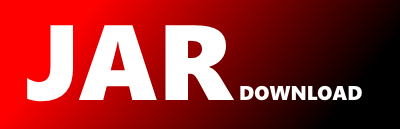
software.amazon.awssdk.services.codestar.CodeStarAsyncClient Maven / Gradle / Ivy
Show all versions of codestar Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codestar;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.codestar.model.AssociateTeamMemberRequest;
import software.amazon.awssdk.services.codestar.model.AssociateTeamMemberResponse;
import software.amazon.awssdk.services.codestar.model.CreateProjectRequest;
import software.amazon.awssdk.services.codestar.model.CreateProjectResponse;
import software.amazon.awssdk.services.codestar.model.CreateUserProfileRequest;
import software.amazon.awssdk.services.codestar.model.CreateUserProfileResponse;
import software.amazon.awssdk.services.codestar.model.DeleteProjectRequest;
import software.amazon.awssdk.services.codestar.model.DeleteProjectResponse;
import software.amazon.awssdk.services.codestar.model.DeleteUserProfileRequest;
import software.amazon.awssdk.services.codestar.model.DeleteUserProfileResponse;
import software.amazon.awssdk.services.codestar.model.DescribeProjectRequest;
import software.amazon.awssdk.services.codestar.model.DescribeProjectResponse;
import software.amazon.awssdk.services.codestar.model.DescribeUserProfileRequest;
import software.amazon.awssdk.services.codestar.model.DescribeUserProfileResponse;
import software.amazon.awssdk.services.codestar.model.DisassociateTeamMemberRequest;
import software.amazon.awssdk.services.codestar.model.DisassociateTeamMemberResponse;
import software.amazon.awssdk.services.codestar.model.ListProjectsRequest;
import software.amazon.awssdk.services.codestar.model.ListProjectsResponse;
import software.amazon.awssdk.services.codestar.model.ListResourcesRequest;
import software.amazon.awssdk.services.codestar.model.ListResourcesResponse;
import software.amazon.awssdk.services.codestar.model.ListTagsForProjectRequest;
import software.amazon.awssdk.services.codestar.model.ListTagsForProjectResponse;
import software.amazon.awssdk.services.codestar.model.ListTeamMembersRequest;
import software.amazon.awssdk.services.codestar.model.ListTeamMembersResponse;
import software.amazon.awssdk.services.codestar.model.ListUserProfilesRequest;
import software.amazon.awssdk.services.codestar.model.ListUserProfilesResponse;
import software.amazon.awssdk.services.codestar.model.TagProjectRequest;
import software.amazon.awssdk.services.codestar.model.TagProjectResponse;
import software.amazon.awssdk.services.codestar.model.UntagProjectRequest;
import software.amazon.awssdk.services.codestar.model.UntagProjectResponse;
import software.amazon.awssdk.services.codestar.model.UpdateProjectRequest;
import software.amazon.awssdk.services.codestar.model.UpdateProjectResponse;
import software.amazon.awssdk.services.codestar.model.UpdateTeamMemberRequest;
import software.amazon.awssdk.services.codestar.model.UpdateTeamMemberResponse;
import software.amazon.awssdk.services.codestar.model.UpdateUserProfileRequest;
import software.amazon.awssdk.services.codestar.model.UpdateUserProfileResponse;
/**
* Service client for accessing CodeStar asynchronously. This can be created using the static {@link #builder()} method.
*
* AWS CodeStar
*
* This is the API reference for AWS CodeStar. This reference provides descriptions of the operations and data types for
* the AWS CodeStar API along with usage examples.
*
*
* You can use the AWS CodeStar API to work with:
*
*
* Projects and their resources, by calling the following:
*
*
* -
*
* DeleteProject
, which deletes a project.
*
*
* -
*
* DescribeProject
, which lists the attributes of a project.
*
*
* -
*
* ListProjects
, which lists all projects associated with your AWS account.
*
*
* -
*
* ListResources
, which lists the resources associated with a project.
*
*
* -
*
* ListTagsForProject
, which lists the tags associated with a project.
*
*
* -
*
* TagProject
, which adds tags to a project.
