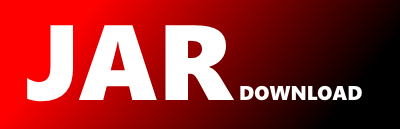
software.amazon.awssdk.services.codestarconnections.DefaultCodeStarConnectionsClient Maven / Gradle / Ivy
Show all versions of codestarconnections Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codestarconnections;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.codestarconnections.model.CodeStarConnectionsException;
import software.amazon.awssdk.services.codestarconnections.model.CodeStarConnectionsRequest;
import software.amazon.awssdk.services.codestarconnections.model.ConflictException;
import software.amazon.awssdk.services.codestarconnections.model.CreateConnectionRequest;
import software.amazon.awssdk.services.codestarconnections.model.CreateConnectionResponse;
import software.amazon.awssdk.services.codestarconnections.model.CreateHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.CreateHostResponse;
import software.amazon.awssdk.services.codestarconnections.model.DeleteConnectionRequest;
import software.amazon.awssdk.services.codestarconnections.model.DeleteConnectionResponse;
import software.amazon.awssdk.services.codestarconnections.model.DeleteHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.DeleteHostResponse;
import software.amazon.awssdk.services.codestarconnections.model.GetConnectionRequest;
import software.amazon.awssdk.services.codestarconnections.model.GetConnectionResponse;
import software.amazon.awssdk.services.codestarconnections.model.GetHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.GetHostResponse;
import software.amazon.awssdk.services.codestarconnections.model.LimitExceededException;
import software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest;
import software.amazon.awssdk.services.codestarconnections.model.ListConnectionsResponse;
import software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest;
import software.amazon.awssdk.services.codestarconnections.model.ListHostsResponse;
import software.amazon.awssdk.services.codestarconnections.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.codestarconnections.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.codestarconnections.model.ResourceNotFoundException;
import software.amazon.awssdk.services.codestarconnections.model.ResourceUnavailableException;
import software.amazon.awssdk.services.codestarconnections.model.TagResourceRequest;
import software.amazon.awssdk.services.codestarconnections.model.TagResourceResponse;
import software.amazon.awssdk.services.codestarconnections.model.UnsupportedOperationException;
import software.amazon.awssdk.services.codestarconnections.model.UntagResourceRequest;
import software.amazon.awssdk.services.codestarconnections.model.UntagResourceResponse;
import software.amazon.awssdk.services.codestarconnections.model.UpdateHostRequest;
import software.amazon.awssdk.services.codestarconnections.model.UpdateHostResponse;
import software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsIterable;
import software.amazon.awssdk.services.codestarconnections.paginators.ListHostsIterable;
import software.amazon.awssdk.services.codestarconnections.transform.CreateConnectionRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.CreateHostRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.DeleteConnectionRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.DeleteHostRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.GetConnectionRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.GetHostRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.ListConnectionsRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.ListHostsRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.codestarconnections.transform.UpdateHostRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link CodeStarConnectionsClient}.
*
* @see CodeStarConnectionsClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCodeStarConnectionsClient implements CodeStarConnectionsClient {
private static final Logger log = Logger.loggerFor(DefaultCodeStarConnectionsClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final CodeStarConnectionsServiceClientConfiguration serviceClientConfiguration;
protected DefaultCodeStarConnectionsClient(CodeStarConnectionsServiceClientConfiguration serviceClientConfiguration,
SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.serviceClientConfiguration = serviceClientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Creates a connection that can then be given to other AWS services like CodePipeline so that it can access
* third-party code repositories. The connection is in pending status until the third-party connection handshake is
* completed from the console.
*
*
* @param createConnectionRequest
* @return Result of the CreateConnection operation returned by the service.
* @throws LimitExceededException
* Exceeded the maximum limit for connections.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws ResourceUnavailableException
* Resource not found. Verify the ARN for the host resource and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.CreateConnection
* @see AWS API Documentation
*/
@Override
public CreateConnectionResponse createConnection(CreateConnectionRequest createConnectionRequest)
throws LimitExceededException, ResourceNotFoundException, ResourceUnavailableException, AwsServiceException,
SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConnection");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateConnection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a resource that represents the infrastructure where a third-party provider is installed. The host is used
* when you create connections to an installed third-party provider type, such as GitHub Enterprise Server. You
* create one host for all connections to that provider.
*
*
*
* A host created through the CLI or the SDK is in `PENDING` status by default. You can make its status `AVAILABLE`
* by setting up the host in the console.
*
*
*
* @param createHostRequest
* @return Result of the CreateHost operation returned by the service.
* @throws LimitExceededException
* Exceeded the maximum limit for connections.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.CreateHost
* @see AWS API Documentation
*/
@Override
public CreateHostResponse createHost(CreateHostRequest createHostRequest) throws LimitExceededException, AwsServiceException,
SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateHostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHost");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateHost")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createHostRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateHostRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* The connection to be deleted.
*
*
* @param deleteConnectionRequest
* @return Result of the DeleteConnection operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.DeleteConnection
* @see AWS API Documentation
*/
@Override
public DeleteConnectionResponse deleteConnection(DeleteConnectionRequest deleteConnectionRequest)
throws ResourceNotFoundException, AwsServiceException, SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConnection");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteConnection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* The host to be deleted. Before you delete a host, all connections associated to the host must be deleted.
*
*
*
* A host cannot be deleted if it is in the VPC_CONFIG_INITIALIZING or VPC_CONFIG_DELETING state.
