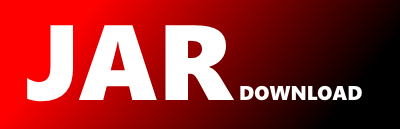
software.amazon.awssdk.services.codebuild.model.Webhook Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a webhook that connects repository events to a build project in CodeBuild.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Webhook implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField URL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("url")
.getter(getter(Webhook::url)).setter(setter(Builder::url))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("url").build()).build();
private static final SdkField PAYLOAD_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("payloadUrl").getter(getter(Webhook::payloadUrl)).setter(setter(Builder::payloadUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("payloadUrl").build()).build();
private static final SdkField SECRET_FIELD = SdkField. builder(MarshallingType.STRING).memberName("secret")
.getter(getter(Webhook::secret)).setter(setter(Builder::secret))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secret").build()).build();
private static final SdkField BRANCH_FILTER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("branchFilter").getter(getter(Webhook::branchFilter)).setter(setter(Builder::branchFilter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("branchFilter").build()).build();
private static final SdkField>> FILTER_GROUPS_FIELD = SdkField
.>> builder(MarshallingType.LIST)
.memberName("filterGroups")
.getter(getter(Webhook::filterGroups))
.setter(setter(Builder::filterGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("filterGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField.> builder(MarshallingType.LIST)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(WebhookFilter::builder)
.traits(LocationTrait.builder()
.location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build())
.build()).build()).build()).build();
private static final SdkField BUILD_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildType").getter(getter(Webhook::buildTypeAsString)).setter(setter(Builder::buildType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildType").build()).build();
private static final SdkField MANUAL_CREATION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("manualCreation").getter(getter(Webhook::manualCreation)).setter(setter(Builder::manualCreation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("manualCreation").build()).build();
private static final SdkField LAST_MODIFIED_SECRET_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastModifiedSecret").getter(getter(Webhook::lastModifiedSecret))
.setter(setter(Builder::lastModifiedSecret))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastModifiedSecret").build())
.build();
private static final SdkField SCOPE_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("scopeConfiguration")
.getter(getter(Webhook::scopeConfiguration)).setter(setter(Builder::scopeConfiguration))
.constructor(ScopeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scopeConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(URL_FIELD, PAYLOAD_URL_FIELD,
SECRET_FIELD, BRANCH_FILTER_FIELD, FILTER_GROUPS_FIELD, BUILD_TYPE_FIELD, MANUAL_CREATION_FIELD,
LAST_MODIFIED_SECRET_FIELD, SCOPE_CONFIGURATION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String url;
private final String payloadUrl;
private final String secret;
private final String branchFilter;
private final List> filterGroups;
private final String buildType;
private final Boolean manualCreation;
private final Instant lastModifiedSecret;
private final ScopeConfiguration scopeConfiguration;
private Webhook(BuilderImpl builder) {
this.url = builder.url;
this.payloadUrl = builder.payloadUrl;
this.secret = builder.secret;
this.branchFilter = builder.branchFilter;
this.filterGroups = builder.filterGroups;
this.buildType = builder.buildType;
this.manualCreation = builder.manualCreation;
this.lastModifiedSecret = builder.lastModifiedSecret;
this.scopeConfiguration = builder.scopeConfiguration;
}
/**
*
* The URL to the webhook.
*
*
* @return The URL to the webhook.
*/
public final String url() {
return url;
}
/**
*
* The CodeBuild endpoint where webhook events are sent.
*
*
* @return The CodeBuild endpoint where webhook events are sent.
*/
public final String payloadUrl() {
return payloadUrl;
}
/**
*
* The secret token of the associated repository.
*
*
*
* A Bitbucket webhook does not support secret
.
*
*
*
* @return The secret token of the associated repository.
*
* A Bitbucket webhook does not support secret
.
*
*/
public final String secret() {
return secret;
}
/**
*
* A regular expression used to determine which repository branches are built when a webhook is triggered. If the
* name of a branch matches the regular expression, then it is built. If branchFilter
is empty, then
* all branches are built.
*
*
*
* It is recommended that you use filterGroups
instead of branchFilter
.
*
*
*
* @return A regular expression used to determine which repository branches are built when a webhook is triggered.
* If the name of a branch matches the regular expression, then it is built. If branchFilter
is
* empty, then all branches are built.
*
* It is recommended that you use filterGroups
instead of branchFilter
.
*
*/
public final String branchFilter() {
return branchFilter;
}
/**
* For responses, this returns true if the service returned a value for the FilterGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasFilterGroups() {
return filterGroups != null && !(filterGroups instanceof SdkAutoConstructList);
}
/**
*
* An array of arrays of WebhookFilter
objects used to determine which webhooks are triggered. At least
* one WebhookFilter
in the array must specify EVENT
as its type
.
*
*
* For a build to be triggered, at least one filter group in the filterGroups
array must pass. For a
* filter group to pass, each of its filters must pass.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFilterGroups} method.
*
*
* @return An array of arrays of WebhookFilter
objects used to determine which webhooks are triggered.
* At least one WebhookFilter
in the array must specify EVENT
as its
* type
.
