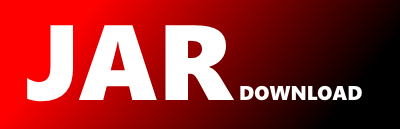
software.amazon.awssdk.services.codedeploy.DefaultCodeDeployClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codedeploy;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.codedeploy.internal.CodeDeployServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.codedeploy.model.AddTagsToOnPremisesInstancesRequest;
import software.amazon.awssdk.services.codedeploy.model.AddTagsToOnPremisesInstancesResponse;
import software.amazon.awssdk.services.codedeploy.model.AlarmsLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.ApplicationAlreadyExistsException;
import software.amazon.awssdk.services.codedeploy.model.ApplicationDoesNotExistException;
import software.amazon.awssdk.services.codedeploy.model.ApplicationLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.ApplicationNameRequiredException;
import software.amazon.awssdk.services.codedeploy.model.ArnNotSupportedException;
import software.amazon.awssdk.services.codedeploy.model.BatchGetApplicationRevisionsRequest;
import software.amazon.awssdk.services.codedeploy.model.BatchGetApplicationRevisionsResponse;
import software.amazon.awssdk.services.codedeploy.model.BatchGetApplicationsRequest;
import software.amazon.awssdk.services.codedeploy.model.BatchGetApplicationsResponse;
import software.amazon.awssdk.services.codedeploy.model.BatchGetDeploymentGroupsRequest;
import software.amazon.awssdk.services.codedeploy.model.BatchGetDeploymentGroupsResponse;
import software.amazon.awssdk.services.codedeploy.model.BatchGetDeploymentTargetsRequest;
import software.amazon.awssdk.services.codedeploy.model.BatchGetDeploymentTargetsResponse;
import software.amazon.awssdk.services.codedeploy.model.BatchGetDeploymentsRequest;
import software.amazon.awssdk.services.codedeploy.model.BatchGetDeploymentsResponse;
import software.amazon.awssdk.services.codedeploy.model.BatchGetOnPremisesInstancesRequest;
import software.amazon.awssdk.services.codedeploy.model.BatchGetOnPremisesInstancesResponse;
import software.amazon.awssdk.services.codedeploy.model.BatchLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.BucketNameFilterRequiredException;
import software.amazon.awssdk.services.codedeploy.model.CodeDeployException;
import software.amazon.awssdk.services.codedeploy.model.ContinueDeploymentRequest;
import software.amazon.awssdk.services.codedeploy.model.ContinueDeploymentResponse;
import software.amazon.awssdk.services.codedeploy.model.CreateApplicationRequest;
import software.amazon.awssdk.services.codedeploy.model.CreateApplicationResponse;
import software.amazon.awssdk.services.codedeploy.model.CreateDeploymentConfigRequest;
import software.amazon.awssdk.services.codedeploy.model.CreateDeploymentConfigResponse;
import software.amazon.awssdk.services.codedeploy.model.CreateDeploymentGroupRequest;
import software.amazon.awssdk.services.codedeploy.model.CreateDeploymentGroupResponse;
import software.amazon.awssdk.services.codedeploy.model.CreateDeploymentRequest;
import software.amazon.awssdk.services.codedeploy.model.CreateDeploymentResponse;
import software.amazon.awssdk.services.codedeploy.model.DeleteApplicationRequest;
import software.amazon.awssdk.services.codedeploy.model.DeleteApplicationResponse;
import software.amazon.awssdk.services.codedeploy.model.DeleteDeploymentConfigRequest;
import software.amazon.awssdk.services.codedeploy.model.DeleteDeploymentConfigResponse;
import software.amazon.awssdk.services.codedeploy.model.DeleteDeploymentGroupRequest;
import software.amazon.awssdk.services.codedeploy.model.DeleteDeploymentGroupResponse;
import software.amazon.awssdk.services.codedeploy.model.DeleteGitHubAccountTokenRequest;
import software.amazon.awssdk.services.codedeploy.model.DeleteGitHubAccountTokenResponse;
import software.amazon.awssdk.services.codedeploy.model.DeleteResourcesByExternalIdRequest;
import software.amazon.awssdk.services.codedeploy.model.DeleteResourcesByExternalIdResponse;
import software.amazon.awssdk.services.codedeploy.model.DeploymentAlreadyCompletedException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentConfigAlreadyExistsException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentConfigDoesNotExistException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentConfigInUseException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentConfigLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentConfigNameRequiredException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentDoesNotExistException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentGroupAlreadyExistsException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentGroupDoesNotExistException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentGroupLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentGroupNameRequiredException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentIdRequiredException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentIsNotInReadyStateException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentNotStartedException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentTargetDoesNotExistException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentTargetIdRequiredException;
import software.amazon.awssdk.services.codedeploy.model.DeploymentTargetListSizeExceededException;
import software.amazon.awssdk.services.codedeploy.model.DeregisterOnPremisesInstanceRequest;
import software.amazon.awssdk.services.codedeploy.model.DeregisterOnPremisesInstanceResponse;
import software.amazon.awssdk.services.codedeploy.model.DescriptionTooLongException;
import software.amazon.awssdk.services.codedeploy.model.EcsServiceMappingLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.GetApplicationRequest;
import software.amazon.awssdk.services.codedeploy.model.GetApplicationResponse;
import software.amazon.awssdk.services.codedeploy.model.GetApplicationRevisionRequest;
import software.amazon.awssdk.services.codedeploy.model.GetApplicationRevisionResponse;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentConfigRequest;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentConfigResponse;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentGroupRequest;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentGroupResponse;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentRequest;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentResponse;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentTargetRequest;
import software.amazon.awssdk.services.codedeploy.model.GetDeploymentTargetResponse;
import software.amazon.awssdk.services.codedeploy.model.GetOnPremisesInstanceRequest;
import software.amazon.awssdk.services.codedeploy.model.GetOnPremisesInstanceResponse;
import software.amazon.awssdk.services.codedeploy.model.GitHubAccountTokenDoesNotExistException;
import software.amazon.awssdk.services.codedeploy.model.GitHubAccountTokenNameRequiredException;
import software.amazon.awssdk.services.codedeploy.model.IamArnRequiredException;
import software.amazon.awssdk.services.codedeploy.model.IamSessionArnAlreadyRegisteredException;
import software.amazon.awssdk.services.codedeploy.model.IamUserArnAlreadyRegisteredException;
import software.amazon.awssdk.services.codedeploy.model.IamUserArnRequiredException;
import software.amazon.awssdk.services.codedeploy.model.InstanceLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.InstanceNameAlreadyRegisteredException;
import software.amazon.awssdk.services.codedeploy.model.InstanceNameRequiredException;
import software.amazon.awssdk.services.codedeploy.model.InstanceNotRegisteredException;
import software.amazon.awssdk.services.codedeploy.model.InvalidAlarmConfigException;
import software.amazon.awssdk.services.codedeploy.model.InvalidApplicationNameException;
import software.amazon.awssdk.services.codedeploy.model.InvalidArnException;
import software.amazon.awssdk.services.codedeploy.model.InvalidAutoRollbackConfigException;
import software.amazon.awssdk.services.codedeploy.model.InvalidAutoScalingGroupException;
import software.amazon.awssdk.services.codedeploy.model.InvalidBlueGreenDeploymentConfigurationException;
import software.amazon.awssdk.services.codedeploy.model.InvalidBucketNameFilterException;
import software.amazon.awssdk.services.codedeploy.model.InvalidComputePlatformException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeployedStateFilterException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentConfigNameException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentGroupNameException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentIdException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentInstanceTypeException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentStatusException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentStyleException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentTargetIdException;
import software.amazon.awssdk.services.codedeploy.model.InvalidDeploymentWaitTypeException;
import software.amazon.awssdk.services.codedeploy.model.InvalidEc2TagCombinationException;
import software.amazon.awssdk.services.codedeploy.model.InvalidEc2TagException;
import software.amazon.awssdk.services.codedeploy.model.InvalidEcsServiceException;
import software.amazon.awssdk.services.codedeploy.model.InvalidExternalIdException;
import software.amazon.awssdk.services.codedeploy.model.InvalidFileExistsBehaviorException;
import software.amazon.awssdk.services.codedeploy.model.InvalidGitHubAccountTokenException;
import software.amazon.awssdk.services.codedeploy.model.InvalidGitHubAccountTokenNameException;
import software.amazon.awssdk.services.codedeploy.model.InvalidIamSessionArnException;
import software.amazon.awssdk.services.codedeploy.model.InvalidIamUserArnException;
import software.amazon.awssdk.services.codedeploy.model.InvalidIgnoreApplicationStopFailuresValueException;
import software.amazon.awssdk.services.codedeploy.model.InvalidInputException;
import software.amazon.awssdk.services.codedeploy.model.InvalidInstanceNameException;
import software.amazon.awssdk.services.codedeploy.model.InvalidInstanceStatusException;
import software.amazon.awssdk.services.codedeploy.model.InvalidInstanceTypeException;
import software.amazon.awssdk.services.codedeploy.model.InvalidKeyPrefixFilterException;
import software.amazon.awssdk.services.codedeploy.model.InvalidLifecycleEventHookExecutionIdException;
import software.amazon.awssdk.services.codedeploy.model.InvalidLifecycleEventHookExecutionStatusException;
import software.amazon.awssdk.services.codedeploy.model.InvalidLoadBalancerInfoException;
import software.amazon.awssdk.services.codedeploy.model.InvalidMinimumHealthyHostValueException;
import software.amazon.awssdk.services.codedeploy.model.InvalidNextTokenException;
import software.amazon.awssdk.services.codedeploy.model.InvalidOnPremisesTagCombinationException;
import software.amazon.awssdk.services.codedeploy.model.InvalidOperationException;
import software.amazon.awssdk.services.codedeploy.model.InvalidRegistrationStatusException;
import software.amazon.awssdk.services.codedeploy.model.InvalidRevisionException;
import software.amazon.awssdk.services.codedeploy.model.InvalidRoleException;
import software.amazon.awssdk.services.codedeploy.model.InvalidSortByException;
import software.amazon.awssdk.services.codedeploy.model.InvalidSortOrderException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTagException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTagFilterException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTagsToAddException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTargetFilterNameException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTargetGroupPairException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTargetInstancesException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTimeRangeException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTrafficRoutingConfigurationException;
import software.amazon.awssdk.services.codedeploy.model.InvalidTriggerConfigException;
import software.amazon.awssdk.services.codedeploy.model.InvalidUpdateOutdatedInstancesOnlyValueException;
import software.amazon.awssdk.services.codedeploy.model.InvalidZonalDeploymentConfigurationException;
import software.amazon.awssdk.services.codedeploy.model.LifecycleEventAlreadyCompletedException;
import software.amazon.awssdk.services.codedeploy.model.LifecycleHookLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.ListApplicationRevisionsRequest;
import software.amazon.awssdk.services.codedeploy.model.ListApplicationRevisionsResponse;
import software.amazon.awssdk.services.codedeploy.model.ListApplicationsRequest;
