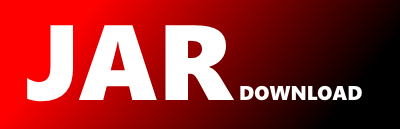
software.amazon.awssdk.services.codebuild.model.UpdateWebhookRequest Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateWebhookRequest extends CodeBuildRequest implements
ToCopyableBuilder {
private static final SdkField PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("projectName").getter(getter(UpdateWebhookRequest::projectName)).setter(setter(Builder::projectName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("projectName").build()).build();
private static final SdkField BRANCH_FILTER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("branchFilter").getter(getter(UpdateWebhookRequest::branchFilter)).setter(setter(Builder::branchFilter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("branchFilter").build()).build();
private static final SdkField ROTATE_SECRET_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("rotateSecret").getter(getter(UpdateWebhookRequest::rotateSecret)).setter(setter(Builder::rotateSecret))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rotateSecret").build()).build();
private static final SdkField>> FILTER_GROUPS_FIELD = SdkField
.>> builder(MarshallingType.LIST)
.memberName("filterGroups")
.getter(getter(UpdateWebhookRequest::filterGroups))
.setter(setter(Builder::filterGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("filterGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField.> builder(MarshallingType.LIST)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(WebhookFilter::builder)
.traits(LocationTrait.builder()
.location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build())
.build()).build()).build()).build();
private static final SdkField BUILD_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildType").getter(getter(UpdateWebhookRequest::buildTypeAsString)).setter(setter(Builder::buildType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PROJECT_NAME_FIELD,
BRANCH_FILTER_FIELD, ROTATE_SECRET_FIELD, FILTER_GROUPS_FIELD, BUILD_TYPE_FIELD));
private final String projectName;
private final String branchFilter;
private final Boolean rotateSecret;
private final List> filterGroups;
private final String buildType;
private UpdateWebhookRequest(BuilderImpl builder) {
super(builder);
this.projectName = builder.projectName;
this.branchFilter = builder.branchFilter;
this.rotateSecret = builder.rotateSecret;
this.filterGroups = builder.filterGroups;
this.buildType = builder.buildType;
}
/**
*
* The name of the CodeBuild project.
*
*
* @return The name of the CodeBuild project.
*/
public final String projectName() {
return projectName;
}
/**
*
* A regular expression used to determine which repository branches are built when a webhook is triggered. If the
* name of a branch matches the regular expression, then it is built. If branchFilter
is empty, then
* all branches are built.
*
*
*
* It is recommended that you use filterGroups
instead of branchFilter
.
*
*
*
* @return A regular expression used to determine which repository branches are built when a webhook is triggered.
* If the name of a branch matches the regular expression, then it is built. If branchFilter
is
* empty, then all branches are built.
*
* It is recommended that you use filterGroups
instead of branchFilter
.
*
*/
public final String branchFilter() {
return branchFilter;
}
/**
*
* A boolean value that specifies whether the associated GitHub repository's secret token should be updated. If you
* use Bitbucket for your repository, rotateSecret
is ignored.
*
*
* @return A boolean value that specifies whether the associated GitHub repository's secret token should be updated.
* If you use Bitbucket for your repository, rotateSecret
is ignored.
*/
public final Boolean rotateSecret() {
return rotateSecret;
}
/**
* For responses, this returns true if the service returned a value for the FilterGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasFilterGroups() {
return filterGroups != null && !(filterGroups instanceof SdkAutoConstructList);
}
/**
*
* An array of arrays of WebhookFilter
objects used to determine if a webhook event can trigger a
* build. A filter group must contain at least one EVENT
WebhookFilter
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFilterGroups} method.
*
*
* @return An array of arrays of WebhookFilter
objects used to determine if a webhook event can trigger
* a build. A filter group must contain at least one EVENT
WebhookFilter
.
*/
public final List> filterGroups() {
return filterGroups;
}
/**
*
* Specifies the type of build this webhook will trigger.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildType} will
* return {@link WebhookBuildType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildTypeAsString}.
*
*
* @return Specifies the type of build this webhook will trigger.
* @see WebhookBuildType
*/
public final WebhookBuildType buildType() {
return WebhookBuildType.fromValue(buildType);
}
/**
*
* Specifies the type of build this webhook will trigger.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildType} will
* return {@link WebhookBuildType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildTypeAsString}.
*
*
* @return Specifies the type of build this webhook will trigger.
* @see WebhookBuildType
*/
public final String buildTypeAsString() {
return buildType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(projectName());
hashCode = 31 * hashCode + Objects.hashCode(branchFilter());
hashCode = 31 * hashCode + Objects.hashCode(rotateSecret());
hashCode = 31 * hashCode + Objects.hashCode(hasFilterGroups() ? filterGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(buildTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateWebhookRequest)) {
return false;
}
UpdateWebhookRequest other = (UpdateWebhookRequest) obj;
return Objects.equals(projectName(), other.projectName()) && Objects.equals(branchFilter(), other.branchFilter())
&& Objects.equals(rotateSecret(), other.rotateSecret()) && hasFilterGroups() == other.hasFilterGroups()
&& Objects.equals(filterGroups(), other.filterGroups())
&& Objects.equals(buildTypeAsString(), other.buildTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateWebhookRequest").add("ProjectName", projectName()).add("BranchFilter", branchFilter())
.add("RotateSecret", rotateSecret()).add("FilterGroups", hasFilterGroups() ? filterGroups() : null)
.add("BuildType", buildTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "projectName":
return Optional.ofNullable(clazz.cast(projectName()));
case "branchFilter":
return Optional.ofNullable(clazz.cast(branchFilter()));
case "rotateSecret":
return Optional.ofNullable(clazz.cast(rotateSecret()));
case "filterGroups":
return Optional.ofNullable(clazz.cast(filterGroups()));
case "buildType":
return Optional.ofNullable(clazz.cast(buildTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function