
*
*
* -
*
* For CodePipeline, the source revision provided by CodePipeline.
*
*
* -
*
* For Amazon S3, this does not apply.
*
*
*
*
* @param resolvedSourceVersion
* An identifier for the version of this build's source code.
*
* -
*
* For CodeCommit, GitHub, GitHub Enterprise, and BitBucket, the commit ID.
*
*
* -
*
* For CodePipeline, the source revision provided by CodePipeline.
*
*
* -
*
* For Amazon S3, this does not apply.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resolvedSourceVersion(String resolvedSourceVersion);
/**
*
* The name of the CodeBuild project.
*
*
* @param projectName
* The name of the CodeBuild project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder projectName(String projectName);
/**
*
* Information about all previous build phases that are complete and information about any current build phase
* that is not yet complete.
*
*
* @param phases
* Information about all previous build phases that are complete and information about any current build
* phase that is not yet complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder phases(Collection phases);
/**
*
* Information about all previous build phases that are complete and information about any current build phase
* that is not yet complete.
*
*
* @param phases
* Information about all previous build phases that are complete and information about any current build
* phase that is not yet complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder phases(BuildPhase... phases);
/**
*
* Information about all previous build phases that are complete and information about any current build phase
* that is not yet complete.
*
* This is a convenience method that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its
* result is passed to {@link #phases(List)}.
*
* @param phases
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #phases(List)
*/
Builder phases(Consumer... phases);
/**
*
* Information about the source code to be built.
*
*
* @param source
* Information about the source code to be built.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder source(ProjectSource source);
/**
*
* Information about the source code to be built.
*
* This is a convenience method that creates an instance of the {@link ProjectSource.Builder} avoiding the need
* to create one manually via {@link ProjectSource#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectSource.Builder#build()} is called immediately and its
* result is passed to {@link #source(ProjectSource)}.
*
* @param source
* a consumer that will call methods on {@link ProjectSource.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #source(ProjectSource)
*/
default Builder source(Consumer source) {
return source(ProjectSource.builder().applyMutation(source).build());
}
/**
*
* An array of ProjectSource
objects.
*
*
* @param secondarySources
* An array of ProjectSource
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySources(Collection secondarySources);
/**
*
* An array of ProjectSource
objects.
*
*
* @param secondarySources
* An array of ProjectSource
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySources(ProjectSource... secondarySources);
/**
*
* An array of ProjectSource
objects.
*
* This is a convenience method that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and
* its result is passed to {@link #secondarySources(List)}.
*
* @param secondarySources
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #secondarySources(List)
*/
Builder secondarySources(Consumer... secondarySources);
/**
*
* An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be one of:
*
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the
* default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* @param secondarySourceVersions
* An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be
* one of:
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version
* of the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source
* code you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not
* specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySourceVersions(Collection secondarySourceVersions);
/**
*
* An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be one of:
*
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the
* default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* @param secondarySourceVersions
* An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be
* one of:
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version
* of the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source
* code you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not
* specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondarySourceVersions(ProjectSourceVersion... secondarySourceVersions);
/**
*
* An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be one of:
*
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the
* default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
* This is a convenience method that creates an instance of the {@link List.Builder}
* avoiding the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately
* and its result is passed to {@link #secondarySourceVersions(List)}.
*
* @param secondarySourceVersions
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #secondarySourceVersions(List)
*/
Builder secondarySourceVersions(Consumer... secondarySourceVersions);
/**
*
* Information about the output artifacts for the build.
*
*
* @param artifacts
* Information about the output artifacts for the build.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder artifacts(BuildArtifacts artifacts);
/**
*
* Information about the output artifacts for the build.
*
* This is a convenience method that creates an instance of the {@link BuildArtifacts.Builder} avoiding the need
* to create one manually via {@link BuildArtifacts#builder()}.
*
* When the {@link Consumer} completes, {@link BuildArtifacts.Builder#build()} is called immediately and its
* result is passed to {@link #artifacts(BuildArtifacts)}.
