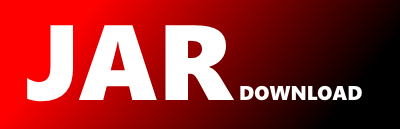
software.amazon.awssdk.services.codebuild.model.BuildArtifacts Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about build output artifacts.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BuildArtifacts implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField LOCATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("location").getter(getter(BuildArtifacts::location)).setter(setter(Builder::location))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("location").build()).build();
private static final SdkField SHA256_SUM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sha256sum").getter(getter(BuildArtifacts::sha256sum)).setter(setter(Builder::sha256sum))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sha256sum").build()).build();
private static final SdkField MD5_SUM_FIELD = SdkField. builder(MarshallingType.STRING).memberName("md5sum")
.getter(getter(BuildArtifacts::md5sum)).setter(setter(Builder::md5sum))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("md5sum").build()).build();
private static final SdkField OVERRIDE_ARTIFACT_NAME_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("overrideArtifactName").getter(getter(BuildArtifacts::overrideArtifactName))
.setter(setter(Builder::overrideArtifactName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("overrideArtifactName").build())
.build();
private static final SdkField ENCRYPTION_DISABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("encryptionDisabled").getter(getter(BuildArtifacts::encryptionDisabled))
.setter(setter(Builder::encryptionDisabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionDisabled").build())
.build();
private static final SdkField ARTIFACT_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("artifactIdentifier").getter(getter(BuildArtifacts::artifactIdentifier))
.setter(setter(Builder::artifactIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("artifactIdentifier").build())
.build();
private static final SdkField BUCKET_OWNER_ACCESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("bucketOwnerAccess").getter(getter(BuildArtifacts::bucketOwnerAccessAsString))
.setter(setter(Builder::bucketOwnerAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("bucketOwnerAccess").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOCATION_FIELD,
SHA256_SUM_FIELD, MD5_SUM_FIELD, OVERRIDE_ARTIFACT_NAME_FIELD, ENCRYPTION_DISABLED_FIELD, ARTIFACT_IDENTIFIER_FIELD,
BUCKET_OWNER_ACCESS_FIELD));
private static final long serialVersionUID = 1L;
private final String location;
private final String sha256sum;
private final String md5sum;
private final Boolean overrideArtifactName;
private final Boolean encryptionDisabled;
private final String artifactIdentifier;
private final String bucketOwnerAccess;
private BuildArtifacts(BuilderImpl builder) {
this.location = builder.location;
this.sha256sum = builder.sha256sum;
this.md5sum = builder.md5sum;
this.overrideArtifactName = builder.overrideArtifactName;
this.encryptionDisabled = builder.encryptionDisabled;
this.artifactIdentifier = builder.artifactIdentifier;
this.bucketOwnerAccess = builder.bucketOwnerAccess;
}
/**
*
* Information about the location of the build artifacts.
*
*
* @return Information about the location of the build artifacts.
*/
public final String location() {
return location;
}
/**
*
* The SHA-256 hash of the build artifact.
*
*
* You can use this hash along with a checksum tool to confirm file integrity and authenticity.
*
*
*
* This value is available only if the build project's packaging
value is set to ZIP
.
*
*
*
* @return The SHA-256 hash of the build artifact.
*
* You can use this hash along with a checksum tool to confirm file integrity and authenticity.
*
*
*
* This value is available only if the build project's packaging
value is set to
* ZIP
.
*
*/
public final String sha256sum() {
return sha256sum;
}
/**
*
* The MD5 hash of the build artifact.
*
*
* You can use this hash along with a checksum tool to confirm file integrity and authenticity.
*
*
*
* This value is available only if the build project's packaging
value is set to ZIP
.
*
*
*
* @return The MD5 hash of the build artifact.
*
* You can use this hash along with a checksum tool to confirm file integrity and authenticity.
*
*
*
* This value is available only if the build project's packaging
value is set to
* ZIP
.
