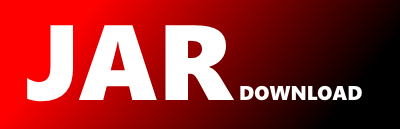
software.amazon.awssdk.services.codedeploy.model.DeploymentGroupInfo Maven / Gradle / Ivy
Show all versions of codedeploy Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codedeploy.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a deployment group.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeploymentGroupInfo implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DeploymentGroupInfo::applicationName)).setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("applicationName").build()).build();
private static final SdkField DEPLOYMENT_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DeploymentGroupInfo::deploymentGroupId)).setter(setter(Builder::deploymentGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentGroupId").build()).build();
private static final SdkField DEPLOYMENT_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DeploymentGroupInfo::deploymentGroupName)).setter(setter(Builder::deploymentGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentGroupName").build())
.build();
private static final SdkField DEPLOYMENT_CONFIG_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DeploymentGroupInfo::deploymentConfigName)).setter(setter(Builder::deploymentConfigName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentConfigName").build())
.build();
private static final SdkField> EC2_TAG_FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DeploymentGroupInfo::ec2TagFilters))
.setter(setter(Builder::ec2TagFilters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ec2TagFilters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EC2TagFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ON_PREMISES_INSTANCE_TAG_FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DeploymentGroupInfo::onPremisesInstanceTagFilters))
.setter(setter(Builder::onPremisesInstanceTagFilters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("onPremisesInstanceTagFilters")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TagFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> AUTO_SCALING_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DeploymentGroupInfo::autoScalingGroups))
.setter(setter(Builder::autoScalingGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("autoScalingGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AutoScalingGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SERVICE_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DeploymentGroupInfo::serviceRoleArn)).setter(setter(Builder::serviceRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRoleArn").build()).build();
private static final SdkField TARGET_REVISION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(DeploymentGroupInfo::targetRevision))
.setter(setter(Builder::targetRevision)).constructor(RevisionLocation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetRevision").build()).build();
private static final SdkField> TRIGGER_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DeploymentGroupInfo::triggerConfigurations))
.setter(setter(Builder::triggerConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("triggerConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TriggerConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ALARM_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(DeploymentGroupInfo::alarmConfiguration))
.setter(setter(Builder::alarmConfiguration)).constructor(AlarmConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("alarmConfiguration").build())
.build();
private static final SdkField AUTO_ROLLBACK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(DeploymentGroupInfo::autoRollbackConfiguration)).setter(setter(Builder::autoRollbackConfiguration))
.constructor(AutoRollbackConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("autoRollbackConfiguration").build())
.build();
private static final SdkField DEPLOYMENT_STYLE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(DeploymentGroupInfo::deploymentStyle))
.setter(setter(Builder::deploymentStyle)).constructor(DeploymentStyle::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentStyle").build()).build();
private static final SdkField BLUE_GREEN_DEPLOYMENT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(DeploymentGroupInfo::blueGreenDeploymentConfiguration))
.setter(setter(Builder::blueGreenDeploymentConfiguration))
.constructor(BlueGreenDeploymentConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("blueGreenDeploymentConfiguration")
.build()).build();
private static final SdkField LOAD_BALANCER_INFO_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(DeploymentGroupInfo::loadBalancerInfo))
.setter(setter(Builder::loadBalancerInfo)).constructor(LoadBalancerInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancerInfo").build()).build();