*
* @param artifacts
* a consumer that will call methods on {@link BuildArtifacts.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #artifacts(BuildArtifacts)
*/
default Builder artifacts(Consumer artifacts) {
return artifacts(BuildArtifacts.builder().applyMutation(artifacts).build());
}
/**
*
* An array of ProjectArtifacts
objects.
*
*
* @param secondaryArtifacts
* An array of ProjectArtifacts
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondaryArtifacts(Collection secondaryArtifacts);
/**
*
* An array of ProjectArtifacts
objects.
*
*
* @param secondaryArtifacts
* An array of ProjectArtifacts
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder secondaryArtifacts(BuildArtifacts... secondaryArtifacts);
/**
*
* An array of ProjectArtifacts
objects.
*
* This is a convenience method that creates an instance of the {@link List.Builder} avoiding
* the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and
* its result is passed to {@link #secondaryArtifacts(List)}.
*
* @param secondaryArtifacts
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #secondaryArtifacts(List)
*/
Builder secondaryArtifacts(Consumer... secondaryArtifacts);
/**
*
* Information about the cache for the build.
*
*
* @param cache
* Information about the cache for the build.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cache(ProjectCache cache);
/**
*
* Information about the cache for the build.
*
* This is a convenience method that creates an instance of the {@link ProjectCache.Builder} avoiding the need
* to create one manually via {@link ProjectCache#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectCache.Builder#build()} is called immediately and its
* result is passed to {@link #cache(ProjectCache)}.
*
* @param cache
* a consumer that will call methods on {@link ProjectCache.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #cache(ProjectCache)
*/
default Builder cache(Consumer cache) {
return cache(ProjectCache.builder().applyMutation(cache).build());
}
/**
*
* Information about the build environment for this build.
*
*
* @param environment
* Information about the build environment for this build.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder environment(ProjectEnvironment environment);
/**
*
* Information about the build environment for this build.
*
* This is a convenience method that creates an instance of the {@link ProjectEnvironment.Builder} avoiding the
* need to create one manually via {@link ProjectEnvironment#builder()}.
*
* When the {@link Consumer} completes, {@link ProjectEnvironment.Builder#build()} is called immediately and its
* result is passed to {@link #environment(ProjectEnvironment)}.
*
* @param environment
* a consumer that will call methods on {@link ProjectEnvironment.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #environment(ProjectEnvironment)
*/
default Builder environment(Consumer environment) {
return environment(ProjectEnvironment.builder().applyMutation(environment).build());
}
/**
*
* The name of a service role used for this build.
*
*
* @param serviceRole
* The name of a service role used for this build.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder serviceRole(String serviceRole);
/**
*
* Information about the build's logs in CloudWatch Logs.
*
*
* @param logs
* Information about the build's logs in CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder logs(LogsLocation logs);
/**
*
* Information about the build's logs in CloudWatch Logs.
*
* This is a convenience method that creates an instance of the {@link LogsLocation.Builder} avoiding the need
* to create one manually via {@link LogsLocation#builder()}.
*
* When the {@link Consumer} completes, {@link LogsLocation.Builder#build()} is called immediately and its
* result is passed to {@link #logs(LogsLocation)}.
*
* @param logs
* a consumer that will call methods on {@link LogsLocation.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #logs(LogsLocation)
*/
default Builder logs(Consumer logs) {
return logs(LogsLocation.builder().applyMutation(logs).build());
}
/**
*
* How long, in minutes, for CodeBuild to wait before timing out this build if it does not get marked as
* completed.
*
*
* @param timeoutInMinutes
* How long, in minutes, for CodeBuild to wait before timing out this build if it does not get marked as
* completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timeoutInMinutes(Integer timeoutInMinutes);
/**
*
* The number of minutes a build is allowed to be queued before it times out.
*
*
* @param queuedTimeoutInMinutes
* The number of minutes a build is allowed to be queued before it times out.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queuedTimeoutInMinutes(Integer queuedTimeoutInMinutes);
/**
*
* Whether the build is complete. True if complete; otherwise, false.
