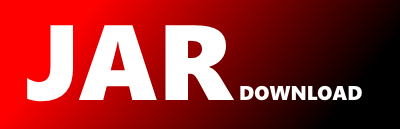
software.amazon.awssdk.services.codepipeline.model.RuleExecutionDetail Maven / Gradle / Ivy
Show all versions of codepipeline Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codepipeline.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of the runs for a rule and the results produced on an artifact as it passes through stages in the
* pipeline.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RuleExecutionDetail implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField PIPELINE_EXECUTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("pipelineExecutionId").getter(getter(RuleExecutionDetail::pipelineExecutionId))
.setter(setter(Builder::pipelineExecutionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pipelineExecutionId").build())
.build();
private static final SdkField RULE_EXECUTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ruleExecutionId").getter(getter(RuleExecutionDetail::ruleExecutionId))
.setter(setter(Builder::ruleExecutionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ruleExecutionId").build()).build();
private static final SdkField PIPELINE_VERSION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("pipelineVersion").getter(getter(RuleExecutionDetail::pipelineVersion))
.setter(setter(Builder::pipelineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pipelineVersion").build()).build();
private static final SdkField STAGE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("stageName").getter(getter(RuleExecutionDetail::stageName)).setter(setter(Builder::stageName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stageName").build()).build();
private static final SdkField RULE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ruleName").getter(getter(RuleExecutionDetail::ruleName)).setter(setter(Builder::ruleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ruleName").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startTime").getter(getter(RuleExecutionDetail::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField LAST_UPDATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastUpdateTime").getter(getter(RuleExecutionDetail::lastUpdateTime))
.setter(setter(Builder::lastUpdateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastUpdateTime").build()).build();
private static final SdkField UPDATED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("updatedBy").getter(getter(RuleExecutionDetail::updatedBy)).setter(setter(Builder::updatedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedBy").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(RuleExecutionDetail::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField INPUT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("input")
.getter(getter(RuleExecutionDetail::input)).setter(setter(Builder::input)).constructor(RuleExecutionInput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("input").build()).build();
private static final SdkField OUTPUT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("output")
.getter(getter(RuleExecutionDetail::output)).setter(setter(Builder::output))
.constructor(RuleExecutionOutput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("output").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PIPELINE_EXECUTION_ID_FIELD,
RULE_EXECUTION_ID_FIELD, PIPELINE_VERSION_FIELD, STAGE_NAME_FIELD, RULE_NAME_FIELD, START_TIME_FIELD,
LAST_UPDATE_TIME_FIELD, UPDATED_BY_FIELD, STATUS_FIELD, INPUT_FIELD, OUTPUT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("pipelineExecutionId", PIPELINE_EXECUTION_ID_FIELD);
put("ruleExecutionId", RULE_EXECUTION_ID_FIELD);
put("pipelineVersion", PIPELINE_VERSION_FIELD);
put("stageName", STAGE_NAME_FIELD);
put("ruleName", RULE_NAME_FIELD);
put("startTime", START_TIME_FIELD);
put("lastUpdateTime", LAST_UPDATE_TIME_FIELD);
put("updatedBy", UPDATED_BY_FIELD);
put("status", STATUS_FIELD);
put("input", INPUT_FIELD);
put("output", OUTPUT_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String pipelineExecutionId;
private final String ruleExecutionId;
private final Integer pipelineVersion;
private final String stageName;
private final String ruleName;
private final Instant startTime;
private final Instant lastUpdateTime;
private final String updatedBy;
private final String status;
private final RuleExecutionInput input;
private final RuleExecutionOutput output;
private RuleExecutionDetail(BuilderImpl builder) {
this.pipelineExecutionId = builder.pipelineExecutionId;
this.ruleExecutionId = builder.ruleExecutionId;
this.pipelineVersion = builder.pipelineVersion;
this.stageName = builder.stageName;
this.ruleName = builder.ruleName;
this.startTime = builder.startTime;
this.lastUpdateTime = builder.lastUpdateTime;
this.updatedBy = builder.updatedBy;
this.status = builder.status;
this.input = builder.input;
this.output = builder.output;
}
/**
*
* The ID of the pipeline execution in the stage where the rule was run. Use the GetPipelineState action to
* retrieve the current pipelineExecutionId of the stage.
*
*
* @return The ID of the pipeline execution in the stage where the rule was run. Use the GetPipelineState
* action to retrieve the current pipelineExecutionId of the stage.
*/
public final String pipelineExecutionId() {
return pipelineExecutionId;
}
/**
*
* The ID of the run for the rule.