*
*
* -
*
* UntagProject
, which removes tags from a project.
*
*
* -
*
* UpdateProject
, which updates the attributes of a project.
*
*
*
*
* Teams and team members, by calling the following:
*
*
* -
*
* AssociateTeamMember
, which adds an IAM user to the team for a project.
*
*
* -
*
* DisassociateTeamMember
, which removes an IAM user from the team for a project.
*
*
* -
*
* ListTeamMembers
, which lists all the IAM users in the team for a project, including their roles and
* attributes.
*
*
* -
*
* UpdateTeamMember
, which updates a team member's attributes in a project.
*
*
*
*
* Users, by calling the following:
*
*
* -
*
* CreateUserProfile
, which creates a user profile that contains data associated with the user across all
* projects.
*
*
* -
*
* DeleteUserProfile
, which deletes all user profile information across all projects.
*
*
* -
*
* DescribeUserProfile
, which describes the profile of a user.
*
*
* -
*
* ListUserProfiles
, which lists all user profiles.
*
*
* -
*
* UpdateUserProfile
, which updates the profile for a user.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface CodeStarAsyncClient extends SdkClient {
String SERVICE_NAME = "codestar";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "codestar";
/**
* Create a {@link CodeStarAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CodeStarAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CodeStarAsyncClient}.
*/
static CodeStarAsyncClientBuilder builder() {
return new DefaultCodeStarAsyncClientBuilder();
}
/**
*
* Adds an IAM user to the team for an AWS CodeStar project.
*
*
* @param associateTeamMemberRequest
* @return A Java Future containing the result of the AssociateTeamMember operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException A resource limit has been exceeded.
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - TeamMemberAlreadyAssociatedException The team member is already associated with a role in this
* project.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.AssociateTeamMember
* @see AWS
* API Documentation
*/
default CompletableFuture associateTeamMember(
AssociateTeamMemberRequest associateTeamMemberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds an IAM user to the team for an AWS CodeStar project.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateTeamMemberRequest.Builder} avoiding the
* need to create one manually via {@link AssociateTeamMemberRequest#builder()}
*
*
* @param associateTeamMemberRequest
* A {@link Consumer} that will call methods on {@link AssociateTeamMemberRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateTeamMember operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException A resource limit has been exceeded.
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - TeamMemberAlreadyAssociatedException The team member is already associated with a role in this
* project.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.AssociateTeamMember
* @see AWS
* API Documentation
*/
default CompletableFuture associateTeamMember(
Consumer associateTeamMemberRequest) {
return associateTeamMember(AssociateTeamMemberRequest.builder().applyMutation(associateTeamMemberRequest).build());
}
/**
*
* Creates a project, including project resources. This action creates a project based on a submitted project
* request. A set of source code files and a toolchain template file can be included with the project request. If
* these are not provided, an empty project is created.
*
*
* @param createProjectRequest
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectAlreadyExistsException An AWS CodeStar project with the same ID already exists in this region
* for the AWS account. AWS CodeStar project IDs must be unique within a region for the AWS account.
* - LimitExceededException A resource limit has been exceeded.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - ProjectCreationFailedException The project creation request was valid, but a nonspecific exception or
* error occurred during project creation. The project could not be created in AWS CodeStar.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.CreateProject
* @see AWS API
* Documentation
*/
default CompletableFuture createProject(CreateProjectRequest createProjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a project, including project resources. This action creates a project based on a submitted project
* request. A set of source code files and a toolchain template file can be included with the project request. If
* these are not provided, an empty project is created.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProjectRequest.Builder} avoiding the need to
* create one manually via {@link CreateProjectRequest#builder()}
*
*
* @param createProjectRequest
* A {@link Consumer} that will call methods on {@link CreateProjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectAlreadyExistsException An AWS CodeStar project with the same ID already exists in this region
* for the AWS account. AWS CodeStar project IDs must be unique within a region for the AWS account.
