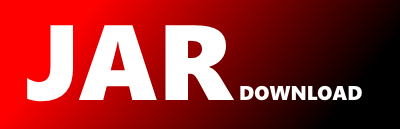
software.amazon.awssdk.services.codebuild.model.StartBuildBatchRequest Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartBuildBatchRequest extends CodeBuildRequest implements
ToCopyableBuilder {
private static final SdkField PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("projectName").getter(getter(StartBuildBatchRequest::projectName)).setter(setter(Builder::projectName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("projectName").build()).build();
private static final SdkField> SECONDARY_SOURCES_OVERRIDE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondarySourcesOverride")
.getter(getter(StartBuildBatchRequest::secondarySourcesOverride))
.setter(setter(Builder::secondarySourcesOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySourcesOverride").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SECONDARY_SOURCES_VERSION_OVERRIDE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondarySourcesVersionOverride")
.getter(getter(StartBuildBatchRequest::secondarySourcesVersionOverride))
.setter(setter(Builder::secondarySourcesVersionOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondarySourcesVersionOverride")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectSourceVersion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SOURCE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceVersion").getter(getter(StartBuildBatchRequest::sourceVersion))
.setter(setter(Builder::sourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceVersion").build()).build();
private static final SdkField ARTIFACTS_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("artifactsOverride")
.getter(getter(StartBuildBatchRequest::artifactsOverride)).setter(setter(Builder::artifactsOverride))
.constructor(ProjectArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("artifactsOverride").build()).build();
private static final SdkField> SECONDARY_ARTIFACTS_OVERRIDE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("secondaryArtifactsOverride")
.getter(getter(StartBuildBatchRequest::secondaryArtifactsOverride))
.setter(setter(Builder::secondaryArtifactsOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secondaryArtifactsOverride").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ProjectArtifacts::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ENVIRONMENT_VARIABLES_OVERRIDE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("environmentVariablesOverride")
.getter(getter(StartBuildBatchRequest::environmentVariablesOverride))
.setter(setter(Builder::environmentVariablesOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environmentVariablesOverride")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EnvironmentVariable::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SOURCE_TYPE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceTypeOverride").getter(getter(StartBuildBatchRequest::sourceTypeOverrideAsString))
.setter(setter(Builder::sourceTypeOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceTypeOverride").build())
.build();
private static final SdkField SOURCE_LOCATION_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceLocationOverride").getter(getter(StartBuildBatchRequest::sourceLocationOverride))
.setter(setter(Builder::sourceLocationOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceLocationOverride").build())
.build();
private static final SdkField SOURCE_AUTH_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("sourceAuthOverride")
.getter(getter(StartBuildBatchRequest::sourceAuthOverride)).setter(setter(Builder::sourceAuthOverride))
.constructor(SourceAuth::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceAuthOverride").build())
.build();
private static final SdkField GIT_CLONE_DEPTH_OVERRIDE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("gitCloneDepthOverride").getter(getter(StartBuildBatchRequest::gitCloneDepthOverride))
.setter(setter(Builder::gitCloneDepthOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("gitCloneDepthOverride").build())
.build();
private static final SdkField GIT_SUBMODULES_CONFIG_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("gitSubmodulesConfigOverride")
.getter(getter(StartBuildBatchRequest::gitSubmodulesConfigOverride))
.setter(setter(Builder::gitSubmodulesConfigOverride))
.constructor(GitSubmodulesConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("gitSubmodulesConfigOverride")
.build()).build();
private static final SdkField BUILDSPEC_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildspecOverride").getter(getter(StartBuildBatchRequest::buildspecOverride))
.setter(setter(Builder::buildspecOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildspecOverride").build()).build();
private static final SdkField INSECURE_SSL_OVERRIDE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("insecureSslOverride").getter(getter(StartBuildBatchRequest::insecureSslOverride))
.setter(setter(Builder::insecureSslOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("insecureSslOverride").build())
.build();
private static final SdkField REPORT_BUILD_BATCH_STATUS_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("reportBuildBatchStatusOverride")
.getter(getter(StartBuildBatchRequest::reportBuildBatchStatusOverride))
.setter(setter(Builder::reportBuildBatchStatusOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reportBuildBatchStatusOverride")
.build()).build();
private static final SdkField ENVIRONMENT_TYPE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("environmentTypeOverride").getter(getter(StartBuildBatchRequest::environmentTypeOverrideAsString))
.setter(setter(Builder::environmentTypeOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environmentTypeOverride").build())
.build();
private static final SdkField IMAGE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("imageOverride").getter(getter(StartBuildBatchRequest::imageOverride))
.setter(setter(Builder::imageOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageOverride").build()).build();
private static final SdkField COMPUTE_TYPE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("computeTypeOverride").getter(getter(StartBuildBatchRequest::computeTypeOverrideAsString))
.setter(setter(Builder::computeTypeOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("computeTypeOverride").build())
.build();
private static final SdkField CERTIFICATE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("certificateOverride").getter(getter(StartBuildBatchRequest::certificateOverride))
.setter(setter(Builder::certificateOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificateOverride").build())
