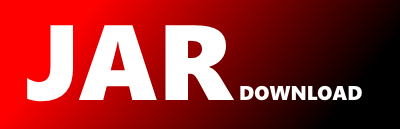
software.amazon.awssdk.services.codebuild.model.BuildBatch Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about a batch build.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BuildBatch implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(BuildBatch::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(BuildBatch::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startTime").getter(getter(BuildBatch::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("endTime").getter(getter(BuildBatch::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build()).build();
private static final SdkField CURRENT_PHASE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("currentPhase").getter(getter(BuildBatch::currentPhase)).setter(setter(Builder::currentPhase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("currentPhase").build()).build();
private static final SdkField BUILD_BATCH_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildBatchStatus").getter(getter(BuildBatch::buildBatchStatusAsString))
.setter(setter(Builder::buildBatchStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildBatchStatus").build()).build();
private static final SdkField SOURCE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceVersion").getter(getter(BuildBatch::sourceVersion)).setter(setter(Builder::sourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceVersion").build()).build();
private static final SdkField RESOLVED_SOURCE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resolvedSourceVersion").getter(getter(BuildBatch::resolvedSourceVersion))
.setter(setter(Builder::resolvedSourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resolvedSourceVersion").build())
.build();
private static final SdkField PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("projectName").getter(getter(BuildBatch::projectName)).setter(setter(Builder::projectName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("projectName").build()).build();
private static final SdkField> PHASES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("phases")
.getter(getter(BuildBatch::phases))
.setter(setter(Builder::phases))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("phases").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BuildBatchPhase::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("source").getter(getter(BuildBatch::source)).setter(setter(Builder::source))
.constructor(ProjectSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("source").build()).build();
private static final SdkField> SECONDARY_SOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondarySources")
.getter(getter(BuildBatch::secondarySources))
.setter(setter(Builder::secondarySources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySources").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SECONDARY_SOURCE_VERSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondarySourceVersions")
.getter(getter(BuildBatch::secondarySourceVersions))
.setter(setter(Builder::secondarySourceVersions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySourceVersions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSourceVersion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ARTIFACTS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("artifacts").getter(getter(BuildBatch::artifacts)).setter(setter(Builder::artifacts))
.constructor(BuildArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("artifacts").build()).build();
private static final SdkField> SECONDARY_ARTIFACTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondaryArtifacts")
.getter(getter(BuildBatch::secondaryArtifacts))
.setter(setter(Builder::secondaryArtifacts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondaryArtifacts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BuildArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CACHE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("cache").getter(getter(BuildBatch::cache)).setter(setter(Builder::cache))
.constructor(ProjectCache::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cache").build()).build();
private static final SdkField ENVIRONMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("environment")
.getter(getter(BuildBatch::environment)).setter(setter(Builder::environment))
.constructor(ProjectEnvironment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environment").build()).build();
private static final SdkField SERVICE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceRole").getter(getter(BuildBatch::serviceRole)).setter(setter(Builder::serviceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRole").build()).build();
private static final SdkField LOG_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("logConfig").getter(getter(BuildBatch::logConfig)).setter(setter(Builder::logConfig))
.constructor(LogsConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logConfig").build()).build();
private static final SdkField BUILD_TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("buildTimeoutInMinutes").getter(getter(BuildBatch::buildTimeoutInMinutes))
.setter(setter(Builder::buildTimeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildTimeoutInMinutes").build())
.build();
private static final SdkField QUEUED_TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("queuedTimeoutInMinutes").getter(getter(BuildBatch::queuedTimeoutInMinutes))
.setter(setter(Builder::queuedTimeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("queuedTimeoutInMinutes").build())
.build();