import software.amazon.awssdk.services.codedeploy.model.ListApplicationsResponse;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentConfigsRequest;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentConfigsResponse;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentGroupsRequest;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentGroupsResponse;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentTargetsRequest;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentTargetsResponse;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentsRequest;
import software.amazon.awssdk.services.codedeploy.model.ListDeploymentsResponse;
import software.amazon.awssdk.services.codedeploy.model.ListGitHubAccountTokenNamesRequest;
import software.amazon.awssdk.services.codedeploy.model.ListGitHubAccountTokenNamesResponse;
import software.amazon.awssdk.services.codedeploy.model.ListOnPremisesInstancesRequest;
import software.amazon.awssdk.services.codedeploy.model.ListOnPremisesInstancesResponse;
import software.amazon.awssdk.services.codedeploy.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.codedeploy.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.codedeploy.model.MultipleIamArnsProvidedException;
import software.amazon.awssdk.services.codedeploy.model.OperationNotSupportedException;
import software.amazon.awssdk.services.codedeploy.model.PutLifecycleEventHookExecutionStatusRequest;
import software.amazon.awssdk.services.codedeploy.model.PutLifecycleEventHookExecutionStatusResponse;
import software.amazon.awssdk.services.codedeploy.model.RegisterApplicationRevisionRequest;
import software.amazon.awssdk.services.codedeploy.model.RegisterApplicationRevisionResponse;
import software.amazon.awssdk.services.codedeploy.model.RegisterOnPremisesInstanceRequest;
import software.amazon.awssdk.services.codedeploy.model.RegisterOnPremisesInstanceResponse;
import software.amazon.awssdk.services.codedeploy.model.RemoveTagsFromOnPremisesInstancesRequest;
import software.amazon.awssdk.services.codedeploy.model.RemoveTagsFromOnPremisesInstancesResponse;
import software.amazon.awssdk.services.codedeploy.model.ResourceArnRequiredException;
import software.amazon.awssdk.services.codedeploy.model.ResourceValidationException;
import software.amazon.awssdk.services.codedeploy.model.RevisionDoesNotExistException;
import software.amazon.awssdk.services.codedeploy.model.RevisionRequiredException;
import software.amazon.awssdk.services.codedeploy.model.RoleRequiredException;
import software.amazon.awssdk.services.codedeploy.model.StopDeploymentRequest;
import software.amazon.awssdk.services.codedeploy.model.StopDeploymentResponse;
import software.amazon.awssdk.services.codedeploy.model.TagLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.TagRequiredException;
import software.amazon.awssdk.services.codedeploy.model.TagResourceRequest;
import software.amazon.awssdk.services.codedeploy.model.TagResourceResponse;
import software.amazon.awssdk.services.codedeploy.model.TagSetListLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.ThrottlingException;
import software.amazon.awssdk.services.codedeploy.model.TriggerTargetsLimitExceededException;
import software.amazon.awssdk.services.codedeploy.model.UnsupportedActionForDeploymentTypeException;
import software.amazon.awssdk.services.codedeploy.model.UntagResourceRequest;
import software.amazon.awssdk.services.codedeploy.model.UntagResourceResponse;
import software.amazon.awssdk.services.codedeploy.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.codedeploy.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.codedeploy.model.UpdateDeploymentGroupRequest;
import software.amazon.awssdk.services.codedeploy.model.UpdateDeploymentGroupResponse;
import software.amazon.awssdk.services.codedeploy.transform.AddTagsToOnPremisesInstancesRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.BatchGetApplicationRevisionsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.BatchGetApplicationsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.BatchGetDeploymentGroupsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.BatchGetDeploymentTargetsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.BatchGetDeploymentsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.BatchGetOnPremisesInstancesRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ContinueDeploymentRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.CreateApplicationRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.CreateDeploymentConfigRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.CreateDeploymentGroupRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.CreateDeploymentRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.DeleteApplicationRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.DeleteDeploymentConfigRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.DeleteDeploymentGroupRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.DeleteGitHubAccountTokenRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.DeleteResourcesByExternalIdRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.DeregisterOnPremisesInstanceRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.GetApplicationRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.GetApplicationRevisionRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.GetDeploymentConfigRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.GetDeploymentGroupRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.GetDeploymentRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.GetDeploymentTargetRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.GetOnPremisesInstanceRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListApplicationRevisionsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListApplicationsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListDeploymentConfigsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListDeploymentGroupsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListDeploymentTargetsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListDeploymentsRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListGitHubAccountTokenNamesRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListOnPremisesInstancesRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.PutLifecycleEventHookExecutionStatusRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.RegisterApplicationRevisionRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.RegisterOnPremisesInstanceRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.RemoveTagsFromOnPremisesInstancesRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.StopDeploymentRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.UpdateApplicationRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.transform.UpdateDeploymentGroupRequestMarshaller;
import software.amazon.awssdk.services.codedeploy.waiters.CodeDeployWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link CodeDeployClient}.
*
* @see CodeDeployClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCodeDeployClient implements CodeDeployClient {
private static final Logger log = Logger.loggerFor(DefaultCodeDeployClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCodeDeployClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Adds tags to on-premises instances.
*
*
* @param addTagsToOnPremisesInstancesRequest
* Represents the input of, and adds tags to, an on-premises instance operation.
* @return Result of the AddTagsToOnPremisesInstances operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws TagLimitExceededException
* The maximum allowed number of tags was exceeded.
* @throws InstanceLimitExceededException
* The maximum number of allowed on-premises instances in a single call was exceeded.
* @throws InstanceNotRegisteredException
* The specified on-premises instance is not registered.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.AddTagsToOnPremisesInstances
* @see AWS API Documentation
*/
@Override
public AddTagsToOnPremisesInstancesResponse addTagsToOnPremisesInstances(
AddTagsToOnPremisesInstancesRequest addTagsToOnPremisesInstancesRequest) throws InstanceNameRequiredException,
InvalidInstanceNameException, TagRequiredException, InvalidTagException, TagLimitExceededException,
InstanceLimitExceededException, InstanceNotRegisteredException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddTagsToOnPremisesInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addTagsToOnPremisesInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addTagsToOnPremisesInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddTagsToOnPremisesInstances");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddTagsToOnPremisesInstances").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(addTagsToOnPremisesInstancesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddTagsToOnPremisesInstancesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about one or more application revisions. The maximum number of application revisions that can be
* returned is 25.
*
*
* @param batchGetApplicationRevisionsRequest
* Represents the input of a BatchGetApplicationRevisions
operation.
* @return Result of the BatchGetApplicationRevisions operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.BatchGetApplicationRevisions
* @see AWS API Documentation
*/
@Override
public BatchGetApplicationRevisionsResponse batchGetApplicationRevisions(
BatchGetApplicationRevisionsRequest batchGetApplicationRevisionsRequest) throws ApplicationDoesNotExistException,
ApplicationNameRequiredException, InvalidApplicationNameException, RevisionRequiredException,
InvalidRevisionException, BatchLimitExceededException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetApplicationRevisionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetApplicationRevisionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetApplicationRevisionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetApplicationRevisions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetApplicationRevisions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchGetApplicationRevisionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetApplicationRevisionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about one or more applications. The maximum number of applications that can be returned is 100.
*
*
* @param batchGetApplicationsRequest
* Represents the input of a BatchGetApplications
operation.
* @return Result of the BatchGetApplications operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.BatchGetApplications
* @see AWS API Documentation
*/
@Override
public BatchGetApplicationsResponse batchGetApplications(BatchGetApplicationsRequest batchGetApplicationsRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
BatchLimitExceededException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetApplicationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetApplicationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetApplicationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetApplications");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchGetApplications").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchGetApplicationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetApplicationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about one or more deployment groups.
*
*
* @param batchGetDeploymentGroupsRequest
* Represents the input of a BatchGetDeploymentGroups
operation.
* @return Result of the BatchGetDeploymentGroups operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.BatchGetDeploymentGroups
* @see AWS API Documentation
*/
@Override
public BatchGetDeploymentGroupsResponse batchGetDeploymentGroups(
BatchGetDeploymentGroupsRequest batchGetDeploymentGroupsRequest) throws ApplicationNameRequiredException,
InvalidApplicationNameException, ApplicationDoesNotExistException, DeploymentGroupNameRequiredException,
InvalidDeploymentGroupNameException, BatchLimitExceededException, DeploymentConfigDoesNotExistException,
AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetDeploymentGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetDeploymentGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetDeploymentGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetDeploymentGroups");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetDeploymentGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchGetDeploymentGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetDeploymentGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns an array of one or more targets associated with a deployment. This method works with all compute types
* and should be used instead of the deprecated BatchGetDeploymentInstances
. The maximum number of
* targets that can be returned is 25.