private static final SdkField LAST_SUCCESSFUL_DEPLOYMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(DeploymentGroupInfo::lastSuccessfulDeployment))
.setter(setter(Builder::lastSuccessfulDeployment)).constructor(LastDeploymentInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastSuccessfulDeployment").build())
.build();
private static final SdkField LAST_ATTEMPTED_DEPLOYMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(DeploymentGroupInfo::lastAttemptedDeployment))
.setter(setter(Builder::lastAttemptedDeployment)).constructor(LastDeploymentInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastAttemptedDeployment").build())
.build();
private static final SdkField EC2_TAG_SET_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(DeploymentGroupInfo::ec2TagSet)).setter(setter(Builder::ec2TagSet)).constructor(EC2TagSet::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ec2TagSet").build()).build();
private static final SdkField ON_PREMISES_TAG_SET_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(DeploymentGroupInfo::onPremisesTagSet))
.setter(setter(Builder::onPremisesTagSet)).constructor(OnPremisesTagSet::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("onPremisesTagSet").build()).build();
private static final SdkField COMPUTE_PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DeploymentGroupInfo::computePlatformAsString)).setter(setter(Builder::computePlatform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("computePlatform").build()).build();
private static final SdkField> ECS_SERVICES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DeploymentGroupInfo::ecsServices))
.setter(setter(Builder::ecsServices))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ecsServices").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ECSService::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_NAME_FIELD,
DEPLOYMENT_GROUP_ID_FIELD, DEPLOYMENT_GROUP_NAME_FIELD, DEPLOYMENT_CONFIG_NAME_FIELD, EC2_TAG_FILTERS_FIELD,
ON_PREMISES_INSTANCE_TAG_FILTERS_FIELD, AUTO_SCALING_GROUPS_FIELD, SERVICE_ROLE_ARN_FIELD, TARGET_REVISION_FIELD,
TRIGGER_CONFIGURATIONS_FIELD, ALARM_CONFIGURATION_FIELD, AUTO_ROLLBACK_CONFIGURATION_FIELD, DEPLOYMENT_STYLE_FIELD,
BLUE_GREEN_DEPLOYMENT_CONFIGURATION_FIELD, LOAD_BALANCER_INFO_FIELD, LAST_SUCCESSFUL_DEPLOYMENT_FIELD,
LAST_ATTEMPTED_DEPLOYMENT_FIELD, EC2_TAG_SET_FIELD, ON_PREMISES_TAG_SET_FIELD, COMPUTE_PLATFORM_FIELD,
ECS_SERVICES_FIELD));
private static final long serialVersionUID = 1L;
private final String applicationName;
private final String deploymentGroupId;
private final String deploymentGroupName;
private final String deploymentConfigName;
private final List ec2TagFilters;
private final List onPremisesInstanceTagFilters;
private final List autoScalingGroups;
private final String serviceRoleArn;
private final RevisionLocation targetRevision;
private final List triggerConfigurations;
private final AlarmConfiguration alarmConfiguration;
private final AutoRollbackConfiguration autoRollbackConfiguration;
private final DeploymentStyle deploymentStyle;
private final BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration;
private final LoadBalancerInfo loadBalancerInfo;
private final LastDeploymentInfo lastSuccessfulDeployment;
private final LastDeploymentInfo lastAttemptedDeployment;
private final EC2TagSet ec2TagSet;
private final OnPremisesTagSet onPremisesTagSet;
private final String computePlatform;
private final List ecsServices;
private DeploymentGroupInfo(BuilderImpl builder) {
this.applicationName = builder.applicationName;
this.deploymentGroupId = builder.deploymentGroupId;
this.deploymentGroupName = builder.deploymentGroupName;
this.deploymentConfigName = builder.deploymentConfigName;
this.ec2TagFilters = builder.ec2TagFilters;
this.onPremisesInstanceTagFilters = builder.onPremisesInstanceTagFilters;
this.autoScalingGroups = builder.autoScalingGroups;
this.serviceRoleArn = builder.serviceRoleArn;
this.targetRevision = builder.targetRevision;
this.triggerConfigurations = builder.triggerConfigurations;
this.alarmConfiguration = builder.alarmConfiguration;
this.autoRollbackConfiguration = builder.autoRollbackConfiguration;
this.deploymentStyle = builder.deploymentStyle;
this.blueGreenDeploymentConfiguration = builder.blueGreenDeploymentConfiguration;
this.loadBalancerInfo = builder.loadBalancerInfo;
this.lastSuccessfulDeployment = builder.lastSuccessfulDeployment;
this.lastAttemptedDeployment = builder.lastAttemptedDeployment;
this.ec2TagSet = builder.ec2TagSet;
this.onPremisesTagSet = builder.onPremisesTagSet;
this.computePlatform = builder.computePlatform;
this.ecsServices = builder.ecsServices;
}
/**
*
* The application name.
