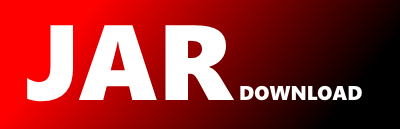
software.amazon.awssdk.services.codebuild.model.ProjectSourceVersion Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codebuild.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A source identifier and its corresponding version.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProjectSourceVersion implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SOURCE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceIdentifier").getter(getter(ProjectSourceVersion::sourceIdentifier))
.setter(setter(Builder::sourceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceIdentifier").build()).build();
private static final SdkField SOURCE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceVersion").getter(getter(ProjectSourceVersion::sourceVersion))
.setter(setter(Builder::sourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceVersion").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SOURCE_IDENTIFIER_FIELD,
SOURCE_VERSION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String sourceIdentifier;
private final String sourceVersion;
private ProjectSourceVersion(BuilderImpl builder) {
this.sourceIdentifier = builder.sourceIdentifier;
this.sourceVersion = builder.sourceVersion;
}
/**
*
* An identifier for a source in the build project. The identifier can only contain alphanumeric characters and
* underscores, and must be less than 128 characters in length.
*
*
* @return An identifier for a source in the build project. The identifier can only contain alphanumeric characters
* and underscores, and must be less than 128 characters in length.
*/
public final String sourceIdentifier() {
return sourceIdentifier;
}
/**
*
* The source version for the corresponding source identifier. If specified, must be one of:
*
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the
* source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the branch's
* HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For GitLab: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you
* want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default
* branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* For more information, see Source Version Sample
* with CodeBuild in the CodeBuild User Guide.
*
*
* @return The source version for the corresponding source identifier. If specified, must be one of:
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of
* the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For GitLab: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code
* you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified,
* the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* For more information, see Source Version
* Sample with CodeBuild in the CodeBuild User Guide.
*/
public final String sourceVersion() {
return sourceVersion;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(sourceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(sourceVersion());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProjectSourceVersion)) {
return false;
}
ProjectSourceVersion other = (ProjectSourceVersion) obj;
return Objects.equals(sourceIdentifier(), other.sourceIdentifier())
&& Objects.equals(sourceVersion(), other.sourceVersion());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProjectSourceVersion").add("SourceIdentifier", sourceIdentifier())
.add("SourceVersion", sourceVersion()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "sourceIdentifier":
return Optional.ofNullable(clazz.cast(sourceIdentifier()));
case "sourceVersion":
return Optional.ofNullable(clazz.cast(sourceVersion()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("sourceIdentifier", SOURCE_IDENTIFIER_FIELD);
map.put("sourceVersion", SOURCE_VERSION_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* -
*
* For CodeCommit: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version
* of the source code you want to build. If a pull request ID is specified, it must use the format
* pr/pull-request-ID
(for example, pr/25
). If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For GitLab: the commit ID, branch, or Git tag to use.
*
*
* -
*
* For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source
* code you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not
* specified, the default branch's HEAD commit ID is used.
*
*
* -
*
* For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
*
*
* For more information, see Source
* Version Sample with CodeBuild in the CodeBuild User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceVersion(String sourceVersion);
}
static final class BuilderImpl implements Builder {
private String sourceIdentifier;
private String sourceVersion;
private BuilderImpl() {
}
private BuilderImpl(ProjectSourceVersion model) {
sourceIdentifier(model.sourceIdentifier);
sourceVersion(model.sourceVersion);
}
public final String getSourceIdentifier() {
return sourceIdentifier;
}
public final void setSourceIdentifier(String sourceIdentifier) {
this.sourceIdentifier = sourceIdentifier;
}
@Override
public final Builder sourceIdentifier(String sourceIdentifier) {
this.sourceIdentifier = sourceIdentifier;
return this;
}
public final String getSourceVersion() {
return sourceVersion;
}
public final void setSourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
}
@Override
public final Builder sourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
return this;
}
@Override
public ProjectSourceVersion build() {
return new ProjectSourceVersion(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}