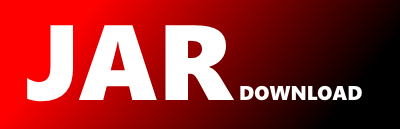
software.amazon.awssdk.services.codepipeline.model.PipelineSummary Maven / Gradle / Ivy
Show all versions of codepipeline Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codepipeline.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Returns a summary of a pipeline.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PipelineSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(PipelineSummary::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("version").getter(getter(PipelineSummary::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField PIPELINE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("pipelineType").getter(getter(PipelineSummary::pipelineTypeAsString))
.setter(setter(Builder::pipelineType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pipelineType").build()).build();
private static final SdkField EXECUTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("executionMode").getter(getter(PipelineSummary::executionModeAsString))
.setter(setter(Builder::executionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionMode").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("created").getter(getter(PipelineSummary::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("created").build()).build();
private static final SdkField UPDATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updated").getter(getter(PipelineSummary::updated)).setter(setter(Builder::updated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updated").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, VERSION_FIELD,
PIPELINE_TYPE_FIELD, EXECUTION_MODE_FIELD, CREATED_FIELD, UPDATED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("name", NAME_FIELD);
put("version", VERSION_FIELD);
put("pipelineType", PIPELINE_TYPE_FIELD);
put("executionMode", EXECUTION_MODE_FIELD);
put("created", CREATED_FIELD);
put("updated", UPDATED_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String name;
private final Integer version;
private final String pipelineType;
private final String executionMode;
private final Instant created;
private final Instant updated;
private PipelineSummary(BuilderImpl builder) {
this.name = builder.name;
this.version = builder.version;
this.pipelineType = builder.pipelineType;
this.executionMode = builder.executionMode;
this.created = builder.created;
this.updated = builder.updated;
}
/**
*
* The name of the pipeline.
*
*
* @return The name of the pipeline.
*/
public final String name() {
return name;
}
/**
*
* The version number of the pipeline.
*
*
* @return The version number of the pipeline.
*/
public final Integer version() {
return version;
}
/**
*
* CodePipeline provides the following pipeline types, which differ in characteristics and price, so that you can
* tailor your pipeline features and cost to the needs of your applications.
*
*
* -
*
* V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level parameters.
*
*
* -
*
* V2 type pipelines have the same structure as a V1 type, along with additional parameters for release safety and
* trigger configuration.
*
*
*
*
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a pipeline
* will result in the pipeline having the V2 type of pipeline and the associated costs.
*
*
*
* For information about pricing for CodePipeline, see Pricing.
*
*
* For information about which type of pipeline to choose, see What type of
* pipeline is right for me?.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pipelineType} will
* return {@link PipelineType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pipelineTypeAsString}.
*
*
* @return CodePipeline provides the following pipeline types, which differ in characteristics and price, so that
* you can tailor your pipeline features and cost to the needs of your applications.
*
* -
*
* V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level
* parameters.
*
*
* -
*
* V2 type pipelines have the same structure as a V1 type, along with additional parameters for release
* safety and trigger configuration.
*
*
*
*
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a
* pipeline will result in the pipeline having the V2 type of pipeline and the associated costs.
*
*
*
* For information about pricing for CodePipeline, see Pricing.
*
*
* For information about which type of pipeline to choose, see What type
* of pipeline is right for me?.
* @see PipelineType
*/
public final PipelineType pipelineType() {
return PipelineType.fromValue(pipelineType);
}
/**
*
* CodePipeline provides the following pipeline types, which differ in characteristics and price, so that you can
* tailor your pipeline features and cost to the needs of your applications.
*
*
* -
*
* V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level parameters.
*
*
* -
*
* V2 type pipelines have the same structure as a V1 type, along with additional parameters for release safety and
* trigger configuration.
*
*
*
*
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a pipeline
* will result in the pipeline having the V2 type of pipeline and the associated costs.
*
*
*
* For information about pricing for CodePipeline, see Pricing.
*
*
* For information about which type of pipeline to choose, see What type of
* pipeline is right for me?.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pipelineType} will
* return {@link PipelineType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pipelineTypeAsString}.
*
*
* @return CodePipeline provides the following pipeline types, which differ in characteristics and price, so that
* you can tailor your pipeline features and cost to the needs of your applications.
*
* -
*
* V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level
* parameters.
*
*
* -
*
* V2 type pipelines have the same structure as a V1 type, along with additional parameters for release
* safety and trigger configuration.
*
*
*
*
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a
* pipeline will result in the pipeline having the V2 type of pipeline and the associated costs.
*
*
*
* For information about pricing for CodePipeline, see Pricing.
*
*
* For information about which type of pipeline to choose, see What type
* of pipeline is right for me?.
* @see PipelineType
*/
public final String pipelineTypeAsString() {
return pipelineType;
}
/**
*
* The method that the pipeline will use to handle multiple executions. The default mode is SUPERSEDED.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #executionMode}
* will return {@link ExecutionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #executionModeAsString}.
*
*
* @return The method that the pipeline will use to handle multiple executions. The default mode is SUPERSEDED.
* @see ExecutionMode
*/
public final ExecutionMode executionMode() {
return ExecutionMode.fromValue(executionMode);
}
/**
*
* The method that the pipeline will use to handle multiple executions. The default mode is SUPERSEDED.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #executionMode}
* will return {@link ExecutionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #executionModeAsString}.
*
*
* @return The method that the pipeline will use to handle multiple executions. The default mode is SUPERSEDED.
* @see ExecutionMode
*/
public final String executionModeAsString() {
return executionMode;
}
/**
*
* The date and time the pipeline was created, in timestamp format.
*
*
* @return The date and time the pipeline was created, in timestamp format.
*/
public final Instant created() {
return created;
}
/**
*
* The date and time of the last update to the pipeline, in timestamp format.
*
*
* @return The date and time of the last update to the pipeline, in timestamp format.
*/
public final Instant updated() {
return updated;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(pipelineTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(executionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(updated());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PipelineSummary)) {
return false;
}
PipelineSummary other = (PipelineSummary) obj;
return Objects.equals(name(), other.name()) && Objects.equals(version(), other.version())
&& Objects.equals(pipelineTypeAsString(), other.pipelineTypeAsString())
&& Objects.equals(executionModeAsString(), other.executionModeAsString())
&& Objects.equals(created(), other.created()) && Objects.equals(updated(), other.updated());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PipelineSummary").add("Name", name()).add("Version", version())
.add("PipelineType", pipelineTypeAsString()).add("ExecutionMode", executionModeAsString())
.add("Created", created()).add("Updated", updated()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "pipelineType":
return Optional.ofNullable(clazz.cast(pipelineTypeAsString()));
case "executionMode":
return Optional.ofNullable(clazz.cast(executionModeAsString()));
case "created":
return Optional.ofNullable(clazz.cast(created()));
case "updated":
return Optional.ofNullable(clazz.cast(updated()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function