*
*
* The type of targets returned depends on the deployment's compute platform or deployment method:
*
*
* -
*
* EC2/On-premises: Information about Amazon EC2 instance targets.
*
*
* -
*
* Lambda: Information about Lambda functions targets.
*
*
* -
*
* Amazon ECS: Information about Amazon ECS service targets.
*
*
* -
*
* CloudFormation: Information about targets of blue/green deployments initiated by a CloudFormation stack
* update.
*
*
*
*
* @param batchGetDeploymentTargetsRequest
* @return Result of the BatchGetDeploymentTargets operation returned by the service.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws DeploymentTargetIdRequiredException
* A deployment target ID was not provided.
* @throws InvalidDeploymentTargetIdException
* The target ID provided was not valid.
* @throws DeploymentTargetDoesNotExistException
* The provided target ID does not belong to the attempted deployment.
* @throws DeploymentTargetListSizeExceededException
* The maximum number of targets that can be associated with an Amazon ECS or Lambda deployment was
* exceeded. The target list of both types of deployments must have exactly one item. This exception does
* not apply to EC2/On-premises deployments.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.BatchGetDeploymentTargets
* @see AWS API Documentation
*/
@Override
public BatchGetDeploymentTargetsResponse batchGetDeploymentTargets(
BatchGetDeploymentTargetsRequest batchGetDeploymentTargetsRequest) throws InvalidDeploymentIdException,
DeploymentIdRequiredException, DeploymentDoesNotExistException, DeploymentNotStartedException,
DeploymentTargetIdRequiredException, InvalidDeploymentTargetIdException, DeploymentTargetDoesNotExistException,
DeploymentTargetListSizeExceededException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetDeploymentTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetDeploymentTargetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetDeploymentTargetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetDeploymentTargets");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetDeploymentTargets").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchGetDeploymentTargetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetDeploymentTargetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about one or more deployments. The maximum number of deployments that can be returned is 25.
*
*
* @param batchGetDeploymentsRequest
* Represents the input of a BatchGetDeployments
operation.
* @return Result of the BatchGetDeployments operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.BatchGetDeployments
* @see AWS API Documentation
*/
@Override
public BatchGetDeploymentsResponse batchGetDeployments(BatchGetDeploymentsRequest batchGetDeploymentsRequest)
throws DeploymentIdRequiredException, InvalidDeploymentIdException, BatchLimitExceededException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetDeploymentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetDeploymentsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetDeploymentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetDeployments");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchGetDeployments").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchGetDeploymentsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetDeploymentsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about one or more on-premises instances. The maximum number of on-premises instances that can be
* returned is 25.
*
*
* @param batchGetOnPremisesInstancesRequest
* Represents the input of a BatchGetOnPremisesInstances
operation.
* @return Result of the BatchGetOnPremisesInstances operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.BatchGetOnPremisesInstances
* @see AWS API Documentation
*/
@Override
public BatchGetOnPremisesInstancesResponse batchGetOnPremisesInstances(
BatchGetOnPremisesInstancesRequest batchGetOnPremisesInstancesRequest) throws InstanceNameRequiredException,
InvalidInstanceNameException, BatchLimitExceededException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchGetOnPremisesInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchGetOnPremisesInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetOnPremisesInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetOnPremisesInstances");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchGetOnPremisesInstances").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchGetOnPremisesInstancesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetOnPremisesInstancesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* For a blue/green deployment, starts the process of rerouting traffic from instances in the original environment
* to instances in the replacement environment without waiting for a specified wait time to elapse. (Traffic
* rerouting, which is achieved by registering instances in the replacement environment with the load balancer, can
* start as soon as all instances have a status of Ready.)
*
*
* @param continueDeploymentRequest
* @return Result of the ContinueDeployment operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentAlreadyCompletedException
* The deployment is already complete.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentIsNotInReadyStateException
* The deployment does not have a status of Ready and can't continue yet.
* @throws UnsupportedActionForDeploymentTypeException
* A call was submitted that is not supported for the specified deployment type.
* @throws InvalidDeploymentWaitTypeException
* The wait type is invalid.
* @throws InvalidDeploymentStatusException
* The specified deployment status doesn't exist or cannot be determined.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ContinueDeployment
* @see AWS
* API Documentation
*/
@Override
public ContinueDeploymentResponse continueDeployment(ContinueDeploymentRequest continueDeploymentRequest)
throws DeploymentIdRequiredException, DeploymentDoesNotExistException, DeploymentAlreadyCompletedException,
InvalidDeploymentIdException, DeploymentIsNotInReadyStateException, UnsupportedActionForDeploymentTypeException,
InvalidDeploymentWaitTypeException, InvalidDeploymentStatusException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ContinueDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(continueDeploymentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, continueDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ContinueDeployment");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ContinueDeployment").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(continueDeploymentRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ContinueDeploymentRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* Represents the input of a CreateApplication
operation.
* @return Result of the CreateApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationAlreadyExistsException
* An application with the specified name with the user or Amazon Web Services account already exists.
* @throws ApplicationLimitExceededException
* More applications were attempted to be created than are allowed.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.CreateApplication
* @see AWS
* API Documentation
*/
@Override
public CreateApplicationResponse createApplication(CreateApplicationRequest createApplicationRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationAlreadyExistsException,
ApplicationLimitExceededException, InvalidComputePlatformException, InvalidTagsToAddException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateApplication").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createApplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateApplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deploys an application revision through the specified deployment group.
*
*
* @param createDeploymentRequest
* Represents the input of a CreateDeployment
operation.
* @return Result of the CreateDeployment operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws RevisionDoesNotExistException
* The named revision does not exist with the user or Amazon Web Services account.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws DescriptionTooLongException
* The description is too long.
* @throws DeploymentLimitExceededException
* The number of allowed deployments was exceeded.
* @throws InvalidTargetInstancesException
* The target instance configuration is invalid. Possible causes include:
*
* -
*
* Configuration data for target instances was entered for an in-place deployment.
*
*
* -
*
* The limit of 10 tags for a tag type was exceeded.
*
*
* -
*
* The combined length of the tag names exceeded the limit.
*
*
* -
*
* A specified tag is not currently applied to any instances.
*
*
* @throws InvalidAlarmConfigException
* The format of the alarm configuration is invalid. Possible causes include:
*
* -
*
* The alarm list is null.
*
*
* -
*
* The alarm object is null.
*
*
* -
*
* The alarm name is empty or null or exceeds the limit of 255 characters.
*
*
* -
*
* Two alarms with the same name have been specified.
*
*
* -
*
* The alarm configuration is enabled, but the alarm list is empty.
*
*
* @throws AlarmsLimitExceededException
* The maximum number of alarms for a deployment group (10) was exceeded.
* @throws InvalidAutoRollbackConfigException
* The automatic rollback configuration was specified in an invalid format. For example, automatic rollback
* is enabled, but an invalid triggering event type or no event types were listed.
* @throws InvalidLoadBalancerInfoException
* An invalid load balancer name, or no load balancer name, was specified.
* @throws InvalidFileExistsBehaviorException
* An invalid fileExistsBehavior option was specified to determine how CodeDeploy handles files or
* directories that already exist in a deployment target location, but weren't part of the previous
* successful deployment. Valid values include "DISALLOW," "OVERWRITE," and "RETAIN."
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws InvalidAutoScalingGroupException
* The Auto Scaling group was specified in an invalid format or does not exist.
* @throws ThrottlingException
* An API function was called too frequently.
* @throws InvalidUpdateOutdatedInstancesOnlyValueException
* The UpdateOutdatedInstancesOnly value is invalid. For Lambda deployments, false
is expected.
* For EC2/On-premises deployments, true
or false
is expected.
* @throws InvalidIgnoreApplicationStopFailuresValueException
* The IgnoreApplicationStopFailures value is invalid. For Lambda deployments, false
is
* expected. For EC2/On-premises deployments, true
or false
is expected.
* @throws InvalidGitHubAccountTokenException
* The GitHub token is not valid.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.CreateDeployment
* @see AWS
* API Documentation
*/
@Override
public CreateDeploymentResponse createDeployment(CreateDeploymentRequest createDeploymentRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
DeploymentGroupNameRequiredException, InvalidDeploymentGroupNameException, DeploymentGroupDoesNotExistException,
RevisionRequiredException, RevisionDoesNotExistException, InvalidRevisionException,
InvalidDeploymentConfigNameException, DeploymentConfigDoesNotExistException, DescriptionTooLongException,
DeploymentLimitExceededException, InvalidTargetInstancesException, InvalidAlarmConfigException,
AlarmsLimitExceededException, InvalidAutoRollbackConfigException, InvalidLoadBalancerInfoException,
InvalidFileExistsBehaviorException, InvalidRoleException, InvalidAutoScalingGroupException, ThrottlingException,
InvalidUpdateOutdatedInstancesOnlyValueException, InvalidIgnoreApplicationStopFailuresValueException,
InvalidGitHubAccountTokenException, InvalidTrafficRoutingConfigurationException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDeploymentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDeployment");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateDeployment").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createDeploymentRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateDeploymentRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a deployment configuration.
*
*
* @param createDeploymentConfigRequest
* Represents the input of a CreateDeploymentConfig
operation.
* @return Result of the CreateDeploymentConfig operation returned by the service.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigNameRequiredException
* The deployment configuration name was not specified.