*
*
*
* @param deleteHostRequest
* @return Result of the DeleteHost operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws ResourceUnavailableException
* Resource not found. Verify the ARN for the host resource and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.DeleteHost
* @see AWS API Documentation
*/
@Override
public DeleteHostResponse deleteHost(DeleteHostRequest deleteHostRequest) throws ResourceNotFoundException,
ResourceUnavailableException, AwsServiceException, SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteHostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteHostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteHost");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteHost")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteHostRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteHostRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the connection ARN and details such as status, owner, and provider type.
*
*
* @param getConnectionRequest
* @return Result of the GetConnection operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws ResourceUnavailableException
* Resource not found. Verify the ARN for the host resource and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.GetConnection
* @see AWS API Documentation
*/
@Override
public GetConnectionResponse getConnection(GetConnectionRequest getConnectionRequest) throws ResourceNotFoundException,
ResourceUnavailableException, AwsServiceException, SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetConnection");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetConnection").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getConnectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetConnectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the host ARN and details such as status, provider type, endpoint, and, if applicable, the VPC
* configuration.
*
*
* @param getHostRequest
* @return Result of the GetHost operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws ResourceUnavailableException
* Resource not found. Verify the ARN for the host resource and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.GetHost
* @see AWS
* API Documentation
*/
@Override
public GetHostResponse getHost(GetHostRequest getHostRequest) throws ResourceNotFoundException, ResourceUnavailableException,
AwsServiceException, SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetHostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getHostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetHost");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetHost").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getHostRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new GetHostRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the connections associated with your account.
*
*
* @param listConnectionsRequest
* @return Result of the ListConnections operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.ListConnections
* @see AWS API Documentation
*/
@Override
public ListConnectionsResponse listConnections(ListConnectionsRequest listConnectionsRequest) throws AwsServiceException,
SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListConnectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listConnectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListConnections");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListConnections").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listConnectionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListConnectionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the connections associated with your account.
*
*
*
* This is a variant of
* {@link #listConnections(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsIterable responses = client.listConnectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsIterable responses = client
* .listConnectionsPaginator(request);
* for (software.amazon.awssdk.services.codestarconnections.model.ListConnectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListConnectionsIterable responses = client.listConnectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConnections(software.amazon.awssdk.services.codestarconnections.model.ListConnectionsRequest)}
* operation.
*
*
* @param listConnectionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.ListConnections
* @see AWS API Documentation
*/
@Override
public ListConnectionsIterable listConnectionsPaginator(ListConnectionsRequest listConnectionsRequest)
throws AwsServiceException, SdkClientException, CodeStarConnectionsException {
return new ListConnectionsIterable(this, applyPaginatorUserAgent(listConnectionsRequest));
}
/**
*
* Lists the hosts associated with your account.
*
*
* @param listHostsRequest
* @return Result of the ListHosts operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.ListHosts
* @see AWS API Documentation
*/
@Override
public ListHostsResponse listHosts(ListHostsRequest listHostsRequest) throws AwsServiceException, SdkClientException,
CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListHostsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listHostsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListHosts");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListHosts").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listHostsRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new ListHostsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the hosts associated with your account.
*
*
*
* This is a variant of
* {@link #listHosts(software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListHostsIterable responses = client.listHostsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.codestarconnections.paginators.ListHostsIterable responses = client
* .listHostsPaginator(request);
* for (software.amazon.awssdk.services.codestarconnections.model.ListHostsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.codestarconnections.paginators.ListHostsIterable responses = client.listHostsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHosts(software.amazon.awssdk.services.codestarconnections.model.ListHostsRequest)} operation.
*
*
* @param listHostsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.ListHosts
* @see AWS API Documentation
*/
@Override
public ListHostsIterable listHostsPaginator(ListHostsRequest listHostsRequest) throws AwsServiceException,
SdkClientException, CodeStarConnectionsException {
return new ListHostsIterable(this, applyPaginatorUserAgent(listHostsRequest));
}
/**
*
* Gets the set of key-value pairs (metadata) that are used to manage the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ResourceNotFoundException, AwsServiceException, SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds to or modifies the tags of the given resource. Tags are metadata that can be used to manage a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws LimitExceededException
* Exceeded the maximum limit for connections.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.TagResource
* @see AWS API Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ResourceNotFoundException,
LimitExceededException, AwsServiceException, SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes tags from an AWS resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.UntagResource
* @see AWS API Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a specified host with the provided configurations.
*
*
* @param updateHostRequest
* @return Result of the UpdateHost operation returned by the service.
* @throws ConflictException
* Two conflicting operations have been made on the same resource.
* @throws ResourceNotFoundException
* Resource not found. Verify the connection resource ARN and try again.
* @throws ResourceUnavailableException
* Resource not found. Verify the ARN for the host resource and try again.
* @throws UnsupportedOperationException
* The operation is not supported. Check the connection status and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CodeStarConnectionsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CodeStarConnectionsClient.UpdateHost
* @see AWS API Documentation
*/
@Override
public UpdateHostResponse updateHost(UpdateHostRequest updateHostRequest) throws ConflictException,
ResourceNotFoundException, ResourceUnavailableException, UnsupportedOperationException, AwsServiceException,
SdkClientException, CodeStarConnectionsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateHostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateHostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CodeStar connections");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateHost");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("UpdateHost")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(updateHostRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateHostRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(CodeStarConnectionsException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.0")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnsupportedOperationException")
.exceptionBuilderSupplier(UnsupportedOperationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceUnavailableException")
.exceptionBuilderSupplier(ResourceUnavailableException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).httpStatusCode(400).build());
}
@Override
public final CodeStarConnectionsServiceClientConfiguration serviceClientConfiguration() {
return this.serviceClientConfiguration;
}
@Override
public void close() {
clientHandler.close();
}
}