private static final SdkField COMPLETE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("complete").getter(getter(BuildBatch::complete)).setter(setter(Builder::complete))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("complete").build()).build();
private static final SdkField INITIATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("initiator").getter(getter(BuildBatch::initiator)).setter(setter(Builder::initiator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("initiator").build()).build();
private static final SdkField VPC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("vpcConfig").getter(getter(BuildBatch::vpcConfig)).setter(setter(Builder::vpcConfig))
.constructor(VpcConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpcConfig").build()).build();
private static final SdkField ENCRYPTION_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("encryptionKey").getter(getter(BuildBatch::encryptionKey)).setter(setter(Builder::encryptionKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionKey").build()).build();
private static final SdkField BUILD_BATCH_NUMBER_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("buildBatchNumber").getter(getter(BuildBatch::buildBatchNumber))
.setter(setter(Builder::buildBatchNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildBatchNumber").build()).build();
private static final SdkField> FILE_SYSTEM_LOCATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("fileSystemLocations")
.getter(getter(BuildBatch::fileSystemLocations))
.setter(setter(Builder::fileSystemLocations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fileSystemLocations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectFileSystemLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField BUILD_BATCH_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("buildBatchConfig")
.getter(getter(BuildBatch::buildBatchConfig)).setter(setter(Builder::buildBatchConfig))
.constructor(ProjectBuildBatchConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildBatchConfig").build()).build();
private static final SdkField> BUILD_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("buildGroups")
.getter(getter(BuildBatch::buildGroups))
.setter(setter(Builder::buildGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BuildGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DEBUG_SESSION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("debugSessionEnabled").getter(getter(BuildBatch::debugSessionEnabled))
.setter(setter(Builder::debugSessionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("debugSessionEnabled").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ARN_FIELD,
START_TIME_FIELD, END_TIME_FIELD, CURRENT_PHASE_FIELD, BUILD_BATCH_STATUS_FIELD, SOURCE_VERSION_FIELD,
RESOLVED_SOURCE_VERSION_FIELD, PROJECT_NAME_FIELD, PHASES_FIELD, SOURCE_FIELD, SECONDARY_SOURCES_FIELD,
SECONDARY_SOURCE_VERSIONS_FIELD, ARTIFACTS_FIELD, SECONDARY_ARTIFACTS_FIELD, CACHE_FIELD, ENVIRONMENT_FIELD,
SERVICE_ROLE_FIELD, LOG_CONFIG_FIELD, BUILD_TIMEOUT_IN_MINUTES_FIELD, QUEUED_TIMEOUT_IN_MINUTES_FIELD,
COMPLETE_FIELD, INITIATOR_FIELD, VPC_CONFIG_FIELD, ENCRYPTION_KEY_FIELD, BUILD_BATCH_NUMBER_FIELD,
FILE_SYSTEM_LOCATIONS_FIELD, BUILD_BATCH_CONFIG_FIELD, BUILD_GROUPS_FIELD, DEBUG_SESSION_ENABLED_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String arn;
private final Instant startTime;
private final Instant endTime;
private final String currentPhase;
private final String buildBatchStatus;
private final String sourceVersion;
private final String resolvedSourceVersion;
private final String projectName;
private final List phases;
private final ProjectSource source;
private final List secondarySources;
private final List secondarySourceVersions;
private final BuildArtifacts artifacts;
private final List secondaryArtifacts;
private final ProjectCache cache;
private final ProjectEnvironment environment;
private final String serviceRole;
private final LogsConfig logConfig;
private final Integer buildTimeoutInMinutes;
private final Integer queuedTimeoutInMinutes;
private final Boolean complete;
private final String initiator;
private final VpcConfig vpcConfig;
private final String encryptionKey;
private final Long buildBatchNumber;
private final List fileSystemLocations;
private final ProjectBuildBatchConfig buildBatchConfig;
private final List buildGroups;
private final Boolean debugSessionEnabled;
private BuildBatch(BuilderImpl builder) {
this.id = builder.id;
this.arn = builder.arn;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.currentPhase = builder.currentPhase;
this.buildBatchStatus = builder.buildBatchStatus;
this.sourceVersion = builder.sourceVersion;
this.resolvedSourceVersion = builder.resolvedSourceVersion;
this.projectName = builder.projectName;
this.phases = builder.phases;
this.source = builder.source;
this.secondarySources = builder.secondarySources;
this.secondarySourceVersions = builder.secondarySourceVersions;
this.artifacts = builder.artifacts;
this.secondaryArtifacts = builder.secondaryArtifacts;
this.cache = builder.cache;
this.environment = builder.environment;
this.serviceRole = builder.serviceRole;
this.logConfig = builder.logConfig;
this.buildTimeoutInMinutes = builder.buildTimeoutInMinutes;
this.queuedTimeoutInMinutes = builder.queuedTimeoutInMinutes;
this.complete = builder.complete;
this.initiator = builder.initiator;
this.vpcConfig = builder.vpcConfig;
this.encryptionKey = builder.encryptionKey;
this.buildBatchNumber = builder.buildBatchNumber;
this.fileSystemLocations = builder.fileSystemLocations;
this.buildBatchConfig = builder.buildBatchConfig;
this.buildGroups = builder.buildGroups;
this.debugSessionEnabled = builder.debugSessionEnabled;
}
/**
*
* The identifier of the batch build.