* @throws DeploymentConfigAlreadyExistsException
* A deployment configuration with the specified name with the user or Amazon Web Services account already
* exists.
* @throws InvalidMinimumHealthyHostValueException
* The minimum healthy instance value was specified in an invalid format.
* @throws DeploymentConfigLimitExceededException
* The deployment configurations limit was exceeded.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @throws InvalidZonalDeploymentConfigurationException
* The ZonalConfig
object is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.CreateDeploymentConfig
* @see AWS API Documentation
*/
@Override
public CreateDeploymentConfigResponse createDeploymentConfig(CreateDeploymentConfigRequest createDeploymentConfigRequest)
throws InvalidDeploymentConfigNameException, DeploymentConfigNameRequiredException,
DeploymentConfigAlreadyExistsException, InvalidMinimumHealthyHostValueException,
DeploymentConfigLimitExceededException, InvalidComputePlatformException, InvalidTrafficRoutingConfigurationException,
InvalidZonalDeploymentConfigurationException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDeploymentConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDeploymentConfigRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDeploymentConfigRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDeploymentConfig");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDeploymentConfig").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createDeploymentConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateDeploymentConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a deployment group to which application revisions are deployed.
*
*
* @param createDeploymentGroupRequest
* Represents the input of a CreateDeploymentGroup
operation.
* @return Result of the CreateDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupAlreadyExistsException
* A deployment group with the specified name with the user or Amazon Web Services account already exists.
* @throws InvalidEc2TagException
* The tag was specified in an invalid format.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws InvalidAutoScalingGroupException
* The Auto Scaling group was specified in an invalid format or does not exist.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws RoleRequiredException
* The role ID was not specified.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws DeploymentGroupLimitExceededException
* The deployment groups limit was exceeded.
* @throws LifecycleHookLimitExceededException
* The limit for lifecycle hooks was exceeded.
* @throws InvalidTriggerConfigException
* The trigger was specified in an invalid format.
* @throws TriggerTargetsLimitExceededException
* The maximum allowed number of triggers was exceeded.
* @throws InvalidAlarmConfigException
* The format of the alarm configuration is invalid. Possible causes include:
*
* -
*
* The alarm list is null.
*
*
* -
*
* The alarm object is null.
*
*
* -
*
* The alarm name is empty or null or exceeds the limit of 255 characters.
*
*
* -
*
* Two alarms with the same name have been specified.
*
*
* -
*
* The alarm configuration is enabled, but the alarm list is empty.
*
*
* @throws AlarmsLimitExceededException
* The maximum number of alarms for a deployment group (10) was exceeded.
* @throws InvalidAutoRollbackConfigException
* The automatic rollback configuration was specified in an invalid format. For example, automatic rollback
* is enabled, but an invalid triggering event type or no event types were listed.
* @throws InvalidLoadBalancerInfoException
* An invalid load balancer name, or no load balancer name, was specified.
* @throws InvalidDeploymentStyleException
* An invalid deployment style was specified. Valid deployment types include "IN_PLACE" and "BLUE_GREEN."
* Valid deployment options include "WITH_TRAFFIC_CONTROL" and "WITHOUT_TRAFFIC_CONTROL."
* @throws InvalidBlueGreenDeploymentConfigurationException
* The configuration for the blue/green deployment group was provided in an invalid format. For information
* about deployment configuration format, see CreateDeploymentConfig.
* @throws InvalidEc2TagCombinationException
* A call was submitted that specified both Ec2TagFilters and Ec2TagSet, but only one of these data types
* can be used in a single call.
* @throws InvalidOnPremisesTagCombinationException
* A call was submitted that specified both OnPremisesTagFilters and OnPremisesTagSet, but only one of these
* data types can be used in a single call.
* @throws TagSetListLimitExceededException
* The number of tag groups included in the tag set list exceeded the maximum allowed limit of 3.
* @throws InvalidInputException
* The input was specified in an invalid format.
* @throws ThrottlingException
* An API function was called too frequently.
* @throws InvalidEcsServiceException
* The Amazon ECS service identifier is not valid.
* @throws InvalidTargetGroupPairException
* A target group pair associated with this deployment is not valid.
* @throws EcsServiceMappingLimitExceededException
* The Amazon ECS service is associated with more than one deployment groups. An Amazon ECS service can be
* associated with only one deployment group.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.CreateDeploymentGroup
* @see AWS API Documentation
*/
@Override
public CreateDeploymentGroupResponse createDeploymentGroup(CreateDeploymentGroupRequest createDeploymentGroupRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
DeploymentGroupNameRequiredException, InvalidDeploymentGroupNameException, DeploymentGroupAlreadyExistsException,
InvalidEc2TagException, InvalidTagException, InvalidAutoScalingGroupException, InvalidDeploymentConfigNameException,
DeploymentConfigDoesNotExistException, RoleRequiredException, InvalidRoleException,
DeploymentGroupLimitExceededException, LifecycleHookLimitExceededException, InvalidTriggerConfigException,
TriggerTargetsLimitExceededException, InvalidAlarmConfigException, AlarmsLimitExceededException,
InvalidAutoRollbackConfigException, InvalidLoadBalancerInfoException, InvalidDeploymentStyleException,
InvalidBlueGreenDeploymentConfigurationException, InvalidEc2TagCombinationException,
InvalidOnPremisesTagCombinationException, TagSetListLimitExceededException, InvalidInputException,
ThrottlingException, InvalidEcsServiceException, InvalidTargetGroupPairException,
EcsServiceMappingLimitExceededException, InvalidTagsToAddException, InvalidTrafficRoutingConfigurationException,
AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDeploymentGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDeploymentGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDeploymentGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDeploymentGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateDeploymentGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createDeploymentGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateDeploymentGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* Represents the input of a DeleteApplication
operation.
* @return Result of the DeleteApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.DeleteApplication
* @see AWS
* API Documentation
*/
@Override
public DeleteApplicationResponse deleteApplication(DeleteApplicationRequest deleteApplicationRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, InvalidRoleException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteApplication").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteApplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteApplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a deployment configuration.
*
*
*
* A deployment configuration cannot be deleted if it is currently in use. Predefined configurations cannot be
* deleted.
*
*
*
* @param deleteDeploymentConfigRequest
* Represents the input of a DeleteDeploymentConfig
operation.
* @return Result of the DeleteDeploymentConfig operation returned by the service.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigNameRequiredException
* The deployment configuration name was not specified.
* @throws DeploymentConfigInUseException
* The deployment configuration is still in use.
* @throws InvalidOperationException
* An invalid operation was detected.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.DeleteDeploymentConfig
* @see AWS API Documentation
*/
@Override
public DeleteDeploymentConfigResponse deleteDeploymentConfig(DeleteDeploymentConfigRequest deleteDeploymentConfigRequest)
throws InvalidDeploymentConfigNameException, DeploymentConfigNameRequiredException, DeploymentConfigInUseException,
InvalidOperationException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDeploymentConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDeploymentConfigRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDeploymentConfigRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDeploymentConfig");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDeploymentConfig").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteDeploymentConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteDeploymentConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a deployment group.
*
*
* @param deleteDeploymentGroupRequest
* Represents the input of a DeleteDeploymentGroup
operation.
* @return Result of the DeleteDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.DeleteDeploymentGroup
* @see AWS API Documentation
*/
@Override
public DeleteDeploymentGroupResponse deleteDeploymentGroup(DeleteDeploymentGroupRequest deleteDeploymentGroupRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, DeploymentGroupNameRequiredException,
InvalidDeploymentGroupNameException, InvalidRoleException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDeploymentGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDeploymentGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDeploymentGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDeploymentGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteDeploymentGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteDeploymentGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteDeploymentGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a GitHub account connection.
*
*
* @param deleteGitHubAccountTokenRequest
* Represents the input of a DeleteGitHubAccount
operation.
* @return Result of the DeleteGitHubAccountToken operation returned by the service.
* @throws GitHubAccountTokenNameRequiredException
* The call is missing a required GitHub account connection name.
* @throws GitHubAccountTokenDoesNotExistException
* No GitHub account connection exists with the named specified in the call.
* @throws InvalidGitHubAccountTokenNameException
* The format of the specified GitHub account connection name is invalid.
* @throws ResourceValidationException
* The specified resource could not be validated.
* @throws OperationNotSupportedException
* The API used does not support the deployment.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.DeleteGitHubAccountToken
* @see AWS API Documentation
*/
@Override
public DeleteGitHubAccountTokenResponse deleteGitHubAccountToken(
DeleteGitHubAccountTokenRequest deleteGitHubAccountTokenRequest) throws GitHubAccountTokenNameRequiredException,
GitHubAccountTokenDoesNotExistException, InvalidGitHubAccountTokenNameException, ResourceValidationException,
OperationNotSupportedException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteGitHubAccountTokenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteGitHubAccountTokenRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGitHubAccountTokenRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGitHubAccountToken");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteGitHubAccountToken").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteGitHubAccountTokenRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteGitHubAccountTokenRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes resources linked to an external ID. This action only applies if you have configured blue/green
* deployments through CloudFormation.
*
*
*
* It is not necessary to call this action directly. CloudFormation calls it on your behalf when it needs to delete
* stack resources. This action is offered publicly in case you need to delete resources to comply with General Data
* Protection Regulation (GDPR) requirements.
*
*
*
* @param deleteResourcesByExternalIdRequest
* @return Result of the DeleteResourcesByExternalId operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.DeleteResourcesByExternalId
* @see AWS API Documentation
*/
@Override
public DeleteResourcesByExternalIdResponse deleteResourcesByExternalId(
DeleteResourcesByExternalIdRequest deleteResourcesByExternalIdRequest) throws AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteResourcesByExternalIdResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteResourcesByExternalIdRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteResourcesByExternalIdRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteResourcesByExternalId");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteResourcesByExternalId").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteResourcesByExternalIdRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteResourcesByExternalIdRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deregisters an on-premises instance.