*
*
* @param buildComplete
* Whether the build is complete. True if complete; otherwise, false.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder buildComplete(Boolean buildComplete);
/**
*
* The entity that started the build. Valid values include:
*
*
* -
*
* If CodePipeline started the build, the pipeline's name (for example,
* codepipeline/my-demo-pipeline
).
*
*
* -
*
* If an IAM user started the build, the user's name (for example, MyUserName
).
*
*
* -
*
* If the Jenkins plugin for CodeBuild started the build, the string CodeBuild-Jenkins-Plugin
.
*
*
*
*
* @param initiator
* The entity that started the build. Valid values include:
*
* -
*
* If CodePipeline started the build, the pipeline's name (for example,
* codepipeline/my-demo-pipeline
).
*
*
* -
*
* If an IAM user started the build, the user's name (for example, MyUserName
).
*
*
* -
*
* If the Jenkins plugin for CodeBuild started the build, the string
* CodeBuild-Jenkins-Plugin
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder initiator(String initiator);
/**
*
* If your CodeBuild project accesses resources in an Amazon VPC, you provide this parameter that identifies the
* VPC ID and the list of security group IDs and subnet IDs. The security groups and subnets must belong to the
* same VPC. You must provide at least one security group and one subnet ID.
*
*
* @param vpcConfig
* If your CodeBuild project accesses resources in an Amazon VPC, you provide this parameter that
* identifies the VPC ID and the list of security group IDs and subnet IDs. The security groups and
* subnets must belong to the same VPC. You must provide at least one security group and one subnet ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vpcConfig(VpcConfig vpcConfig);
/**
*
* If your CodeBuild project accesses resources in an Amazon VPC, you provide this parameter that identifies the
* VPC ID and the list of security group IDs and subnet IDs. The security groups and subnets must belong to the
* same VPC. You must provide at least one security group and one subnet ID.
*
* This is a convenience method that creates an instance of the {@link VpcConfig.Builder} avoiding the need to
* create one manually via {@link VpcConfig#builder()}.
*
* When the {@link Consumer} completes, {@link VpcConfig.Builder#build()} is called immediately and its result
* is passed to {@link #vpcConfig(VpcConfig)}.
*
* @param vpcConfig
* a consumer that will call methods on {@link VpcConfig.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #vpcConfig(VpcConfig)
*/
default Builder vpcConfig(Consumer vpcConfig) {
return vpcConfig(VpcConfig.builder().applyMutation(vpcConfig).build());
}
/**
*
* Describes a network interface.
*
*
* @param networkInterface
* Describes a network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder networkInterface(NetworkInterface networkInterface);
/**
*
* Describes a network interface.
*
* This is a convenience method that creates an instance of the {@link NetworkInterface.Builder} avoiding the
* need to create one manually via {@link NetworkInterface#builder()}.
*
* When the {@link Consumer} completes, {@link NetworkInterface.Builder#build()} is called immediately and its
* result is passed to {@link #networkInterface(NetworkInterface)}.
*
* @param networkInterface
* a consumer that will call methods on {@link NetworkInterface.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #networkInterface(NetworkInterface)
*/
default Builder networkInterface(Consumer networkInterface) {
return networkInterface(NetworkInterface.builder().applyMutation(networkInterface).build());
}
/**
*
* The Key Management Service customer master key (CMK) to be used for encrypting the build output artifacts.
*
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission
* to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the
* format alias/<alias-name>
).
*
*
* @param encryptionKey
* The Key Management Service customer master key (CMK) to be used for encrypting the build output
* artifacts.
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has
* permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias
* (using the format alias/<alias-name>
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder encryptionKey(String encryptionKey);
/**
*
* A list of exported environment variables for this build.
*
*
* Exported environment variables are used in conjunction with CodePipeline to export environment variables from
* the current build stage to subsequent stages in the pipeline. For more information, see Working with
* variables in the CodePipeline User Guide.