* - LimitExceededException A resource limit has been exceeded.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - ProjectCreationFailedException The project creation request was valid, but a nonspecific exception or
* error occurred during project creation. The project could not be created in AWS CodeStar.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.CreateProject
* @see AWS API
* Documentation
*/
default CompletableFuture createProject(Consumer createProjectRequest) {
return createProject(CreateProjectRequest.builder().applyMutation(createProjectRequest).build());
}
/**
*
* Creates a profile for a user that includes user preferences, such as the display name and email address
* assocciated with the user, in AWS CodeStar. The user profile is not project-specific. Information in the user
* profile is displayed wherever the user's information appears to other users in AWS CodeStar.
*
*
* @param createUserProfileRequest
* @return A Java Future containing the result of the CreateUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UserProfileAlreadyExistsException A user profile with that name already exists in this region for the
* AWS account. AWS CodeStar user profile names must be unique within a region for the AWS account.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.CreateUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture createUserProfile(CreateUserProfileRequest createUserProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a profile for a user that includes user preferences, such as the display name and email address
* assocciated with the user, in AWS CodeStar. The user profile is not project-specific. Information in the user
* profile is displayed wherever the user's information appears to other users in AWS CodeStar.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserProfileRequest.Builder} avoiding the need
* to create one manually via {@link CreateUserProfileRequest#builder()}
*
*
* @param createUserProfileRequest
* A {@link Consumer} that will call methods on {@link CreateUserProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UserProfileAlreadyExistsException A user profile with that name already exists in this region for the
* AWS account. AWS CodeStar user profile names must be unique within a region for the AWS account.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.CreateUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture createUserProfile(
Consumer createUserProfileRequest) {
return createUserProfile(CreateUserProfileRequest.builder().applyMutation(createUserProfileRequest).build());
}
/**
*
* Deletes a project, including project resources. Does not delete users associated with the project, but does
* delete the IAM roles that allowed access to the project.
*
*
* @param deleteProjectRequest
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DeleteProject
* @see AWS API
* Documentation
*/
default CompletableFuture deleteProject(DeleteProjectRequest deleteProjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a project, including project resources. Does not delete users associated with the project, but does
* delete the IAM roles that allowed access to the project.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProjectRequest.Builder} avoiding the need to
* create one manually via {@link DeleteProjectRequest#builder()}
*
*
* @param deleteProjectRequest
* A {@link Consumer} that will call methods on {@link DeleteProjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DeleteProject
* @see AWS API
* Documentation
*/
default CompletableFuture deleteProject(Consumer deleteProjectRequest) {
return deleteProject(DeleteProjectRequest.builder().applyMutation(deleteProjectRequest).build());
}
/**
*
* Deletes a user profile in AWS CodeStar, including all personal preference data associated with that profile, such
* as display name and email address. It does not delete the history of that user, for example the history of
* commits made by that user.
*
*
* @param deleteUserProfileRequest
* @return A Java Future containing the result of the DeleteUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DeleteUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture deleteUserProfile(DeleteUserProfileRequest deleteUserProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a user profile in AWS CodeStar, including all personal preference data associated with that profile, such
* as display name and email address. It does not delete the history of that user, for example the history of
* commits made by that user.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserProfileRequest.Builder} avoiding the need
* to create one manually via {@link DeleteUserProfileRequest#builder()}
*
*
* @param deleteUserProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteUserProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DeleteUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture deleteUserProfile(
Consumer deleteUserProfileRequest) {
return deleteUserProfile(DeleteUserProfileRequest.builder().applyMutation(deleteUserProfileRequest).build());
}
/**
*
* Describes a project and its resources.
*
*
* @param describeProjectRequest
* @return A Java Future containing the result of the DescribeProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DescribeProject
* @see AWS API
* Documentation
*/
default CompletableFuture describeProject(DescribeProjectRequest describeProjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a project and its resources.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeProjectRequest.Builder} avoiding the need
* to create one manually via {@link DescribeProjectRequest#builder()}
*
*
* @param describeProjectRequest
* A {@link Consumer} that will call methods on {@link DescribeProjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DescribeProject
* @see AWS API
* Documentation
*/
default CompletableFuture describeProject(
Consumer describeProjectRequest) {
return describeProject(DescribeProjectRequest.builder().applyMutation(describeProjectRequest).build());
}
/**
*
* Describes a user in AWS CodeStar and the user attributes across all projects.