*
*/
public final String md5sum() {
return md5sum;
}
/**
*
* If this flag is set, a name specified in the buildspec file overrides the artifact name. The name specified in a
* buildspec file is calculated at build time and uses the Shell Command Language. For example, you can append a
* date and time to your artifact name so that it is always unique.
*
*
* @return If this flag is set, a name specified in the buildspec file overrides the artifact name. The name
* specified in a buildspec file is calculated at build time and uses the Shell Command Language. For
* example, you can append a date and time to your artifact name so that it is always unique.
*/
public final Boolean overrideArtifactName() {
return overrideArtifactName;
}
/**
*
* Information that tells you if encryption for build artifacts is disabled.
*
*
* @return Information that tells you if encryption for build artifacts is disabled.
*/
public final Boolean encryptionDisabled() {
return encryptionDisabled;
}
/**
*
* An identifier for this artifact definition.
*
*
* @return An identifier for this artifact definition.
*/
public final String artifactIdentifier() {
return artifactIdentifier;
}
/**
* Returns the value of the BucketOwnerAccess property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #bucketOwnerAccess}
* will return {@link BucketOwnerAccess#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #bucketOwnerAccessAsString}.
*
*
* @return The value of the BucketOwnerAccess property for this object.
* @see BucketOwnerAccess
*/
public final BucketOwnerAccess bucketOwnerAccess() {
return BucketOwnerAccess.fromValue(bucketOwnerAccess);
}
/**
* Returns the value of the BucketOwnerAccess property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #bucketOwnerAccess}
* will return {@link BucketOwnerAccess#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #bucketOwnerAccessAsString}.
*
*
* @return The value of the BucketOwnerAccess property for this object.
* @see BucketOwnerAccess
*/
public final String bucketOwnerAccessAsString() {
return bucketOwnerAccess;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(location());
hashCode = 31 * hashCode + Objects.hashCode(sha256sum());
hashCode = 31 * hashCode + Objects.hashCode(md5sum());
hashCode = 31 * hashCode + Objects.hashCode(overrideArtifactName());
hashCode = 31 * hashCode + Objects.hashCode(encryptionDisabled());
hashCode = 31 * hashCode + Objects.hashCode(artifactIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(bucketOwnerAccessAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BuildArtifacts)) {
return false;
}
BuildArtifacts other = (BuildArtifacts) obj;
return Objects.equals(location(), other.location()) && Objects.equals(sha256sum(), other.sha256sum())
&& Objects.equals(md5sum(), other.md5sum())
&& Objects.equals(overrideArtifactName(), other.overrideArtifactName())
&& Objects.equals(encryptionDisabled(), other.encryptionDisabled())
&& Objects.equals(artifactIdentifier(), other.artifactIdentifier())
&& Objects.equals(bucketOwnerAccessAsString(), other.bucketOwnerAccessAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BuildArtifacts").add("Location", location()).add("Sha256sum", sha256sum())
.add("Md5sum", md5sum()).add("OverrideArtifactName", overrideArtifactName())
.add("EncryptionDisabled", encryptionDisabled()).add("ArtifactIdentifier", artifactIdentifier())
.add("BucketOwnerAccess", bucketOwnerAccessAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "location":
return Optional.ofNullable(clazz.cast(location()));
case "sha256sum":
return Optional.ofNullable(clazz.cast(sha256sum()));
case "md5sum":
return Optional.ofNullable(clazz.cast(md5sum()));
case "overrideArtifactName":
return Optional.ofNullable(clazz.cast(overrideArtifactName()));
case "encryptionDisabled":
return Optional.ofNullable(clazz.cast(encryptionDisabled()));
case "artifactIdentifier":
return Optional.ofNullable(clazz.cast(artifactIdentifier()));
case "bucketOwnerAccess":
return Optional.ofNullable(clazz.cast(bucketOwnerAccessAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function