*
*
* @param exportedEnvironmentVariables
* A list of exported environment variables for this build.
*
* Exported environment variables are used in conjunction with CodePipeline to export environment
* variables from the current build stage to subsequent stages in the pipeline. For more information, see
* Working
* with variables in the CodePipeline User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder exportedEnvironmentVariables(Collection exportedEnvironmentVariables);
/**
*
* A list of exported environment variables for this build.
*
*
* Exported environment variables are used in conjunction with CodePipeline to export environment variables from
* the current build stage to subsequent stages in the pipeline. For more information, see Working with
* variables in the CodePipeline User Guide.
*
*
* @param exportedEnvironmentVariables
* A list of exported environment variables for this build.
*
* Exported environment variables are used in conjunction with CodePipeline to export environment
* variables from the current build stage to subsequent stages in the pipeline. For more information, see
* Working
* with variables in the CodePipeline User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder exportedEnvironmentVariables(ExportedEnvironmentVariable... exportedEnvironmentVariables);
/**
*
* A list of exported environment variables for this build.
*
*
* Exported environment variables are used in conjunction with CodePipeline to export environment variables from
* the current build stage to subsequent stages in the pipeline. For more information, see Working with
* variables in the CodePipeline User Guide.
*
* This is a convenience method that creates an instance of the {@link List
* .Builder} avoiding the need to create one manually via {@link List
* #builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called
* immediately and its result is passed to {@link
* #exportedEnvironmentVariables(List)}.
*
* @param exportedEnvironmentVariables
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #exportedEnvironmentVariables(List)
*/
Builder exportedEnvironmentVariables(Consumer... exportedEnvironmentVariables);
/**
*
* An array of the ARNs associated with this build's reports.
*
*
* @param reportArns
* An array of the ARNs associated with this build's reports.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder reportArns(Collection reportArns);
/**
*
* An array of the ARNs associated with this build's reports.
*
*
* @param reportArns
* An array of the ARNs associated with this build's reports.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder reportArns(String... reportArns);
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using
* Amazon Elastic File System.
*
*
* @param fileSystemLocations
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
,
* location
, mountOptions
, mountPoint
, and type
of a
* file system created using Amazon Elastic File System.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder fileSystemLocations(Collection fileSystemLocations);
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using
* Amazon Elastic File System.
*
*
* @param fileSystemLocations
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
,
* location
, mountOptions
, mountPoint
, and type
of a
* file system created using Amazon Elastic File System.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder fileSystemLocations(ProjectFileSystemLocation... fileSystemLocations);
/**
*
* An array of ProjectFileSystemLocation
objects for a CodeBuild build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using
* Amazon Elastic File System.
*
* This is a convenience method that creates an instance of the {@link List.Builder}
* avoiding the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called
* immediately and its result is passed to {@link #fileSystemLocations(List)}.
*
* @param fileSystemLocations
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #fileSystemLocations(List)
*/
Builder fileSystemLocations(Consumer... fileSystemLocations);
/**
*
* Contains information about the debug session for this build.
*
*
* @param debugSession
* Contains information about the debug session for this build.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder debugSession(DebugSession debugSession);
/**
*
* Contains information about the debug session for this build.
*
* This is a convenience method that creates an instance of the {@link DebugSession.Builder} avoiding the need
* to create one manually via {@link DebugSession#builder()}.
*
* When the {@link Consumer} completes, {@link DebugSession.Builder#build()} is called immediately and its
* result is passed to {@link #debugSession(DebugSession)}.
*
* @param debugSession
* a consumer that will call methods on {@link DebugSession.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #debugSession(DebugSession)
*/
default Builder debugSession(Consumer debugSession) {
return debugSession(DebugSession.builder().applyMutation(debugSession).build());
}
/**
*
* The ARN of the batch build that this build is a member of, if applicable.
