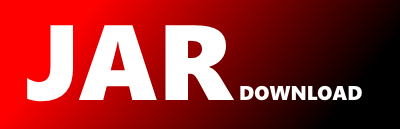
software.amazon.awssdk.services.codebuild.model.S3ReportExportConfig Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the S3 bucket where the raw data of a report are exported.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class S3ReportExportConfig implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BUCKET_FIELD = SdkField. builder(MarshallingType.STRING).memberName("bucket")
.getter(getter(S3ReportExportConfig::bucket)).setter(setter(Builder::bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("bucket").build()).build();
private static final SdkField BUCKET_OWNER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("bucketOwner").getter(getter(S3ReportExportConfig::bucketOwner)).setter(setter(Builder::bucketOwner))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("bucketOwner").build()).build();
private static final SdkField PATH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("path")
.getter(getter(S3ReportExportConfig::path)).setter(setter(Builder::path))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("path").build()).build();
private static final SdkField PACKAGING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("packaging").getter(getter(S3ReportExportConfig::packagingAsString)).setter(setter(Builder::packaging))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("packaging").build()).build();
private static final SdkField ENCRYPTION_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("encryptionKey").getter(getter(S3ReportExportConfig::encryptionKey))
.setter(setter(Builder::encryptionKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionKey").build()).build();
private static final SdkField ENCRYPTION_DISABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("encryptionDisabled").getter(getter(S3ReportExportConfig::encryptionDisabled))
.setter(setter(Builder::encryptionDisabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionDisabled").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_FIELD,
BUCKET_OWNER_FIELD, PATH_FIELD, PACKAGING_FIELD, ENCRYPTION_KEY_FIELD, ENCRYPTION_DISABLED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String bucket;
private final String bucketOwner;
private final String path;
private final String packaging;
private final String encryptionKey;
private final Boolean encryptionDisabled;
private S3ReportExportConfig(BuilderImpl builder) {
this.bucket = builder.bucket;
this.bucketOwner = builder.bucketOwner;
this.path = builder.path;
this.packaging = builder.packaging;
this.encryptionKey = builder.encryptionKey;
this.encryptionDisabled = builder.encryptionDisabled;
}
/**
*
* The name of the S3 bucket where the raw data of a report are exported.
*
*
* @return The name of the S3 bucket where the raw data of a report are exported.
*/
public final String bucket() {
return bucket;
}
/**
*
* The Amazon Web Services account identifier of the owner of the Amazon S3 bucket. This allows report data to be
* exported to an Amazon S3 bucket that is owned by an account other than the account running the build.
*
*
* @return The Amazon Web Services account identifier of the owner of the Amazon S3 bucket. This allows report data
* to be exported to an Amazon S3 bucket that is owned by an account other than the account running the
* build.
*/
public final String bucketOwner() {
return bucketOwner;
}
/**
*
* The path to the exported report's raw data results.
*
*
* @return The path to the exported report's raw data results.
*/
public final String path() {
return path;
}
/**
*
* The type of build output artifact to create. Valid values include:
*
*
* -
*
* NONE
: CodeBuild creates the raw data in the output bucket. This is the default if packaging is not
* specified.
*
*
* -
*
* ZIP
: CodeBuild creates a ZIP file with the raw data in the output bucket.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #packaging} will
* return {@link ReportPackagingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #packagingAsString}.
*
*
* @return The type of build output artifact to create. Valid values include:
*
* -
*
* NONE
: CodeBuild creates the raw data in the output bucket. This is the default if packaging
* is not specified.
*
*
* -
*
* ZIP
: CodeBuild creates a ZIP file with the raw data in the output bucket.
*
*
* @see ReportPackagingType
*/
public final ReportPackagingType packaging() {
return ReportPackagingType.fromValue(packaging);
}
/**
*
* The type of build output artifact to create. Valid values include:
*
*
* -
*
* NONE
: CodeBuild creates the raw data in the output bucket. This is the default if packaging is not
* specified.
*
*
* -
*
* ZIP
: CodeBuild creates a ZIP file with the raw data in the output bucket.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #packaging} will
* return {@link ReportPackagingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #packagingAsString}.
*
*
* @return The type of build output artifact to create. Valid values include:
*
* -
*
* NONE
: CodeBuild creates the raw data in the output bucket. This is the default if packaging
* is not specified.
*
*
* -
*
* ZIP
: CodeBuild creates a ZIP file with the raw data in the output bucket.
*
*
* @see ReportPackagingType
*/
public final String packagingAsString() {
return packaging;
}
/**
*
* The encryption key for the report's encrypted raw data.
*
*
* @return The encryption key for the report's encrypted raw data.
*/
public final String encryptionKey() {
return encryptionKey;
}
/**
*
* A boolean value that specifies if the results of a report are encrypted.
*
*
* @return A boolean value that specifies if the results of a report are encrypted.
*/
public final Boolean encryptionDisabled() {
return encryptionDisabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(bucket());
hashCode = 31 * hashCode + Objects.hashCode(bucketOwner());
hashCode = 31 * hashCode + Objects.hashCode(path());
hashCode = 31 * hashCode + Objects.hashCode(packagingAsString());
hashCode = 31 * hashCode + Objects.hashCode(encryptionKey());
hashCode = 31 * hashCode + Objects.hashCode(encryptionDisabled());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof S3ReportExportConfig)) {
return false;
}
S3ReportExportConfig other = (S3ReportExportConfig) obj;
return Objects.equals(bucket(), other.bucket()) && Objects.equals(bucketOwner(), other.bucketOwner())
&& Objects.equals(path(), other.path()) && Objects.equals(packagingAsString(), other.packagingAsString())
&& Objects.equals(encryptionKey(), other.encryptionKey())
&& Objects.equals(encryptionDisabled(), other.encryptionDisabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("S3ReportExportConfig").add("Bucket", bucket()).add("BucketOwner", bucketOwner())
.add("Path", path()).add("Packaging", packagingAsString()).add("EncryptionKey", encryptionKey())
.add("EncryptionDisabled", encryptionDisabled()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "bucket":
return Optional.ofNullable(clazz.cast(bucket()));
case "bucketOwner":
return Optional.ofNullable(clazz.cast(bucketOwner()));
case "path":
return Optional.ofNullable(clazz.cast(path()));
case "packaging":
return Optional.ofNullable(clazz.cast(packagingAsString()));
case "encryptionKey":
return Optional.ofNullable(clazz.cast(encryptionKey()));
case "encryptionDisabled":
return Optional.ofNullable(clazz.cast(encryptionDisabled()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("bucket", BUCKET_FIELD);
map.put("bucketOwner", BUCKET_OWNER_FIELD);
map.put("path", PATH_FIELD);
map.put("packaging", PACKAGING_FIELD);
map.put("encryptionKey", ENCRYPTION_KEY_FIELD);
map.put("encryptionDisabled", ENCRYPTION_DISABLED_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function