*
*
* @return The application name.
*/
public String applicationName() {
return applicationName;
}
/**
*
* The deployment group ID.
*
*
* @return The deployment group ID.
*/
public String deploymentGroupId() {
return deploymentGroupId;
}
/**
*
* The deployment group name.
*
*
* @return The deployment group name.
*/
public String deploymentGroupName() {
return deploymentGroupName;
}
/**
*
* The deployment configuration name.
*
*
* @return The deployment configuration name.
*/
public String deploymentConfigName() {
return deploymentConfigName;
}
/**
*
* The Amazon EC2 tags on which to filter. The deployment group includes EC2 instances with any of the specified
* tags.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The Amazon EC2 tags on which to filter. The deployment group includes EC2 instances with any of the
* specified tags.
*/
public List ec2TagFilters() {
return ec2TagFilters;
}
/**
*
* The on-premises instance tags on which to filter. The deployment group includes on-premises instances with any of
* the specified tags.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The on-premises instance tags on which to filter. The deployment group includes on-premises instances
* with any of the specified tags.
*/
public List onPremisesInstanceTagFilters() {
return onPremisesInstanceTagFilters;
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of associated Auto Scaling groups.
*/
public List autoScalingGroups() {
return autoScalingGroups;
}
/**
*
* A service role ARN.
*
*
* @return A service role ARN.
*/
public String serviceRoleArn() {
return serviceRoleArn;
}
/**
*
* Information about the deployment group's target revision, including type and location.
*
*
* @return Information about the deployment group's target revision, including type and location.
*/
public RevisionLocation targetRevision() {
return targetRevision;
}
/**
*
* Information about triggers associated with the deployment group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Information about triggers associated with the deployment group.
*/
public List triggerConfigurations() {
return triggerConfigurations;
}
/**
*
* A list of alarms associated with the deployment group.
*
*
* @return A list of alarms associated with the deployment group.
*/
public AlarmConfiguration alarmConfiguration() {
return alarmConfiguration;
}
/**
*
* Information about the automatic rollback configuration associated with the deployment group.
*
*
* @return Information about the automatic rollback configuration associated with the deployment group.
*/
public AutoRollbackConfiguration autoRollbackConfiguration() {
return autoRollbackConfiguration;
}
/**
*
* Information about the type of deployment, either in-place or blue/green, you want to run and whether to route
* deployment traffic behind a load balancer.
*
*
* @return Information about the type of deployment, either in-place or blue/green, you want to run and whether to
* route deployment traffic behind a load balancer.
*/
public DeploymentStyle deploymentStyle() {
return deploymentStyle;
}
/**
*
* Information about blue/green deployment options for a deployment group.
*
*
* @return Information about blue/green deployment options for a deployment group.
*/
public BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration() {
return blueGreenDeploymentConfiguration;
}
/**
*
* Information about the load balancer to use in a deployment.
*
*
* @return Information about the load balancer to use in a deployment.
*/
public LoadBalancerInfo loadBalancerInfo() {
return loadBalancerInfo;
}
/**
*
* Information about the most recent successful deployment to the deployment group.
*
*
* @return Information about the most recent successful deployment to the deployment group.
*/
public LastDeploymentInfo lastSuccessfulDeployment() {
return lastSuccessfulDeployment;
}
/**
*
* Information about the most recent attempted deployment to the deployment group.
*
*
* @return Information about the most recent attempted deployment to the deployment group.
*/
public LastDeploymentInfo lastAttemptedDeployment() {
return lastAttemptedDeployment;
}
/**
*
* Information about groups of tags applied to an EC2 instance. The deployment group includes only EC2 instances
* identified by all of the tag groups. Cannot be used in the same call as ec2TagFilters.
*
*
* @return Information about groups of tags applied to an EC2 instance. The deployment group includes only EC2
* instances identified by all of the tag groups. Cannot be used in the same call as ec2TagFilters.