*
*
* @return The identifier of the batch build.
*/
public final String id() {
return id;
}
/**
*
* The ARN of the batch build.
*
*
* @return The ARN of the batch build.
*/
public final String arn() {
return arn;
}
/**
*
* The date and time that the batch build started.
*
*
* @return The date and time that the batch build started.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The date and time that the batch build ended.
*
*
* @return The date and time that the batch build ended.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* The current phase of the batch build.
*
*
* @return The current phase of the batch build.
*/
public final String currentPhase() {
return currentPhase;
}
/**
*
* The status of the batch build.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildBatchStatus}
* will return {@link StatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildBatchStatusAsString}.
*
*
* @return The status of the batch build.
* @see StatusType
*/
public final StatusType buildBatchStatus() {
return StatusType.fromValue(buildBatchStatus);
}
/**
*
* The status of the batch build.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildBatchStatus}
* will return {@link StatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildBatchStatusAsString}.
*
*
* @return The status of the batch build.
* @see StatusType
*/
public final String buildBatchStatusAsString() {
return buildBatchStatus;
}
/**
*
* The identifier of the version of the source code to be built.
*
*
* @return The identifier of the version of the source code to be built.
*/
public final String sourceVersion() {
return sourceVersion;
}
/**
*
* The identifier of the resolved version of this batch build's source code.
*
*
* -
*
* For CodeCommit, GitHub, GitHub Enterprise, and BitBucket, the commit ID.
*
*
* -
*
* For CodePipeline, the source revision provided by CodePipeline.
*
*
* -
*
* For Amazon S3, this does not apply.
*
*
*
*
* @return The identifier of the resolved version of this batch build's source code.
*
* -
*
* For CodeCommit, GitHub, GitHub Enterprise, and BitBucket, the commit ID.
*
*
* -
*
* For CodePipeline, the source revision provided by CodePipeline.
*
*
* -
*
* For Amazon S3, this does not apply.
*
*
*/
public final String resolvedSourceVersion() {
return resolvedSourceVersion;
}
/**
*
* The name of the batch build project.
*
*
* @return The name of the batch build project.
*/
public final String projectName() {
return projectName;
}
/**
* For responses, this returns true if the service returned a value for the Phases property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasPhases() {
return phases != null && !(phases instanceof SdkAutoConstructList);
}
/**
*
* An array of BuildBatchPhase
objects the specify the phases of the batch build.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPhases} method.
*
*
* @return An array of BuildBatchPhase
objects the specify the phases of the batch build.
*/
public final List phases() {
return phases;
}
/**
* Returns the value of the Source property for this object.
*
* @return The value of the Source property for this object.
*/
public final ProjectSource source() {
return source;
}
/**
* For responses, this returns true if the service returned a value for the SecondarySources property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecondarySources() {
return secondarySources != null && !(secondarySources instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSource
objects that define the sources for the batch build.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondarySources} method.
*
*
* @return An array of ProjectSource
objects that define the sources for the batch build.
*/
public final List secondarySources() {
return secondarySources;
}
/**
* For responses, this returns true if the service returned a value for the SecondarySourceVersions property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasSecondarySourceVersions() {
return secondarySourceVersions != null && !(secondarySourceVersions instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be one of:
*
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the branch's
* HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default
* branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondarySourceVersions} method.
*
*
* @return An array of ProjectSourceVersion
objects. Each ProjectSourceVersion
must be one
* of:
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of
* the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code
* you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified,
* the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*/
public final List secondarySourceVersions() {
return secondarySourceVersions;
}
/**
*
* A BuildArtifacts
object the defines the build artifacts for this batch build.
*
*
* @return A BuildArtifacts
object the defines the build artifacts for this batch build.
*/
public final BuildArtifacts artifacts() {
return artifacts;
}
/**
* For responses, this returns true if the service returned a value for the SecondaryArtifacts property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecondaryArtifacts() {
return secondaryArtifacts != null && !(secondaryArtifacts instanceof SdkAutoConstructList);
}
/**
*
* An array of BuildArtifacts
objects the define the build artifacts for this batch build.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondaryArtifacts} method.
*
*
* @return An array of BuildArtifacts
objects the define the build artifacts for this batch build.