.build();
private static final SdkField CACHE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("cacheOverride").getter(getter(StartBuildBatchRequest::cacheOverride))
.setter(setter(Builder::cacheOverride)).constructor(ProjectCache::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cacheOverride").build()).build();
private static final SdkField SERVICE_ROLE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceRoleOverride").getter(getter(StartBuildBatchRequest::serviceRoleOverride))
.setter(setter(Builder::serviceRoleOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRoleOverride").build())
.build();
private static final SdkField PRIVILEGED_MODE_OVERRIDE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("privilegedModeOverride").getter(getter(StartBuildBatchRequest::privilegedModeOverride))
.setter(setter(Builder::privilegedModeOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("privilegedModeOverride").build())
.build();
private static final SdkField BUILD_TIMEOUT_IN_MINUTES_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("buildTimeoutInMinutesOverride")
.getter(getter(StartBuildBatchRequest::buildTimeoutInMinutesOverride))
.setter(setter(Builder::buildTimeoutInMinutesOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildTimeoutInMinutesOverride")
.build()).build();
private static final SdkField QUEUED_TIMEOUT_IN_MINUTES_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("queuedTimeoutInMinutesOverride")
.getter(getter(StartBuildBatchRequest::queuedTimeoutInMinutesOverride))
.setter(setter(Builder::queuedTimeoutInMinutesOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("queuedTimeoutInMinutesOverride")
.build()).build();
private static final SdkField ENCRYPTION_KEY_OVERRIDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("encryptionKeyOverride").getter(getter(StartBuildBatchRequest::encryptionKeyOverride))
.setter(setter(Builder::encryptionKeyOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionKeyOverride").build())
.build();
private static final SdkField IDEMPOTENCY_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("idempotencyToken").getter(getter(StartBuildBatchRequest::idempotencyToken))
.setter(setter(Builder::idempotencyToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("idempotencyToken").build()).build();
private static final SdkField LOGS_CONFIG_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("logsConfigOverride")
.getter(getter(StartBuildBatchRequest::logsConfigOverride)).setter(setter(Builder::logsConfigOverride))
.constructor(LogsConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logsConfigOverride").build())
.build();
private static final SdkField REGISTRY_CREDENTIAL_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("registryCredentialOverride")
.getter(getter(StartBuildBatchRequest::registryCredentialOverride))
.setter(setter(Builder::registryCredentialOverride))
.constructor(RegistryCredential::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryCredentialOverride").build())
.build();
private static final SdkField IMAGE_PULL_CREDENTIALS_TYPE_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("imagePullCredentialsTypeOverride")
.getter(getter(StartBuildBatchRequest::imagePullCredentialsTypeOverrideAsString))
.setter(setter(Builder::imagePullCredentialsTypeOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imagePullCredentialsTypeOverride")
.build()).build();
private static final SdkField BUILD_BATCH_CONFIG_OVERRIDE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("buildBatchConfigOverride")
.getter(getter(StartBuildBatchRequest::buildBatchConfigOverride)).setter(setter(Builder::buildBatchConfigOverride))
.constructor(ProjectBuildBatchConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildBatchConfigOverride").build())
.build();
private static final SdkField DEBUG_SESSION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("debugSessionEnabled").getter(getter(StartBuildBatchRequest::debugSessionEnabled))
.setter(setter(Builder::debugSessionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("debugSessionEnabled").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PROJECT_NAME_FIELD,
SECONDARY_SOURCES_OVERRIDE_FIELD, SECONDARY_SOURCES_VERSION_OVERRIDE_FIELD, SOURCE_VERSION_FIELD,
ARTIFACTS_OVERRIDE_FIELD, SECONDARY_ARTIFACTS_OVERRIDE_FIELD, ENVIRONMENT_VARIABLES_OVERRIDE_FIELD,
SOURCE_TYPE_OVERRIDE_FIELD, SOURCE_LOCATION_OVERRIDE_FIELD, SOURCE_AUTH_OVERRIDE_FIELD,
GIT_CLONE_DEPTH_OVERRIDE_FIELD, GIT_SUBMODULES_CONFIG_OVERRIDE_FIELD, BUILDSPEC_OVERRIDE_FIELD,
INSECURE_SSL_OVERRIDE_FIELD, REPORT_BUILD_BATCH_STATUS_OVERRIDE_FIELD, ENVIRONMENT_TYPE_OVERRIDE_FIELD,
IMAGE_OVERRIDE_FIELD, COMPUTE_TYPE_OVERRIDE_FIELD, CERTIFICATE_OVERRIDE_FIELD, CACHE_OVERRIDE_FIELD,
SERVICE_ROLE_OVERRIDE_FIELD, PRIVILEGED_MODE_OVERRIDE_FIELD, BUILD_TIMEOUT_IN_MINUTES_OVERRIDE_FIELD,
QUEUED_TIMEOUT_IN_MINUTES_OVERRIDE_FIELD, ENCRYPTION_KEY_OVERRIDE_FIELD, IDEMPOTENCY_TOKEN_FIELD,
LOGS_CONFIG_OVERRIDE_FIELD, REGISTRY_CREDENTIAL_OVERRIDE_FIELD, IMAGE_PULL_CREDENTIALS_TYPE_OVERRIDE_FIELD,
BUILD_BATCH_CONFIG_OVERRIDE_FIELD, DEBUG_SESSION_ENABLED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String projectName;
private final List secondarySourcesOverride;
private final List secondarySourcesVersionOverride;
private final String sourceVersion;
private final ProjectArtifacts artifactsOverride;
private final List secondaryArtifactsOverride;
private final List environmentVariablesOverride;
private final String sourceTypeOverride;
private final String sourceLocationOverride;
private final SourceAuth sourceAuthOverride;
private final Integer gitCloneDepthOverride;
private final GitSubmodulesConfig gitSubmodulesConfigOverride;
private final String buildspecOverride;
private final Boolean insecureSslOverride;
private final Boolean reportBuildBatchStatusOverride;
private final String environmentTypeOverride;
private final String imageOverride;
private final String computeTypeOverride;
private final String certificateOverride;
private final ProjectCache cacheOverride;
private final String serviceRoleOverride;
private final Boolean privilegedModeOverride;
private final Integer buildTimeoutInMinutesOverride;
private final Integer queuedTimeoutInMinutesOverride;
private final String encryptionKeyOverride;
private final String idempotencyToken;
private final LogsConfig logsConfigOverride;
private final RegistryCredential registryCredentialOverride;
private final String imagePullCredentialsTypeOverride;
private final ProjectBuildBatchConfig buildBatchConfigOverride;
private final Boolean debugSessionEnabled;
