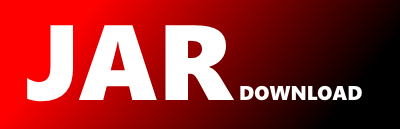
software.amazon.awssdk.services.codebuild.model.BuildBatchPhase Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.beans.Transient;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about a stage for a batch build.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BuildBatchPhase implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField PHASE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("phaseType").getter(getter(BuildBatchPhase::phaseTypeAsString)).setter(setter(Builder::phaseType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("phaseType").build()).build();
private static final SdkField PHASE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("phaseStatus").getter(getter(BuildBatchPhase::phaseStatusAsString)).setter(setter(Builder::phaseStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("phaseStatus").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startTime").getter(getter(BuildBatchPhase::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("endTime").getter(getter(BuildBatchPhase::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build()).build();
private static final SdkField DURATION_IN_SECONDS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("durationInSeconds").getter(getter(BuildBatchPhase::durationInSeconds))
.setter(setter(Builder::durationInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("durationInSeconds").build()).build();
private static final SdkField> CONTEXTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("contexts")
.getter(getter(BuildBatchPhase::contexts))
.setter(setter(Builder::contexts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("contexts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PhaseContext::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PHASE_TYPE_FIELD,
PHASE_STATUS_FIELD, START_TIME_FIELD, END_TIME_FIELD, DURATION_IN_SECONDS_FIELD, CONTEXTS_FIELD));
private static final long serialVersionUID = 1L;
private final String phaseType;
private final String phaseStatus;
private final Instant startTime;
private final Instant endTime;
private final Long durationInSeconds;
private final List contexts;
private BuildBatchPhase(BuilderImpl builder) {
this.phaseType = builder.phaseType;
this.phaseStatus = builder.phaseStatus;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.durationInSeconds = builder.durationInSeconds;
this.contexts = builder.contexts;
}
/**
*
* The name of the batch build phase. Valid values include:
*
*
* - COMBINE_ARTIFACTS
* -
*
* Build output artifacts are being combined and uploaded to the output location.
*
*
* - DOWNLOAD_BATCHSPEC
* -
*
* The batch build specification is being downloaded.
*
*
* - FAILED
* -
*
* One or more of the builds failed.
*
*
* - IN_PROGRESS
* -
*
* The batch build is in progress.
*
*
* - STOPPED
* -
*
* The batch build was stopped.
*
*
* - SUBMITTED
* -
*
* The btach build has been submitted.
*
*
* - SUCCEEDED
* -
*
* The batch build succeeded.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #phaseType} will
* return {@link BuildBatchPhaseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #phaseTypeAsString}.
*
*
* @return The name of the batch build phase. Valid values include:
*
* - COMBINE_ARTIFACTS
* -
*
* Build output artifacts are being combined and uploaded to the output location.
*
*
* - DOWNLOAD_BATCHSPEC
* -
*
* The batch build specification is being downloaded.
*
*
* - FAILED
* -
*
* One or more of the builds failed.
*
*
* - IN_PROGRESS
* -
*
* The batch build is in progress.
*
*
* - STOPPED
* -
*
* The batch build was stopped.
*
*
* - SUBMITTED
* -
*
* The btach build has been submitted.
*
*
* - SUCCEEDED
* -
*
* The batch build succeeded.
*
*
* @see BuildBatchPhaseType
*/
public final BuildBatchPhaseType phaseType() {
return BuildBatchPhaseType.fromValue(phaseType);
}
/**
*
* The name of the batch build phase. Valid values include:
*
*
* - COMBINE_ARTIFACTS
* -
*
* Build output artifacts are being combined and uploaded to the output location.
*
*
* - DOWNLOAD_BATCHSPEC
* -
*
* The batch build specification is being downloaded.
*
*
* - FAILED
* -
*
* One or more of the builds failed.
*
*
* - IN_PROGRESS
* -
*
* The batch build is in progress.
*
*
* - STOPPED
* -
*
* The batch build was stopped.
*
*
* - SUBMITTED
* -
*
* The btach build has been submitted.
*
*
* - SUCCEEDED
* -
*
* The batch build succeeded.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #phaseType} will
* return {@link BuildBatchPhaseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #phaseTypeAsString}.
*
*
* @return The name of the batch build phase. Valid values include:
*
* - COMBINE_ARTIFACTS
* -
*
* Build output artifacts are being combined and uploaded to the output location.
*
*
* - DOWNLOAD_BATCHSPEC
* -
*
* The batch build specification is being downloaded.
*
*
* - FAILED
* -
*
* One or more of the builds failed.
*
*
* - IN_PROGRESS
* -
*
* The batch build is in progress.
*
*
* - STOPPED
* -
*
* The batch build was stopped.