*/
public final List secondaryArtifacts() {
return secondaryArtifacts;
}
/**
* Returns the value of the Cache property for this object.
*
* @return The value of the Cache property for this object.
*/
public final ProjectCache cache() {
return cache;
}
/**
* Returns the value of the Environment property for this object.
*
* @return The value of the Environment property for this object.
*/
public final ProjectEnvironment environment() {
return environment;
}
/**
*
* The name of a service role used for builds in the batch.
*
*
* @return The name of a service role used for builds in the batch.
*/
public final String serviceRole() {
return serviceRole;
}
/**
* Returns the value of the LogConfig property for this object.
*
* @return The value of the LogConfig property for this object.
*/
public final LogsConfig logConfig() {
return logConfig;
}
/**
*
* Specifies the maximum amount of time, in minutes, that the build in a batch must be completed in.
*
*
* @return Specifies the maximum amount of time, in minutes, that the build in a batch must be completed in.
*/
public final Integer buildTimeoutInMinutes() {
return buildTimeoutInMinutes;
}
/**
*
* Specifies the amount of time, in minutes, that the batch build is allowed to be queued before it times out.
*
*
* @return Specifies the amount of time, in minutes, that the batch build is allowed to be queued before it times
* out.
*/
public final Integer queuedTimeoutInMinutes() {
return queuedTimeoutInMinutes;
}
/**
*
* Indicates if the batch build is complete.
*
*
* @return Indicates if the batch build is complete.
*/
public final Boolean complete() {
return complete;
}
/**
*
* The entity that started the batch build. Valid values include:
*
*
* -
*
* If CodePipeline started the build, the pipeline's name (for example, codepipeline/my-demo-pipeline
).
*
*
* -
*
* If an IAM user started the build, the user's name.
*
*
* -
*
* If the Jenkins plugin for CodeBuild started the build, the string CodeBuild-Jenkins-Plugin
.
*
*
*
*
* @return The entity that started the batch build. Valid values include:
*
* -
*
* If CodePipeline started the build, the pipeline's name (for example,
* codepipeline/my-demo-pipeline
).
*
*
* -
*
* If an IAM user started the build, the user's name.
*
*
* -
*
* If the Jenkins plugin for CodeBuild started the build, the string CodeBuild-Jenkins-Plugin
.
*
*
*/
public final String initiator() {
return initiator;
}
/**
* Returns the value of the VpcConfig property for this object.
*
* @return The value of the VpcConfig property for this object.
*/
public final VpcConfig vpcConfig() {
return vpcConfig;
}
/**
*
* The Key Management Service customer master key (CMK) to be used for encrypting the batch build output artifacts.
*
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission to
* that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the
* format alias/<alias-name>
).
*
*
* @return The Key Management Service customer master key (CMK) to be used for encrypting the batch build output
* artifacts.
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has
* permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using
* the format alias/<alias-name>
).
*/
public final String encryptionKey() {
return encryptionKey;
}
/**
*
* The number of the batch build. For each project, the buildBatchNumber
of its first batch build is
* 1
. The buildBatchNumber
of each subsequent batch build is incremented by 1
* . If a batch build is deleted, the buildBatchNumber
of other batch builds does not change.
*
*
* @return The number of the batch build. For each project, the buildBatchNumber
of its first batch
* build is 1
. The buildBatchNumber
of each subsequent batch build is incremented
* by 1
. If a batch build is deleted, the buildBatchNumber
of other batch builds
* does not change.
*/
public final Long buildBatchNumber() {
return buildBatchNumber;
}
/**
* For responses, this returns true if the service returned a value for the FileSystemLocations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasFileSystemLocations() {
return fileSystemLocations != null && !(fileSystemLocations instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectFileSystemLocation
objects for the batch build project. A
* ProjectFileSystemLocation
object specifies the identifier
, location
,
* mountOptions
, mountPoint
, and type
of a file system created using Amazon
* Elastic File System.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFileSystemLocations} method.
*
*
* @return An array of ProjectFileSystemLocation
objects for the batch build project. A
* ProjectFileSystemLocation
object specifies the identifier
,
* location
, mountOptions
, mountPoint
, and type
of a
* file system created using Amazon Elastic File System.
*/
public final List fileSystemLocations() {
return fileSystemLocations;
}
/**
* Returns the value of the BuildBatchConfig property for this object.
*
* @return The value of the BuildBatchConfig property for this object.