private StartBuildBatchRequest(BuilderImpl builder) {
super(builder);
this.projectName = builder.projectName;
this.secondarySourcesOverride = builder.secondarySourcesOverride;
this.secondarySourcesVersionOverride = builder.secondarySourcesVersionOverride;
this.sourceVersion = builder.sourceVersion;
this.artifactsOverride = builder.artifactsOverride;
this.secondaryArtifactsOverride = builder.secondaryArtifactsOverride;
this.environmentVariablesOverride = builder.environmentVariablesOverride;
this.sourceTypeOverride = builder.sourceTypeOverride;
this.sourceLocationOverride = builder.sourceLocationOverride;
this.sourceAuthOverride = builder.sourceAuthOverride;
this.gitCloneDepthOverride = builder.gitCloneDepthOverride;
this.gitSubmodulesConfigOverride = builder.gitSubmodulesConfigOverride;
this.buildspecOverride = builder.buildspecOverride;
this.insecureSslOverride = builder.insecureSslOverride;
this.reportBuildBatchStatusOverride = builder.reportBuildBatchStatusOverride;
this.environmentTypeOverride = builder.environmentTypeOverride;
this.imageOverride = builder.imageOverride;
this.computeTypeOverride = builder.computeTypeOverride;
this.certificateOverride = builder.certificateOverride;
this.cacheOverride = builder.cacheOverride;
this.serviceRoleOverride = builder.serviceRoleOverride;
this.privilegedModeOverride = builder.privilegedModeOverride;
this.buildTimeoutInMinutesOverride = builder.buildTimeoutInMinutesOverride;
this.queuedTimeoutInMinutesOverride = builder.queuedTimeoutInMinutesOverride;
this.encryptionKeyOverride = builder.encryptionKeyOverride;
this.idempotencyToken = builder.idempotencyToken;
this.logsConfigOverride = builder.logsConfigOverride;
this.registryCredentialOverride = builder.registryCredentialOverride;
this.imagePullCredentialsTypeOverride = builder.imagePullCredentialsTypeOverride;
this.buildBatchConfigOverride = builder.buildBatchConfigOverride;
this.debugSessionEnabled = builder.debugSessionEnabled;
}
/**
*
* The name of the project.
*
*
* @return The name of the project.
*/
public final String projectName() {
return projectName;
}
/**
* For responses, this returns true if the service returned a value for the SecondarySourcesOverride property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasSecondarySourcesOverride() {
return secondarySourcesOverride != null && !(secondarySourcesOverride instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSource
objects that override the secondary sources defined in the batch build
* project.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondarySourcesOverride} method.
*
*
* @return An array of ProjectSource
objects that override the secondary sources defined in the batch
* build project.
*/
public final List secondarySourcesOverride() {
return secondarySourcesOverride;
}
/**
* For responses, this returns true if the service returned a value for the SecondarySourcesVersionOverride
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasSecondarySourcesVersionOverride() {
return secondarySourcesVersionOverride != null && !(secondarySourcesVersionOverride instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectSourceVersion
objects that override the secondary source versions in the batch
* build project.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondarySourcesVersionOverride}
* method.
*
*
* @return An array of ProjectSourceVersion
objects that override the secondary source versions in the
* batch build project.
*/
public final List secondarySourcesVersionOverride() {
return secondarySourcesVersionOverride;
}
/**
*
* The version of the batch build input to be built, for this build only. If not specified, the latest version is
* used. If specified, the contents depends on the source provider:
*
*
* - CodeCommit
* -
*
* The commit ID, branch, or Git tag to use.
*
*
* - GitHub
* -
*
* The commit ID, pull request ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a pull request ID is specified, it must use the format pr/pull-request-ID
(for
* example pr/25
). If a branch name is specified, the branch's HEAD commit ID is used. If not
* specified, the default branch's HEAD commit ID is used.
*
*
* - Bitbucket
* -
*
* The commit ID, branch name, or tag name that corresponds to the version of the source code you want to build. If
* a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default branch's HEAD
* commit ID is used.
*
*
* - Amazon S3
* -
*
* The version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* If sourceVersion
is specified at the project level, then this sourceVersion
(at the
* build level) takes precedence.
*
*
* For more information, see Source Version Sample
* with CodeBuild in the CodeBuild User Guide.
*
*
* @return The version of the batch build input to be built, for this build only. If not specified, the latest
* version is used. If specified, the contents depends on the source provider:
*
* - CodeCommit
* -
*
* The commit ID, branch, or Git tag to use.
*
*
* - GitHub
* -
*
* The commit ID, pull request ID, branch name, or tag name that corresponds to the version of the source
* code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* - Bitbucket
* -
*
* The commit ID, branch name, or tag name that corresponds to the version of the source code you want to
* build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default
* branch's HEAD commit ID is used.
*
*
* - Amazon S3
* -
*
* The version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* If sourceVersion
is specified at the project level, then this sourceVersion
(at
* the build level) takes precedence.
*
*
* For more information, see Source Version
* Sample with CodeBuild in the CodeBuild User Guide.
*/
public final String sourceVersion() {
return sourceVersion;
}
/**
*
* An array of ProjectArtifacts
objects that contains information about the build output artifact
* overrides for the build project.
*
*
* @return An array of ProjectArtifacts
objects that contains information about the build output
* artifact overrides for the build project.
*/
public final ProjectArtifacts artifactsOverride() {
return artifactsOverride;
}
/**
* For responses, this returns true if the service returned a value for the SecondaryArtifactsOverride property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasSecondaryArtifactsOverride() {
return secondaryArtifactsOverride != null && !(secondaryArtifactsOverride instanceof SdkAutoConstructList);
}
/**
*
* An array of ProjectArtifacts
objects that override the secondary artifacts defined in the batch
* build project.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecondaryArtifactsOverride} method.