*
* For a build to be triggered, at least one filter group in the filterGroups
array must pass.
* For a filter group to pass, each of its filters must pass.
*/
public final List> filterGroups() {
return filterGroups;
}
/**
*
* Specifies the type of build this webhook will trigger.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildType} will
* return {@link WebhookBuildType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildTypeAsString}.
*
*
* @return Specifies the type of build this webhook will trigger.
* @see WebhookBuildType
*/
public final WebhookBuildType buildType() {
return WebhookBuildType.fromValue(buildType);
}
/**
*
* Specifies the type of build this webhook will trigger.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #buildType} will
* return {@link WebhookBuildType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #buildTypeAsString}.
*
*
* @return Specifies the type of build this webhook will trigger.
* @see WebhookBuildType
*/
public final String buildTypeAsString() {
return buildType;
}
/**
*
* If manualCreation is true, CodeBuild doesn't create a webhook in GitHub and instead returns
* payloadUrl
and secret
values for the webhook. The payloadUrl
and
* secret
values in the output can be used to manually create a webhook within GitHub.
*
*
*
* manualCreation is only available for GitHub webhooks.
*
*
*
* @return If manualCreation is true, CodeBuild doesn't create a webhook in GitHub and instead returns
* payloadUrl
and secret
values for the webhook. The payloadUrl
and
* secret
values in the output can be used to manually create a webhook within GitHub.
*
*
* manualCreation is only available for GitHub webhooks.
*
*/
public final Boolean manualCreation() {
return manualCreation;
}
/**
*
* A timestamp that indicates the last time a repository's secret token was modified.
*
*
* @return A timestamp that indicates the last time a repository's secret token was modified.
*/
public final Instant lastModifiedSecret() {
return lastModifiedSecret;
}
/**
*
* The scope configuration for global or organization webhooks.
*
*
*
* Global or organization webhooks are only available for GitHub and Github Enterprise webhooks.
*
*
*
* @return The scope configuration for global or organization webhooks.
*
* Global or organization webhooks are only available for GitHub and Github Enterprise webhooks.
*
*/
public final ScopeConfiguration scopeConfiguration() {
return scopeConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(url());
hashCode = 31 * hashCode + Objects.hashCode(payloadUrl());
hashCode = 31 * hashCode + Objects.hashCode(secret());
hashCode = 31 * hashCode + Objects.hashCode(branchFilter());
hashCode = 31 * hashCode + Objects.hashCode(hasFilterGroups() ? filterGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(buildTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(manualCreation());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedSecret());
hashCode = 31 * hashCode + Objects.hashCode(scopeConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Webhook)) {
return false;
}
Webhook other = (Webhook) obj;
return Objects.equals(url(), other.url()) && Objects.equals(payloadUrl(), other.payloadUrl())
&& Objects.equals(secret(), other.secret()) && Objects.equals(branchFilter(), other.branchFilter())
&& hasFilterGroups() == other.hasFilterGroups() && Objects.equals(filterGroups(), other.filterGroups())
&& Objects.equals(buildTypeAsString(), other.buildTypeAsString())
&& Objects.equals(manualCreation(), other.manualCreation())
&& Objects.equals(lastModifiedSecret(), other.lastModifiedSecret())
&& Objects.equals(scopeConfiguration(), other.scopeConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Webhook").add("Url", url()).add("PayloadUrl", payloadUrl()).add("Secret", secret())
.add("BranchFilter", branchFilter()).add("FilterGroups", hasFilterGroups() ? filterGroups() : null)
.add("BuildType", buildTypeAsString()).add("ManualCreation", manualCreation())
.add("LastModifiedSecret", lastModifiedSecret()).add("ScopeConfiguration", scopeConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "url":
return Optional.ofNullable(clazz.cast(url()));
case "payloadUrl":
return Optional.ofNullable(clazz.cast(payloadUrl()));
case "secret":
return Optional.ofNullable(clazz.cast(secret()));
case "branchFilter":
return Optional.ofNullable(clazz.cast(branchFilter()));
case "filterGroups":
return Optional.ofNullable(clazz.cast(filterGroups()));
case "buildType":
return Optional.ofNullable(clazz.cast(buildTypeAsString()));
case "manualCreation":
return Optional.ofNullable(clazz.cast(manualCreation()));
case "lastModifiedSecret":
return Optional.ofNullable(clazz.cast(lastModifiedSecret()));
case "scopeConfiguration":
return Optional.ofNullable(clazz.cast(scopeConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("url", URL_FIELD);
map.put("payloadUrl", PAYLOAD_URL_FIELD);
map.put("secret", SECRET_FIELD);
map.put("branchFilter", BRANCH_FILTER_FIELD);
map.put("filterGroups", FILTER_GROUPS_FIELD);
map.put("buildType", BUILD_TYPE_FIELD);
map.put("manualCreation", MANUAL_CREATION_FIELD);
map.put("lastModifiedSecret", LAST_MODIFIED_SECRET_FIELD);
map.put("scopeConfiguration", SCOPE_CONFIGURATION_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function