*
*
* @return The ID of the run for the rule.
*/
public final String ruleExecutionId() {
return ruleExecutionId;
}
/**
*
* The version number of the pipeline with the stage where the rule was run.
*
*
* @return The version number of the pipeline with the stage where the rule was run.
*/
public final Integer pipelineVersion() {
return pipelineVersion;
}
/**
*
* The name of the stage where the rule was run.
*
*
* @return The name of the stage where the rule was run.
*/
public final String stageName() {
return stageName;
}
/**
*
* The name of the rule that was run in the stage.
*
*
* @return The name of the rule that was run in the stage.
*/
public final String ruleName() {
return ruleName;
}
/**
*
* The start time of the rule execution.
*
*
* @return The start time of the rule execution.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The date and time of the last change to the rule execution, in timestamp format.
*
*
* @return The date and time of the last change to the rule execution, in timestamp format.
*/
public final Instant lastUpdateTime() {
return lastUpdateTime;
}
/**
*
* The ARN of the user who changed the rule execution details.
*
*
* @return The ARN of the user who changed the rule execution details.
*/
public final String updatedBy() {
return updatedBy;
}
/**
*
* The status of the rule execution. Status categories are InProgress
, Succeeded
, and
* Failed
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RuleExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the rule execution. Status categories are InProgress
, Succeeded
,
* and Failed
.
* @see RuleExecutionStatus
*/
public final RuleExecutionStatus status() {
return RuleExecutionStatus.fromValue(status);
}
/**
*
* The status of the rule execution. Status categories are InProgress
, Succeeded
, and
* Failed
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RuleExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the rule execution. Status categories are InProgress
, Succeeded
,
* and Failed
.
* @see RuleExecutionStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* Input details for the rule execution, such as role ARN, Region, and input artifacts.
*
*
* @return Input details for the rule execution, such as role ARN, Region, and input artifacts.
*/
public final RuleExecutionInput input() {
return input;
}
/**
*
* Output details for the rule execution, such as the rule execution result.
*
*
* @return Output details for the rule execution, such as the rule execution result.
*/
public final RuleExecutionOutput output() {
return output;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(pipelineExecutionId());
hashCode = 31 * hashCode + Objects.hashCode(ruleExecutionId());
hashCode = 31 * hashCode + Objects.hashCode(pipelineVersion());
hashCode = 31 * hashCode + Objects.hashCode(stageName());
hashCode = 31 * hashCode + Objects.hashCode(ruleName());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdateTime());
hashCode = 31 * hashCode + Objects.hashCode(updatedBy());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(input());
hashCode = 31 * hashCode + Objects.hashCode(output());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RuleExecutionDetail)) {
return false;
}
RuleExecutionDetail other = (RuleExecutionDetail) obj;
return Objects.equals(pipelineExecutionId(), other.pipelineExecutionId())
&& Objects.equals(ruleExecutionId(), other.ruleExecutionId())
&& Objects.equals(pipelineVersion(), other.pipelineVersion()) && Objects.equals(stageName(), other.stageName())
&& Objects.equals(ruleName(), other.ruleName()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(lastUpdateTime(), other.lastUpdateTime()) && Objects.equals(updatedBy(), other.updatedBy())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(input(), other.input())
&& Objects.equals(output(), other.output());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RuleExecutionDetail").add("PipelineExecutionId", pipelineExecutionId())
.add("RuleExecutionId", ruleExecutionId()).add("PipelineVersion", pipelineVersion())
.add("StageName", stageName()).add("RuleName", ruleName()).add("StartTime", startTime())
.add("LastUpdateTime", lastUpdateTime()).add("UpdatedBy", updatedBy()).add("Status", statusAsString())
.add("Input", input()).add("Output", output()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "pipelineExecutionId":
return Optional.ofNullable(clazz.cast(pipelineExecutionId()));
case "ruleExecutionId":
return Optional.ofNullable(clazz.cast(ruleExecutionId()));
case "pipelineVersion":
return Optional.ofNullable(clazz.cast(pipelineVersion()));
case "stageName":
return Optional.ofNullable(clazz.cast(stageName()));
case "ruleName":
return Optional.ofNullable(clazz.cast(ruleName()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "lastUpdateTime":
return Optional.ofNullable(clazz.cast(lastUpdateTime()));
case "updatedBy":
return Optional.ofNullable(clazz.cast(updatedBy()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "input":
return Optional.ofNullable(clazz.cast(input()));
case "output":
return Optional.ofNullable(clazz.cast(output()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function