*
*
* @param describeUserProfileRequest
* @return A Java Future containing the result of the DescribeUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UserProfileNotFoundException The user profile was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DescribeUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture describeUserProfile(
DescribeUserProfileRequest describeUserProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a user in AWS CodeStar and the user attributes across all projects.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserProfileRequest.Builder} avoiding the
* need to create one manually via {@link DescribeUserProfileRequest#builder()}
*
*
* @param describeUserProfileRequest
* A {@link Consumer} that will call methods on {@link DescribeUserProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UserProfileNotFoundException The user profile was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DescribeUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture describeUserProfile(
Consumer describeUserProfileRequest) {
return describeUserProfile(DescribeUserProfileRequest.builder().applyMutation(describeUserProfileRequest).build());
}
/**
*
* Removes a user from a project. Removing a user from a project also removes the IAM policies from that user that
* allowed access to the project and its resources. Disassociating a team member does not remove that user's profile
* from AWS CodeStar. It does not remove the user from IAM.
*
*
* @param disassociateTeamMemberRequest
* @return A Java Future containing the result of the DisassociateTeamMember operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DisassociateTeamMember
* @see AWS API Documentation
*/
default CompletableFuture disassociateTeamMember(
DisassociateTeamMemberRequest disassociateTeamMemberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes a user from a project. Removing a user from a project also removes the IAM policies from that user that
* allowed access to the project and its resources. Disassociating a team member does not remove that user's profile
* from AWS CodeStar. It does not remove the user from IAM.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateTeamMemberRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateTeamMemberRequest#builder()}
*
*
* @param disassociateTeamMemberRequest
* A {@link Consumer} that will call methods on {@link DisassociateTeamMemberRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateTeamMember operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.DisassociateTeamMember
* @see AWS API Documentation
*/
default CompletableFuture disassociateTeamMember(
Consumer disassociateTeamMemberRequest) {
return disassociateTeamMember(DisassociateTeamMemberRequest.builder().applyMutation(disassociateTeamMemberRequest)
.build());
}
/**
*
* Lists all projects in AWS CodeStar associated with your AWS account.
*
*
* @param listProjectsRequest
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListProjects
* @see AWS API
* Documentation
*/
default CompletableFuture listProjects(ListProjectsRequest listProjectsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all projects in AWS CodeStar associated with your AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListProjectsRequest.Builder} avoiding the need to
* create one manually via {@link ListProjectsRequest#builder()}
*
*
* @param listProjectsRequest
* A {@link Consumer} that will call methods on {@link ListProjectsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListProjects
* @see AWS API
* Documentation
*/
default CompletableFuture listProjects(Consumer listProjectsRequest) {
return listProjects(ListProjectsRequest.builder().applyMutation(listProjectsRequest).build());
}
/**
*
* Lists all projects in AWS CodeStar associated with your AWS account.
*
*
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListProjects
* @see AWS API
* Documentation
*/
default CompletableFuture listProjects() {
return listProjects(ListProjectsRequest.builder().build());
}
/**
*
* Lists resources associated with a project in AWS CodeStar.
*
*
* @param listResourcesRequest
* @return A Java Future containing the result of the ListResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListResources
* @see AWS API
* Documentation
*/
default CompletableFuture listResources(ListResourcesRequest listResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists resources associated with a project in AWS CodeStar.
*
*
*
* This is a convenience which creates an instance of the {@link ListResourcesRequest.Builder} avoiding the need to
* create one manually via {@link ListResourcesRequest#builder()}
*
*
* @param listResourcesRequest
* A {@link Consumer} that will call methods on {@link ListResourcesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListResources
* @see AWS API
* Documentation
*/
default CompletableFuture listResources(Consumer listResourcesRequest) {
return listResources(ListResourcesRequest.builder().applyMutation(listResourcesRequest).build());
}
/**
*
* Gets the tags for a project.
