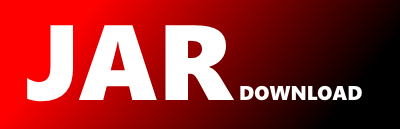
software.amazon.awssdk.services.codegurureviewer.CodeGuruReviewerClient Maven / Gradle / Ivy
Show all versions of codegurureviewer Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codegurureviewer;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.codegurureviewer.model.AccessDeniedException;
import software.amazon.awssdk.services.codegurureviewer.model.AssociateRepositoryRequest;
import software.amazon.awssdk.services.codegurureviewer.model.AssociateRepositoryResponse;
import software.amazon.awssdk.services.codegurureviewer.model.CodeGuruReviewerException;
import software.amazon.awssdk.services.codegurureviewer.model.ConflictException;
import software.amazon.awssdk.services.codegurureviewer.model.DescribeRepositoryAssociationRequest;
import software.amazon.awssdk.services.codegurureviewer.model.DescribeRepositoryAssociationResponse;
import software.amazon.awssdk.services.codegurureviewer.model.DisassociateRepositoryRequest;
import software.amazon.awssdk.services.codegurureviewer.model.DisassociateRepositoryResponse;
import software.amazon.awssdk.services.codegurureviewer.model.InternalServerException;
import software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsRequest;
import software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsResponse;
import software.amazon.awssdk.services.codegurureviewer.model.NotFoundException;
import software.amazon.awssdk.services.codegurureviewer.model.ThrottlingException;
import software.amazon.awssdk.services.codegurureviewer.model.ValidationException;
import software.amazon.awssdk.services.codegurureviewer.paginators.ListRepositoryAssociationsIterable;
/**
* Service client for accessing CodeGuruReviewer. This can be created using the static {@link #builder()} method.
*
*
* This section provides documentation for the Amazon CodeGuru Reviewer API operations.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface CodeGuruReviewerClient extends SdkClient {
String SERVICE_NAME = "codeguru-reviewer";
/**
* Create a {@link CodeGuruReviewerClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CodeGuruReviewerClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CodeGuruReviewerClient}.
*/
static CodeGuruReviewerClientBuilder builder() {
return new DefaultCodeGuruReviewerClientBuilder();
}
/**
*
* Associates an AWS CodeCommit repository with Amazon CodeGuru Reviewer. When you associate an AWS CodeCommit
* repository with Amazon CodeGuru Reviewer, Amazon CodeGuru Reviewer will provide recommendations for each pull
* request. You can view recommendations in the AWS CodeCommit repository.
*
*
* You can associate a GitHub repository using the Amazon CodeGuru Reviewer console.
*
*
* @param associateRepositoryRequest
* @return Result of the AssociateRepository operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.AssociateRepository
* @see AWS API Documentation
*/
default AssociateRepositoryResponse associateRepository(AssociateRepositoryRequest associateRepositoryRequest)
throws InternalServerException, ValidationException, AccessDeniedException, ConflictException, ThrottlingException,
AwsServiceException, SdkClientException, CodeGuruReviewerException {
throw new UnsupportedOperationException();
}
/**
*
* Associates an AWS CodeCommit repository with Amazon CodeGuru Reviewer. When you associate an AWS CodeCommit
* repository with Amazon CodeGuru Reviewer, Amazon CodeGuru Reviewer will provide recommendations for each pull
* request. You can view recommendations in the AWS CodeCommit repository.
*
*
* You can associate a GitHub repository using the Amazon CodeGuru Reviewer console.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateRepositoryRequest.Builder} avoiding the
* need to create one manually via {@link AssociateRepositoryRequest#builder()}
*
*
* @param associateRepositoryRequest
* A {@link Consumer} that will call methods on {@link AssociateRepositoryRequest.Builder} to create a
* request.
* @return Result of the AssociateRepository operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.AssociateRepository
* @see AWS API Documentation
*/
default AssociateRepositoryResponse associateRepository(
Consumer associateRepositoryRequest) throws InternalServerException,
ValidationException, AccessDeniedException, ConflictException, ThrottlingException, AwsServiceException,
SdkClientException, CodeGuruReviewerException {
return associateRepository(AssociateRepositoryRequest.builder().applyMutation(associateRepositoryRequest).build());
}
/**
*
* Describes a repository association.
*
*
* @param describeRepositoryAssociationRequest
* @return Result of the DescribeRepositoryAssociation operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.DescribeRepositoryAssociation
* @see AWS API Documentation
*/
default DescribeRepositoryAssociationResponse describeRepositoryAssociation(
DescribeRepositoryAssociationRequest describeRepositoryAssociationRequest) throws NotFoundException,
InternalServerException, ValidationException, AccessDeniedException, ThrottlingException, AwsServiceException,
SdkClientException, CodeGuruReviewerException {
throw new UnsupportedOperationException();
}
/**
*
* Describes a repository association.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoryAssociationRequest.Builder}
* avoiding the need to create one manually via {@link DescribeRepositoryAssociationRequest#builder()}
*
*
* @param describeRepositoryAssociationRequest
* A {@link Consumer} that will call methods on {@link DescribeRepositoryAssociationRequest.Builder} to
* create a request.
* @return Result of the DescribeRepositoryAssociation operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.DescribeRepositoryAssociation
* @see AWS API Documentation
*/
default DescribeRepositoryAssociationResponse describeRepositoryAssociation(
Consumer describeRepositoryAssociationRequest)
throws NotFoundException, InternalServerException, ValidationException, AccessDeniedException, ThrottlingException,
AwsServiceException, SdkClientException, CodeGuruReviewerException {
return describeRepositoryAssociation(DescribeRepositoryAssociationRequest.builder()
.applyMutation(describeRepositoryAssociationRequest).build());
}
/**
*
* Removes the association between Amazon CodeGuru Reviewer and a repository.
