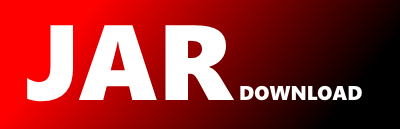
software.amazon.awssdk.services.codestarconnections.CodeStarConnectionsAsyncClient Maven / Gradle / Ivy
Show all versions of codestarconnections Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codestarconnections;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.codestarconnections.model.CreateConnectionRequest;
import software.amazon.awssdk.services.codestarconnections.model.CreateConnectionResponse;
import software.amazon.awssdk.services.codestarconnections.model.CreateHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.CreateHostResponse;
import software.amazon.awssdk.services.codestarconnections.model.DeleteConnectionRequest;
import software.amazon.awssdk.services.codestarconnections.model.DeleteConnectionResponse;
import software.amazon.awssdk.services.codestarconnections.model.DeleteHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.DeleteHostResponse;
import software.amazon.awssdk.services.codestarconnections.model.GetConnectionRequest;
import software.amazon.awssdk.services.codestarconnections.model.GetConnectionResponse;
import software.amazon.awssdk.services.codestarconnections.model.GetHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.GetHostResponse;
import software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest;
import software.amazon.awssdk.services.codestarconnections.model.ListConnectionsResponse;
import software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest;
import software.amazon.awssdk.services.codestarconnections.model.ListHostsResponse;
import software.amazon.awssdk.services.codestarconnections.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.codestarconnections.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.codestarconnections.model.TagResourceRequest;
import software.amazon.awssdk.services.codestarconnections.model.TagResourceResponse;
import software.amazon.awssdk.services.codestarconnections.model.UntagResourceRequest;
import software.amazon.awssdk.services.codestarconnections.model.UntagResourceResponse;
import software.amazon.awssdk.services.codestarconnections.model.UpdateHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.UpdateHostResponse;
import software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsPublisher;
import software.amazon.awssdk.services.codestarconnections.paginators.ListHostsPublisher;
/**
* Service client for accessing AWS CodeStar connections asynchronously. This can be created using the static
* {@link #builder()} method.
*
* AWS CodeStar Connections
*
* This AWS CodeStar Connections API Reference provides descriptions and usage examples of the operations and data types
* for the AWS CodeStar Connections API. You can use the connections API to work with connections and installations.
*
*
* Connections are configurations that you use to connect AWS resources to external code repositories. Each
* connection is a resource that can be given to services such as CodePipeline to connect to a third-party repository
* such as Bitbucket. For example, you can add the connection in CodePipeline so that it triggers your pipeline when a
* code change is made to your third-party code repository. Each connection is named and associated with a unique ARN
* that is used to reference the connection.
*
*
* When you create a connection, the console initiates a third-party connection handshake. Installations are the
* apps that are used to conduct this handshake. For example, the installation for the Bitbucket provider type is the
* Bitbucket app. When you create a connection, you can choose an existing installation or create one.
*
*
* When you want to create a connection to an installed provider type such as GitHub Enterprise Server, you create a
* host for your connections.
*
*
* You can work with connections by calling:
*
*
* -
*
* CreateConnection, which creates a uniquely named connection that can be referenced by services such as
* CodePipeline.
*
*
* -
*
* DeleteConnection, which deletes the specified connection.
*
*
* -
*
* GetConnection, which returns information about the connection, including the connection status.
*
*
* -
*
* ListConnections, which lists the connections associated with your account.
*
*
*
*
* You can work with hosts by calling:
*
*
* -
*
* CreateHost, which creates a host that represents the infrastructure where your provider is installed.
*
*
* -
*
* DeleteHost, which deletes the specified host.
*
*
* -
*
* GetHost, which returns information about the host, including the setup status.
*
*
* -
*
* ListHosts, which lists the hosts associated with your account.
*
*
*
*
* You can work with tags in AWS CodeStar Connections by calling the following:
*
*
* -
*
* ListTagsForResource, which gets information about AWS tags for a specified Amazon Resource Name (ARN) in AWS
* CodeStar Connections.
*
*
* -
*
* TagResource, which adds or updates tags for a resource in AWS CodeStar Connections.
*
*
* -
*
* UntagResource, which removes tags for a resource in AWS CodeStar Connections.