*
*
* @return An array of ProjectArtifacts
objects that override the secondary artifacts defined in the
* batch build project.
*/
public final List secondaryArtifactsOverride() {
return secondaryArtifactsOverride;
}
/**
* For responses, this returns true if the service returned a value for the EnvironmentVariablesOverride property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasEnvironmentVariablesOverride() {
return environmentVariablesOverride != null && !(environmentVariablesOverride instanceof SdkAutoConstructList);
}
/**
*
* An array of EnvironmentVariable
objects that override, or add to, the environment variables defined
* in the batch build project.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEnvironmentVariablesOverride} method.
*
*
* @return An array of EnvironmentVariable
objects that override, or add to, the environment variables
* defined in the batch build project.
*/
public final List environmentVariablesOverride() {
return environmentVariablesOverride;
}
/**
*
* The source input type that overrides the source input defined in the batch build project.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #sourceTypeOverride} will return {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #sourceTypeOverrideAsString}.
*
*
* @return The source input type that overrides the source input defined in the batch build project.
* @see SourceType
*/
public final SourceType sourceTypeOverride() {
return SourceType.fromValue(sourceTypeOverride);
}
/**
*
* The source input type that overrides the source input defined in the batch build project.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #sourceTypeOverride} will return {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #sourceTypeOverrideAsString}.
*
*
* @return The source input type that overrides the source input defined in the batch build project.
* @see SourceType
*/
public final String sourceTypeOverrideAsString() {
return sourceTypeOverride;
}
/**
*
* A location that overrides, for this batch build, the source location defined in the batch build project.
*
*
* @return A location that overrides, for this batch build, the source location defined in the batch build project.
*/
public final String sourceLocationOverride() {
return sourceLocationOverride;
}
/**
*
* A SourceAuth
object that overrides the one defined in the batch build project. This override applies
* only if the build project's source is BitBucket or GitHub.
*
*
* @return A SourceAuth
object that overrides the one defined in the batch build project. This override
* applies only if the build project's source is BitBucket or GitHub.
*/
public final SourceAuth sourceAuthOverride() {
return sourceAuthOverride;
}
/**
*
* The user-defined depth of history, with a minimum value of 0, that overrides, for this batch build only, any
* previous depth of history defined in the batch build project.
*
*
* @return The user-defined depth of history, with a minimum value of 0, that overrides, for this batch build only,
* any previous depth of history defined in the batch build project.
*/
public final Integer gitCloneDepthOverride() {
return gitCloneDepthOverride;
}
/**
*
* A GitSubmodulesConfig
object that overrides the Git submodules configuration for this batch build.
*
*
* @return A GitSubmodulesConfig
object that overrides the Git submodules configuration for this batch
* build.
*/
public final GitSubmodulesConfig gitSubmodulesConfigOverride() {
return gitSubmodulesConfigOverride;
}
/**
*
* A buildspec file declaration that overrides, for this build only, the latest one already defined in the build
* project.
*
*
* If this value is set, it can be either an inline buildspec definition, the path to an alternate buildspec file
* relative to the value of the built-in CODEBUILD_SRC_DIR
environment variable, or the path to an S3
* bucket. The bucket must be in the same Amazon Web Services Region as the build project. Specify the buildspec
* file using its ARN (for example, arn:aws:s3:::my-codebuild-sample2/buildspec.yml
). If this value is
* not provided or is set to an empty string, the source code must contain a buildspec file in its root directory.
* For more information, see Buildspec File Name and Storage Location.
*
*
* @return A buildspec file declaration that overrides, for this build only, the latest one already defined in the
* build project.
*
* If this value is set, it can be either an inline buildspec definition, the path to an alternate buildspec
* file relative to the value of the built-in CODEBUILD_SRC_DIR
environment variable, or the
* path to an S3 bucket. The bucket must be in the same Amazon Web Services Region as the build project.
* Specify the buildspec file using its ARN (for example,
* arn:aws:s3:::my-codebuild-sample2/buildspec.yml
). If this value is not provided or is set to
* an empty string, the source code must contain a buildspec file in its root directory. For more
* information, see Buildspec File Name and Storage Location.
*/
public final String buildspecOverride() {
return buildspecOverride;
}
/**
*
* Enable this flag to override the insecure SSL setting that is specified in the batch build project. The insecure
* SSL setting determines whether to ignore SSL warnings while connecting to the project source code. This override
* applies only if the build's source is GitHub Enterprise.
*
*
* @return Enable this flag to override the insecure SSL setting that is specified in the batch build project. The
* insecure SSL setting determines whether to ignore SSL warnings while connecting to the project source
* code. This override applies only if the build's source is GitHub Enterprise.
*/
public final Boolean insecureSslOverride() {
return insecureSslOverride;
}
/**
*
* Set to true
to report to your source provider the status of a batch build's start and completion. If
* you use this option with a source provider other than GitHub, GitHub Enterprise, or Bitbucket, an
* invalidInputException
is thrown.
*
*
*
* The status of a build triggered by a webhook is always reported to your source provider.
*
*
*
* @return Set to true
to report to your source provider the status of a batch build's start and
* completion. If you use this option with a source provider other than GitHub, GitHub Enterprise, or
* Bitbucket, an invalidInputException
is thrown.
*
* The status of a build triggered by a webhook is always reported to your source provider.
*
*/
public final Boolean reportBuildBatchStatusOverride() {
return reportBuildBatchStatusOverride;
}
/**
*
* A container type for this batch build that overrides the one specified in the batch build project.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #environmentTypeOverride} will return {@link EnvironmentType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #environmentTypeOverrideAsString}.
*
*
* @return A container type for this batch build that overrides the one specified in the batch build project.
