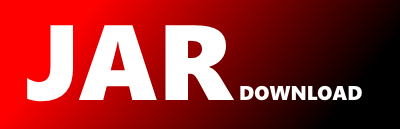
software.amazon.awssdk.services.codedeploy.model.ECSTarget Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codedeploy.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the target of an Amazon ECS deployment.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ECSTarget implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DEPLOYMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("deploymentId").getter(getter(ECSTarget::deploymentId)).setter(setter(Builder::deploymentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentId").build()).build();
private static final SdkField TARGET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("targetId").getter(getter(ECSTarget::targetId)).setter(setter(Builder::targetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetId").build()).build();
private static final SdkField TARGET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("targetArn").getter(getter(ECSTarget::targetArn)).setter(setter(Builder::targetArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetArn").build()).build();
private static final SdkField LAST_UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastUpdatedAt").getter(getter(ECSTarget::lastUpdatedAt)).setter(setter(Builder::lastUpdatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastUpdatedAt").build()).build();
private static final SdkField> LIFECYCLE_EVENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("lifecycleEvents")
.getter(getter(ECSTarget::lifecycleEvents))
.setter(setter(Builder::lifecycleEvents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lifecycleEvents").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LifecycleEvent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ECSTarget::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField> TASK_SETS_INFO_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("taskSetsInfo")
.getter(getter(ECSTarget::taskSetsInfo))
.setter(setter(Builder::taskSetsInfo))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskSetsInfo").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ECSTaskSet::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections
.unmodifiableList(Arrays.asList(DEPLOYMENT_ID_FIELD, TARGET_ID_FIELD, TARGET_ARN_FIELD, LAST_UPDATED_AT_FIELD,
LIFECYCLE_EVENTS_FIELD, STATUS_FIELD, TASK_SETS_INFO_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("deploymentId", DEPLOYMENT_ID_FIELD);
put("targetId", TARGET_ID_FIELD);
put("targetArn", TARGET_ARN_FIELD);
put("lastUpdatedAt", LAST_UPDATED_AT_FIELD);
put("lifecycleEvents", LIFECYCLE_EVENTS_FIELD);
put("status", STATUS_FIELD);
put("taskSetsInfo", TASK_SETS_INFO_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String deploymentId;
private final String targetId;
private final String targetArn;
private final Instant lastUpdatedAt;
private final List lifecycleEvents;
private final String status;
private final List taskSetsInfo;
private ECSTarget(BuilderImpl builder) {
this.deploymentId = builder.deploymentId;
this.targetId = builder.targetId;
this.targetArn = builder.targetArn;
this.lastUpdatedAt = builder.lastUpdatedAt;
this.lifecycleEvents = builder.lifecycleEvents;
this.status = builder.status;
this.taskSetsInfo = builder.taskSetsInfo;
}
/**
*
* The unique ID of a deployment.
*
*
* @return The unique ID of a deployment.
*/
public final String deploymentId() {
return deploymentId;
}
/**
*
* The unique ID of a deployment target that has a type of ecsTarget
.
*
*
* @return The unique ID of a deployment target that has a type of ecsTarget
.
*/
public final String targetId() {
return targetId;
}
/**
*
* The Amazon Resource Name (ARN) of the target.
*
*
* @return The Amazon Resource Name (ARN) of the target.
*/
public final String targetArn() {
return targetArn;
}
/**
*
* The date and time when the target Amazon ECS application was updated by a deployment.
*
*
* @return The date and time when the target Amazon ECS application was updated by a deployment.
*/
public final Instant lastUpdatedAt() {
return lastUpdatedAt;
}
/**
* For responses, this returns true if the service returned a value for the LifecycleEvents property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLifecycleEvents() {
return lifecycleEvents != null && !(lifecycleEvents instanceof SdkAutoConstructList);
}
/**
*
* The lifecycle events of the deployment to this target Amazon ECS application.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLifecycleEvents} method.
*
*
* @return The lifecycle events of the deployment to this target Amazon ECS application.
*/
public final List lifecycleEvents() {
return lifecycleEvents;
}
/**
*
* The status an Amazon ECS deployment's target ECS application.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TargetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status an Amazon ECS deployment's target ECS application.
* @see TargetStatus
*/
public final TargetStatus status() {
return TargetStatus.fromValue(status);
}
/**
*
* The status an Amazon ECS deployment's target ECS application.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TargetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status an Amazon ECS deployment's target ECS application.
* @see TargetStatus
*/
public final String statusAsString() {
return status;
}
/**
* For responses, this returns true if the service returned a value for the TaskSetsInfo property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTaskSetsInfo() {
return taskSetsInfo != null && !(taskSetsInfo instanceof SdkAutoConstructList);
}
/**
*
* The ECSTaskSet
objects associated with the ECS target.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTaskSetsInfo} method.
*
*
* @return The ECSTaskSet
objects associated with the ECS target.
*/
public final List taskSetsInfo() {
return taskSetsInfo;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(deploymentId());
hashCode = 31 * hashCode + Objects.hashCode(targetId());
hashCode = 31 * hashCode + Objects.hashCode(targetArn());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedAt());
hashCode = 31 * hashCode + Objects.hashCode(hasLifecycleEvents() ? lifecycleEvents() : null);
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTaskSetsInfo() ? taskSetsInfo() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ECSTarget)) {
return false;
}
ECSTarget other = (ECSTarget) obj;
return Objects.equals(deploymentId(), other.deploymentId()) && Objects.equals(targetId(), other.targetId())
&& Objects.equals(targetArn(), other.targetArn()) && Objects.equals(lastUpdatedAt(), other.lastUpdatedAt())
&& hasLifecycleEvents() == other.hasLifecycleEvents()
&& Objects.equals(lifecycleEvents(), other.lifecycleEvents())
&& Objects.equals(statusAsString(), other.statusAsString()) && hasTaskSetsInfo() == other.hasTaskSetsInfo()
&& Objects.equals(taskSetsInfo(), other.taskSetsInfo());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ECSTarget").add("DeploymentId", deploymentId()).add("TargetId", targetId())
.add("TargetArn", targetArn()).add("LastUpdatedAt", lastUpdatedAt())
.add("LifecycleEvents", hasLifecycleEvents() ? lifecycleEvents() : null).add("Status", statusAsString())
.add("TaskSetsInfo", hasTaskSetsInfo() ? taskSetsInfo() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "deploymentId":
return Optional.ofNullable(clazz.cast(deploymentId()));
case "targetId":
return Optional.ofNullable(clazz.cast(targetId()));
case "targetArn":
return Optional.ofNullable(clazz.cast(targetArn()));
case "lastUpdatedAt":
return Optional.ofNullable(clazz.cast(lastUpdatedAt()));
case "lifecycleEvents":
return Optional.ofNullable(clazz.cast(lifecycleEvents()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "taskSetsInfo":
return Optional.ofNullable(clazz.cast(taskSetsInfo()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function