*/
public final ProjectBuildBatchConfig buildBatchConfig() {
return buildBatchConfig;
}
/**
* For responses, this returns true if the service returned a value for the BuildGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasBuildGroups() {
return buildGroups != null && !(buildGroups instanceof SdkAutoConstructList);
}
/**
*
* An array of BuildGroup
objects that define the build groups for the batch build.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasBuildGroups} method.
*
*
* @return An array of BuildGroup
objects that define the build groups for the batch build.
*/
public final List buildGroups() {
return buildGroups;
}
/**
*
* Specifies if session debugging is enabled for this batch build. For more information, see Viewing a running build in
* Session Manager. Batch session debugging is not supported for matrix batch builds.
*
*
* @return Specifies if session debugging is enabled for this batch build. For more information, see Viewing a running
* build in Session Manager. Batch session debugging is not supported for matrix batch builds.
*/
public final Boolean debugSessionEnabled() {
return debugSessionEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(currentPhase());
hashCode = 31 * hashCode + Objects.hashCode(buildBatchStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(sourceVersion());
hashCode = 31 * hashCode + Objects.hashCode(resolvedSourceVersion());
hashCode = 31 * hashCode + Objects.hashCode(projectName());
hashCode = 31 * hashCode + Objects.hashCode(hasPhases() ? phases() : null);
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(hasSecondarySources() ? secondarySources() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSecondarySourceVersions() ? secondarySourceVersions() : null);
hashCode = 31 * hashCode + Objects.hashCode(artifacts());
hashCode = 31 * hashCode + Objects.hashCode(hasSecondaryArtifacts() ? secondaryArtifacts() : null);
hashCode = 31 * hashCode + Objects.hashCode(cache());
hashCode = 31 * hashCode + Objects.hashCode(environment());
hashCode = 31 * hashCode + Objects.hashCode(serviceRole());
hashCode = 31 * hashCode + Objects.hashCode(logConfig());
hashCode = 31 * hashCode + Objects.hashCode(buildTimeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(queuedTimeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(complete());
hashCode = 31 * hashCode + Objects.hashCode(initiator());
hashCode = 31 * hashCode + Objects.hashCode(vpcConfig());
hashCode = 31 * hashCode + Objects.hashCode(encryptionKey());
hashCode = 31 * hashCode + Objects.hashCode(buildBatchNumber());
hashCode = 31 * hashCode + Objects.hashCode(hasFileSystemLocations() ? fileSystemLocations() : null);
hashCode = 31 * hashCode + Objects.hashCode(buildBatchConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasBuildGroups() ? buildGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(debugSessionEnabled());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BuildBatch)) {
return false;
}
BuildBatch other = (BuildBatch) obj;
return Objects.equals(id(), other.id()) && Objects.equals(arn(), other.arn())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(currentPhase(), other.currentPhase())
&& Objects.equals(buildBatchStatusAsString(), other.buildBatchStatusAsString())
&& Objects.equals(sourceVersion(), other.sourceVersion())
&& Objects.equals(resolvedSourceVersion(), other.resolvedSourceVersion())
&& Objects.equals(projectName(), other.projectName()) && hasPhases() == other.hasPhases()
&& Objects.equals(phases(), other.phases()) && Objects.equals(source(), other.source())
&& hasSecondarySources() == other.hasSecondarySources()
&& Objects.equals(secondarySources(), other.secondarySources())
&& hasSecondarySourceVersions() == other.hasSecondarySourceVersions()
&& Objects.equals(secondarySourceVersions(), other.secondarySourceVersions())
&& Objects.equals(artifacts(), other.artifacts()) && hasSecondaryArtifacts() == other.hasSecondaryArtifacts()
&& Objects.equals(secondaryArtifacts(), other.secondaryArtifacts()) && Objects.equals(cache(), other.cache())
&& Objects.equals(environment(), other.environment()) && Objects.equals(serviceRole(), other.serviceRole())
&& Objects.equals(logConfig(), other.logConfig())
&& Objects.equals(buildTimeoutInMinutes(), other.buildTimeoutInMinutes())