*
*
* - SUBMITTED
* -
*
* The btach build has been submitted.
*
*
* - SUCCEEDED
* -
*
* The batch build succeeded.
*
*
* @see BuildBatchPhaseType
*/
public final String phaseTypeAsString() {
return phaseType;
}
/**
*
* The current status of the batch build phase. Valid values include:
*
*
* - FAILED
* -
*
* The build phase failed.
*
*
* - FAULT
* -
*
* The build phase faulted.
*
*
* - IN_PROGRESS
* -
*
* The build phase is still in progress.
*
*
* - STOPPED
* -
*
* The build phase stopped.
*
*
* - SUCCEEDED
* -
*
* The build phase succeeded.
*
*
* - TIMED_OUT
* -
*
* The build phase timed out.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #phaseStatus} will
* return {@link StatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #phaseStatusAsString}.
*
*
* @return The current status of the batch build phase. Valid values include:
*
* - FAILED
* -
*
* The build phase failed.
*
*
* - FAULT
* -
*
* The build phase faulted.
*
*
* - IN_PROGRESS
* -
*
* The build phase is still in progress.
*
*
* - STOPPED
* -
*
* The build phase stopped.
*
*
* - SUCCEEDED
* -
*
* The build phase succeeded.
*
*
* - TIMED_OUT
* -
*
* The build phase timed out.
*
*
* @see StatusType
*/
public final StatusType phaseStatus() {
return StatusType.fromValue(phaseStatus);
}
/**
*
* The current status of the batch build phase. Valid values include:
*
*
* - FAILED
* -
*
* The build phase failed.
*
*
* - FAULT
* -
*
* The build phase faulted.
*
*
* - IN_PROGRESS
* -
*
* The build phase is still in progress.
*
*
* - STOPPED
* -
*
* The build phase stopped.
*
*
* - SUCCEEDED
* -
*
* The build phase succeeded.
*
*
* - TIMED_OUT
* -
*
* The build phase timed out.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #phaseStatus} will
* return {@link StatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #phaseStatusAsString}.
*
*
* @return The current status of the batch build phase. Valid values include:
*
* - FAILED
* -
*
* The build phase failed.
*
*
* - FAULT
* -
*
* The build phase faulted.
*
*
* - IN_PROGRESS
* -
*
* The build phase is still in progress.
*
*
* - STOPPED
* -
*
* The build phase stopped.
*
*
* - SUCCEEDED
* -
*
* The build phase succeeded.
*
*
* - TIMED_OUT
* -
*
* The build phase timed out.
*
*
* @see StatusType
*/
public final String phaseStatusAsString() {
return phaseStatus;
}
/**
*
* When the batch build phase started, expressed in Unix time format.
*
*
* @return When the batch build phase started, expressed in Unix time format.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* When the batch build phase ended, expressed in Unix time format.
*
*
* @return When the batch build phase ended, expressed in Unix time format.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* How long, in seconds, between the starting and ending times of the batch build's phase.
*
*
* @return How long, in seconds, between the starting and ending times of the batch build's phase.
*/
public final Long durationInSeconds() {
return durationInSeconds;
}
/**
* For responses, this returns true if the service returned a value for the Contexts property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasContexts() {
return contexts != null && !(contexts instanceof SdkAutoConstructList);
}
/**
*
* Additional information about the batch build phase. Especially to help troubleshoot a failed batch build.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContexts} method.
*
*
* @return Additional information about the batch build phase. Especially to help troubleshoot a failed batch build.
*/
public final List contexts() {
return contexts;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(phaseTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(phaseStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(durationInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(hasContexts() ? contexts() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BuildBatchPhase)) {
return false;
}
BuildBatchPhase other = (BuildBatchPhase) obj;
return Objects.equals(phaseTypeAsString(), other.phaseTypeAsString())
&& Objects.equals(phaseStatusAsString(), other.phaseStatusAsString())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(durationInSeconds(), other.durationInSeconds()) && hasContexts() == other.hasContexts()
&& Objects.equals(contexts(), other.contexts());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BuildBatchPhase").add("PhaseType", phaseTypeAsString())
.add("PhaseStatus", phaseStatusAsString()).add("StartTime", startTime()).add("EndTime", endTime())
.add("DurationInSeconds", durationInSeconds()).add("Contexts", hasContexts() ? contexts() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "phaseType":
return Optional.ofNullable(clazz.cast(phaseTypeAsString()));
case "phaseStatus":
return Optional.ofNullable(clazz.cast(phaseStatusAsString()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "endTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "durationInSeconds":
return Optional.ofNullable(clazz.cast(durationInSeconds()));
case "contexts":
return Optional.ofNullable(clazz.cast(contexts()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function