*
*
* @param deregisterOnPremisesInstanceRequest
* Represents the input of a DeregisterOnPremisesInstance
operation.
* @return Result of the DeregisterOnPremisesInstance operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.DeregisterOnPremisesInstance
* @see AWS API Documentation
*/
@Override
public DeregisterOnPremisesInstanceResponse deregisterOnPremisesInstance(
DeregisterOnPremisesInstanceRequest deregisterOnPremisesInstanceRequest) throws InstanceNameRequiredException,
InvalidInstanceNameException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterOnPremisesInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterOnPremisesInstanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterOnPremisesInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterOnPremisesInstance");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterOnPremisesInstance").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deregisterOnPremisesInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeregisterOnPremisesInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about an application.
*
*
* @param getApplicationRequest
* Represents the input of a GetApplication
operation.
* @return Result of the GetApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.GetApplication
* @see AWS API
* Documentation
*/
@Override
public GetApplicationResponse getApplication(GetApplicationRequest getApplicationRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getApplicationRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetApplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetApplication").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getApplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetApplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about an application revision.
*
*
* @param getApplicationRevisionRequest
* Represents the input of a GetApplicationRevision
operation.
* @return Result of the GetApplicationRevision operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws RevisionDoesNotExistException
* The named revision does not exist with the user or Amazon Web Services account.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.GetApplicationRevision
* @see AWS API Documentation
*/
@Override
public GetApplicationRevisionResponse getApplicationRevision(GetApplicationRevisionRequest getApplicationRevisionRequest)
throws ApplicationDoesNotExistException, ApplicationNameRequiredException, InvalidApplicationNameException,
RevisionDoesNotExistException, RevisionRequiredException, InvalidRevisionException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetApplicationRevisionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getApplicationRevisionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getApplicationRevisionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetApplicationRevision");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetApplicationRevision").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getApplicationRevisionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetApplicationRevisionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about a deployment.
*
*
*
* The content
property of the appSpecContent
object in the returned revision is always
* null. Use GetApplicationRevision
and the sha256
property of the returned
* appSpecContent
object to get the content of the deployment’s AppSpec file.
*
*
*
* @param getDeploymentRequest
* Represents the input of a GetDeployment
operation.
* @return Result of the GetDeployment operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.GetDeployment
* @see AWS API
* Documentation
*/
@Override
public GetDeploymentResponse getDeployment(GetDeploymentRequest getDeploymentRequest) throws DeploymentIdRequiredException,
InvalidDeploymentIdException, DeploymentDoesNotExistException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeploymentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeployment");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDeployment").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getDeploymentRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDeploymentRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about a deployment configuration.
*
*
* @param getDeploymentConfigRequest
* Represents the input of a GetDeploymentConfig
operation.
* @return Result of the GetDeploymentConfig operation returned by the service.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigNameRequiredException
* The deployment configuration name was not specified.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.GetDeploymentConfig
* @see AWS API Documentation
*/
@Override
public GetDeploymentConfigResponse getDeploymentConfig(GetDeploymentConfigRequest getDeploymentConfigRequest)
throws InvalidDeploymentConfigNameException, DeploymentConfigNameRequiredException,
DeploymentConfigDoesNotExistException, InvalidComputePlatformException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDeploymentConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeploymentConfigRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeploymentConfigRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeploymentConfig");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDeploymentConfig").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getDeploymentConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDeploymentConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about a deployment group.
*
*
* @param getDeploymentGroupRequest
* Represents the input of a GetDeploymentGroup
operation.
* @return Result of the GetDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.GetDeploymentGroup
* @see AWS
* API Documentation
*/
@Override
public GetDeploymentGroupResponse getDeploymentGroup(GetDeploymentGroupRequest getDeploymentGroupRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
DeploymentGroupNameRequiredException, InvalidDeploymentGroupNameException, DeploymentGroupDoesNotExistException,
DeploymentConfigDoesNotExistException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDeploymentGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeploymentGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeploymentGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeploymentGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDeploymentGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getDeploymentGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDeploymentGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about a deployment target.
*
*
* @param getDeploymentTargetRequest
* @return Result of the GetDeploymentTarget operation returned by the service.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws DeploymentTargetIdRequiredException
* A deployment target ID was not provided.
* @throws InvalidDeploymentTargetIdException
* The target ID provided was not valid.
* @throws DeploymentTargetDoesNotExistException
* The provided target ID does not belong to the attempted deployment.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.GetDeploymentTarget
* @see AWS API Documentation
*/
@Override
public GetDeploymentTargetResponse getDeploymentTarget(GetDeploymentTargetRequest getDeploymentTargetRequest)
throws InvalidDeploymentIdException, DeploymentIdRequiredException, DeploymentDoesNotExistException,
DeploymentNotStartedException, DeploymentTargetIdRequiredException, InvalidDeploymentTargetIdException,
DeploymentTargetDoesNotExistException, InvalidInstanceNameException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDeploymentTargetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeploymentTargetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeploymentTargetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeploymentTarget");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDeploymentTarget").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getDeploymentTargetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDeploymentTargetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets information about an on-premises instance.
*
*
* @param getOnPremisesInstanceRequest
* Represents the input of a GetOnPremisesInstance
operation.
* @return Result of the GetOnPremisesInstance operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InstanceNotRegisteredException
* The specified on-premises instance is not registered.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.GetOnPremisesInstance
* @see AWS API Documentation
*/
@Override
public GetOnPremisesInstanceResponse getOnPremisesInstance(GetOnPremisesInstanceRequest getOnPremisesInstanceRequest)
throws InstanceNameRequiredException, InstanceNotRegisteredException, InvalidInstanceNameException,
AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetOnPremisesInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getOnPremisesInstanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getOnPremisesInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOnPremisesInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetOnPremisesInstance").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getOnPremisesInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetOnPremisesInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists information about revisions for an application.
*
*
* @param listApplicationRevisionsRequest
* Represents the input of a ListApplicationRevisions
operation.
* @return Result of the ListApplicationRevisions operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws InvalidSortByException
* The column name to sort by is either not present or was specified in an invalid format.
* @throws InvalidSortOrderException
* The sort order was specified in an invalid format.
* @throws InvalidBucketNameFilterException
* The bucket name either doesn't exist or was specified in an invalid format.
* @throws InvalidKeyPrefixFilterException
* The specified key prefix filter was specified in an invalid format.
* @throws BucketNameFilterRequiredException
* A bucket name is required, but was not provided.
* @throws InvalidDeployedStateFilterException
* The deployed state filter was specified in an invalid format.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListApplicationRevisions
* @see AWS API Documentation
*/
@Override
public ListApplicationRevisionsResponse listApplicationRevisions(
ListApplicationRevisionsRequest listApplicationRevisionsRequest) throws ApplicationDoesNotExistException,
ApplicationNameRequiredException, InvalidApplicationNameException, InvalidSortByException, InvalidSortOrderException,
InvalidBucketNameFilterException, InvalidKeyPrefixFilterException, BucketNameFilterRequiredException,
InvalidDeployedStateFilterException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListApplicationRevisionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listApplicationRevisionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listApplicationRevisionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListApplicationRevisions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListApplicationRevisions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listApplicationRevisionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListApplicationRevisionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the applications registered with the user or Amazon Web Services account.
*
*
* @param listApplicationsRequest
* Represents the input of a ListApplications
operation.
* @return Result of the ListApplications operation returned by the service.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListApplications
* @see AWS
* API Documentation
*/
@Override
public ListApplicationsResponse listApplications(ListApplicationsRequest listApplicationsRequest)
throws InvalidNextTokenException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListApplicationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listApplicationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listApplicationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListApplications");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListApplications").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listApplicationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListApplicationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the deployment configurations with the user or Amazon Web Services account.
*
*
* @param listDeploymentConfigsRequest
* Represents the input of a ListDeploymentConfigs
operation.
* @return Result of the ListDeploymentConfigs operation returned by the service.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListDeploymentConfigs
* @see AWS API Documentation
*/
@Override
public ListDeploymentConfigsResponse listDeploymentConfigs(ListDeploymentConfigsRequest listDeploymentConfigsRequest)
throws InvalidNextTokenException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDeploymentConfigsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDeploymentConfigsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDeploymentConfigsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDeploymentConfigs");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListDeploymentConfigs").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listDeploymentConfigsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDeploymentConfigsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the deployment groups for an application registered with the Amazon Web Services user or Amazon Web
* Services account.
*
*
* @param listDeploymentGroupsRequest
* Represents the input of a ListDeploymentGroups
operation.
* @return Result of the ListDeploymentGroups operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListDeploymentGroups
* @see AWS API Documentation
*/
@Override
public ListDeploymentGroupsResponse listDeploymentGroups(ListDeploymentGroupsRequest listDeploymentGroupsRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDeploymentGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDeploymentGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDeploymentGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDeploymentGroups");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListDeploymentGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listDeploymentGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDeploymentGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns an array of target IDs that are associated a deployment.
*
*
* @param listDeploymentTargetsRequest
* @return Result of the ListDeploymentTargets operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws InvalidInstanceStatusException
* The specified instance status does not exist.
* @throws InvalidInstanceTypeException
* An invalid instance type was specified for instances in a blue/green deployment. Valid values include
* "Blue" for an original environment and "Green" for a replacement environment.
* @throws InvalidDeploymentInstanceTypeException
* An instance type was specified for an in-place deployment. Instance types are supported for blue/green
* deployments only.