*
*
*
*
* For information about how to use AWS CodeStar Connections, see the Developer Tools User
* Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface CodeStarConnectionsAsyncClient extends SdkClient {
String SERVICE_NAME = "codestar-connections";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "codestar-connections";
/**
* Create a {@link CodeStarConnectionsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CodeStarConnectionsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CodeStarConnectionsAsyncClient}.
*/
static CodeStarConnectionsAsyncClientBuilder builder() {
return new DefaultCodeStarConnectionsAsyncClientBuilder();
}
/**
*
* Creates a connection that can then be given to other AWS services like CodePipeline so that it can access
* third-party code repositories. The connection is in pending status until the third-party connection handshake is
* completed from the console.
*
*
* @param createConnectionRequest
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException Exceeded the maximum limit for connections.
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.CreateConnection
* @see AWS API Documentation
*/
default CompletableFuture createConnection(CreateConnectionRequest createConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a connection that can then be given to other AWS services like CodePipeline so that it can access
* third-party code repositories. The connection is in pending status until the third-party connection handshake is
* completed from the console.
*
*
*
* This is a convenience which creates an instance of the {@link CreateConnectionRequest.Builder} avoiding the need
* to create one manually via {@link CreateConnectionRequest#builder()}
*
*
* @param createConnectionRequest
* A {@link Consumer} that will call methods on {@link CreateConnectionInput.Builder} to create a request.
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException Exceeded the maximum limit for connections.
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.CreateConnection
* @see AWS API Documentation
*/
default CompletableFuture createConnection(
Consumer createConnectionRequest) {
return createConnection(CreateConnectionRequest.builder().applyMutation(createConnectionRequest).build());
}
/**
*
* Creates a resource that represents the infrastructure where a third-party provider is installed. The host is used
* when you create connections to an installed third-party provider type, such as GitHub Enterprise Server. You
* create one host for all connections to that provider.
*
*
*
* A host created through the CLI or the SDK is in `PENDING` status by default. You can make its status `AVAILABLE`
* by setting up the host in the console.
*
*
*
* @param createHostRequest
* @return A Java Future containing the result of the CreateHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException Exceeded the maximum limit for connections.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.CreateHost
* @see AWS API Documentation
*/
default CompletableFuture createHost(CreateHostRequest createHostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a resource that represents the infrastructure where a third-party provider is installed. The host is used
* when you create connections to an installed third-party provider type, such as GitHub Enterprise Server. You
* create one host for all connections to that provider.
*
*
*
* A host created through the CLI or the SDK is in `PENDING` status by default. You can make its status `AVAILABLE`
* by setting up the host in the console.
*
*
*
* This is a convenience which creates an instance of the {@link CreateHostRequest.Builder} avoiding the need to
* create one manually via {@link CreateHostRequest#builder()}
*
*
* @param createHostRequest
* A {@link Consumer} that will call methods on {@link CreateHostInput.Builder} to create a request.
* @return A Java Future containing the result of the CreateHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException Exceeded the maximum limit for connections.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.CreateHost
* @see AWS API Documentation
*/
default CompletableFuture createHost(Consumer createHostRequest) {
return createHost(CreateHostRequest.builder().applyMutation(createHostRequest).build());
}
/**
*
* The connection to be deleted.
*
*
* @param deleteConnectionRequest
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.DeleteConnection
* @see AWS API Documentation
*/
default CompletableFuture deleteConnection(DeleteConnectionRequest deleteConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The connection to be deleted.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteConnectionRequest.Builder} avoiding the need
* to create one manually via {@link DeleteConnectionRequest#builder()}
*
*
* @param deleteConnectionRequest
* A {@link Consumer} that will call methods on {@link DeleteConnectionInput.Builder} to create a request.
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.DeleteConnection
* @see AWS API Documentation
*/
default CompletableFuture deleteConnection(
Consumer deleteConnectionRequest) {
return deleteConnection(DeleteConnectionRequest.builder().applyMutation(deleteConnectionRequest).build());
}
/**
*
* The host to be deleted. Before you delete a host, all connections associated to the host must be deleted.
*
*
*
* A host cannot be deleted if it is in the VPC_CONFIG_INITIALIZING or VPC_CONFIG_DELETING state.
*
*
*
* @param deleteHostRequest
* @return A Java Future containing the result of the DeleteHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.DeleteHost
* @see AWS API Documentation
*/
default CompletableFuture deleteHost(DeleteHostRequest deleteHostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The host to be deleted. Before you delete a host, all connections associated to the host must be deleted.