*
*
* @param buildBatchArn
* The ARN of the batch build that this build is a member of, if applicable.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder buildBatchArn(String buildBatchArn);
}
static final class BuilderImpl implements Builder {
private String id;
private String arn;
private Long buildNumber;
private Instant startTime;
private Instant endTime;
private String currentPhase;
private String buildStatus;
private String sourceVersion;
private String resolvedSourceVersion;
private String projectName;
private List phases = DefaultSdkAutoConstructList.getInstance();
private ProjectSource source;
private List secondarySources = DefaultSdkAutoConstructList.getInstance();
private List secondarySourceVersions = DefaultSdkAutoConstructList.getInstance();
private BuildArtifacts artifacts;
private List secondaryArtifacts = DefaultSdkAutoConstructList.getInstance();
private ProjectCache cache;
private ProjectEnvironment environment;
private String serviceRole;
private LogsLocation logs;
private Integer timeoutInMinutes;
private Integer queuedTimeoutInMinutes;
private Boolean buildComplete;
private String initiator;
private VpcConfig vpcConfig;
private NetworkInterface networkInterface;
private String encryptionKey;
private List exportedEnvironmentVariables = DefaultSdkAutoConstructList.getInstance();
private List reportArns = DefaultSdkAutoConstructList.getInstance();
private List fileSystemLocations = DefaultSdkAutoConstructList.getInstance();
private DebugSession debugSession;
private String buildBatchArn;
private BuilderImpl() {
}
private BuilderImpl(Build model) {
id(model.id);
arn(model.arn);
buildNumber(model.buildNumber);
startTime(model.startTime);
endTime(model.endTime);
currentPhase(model.currentPhase);
buildStatus(model.buildStatus);
sourceVersion(model.sourceVersion);
resolvedSourceVersion(model.resolvedSourceVersion);
projectName(model.projectName);
phases(model.phases);
source(model.source);
secondarySources(model.secondarySources);
secondarySourceVersions(model.secondarySourceVersions);
artifacts(model.artifacts);
secondaryArtifacts(model.secondaryArtifacts);
cache(model.cache);
environment(model.environment);
serviceRole(model.serviceRole);
logs(model.logs);
timeoutInMinutes(model.timeoutInMinutes);
queuedTimeoutInMinutes(model.queuedTimeoutInMinutes);
buildComplete(model.buildComplete);
initiator(model.initiator);
vpcConfig(model.vpcConfig);
networkInterface(model.networkInterface);
encryptionKey(model.encryptionKey);
exportedEnvironmentVariables(model.exportedEnvironmentVariables);
reportArns(model.reportArns);
fileSystemLocations(model.fileSystemLocations);
debugSession(model.debugSession);
buildBatchArn(model.buildBatchArn);
}
public final String getId() {
return id;
}
public final void setId(String id) {
this.id = id;
}
@Override
public final Builder id(String id) {
this.id = id;
return this;
}
public final String getArn() {
return arn;
}
public final void setArn(String arn) {
this.arn = arn;
}
@Override
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
public final Long getBuildNumber() {
return buildNumber;
}
public final void setBuildNumber(Long buildNumber) {
this.buildNumber = buildNumber;
}
@Override
public final Builder buildNumber(Long buildNumber) {
this.buildNumber = buildNumber;
return this;
}
public final Instant getStartTime() {
return startTime;
}
public final void setStartTime(Instant startTime) {
this.startTime = startTime;
}
@Override
public final Builder startTime(Instant startTime) {
this.startTime = startTime;
return this;
}
public final Instant getEndTime() {
return endTime;
}
public final void setEndTime(Instant endTime) {
this.endTime = endTime;
}
@Override
public final Builder endTime(Instant endTime) {
this.endTime = endTime;
return this;
}
public final String getCurrentPhase() {
return currentPhase;
}
public final void setCurrentPhase(String currentPhase) {
this.currentPhase = currentPhase;
}
@Override
public final Builder currentPhase(String currentPhase) {
this.currentPhase = currentPhase;
return this;
}
public final String getBuildStatus() {