&& Objects.equals(queuedTimeoutInMinutes(), other.queuedTimeoutInMinutes())
&& Objects.equals(complete(), other.complete()) && Objects.equals(initiator(), other.initiator())
&& Objects.equals(vpcConfig(), other.vpcConfig()) && Objects.equals(encryptionKey(), other.encryptionKey())
&& Objects.equals(buildBatchNumber(), other.buildBatchNumber())
&& hasFileSystemLocations() == other.hasFileSystemLocations()
&& Objects.equals(fileSystemLocations(), other.fileSystemLocations())
&& Objects.equals(buildBatchConfig(), other.buildBatchConfig()) && hasBuildGroups() == other.hasBuildGroups()
&& Objects.equals(buildGroups(), other.buildGroups())
&& Objects.equals(debugSessionEnabled(), other.debugSessionEnabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BuildBatch").add("Id", id()).add("Arn", arn()).add("StartTime", startTime())
.add("EndTime", endTime()).add("CurrentPhase", currentPhase())
.add("BuildBatchStatus", buildBatchStatusAsString()).add("SourceVersion", sourceVersion())
.add("ResolvedSourceVersion", resolvedSourceVersion()).add("ProjectName", projectName())
.add("Phases", hasPhases() ? phases() : null).add("Source", source())
.add("SecondarySources", hasSecondarySources() ? secondarySources() : null)
.add("SecondarySourceVersions", hasSecondarySourceVersions() ? secondarySourceVersions() : null)
.add("Artifacts", artifacts()).add("SecondaryArtifacts", hasSecondaryArtifacts() ? secondaryArtifacts() : null)
.add("Cache", cache()).add("Environment", environment()).add("ServiceRole", serviceRole())
.add("LogConfig", logConfig()).add("BuildTimeoutInMinutes", buildTimeoutInMinutes())
.add("QueuedTimeoutInMinutes", queuedTimeoutInMinutes()).add("Complete", complete())
.add("Initiator", initiator()).add("VpcConfig", vpcConfig()).add("EncryptionKey", encryptionKey())
.add("BuildBatchNumber", buildBatchNumber())
.add("FileSystemLocations", hasFileSystemLocations() ? fileSystemLocations() : null)
.add("BuildBatchConfig", buildBatchConfig()).add("BuildGroups", hasBuildGroups() ? buildGroups() : null)
.add("DebugSessionEnabled", debugSessionEnabled()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "endTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "currentPhase":
return Optional.ofNullable(clazz.cast(currentPhase()));
case "buildBatchStatus":
return Optional.ofNullable(clazz.cast(buildBatchStatusAsString()));
case "sourceVersion":
return Optional.ofNullable(clazz.cast(sourceVersion()));
case "resolvedSourceVersion":
return Optional.ofNullable(clazz.cast(resolvedSourceVersion()));
case "projectName":
return Optional.ofNullable(clazz.cast(projectName()));
case "phases":
return Optional.ofNullable(clazz.cast(phases()));
case "source":
return Optional.ofNullable(clazz.cast(source()));
case "secondarySources":
return Optional.ofNullable(clazz.cast(secondarySources()));
case "secondarySourceVersions":
return Optional.ofNullable(clazz.cast(secondarySourceVersions()));
case "artifacts":
return Optional.ofNullable(clazz.cast(artifacts()));
case "secondaryArtifacts":
return Optional.ofNullable(clazz.cast(secondaryArtifacts()));
case "cache":
return Optional.ofNullable(clazz.cast(cache()));
case "environment":
return Optional.ofNullable(clazz.cast(environment()));
case "serviceRole":
return Optional.ofNullable(clazz.cast(serviceRole()));
case "logConfig":
return Optional.ofNullable(clazz.cast(logConfig()));
case "buildTimeoutInMinutes":
return Optional.ofNullable(clazz.cast(buildTimeoutInMinutes()));
case "queuedTimeoutInMinutes":
return Optional.ofNullable(clazz.cast(queuedTimeoutInMinutes()));
case "complete":
return Optional.ofNullable(clazz.cast(complete()));
case "initiator":
return Optional.ofNullable(clazz.cast(initiator()));
case "vpcConfig":
return Optional.ofNullable(clazz.cast(vpcConfig()));
case "encryptionKey":
return Optional.ofNullable(clazz.cast(encryptionKey()));
case "buildBatchNumber":
return Optional.ofNullable(clazz.cast(buildBatchNumber()));
case "fileSystemLocations":
return Optional.ofNullable(clazz.cast(fileSystemLocations()));
case "buildBatchConfig":
return Optional.ofNullable(clazz.cast(buildBatchConfig()));
case "buildGroups":
return Optional.ofNullable(clazz.cast(buildGroups()));
case "debugSessionEnabled":
return Optional.ofNullable(clazz.cast(debugSessionEnabled()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function