*
*
* @param listTagsForProjectRequest
* @return A Java Future containing the result of the ListTagsForProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidNextTokenException The next token is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListTagsForProject
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForProject(ListTagsForProjectRequest listTagsForProjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the tags for a project.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForProjectRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForProjectRequest#builder()}
*
*
* @param listTagsForProjectRequest
* A {@link Consumer} that will call methods on {@link ListTagsForProjectRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidNextTokenException The next token is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListTagsForProject
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForProject(
Consumer listTagsForProjectRequest) {
return listTagsForProject(ListTagsForProjectRequest.builder().applyMutation(listTagsForProjectRequest).build());
}
/**
*
* Lists all team members associated with a project.
*
*
* @param listTeamMembersRequest
* @return A Java Future containing the result of the ListTeamMembers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListTeamMembers
* @see AWS API
* Documentation
*/
default CompletableFuture listTeamMembers(ListTeamMembersRequest listTeamMembersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all team members associated with a project.
*
*
*
* This is a convenience which creates an instance of the {@link ListTeamMembersRequest.Builder} avoiding the need
* to create one manually via {@link ListTeamMembersRequest#builder()}
*
*
* @param listTeamMembersRequest
* A {@link Consumer} that will call methods on {@link ListTeamMembersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListTeamMembers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListTeamMembers
* @see AWS API
* Documentation
*/
default CompletableFuture listTeamMembers(
Consumer listTeamMembersRequest) {
return listTeamMembers(ListTeamMembersRequest.builder().applyMutation(listTeamMembersRequest).build());
}
/**
*
* Lists all the user profiles configured for your AWS account in AWS CodeStar.
*
*
* @param listUserProfilesRequest
* @return A Java Future containing the result of the ListUserProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListUserProfiles
* @see AWS API
* Documentation
*/
default CompletableFuture listUserProfiles(ListUserProfilesRequest listUserProfilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the user profiles configured for your AWS account in AWS CodeStar.
*
*
*
* This is a convenience which creates an instance of the {@link ListUserProfilesRequest.Builder} avoiding the need
* to create one manually via {@link ListUserProfilesRequest#builder()}
*
*
* @param listUserProfilesRequest
* A {@link Consumer} that will call methods on {@link ListUserProfilesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListUserProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListUserProfiles
* @see AWS API
* Documentation
*/
default CompletableFuture listUserProfiles(
Consumer listUserProfilesRequest) {
return listUserProfiles(ListUserProfilesRequest.builder().applyMutation(listUserProfilesRequest).build());
}
/**
*
* Lists all the user profiles configured for your AWS account in AWS CodeStar.
*
*
* @return A Java Future containing the result of the ListUserProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The next token is not valid.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.ListUserProfiles
* @see AWS API
* Documentation
*/
default CompletableFuture listUserProfiles() {
return listUserProfiles(ListUserProfilesRequest.builder().build());
}
/**
*
* Adds tags to a project.
*
*
* @param tagProjectRequest
* @return A Java Future containing the result of the TagProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - LimitExceededException A resource limit has been exceeded.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.TagProject
* @see AWS API
* Documentation
*/
default CompletableFuture tagProject(TagProjectRequest tagProjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds tags to a project.
*
*
*
* This is a convenience which creates an instance of the {@link TagProjectRequest.Builder} avoiding the need to
* create one manually via {@link TagProjectRequest#builder()}
*
*
* @param tagProjectRequest
* A {@link Consumer} that will call methods on {@link TagProjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - LimitExceededException A resource limit has been exceeded.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.TagProject
* @see AWS API
* Documentation
*/
default CompletableFuture tagProject(Consumer tagProjectRequest) {
return tagProject(TagProjectRequest.builder().applyMutation(tagProjectRequest).build());
}
/**
*
* Removes tags from a project.
*
*
* @param untagProjectRequest
* @return A Java Future containing the result of the UntagProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - LimitExceededException A resource limit has been exceeded.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UntagProject
* @see AWS API
* Documentation
*/
default CompletableFuture untagProject(UntagProjectRequest untagProjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a project.