*
*
* @param disassociateRepositoryRequest
* @return Result of the DisassociateRepository operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.DisassociateRepository
* @see AWS API Documentation
*/
default DisassociateRepositoryResponse disassociateRepository(DisassociateRepositoryRequest disassociateRepositoryRequest)
throws NotFoundException, InternalServerException, ValidationException, AccessDeniedException, ConflictException,
ThrottlingException, AwsServiceException, SdkClientException, CodeGuruReviewerException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the association between Amazon CodeGuru Reviewer and a repository.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateRepositoryRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateRepositoryRequest#builder()}
*
*
* @param disassociateRepositoryRequest
* A {@link Consumer} that will call methods on {@link DisassociateRepositoryRequest.Builder} to create a
* request.
* @return Result of the DisassociateRepository operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.DisassociateRepository
* @see AWS API Documentation
*/
default DisassociateRepositoryResponse disassociateRepository(
Consumer disassociateRepositoryRequest) throws NotFoundException,
InternalServerException, ValidationException, AccessDeniedException, ConflictException, ThrottlingException,
AwsServiceException, SdkClientException, CodeGuruReviewerException {
return disassociateRepository(DisassociateRepositoryRequest.builder().applyMutation(disassociateRepositoryRequest)
.build());
}
/**
*
* Lists repository associations. You can optionally filter on one or more of the following recommendation
* properties: provider types, states, names, and owners.
*
*
* @param listRepositoryAssociationsRequest
* @return Result of the ListRepositoryAssociations operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.ListRepositoryAssociations
* @see AWS API Documentation
*/
default ListRepositoryAssociationsResponse listRepositoryAssociations(
ListRepositoryAssociationsRequest listRepositoryAssociationsRequest) throws InternalServerException,
ValidationException, ThrottlingException, AwsServiceException, SdkClientException, CodeGuruReviewerException {
throw new UnsupportedOperationException();
}
/**
*
* Lists repository associations. You can optionally filter on one or more of the following recommendation
* properties: provider types, states, names, and owners.
*
*
*
* This is a convenience which creates an instance of the {@link ListRepositoryAssociationsRequest.Builder} avoiding
* the need to create one manually via {@link ListRepositoryAssociationsRequest#builder()}
*
*
* @param listRepositoryAssociationsRequest
* A {@link Consumer} that will call methods on {@link ListRepositoryAssociationsRequest.Builder} to create a
* request.
* @return Result of the ListRepositoryAssociations operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.ListRepositoryAssociations
* @see AWS API Documentation
*/
default ListRepositoryAssociationsResponse listRepositoryAssociations(
Consumer listRepositoryAssociationsRequest)
throws InternalServerException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
CodeGuruReviewerException {
return listRepositoryAssociations(ListRepositoryAssociationsRequest.builder()
.applyMutation(listRepositoryAssociationsRequest).build());
}
/**
*
* Lists repository associations. You can optionally filter on one or more of the following recommendation
* properties: provider types, states, names, and owners.
*
*
*
* This is a variant of
* {@link #listRepositoryAssociations(software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codegurureviewer.paginators.ListRepositoryAssociationsIterable responses = client.listRepositoryAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codegurureviewer.paginators.ListRepositoryAssociationsIterable responses = client
* .listRepositoryAssociationsPaginator(request);
* for (software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codegurureviewer.paginators.ListRepositoryAssociationsIterable responses = client.listRepositoryAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositoryAssociations(software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsRequest)}
* operation.
*
*
* @param listRepositoryAssociationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.ListRepositoryAssociations
* @see AWS API Documentation
*/
default ListRepositoryAssociationsIterable listRepositoryAssociationsPaginator(
ListRepositoryAssociationsRequest listRepositoryAssociationsRequest) throws InternalServerException,
ValidationException, ThrottlingException, AwsServiceException, SdkClientException, CodeGuruReviewerException {
throw new UnsupportedOperationException();
}
/**
*
* Lists repository associations. You can optionally filter on one or more of the following recommendation
* properties: provider types, states, names, and owners.
*
*
*
* This is a variant of
* {@link #listRepositoryAssociations(software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codegurureviewer.paginators.ListRepositoryAssociationsIterable responses = client.listRepositoryAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codegurureviewer.paginators.ListRepositoryAssociationsIterable responses = client
* .listRepositoryAssociationsPaginator(request);
* for (software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codegurureviewer.paginators.ListRepositoryAssociationsIterable responses = client.listRepositoryAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRepositoryAssociations(software.amazon.awssdk.services.codegurureviewer.model.ListRepositoryAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListRepositoryAssociationsRequest.Builder} avoiding
* the need to create one manually via {@link ListRepositoryAssociationsRequest#builder()}
*
*
* @param listRepositoryAssociationsRequest
* A {@link Consumer} that will call methods on {@link ListRepositoryAssociationsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The input fails to satisfy the specified constraints.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeGuruReviewerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeGuruReviewerClient.ListRepositoryAssociations
* @see AWS API Documentation
*/
default ListRepositoryAssociationsIterable listRepositoryAssociationsPaginator(
Consumer listRepositoryAssociationsRequest)
throws InternalServerException, ValidationException, ThrottlingException, AwsServiceException, SdkClientException,
CodeGuruReviewerException {
return listRepositoryAssociationsPaginator(ListRepositoryAssociationsRequest.builder()
.applyMutation(listRepositoryAssociationsRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("codeguru-reviewer");
}
}