* @see EnvironmentType
*/
public final EnvironmentType environmentTypeOverride() {
return EnvironmentType.fromValue(environmentTypeOverride);
}
/**
*
* A container type for this batch build that overrides the one specified in the batch build project.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #environmentTypeOverride} will return {@link EnvironmentType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #environmentTypeOverrideAsString}.
*
*
* @return A container type for this batch build that overrides the one specified in the batch build project.
* @see EnvironmentType
*/
public final String environmentTypeOverrideAsString() {
return environmentTypeOverride;
}
/**
*
* The name of an image for this batch build that overrides the one specified in the batch build project.
*
*
* @return The name of an image for this batch build that overrides the one specified in the batch build project.
*/
public final String imageOverride() {
return imageOverride;
}
/**
*
* The name of a compute type for this batch build that overrides the one specified in the batch build project.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #computeTypeOverride} will return {@link ComputeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #computeTypeOverrideAsString}.
*
*
* @return The name of a compute type for this batch build that overrides the one specified in the batch build
* project.
* @see ComputeType
*/
public final ComputeType computeTypeOverride() {
return ComputeType.fromValue(computeTypeOverride);
}
/**
*
* The name of a compute type for this batch build that overrides the one specified in the batch build project.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #computeTypeOverride} will return {@link ComputeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #computeTypeOverrideAsString}.
*
*
* @return The name of a compute type for this batch build that overrides the one specified in the batch build
* project.
* @see ComputeType
*/
public final String computeTypeOverrideAsString() {
return computeTypeOverride;
}
/**
*
* The name of a certificate for this batch build that overrides the one specified in the batch build project.
*
*
* @return The name of a certificate for this batch build that overrides the one specified in the batch build
* project.
*/
public final String certificateOverride() {
return certificateOverride;
}
/**
*
* A ProjectCache
object that specifies cache overrides.
*
*
* @return A ProjectCache
object that specifies cache overrides.
*/
public final ProjectCache cacheOverride() {
return cacheOverride;
}
/**
*
* The name of a service role for this batch build that overrides the one specified in the batch build project.
*
*
* @return The name of a service role for this batch build that overrides the one specified in the batch build
* project.
*/
public final String serviceRoleOverride() {
return serviceRoleOverride;
}
/**
*
* Enable this flag to override privileged mode in the batch build project.
*
*
* @return Enable this flag to override privileged mode in the batch build project.
*/
public final Boolean privilegedModeOverride() {
return privilegedModeOverride;
}
/**
*
* Overrides the build timeout specified in the batch build project.
*
*
* @return Overrides the build timeout specified in the batch build project.
*/
public final Integer buildTimeoutInMinutesOverride() {
return buildTimeoutInMinutesOverride;
}
/**
*
* The number of minutes a batch build is allowed to be queued before it times out.
*
*
* @return The number of minutes a batch build is allowed to be queued before it times out.
*/
public final Integer queuedTimeoutInMinutesOverride() {
return queuedTimeoutInMinutesOverride;
}
/**
*
* The Key Management Service customer master key (CMK) that overrides the one specified in the batch build project.
* The CMK key encrypts the build output artifacts.
*
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission to
* that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the
* format alias/<alias-name>
).
*
*
* @return The Key Management Service customer master key (CMK) that overrides the one specified in the batch build
* project. The CMK key encrypts the build output artifacts.
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has
* permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using
* the format alias/<alias-name>
).
*/
public final String encryptionKeyOverride() {
return encryptionKeyOverride;
}
/**
*
* A unique, case sensitive identifier you provide to ensure the idempotency of the StartBuildBatch
* request. The token is included in the StartBuildBatch
request and is valid for five minutes. If you
* repeat the StartBuildBatch
request with the same token, but change a parameter, CodeBuild returns a
* parameter mismatch error.
*
*
* @return A unique, case sensitive identifier you provide to ensure the idempotency of the
* StartBuildBatch
request. The token is included in the StartBuildBatch
request
* and is valid for five minutes. If you repeat the StartBuildBatch
request with the same
* token, but change a parameter, CodeBuild returns a parameter mismatch error.
*/
public final String idempotencyToken() {
return idempotencyToken;
}
/**
*
* A LogsConfig
object that override the log settings defined in the batch build project.
*
*
* @return A LogsConfig
object that override the log settings defined in the batch build project.
*/
public final LogsConfig logsConfigOverride() {
return logsConfigOverride;
}
/**
*
* A RegistryCredential
object that overrides credentials for access to a private registry.
*
*
* @return A RegistryCredential
object that overrides credentials for access to a private registry.
*/
public final RegistryCredential registryCredentialOverride() {
return registryCredentialOverride;
}
/**
*
* The type of credentials CodeBuild uses to pull images in your batch build. There are two valid values:
*
*
* - CODEBUILD
* -
*
* Specifies that CodeBuild uses its own credentials. This requires that you modify your ECR repository policy to
* trust CodeBuild's service principal.
*
*
* - SERVICE_ROLE
* -
*
* Specifies that CodeBuild uses your build project's service role.
*
*
*
*
* When using a cross-account or private registry image, you must use SERVICE_ROLE
credentials. When
* using an CodeBuild curated image, you must use CODEBUILD
credentials.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imagePullCredentialsTypeOverride} will return {@link ImagePullCredentialsType#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #imagePullCredentialsTypeOverrideAsString}.
*
*
* @return The type of credentials CodeBuild uses to pull images in your batch build. There are two valid values:
*
*
* - CODEBUILD
* -
*
* Specifies that CodeBuild uses its own credentials. This requires that you modify your ECR repository
* policy to trust CodeBuild's service principal.
*
*
* - SERVICE_ROLE
* -
*
* Specifies that CodeBuild uses your build project's service role.