*
*
*
* This is a convenience which creates an instance of the {@link UntagProjectRequest.Builder} avoiding the need to
* create one manually via {@link UntagProjectRequest#builder()}
*
*
* @param untagProjectRequest
* A {@link Consumer} that will call methods on {@link UntagProjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - LimitExceededException A resource limit has been exceeded.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UntagProject
* @see AWS API
* Documentation
*/
default CompletableFuture untagProject(Consumer untagProjectRequest) {
return untagProject(UntagProjectRequest.builder().applyMutation(untagProjectRequest).build());
}
/**
*
* Updates a project in AWS CodeStar.
*
*
* @param updateProjectRequest
* @return A Java Future containing the result of the UpdateProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UpdateProject
* @see AWS API
* Documentation
*/
default CompletableFuture updateProject(UpdateProjectRequest updateProjectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a project in AWS CodeStar.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateProjectRequest.Builder} avoiding the need to
* create one manually via {@link UpdateProjectRequest#builder()}
*
*
* @param updateProjectRequest
* A {@link Consumer} that will call methods on {@link UpdateProjectRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UpdateProject
* @see AWS API
* Documentation
*/
default CompletableFuture updateProject(Consumer updateProjectRequest) {
return updateProject(UpdateProjectRequest.builder().applyMutation(updateProjectRequest).build());
}
/**
*
* Updates a team member's attributes in an AWS CodeStar project. For example, you can change a team member's role
* in the project, or change whether they have remote access to project resources.
*
*
* @param updateTeamMemberRequest
* @return A Java Future containing the result of the UpdateTeamMember operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException A resource limit has been exceeded.
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - TeamMemberNotFoundException The specified team member was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UpdateTeamMember
* @see AWS API
* Documentation
*/
default CompletableFuture updateTeamMember(UpdateTeamMemberRequest updateTeamMemberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a team member's attributes in an AWS CodeStar project. For example, you can change a team member's role
* in the project, or change whether they have remote access to project resources.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateTeamMemberRequest.Builder} avoiding the need
* to create one manually via {@link UpdateTeamMemberRequest#builder()}
*
*
* @param updateTeamMemberRequest
* A {@link Consumer} that will call methods on {@link UpdateTeamMemberRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateTeamMember operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException A resource limit has been exceeded.
* - ProjectNotFoundException The specified AWS CodeStar project was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - InvalidServiceRoleException The service role is not valid.
* - ProjectConfigurationException Project configuration information is required but not specified.
* - ConcurrentModificationException Another modification is being made. That modification must complete
* before you can make your change.
* - TeamMemberNotFoundException The specified team member was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UpdateTeamMember
* @see AWS API
* Documentation
*/
default CompletableFuture updateTeamMember(
Consumer updateTeamMemberRequest) {
return updateTeamMember(UpdateTeamMemberRequest.builder().applyMutation(updateTeamMemberRequest).build());
}
/**
*
* Updates a user's profile in AWS CodeStar. The user profile is not project-specific. Information in the user
* profile is displayed wherever the user's information appears to other users in AWS CodeStar.
*
*
* @param updateUserProfileRequest
* @return A Java Future containing the result of the UpdateUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UserProfileNotFoundException The user profile was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UpdateUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture updateUserProfile(UpdateUserProfileRequest updateUserProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a user's profile in AWS CodeStar. The user profile is not project-specific. Information in the user
* profile is displayed wherever the user's information appears to other users in AWS CodeStar.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserProfileRequest.Builder} avoiding the need
* to create one manually via {@link UpdateUserProfileRequest#builder()}
*
*
* @param updateUserProfileRequest
* A {@link Consumer} that will call methods on {@link UpdateUserProfileRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UserProfileNotFoundException The user profile was not found.
* - ValidationException The specified input is either not valid, or it could not be validated.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CodeStarAsyncClient.UpdateUserProfile
* @see AWS
* API Documentation
*/
default CompletableFuture updateUserProfile(
Consumer updateUserProfileRequest) {
return updateUserProfile(UpdateUserProfileRequest.builder().applyMutation(updateUserProfileRequest).build());
}
}