*/
public EC2TagSet ec2TagSet() {
return ec2TagSet;
}
/**
*
* Information about groups of tags applied to an on-premises instance. The deployment group includes only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*
*
* @return Information about groups of tags applied to an on-premises instance. The deployment group includes only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*/
public OnPremisesTagSet onPremisesTagSet() {
return onPremisesTagSet;
}
/**
*
* The destination platform type for the deployment group (Lambda
or Server
).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #computePlatform}
* will return {@link ComputePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #computePlatformAsString}.
*
*
* @return The destination platform type for the deployment group (Lambda
or Server
).
* @see ComputePlatform
*/
public ComputePlatform computePlatform() {
return ComputePlatform.fromValue(computePlatform);
}
/**
*
* The destination platform type for the deployment group (Lambda
or Server
).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #computePlatform}
* will return {@link ComputePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #computePlatformAsString}.
*
*
* @return The destination platform type for the deployment group (Lambda
or Server
).
* @see ComputePlatform
*/
public String computePlatformAsString() {
return computePlatform;
}
/**
*
* The target Amazon ECS services in the deployment group. This applies only to deployment groups that use the
* Amazon ECS compute platform. A target Amazon ECS service is specified as an Amazon ECS cluster and service name
* pair using the format <clustername>:<servicename>
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The target Amazon ECS services in the deployment group. This applies only to deployment groups that use
* the Amazon ECS compute platform. A target Amazon ECS service is specified as an Amazon ECS cluster and
* service name pair using the format <clustername>:<servicename>
.
*/
public List ecsServices() {
return ecsServices;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(applicationName());
hashCode = 31 * hashCode + Objects.hashCode(deploymentGroupId());
hashCode = 31 * hashCode + Objects.hashCode(deploymentGroupName());
hashCode = 31 * hashCode + Objects.hashCode(deploymentConfigName());
hashCode = 31 * hashCode + Objects.hashCode(ec2TagFilters());
hashCode = 31 * hashCode + Objects.hashCode(onPremisesInstanceTagFilters());
hashCode = 31 * hashCode + Objects.hashCode(autoScalingGroups());
hashCode = 31 * hashCode + Objects.hashCode(serviceRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(targetRevision());
hashCode = 31 * hashCode + Objects.hashCode(triggerConfigurations());
hashCode = 31 * hashCode + Objects.hashCode(alarmConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(autoRollbackConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(deploymentStyle());
hashCode = 31 * hashCode + Objects.hashCode(blueGreenDeploymentConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(loadBalancerInfo());
hashCode = 31 * hashCode + Objects.hashCode(lastSuccessfulDeployment());
hashCode = 31 * hashCode + Objects.hashCode(lastAttemptedDeployment());
hashCode = 31 * hashCode + Objects.hashCode(ec2TagSet());
hashCode = 31 * hashCode + Objects.hashCode(onPremisesTagSet());
hashCode = 31 * hashCode + Objects.hashCode(computePlatformAsString());
hashCode = 31 * hashCode + Objects.hashCode(ecsServices());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeploymentGroupInfo)) {
return false;
}
DeploymentGroupInfo other = (DeploymentGroupInfo) obj;
return Objects.equals(applicationName(), other.applicationName())
&& Objects.equals(deploymentGroupId(), other.deploymentGroupId())
&& Objects.equals(deploymentGroupName(), other.deploymentGroupName())
&& Objects.equals(deploymentConfigName(), other.deploymentConfigName())
&& Objects.equals(ec2TagFilters(), other.ec2TagFilters())
&& Objects.equals(onPremisesInstanceTagFilters(), other.onPremisesInstanceTagFilters())
&& Objects.equals(autoScalingGroups(), other.autoScalingGroups())