*
*
*
*
* When using a cross-account or private registry image, you must use SERVICE_ROLE
credentials.
* When using an CodeBuild curated image, you must use CODEBUILD
credentials.
* @see ImagePullCredentialsType
*/
public final ImagePullCredentialsType imagePullCredentialsTypeOverride() {
return ImagePullCredentialsType.fromValue(imagePullCredentialsTypeOverride);
}
/**
*
* The type of credentials CodeBuild uses to pull images in your batch build. There are two valid values:
*
*
* - CODEBUILD
* -
*
* Specifies that CodeBuild uses its own credentials. This requires that you modify your ECR repository policy to
* trust CodeBuild's service principal.
*
*
* - SERVICE_ROLE
* -
*
* Specifies that CodeBuild uses your build project's service role.
*
*
*
*
* When using a cross-account or private registry image, you must use SERVICE_ROLE
credentials. When
* using an CodeBuild curated image, you must use CODEBUILD
credentials.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imagePullCredentialsTypeOverride} will return {@link ImagePullCredentialsType#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #imagePullCredentialsTypeOverrideAsString}.
*
*
* @return The type of credentials CodeBuild uses to pull images in your batch build. There are two valid values:
*
*
* - CODEBUILD
* -
*
* Specifies that CodeBuild uses its own credentials. This requires that you modify your ECR repository
* policy to trust CodeBuild's service principal.
*
*
* - SERVICE_ROLE
* -
*
* Specifies that CodeBuild uses your build project's service role.
*
*
*
*
* When using a cross-account or private registry image, you must use SERVICE_ROLE
credentials.
* When using an CodeBuild curated image, you must use CODEBUILD
credentials.
* @see ImagePullCredentialsType
*/
public final String imagePullCredentialsTypeOverrideAsString() {
return imagePullCredentialsTypeOverride;
}
/**
*
* A BuildBatchConfigOverride
object that contains batch build configuration overrides.
*
*
* @return A BuildBatchConfigOverride
object that contains batch build configuration overrides.
*/
public final ProjectBuildBatchConfig buildBatchConfigOverride() {
return buildBatchConfigOverride;
}
/**
*
* Specifies if session debugging is enabled for this batch build. For more information, see Viewing a running build in
* Session Manager. Batch session debugging is not supported for matrix batch builds.
*
*
* @return Specifies if session debugging is enabled for this batch build. For more information, see Viewing a running
* build in Session Manager. Batch session debugging is not supported for matrix batch builds.
*/
public final Boolean debugSessionEnabled() {
return debugSessionEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(projectName());
hashCode = 31 * hashCode + Objects.hashCode(hasSecondarySourcesOverride() ? secondarySourcesOverride() : null);
hashCode = 31 * hashCode
+ Objects.hashCode(hasSecondarySourcesVersionOverride() ? secondarySourcesVersionOverride() : null);
hashCode = 31 * hashCode + Objects.hashCode(sourceVersion());
hashCode = 31 * hashCode + Objects.hashCode(artifactsOverride());
hashCode = 31 * hashCode + Objects.hashCode(hasSecondaryArtifactsOverride() ? secondaryArtifactsOverride() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasEnvironmentVariablesOverride() ? environmentVariablesOverride() : null);
hashCode = 31 * hashCode + Objects.hashCode(sourceTypeOverrideAsString());
hashCode = 31 * hashCode + Objects.hashCode(sourceLocationOverride());
hashCode = 31 * hashCode + Objects.hashCode(sourceAuthOverride());
hashCode = 31 * hashCode + Objects.hashCode(gitCloneDepthOverride());
hashCode = 31 * hashCode + Objects.hashCode(gitSubmodulesConfigOverride());
hashCode = 31 * hashCode + Objects.hashCode(buildspecOverride());
hashCode = 31 * hashCode + Objects.hashCode(insecureSslOverride());
hashCode = 31 * hashCode + Objects.hashCode(reportBuildBatchStatusOverride());
hashCode = 31 * hashCode + Objects.hashCode(environmentTypeOverrideAsString());
hashCode = 31 * hashCode + Objects.hashCode(imageOverride());
hashCode = 31 * hashCode + Objects.hashCode(computeTypeOverrideAsString());
hashCode = 31 * hashCode + Objects.hashCode(certificateOverride());
hashCode = 31 * hashCode + Objects.hashCode(cacheOverride());
hashCode = 31 * hashCode + Objects.hashCode(serviceRoleOverride());
hashCode = 31 * hashCode + Objects.hashCode(privilegedModeOverride());
hashCode = 31 * hashCode + Objects.hashCode(buildTimeoutInMinutesOverride());
hashCode = 31 * hashCode + Objects.hashCode(queuedTimeoutInMinutesOverride());
hashCode = 31 * hashCode + Objects.hashCode(encryptionKeyOverride());
hashCode = 31 * hashCode + Objects.hashCode(idempotencyToken());
hashCode = 31 * hashCode + Objects.hashCode(logsConfigOverride());
hashCode = 31 * hashCode + Objects.hashCode(registryCredentialOverride());
hashCode = 31 * hashCode + Objects.hashCode(imagePullCredentialsTypeOverrideAsString());
hashCode = 31 * hashCode + Objects.hashCode(buildBatchConfigOverride());