* @throws InvalidTargetFilterNameException
* The target filter name is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListDeploymentTargets
* @see AWS API Documentation
*/
@Override
public ListDeploymentTargetsResponse listDeploymentTargets(ListDeploymentTargetsRequest listDeploymentTargetsRequest)
throws DeploymentIdRequiredException, DeploymentDoesNotExistException, DeploymentNotStartedException,
InvalidNextTokenException, InvalidDeploymentIdException, InvalidInstanceStatusException,
InvalidInstanceTypeException, InvalidDeploymentInstanceTypeException, InvalidTargetFilterNameException,
AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDeploymentTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDeploymentTargetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDeploymentTargetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDeploymentTargets");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListDeploymentTargets").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listDeploymentTargetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDeploymentTargetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the deployments in a deployment group for an application registered with the user or Amazon Web Services
* account.
*
*
* @param listDeploymentsRequest
* Represents the input of a ListDeployments
operation.
* @return Result of the ListDeployments operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidTimeRangeException
* The specified time range was specified in an invalid format.
* @throws InvalidDeploymentStatusException
* The specified deployment status doesn't exist or cannot be determined.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws InvalidExternalIdException
* The external ID was specified in an invalid format.
* @throws InvalidInputException
* The input was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListDeployments
* @see AWS
* API Documentation
*/
@Override
public ListDeploymentsResponse listDeployments(ListDeploymentsRequest listDeploymentsRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
InvalidDeploymentGroupNameException, DeploymentGroupDoesNotExistException, DeploymentGroupNameRequiredException,
InvalidTimeRangeException, InvalidDeploymentStatusException, InvalidNextTokenException, InvalidExternalIdException,
InvalidInputException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListDeploymentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDeploymentsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDeploymentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDeployments");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListDeployments").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listDeploymentsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDeploymentsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the names of stored connections to GitHub accounts.
*
*
* @param listGitHubAccountTokenNamesRequest
* Represents the input of a ListGitHubAccountTokenNames
operation.
* @return Result of the ListGitHubAccountTokenNames operation returned by the service.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws ResourceValidationException
* The specified resource could not be validated.
* @throws OperationNotSupportedException
* The API used does not support the deployment.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListGitHubAccountTokenNames
* @see AWS API Documentation
*/
@Override
public ListGitHubAccountTokenNamesResponse listGitHubAccountTokenNames(
ListGitHubAccountTokenNamesRequest listGitHubAccountTokenNamesRequest) throws InvalidNextTokenException,
ResourceValidationException, OperationNotSupportedException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListGitHubAccountTokenNamesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listGitHubAccountTokenNamesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listGitHubAccountTokenNamesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListGitHubAccountTokenNames");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListGitHubAccountTokenNames").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listGitHubAccountTokenNamesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListGitHubAccountTokenNamesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets a list of names for one or more on-premises instances.
*
*
* Unless otherwise specified, both registered and deregistered on-premises instance names are listed. To list only
* registered or deregistered on-premises instance names, use the registration status parameter.
*
*
* @param listOnPremisesInstancesRequest
* Represents the input of a ListOnPremisesInstances
operation.
* @return Result of the ListOnPremisesInstances operation returned by the service.
* @throws InvalidRegistrationStatusException
* The registration status was specified in an invalid format.
* @throws InvalidTagFilterException
* The tag filter was specified in an invalid format.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListOnPremisesInstances
* @see AWS API Documentation
*/
@Override
public ListOnPremisesInstancesResponse listOnPremisesInstances(ListOnPremisesInstancesRequest listOnPremisesInstancesRequest)
throws InvalidRegistrationStatusException, InvalidTagFilterException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListOnPremisesInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listOnPremisesInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listOnPremisesInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListOnPremisesInstances");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListOnPremisesInstances").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listOnPremisesInstancesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListOnPremisesInstancesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of tags for the resource identified by a specified Amazon Resource Name (ARN). Tags are used to
* organize and categorize your CodeDeploy resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ArnNotSupportedException
* The specified ARN is not supported. For example, it might be an ARN for a resource that is not expected.
* @throws InvalidArnException
* The specified ARN is not in a valid format.
* @throws ResourceArnRequiredException
* The ARN of a resource is required, but was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ArnNotSupportedException, InvalidArnException, ResourceArnRequiredException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sets the result of a Lambda validation function. The function validates lifecycle hooks during a deployment that
* uses the Lambda or Amazon ECS compute platform. For Lambda deployments, the available lifecycle hooks are
* BeforeAllowTraffic
and AfterAllowTraffic
. For Amazon ECS deployments, the available
* lifecycle hooks are BeforeInstall
, AfterInstall
, AfterAllowTestTraffic
,
* BeforeAllowTraffic
, and AfterAllowTraffic
. Lambda validation functions return
* Succeeded
or Failed
. For more information, see AppSpec 'hooks' Section for an Lambda Deployment and AppSpec 'hooks' Section for an Amazon ECS Deployment.
*
*
* @param putLifecycleEventHookExecutionStatusRequest
* @return Result of the PutLifecycleEventHookExecutionStatus operation returned by the service.
* @throws InvalidLifecycleEventHookExecutionStatusException
* The result of a Lambda validation function that verifies a lifecycle event is invalid. It should return
* Succeeded
or Failed
.
* @throws InvalidLifecycleEventHookExecutionIdException
* A lifecycle event hook is invalid. Review the hooks
section in your AppSpec file to ensure
* the lifecycle events and hooks
functions are valid.
* @throws LifecycleEventAlreadyCompletedException
* An attempt to return the status of an already completed lifecycle event occurred.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws UnsupportedActionForDeploymentTypeException
* A call was submitted that is not supported for the specified deployment type.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.PutLifecycleEventHookExecutionStatus
* @see AWS API Documentation
*/
@Override
public PutLifecycleEventHookExecutionStatusResponse putLifecycleEventHookExecutionStatus(
PutLifecycleEventHookExecutionStatusRequest putLifecycleEventHookExecutionStatusRequest)
throws InvalidLifecycleEventHookExecutionStatusException, InvalidLifecycleEventHookExecutionIdException,
LifecycleEventAlreadyCompletedException, DeploymentIdRequiredException, DeploymentDoesNotExistException,
InvalidDeploymentIdException, UnsupportedActionForDeploymentTypeException, AwsServiceException, SdkClientException,
CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, PutLifecycleEventHookExecutionStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putLifecycleEventHookExecutionStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putLifecycleEventHookExecutionStatusRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutLifecycleEventHookExecutionStatus");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutLifecycleEventHookExecutionStatus").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(putLifecycleEventHookExecutionStatusRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutLifecycleEventHookExecutionStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers with CodeDeploy a revision for the specified application.
*
*
* @param registerApplicationRevisionRequest
* Represents the input of a RegisterApplicationRevision operation.
* @return Result of the RegisterApplicationRevision operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws DescriptionTooLongException
* The description is too long.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.RegisterApplicationRevision
* @see AWS API Documentation
*/
@Override
public RegisterApplicationRevisionResponse registerApplicationRevision(
RegisterApplicationRevisionRequest registerApplicationRevisionRequest) throws ApplicationDoesNotExistException,
ApplicationNameRequiredException, InvalidApplicationNameException, DescriptionTooLongException,
RevisionRequiredException, InvalidRevisionException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RegisterApplicationRevisionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(registerApplicationRevisionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerApplicationRevisionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterApplicationRevision");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RegisterApplicationRevision").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(registerApplicationRevisionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterApplicationRevisionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers an on-premises instance.
*
*
*
* Only one IAM ARN (an IAM session ARN or IAM user ARN) is supported in the request. You cannot use both.
*
*
*
* @param registerOnPremisesInstanceRequest
* Represents the input of the register on-premises instance operation.
* @return Result of the RegisterOnPremisesInstance operation returned by the service.
* @throws InstanceNameAlreadyRegisteredException
* The specified on-premises instance name is already registered.
* @throws IamArnRequiredException
* No IAM ARN was included in the request. You must use an IAM session ARN or user ARN in the request.
* @throws IamSessionArnAlreadyRegisteredException
* The request included an IAM session ARN that has already been used to register a different instance.
* @throws IamUserArnAlreadyRegisteredException
* The specified user ARN is already registered with an on-premises instance.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws IamUserArnRequiredException
* An user ARN was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws InvalidIamSessionArnException
* The IAM session ARN was specified in an invalid format.
* @throws InvalidIamUserArnException
* The user ARN was specified in an invalid format.
* @throws MultipleIamArnsProvidedException
* Both an user ARN and an IAM session ARN were included in the request. Use only one ARN type.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.RegisterOnPremisesInstance
* @see AWS API Documentation
*/
@Override
public RegisterOnPremisesInstanceResponse registerOnPremisesInstance(
RegisterOnPremisesInstanceRequest registerOnPremisesInstanceRequest) throws InstanceNameAlreadyRegisteredException,
IamArnRequiredException, IamSessionArnAlreadyRegisteredException, IamUserArnAlreadyRegisteredException,
InstanceNameRequiredException, IamUserArnRequiredException, InvalidInstanceNameException,
InvalidIamSessionArnException, InvalidIamUserArnException, MultipleIamArnsProvidedException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RegisterOnPremisesInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(registerOnPremisesInstanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerOnPremisesInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterOnPremisesInstance");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RegisterOnPremisesInstance").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(registerOnPremisesInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterOnPremisesInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes one or more tags from one or more on-premises instances.
*
*
* @param removeTagsFromOnPremisesInstancesRequest
* Represents the input of a RemoveTagsFromOnPremisesInstances
operation.
* @return Result of the RemoveTagsFromOnPremisesInstances operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws TagLimitExceededException
* The maximum allowed number of tags was exceeded.