return buildStatus;
}
public final void setBuildStatus(String buildStatus) {
this.buildStatus = buildStatus;
}
@Override
public final Builder buildStatus(String buildStatus) {
this.buildStatus = buildStatus;
return this;
}
@Override
public final Builder buildStatus(StatusType buildStatus) {
this.buildStatus(buildStatus == null ? null : buildStatus.toString());
return this;
}
public final String getSourceVersion() {
return sourceVersion;
}
public final void setSourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
}
@Override
public final Builder sourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
return this;
}
public final String getResolvedSourceVersion() {
return resolvedSourceVersion;
}
public final void setResolvedSourceVersion(String resolvedSourceVersion) {
this.resolvedSourceVersion = resolvedSourceVersion;
}
@Override
public final Builder resolvedSourceVersion(String resolvedSourceVersion) {
this.resolvedSourceVersion = resolvedSourceVersion;
return this;
}
public final String getProjectName() {
return projectName;
}
public final void setProjectName(String projectName) {
this.projectName = projectName;
}
@Override
public final Builder projectName(String projectName) {
this.projectName = projectName;
return this;
}
public final List getPhases() {
List result = BuildPhasesCopier.copyToBuilder(this.phases);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setPhases(Collection phases) {
this.phases = BuildPhasesCopier.copyFromBuilder(phases);
}
@Override
public final Builder phases(Collection phases) {
this.phases = BuildPhasesCopier.copy(phases);
return this;
}
@Override
@SafeVarargs
public final Builder phases(BuildPhase... phases) {
phases(Arrays.asList(phases));
return this;
}
@Override
@SafeVarargs
public final Builder phases(Consumer... phases) {
phases(Stream.of(phases).map(c -> BuildPhase.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final ProjectSource.Builder getSource() {
return source != null ? source.toBuilder() : null;
}
public final void setSource(ProjectSource.BuilderImpl source) {
this.source = source != null ? source.build() : null;
}
@Override
public final Builder source(ProjectSource source) {
this.source = source;
return this;
}
public final List getSecondarySources() {
List result = ProjectSourcesCopier.copyToBuilder(this.secondarySources);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setSecondarySources(Collection secondarySources) {
this.secondarySources = ProjectSourcesCopier.copyFromBuilder(secondarySources);
}
@Override
public final Builder secondarySources(Collection secondarySources) {
this.secondarySources = ProjectSourcesCopier.copy(secondarySources);
return this;
}
@Override
@SafeVarargs
public final Builder secondarySources(ProjectSource... secondarySources) {
secondarySources(Arrays.asList(secondarySources));
return this;
}
@Override
@SafeVarargs
public final Builder secondarySources(Consumer... secondarySources) {
secondarySources(Stream.of(secondarySources).map(c -> ProjectSource.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final List getSecondarySourceVersions() {
List result = ProjectSecondarySourceVersionsCopier
.copyToBuilder(this.secondarySourceVersions);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setSecondarySourceVersions(Collection secondarySourceVersions) {
this.secondarySourceVersions = ProjectSecondarySourceVersionsCopier.copyFromBuilder(secondarySourceVersions);
}
@Override
public final Builder secondarySourceVersions(Collection secondarySourceVersions) {
this.secondarySourceVersions = ProjectSecondarySourceVersionsCopier.copy(secondarySourceVersions);
return this;
}
@Override
@SafeVarargs
public final Builder secondarySourceVersions(ProjectSourceVersion... secondarySourceVersions) {
secondarySourceVersions(Arrays.asList(secondarySourceVersions));
return this;
}
@Override
@SafeVarargs
public final Builder secondarySourceVersions(Consumer... secondarySourceVersions) {
secondarySourceVersions(Stream.of(secondarySourceVersions)
.map(c -> ProjectSourceVersion.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final BuildArtifacts.Builder getArtifacts() {