hashCode = 31 * hashCode + Objects.hashCode(debugSessionEnabled());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartBuildBatchRequest)) {
return false;
}
StartBuildBatchRequest other = (StartBuildBatchRequest) obj;
return Objects.equals(projectName(), other.projectName())
&& hasSecondarySourcesOverride() == other.hasSecondarySourcesOverride()
&& Objects.equals(secondarySourcesOverride(), other.secondarySourcesOverride())
&& hasSecondarySourcesVersionOverride() == other.hasSecondarySourcesVersionOverride()
&& Objects.equals(secondarySourcesVersionOverride(), other.secondarySourcesVersionOverride())
&& Objects.equals(sourceVersion(), other.sourceVersion())
&& Objects.equals(artifactsOverride(), other.artifactsOverride())
&& hasSecondaryArtifactsOverride() == other.hasSecondaryArtifactsOverride()
&& Objects.equals(secondaryArtifactsOverride(), other.secondaryArtifactsOverride())
&& hasEnvironmentVariablesOverride() == other.hasEnvironmentVariablesOverride()
&& Objects.equals(environmentVariablesOverride(), other.environmentVariablesOverride())
&& Objects.equals(sourceTypeOverrideAsString(), other.sourceTypeOverrideAsString())
&& Objects.equals(sourceLocationOverride(), other.sourceLocationOverride())
&& Objects.equals(sourceAuthOverride(), other.sourceAuthOverride())
&& Objects.equals(gitCloneDepthOverride(), other.gitCloneDepthOverride())
&& Objects.equals(gitSubmodulesConfigOverride(), other.gitSubmodulesConfigOverride())
&& Objects.equals(buildspecOverride(), other.buildspecOverride())
&& Objects.equals(insecureSslOverride(), other.insecureSslOverride())
&& Objects.equals(reportBuildBatchStatusOverride(), other.reportBuildBatchStatusOverride())
&& Objects.equals(environmentTypeOverrideAsString(), other.environmentTypeOverrideAsString())
&& Objects.equals(imageOverride(), other.imageOverride())
&& Objects.equals(computeTypeOverrideAsString(), other.computeTypeOverrideAsString())
&& Objects.equals(certificateOverride(), other.certificateOverride())
&& Objects.equals(cacheOverride(), other.cacheOverride())
&& Objects.equals(serviceRoleOverride(), other.serviceRoleOverride())
&& Objects.equals(privilegedModeOverride(), other.privilegedModeOverride())
&& Objects.equals(buildTimeoutInMinutesOverride(), other.buildTimeoutInMinutesOverride())
&& Objects.equals(queuedTimeoutInMinutesOverride(), other.queuedTimeoutInMinutesOverride())
&& Objects.equals(encryptionKeyOverride(), other.encryptionKeyOverride())
&& Objects.equals(idempotencyToken(), other.idempotencyToken())
&& Objects.equals(logsConfigOverride(), other.logsConfigOverride())
&& Objects.equals(registryCredentialOverride(), other.registryCredentialOverride())
&& Objects.equals(imagePullCredentialsTypeOverrideAsString(), other.imagePullCredentialsTypeOverrideAsString())
&& Objects.equals(buildBatchConfigOverride(), other.buildBatchConfigOverride())
&& Objects.equals(debugSessionEnabled(), other.debugSessionEnabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("StartBuildBatchRequest")
.add("ProjectName", projectName())
.add("SecondarySourcesOverride", hasSecondarySourcesOverride() ? secondarySourcesOverride() : null)
.add("SecondarySourcesVersionOverride",
hasSecondarySourcesVersionOverride() ? secondarySourcesVersionOverride() : null)
.add("SourceVersion", sourceVersion()).add("ArtifactsOverride", artifactsOverride())
.add("SecondaryArtifactsOverride", hasSecondaryArtifactsOverride() ? secondaryArtifactsOverride() : null)
.add("EnvironmentVariablesOverride", hasEnvironmentVariablesOverride() ? environmentVariablesOverride() : null)
.add("SourceTypeOverride", sourceTypeOverrideAsString()).add("SourceLocationOverride", sourceLocationOverride())
.add("SourceAuthOverride", sourceAuthOverride()).add("GitCloneDepthOverride", gitCloneDepthOverride())
.add("GitSubmodulesConfigOverride", gitSubmodulesConfigOverride()).add("BuildspecOverride", buildspecOverride())
.add("InsecureSslOverride", insecureSslOverride())
.add("ReportBuildBatchStatusOverride", reportBuildBatchStatusOverride())
.add("EnvironmentTypeOverride", environmentTypeOverrideAsString()).add("ImageOverride", imageOverride())
.add("ComputeTypeOverride", computeTypeOverrideAsString()).add("CertificateOverride", certificateOverride())
.add("CacheOverride", cacheOverride()).add("ServiceRoleOverride", serviceRoleOverride())
.add("PrivilegedModeOverride", privilegedModeOverride())
.add("BuildTimeoutInMinutesOverride", buildTimeoutInMinutesOverride())
.add("QueuedTimeoutInMinutesOverride", queuedTimeoutInMinutesOverride())
.add("EncryptionKeyOverride", encryptionKeyOverride()).add("IdempotencyToken", idempotencyToken())
.add("LogsConfigOverride", logsConfigOverride()).add("RegistryCredentialOverride", registryCredentialOverride())
.add("ImagePullCredentialsTypeOverride", imagePullCredentialsTypeOverrideAsString())
.add("BuildBatchConfigOverride", buildBatchConfigOverride()).add("DebugSessionEnabled", debugSessionEnabled())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "projectName":
return Optional.ofNullable(clazz.cast(projectName()));
case "secondarySourcesOverride":