* @throws InstanceLimitExceededException
* The maximum number of allowed on-premises instances in a single call was exceeded.
* @throws InstanceNotRegisteredException
* The specified on-premises instance is not registered.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.RemoveTagsFromOnPremisesInstances
* @see AWS API Documentation
*/
@Override
public RemoveTagsFromOnPremisesInstancesResponse removeTagsFromOnPremisesInstances(
RemoveTagsFromOnPremisesInstancesRequest removeTagsFromOnPremisesInstancesRequest)
throws InstanceNameRequiredException, InvalidInstanceNameException, TagRequiredException, InvalidTagException,
TagLimitExceededException, InstanceLimitExceededException, InstanceNotRegisteredException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveTagsFromOnPremisesInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeTagsFromOnPremisesInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
removeTagsFromOnPremisesInstancesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveTagsFromOnPremisesInstances");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveTagsFromOnPremisesInstances").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(removeTagsFromOnPremisesInstancesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveTagsFromOnPremisesInstancesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Attempts to stop an ongoing deployment.
*
*
* @param stopDeploymentRequest
* Represents the input of a StopDeployment
operation.
* @return Result of the StopDeployment operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentAlreadyCompletedException
* The deployment is already complete.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws UnsupportedActionForDeploymentTypeException
* A call was submitted that is not supported for the specified deployment type.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.StopDeployment
* @see AWS API
* Documentation
*/
@Override
public StopDeploymentResponse stopDeployment(StopDeploymentRequest stopDeploymentRequest)
throws DeploymentIdRequiredException, DeploymentDoesNotExistException, DeploymentGroupDoesNotExistException,
DeploymentAlreadyCompletedException, InvalidDeploymentIdException, UnsupportedActionForDeploymentTypeException,
AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StopDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(stopDeploymentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopDeployment");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StopDeployment").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(stopDeploymentRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StopDeploymentRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates the list of tags in the input Tags
parameter with the resource identified by the
* ResourceArn
input parameter.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceArnRequiredException
* The ARN of a resource is required, but was not found.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @throws ArnNotSupportedException
* The specified ARN is not supported. For example, it might be an ARN for a resource that is not expected.
* @throws InvalidArnException
* The specified ARN is not in a valid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ResourceArnRequiredException,
ApplicationDoesNotExistException, DeploymentGroupDoesNotExistException, DeploymentConfigDoesNotExistException,
TagRequiredException, InvalidTagsToAddException, ArnNotSupportedException, InvalidArnException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(tagResourceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates a resource from a list of tags. The resource is identified by the ResourceArn
input
* parameter. The tags are identified by the list of keys in the TagKeys
input parameter.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceArnRequiredException
* The ARN of a resource is required, but was not found.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @throws ArnNotSupportedException
* The specified ARN is not supported. For example, it might be an ARN for a resource that is not expected.
* @throws InvalidArnException
* The specified ARN is not in a valid format.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ResourceArnRequiredException,
ApplicationDoesNotExistException, DeploymentGroupDoesNotExistException, DeploymentConfigDoesNotExistException,
TagRequiredException, InvalidTagsToAddException, ArnNotSupportedException, InvalidArnException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Changes the name of an application.
*
*
* @param updateApplicationRequest
* Represents the input of an UpdateApplication
operation.
* @return Result of the UpdateApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationAlreadyExistsException
* An application with the specified name with the user or Amazon Web Services account already exists.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.UpdateApplication
* @see AWS
* API Documentation
*/
@Override
public UpdateApplicationResponse updateApplication(UpdateApplicationRequest updateApplicationRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationAlreadyExistsException,
ApplicationDoesNotExistException, AwsServiceException, SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateApplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateApplication").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateApplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateApplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Changes information about a deployment group.
*
*
* @param updateDeploymentGroupRequest
* Represents the input of an UpdateDeploymentGroup
operation.
* @return Result of the UpdateDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupAlreadyExistsException
* A deployment group with the specified name with the user or Amazon Web Services account already exists.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws InvalidEc2TagException
* The tag was specified in an invalid format.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws InvalidAutoScalingGroupException
* The Auto Scaling group was specified in an invalid format or does not exist.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws LifecycleHookLimitExceededException
* The limit for lifecycle hooks was exceeded.
* @throws InvalidTriggerConfigException
* The trigger was specified in an invalid format.
* @throws TriggerTargetsLimitExceededException
* The maximum allowed number of triggers was exceeded.
* @throws InvalidAlarmConfigException
* The format of the alarm configuration is invalid. Possible causes include:
*
* -
*
* The alarm list is null.
*
*
* -
*
* The alarm object is null.
*
*
* -
*
* The alarm name is empty or null or exceeds the limit of 255 characters.
*
*
* -
*
* Two alarms with the same name have been specified.
*
*
* -
*
* The alarm configuration is enabled, but the alarm list is empty.
*
*
* @throws AlarmsLimitExceededException
* The maximum number of alarms for a deployment group (10) was exceeded.
* @throws InvalidAutoRollbackConfigException
* The automatic rollback configuration was specified in an invalid format. For example, automatic rollback
* is enabled, but an invalid triggering event type or no event types were listed.
* @throws InvalidLoadBalancerInfoException
* An invalid load balancer name, or no load balancer name, was specified.
* @throws InvalidDeploymentStyleException
* An invalid deployment style was specified. Valid deployment types include "IN_PLACE" and "BLUE_GREEN."
* Valid deployment options include "WITH_TRAFFIC_CONTROL" and "WITHOUT_TRAFFIC_CONTROL."
* @throws InvalidBlueGreenDeploymentConfigurationException
* The configuration for the blue/green deployment group was provided in an invalid format. For information
* about deployment configuration format, see CreateDeploymentConfig.
* @throws InvalidEc2TagCombinationException
* A call was submitted that specified both Ec2TagFilters and Ec2TagSet, but only one of these data types
* can be used in a single call.
* @throws InvalidOnPremisesTagCombinationException
* A call was submitted that specified both OnPremisesTagFilters and OnPremisesTagSet, but only one of these
* data types can be used in a single call.
* @throws TagSetListLimitExceededException
* The number of tag groups included in the tag set list exceeded the maximum allowed limit of 3.
* @throws InvalidInputException
* The input was specified in an invalid format.
* @throws ThrottlingException
* An API function was called too frequently.
* @throws InvalidEcsServiceException
* The Amazon ECS service identifier is not valid.
* @throws InvalidTargetGroupPairException
* A target group pair associated with this deployment is not valid.
* @throws EcsServiceMappingLimitExceededException
* The Amazon ECS service is associated with more than one deployment groups. An Amazon ECS service can be
* associated with only one deployment group.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeDeployException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeDeployClient.UpdateDeploymentGroup
* @see AWS API Documentation
*/
@Override
public UpdateDeploymentGroupResponse updateDeploymentGroup(UpdateDeploymentGroupRequest updateDeploymentGroupRequest)
throws ApplicationNameRequiredException, InvalidApplicationNameException, ApplicationDoesNotExistException,
InvalidDeploymentGroupNameException, DeploymentGroupAlreadyExistsException, DeploymentGroupNameRequiredException,
DeploymentGroupDoesNotExistException, InvalidEc2TagException, InvalidTagException, InvalidAutoScalingGroupException,
InvalidDeploymentConfigNameException, DeploymentConfigDoesNotExistException, InvalidRoleException,
LifecycleHookLimitExceededException, InvalidTriggerConfigException, TriggerTargetsLimitExceededException,
InvalidAlarmConfigException, AlarmsLimitExceededException, InvalidAutoRollbackConfigException,
InvalidLoadBalancerInfoException, InvalidDeploymentStyleException, InvalidBlueGreenDeploymentConfigurationException,
InvalidEc2TagCombinationException, InvalidOnPremisesTagCombinationException, TagSetListLimitExceededException,
InvalidInputException, ThrottlingException, InvalidEcsServiceException, InvalidTargetGroupPairException,
EcsServiceMappingLimitExceededException, InvalidTrafficRoutingConfigurationException, AwsServiceException,
SdkClientException, CodeDeployException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateDeploymentGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateDeploymentGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateDeploymentGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeDeploy");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateDeploymentGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateDeploymentGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateDeploymentGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateDeploymentGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
* Create an instance of {@link CodeDeployWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link CodeDeployWaiter}
*/
@Override
public CodeDeployWaiter waiter() {
return CodeDeployWaiter.builder().client(this).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
CodeDeployServiceClientConfigurationBuilder serviceConfigBuilder = new CodeDeployServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(CodeDeployException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceValidationException")
.exceptionBuilderSupplier(ResourceValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidZonalDeploymentConfigurationException")
.exceptionBuilderSupplier(InvalidZonalDeploymentConfigurationException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("GitHubAccountTokenDoesNotExistException")
.exceptionBuilderSupplier(GitHubAccountTokenDoesNotExistException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidOperationException")
.exceptionBuilderSupplier(InvalidOperationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTagsToAddException")
.exceptionBuilderSupplier(InvalidTagsToAddException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TagSetListLimitExceededException")
.exceptionBuilderSupplier(TagSetListLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentConfigDoesNotExistException")
.exceptionBuilderSupplier(DeploymentConfigDoesNotExistException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ApplicationNameRequiredException")
.exceptionBuilderSupplier(ApplicationNameRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidLifecycleEventHookExecutionIdException")
.exceptionBuilderSupplier(InvalidLifecycleEventHookExecutionIdException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidAutoRollbackConfigException")
.exceptionBuilderSupplier(InvalidAutoRollbackConfigException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentLimitExceededException")
.exceptionBuilderSupplier(DeploymentLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidECSServiceException")
.exceptionBuilderSupplier(InvalidEcsServiceException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidArnException")
.exceptionBuilderSupplier(InvalidArnException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTagFilterException")
