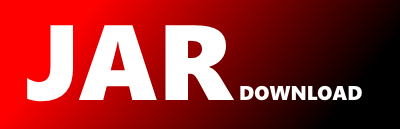
software.amazon.awssdk.services.codegurureviewer.model.MetricsSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codegurureviewer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about metrics summaries.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MetricsSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField METERED_LINES_OF_CODE_COUNT_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("MeteredLinesOfCodeCount").getter(getter(MetricsSummary::meteredLinesOfCodeCount))
.setter(setter(Builder::meteredLinesOfCodeCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MeteredLinesOfCodeCount").build())
.build();
private static final SdkField SUPPRESSED_LINES_OF_CODE_COUNT_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("SuppressedLinesOfCodeCount")
.getter(getter(MetricsSummary::suppressedLinesOfCodeCount))
.setter(setter(Builder::suppressedLinesOfCodeCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SuppressedLinesOfCodeCount").build())
.build();
private static final SdkField FINDINGS_COUNT_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("FindingsCount").getter(getter(MetricsSummary::findingsCount)).setter(setter(Builder::findingsCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FindingsCount").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
METERED_LINES_OF_CODE_COUNT_FIELD, SUPPRESSED_LINES_OF_CODE_COUNT_FIELD, FINDINGS_COUNT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final Long meteredLinesOfCodeCount;
private final Long suppressedLinesOfCodeCount;
private final Long findingsCount;
private MetricsSummary(BuilderImpl builder) {
this.meteredLinesOfCodeCount = builder.meteredLinesOfCodeCount;
this.suppressedLinesOfCodeCount = builder.suppressedLinesOfCodeCount;
this.findingsCount = builder.findingsCount;
}
/**
*
* Lines of code metered in the code review. For the initial code review pull request and all subsequent revisions,
* this includes all lines of code in the files added to the pull request. In subsequent revisions, for files that
* already existed in the pull request, this includes only the changed lines of code. In both cases, this does not
* include non-code lines such as comments and import statements. For example, if you submit a pull request
* containing 5 files, each with 500 lines of code, and in a subsequent revision you added a new file with 200 lines
* of code, and also modified a total of 25 lines across the initial 5 files, MeteredLinesOfCodeCount
* includes the first 5 files (5 * 500 = 2,500 lines), the new file (200 lines) and the 25 changed lines of code for
* a total of 2,725 lines of code.
*
*
* @return Lines of code metered in the code review. For the initial code review pull request and all subsequent
* revisions, this includes all lines of code in the files added to the pull request. In subsequent
* revisions, for files that already existed in the pull request, this includes only the changed lines of
* code. In both cases, this does not include non-code lines such as comments and import statements. For
* example, if you submit a pull request containing 5 files, each with 500 lines of code, and in a
* subsequent revision you added a new file with 200 lines of code, and also modified a total of 25 lines
* across the initial 5 files, MeteredLinesOfCodeCount
includes the first 5 files (5 * 500 =
* 2,500 lines), the new file (200 lines) and the 25 changed lines of code for a total of 2,725 lines of
* code.
*/
public final Long meteredLinesOfCodeCount() {
return meteredLinesOfCodeCount;
}
/**
*
* Lines of code suppressed in the code review based on the excludeFiles
element in the
* aws-codeguru-reviewer.yml
file. For full repository analyses, this number includes all lines of code
* in the files that are suppressed. For pull requests, this number only includes the changed lines of code
* that are suppressed. In both cases, this number does not include non-code lines such as comments and import
* statements. For example, if you initiate a full repository analysis on a repository containing 5 files, each file
* with 100 lines of code, and 2 files are listed as excluded in the aws-codeguru-reviewer.yml
file,
* then SuppressedLinesOfCodeCount
returns 200 (2 * 100) as the total number of lines of code
* suppressed. However, if you submit a pull request for the same repository, then
* SuppressedLinesOfCodeCount
only includes the lines in the 2 files that changed. If only 1 of the 2
* files changed in the pull request, then SuppressedLinesOfCodeCount
returns 100 (1 * 100) as the
* total number of lines of code suppressed.
*
*
* @return Lines of code suppressed in the code review based on the excludeFiles
element in the
* aws-codeguru-reviewer.yml
file. For full repository analyses, this number includes all lines
* of code in the files that are suppressed. For pull requests, this number only includes the changed
* lines of code that are suppressed. In both cases, this number does not include non-code lines such as
* comments and import statements. For example, if you initiate a full repository analysis on a repository
* containing 5 files, each file with 100 lines of code, and 2 files are listed as excluded in the
* aws-codeguru-reviewer.yml
file, then SuppressedLinesOfCodeCount
returns 200 (2
* * 100) as the total number of lines of code suppressed. However, if you submit a pull request for the
* same repository, then SuppressedLinesOfCodeCount
only includes the lines in the 2 files that
* changed. If only 1 of the 2 files changed in the pull request, then
* SuppressedLinesOfCodeCount
returns 100 (1 * 100) as the total number of lines of code
* suppressed.
*/
public final Long suppressedLinesOfCodeCount() {
return suppressedLinesOfCodeCount;
}
/**
*
* Total number of recommendations found in the code review.
*
*
* @return Total number of recommendations found in the code review.
*/
public final Long findingsCount() {
return findingsCount;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(meteredLinesOfCodeCount());
hashCode = 31 * hashCode + Objects.hashCode(suppressedLinesOfCodeCount());
hashCode = 31 * hashCode + Objects.hashCode(findingsCount());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MetricsSummary)) {
return false;
}
MetricsSummary other = (MetricsSummary) obj;
return Objects.equals(meteredLinesOfCodeCount(), other.meteredLinesOfCodeCount())
&& Objects.equals(suppressedLinesOfCodeCount(), other.suppressedLinesOfCodeCount())
&& Objects.equals(findingsCount(), other.findingsCount());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MetricsSummary").add("MeteredLinesOfCodeCount", meteredLinesOfCodeCount())
.add("SuppressedLinesOfCodeCount", suppressedLinesOfCodeCount()).add("FindingsCount", findingsCount()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MeteredLinesOfCodeCount":
return Optional.ofNullable(clazz.cast(meteredLinesOfCodeCount()));
case "SuppressedLinesOfCodeCount":
return Optional.ofNullable(clazz.cast(suppressedLinesOfCodeCount()));
case "FindingsCount":
return Optional.ofNullable(clazz.cast(findingsCount()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("MeteredLinesOfCodeCount", METERED_LINES_OF_CODE_COUNT_FIELD);
map.put("SuppressedLinesOfCodeCount", SUPPRESSED_LINES_OF_CODE_COUNT_FIELD);
map.put("FindingsCount", FINDINGS_COUNT_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function