return artifacts != null ? artifacts.toBuilder() : null;
}
public final void setArtifacts(BuildArtifacts.BuilderImpl artifacts) {
this.artifacts = artifacts != null ? artifacts.build() : null;
}
@Override
public final Builder artifacts(BuildArtifacts artifacts) {
this.artifacts = artifacts;
return this;
}
public final List getSecondaryArtifacts() {
List result = BuildArtifactsListCopier.copyToBuilder(this.secondaryArtifacts);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setSecondaryArtifacts(Collection secondaryArtifacts) {
this.secondaryArtifacts = BuildArtifactsListCopier.copyFromBuilder(secondaryArtifacts);
}
@Override
public final Builder secondaryArtifacts(Collection secondaryArtifacts) {
this.secondaryArtifacts = BuildArtifactsListCopier.copy(secondaryArtifacts);
return this;
}
@Override
@SafeVarargs
public final Builder secondaryArtifacts(BuildArtifacts... secondaryArtifacts) {
secondaryArtifacts(Arrays.asList(secondaryArtifacts));
return this;
}
@Override
@SafeVarargs
public final Builder secondaryArtifacts(Consumer... secondaryArtifacts) {
secondaryArtifacts(Stream.of(secondaryArtifacts).map(c -> BuildArtifacts.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final ProjectCache.Builder getCache() {
return cache != null ? cache.toBuilder() : null;
}
public final void setCache(ProjectCache.BuilderImpl cache) {
this.cache = cache != null ? cache.build() : null;
}
@Override
public final Builder cache(ProjectCache cache) {
this.cache = cache;
return this;
}
public final ProjectEnvironment.Builder getEnvironment() {
return environment != null ? environment.toBuilder() : null;
}
public final void setEnvironment(ProjectEnvironment.BuilderImpl environment) {
this.environment = environment != null ? environment.build() : null;
}
@Override
public final Builder environment(ProjectEnvironment environment) {
this.environment = environment;
return this;
}
public final String getServiceRole() {
return serviceRole;
}
public final void setServiceRole(String serviceRole) {
this.serviceRole = serviceRole;
}
@Override
public final Builder serviceRole(String serviceRole) {
this.serviceRole = serviceRole;
return this;
}
public final LogsLocation.Builder getLogs() {
return logs != null ? logs.toBuilder() : null;
}
public final void setLogs(LogsLocation.BuilderImpl logs) {
this.logs = logs != null ? logs.build() : null;
}
@Override
public final Builder logs(LogsLocation logs) {
this.logs = logs;
return this;
}
public final Integer getTimeoutInMinutes() {
return timeoutInMinutes;
}
public final void setTimeoutInMinutes(Integer timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
}
@Override
public final Builder timeoutInMinutes(Integer timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
return this;
}
public final Integer getQueuedTimeoutInMinutes() {
return queuedTimeoutInMinutes;
}
public final void setQueuedTimeoutInMinutes(Integer queuedTimeoutInMinutes) {
this.queuedTimeoutInMinutes = queuedTimeoutInMinutes;
}
@Override
public final Builder queuedTimeoutInMinutes(Integer queuedTimeoutInMinutes) {
this.queuedTimeoutInMinutes = queuedTimeoutInMinutes;
return this;
}
public final Boolean getBuildComplete() {
return buildComplete;
}
public final void setBuildComplete(Boolean buildComplete) {
this.buildComplete = buildComplete;
}
@Override
public final Builder buildComplete(Boolean buildComplete) {
this.buildComplete = buildComplete;
return this;
}
public final String getInitiator() {
return initiator;
}
public final void setInitiator(String initiator) {
this.initiator = initiator;
}
@Override
public final Builder initiator(String initiator) {
this.initiator = initiator;
return this;
}
public final VpcConfig.Builder getVpcConfig() {
return vpcConfig != null ? vpcConfig.toBuilder() : null;
}
public final void setVpcConfig(VpcConfig.BuilderImpl vpcConfig) {
this.vpcConfig = vpcConfig != null ? vpcConfig.build() : null;
}
@Override
public final Builder vpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