return Optional.ofNullable(clazz.cast(secondarySourcesOverride()));
case "secondarySourcesVersionOverride":
return Optional.ofNullable(clazz.cast(secondarySourcesVersionOverride()));
case "sourceVersion":
return Optional.ofNullable(clazz.cast(sourceVersion()));
case "artifactsOverride":
return Optional.ofNullable(clazz.cast(artifactsOverride()));
case "secondaryArtifactsOverride":
return Optional.ofNullable(clazz.cast(secondaryArtifactsOverride()));
case "environmentVariablesOverride":
return Optional.ofNullable(clazz.cast(environmentVariablesOverride()));
case "sourceTypeOverride":
return Optional.ofNullable(clazz.cast(sourceTypeOverrideAsString()));
case "sourceLocationOverride":
return Optional.ofNullable(clazz.cast(sourceLocationOverride()));
case "sourceAuthOverride":
return Optional.ofNullable(clazz.cast(sourceAuthOverride()));
case "gitCloneDepthOverride":
return Optional.ofNullable(clazz.cast(gitCloneDepthOverride()));
case "gitSubmodulesConfigOverride":
return Optional.ofNullable(clazz.cast(gitSubmodulesConfigOverride()));
case "buildspecOverride":
return Optional.ofNullable(clazz.cast(buildspecOverride()));
case "insecureSslOverride":
return Optional.ofNullable(clazz.cast(insecureSslOverride()));
case "reportBuildBatchStatusOverride":
return Optional.ofNullable(clazz.cast(reportBuildBatchStatusOverride()));
case "environmentTypeOverride":
return Optional.ofNullable(clazz.cast(environmentTypeOverrideAsString()));
case "imageOverride":
return Optional.ofNullable(clazz.cast(imageOverride()));
case "computeTypeOverride":
return Optional.ofNullable(clazz.cast(computeTypeOverrideAsString()));
case "certificateOverride":
return Optional.ofNullable(clazz.cast(certificateOverride()));
case "cacheOverride":
return Optional.ofNullable(clazz.cast(cacheOverride()));
case "serviceRoleOverride":
return Optional.ofNullable(clazz.cast(serviceRoleOverride()));
case "privilegedModeOverride":
return Optional.ofNullable(clazz.cast(privilegedModeOverride()));
case "buildTimeoutInMinutesOverride":
return Optional.ofNullable(clazz.cast(buildTimeoutInMinutesOverride()));
case "queuedTimeoutInMinutesOverride":
return Optional.ofNullable(clazz.cast(queuedTimeoutInMinutesOverride()));
case "encryptionKeyOverride":
return Optional.ofNullable(clazz.cast(encryptionKeyOverride()));
case "idempotencyToken":
return Optional.ofNullable(clazz.cast(idempotencyToken()));
case "logsConfigOverride":
return Optional.ofNullable(clazz.cast(logsConfigOverride()));
case "registryCredentialOverride":
return Optional.ofNullable(clazz.cast(registryCredentialOverride()));
case "imagePullCredentialsTypeOverride":
return Optional.ofNullable(clazz.cast(imagePullCredentialsTypeOverrideAsString()));
case "buildBatchConfigOverride":
return Optional.ofNullable(clazz.cast(buildBatchConfigOverride()));
case "debugSessionEnabled":
return Optional.ofNullable(clazz.cast(debugSessionEnabled()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("projectName", PROJECT_NAME_FIELD);
map.put("secondarySourcesOverride", SECONDARY_SOURCES_OVERRIDE_FIELD);
map.put("secondarySourcesVersionOverride", SECONDARY_SOURCES_VERSION_OVERRIDE_FIELD);
map.put("sourceVersion", SOURCE_VERSION_FIELD);
map.put("artifactsOverride", ARTIFACTS_OVERRIDE_FIELD);
map.put("secondaryArtifactsOverride", SECONDARY_ARTIFACTS_OVERRIDE_FIELD);
map.put("environmentVariablesOverride", ENVIRONMENT_VARIABLES_OVERRIDE_FIELD);
map.put("sourceTypeOverride", SOURCE_TYPE_OVERRIDE_FIELD);
map.put("sourceLocationOverride", SOURCE_LOCATION_OVERRIDE_FIELD);
map.put("sourceAuthOverride", SOURCE_AUTH_OVERRIDE_FIELD);
map.put("gitCloneDepthOverride", GIT_CLONE_DEPTH_OVERRIDE_FIELD);
map.put("gitSubmodulesConfigOverride", GIT_SUBMODULES_CONFIG_OVERRIDE_FIELD);
map.put("buildspecOverride", BUILDSPEC_OVERRIDE_FIELD);
map.put("insecureSslOverride", INSECURE_SSL_OVERRIDE_FIELD);
map.put("reportBuildBatchStatusOverride", REPORT_BUILD_BATCH_STATUS_OVERRIDE_FIELD);
map.put("environmentTypeOverride", ENVIRONMENT_TYPE_OVERRIDE_FIELD);
map.put("imageOverride", IMAGE_OVERRIDE_FIELD);
map.put("computeTypeOverride", COMPUTE_TYPE_OVERRIDE_FIELD);
map.put("certificateOverride", CERTIFICATE_OVERRIDE_FIELD);
map.put("cacheOverride", CACHE_OVERRIDE_FIELD);
map.put("serviceRoleOverride", SERVICE_ROLE_OVERRIDE_FIELD);
map.put("privilegedModeOverride", PRIVILEGED_MODE_OVERRIDE_FIELD);
map.put("buildTimeoutInMinutesOverride", BUILD_TIMEOUT_IN_MINUTES_OVERRIDE_FIELD);
map.put("queuedTimeoutInMinutesOverride", QUEUED_TIMEOUT_IN_MINUTES_OVERRIDE_FIELD);
map.put("encryptionKeyOverride", ENCRYPTION_KEY_OVERRIDE_FIELD);
map.put("idempotencyToken", IDEMPOTENCY_TOKEN_FIELD);
map.put("logsConfigOverride", LOGS_CONFIG_OVERRIDE_FIELD);
map.put("registryCredentialOverride", REGISTRY_CREDENTIAL_OVERRIDE_FIELD);
map.put("imagePullCredentialsTypeOverride", IMAGE_PULL_CREDENTIALS_TYPE_OVERRIDE_FIELD);
map.put("buildBatchConfigOverride", BUILD_BATCH_CONFIG_OVERRIDE_FIELD);
map.put("debugSessionEnabled", DEBUG_SESSION_ENABLED_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function