*
*
*
* A host cannot be deleted if it is in the VPC_CONFIG_INITIALIZING or VPC_CONFIG_DELETING state.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteHostRequest.Builder} avoiding the need to
* create one manually via {@link DeleteHostRequest#builder()}
*
*
* @param deleteHostRequest
* A {@link Consumer} that will call methods on {@link DeleteHostInput.Builder} to create a request.
* @return A Java Future containing the result of the DeleteHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.DeleteHost
* @see AWS API Documentation
*/
default CompletableFuture deleteHost(Consumer deleteHostRequest) {
return deleteHost(DeleteHostRequest.builder().applyMutation(deleteHostRequest).build());
}
/**
*
* Returns the connection ARN and details such as status, owner, and provider type.
*
*
* @param getConnectionRequest
* @return A Java Future containing the result of the GetConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.GetConnection
* @see AWS API Documentation
*/
default CompletableFuture getConnection(GetConnectionRequest getConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the connection ARN and details such as status, owner, and provider type.
*
*
*
* This is a convenience which creates an instance of the {@link GetConnectionRequest.Builder} avoiding the need to
* create one manually via {@link GetConnectionRequest#builder()}
*
*
* @param getConnectionRequest
* A {@link Consumer} that will call methods on {@link GetConnectionInput.Builder} to create a request.
* @return A Java Future containing the result of the GetConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.GetConnection
* @see AWS API Documentation
*/
default CompletableFuture getConnection(Consumer getConnectionRequest) {
return getConnection(GetConnectionRequest.builder().applyMutation(getConnectionRequest).build());
}
/**
*
* Returns the host ARN and details such as status, provider type, endpoint, and, if applicable, the VPC
* configuration.
*
*
* @param getHostRequest
* @return A Java Future containing the result of the GetHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.GetHost
* @see AWS
* API Documentation
*/
default CompletableFuture getHost(GetHostRequest getHostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the host ARN and details such as status, provider type, endpoint, and, if applicable, the VPC
* configuration.
*
*
*
* This is a convenience which creates an instance of the {@link GetHostRequest.Builder} avoiding the need to create
* one manually via {@link GetHostRequest#builder()}
*
*
* @param getHostRequest
* A {@link Consumer} that will call methods on {@link GetHostInput.Builder} to create a request.
* @return A Java Future containing the result of the GetHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.GetHost
* @see AWS
* API Documentation
*/
default CompletableFuture getHost(Consumer getHostRequest) {
return getHost(GetHostRequest.builder().applyMutation(getHostRequest).build());
}
/**
*
* Lists the connections associated with your account.
*
*
* @param listConnectionsRequest
* @return A Java Future containing the result of the ListConnections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListConnections
* @see AWS API Documentation
*/
default CompletableFuture listConnections(ListConnectionsRequest listConnectionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the connections associated with your account.
*
*
*
* This is a convenience which creates an instance of the {@link ListConnectionsRequest.Builder} avoiding the need
* to create one manually via {@link ListConnectionsRequest#builder()}
*
*
* @param listConnectionsRequest
* A {@link Consumer} that will call methods on {@link ListConnectionsInput.Builder} to create a request.
* @return A Java Future containing the result of the ListConnections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListConnections
* @see AWS API Documentation
*/
default CompletableFuture listConnections(
Consumer listConnectionsRequest) {
return listConnections(ListConnectionsRequest.builder().applyMutation(listConnectionsRequest).build());
}
/**
*
* Lists the connections associated with your account.
*
*
*
* This is a variant of
* {@link #listConnections(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsPublisher publisher = client.listConnectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsPublisher publisher = client.listConnectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConnections(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest)}
* operation.
*
*
* @param listConnectionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListConnections
* @see AWS API Documentation
*/
default ListConnectionsPublisher listConnectionsPaginator(ListConnectionsRequest listConnectionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the connections associated with your account.
*
*
*
* This is a variant of
* {@link #listConnections(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsPublisher publisher = client.listConnectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsPublisher publisher = client.listConnectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConnections(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListConnectionsRequest.Builder} avoiding the need
* to create one manually via {@link ListConnectionsRequest#builder()}
*
*
* @param listConnectionsRequest
* A {@link Consumer} that will call methods on {@link ListConnectionsInput.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListConnections
* @see AWS API Documentation
*/
default ListConnectionsPublisher listConnectionsPaginator(Consumer listConnectionsRequest) {
return listConnectionsPaginator(ListConnectionsRequest.builder().applyMutation(listConnectionsRequest).build());
}
/**
*
* Lists the hosts associated with your account.