&& Objects.equals(serviceRoleArn(), other.serviceRoleArn())
&& Objects.equals(targetRevision(), other.targetRevision())
&& Objects.equals(triggerConfigurations(), other.triggerConfigurations())
&& Objects.equals(alarmConfiguration(), other.alarmConfiguration())
&& Objects.equals(autoRollbackConfiguration(), other.autoRollbackConfiguration())
&& Objects.equals(deploymentStyle(), other.deploymentStyle())
&& Objects.equals(blueGreenDeploymentConfiguration(), other.blueGreenDeploymentConfiguration())
&& Objects.equals(loadBalancerInfo(), other.loadBalancerInfo())
&& Objects.equals(lastSuccessfulDeployment(), other.lastSuccessfulDeployment())
&& Objects.equals(lastAttemptedDeployment(), other.lastAttemptedDeployment())
&& Objects.equals(ec2TagSet(), other.ec2TagSet()) && Objects.equals(onPremisesTagSet(), other.onPremisesTagSet())
&& Objects.equals(computePlatformAsString(), other.computePlatformAsString())
&& Objects.equals(ecsServices(), other.ecsServices());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DeploymentGroupInfo").add("ApplicationName", applicationName())
.add("DeploymentGroupId", deploymentGroupId()).add("DeploymentGroupName", deploymentGroupName())
.add("DeploymentConfigName", deploymentConfigName()).add("Ec2TagFilters", ec2TagFilters())
.add("OnPremisesInstanceTagFilters", onPremisesInstanceTagFilters())
.add("AutoScalingGroups", autoScalingGroups()).add("ServiceRoleArn", serviceRoleArn())
.add("TargetRevision", targetRevision()).add("TriggerConfigurations", triggerConfigurations())
.add("AlarmConfiguration", alarmConfiguration()).add("AutoRollbackConfiguration", autoRollbackConfiguration())
.add("DeploymentStyle", deploymentStyle())
.add("BlueGreenDeploymentConfiguration", blueGreenDeploymentConfiguration())
.add("LoadBalancerInfo", loadBalancerInfo()).add("LastSuccessfulDeployment", lastSuccessfulDeployment())
.add("LastAttemptedDeployment", lastAttemptedDeployment()).add("Ec2TagSet", ec2TagSet())
.add("OnPremisesTagSet", onPremisesTagSet()).add("ComputePlatform", computePlatformAsString())
.add("EcsServices", ecsServices()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "applicationName":
return Optional.ofNullable(clazz.cast(applicationName()));
case "deploymentGroupId":
return Optional.ofNullable(clazz.cast(deploymentGroupId()));
case "deploymentGroupName":
return Optional.ofNullable(clazz.cast(deploymentGroupName()));
case "deploymentConfigName":
return Optional.ofNullable(clazz.cast(deploymentConfigName()));
case "ec2TagFilters":
return Optional.ofNullable(clazz.cast(ec2TagFilters()));
case "onPremisesInstanceTagFilters":
return Optional.ofNullable(clazz.cast(onPremisesInstanceTagFilters()));
case "autoScalingGroups":
return Optional.ofNullable(clazz.cast(autoScalingGroups()));
case "serviceRoleArn":
return Optional.ofNullable(clazz.cast(serviceRoleArn()));
case "targetRevision":
return Optional.ofNullable(clazz.cast(targetRevision()));
case "triggerConfigurations":
return Optional.ofNullable(clazz.cast(triggerConfigurations()));
case "alarmConfiguration":
return Optional.ofNullable(clazz.cast(alarmConfiguration()));
case "autoRollbackConfiguration":
return Optional.ofNullable(clazz.cast(autoRollbackConfiguration()));
case "deploymentStyle":
return Optional.ofNullable(clazz.cast(deploymentStyle()));
case "blueGreenDeploymentConfiguration":
return Optional.ofNullable(clazz.cast(blueGreenDeploymentConfiguration()));
case "loadBalancerInfo":
return Optional.ofNullable(clazz.cast(loadBalancerInfo()));
case "lastSuccessfulDeployment":
return Optional.ofNullable(clazz.cast(lastSuccessfulDeployment()));
case "lastAttemptedDeployment":
return Optional.ofNullable(clazz.cast(lastAttemptedDeployment()));
case "ec2TagSet":
return Optional.ofNullable(clazz.cast(ec2TagSet()));
case "onPremisesTagSet":
return Optional.ofNullable(clazz.cast(onPremisesTagSet()));
case "computePlatform":
return Optional.ofNullable(clazz.cast(computePlatformAsString()));
case "ecsServices":
return Optional.ofNullable(clazz.cast(ecsServices()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function