public final NetworkInterface.Builder getNetworkInterface() {
return networkInterface != null ? networkInterface.toBuilder() : null;
}
public final void setNetworkInterface(NetworkInterface.BuilderImpl networkInterface) {
this.networkInterface = networkInterface != null ? networkInterface.build() : null;
}
@Override
public final Builder networkInterface(NetworkInterface networkInterface) {
this.networkInterface = networkInterface;
return this;
}
public final String getEncryptionKey() {
return encryptionKey;
}
public final void setEncryptionKey(String encryptionKey) {
this.encryptionKey = encryptionKey;
}
@Override
public final Builder encryptionKey(String encryptionKey) {
this.encryptionKey = encryptionKey;
return this;
}
public final List getExportedEnvironmentVariables() {
List result = ExportedEnvironmentVariablesCopier
.copyToBuilder(this.exportedEnvironmentVariables);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setExportedEnvironmentVariables(
Collection exportedEnvironmentVariables) {
this.exportedEnvironmentVariables = ExportedEnvironmentVariablesCopier.copyFromBuilder(exportedEnvironmentVariables);
}
@Override
public final Builder exportedEnvironmentVariables(Collection exportedEnvironmentVariables) {
this.exportedEnvironmentVariables = ExportedEnvironmentVariablesCopier.copy(exportedEnvironmentVariables);
return this;
}
@Override
@SafeVarargs
public final Builder exportedEnvironmentVariables(ExportedEnvironmentVariable... exportedEnvironmentVariables) {
exportedEnvironmentVariables(Arrays.asList(exportedEnvironmentVariables));
return this;
}
@Override
@SafeVarargs
public final Builder exportedEnvironmentVariables(
Consumer... exportedEnvironmentVariables) {
exportedEnvironmentVariables(Stream.of(exportedEnvironmentVariables)
.map(c -> ExportedEnvironmentVariable.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Collection getReportArns() {
if (reportArns instanceof SdkAutoConstructList) {
return null;
}
return reportArns;
}
public final void setReportArns(Collection reportArns) {
this.reportArns = BuildReportArnsCopier.copy(reportArns);
}
@Override
public final Builder reportArns(Collection reportArns) {
this.reportArns = BuildReportArnsCopier.copy(reportArns);
return this;
}
@Override
@SafeVarargs
public final Builder reportArns(String... reportArns) {
reportArns(Arrays.asList(reportArns));
return this;
}
public final List getFileSystemLocations() {
List result = ProjectFileSystemLocationsCopier
.copyToBuilder(this.fileSystemLocations);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setFileSystemLocations(Collection fileSystemLocations) {
this.fileSystemLocations = ProjectFileSystemLocationsCopier.copyFromBuilder(fileSystemLocations);
}
@Override
public final Builder fileSystemLocations(Collection fileSystemLocations) {
this.fileSystemLocations = ProjectFileSystemLocationsCopier.copy(fileSystemLocations);
return this;
}
@Override
@SafeVarargs
public final Builder fileSystemLocations(ProjectFileSystemLocation... fileSystemLocations) {
fileSystemLocations(Arrays.asList(fileSystemLocations));
return this;
}
@Override
@SafeVarargs
public final Builder fileSystemLocations(Consumer... fileSystemLocations) {
fileSystemLocations(Stream.of(fileSystemLocations)
.map(c -> ProjectFileSystemLocation.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final DebugSession.Builder getDebugSession() {
return debugSession != null ? debugSession.toBuilder() : null;
}
public final void setDebugSession(DebugSession.BuilderImpl debugSession) {
this.debugSession = debugSession != null ? debugSession.build() : null;
}
@Override
public final Builder debugSession(DebugSession debugSession) {
this.debugSession = debugSession;
return this;
}
public final String getBuildBatchArn() {
return buildBatchArn;
}
public final void setBuildBatchArn(String buildBatchArn) {
this.buildBatchArn = buildBatchArn;
}
@Override
public final Builder buildBatchArn(String buildBatchArn) {
this.buildBatchArn = buildBatchArn;
return this;
}
@Override
public Build build() {
return new Build(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}