*
*
* @param listHostsRequest
* @return A Java Future containing the result of the ListHosts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListHosts
* @see AWS API Documentation
*/
default CompletableFuture listHosts(ListHostsRequest listHostsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the hosts associated with your account.
*
*
*
* This is a convenience which creates an instance of the {@link ListHostsRequest.Builder} avoiding the need to
* create one manually via {@link ListHostsRequest#builder()}
*
*
* @param listHostsRequest
* A {@link Consumer} that will call methods on {@link ListHostsInput.Builder} to create a request.
* @return A Java Future containing the result of the ListHosts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListHosts
* @see AWS API Documentation
*/
default CompletableFuture listHosts(Consumer listHostsRequest) {
return listHosts(ListHostsRequest.builder().applyMutation(listHostsRequest).build());
}
/**
*
* Lists the hosts associated with your account.
*
*
*
* This is a variant of
* {@link #listHosts(software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListHostsPublisher publisher = client.listHostsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListHostsPublisher publisher = client.listHostsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codestarconnections.model.ListHostsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHosts(software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest)} operation.
*
*
* @param listHostsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListHosts
* @see AWS API Documentation
*/
default ListHostsPublisher listHostsPaginator(ListHostsRequest listHostsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the hosts associated with your account.
*
*
*
* This is a variant of
* {@link #listHosts(software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListHostsPublisher publisher = client.listHostsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListHostsPublisher publisher = client.listHostsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.codestarconnections.model.ListHostsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHosts(software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListHostsRequest.Builder} avoiding the need to
* create one manually via {@link ListHostsRequest#builder()}
*
*
* @param listHostsRequest
* A {@link Consumer} that will call methods on {@link ListHostsInput.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListHosts
* @see AWS API Documentation
*/
default ListHostsPublisher listHostsPaginator(Consumer listHostsRequest) {
return listHostsPaginator(ListHostsRequest.builder().applyMutation(listHostsRequest).build());
}
/**
*
* Gets the set of key-value pairs (metadata) that are used to manage the resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the set of key-value pairs (metadata) that are used to manage the resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceInput.Builder} to create a request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Adds to or modifies the tags of the given resource. Tags are metadata that can be used to manage a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - LimitExceededException Exceeded the maximum limit for connections.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.TagResource
* @see AWS API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds to or modifies the tags of the given resource. Tags are metadata that can be used to manage a resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceInput.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - LimitExceededException Exceeded the maximum limit for connections.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.TagResource
* @see AWS API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from an AWS resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from an AWS resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceInput.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a specified host with the provided configurations.
*
*
* @param updateHostRequest
* @return A Java Future containing the result of the UpdateHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConflictException Two conflicting operations have been made on the same resource.
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - UnsupportedOperationException The operation is not supported. Check the connection status and try
* again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.UpdateHost
* @see AWS API Documentation
*/
default CompletableFuture updateHost(UpdateHostRequest updateHostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified host with the provided configurations.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateHostRequest.Builder} avoiding the need to
* create one manually via {@link UpdateHostRequest#builder()}
*
*
* @param updateHostRequest
* A {@link Consumer} that will call methods on {@link UpdateHostInput.Builder} to create a request.
* @return A Java Future containing the result of the UpdateHost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConflictException Two conflicting operations have been made on the same resource.
* - ResourceNotFoundException Resource not found. Verify the connection resource ARN and try again.
* - ResourceUnavailableException Resource not found. Verify the ARN for the host resource and try again.
* - UnsupportedOperationException The operation is not supported. Check the connection status and try
* again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CodeStarConnectionsException Base class for all service exceptions. Unknown exceptions will be thrown
* as an instance of this type.
*
* @sample CodeStarConnectionsAsyncClient.UpdateHost
* @see AWS API Documentation
*/
default CompletableFuture updateHost(Consumer updateHostRequest) {
return updateHost(UpdateHostRequest.builder().applyMutation(updateHostRequest).build());
}
}