.exceptionBuilderSupplier(InvalidTagFilterException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentAlreadyCompletedException")
.exceptionBuilderSupplier(DeploymentAlreadyCompletedException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceArnRequiredException")
.exceptionBuilderSupplier(ResourceArnRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("OperationNotSupportedException")
.exceptionBuilderSupplier(OperationNotSupportedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentGroupLimitExceededException")
.exceptionBuilderSupplier(DeploymentGroupLimitExceededException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentGroupNameException")
.exceptionBuilderSupplier(InvalidDeploymentGroupNameException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentTargetDoesNotExistException")
.exceptionBuilderSupplier(DeploymentTargetDoesNotExistException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AlarmsLimitExceededException")
.exceptionBuilderSupplier(AlarmsLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidNextTokenException")
.exceptionBuilderSupplier(InvalidNextTokenException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidGitHubAccountTokenException")
.exceptionBuilderSupplier(InvalidGitHubAccountTokenException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TriggerTargetsLimitExceededException")
.exceptionBuilderSupplier(TriggerTargetsLimitExceededException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ECSServiceMappingLimitExceededException")
.exceptionBuilderSupplier(EcsServiceMappingLimitExceededException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidLoadBalancerInfoException")
.exceptionBuilderSupplier(InvalidLoadBalancerInfoException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("MultipleIamArnsProvidedException")
.exceptionBuilderSupplier(MultipleIamArnsProvidedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidEC2TagException")
.exceptionBuilderSupplier(InvalidEc2TagException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentConfigNameException")
.exceptionBuilderSupplier(InvalidDeploymentConfigNameException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LifecycleHookLimitExceededException")
.exceptionBuilderSupplier(LifecycleHookLimitExceededException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("IamUserArnAlreadyRegisteredException")
.exceptionBuilderSupplier(IamUserArnAlreadyRegisteredException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RevisionRequiredException")
.exceptionBuilderSupplier(RevisionRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentIsNotInReadyStateException")
.exceptionBuilderSupplier(DeploymentIsNotInReadyStateException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidGitHubAccountTokenNameException")
.exceptionBuilderSupplier(InvalidGitHubAccountTokenNameException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InstanceNotRegisteredException")
.exceptionBuilderSupplier(InstanceNotRegisteredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidBlueGreenDeploymentConfigurationException")
.exceptionBuilderSupplier(InvalidBlueGreenDeploymentConfigurationException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ApplicationAlreadyExistsException")
.exceptionBuilderSupplier(ApplicationAlreadyExistsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeployedStateFilterException")
.exceptionBuilderSupplier(InvalidDeployedStateFilterException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentTargetIdRequiredException")
.exceptionBuilderSupplier(DeploymentTargetIdRequiredException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidAlarmConfigException")
.exceptionBuilderSupplier(InvalidAlarmConfigException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DescriptionTooLongException")
.exceptionBuilderSupplier(DescriptionTooLongException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidInstanceStatusException")
.exceptionBuilderSupplier(InvalidInstanceStatusException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InstanceLimitExceededException")
.exceptionBuilderSupplier(InstanceLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentIdRequiredException")
.exceptionBuilderSupplier(DeploymentIdRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidIgnoreApplicationStopFailuresValueException")
.exceptionBuilderSupplier(InvalidIgnoreApplicationStopFailuresValueException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentConfigNameRequiredException")
.exceptionBuilderSupplier(DeploymentConfigNameRequiredException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTargetGroupPairException")
.exceptionBuilderSupplier(InvalidTargetGroupPairException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTargetFilterNameException")
.exceptionBuilderSupplier(InvalidTargetFilterNameException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ApplicationLimitExceededException")
.exceptionBuilderSupplier(ApplicationLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRegistrationStatusException")
.exceptionBuilderSupplier(InvalidRegistrationStatusException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidLifecycleEventHookExecutionStatusException")
.exceptionBuilderSupplier(InvalidLifecycleEventHookExecutionStatusException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidExternalIdException")
.exceptionBuilderSupplier(InvalidExternalIdException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentGroupDoesNotExistException")
.exceptionBuilderSupplier(DeploymentGroupDoesNotExistException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnsupportedActionForDeploymentTypeException")
.exceptionBuilderSupplier(UnsupportedActionForDeploymentTypeException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidMinimumHealthyHostValueException")
.exceptionBuilderSupplier(InvalidMinimumHealthyHostValueException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BatchLimitExceededException")
.exceptionBuilderSupplier(BatchLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ApplicationDoesNotExistException")
.exceptionBuilderSupplier(ApplicationDoesNotExistException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTargetInstancesException")
.exceptionBuilderSupplier(InvalidTargetInstancesException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TagRequiredException")
.exceptionBuilderSupplier(TagRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BucketNameFilterRequiredException")
.exceptionBuilderSupplier(BucketNameFilterRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidIamUserArnException")
.exceptionBuilderSupplier(InvalidIamUserArnException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidInstanceTypeException")
.exceptionBuilderSupplier(InvalidInstanceTypeException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTriggerConfigException")
.exceptionBuilderSupplier(InvalidTriggerConfigException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("IamArnRequiredException")
.exceptionBuilderSupplier(IamArnRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidKeyPrefixFilterException")
.exceptionBuilderSupplier(InvalidKeyPrefixFilterException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("GitHubAccountTokenNameRequiredException")
.exceptionBuilderSupplier(GitHubAccountTokenNameRequiredException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidBucketNameFilterException")
.exceptionBuilderSupplier(InvalidBucketNameFilterException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidComputePlatformException")
.exceptionBuilderSupplier(InvalidComputePlatformException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RoleRequiredException")
.exceptionBuilderSupplier(RoleRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentNotStartedException")
.exceptionBuilderSupplier(DeploymentNotStartedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LifecycleEventAlreadyCompletedException")
.exceptionBuilderSupplier(LifecycleEventAlreadyCompletedException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidIamSessionArnException")
.exceptionBuilderSupplier(InvalidIamSessionArnException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentGroupAlreadyExistsException")
.exceptionBuilderSupplier(DeploymentGroupAlreadyExistsException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidOnPremisesTagCombinationException")
.exceptionBuilderSupplier(InvalidOnPremisesTagCombinationException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InstanceNameRequiredException")
.exceptionBuilderSupplier(InstanceNameRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentConfigLimitExceededException")
.exceptionBuilderSupplier(DeploymentConfigLimitExceededException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRevisionException")
.exceptionBuilderSupplier(InvalidRevisionException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("IamUserArnRequiredException")
.exceptionBuilderSupplier(IamUserArnRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidUpdateOutdatedInstancesOnlyValueException")
.exceptionBuilderSupplier(InvalidUpdateOutdatedInstancesOnlyValueException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidEC2TagCombinationException")
.exceptionBuilderSupplier(InvalidEc2TagCombinationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentIdException")
.exceptionBuilderSupplier(InvalidDeploymentIdException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ArnNotSupportedException")
.exceptionBuilderSupplier(ArnNotSupportedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentTargetListSizeExceededException")
.exceptionBuilderSupplier(DeploymentTargetListSizeExceededException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentStatusException")
.exceptionBuilderSupplier(InvalidDeploymentStatusException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidInstanceNameException")
.exceptionBuilderSupplier(InvalidInstanceNameException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentConfigInUseException")
.exceptionBuilderSupplier(DeploymentConfigInUseException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentConfigAlreadyExistsException")
.exceptionBuilderSupplier(DeploymentConfigAlreadyExistsException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("IamSessionArnAlreadyRegisteredException")
.exceptionBuilderSupplier(IamSessionArnAlreadyRegisteredException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InstanceNameAlreadyRegisteredException")
.exceptionBuilderSupplier(InstanceNameAlreadyRegisteredException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTagException")
.exceptionBuilderSupplier(InvalidTagException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidApplicationNameException")
.exceptionBuilderSupplier(InvalidApplicationNameException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentStyleException")
.exceptionBuilderSupplier(InvalidDeploymentStyleException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTimeRangeException")
.exceptionBuilderSupplier(InvalidTimeRangeException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidInputException")
.exceptionBuilderSupplier(InvalidInputException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSortByException")
.exceptionBuilderSupplier(InvalidSortByException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentInstanceTypeException")
.exceptionBuilderSupplier(InvalidDeploymentInstanceTypeException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentDoesNotExistException")
.exceptionBuilderSupplier(DeploymentDoesNotExistException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSortOrderException")
.exceptionBuilderSupplier(InvalidSortOrderException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeploymentGroupNameRequiredException")
.exceptionBuilderSupplier(DeploymentGroupNameRequiredException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RevisionDoesNotExistException")
.exceptionBuilderSupplier(RevisionDoesNotExistException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRoleException")
.exceptionBuilderSupplier(InvalidRoleException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidFileExistsBehaviorException")
.exceptionBuilderSupplier(InvalidFileExistsBehaviorException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentTargetIdException")
.exceptionBuilderSupplier(InvalidDeploymentTargetIdException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TagLimitExceededException")
.exceptionBuilderSupplier(TagLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidDeploymentWaitTypeException")
.exceptionBuilderSupplier(InvalidDeploymentWaitTypeException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidAutoScalingGroupException")
.exceptionBuilderSupplier(InvalidAutoScalingGroupException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTrafficRoutingConfigurationException")
.exceptionBuilderSupplier(InvalidTrafficRoutingConfigurationException::builder)
.httpStatusCode(400).build());
}
@Override
public final CodeDeployServiceClientConfiguration serviceClientConfiguration() {
return new CodeDeployServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}