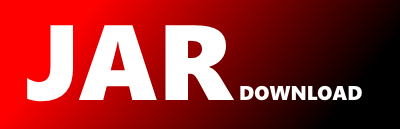
software.amazon.awssdk.services.codestarconnections.model.Connection Maven / Gradle / Ivy
Show all versions of codestarconnections Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codestarconnections.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The configuration that allows a service such as CodePipeline to connect to a third-party code repository.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Connection implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CONNECTION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Connection::connectionName)).setter(setter(Builder::connectionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectionName").build()).build();
private static final SdkField CONNECTION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Connection::connectionArn)).setter(setter(Builder::connectionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectionArn").build()).build();
private static final SdkField PROVIDER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Connection::providerTypeAsString)).setter(setter(Builder::providerType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProviderType").build()).build();
private static final SdkField OWNER_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Connection::ownerAccountId)).setter(setter(Builder::ownerAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerAccountId").build()).build();
private static final SdkField CONNECTION_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Connection::connectionStatusAsString)).setter(setter(Builder::connectionStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectionStatus").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONNECTION_NAME_FIELD,
CONNECTION_ARN_FIELD, PROVIDER_TYPE_FIELD, OWNER_ACCOUNT_ID_FIELD, CONNECTION_STATUS_FIELD));
private static final long serialVersionUID = 1L;
private final String connectionName;
private final String connectionArn;
private final String providerType;
private final String ownerAccountId;
private final String connectionStatus;
private Connection(BuilderImpl builder) {
this.connectionName = builder.connectionName;
this.connectionArn = builder.connectionArn;
this.providerType = builder.providerType;
this.ownerAccountId = builder.ownerAccountId;
this.connectionStatus = builder.connectionStatus;
}
/**
*
* The name of the connection. Connection names must be unique in an AWS user account.
*
*
* @return The name of the connection. Connection names must be unique in an AWS user account.
*/
public String connectionName() {
return connectionName;
}
/**
*
* The Amazon Resource Name (ARN) of the connection. The ARN is used as the connection reference when the connection
* is shared between AWS services.
*
*
*
* The ARN is never reused if the connection is deleted.
*
*
*
* @return The Amazon Resource Name (ARN) of the connection. The ARN is used as the connection reference when the
* connection is shared between AWS services.
*
* The ARN is never reused if the connection is deleted.
*
*/
public String connectionArn() {
return connectionArn;
}
/**
*
* The name of the external provider where your third-party code repository is configured. Currently, the valid
* provider type is Bitbucket.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #providerType} will
* return {@link ProviderType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #providerTypeAsString}.
*
*
* @return The name of the external provider where your third-party code repository is configured. Currently, the
* valid provider type is Bitbucket.
* @see ProviderType
*/
public ProviderType providerType() {
return ProviderType.fromValue(providerType);
}
/**
*
* The name of the external provider where your third-party code repository is configured. Currently, the valid
* provider type is Bitbucket.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #providerType} will
* return {@link ProviderType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #providerTypeAsString}.
*
*
* @return The name of the external provider where your third-party code repository is configured. Currently, the
* valid provider type is Bitbucket.
* @see ProviderType
*/
public String providerTypeAsString() {
return providerType;
}
/**
*
* The name of the external provider where your third-party code repository is configured. For Bitbucket, this is
* the account ID of the owner of the Bitbucket repository.
*
*
* @return The name of the external provider where your third-party code repository is configured. For Bitbucket,
* this is the account ID of the owner of the Bitbucket repository.
*/
public String ownerAccountId() {
return ownerAccountId;
}
/**
*
* The current status of the connection.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectionStatus}
* will return {@link ConnectionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #connectionStatusAsString}.
*
*
* @return The current status of the connection.
* @see ConnectionStatus
*/
public ConnectionStatus connectionStatus() {
return ConnectionStatus.fromValue(connectionStatus);
}
/**
*
* The current status of the connection.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectionStatus}
* will return {@link ConnectionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #connectionStatusAsString}.
*
*
* @return The current status of the connection.
* @see ConnectionStatus
*/
public String connectionStatusAsString() {
return connectionStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(connectionName());
hashCode = 31 * hashCode + Objects.hashCode(connectionArn());
hashCode = 31 * hashCode + Objects.hashCode(providerTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ownerAccountId());
hashCode = 31 * hashCode + Objects.hashCode(connectionStatusAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Connection)) {
return false;
}
Connection other = (Connection) obj;
return Objects.equals(connectionName(), other.connectionName()) && Objects.equals(connectionArn(), other.connectionArn())
&& Objects.equals(providerTypeAsString(), other.providerTypeAsString())
&& Objects.equals(ownerAccountId(), other.ownerAccountId())
&& Objects.equals(connectionStatusAsString(), other.connectionStatusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Connection").add("ConnectionName", connectionName()).add("ConnectionArn", connectionArn())
.add("ProviderType", providerTypeAsString()).add("OwnerAccountId", ownerAccountId())
.add("ConnectionStatus", connectionStatusAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ConnectionName":
return Optional.ofNullable(clazz.cast(connectionName()));
case "ConnectionArn":
return Optional.ofNullable(clazz.cast(connectionArn()));
case "ProviderType":
return Optional.ofNullable(clazz.cast(providerTypeAsString()));
case "OwnerAccountId":
return Optional.ofNullable(clazz.cast(ownerAccountId()));
case "ConnectionStatus":
return Optional.ofNullable(clazz.cast(connectionStatusAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function